2 D ARRAYS AND ARRAYS OF POINTERS Defining
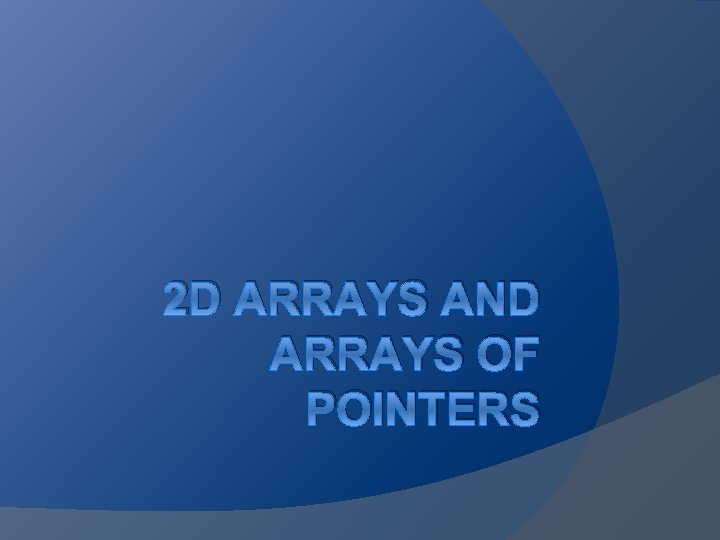
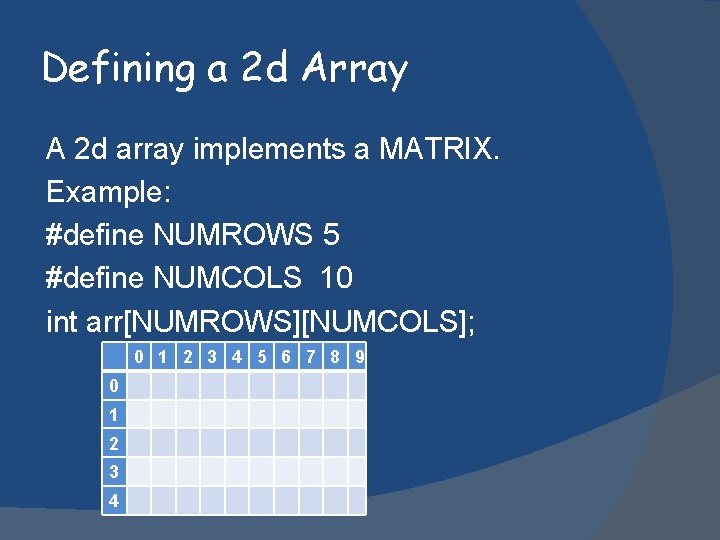
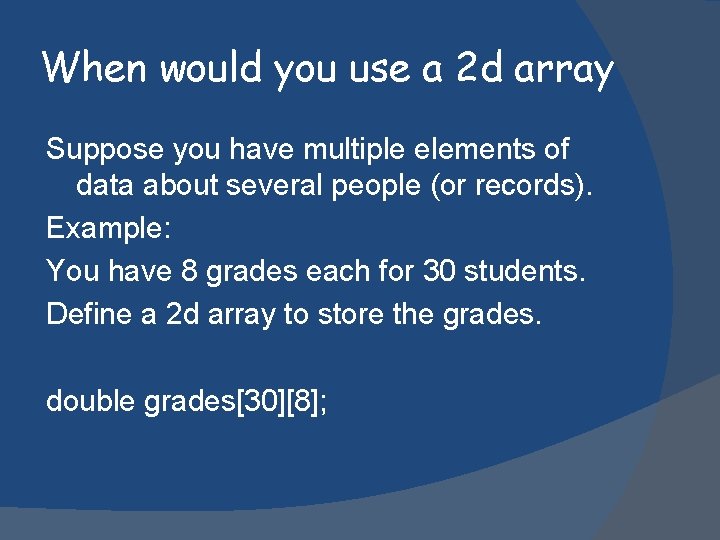
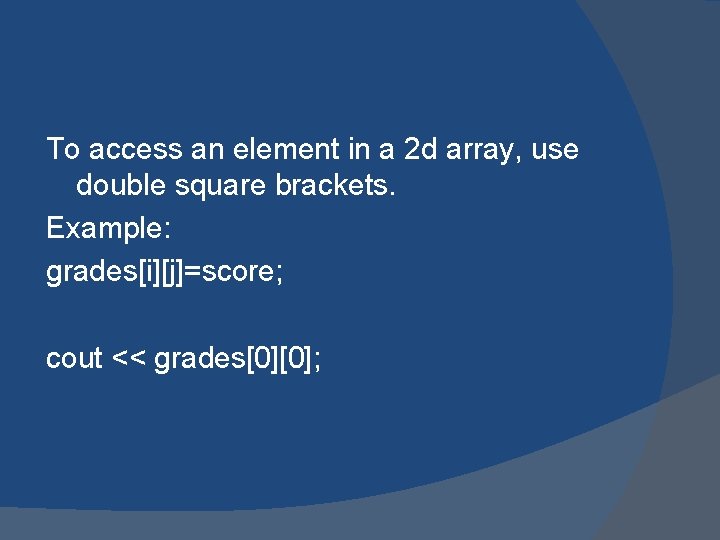
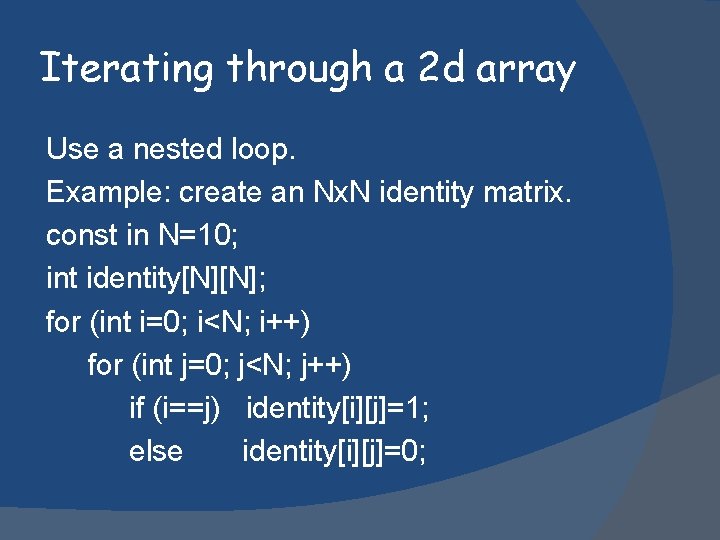
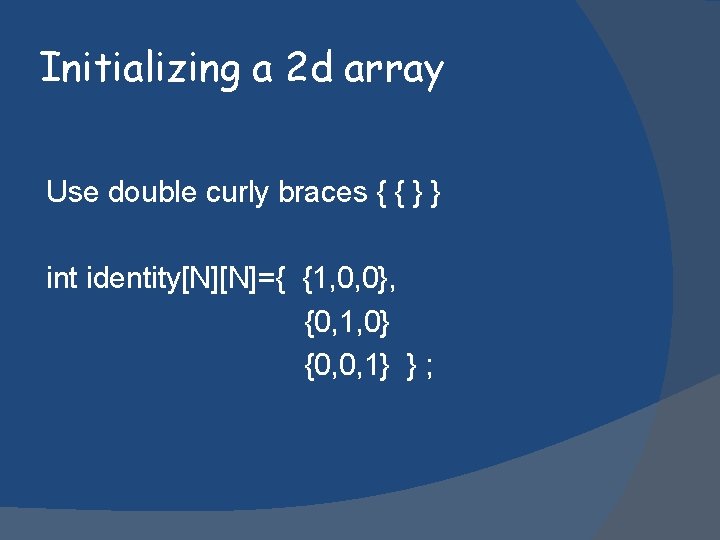
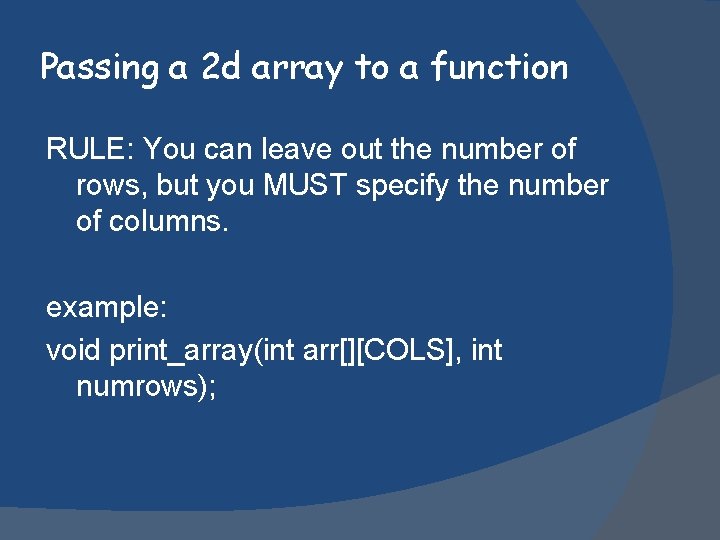
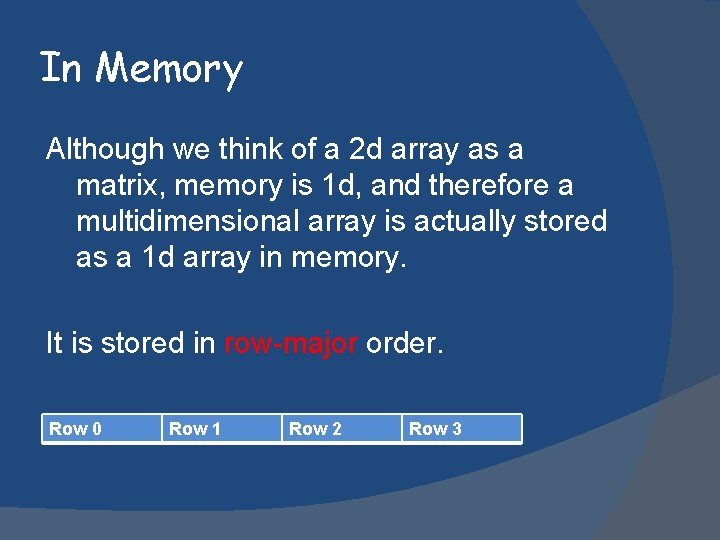
![Relating 2 d arrays to pointers (e. c. ) int arr[numrows][numcols]; arr[0] refers to Relating 2 d arrays to pointers (e. c. ) int arr[numrows][numcols]; arr[0] refers to](https://slidetodoc.com/presentation_image/83f383f171bf30144b4807b74d7f96d1/image-9.jpg)
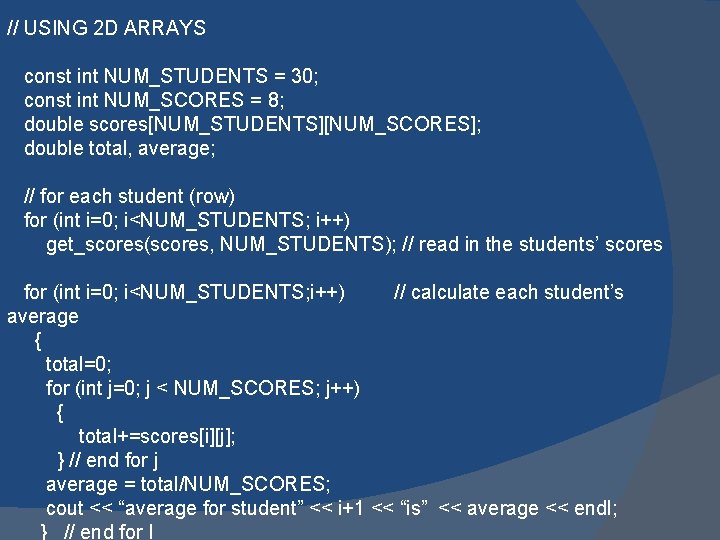
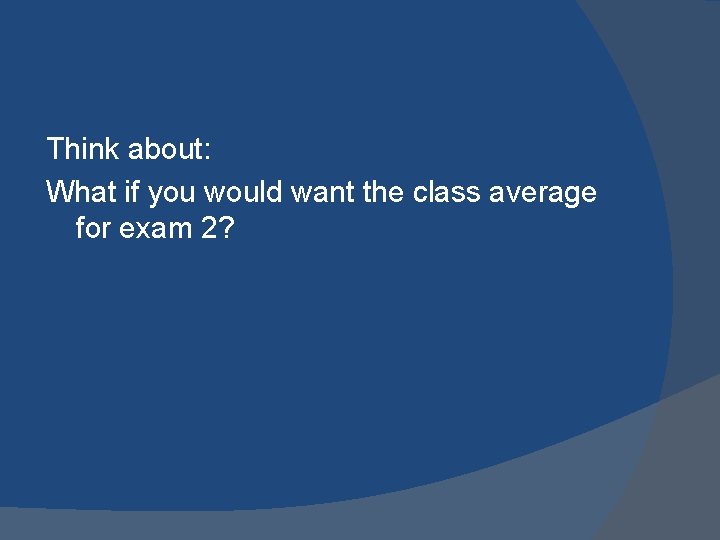
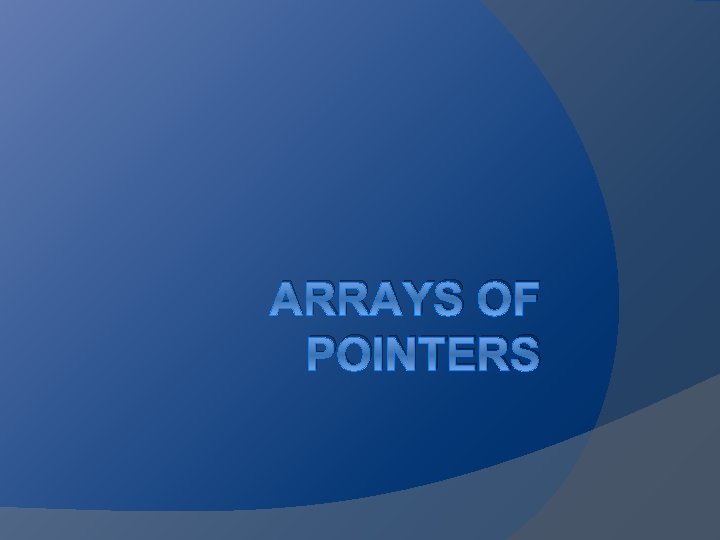
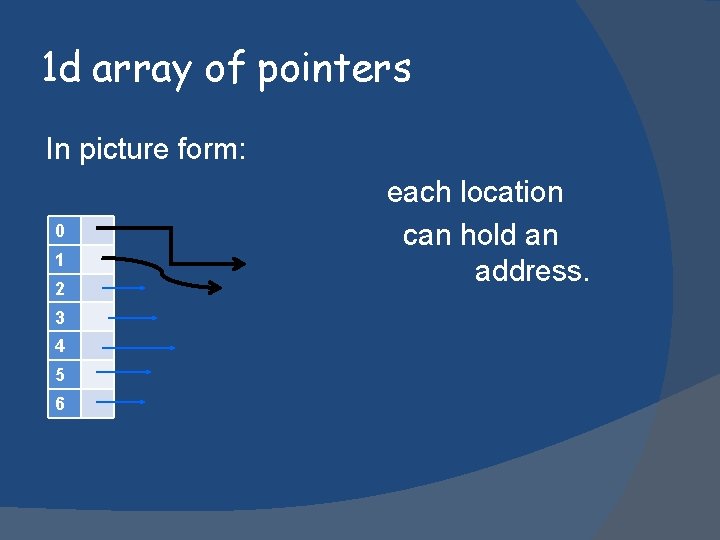
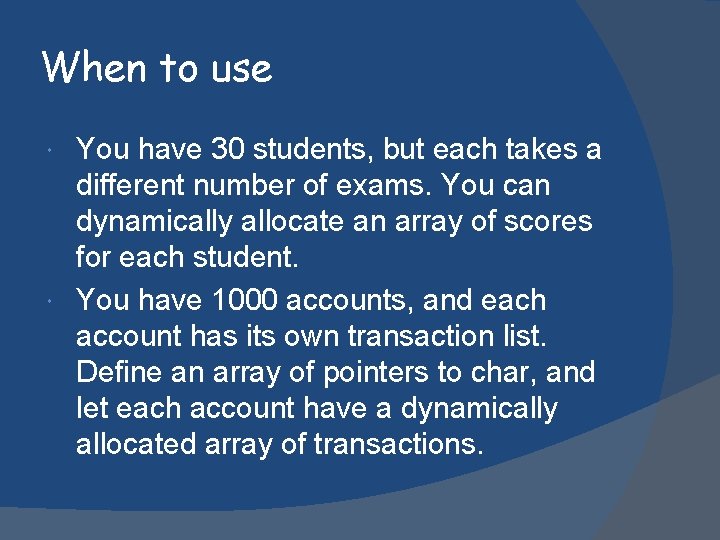
![How can we do that? ? char *transactions[NUM_ACCOUNTS]; // transactions is an array of How can we do that? ? char *transactions[NUM_ACCOUNTS]; // transactions is an array of](https://slidetodoc.com/presentation_image/83f383f171bf30144b4807b74d7f96d1/image-15.jpg)
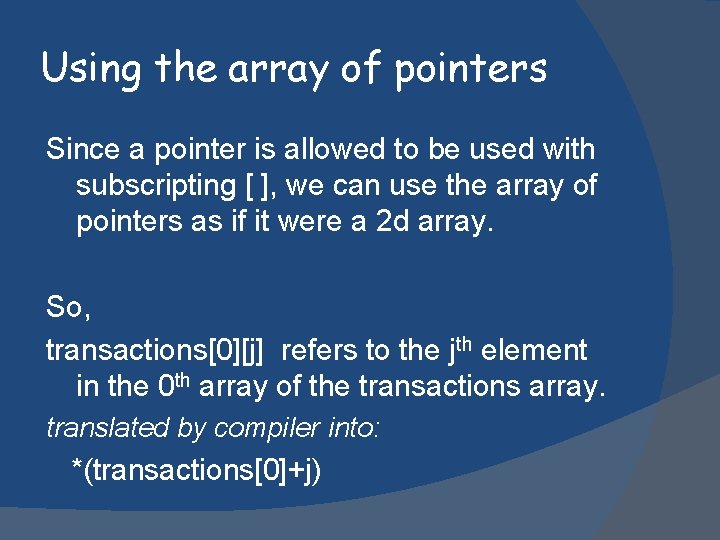
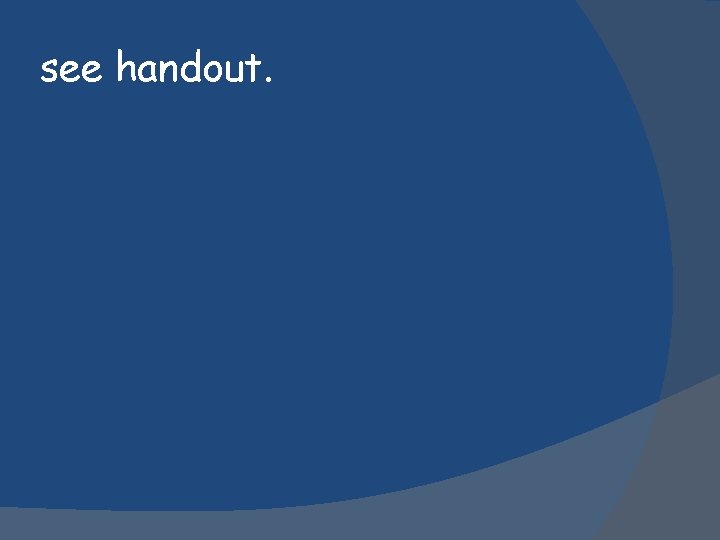
- Slides: 17
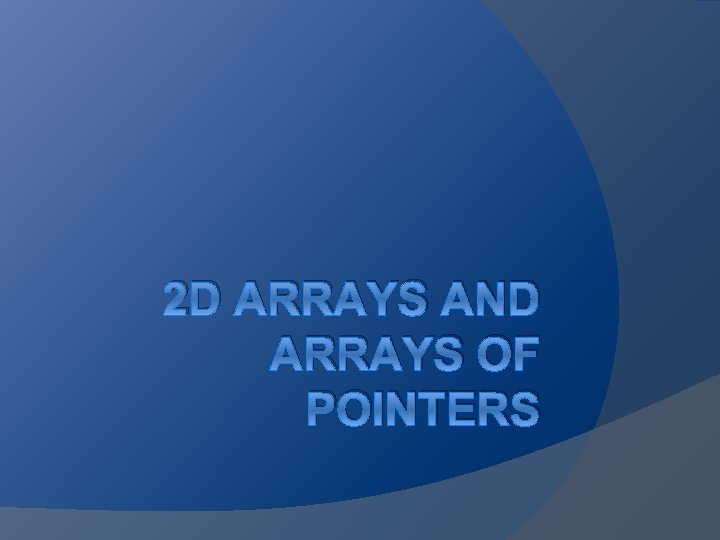
2 D ARRAYS AND ARRAYS OF POINTERS
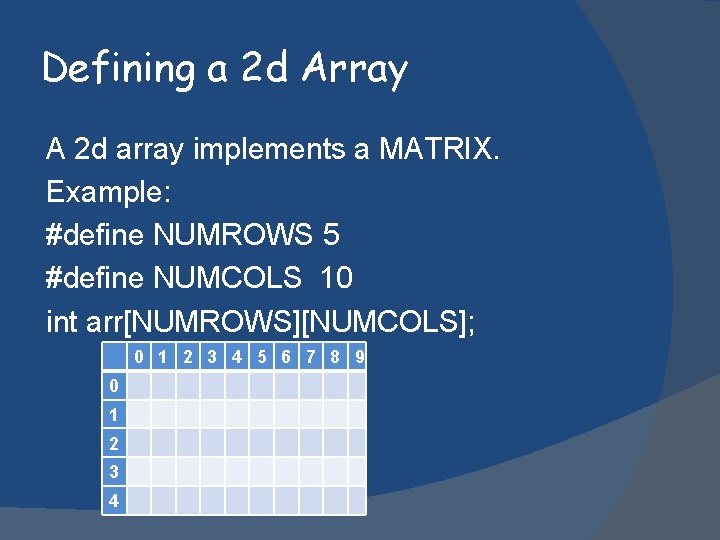
Defining a 2 d Array A 2 d array implements a MATRIX. Example: #define NUMROWS 5 #define NUMCOLS 10 int arr[NUMROWS][NUMCOLS]; 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4
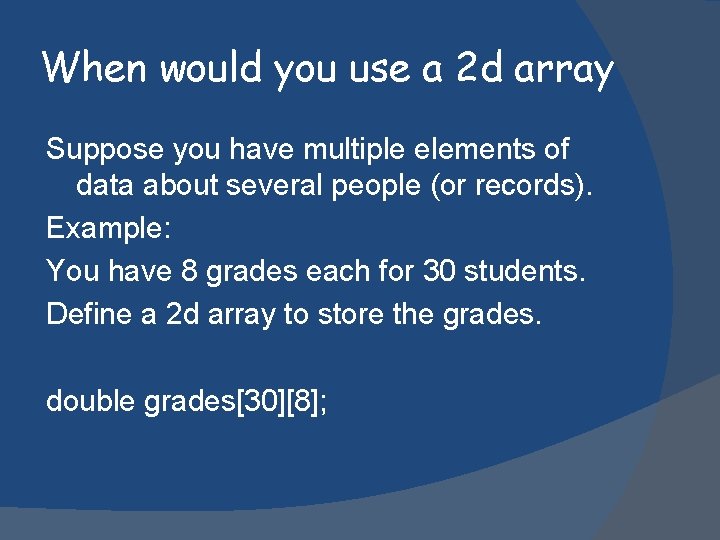
When would you use a 2 d array Suppose you have multiple elements of data about several people (or records). Example: You have 8 grades each for 30 students. Define a 2 d array to store the grades. double grades[30][8];
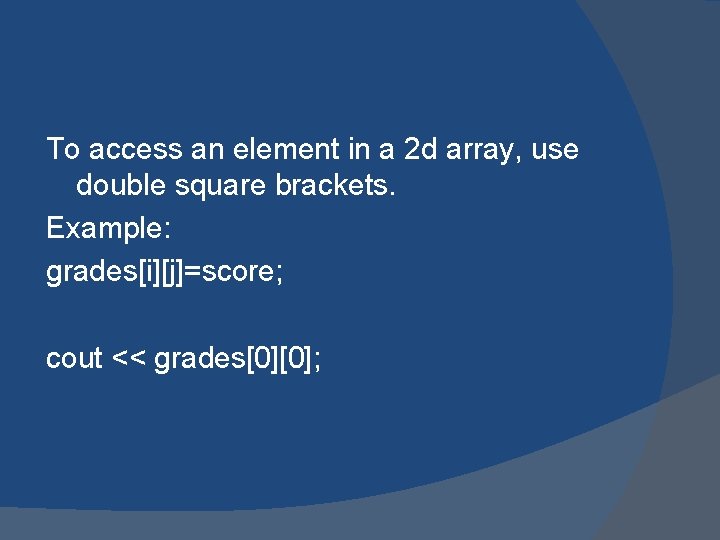
To access an element in a 2 d array, use double square brackets. Example: grades[i][j]=score; cout << grades[0][0];
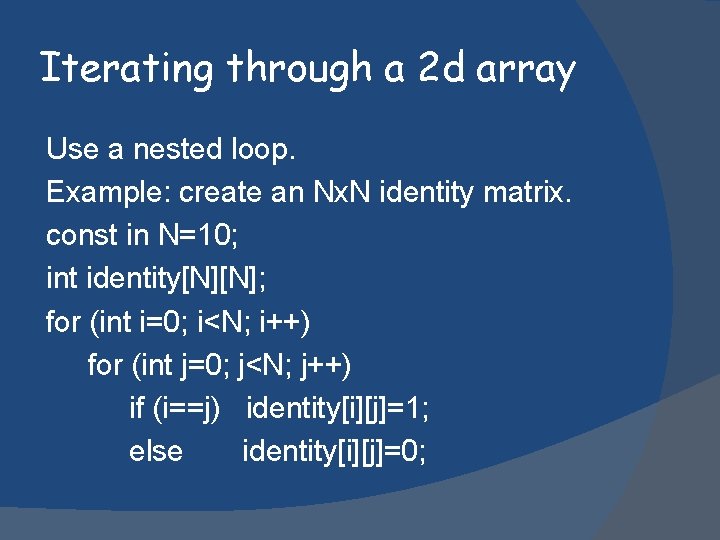
Iterating through a 2 d array Use a nested loop. Example: create an Nx. N identity matrix. const in N=10; int identity[N][N]; for (int i=0; i<N; i++) for (int j=0; j<N; j++) if (i==j) identity[i][j]=1; else identity[i][j]=0;
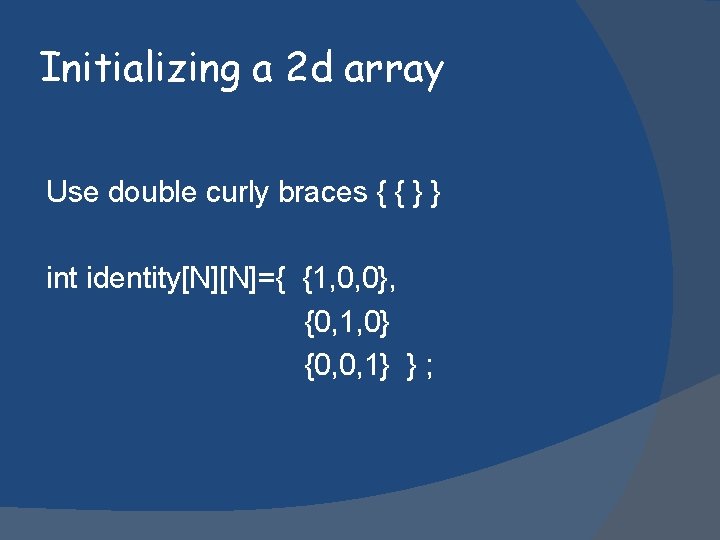
Initializing a 2 d array Use double curly braces { { } } int identity[N][N]={ {1, 0, 0}, {0, 1, 0} {0, 0, 1} } ;
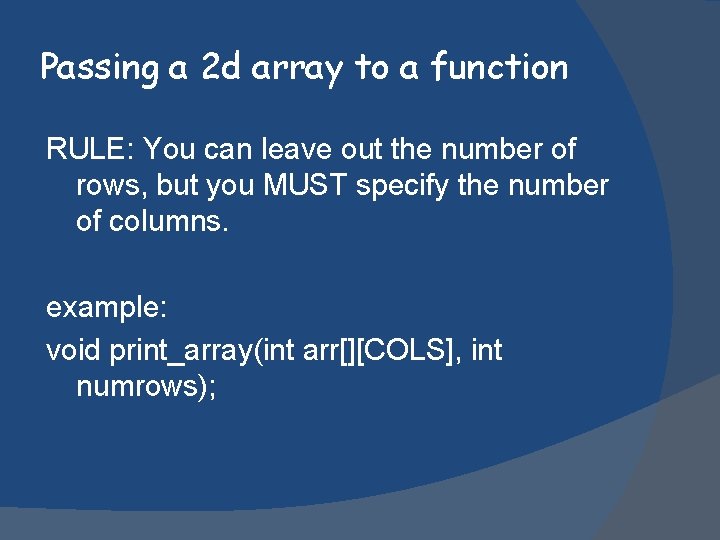
Passing a 2 d array to a function RULE: You can leave out the number of rows, but you MUST specify the number of columns. example: void print_array(int arr[][COLS], int numrows);
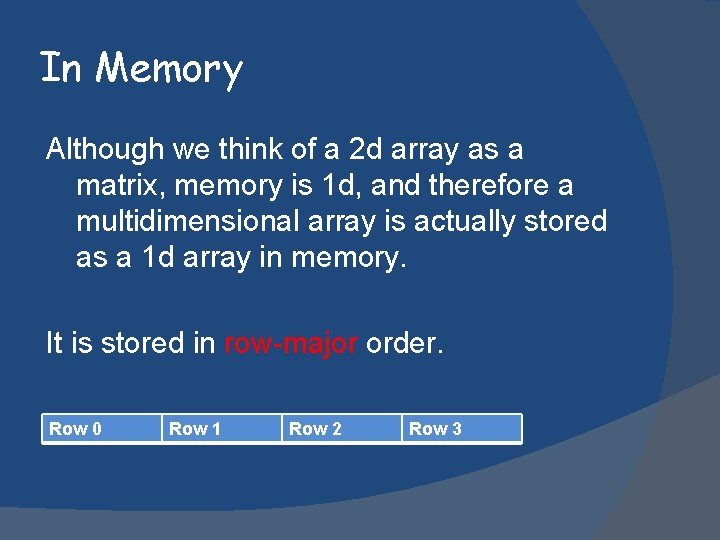
In Memory Although we think of a 2 d array as a matrix, memory is 1 d, and therefore a multidimensional array is actually stored as a 1 d array in memory. It is stored in row-major order. Row 0 Row 1 Row 2 Row 3
![Relating 2 d arrays to pointers e c int arrnumrowsnumcols arr0 refers to Relating 2 d arrays to pointers (e. c. ) int arr[numrows][numcols]; arr[0] refers to](https://slidetodoc.com/presentation_image/83f383f171bf30144b4807b74d7f96d1/image-9.jpg)
Relating 2 d arrays to pointers (e. c. ) int arr[numrows][numcols]; arr[0] refers to the address of row 0. That is, it is of type 1 d array (alternatively int*). So, *arr[0] is the contents of the first element of the first row (i. e. arr[0][0]). arr[i][j] is translated by the compiler into: *(arr[i]+j) *(*(arr+i)+j)
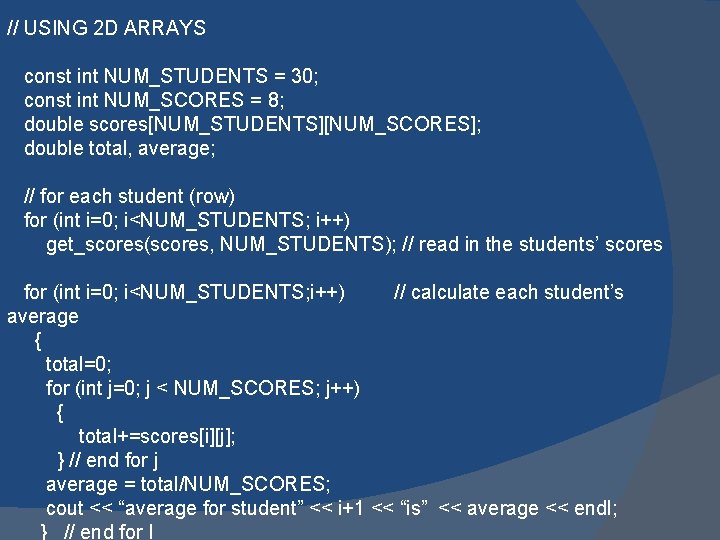
// USING 2 D ARRAYS const int NUM_STUDENTS = 30; const int NUM_SCORES = 8; double scores[NUM_STUDENTS][NUM_SCORES]; double total, average; // for each student (row) for (int i=0; i<NUM_STUDENTS; i++) get_scores(scores, NUM_STUDENTS); // read in the students’ scores for (int i=0; i<NUM_STUDENTS; i++) // calculate each student’s average { total=0; for (int j=0; j < NUM_SCORES; j++) { total+=scores[i][j]; } // end for j average = total/NUM_SCORES; cout << “average for student” << i+1 << “is” << average << endl; } // end for I
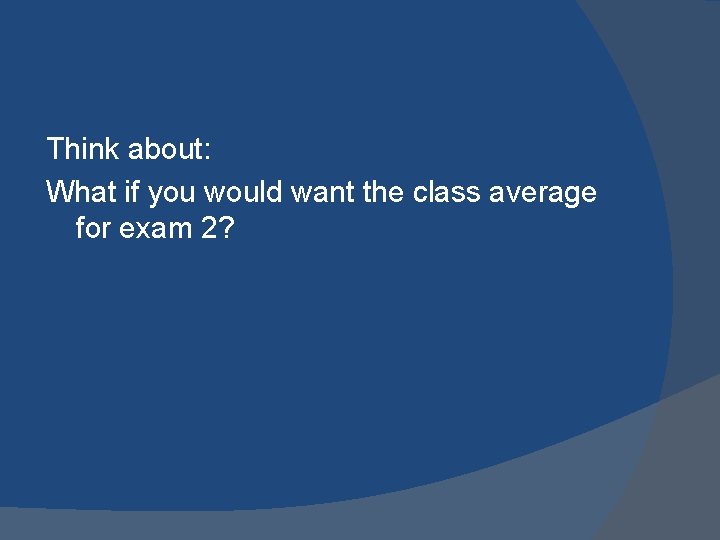
Think about: What if you would want the class average for exam 2?
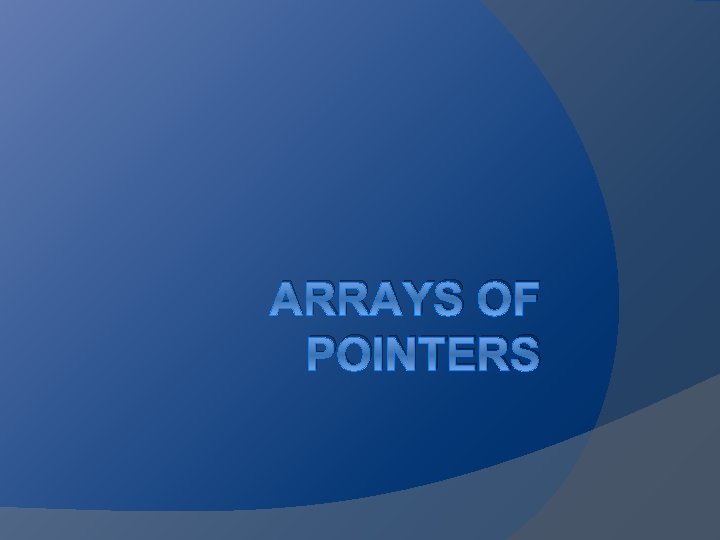
ARRAYS OF POINTERS
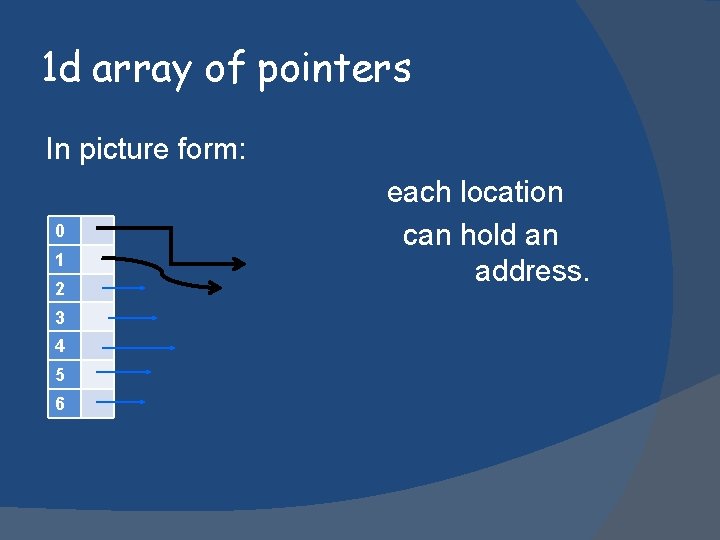
1 d array of pointers In picture form: each location 0 can hold an 1 address. 2 3 4 5 6
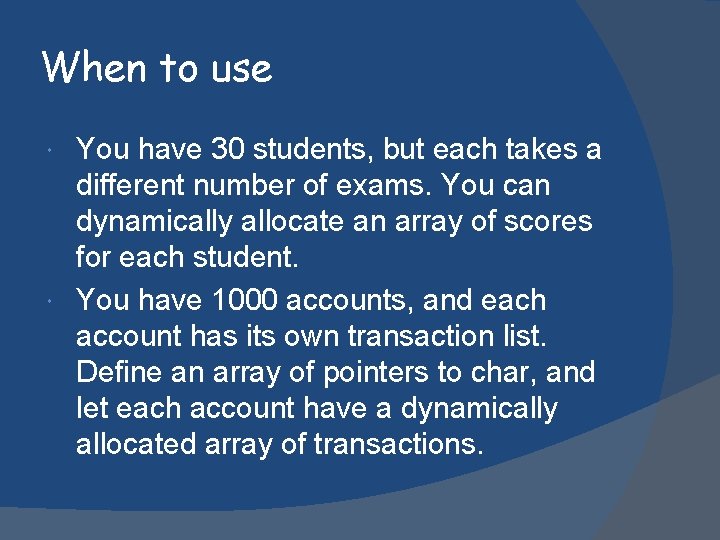
When to use You have 30 students, but each takes a different number of exams. You can dynamically allocate an array of scores for each student. You have 1000 accounts, and each account has its own transaction list. Define an array of pointers to char, and let each account have a dynamically allocated array of transactions.
![How can we do that char transactionsNUMACCOUNTS transactions is an array of How can we do that? ? char *transactions[NUM_ACCOUNTS]; // transactions is an array of](https://slidetodoc.com/presentation_image/83f383f171bf30144b4807b74d7f96d1/image-15.jpg)
How can we do that? ? char *transactions[NUM_ACCOUNTS]; // transactions is an array of type pointer to char transactions[0] = new char [transnumber]; // now, the first location of the transactions array points to the beginning of a dynamically allocated array.
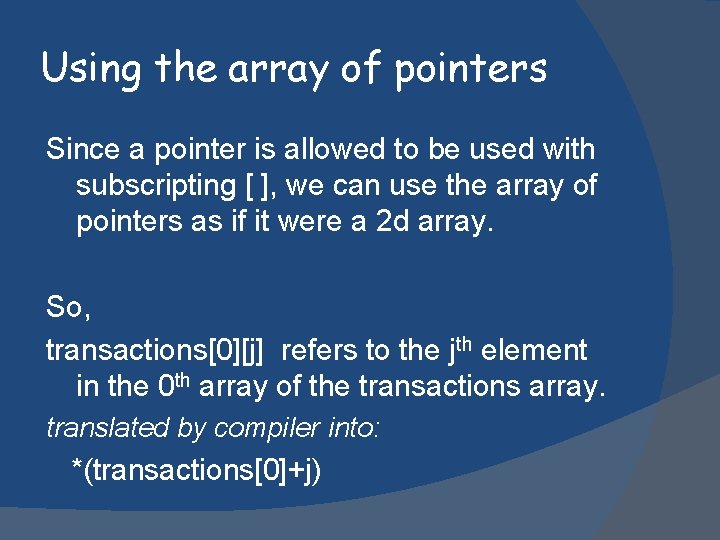
Using the array of pointers Since a pointer is allowed to be used with subscripting [ ], we can use the array of pointers as if it were a 2 d array. So, transactions[0][j] refers to the jth element in the 0 th array of the transactions array. translated by compiler into: *(transactions[0]+j)
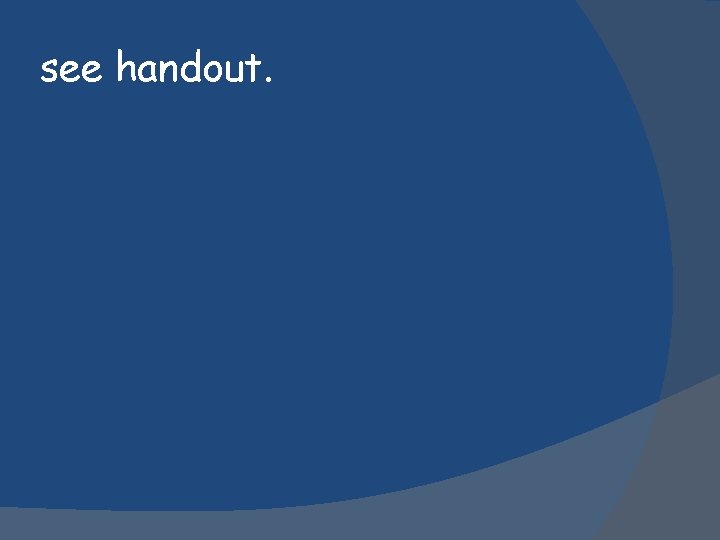
see handout.
Non defining clause examples
Relative clauses defining and non defining
Defining non defining relative clauses
Defining and non defining relative clauses in telugu
Define and non defining relative clauses
Non-defining relative clauses cümleleri
Pointers and strings
Java pointers and references
& vs * in c
Dynamic arrays and amortized analysis
Searching and sorting arrays in c++
Array advantages and disadvantages
Advantages of pointers
Significance of pointer
C array of pointers to structs
Xp1024xp
Which is a good idea for using skip pointers
Skip pointer