2 ADT Stack 0 S Stack item Element
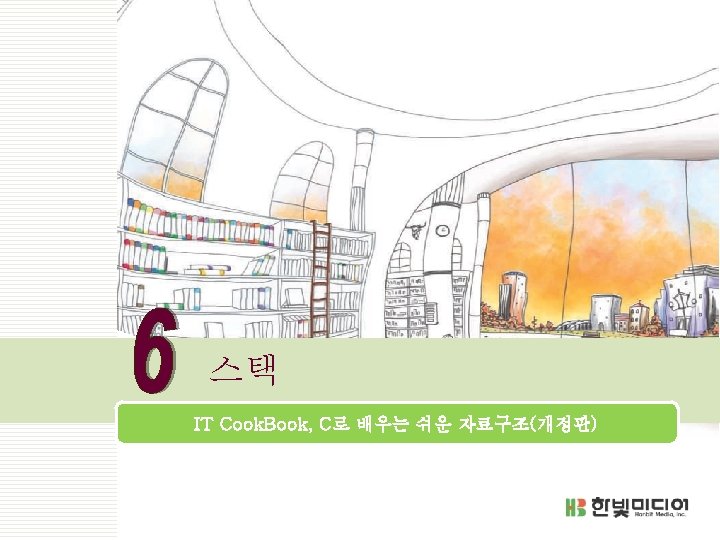
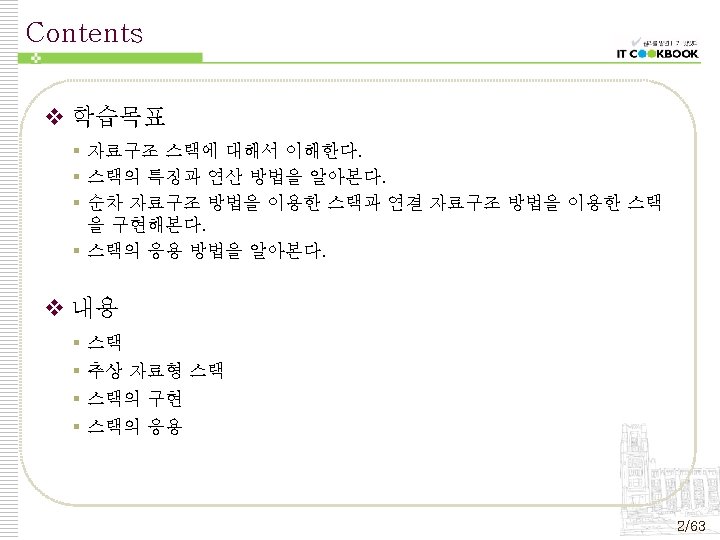
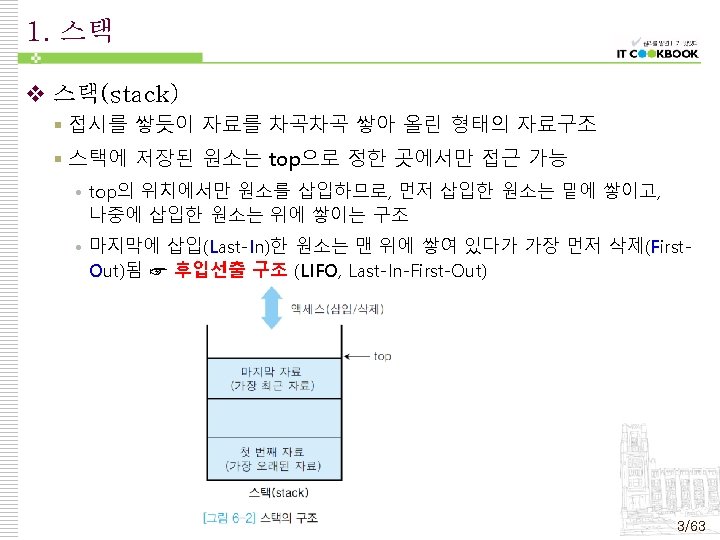
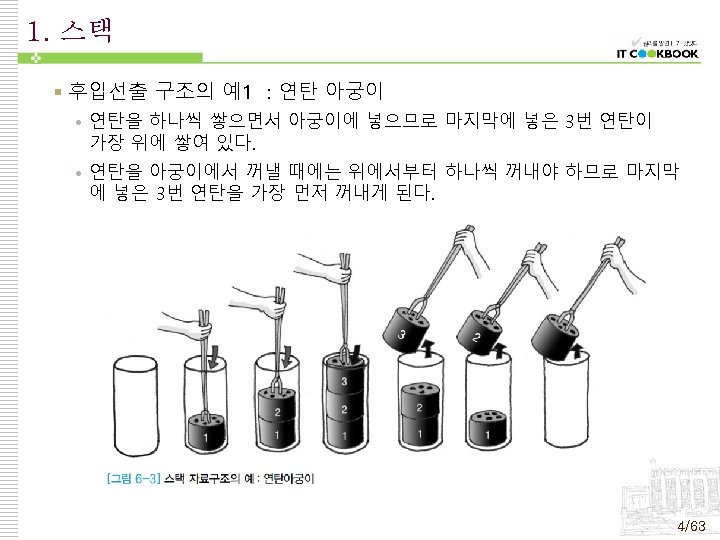
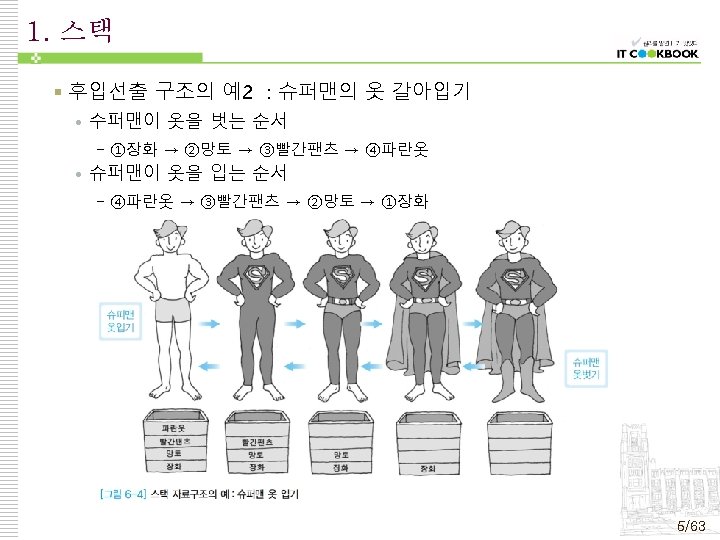
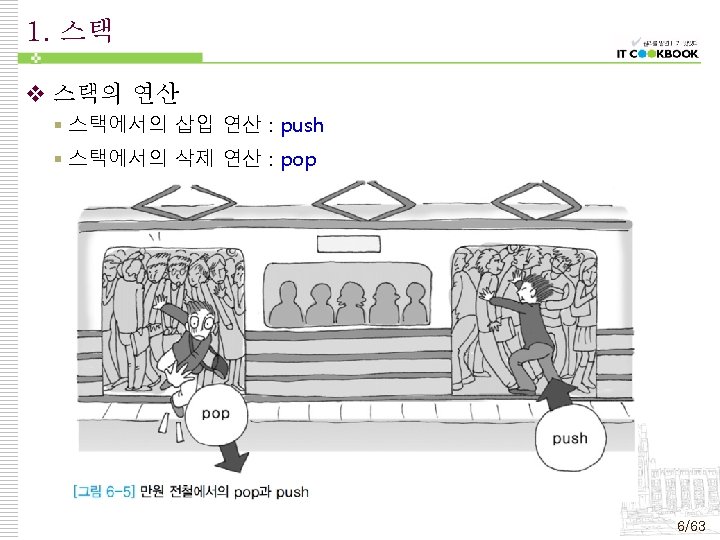
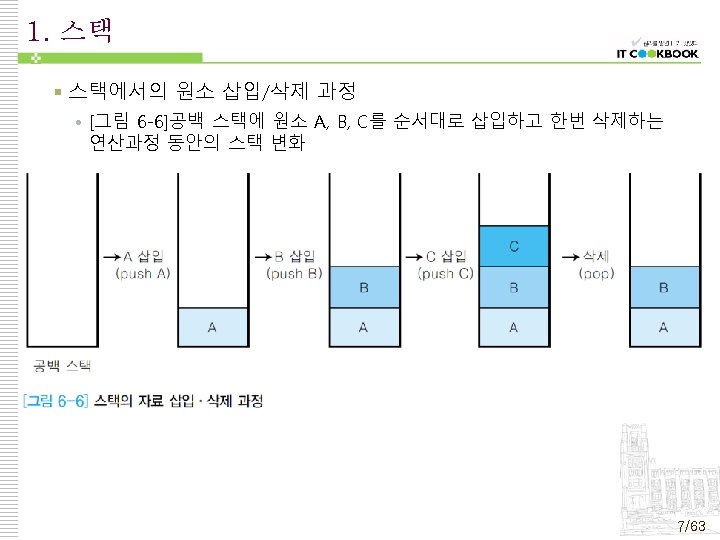
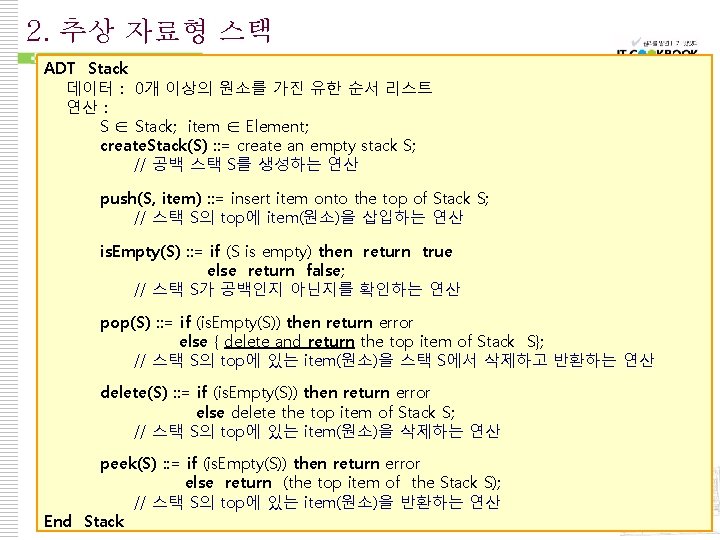
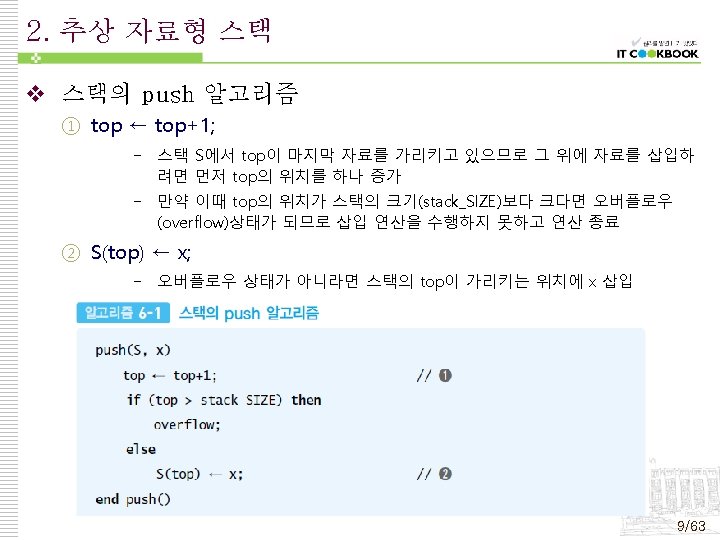
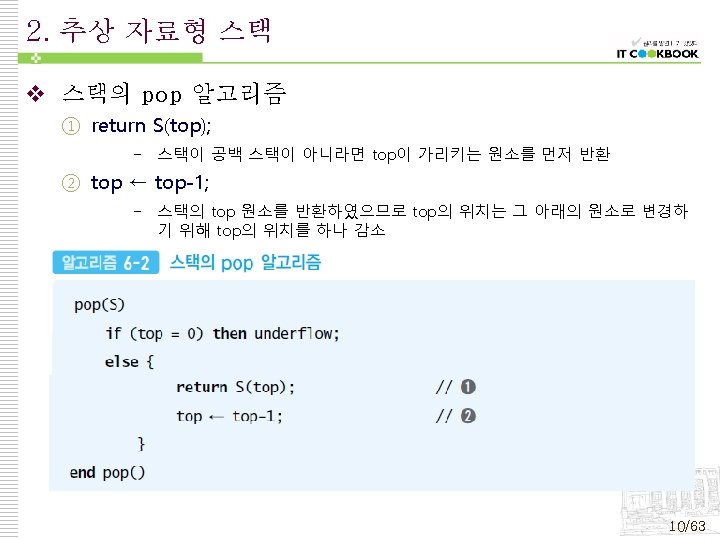
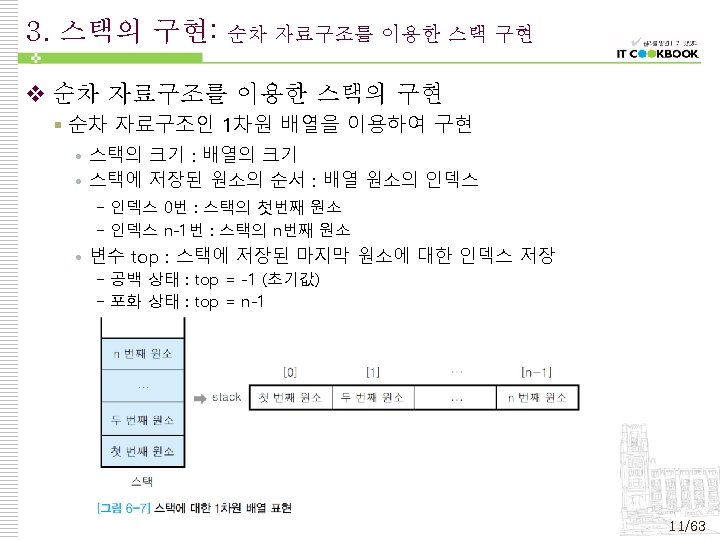
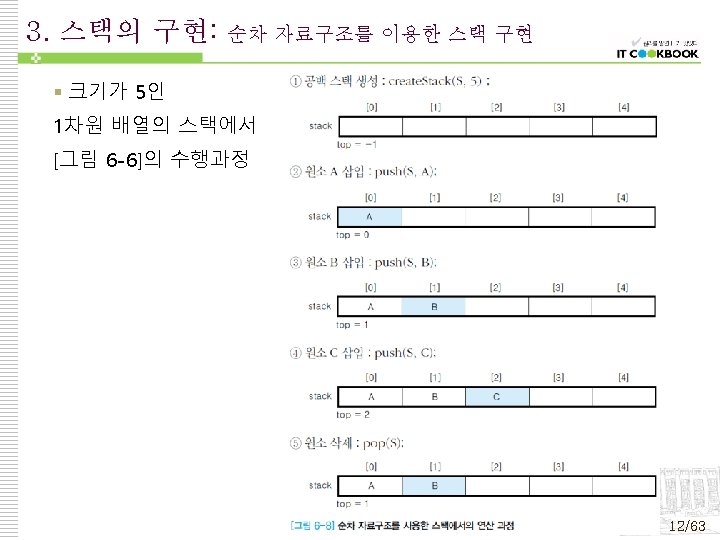
![3. 스택의 구현: [예제 6 -1] § 순차 자료구조를 이용한 스택 프로그램 01 02 3. 스택의 구현: [예제 6 -1] § 순차 자료구조를 이용한 스택 프로그램 01 02](https://slidetodoc.com/presentation_image_h2/7c694be2007e3644f7039628ea8483fd/image-13.jpg)
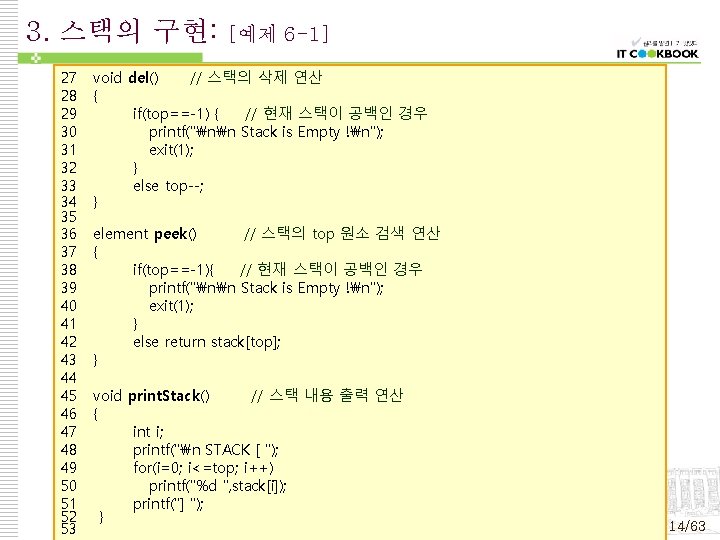
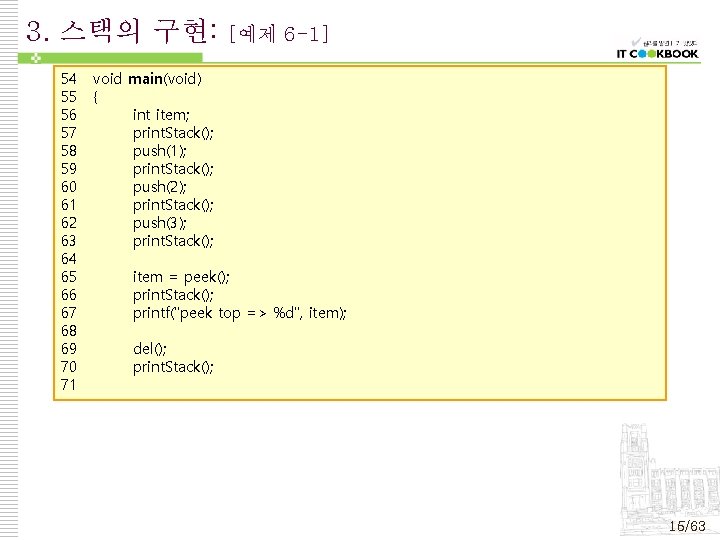
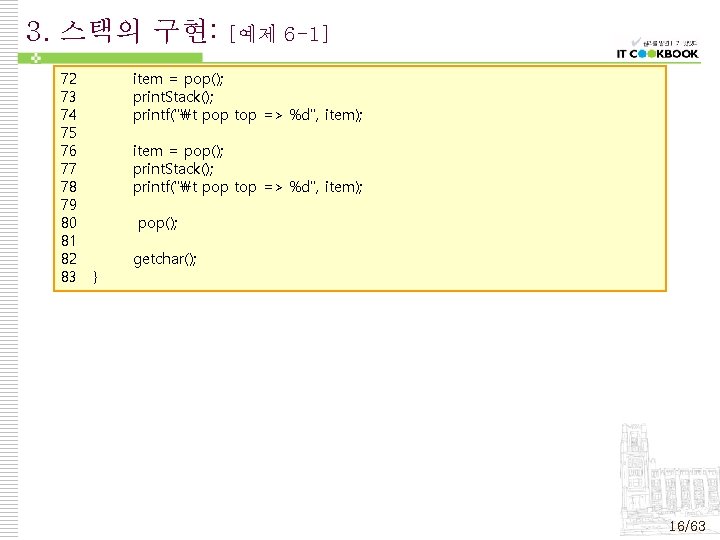
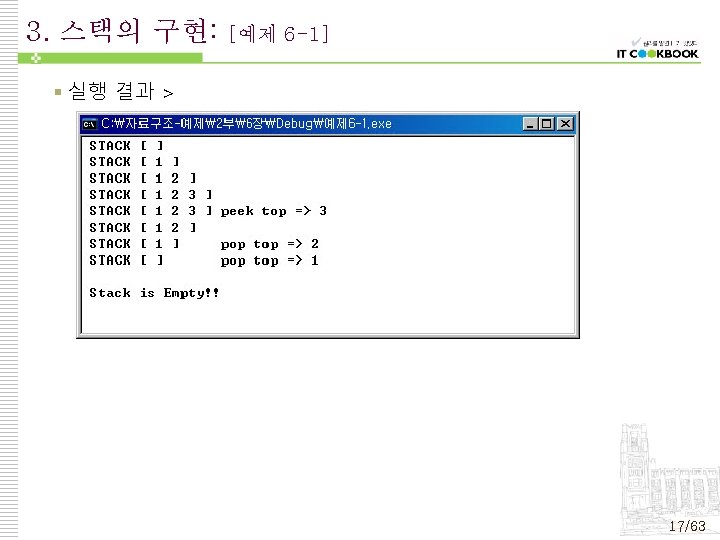
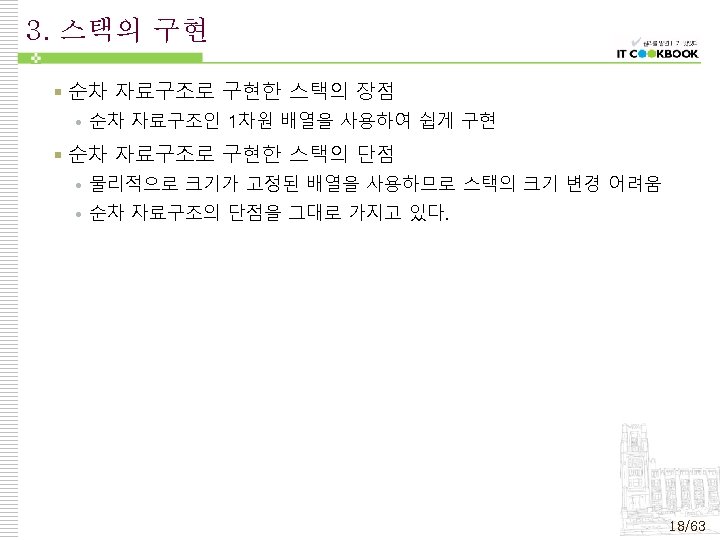
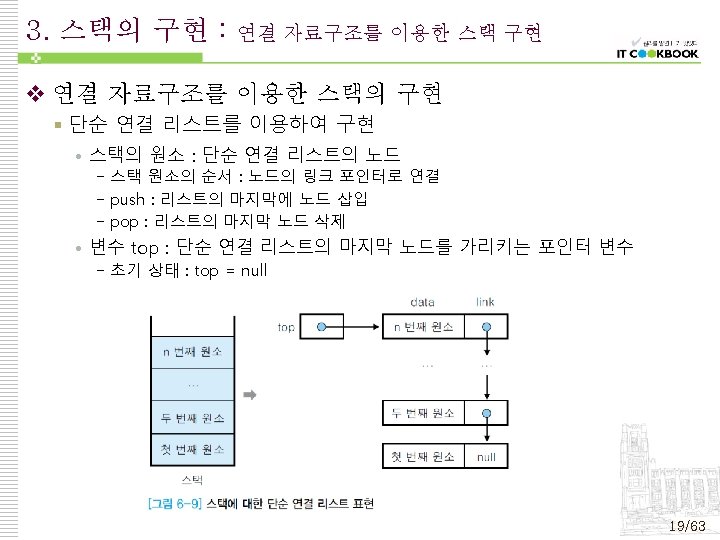
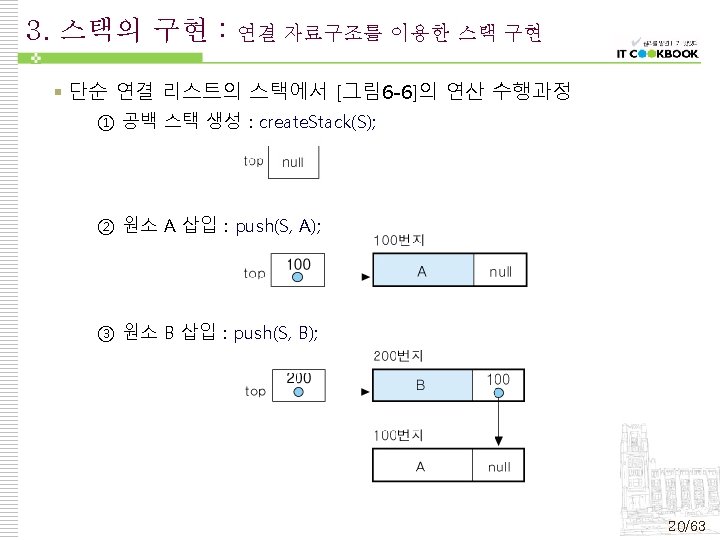
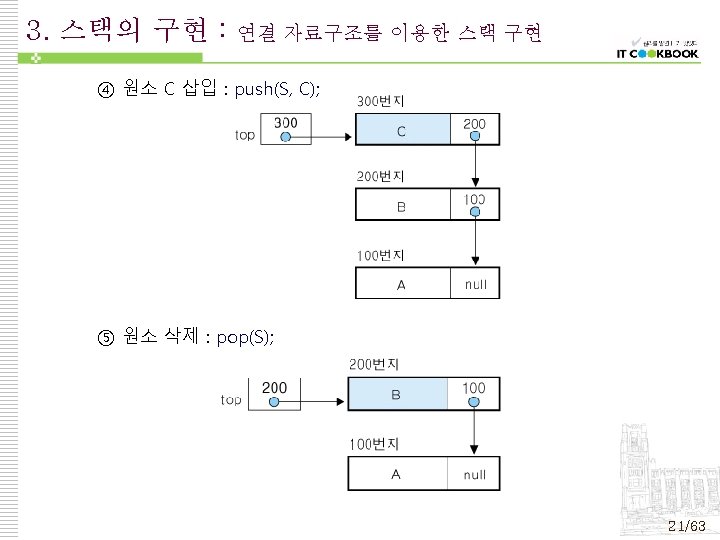
![3. 스택의 구현 : [예제 6 -2] § 단순 연결 리스트를 이용한 스택 프로그램 3. 스택의 구현 : [예제 6 -2] § 단순 연결 리스트를 이용한 스택 프로그램](https://slidetodoc.com/presentation_image_h2/7c694be2007e3644f7039628ea8483fd/image-22.jpg)
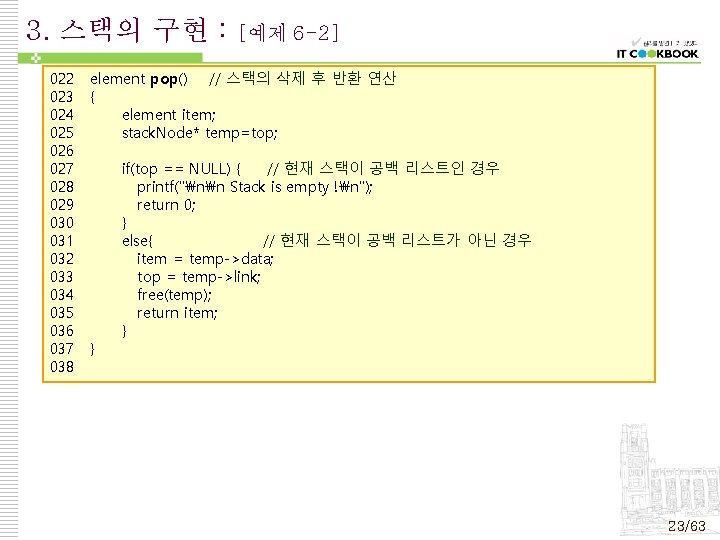
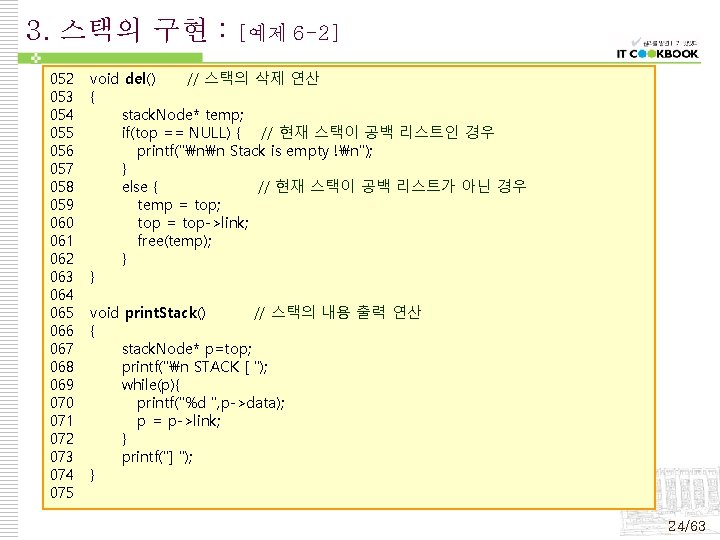
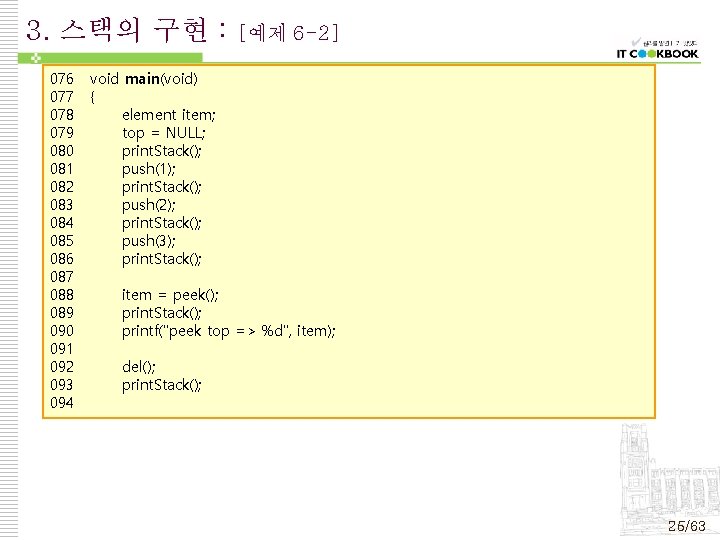
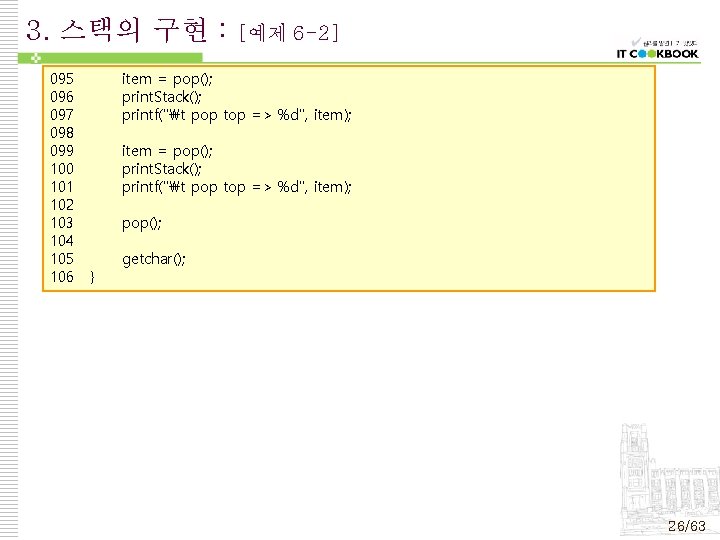
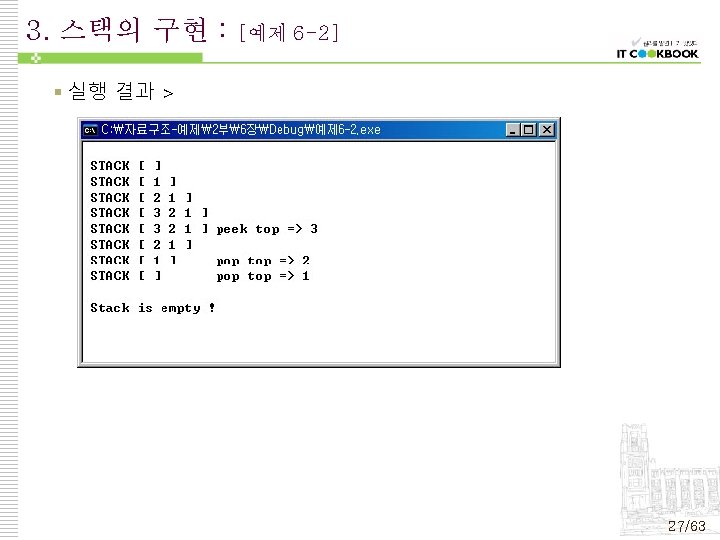
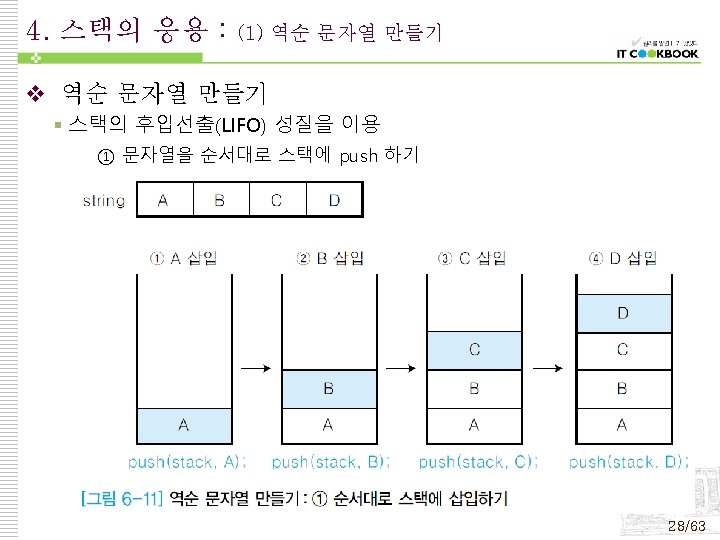
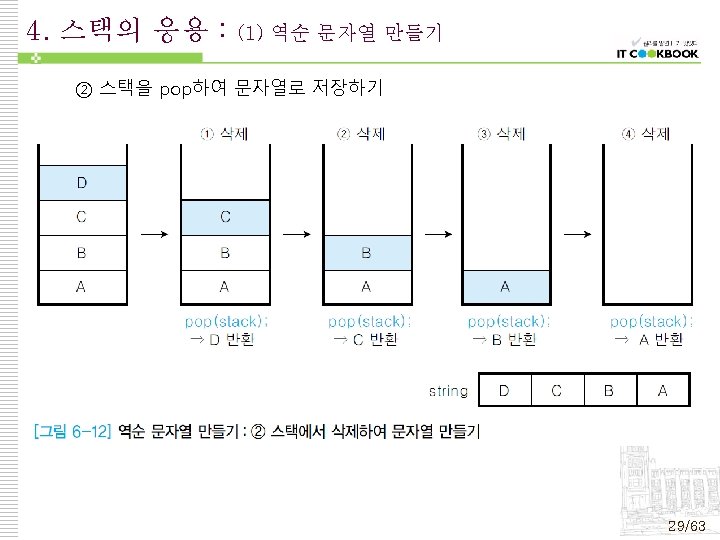
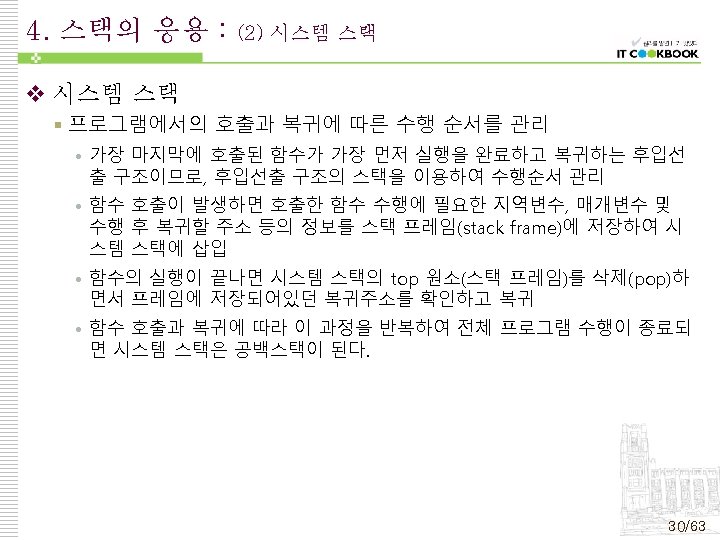
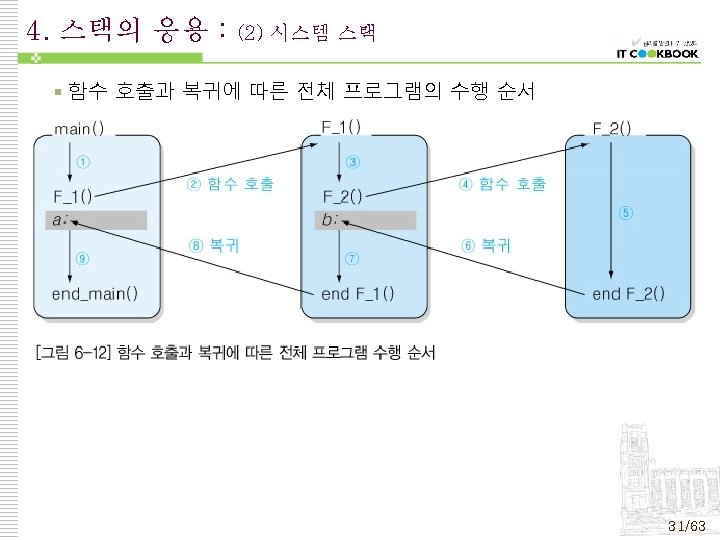
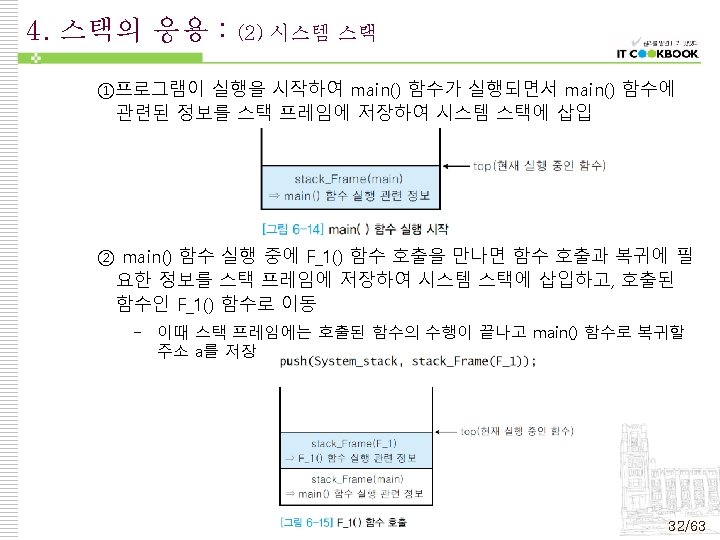
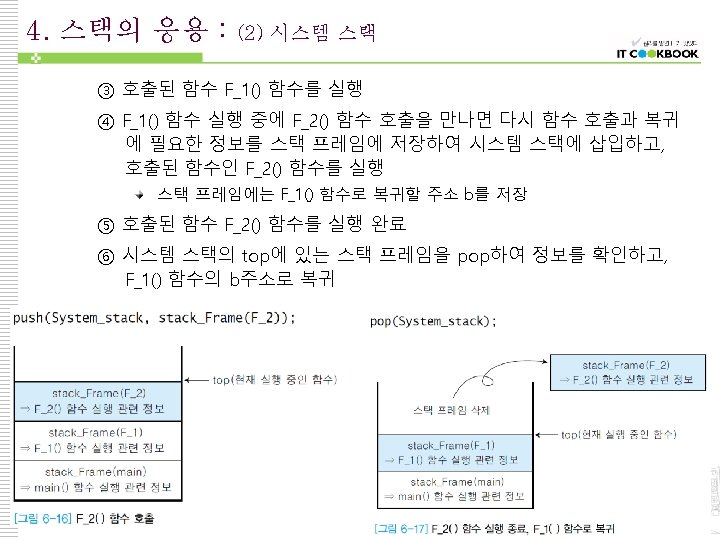
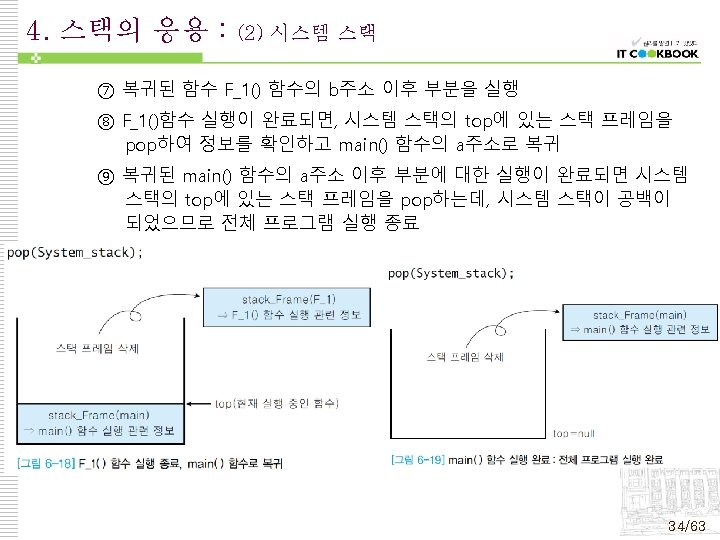
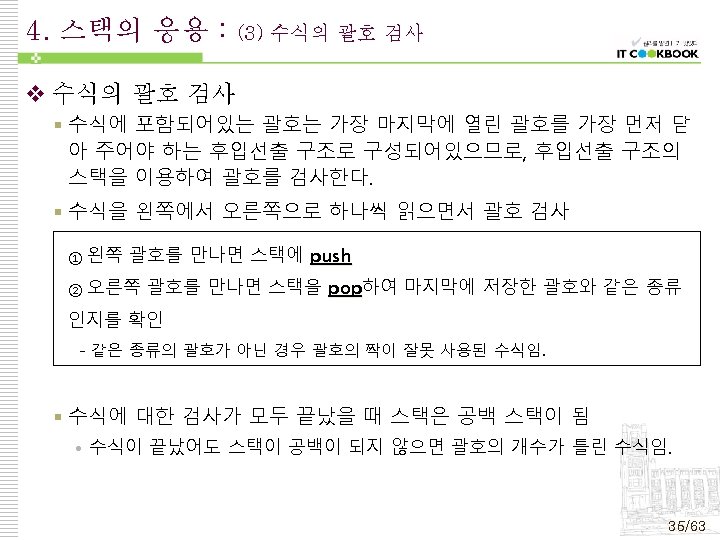
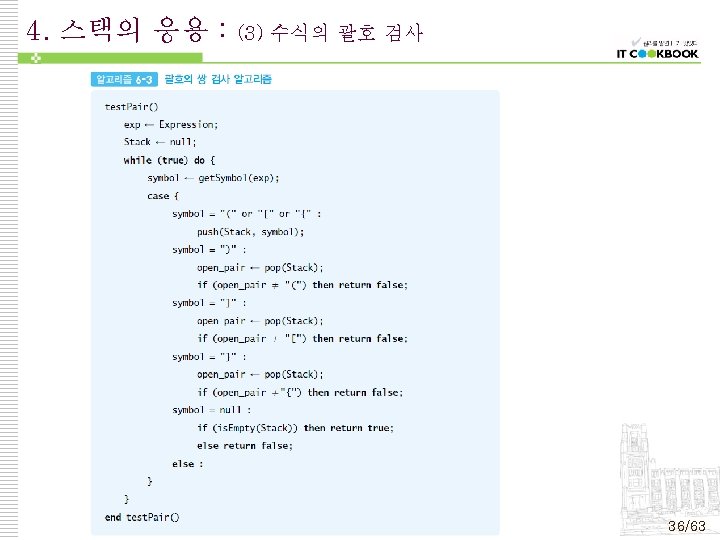
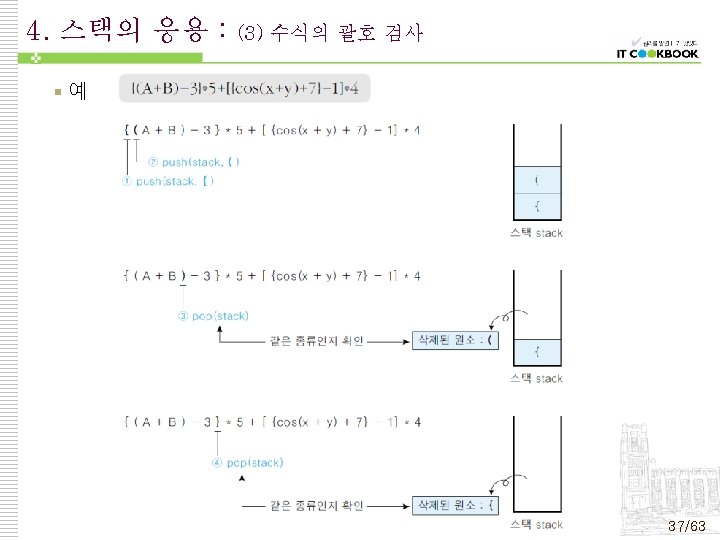
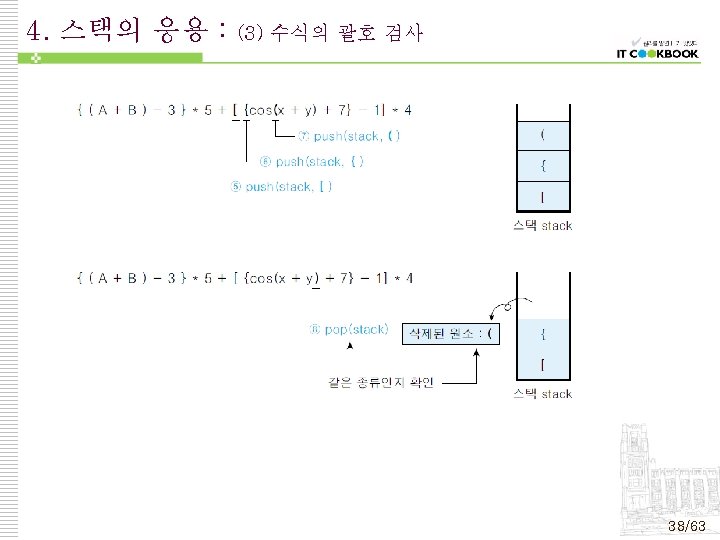
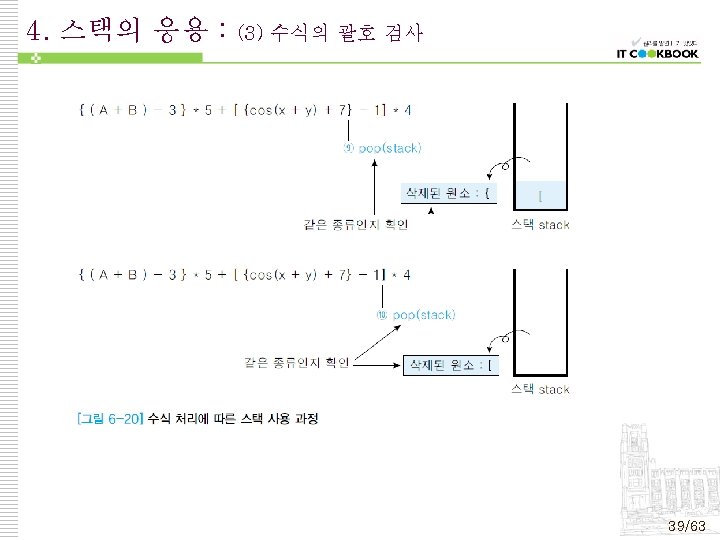
![4. 스택의 응용 : (3) 수식의 괄호 검사 – [예제 6 -3] § 수식의 4. 스택의 응용 : (3) 수식의 괄호 검사 – [예제 6 -3] § 수식의](https://slidetodoc.com/presentation_image_h2/7c694be2007e3644f7039628ea8483fd/image-40.jpg)
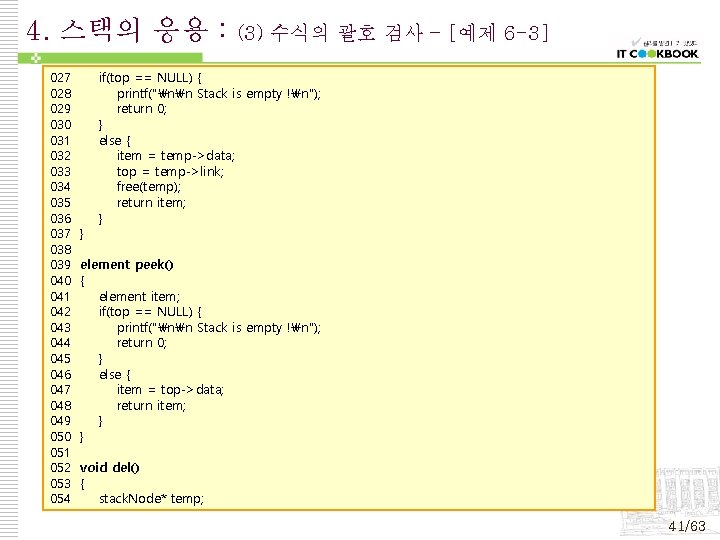
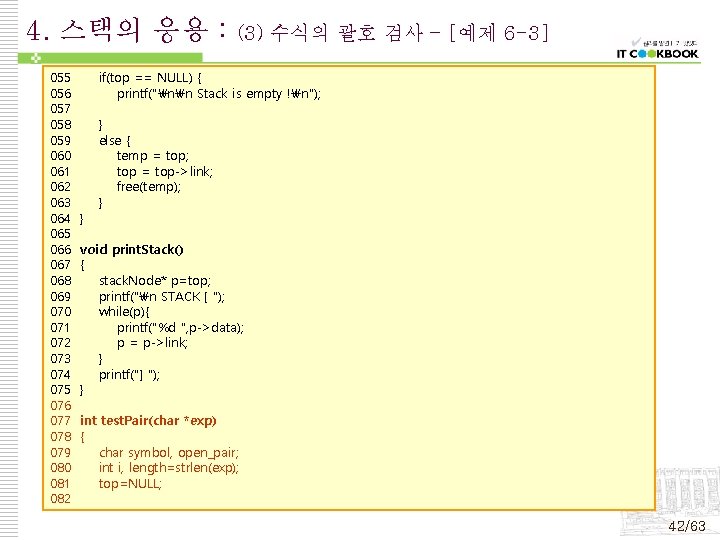
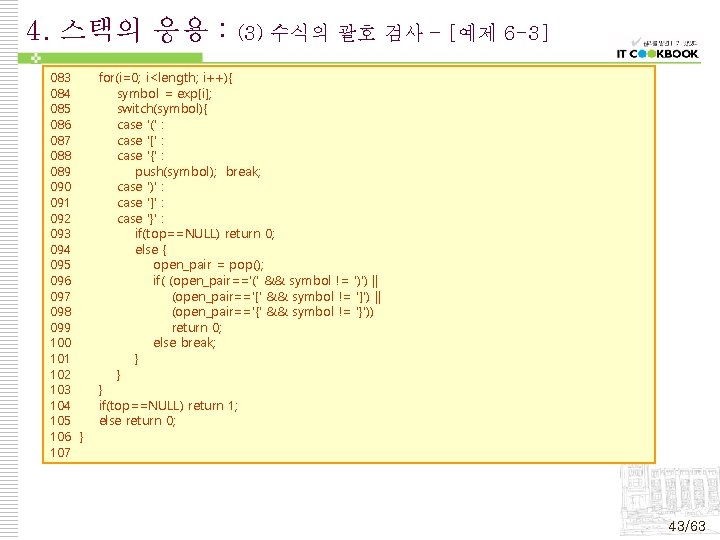
![4. 스택의 응용 : (3) 수식의 괄호 검사 – [예제 6 -3] 108 109 4. 스택의 응용 : (3) 수식의 괄호 검사 – [예제 6 -3] 108 109](https://slidetodoc.com/presentation_image_h2/7c694be2007e3644f7039628ea8483fd/image-44.jpg)
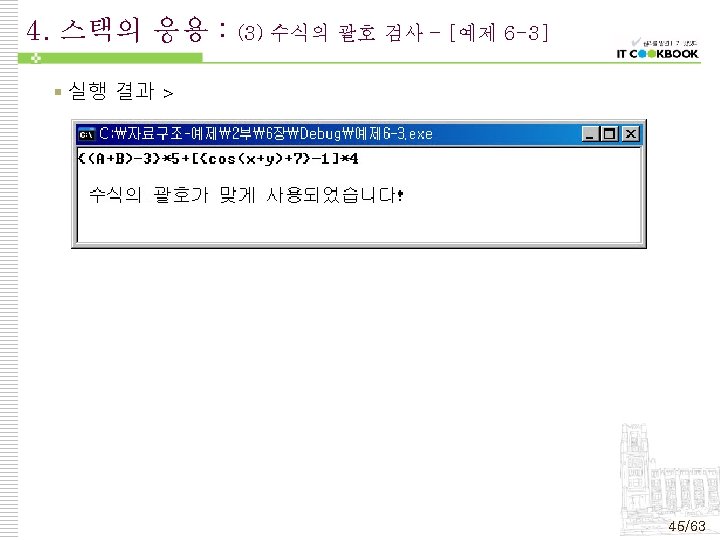
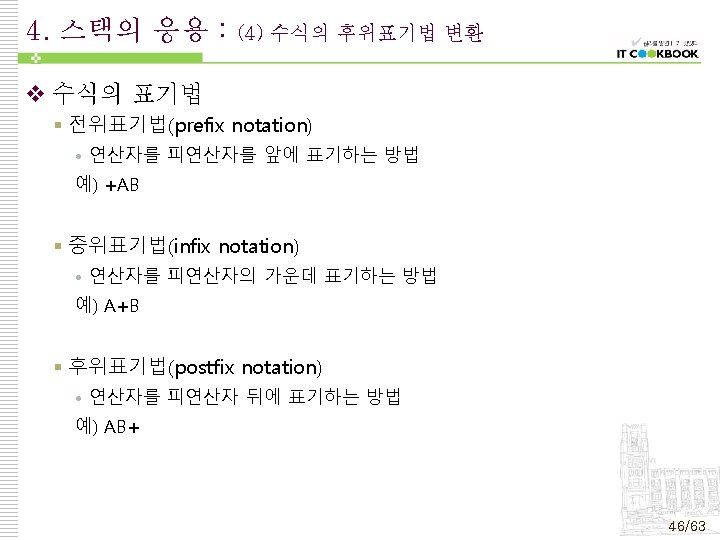
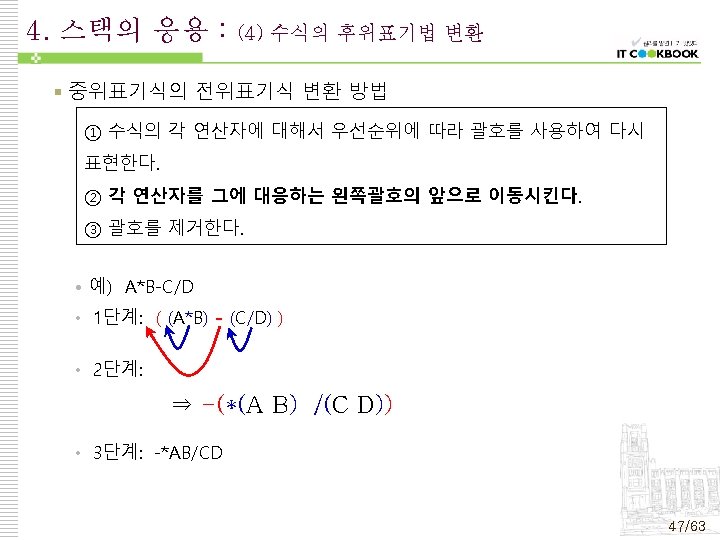
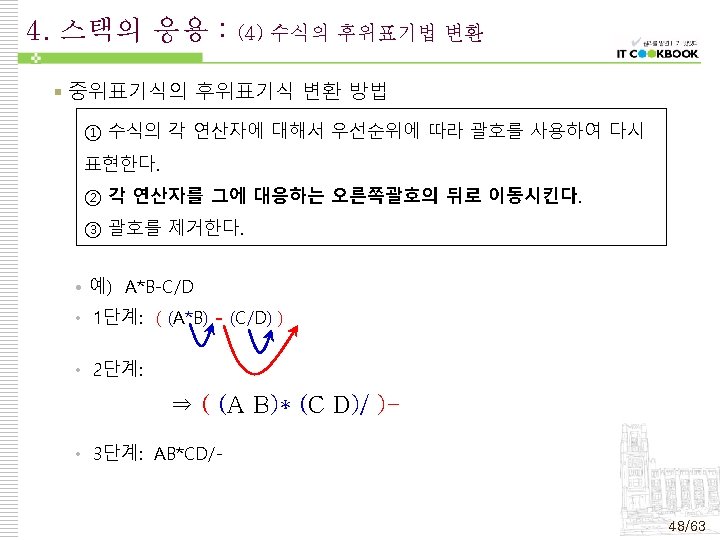
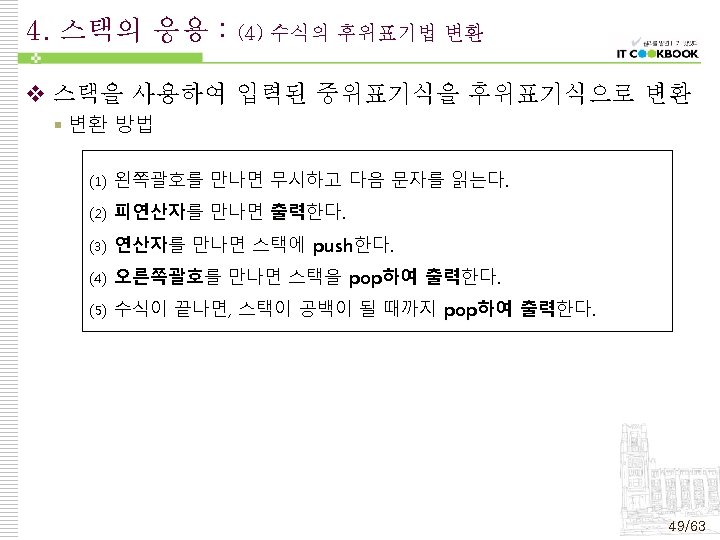
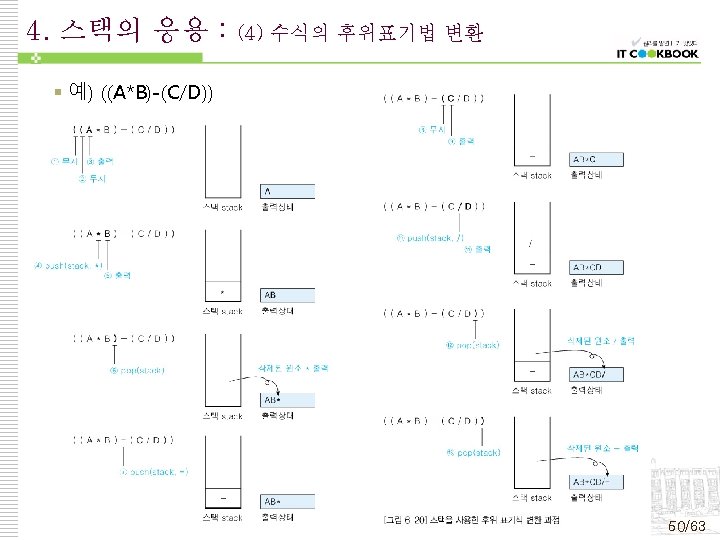
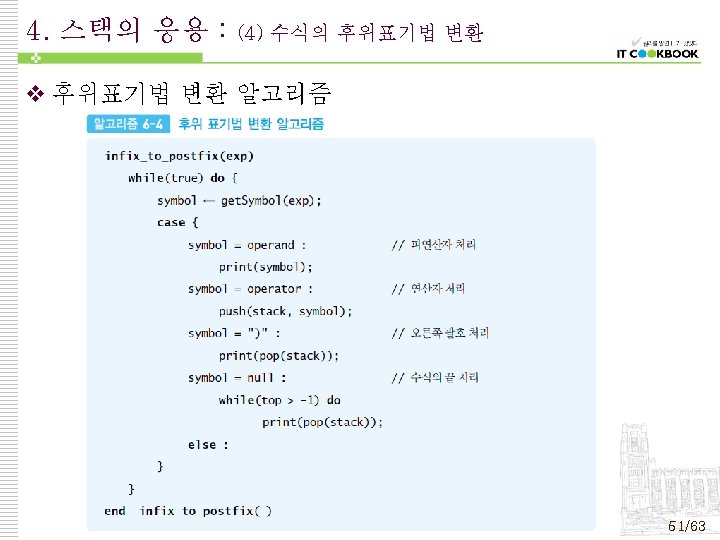
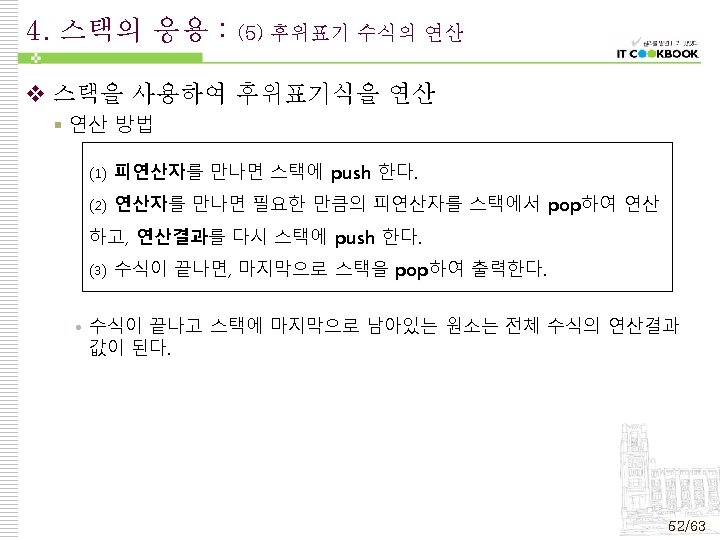
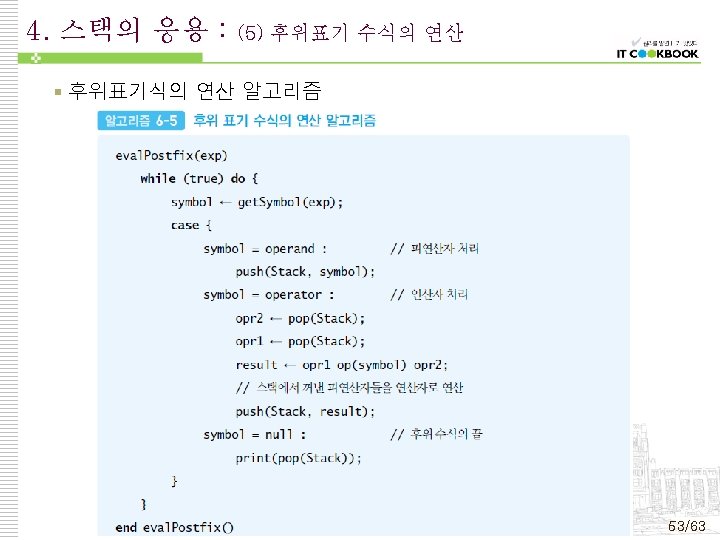
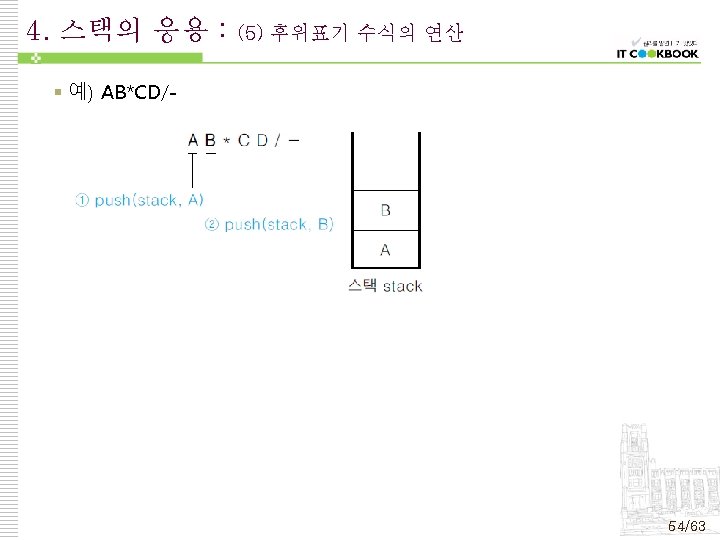
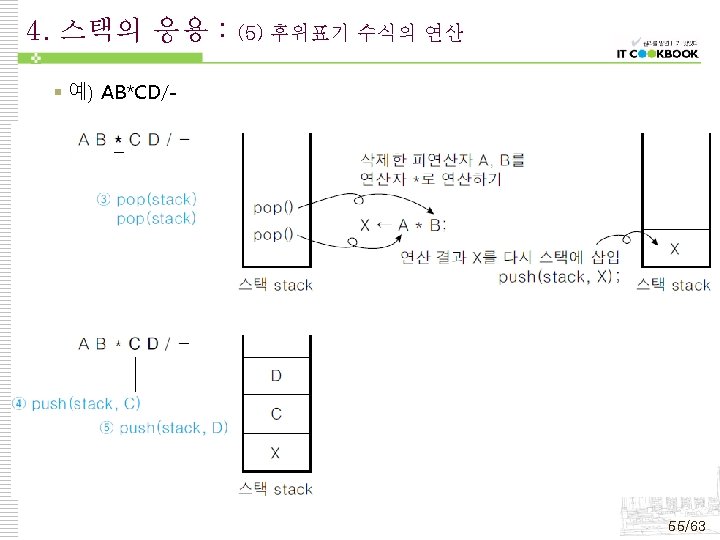
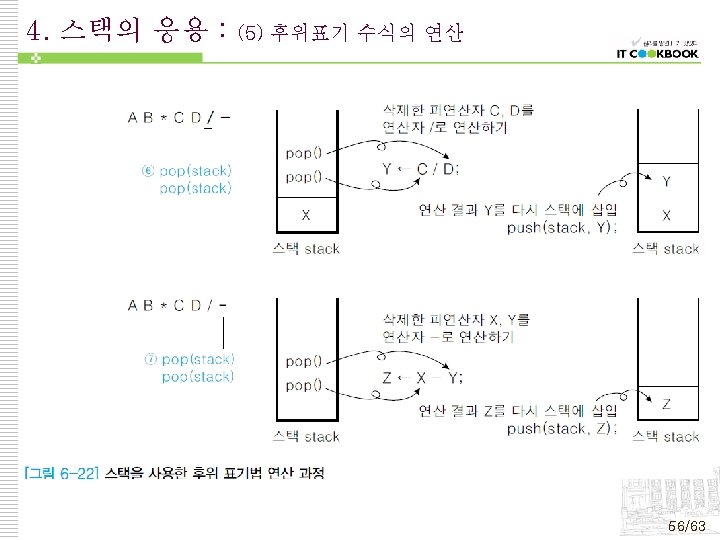
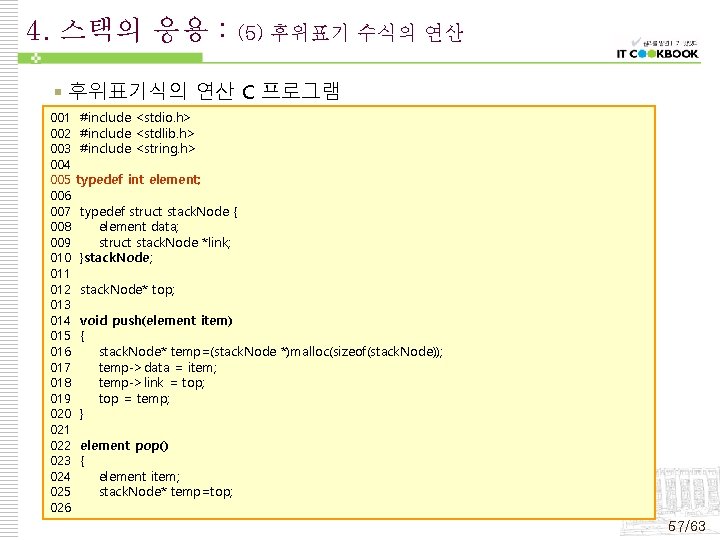
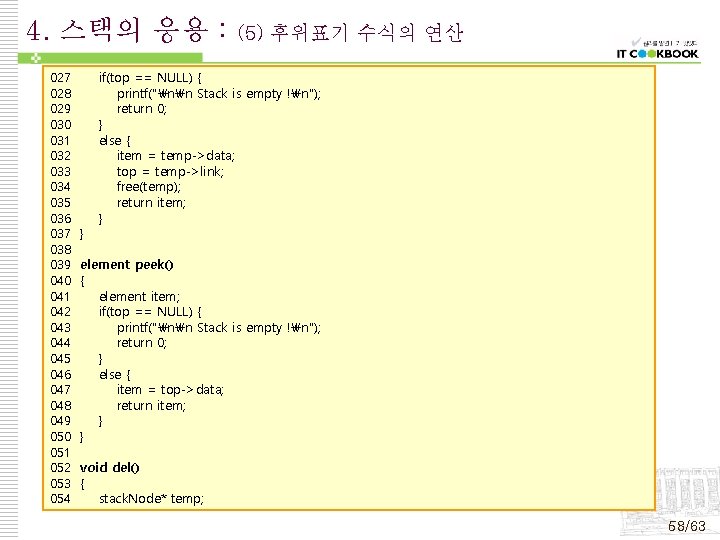
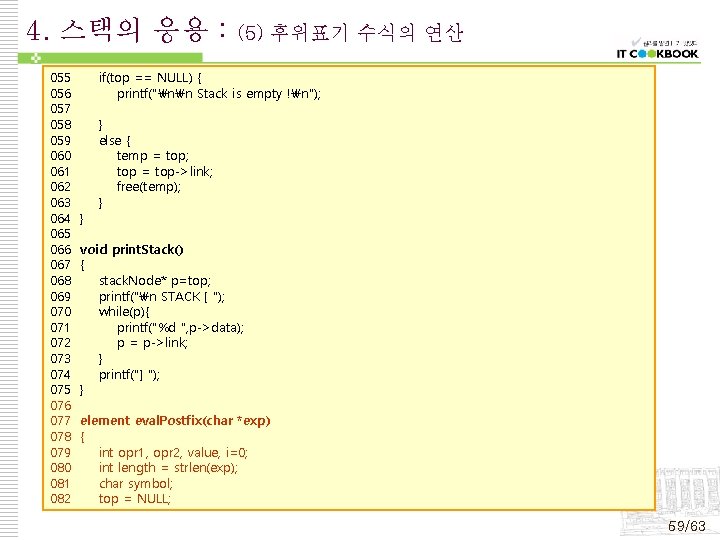
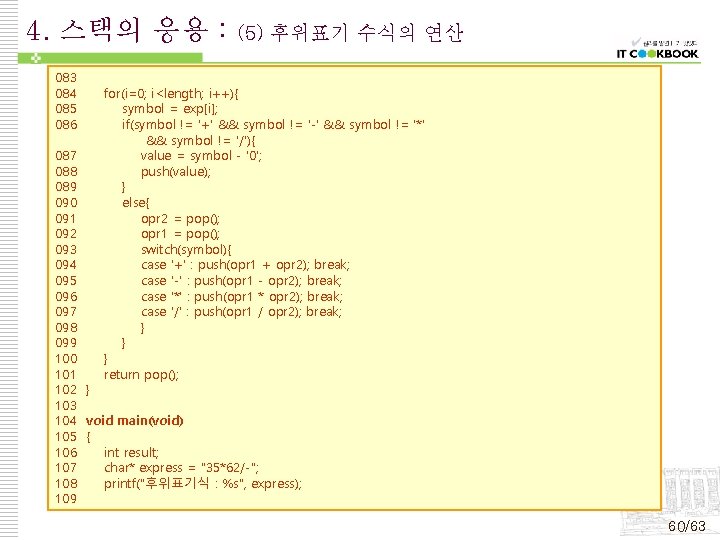
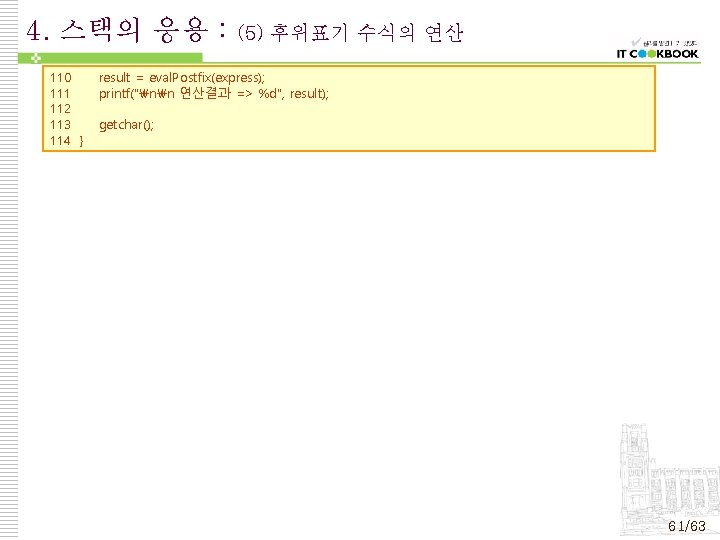
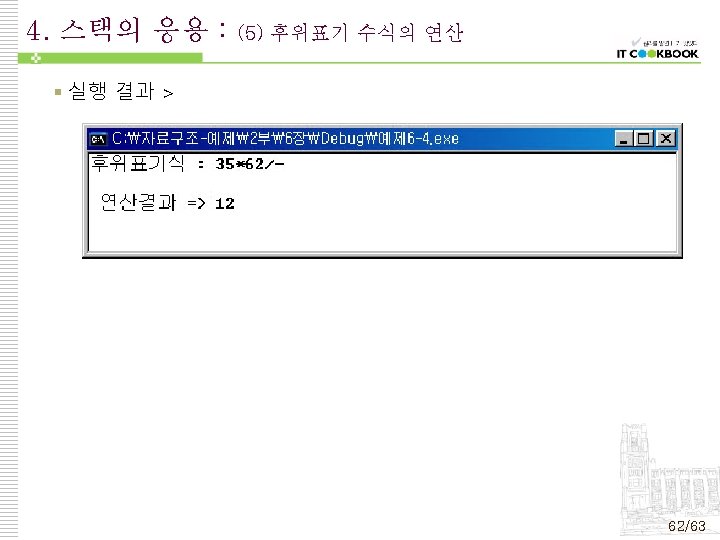
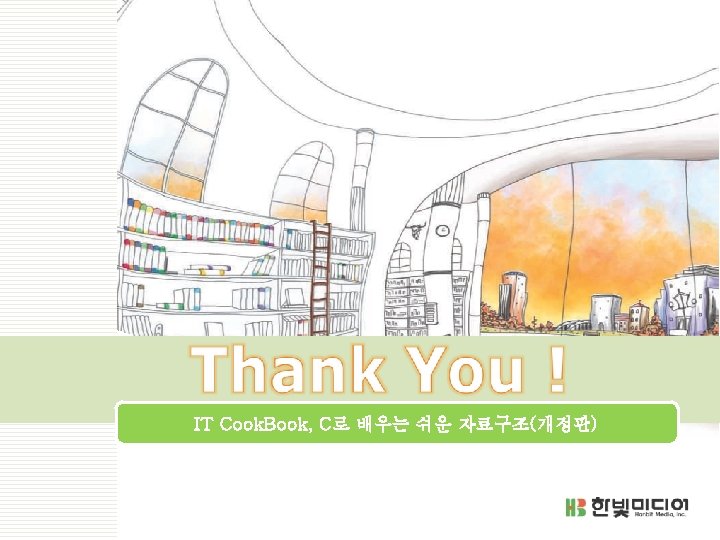
- Slides: 63
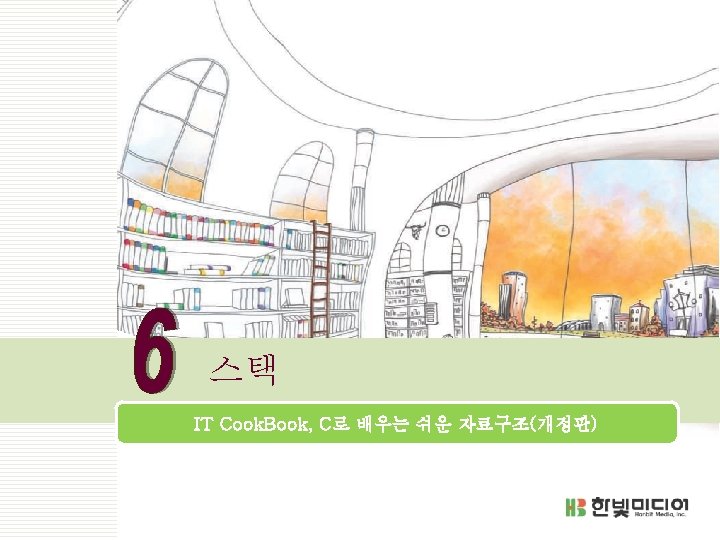
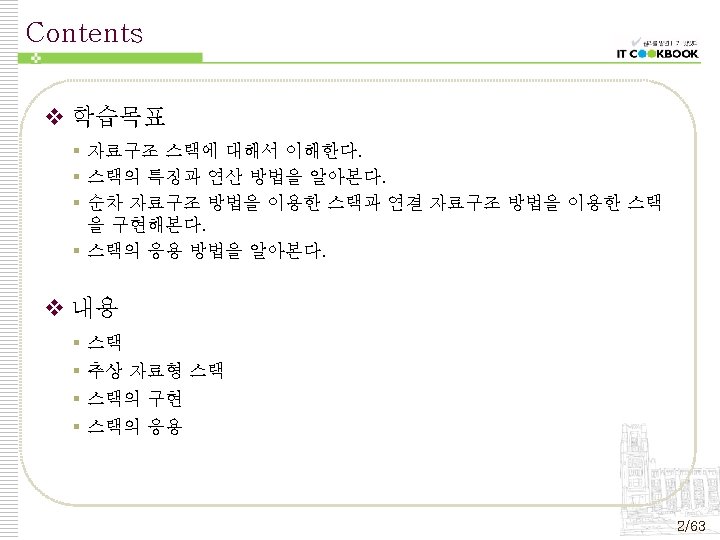
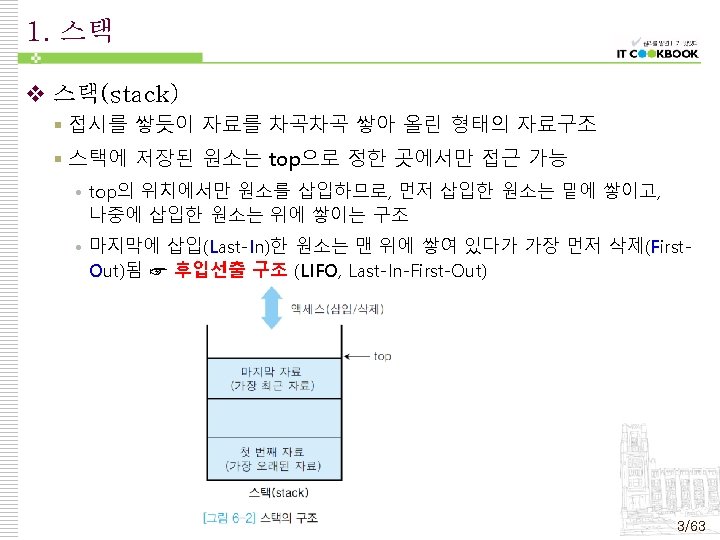
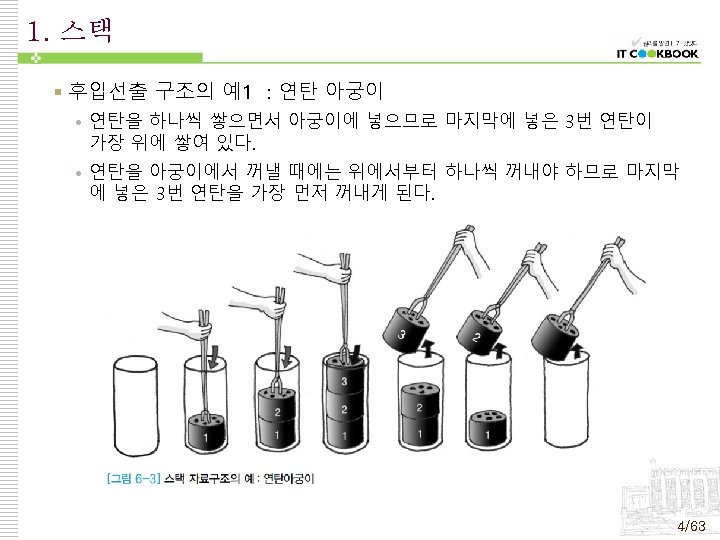
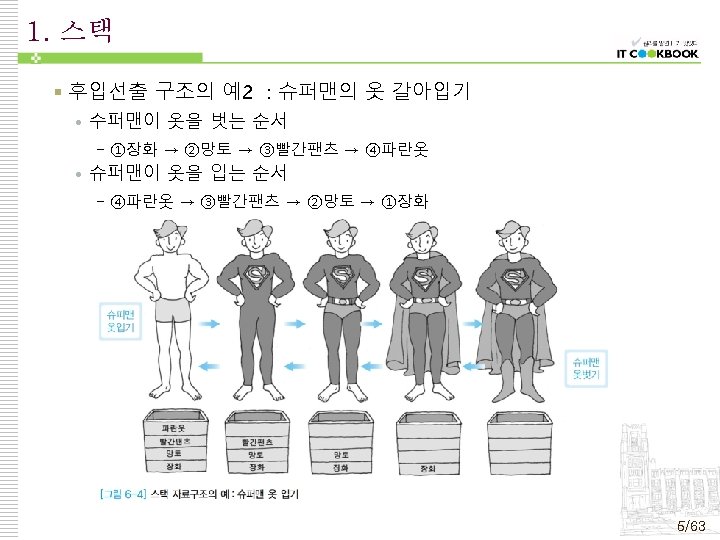
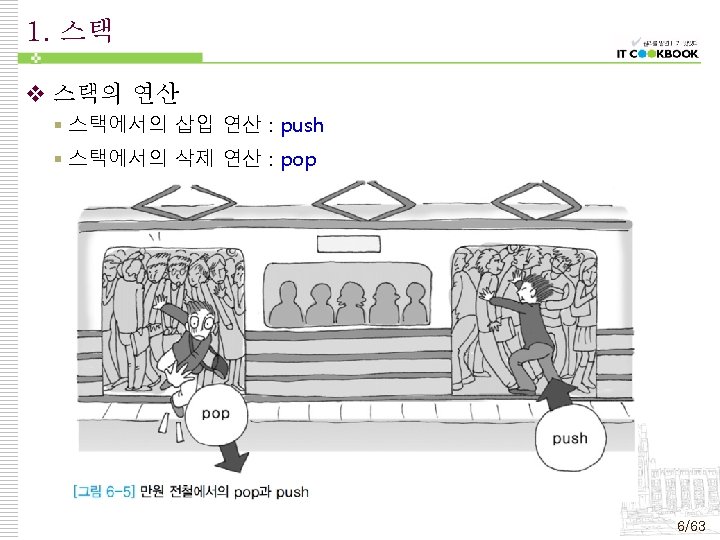
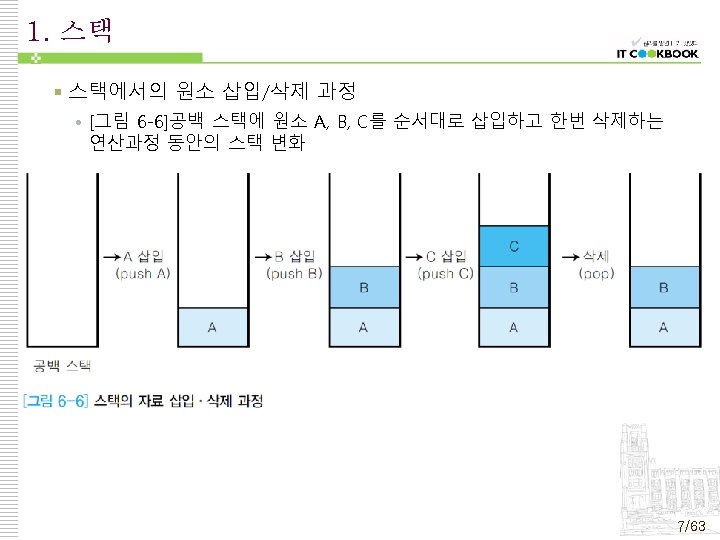
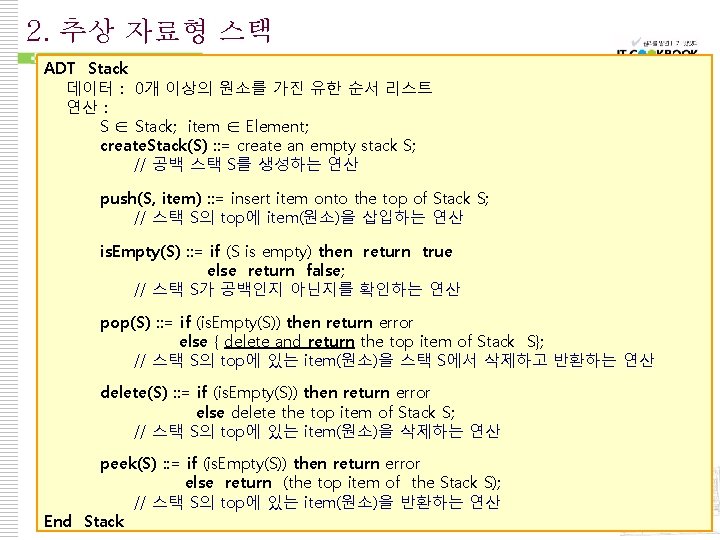
2. 추상 자료형 스택 ADT Stack 데이터 : 0개 이상의 원소를 가진 유한 순서 리스트 연산 : S ∈ Stack; item ∈ Element; create. Stack(S) : : = create an empty stack S; // 공백 스택 S를 생성하는 연산 push(S, item) : : = insert item onto the top of Stack S; // 스택 S의 top에 item(원소)을 삽입하는 연산 is. Empty(S) : : = if (S is empty) then return true else return false; // 스택 S가 공백인지 아닌지를 확인하는 연산 pop(S) : : = if (is. Empty(S)) then return error else { delete and return the top item of Stack S}; // 스택 S의 top에 있는 item(원소)을 스택 S에서 삭제하고 반환하는 연산 delete(S) : : = if (is. Empty(S)) then return error else delete the top item of Stack S; // 스택 S의 top에 있는 item(원소)을 삭제하는 연산 peek(S) : : = if (is. Empty(S)) then return error else return (the top item of the Stack S); // 스택 S의 top에 있는 item(원소)을 반환하는 연산 End Stack 8/63
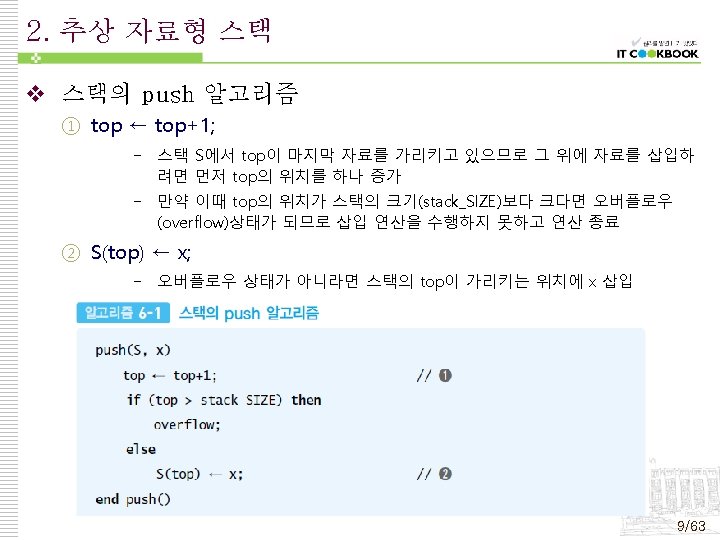
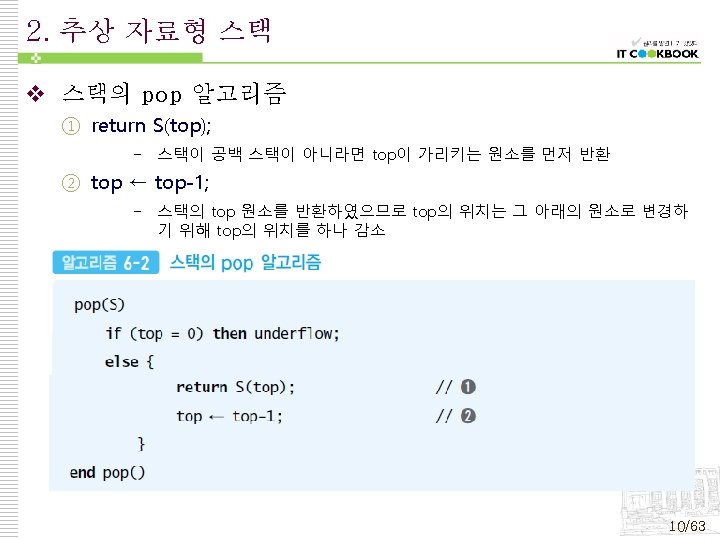
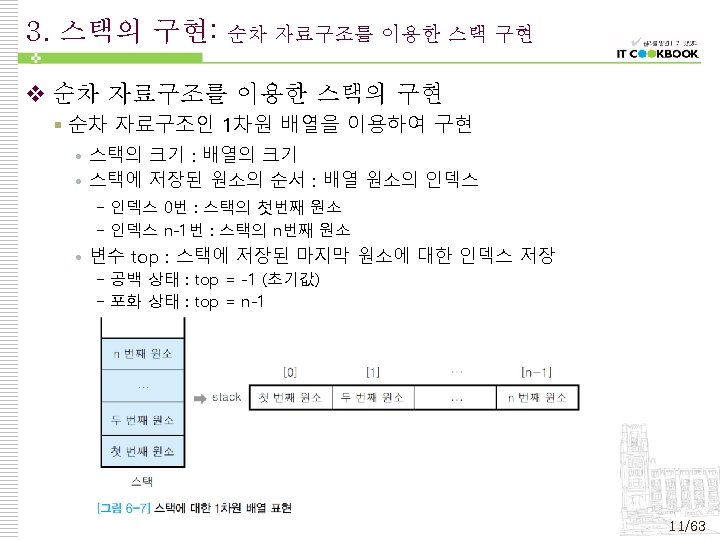
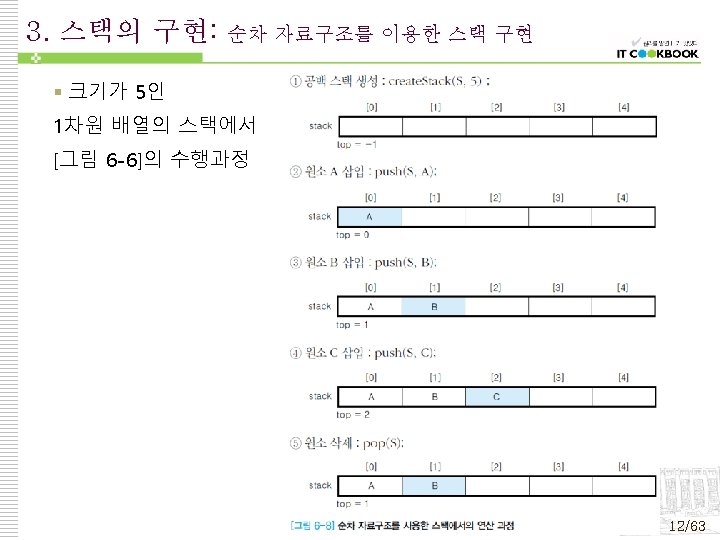
![3 스택의 구현 예제 6 1 순차 자료구조를 이용한 스택 프로그램 01 02 3. 스택의 구현: [예제 6 -1] § 순차 자료구조를 이용한 스택 프로그램 01 02](https://slidetodoc.com/presentation_image_h2/7c694be2007e3644f7039628ea8483fd/image-13.jpg)
3. 스택의 구현: [예제 6 -1] § 순차 자료구조를 이용한 스택 프로그램 01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 #include <stdio. h> #include <stdlib. h> #define STACK_SIZE 100 typedef int element; // int를 스택 element의 자료형으로 정의 element stack[STACK_SIZE]; int top= -1; // 스택의 top의 초기값을-1로 설정 void push(element item) // 스택의 삽입 연산 { if(top >= STACK_SIZE-1) { // 스택이 이미 Full인 경우 printf("nn Stack is FULL ! n"); return; } else stack[++top]=item; } element pop() // 스택의 삭제 후 반환 연산 { if(top==-1) { // 현재 스택이 공백인 경우 printf("nn Stack is Empty!!n"); return 0; } else return stack[top--]; } 13/63
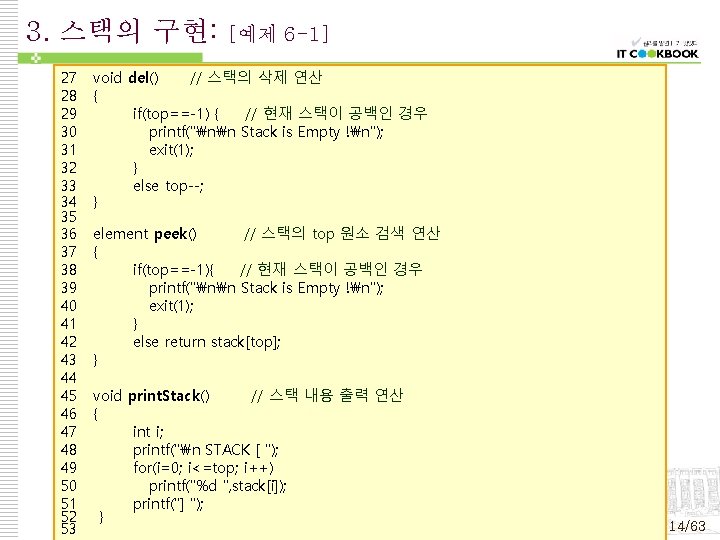
3. 스택의 구현: 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 [예제 6 -1] void del() // 스택의 삭제 연산 { if(top==-1) { // 현재 스택이 공백인 경우 printf("nn Stack is Empty !n"); exit(1); } else top--; } element peek() // 스택의 top 원소 검색 연산 { if(top==-1){ // 현재 스택이 공백인 경우 printf("nn Stack is Empty !n"); exit(1); } else return stack[top]; } void print. Stack() // 스택 내용 출력 연산 { int i; printf("n STACK [ "); for(i=0; i<=top; i++) printf("%d ", stack[i]); printf("] "); } 14/63
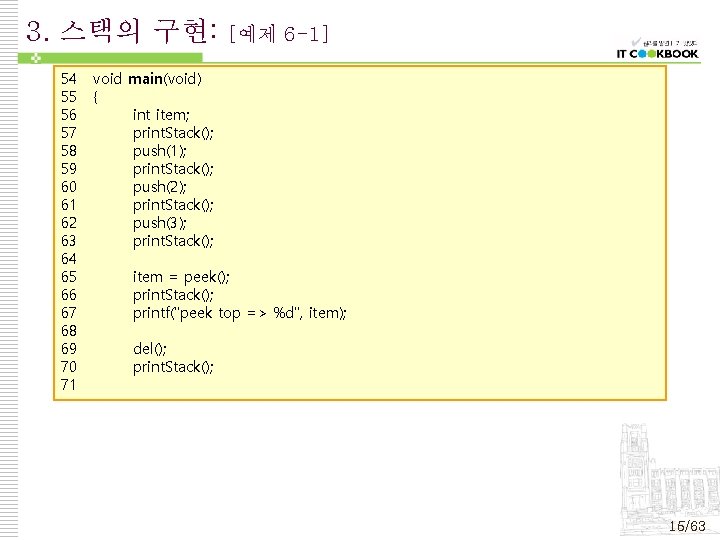
3. 스택의 구현: 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 [예제 6 -1] void main(void) { int item; print. Stack(); push(1); print. Stack(); push(2); print. Stack(); push(3); print. Stack(); item = peek(); print. Stack(); printf("peek top => %d", item); del(); print. Stack(); 15/63
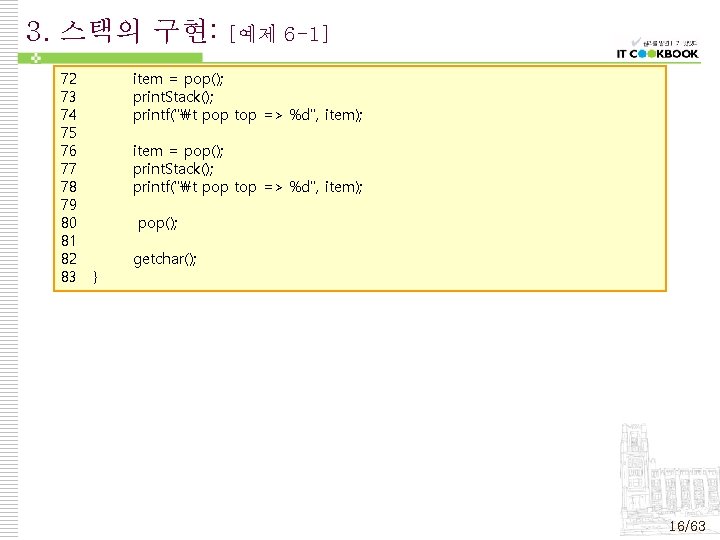
3. 스택의 구현: 72 73 74 75 76 77 78 79 80 81 82 83 [예제 6 -1] item = pop(); print. Stack(); printf("t pop top => %d", item); pop(); } getchar(); 16/63
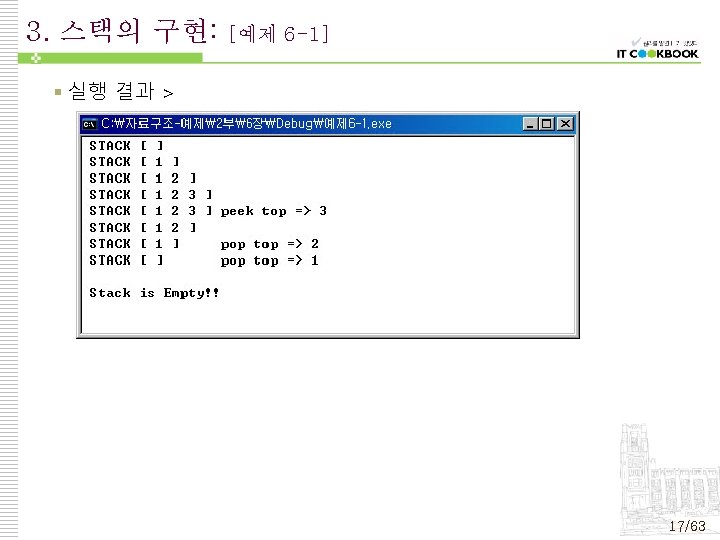
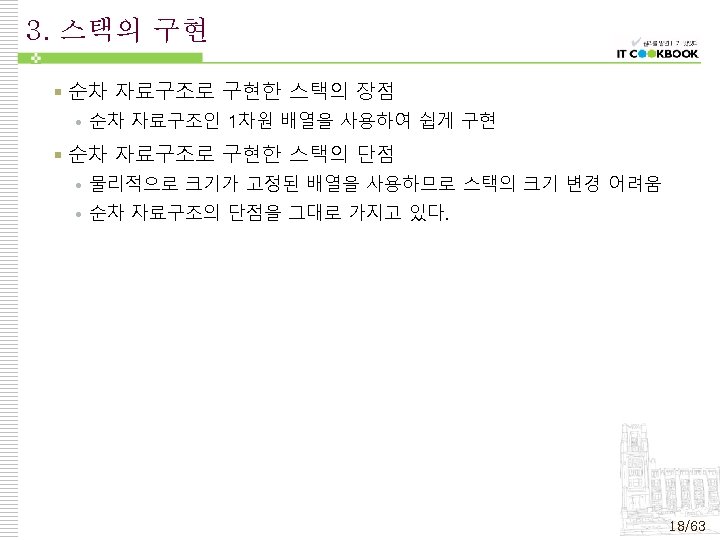
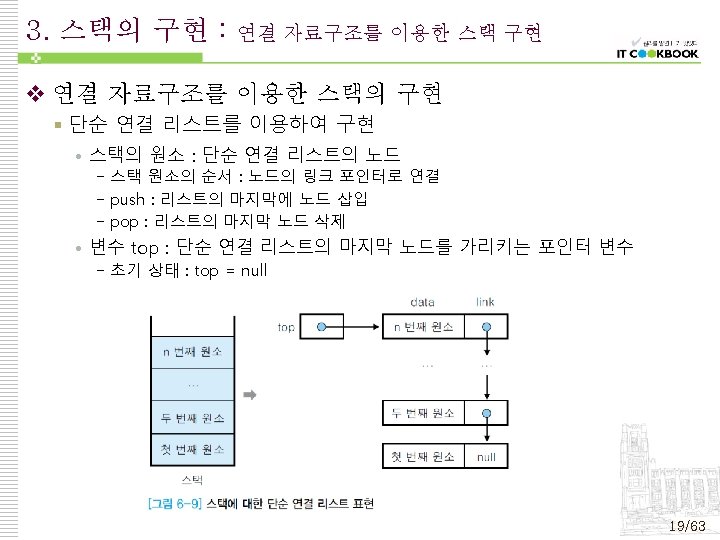
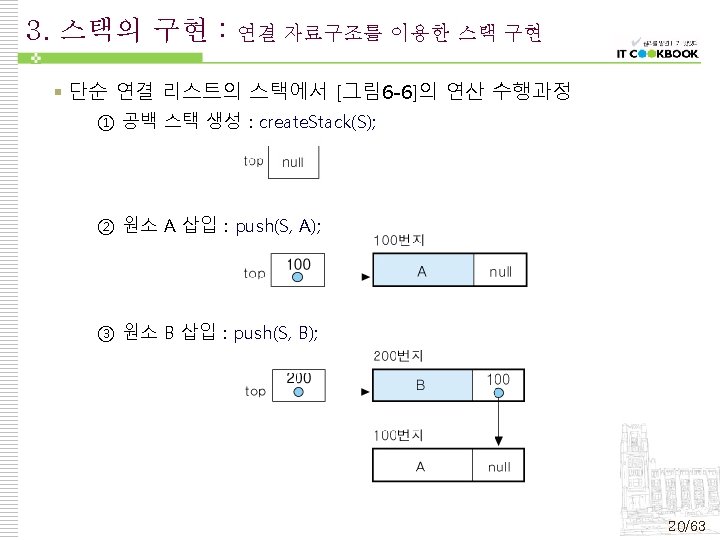
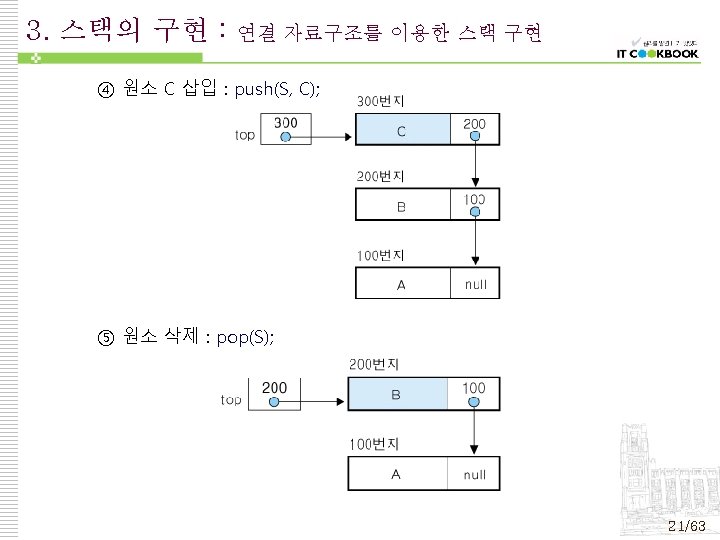
![3 스택의 구현 예제 6 2 단순 연결 리스트를 이용한 스택 프로그램 3. 스택의 구현 : [예제 6 -2] § 단순 연결 리스트를 이용한 스택 프로그램](https://slidetodoc.com/presentation_image_h2/7c694be2007e3644f7039628ea8483fd/image-22.jpg)
3. 스택의 구현 : [예제 6 -2] § 단순 연결 리스트를 이용한 스택 프로그램 001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 #include <stdio. h> #include <stdlib. h> #include <string. h> typedef int element; // int를 스택 element의 자료형으로 정의 typedef struct stack. Node { // 스택의 노드 구조 정의 element data; struct stack. Node *link; }stack. Node; stack. Node* top; // 스택의top 노드를 지정하기 위한 포인터 top 선언 void push(element item) // 스택 삽입 연산 { stack. Node* temp=(stack. Node *)malloc(sizeof(stack. Node)); temp->data = item; temp->link = top; top = temp; } 22/63
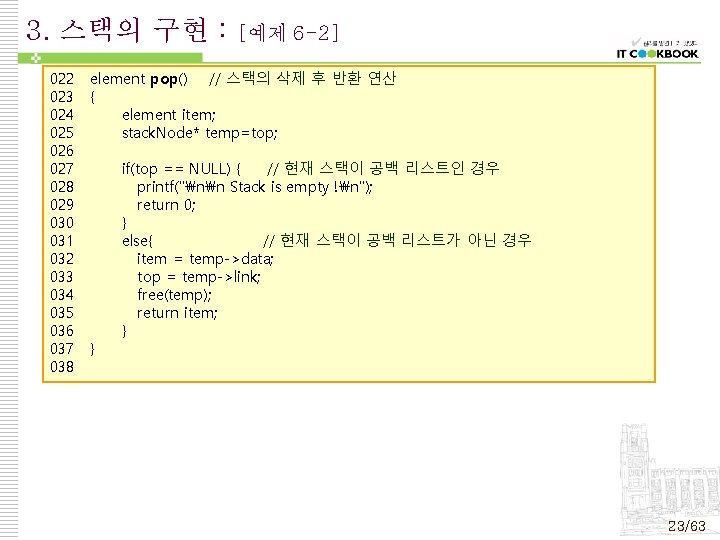
3. 스택의 구현 : 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 [예제 6 -2] element pop() // 스택의 삭제 후 반환 연산 { element item; stack. Node* temp=top; } if(top == NULL) { // 현재 스택이 공백 리스트인 경우 printf("nn Stack is empty !n"); return 0; } else{ // 현재 스택이 공백 리스트가 아닌 경우 item = temp->data; top = temp->link; free(temp); return item; } 23/63
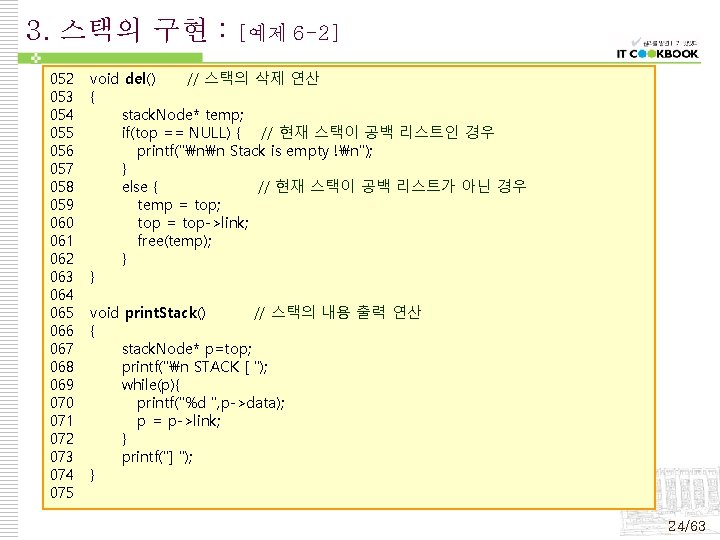
3. 스택의 구현 : 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 [예제 6 -2] void del() // 스택의 삭제 연산 { stack. Node* temp; if(top == NULL) { // 현재 스택이 공백 리스트인 경우 printf("nn Stack is empty !n"); } else { // 현재 스택이 공백 리스트가 아닌 경우 temp = top; top = top->link; free(temp); } } void print. Stack() // 스택의 내용 출력 연산 { stack. Node* p=top; printf("n STACK [ "); while(p){ printf("%d ", p->data); p = p->link; } printf("] "); } 24/63
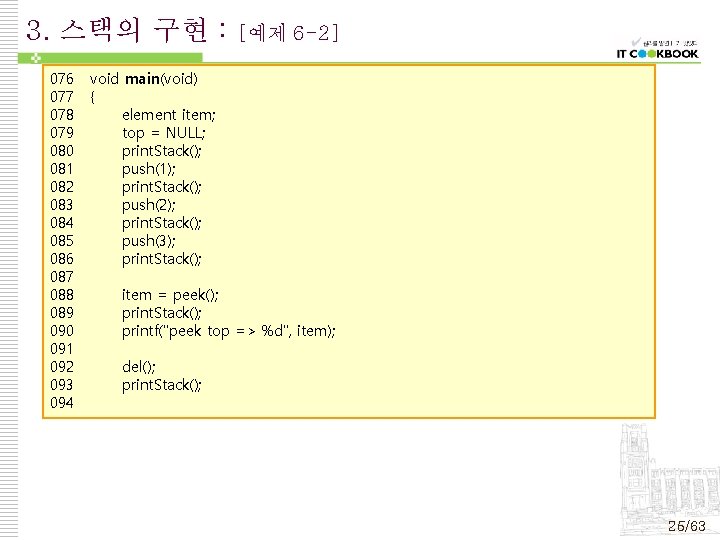
3. 스택의 구현 : 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 [예제 6 -2] void main(void) { element item; top = NULL; print. Stack(); push(1); print. Stack(); push(2); print. Stack(); push(3); print. Stack(); item = peek(); print. Stack(); printf("peek top => %d", item); del(); print. Stack(); 25/63
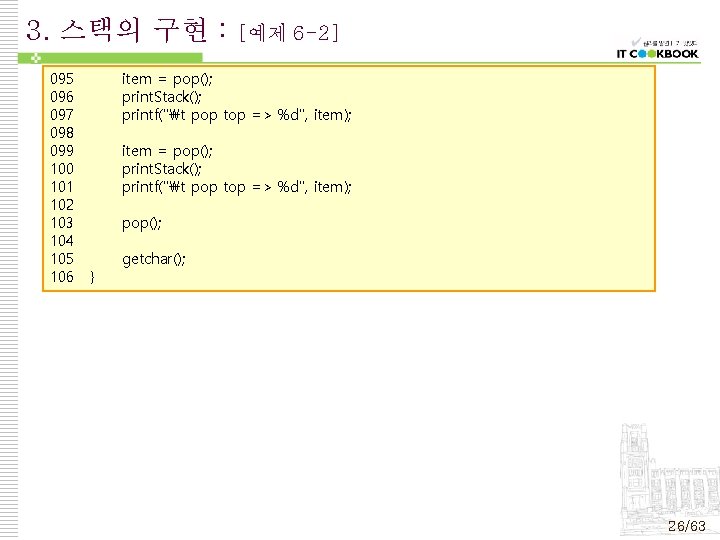
3. 스택의 구현 : 095 096 097 098 099 100 101 102 103 104 105 106 [예제 6 -2] item = pop(); print. Stack(); printf("t pop top => %d", item); pop(); } getchar(); 26/63
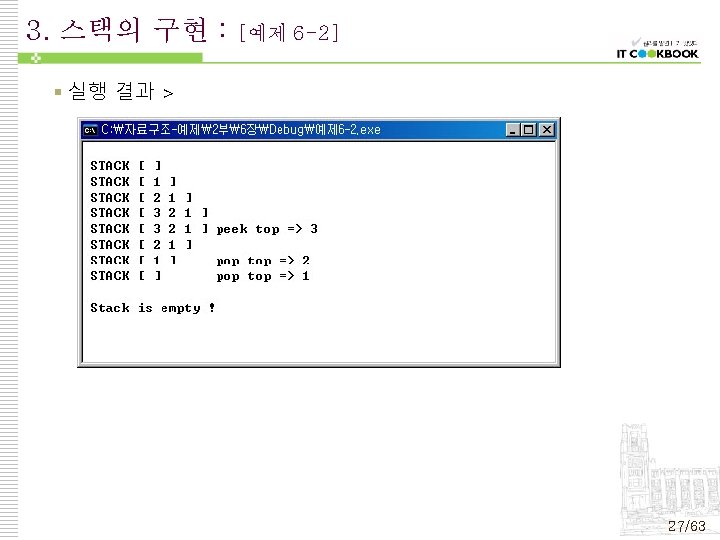
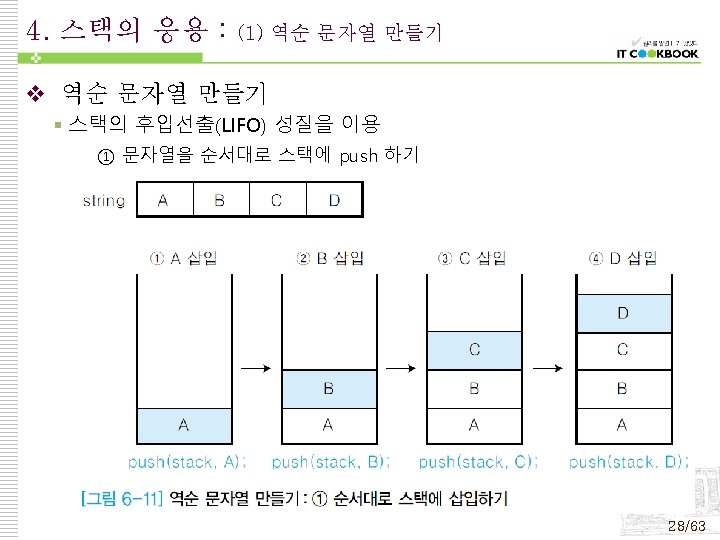
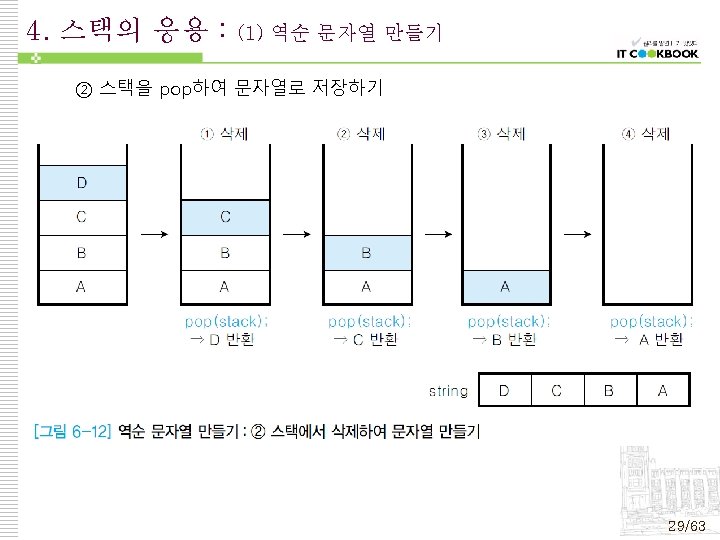
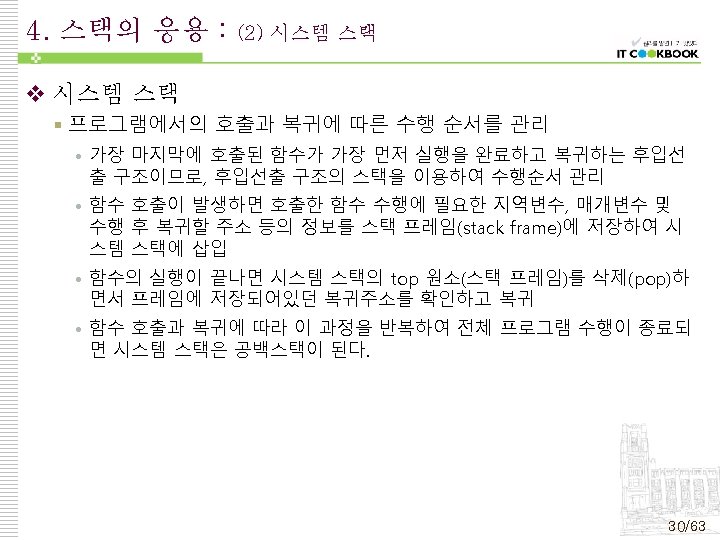
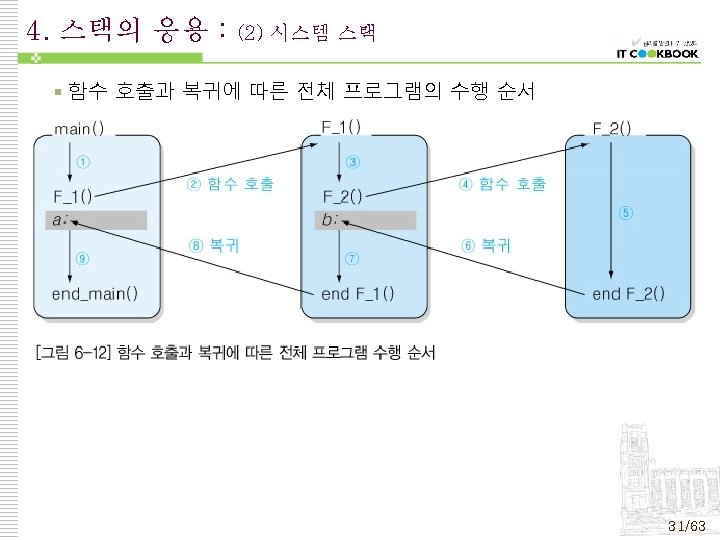
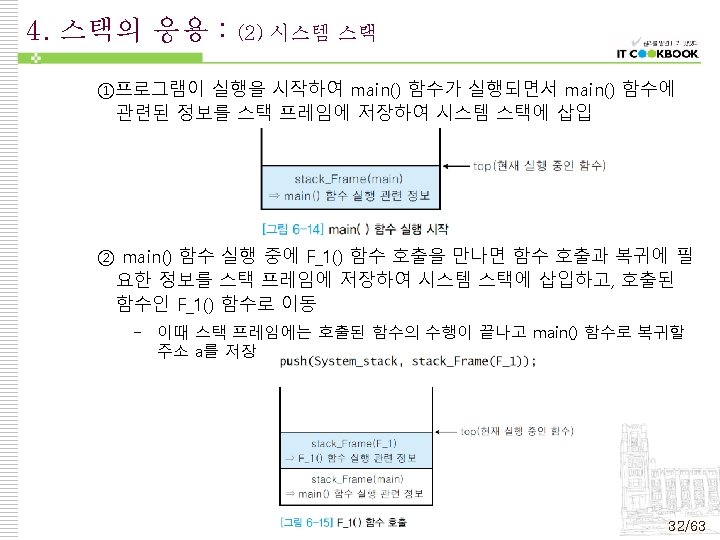
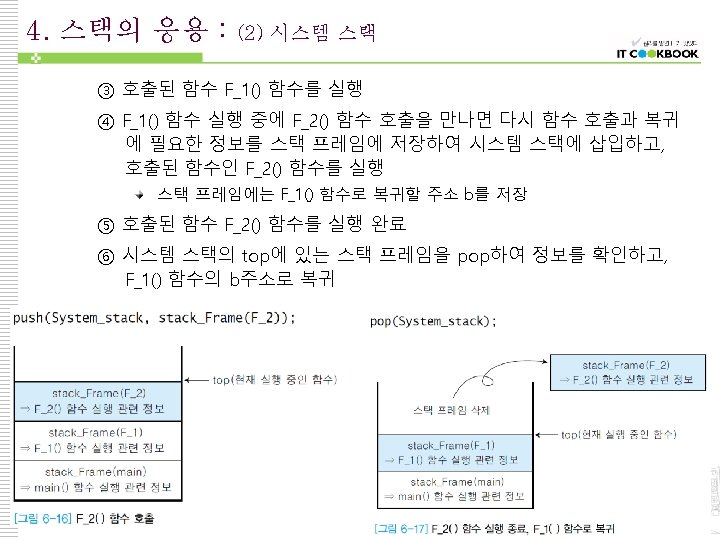
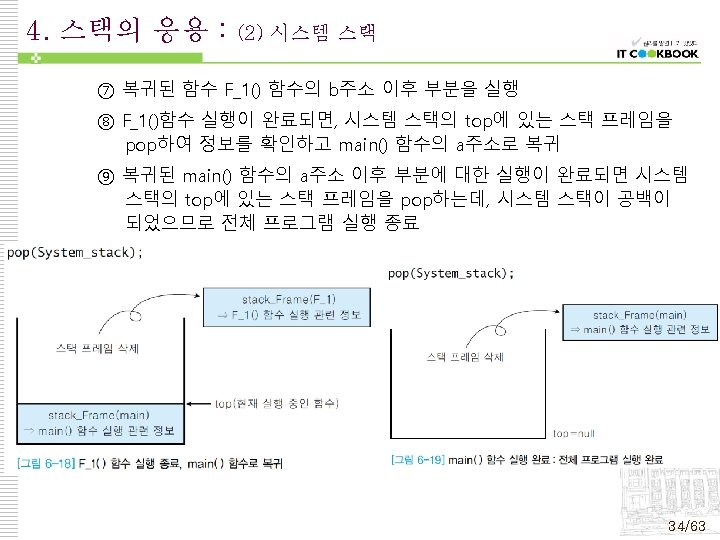
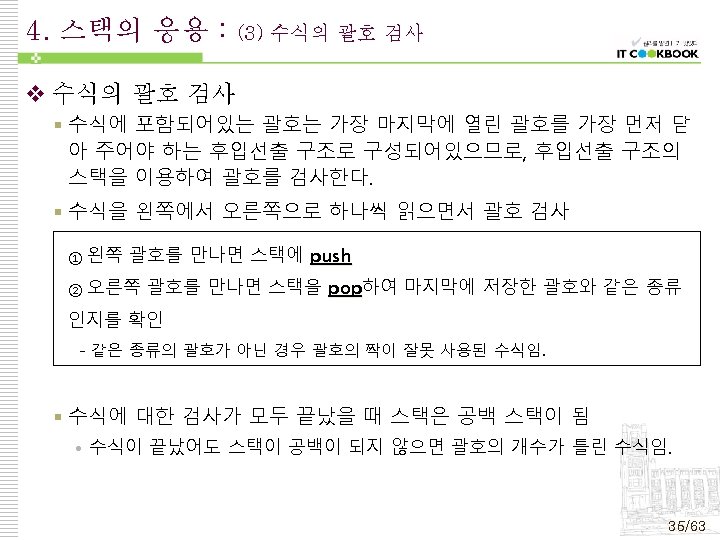
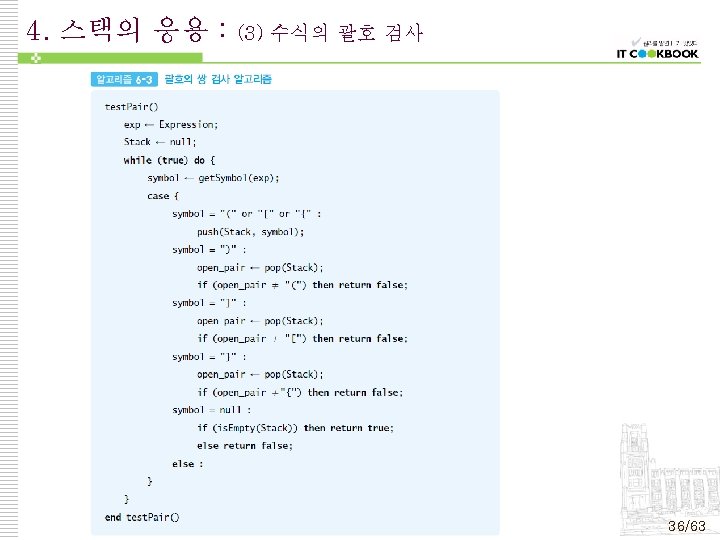
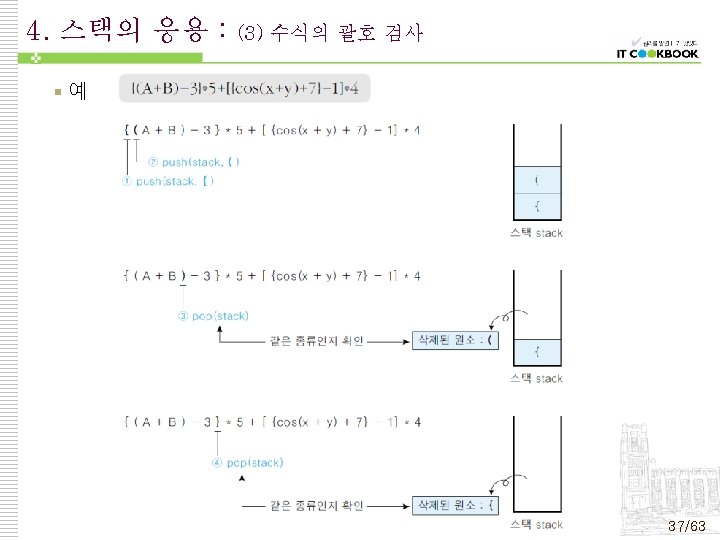
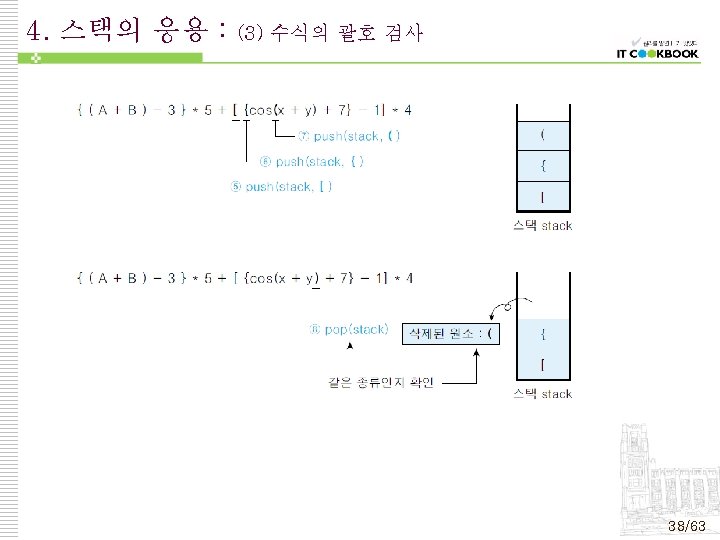
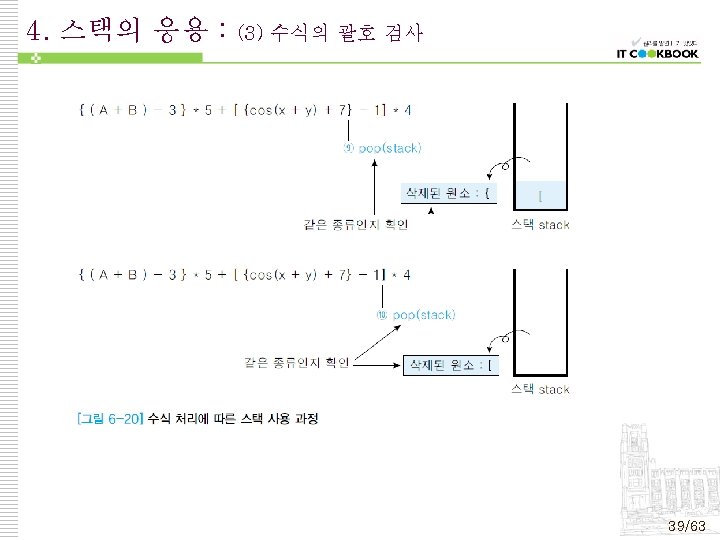
![4 스택의 응용 3 수식의 괄호 검사 예제 6 3 수식의 4. 스택의 응용 : (3) 수식의 괄호 검사 – [예제 6 -3] § 수식의](https://slidetodoc.com/presentation_image_h2/7c694be2007e3644f7039628ea8483fd/image-40.jpg)
4. 스택의 응용 : (3) 수식의 괄호 검사 – [예제 6 -3] § 수식의 괄호 검사 C 프로그램 001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 #include <stdio. h> #include <stdlib. h> #include <string. h> typedef char element; typedef struct stack. Node { element data; struct stack. Node *link; }stack. Node; stack. Node* top; void push(element item) { stack. Node* temp=(stack. Node *)malloc(sizeof(stack. Node)); temp->data = item; temp->link = top; top = temp; } element pop() { element item; stack. Node* temp=top; 40/63
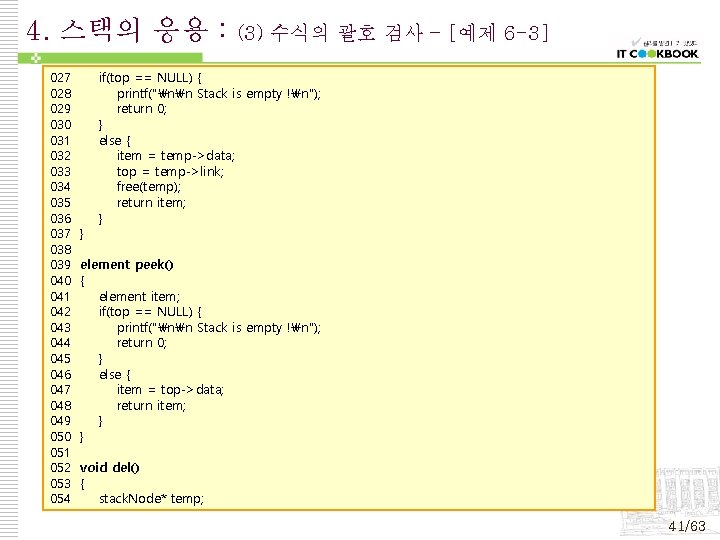
4. 스택의 응용 : 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 } (3) 수식의 괄호 검사 – [예제 6 -3] if(top == NULL) { printf("nn Stack is empty !n"); return 0; } else { item = temp->data; top = temp->link; free(temp); return item; } element peek() { element item; if(top == NULL) { printf("nn Stack is empty !n"); return 0; } else { item = top->data; return item; } } void del() { stack. Node* temp; 41/63
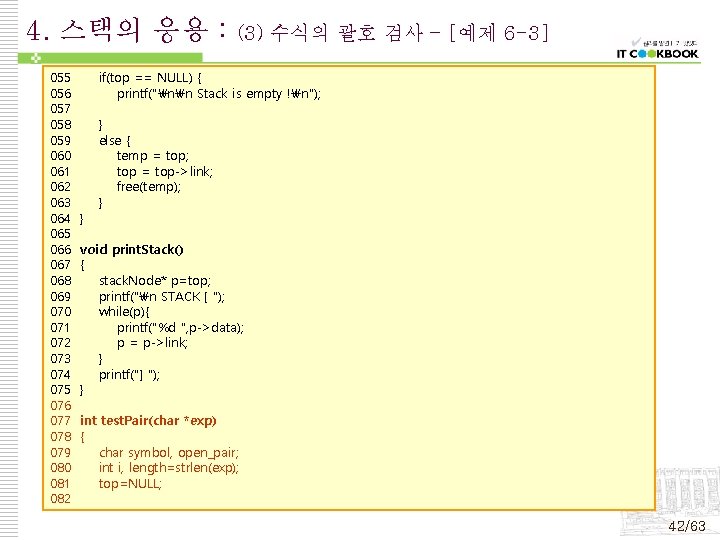
4. 스택의 응용 : 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 (3) 수식의 괄호 검사 – [예제 6 -3] if(top == NULL) { printf("nn Stack is empty !n"); } } else { temp = top; top = top->link; free(temp); } void print. Stack() { stack. Node* p=top; printf("n STACK [ "); while(p){ printf("%d ", p->data); p = p->link; } printf("] "); } int test. Pair(char *exp) { char symbol, open_pair; int i, length=strlen(exp); top=NULL; 42/63
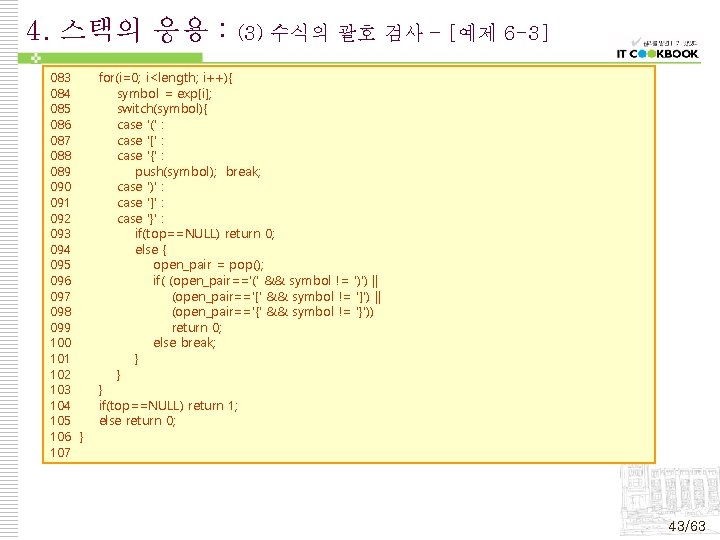
4. 스택의 응용 : 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 } 107 (3) 수식의 괄호 검사 – [예제 6 -3] for(i=0; i<length; i++){ symbol = exp[i]; switch(symbol){ case '(' : case '[' : case '{' : push(symbol); break; case ')' : case ']' : case '}' : if(top==NULL) return 0; else { open_pair = pop(); if( (open_pair=='(' && symbol != ')') || (open_pair=='[' && symbol != ']') || (open_pair=='{' && symbol != '}')) return 0; else break; } } } if(top==NULL) return 1; else return 0; 43/63
![4 스택의 응용 3 수식의 괄호 검사 예제 6 3 108 109 4. 스택의 응용 : (3) 수식의 괄호 검사 – [예제 6 -3] 108 109](https://slidetodoc.com/presentation_image_h2/7c694be2007e3644f7039628ea8483fd/image-44.jpg)
4. 스택의 응용 : (3) 수식의 괄호 검사 – [예제 6 -3] 108 109 void main(void) 110 { 111 char* express = "{(A+B)-3}*5+[{cos(x+y)+7}-1]*4"; 112 printf("%s", express); 113 if(test. Pair(express) == 1) 114 printf("nn 수식의 괄호가 맞게 사용되었습니다!"); 115 else 116 printf("nn 수식의 괄호가 틀렸습니다!"); 117 118 getchar(); 119 } 44/63
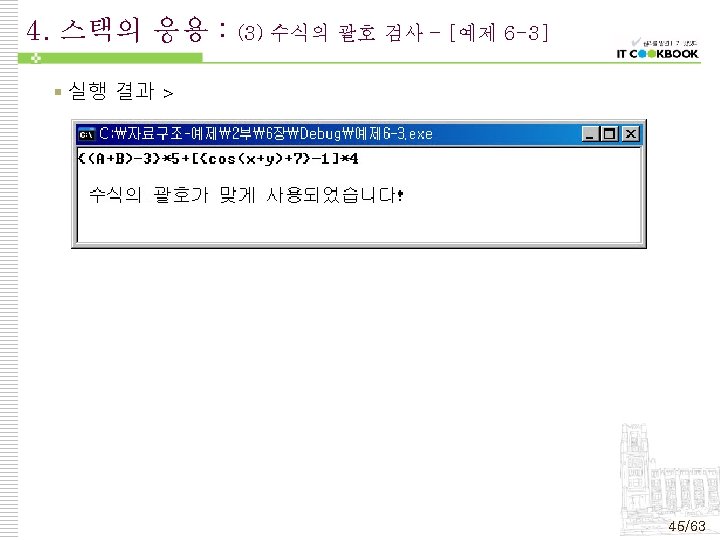
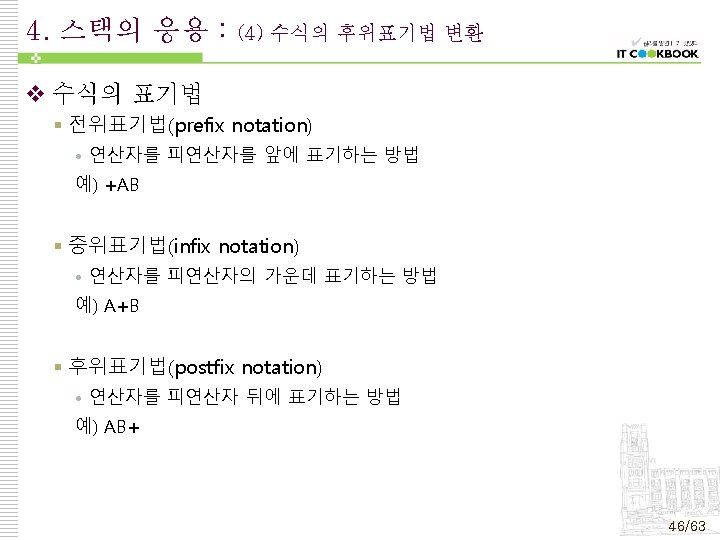
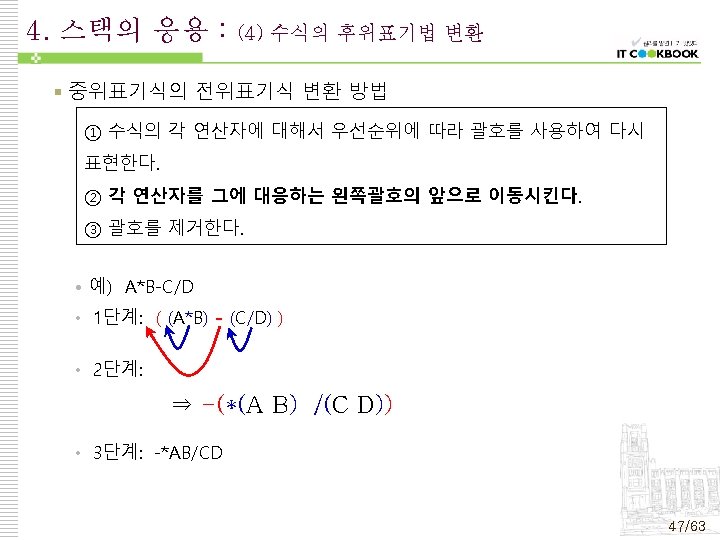
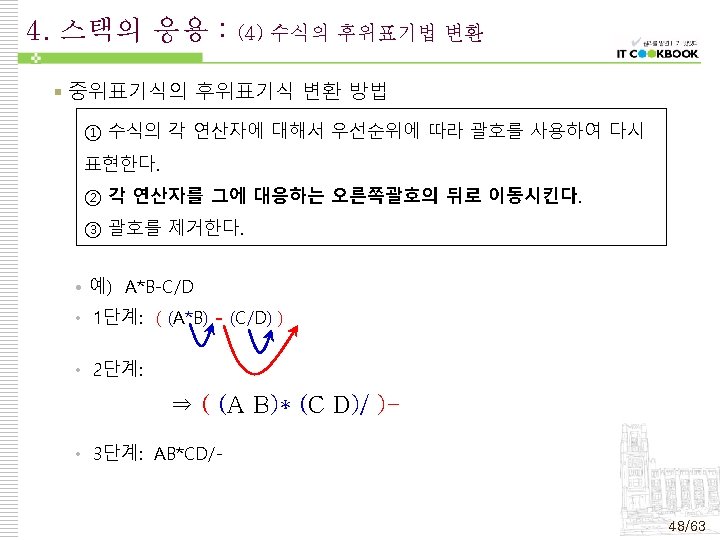
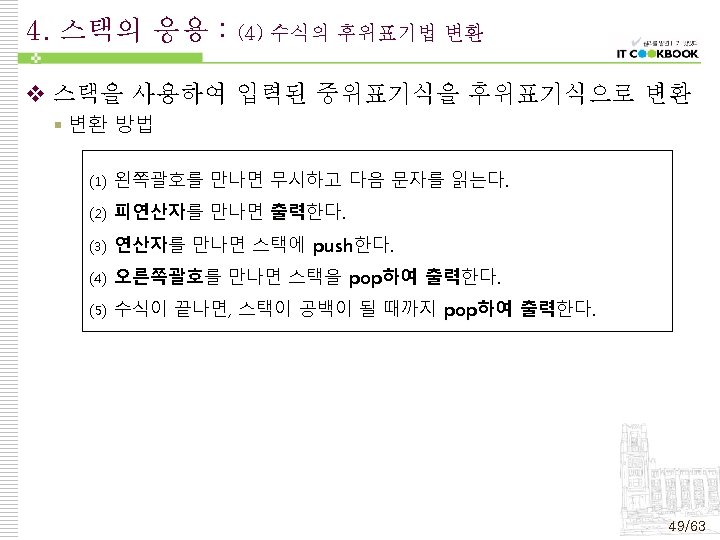
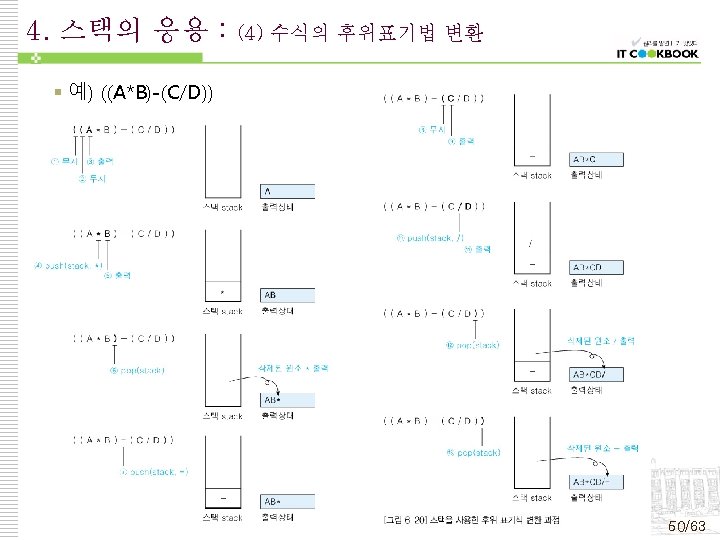
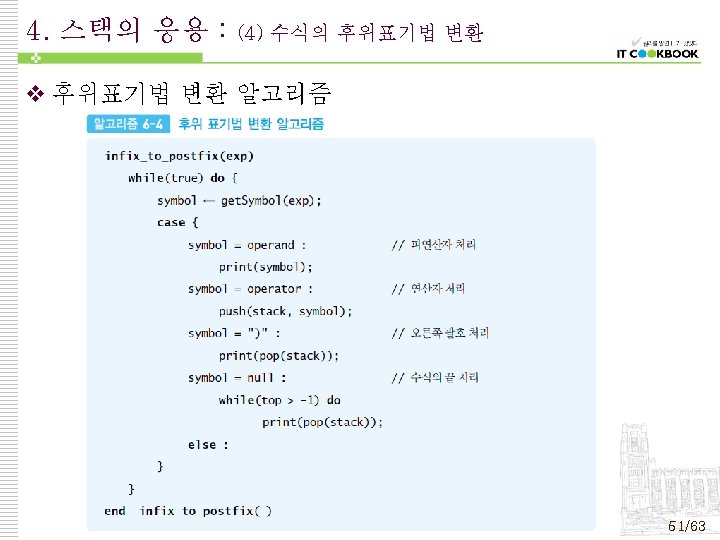
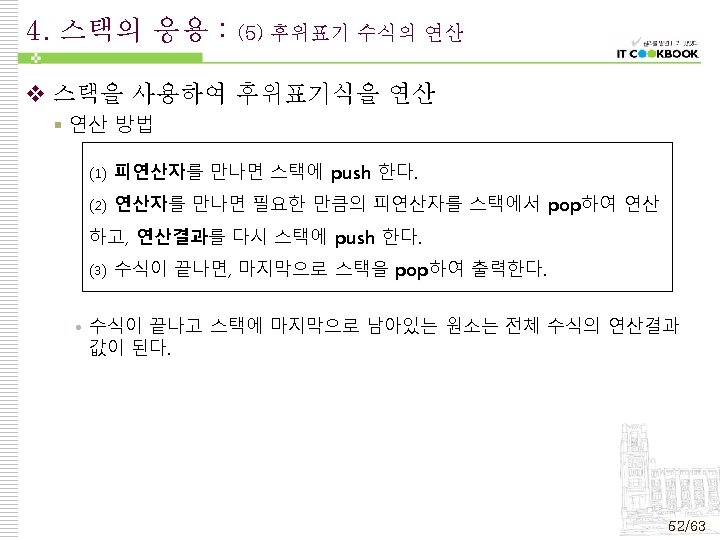
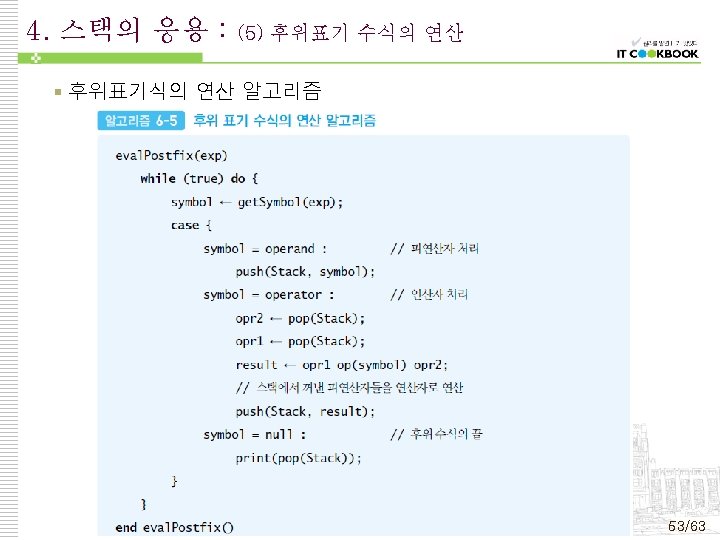
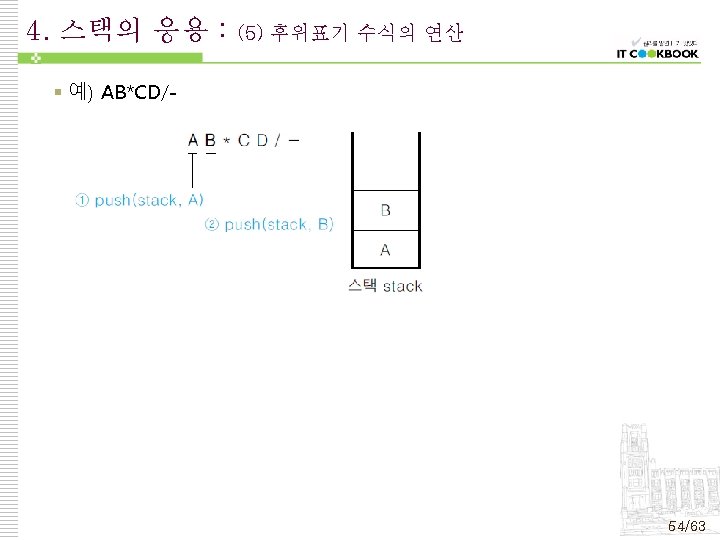
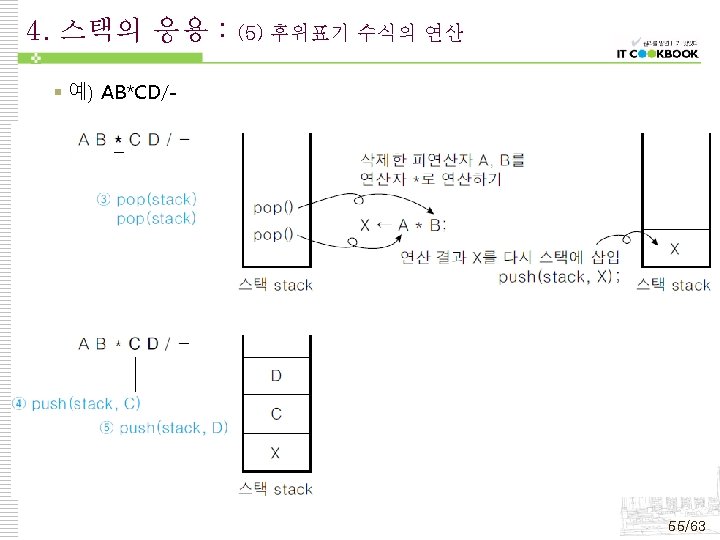
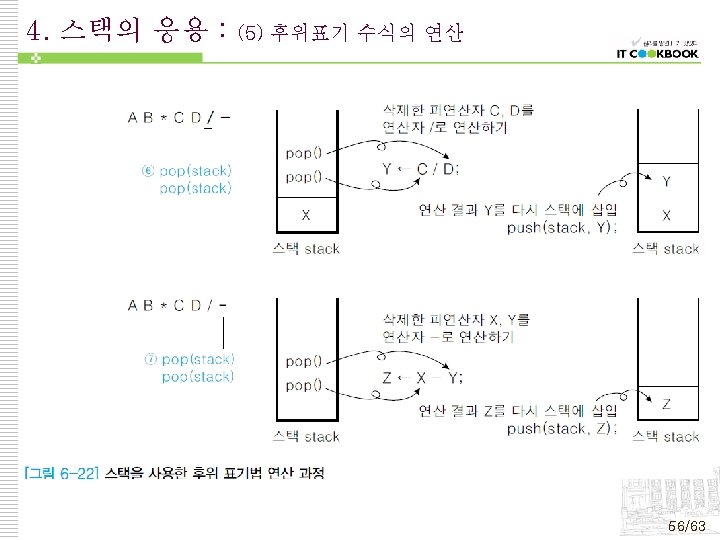
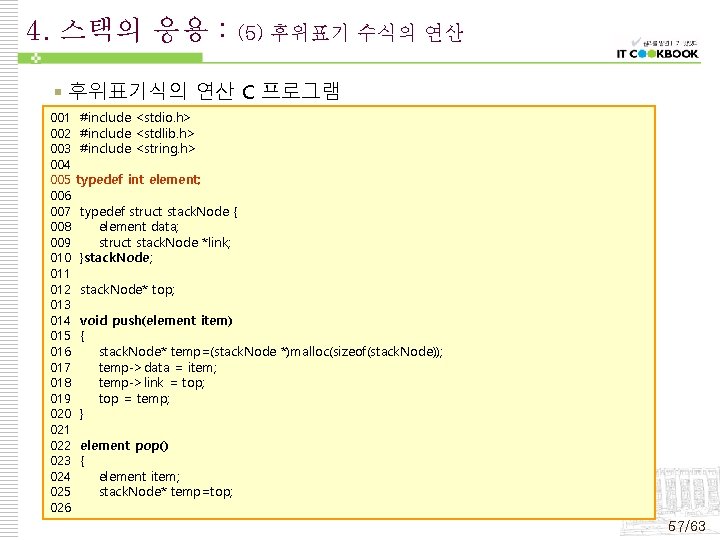
4. 스택의 응용 : (5) 후위표기 수식의 연산 § 후위표기식의 연산 C 프로그램 001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 #include <stdio. h> #include <stdlib. h> #include <string. h> typedef int element; typedef struct stack. Node { element data; struct stack. Node *link; }stack. Node; stack. Node* top; void push(element item) { stack. Node* temp=(stack. Node *)malloc(sizeof(stack. Node)); temp->data = item; temp->link = top; top = temp; } element pop() { element item; stack. Node* temp=top; 57/63
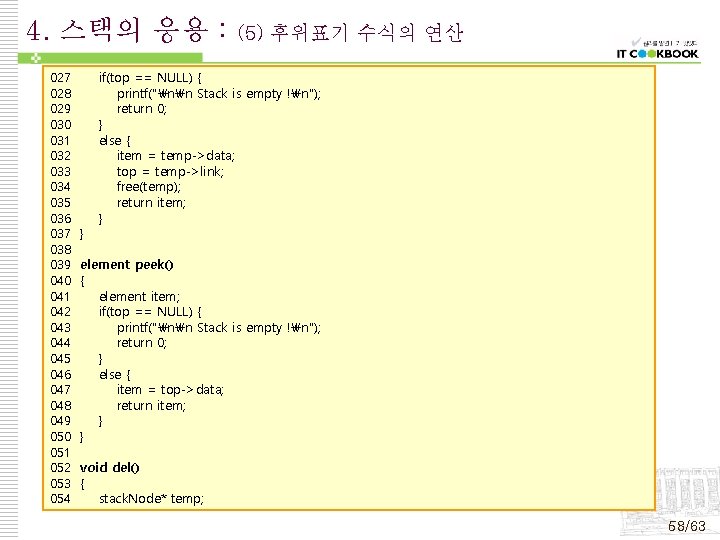
4. 스택의 응용 : 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 } (5) 후위표기 수식의 연산 if(top == NULL) { printf("nn Stack is empty !n"); return 0; } else { item = temp->data; top = temp->link; free(temp); return item; } element peek() { element item; if(top == NULL) { printf("nn Stack is empty !n"); return 0; } else { item = top->data; return item; } } void del() { stack. Node* temp; 58/63
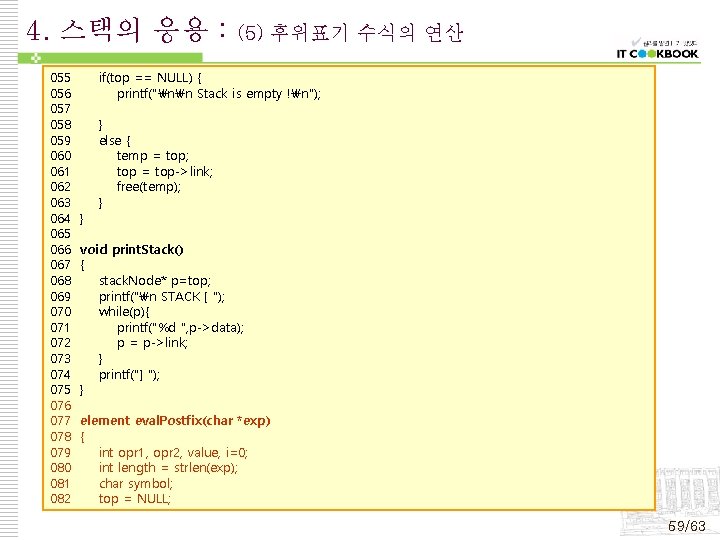
4. 스택의 응용 : 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 (5) 후위표기 수식의 연산 if(top == NULL) { printf("nn Stack is empty !n"); } } else { temp = top; top = top->link; free(temp); } void print. Stack() { stack. Node* p=top; printf("n STACK [ "); while(p){ printf("%d ", p->data); p = p->link; } printf("] "); } element eval. Postfix(char *exp) { int opr 1, opr 2, value, i=0; int length = strlen(exp); char symbol; top = NULL; 59/63
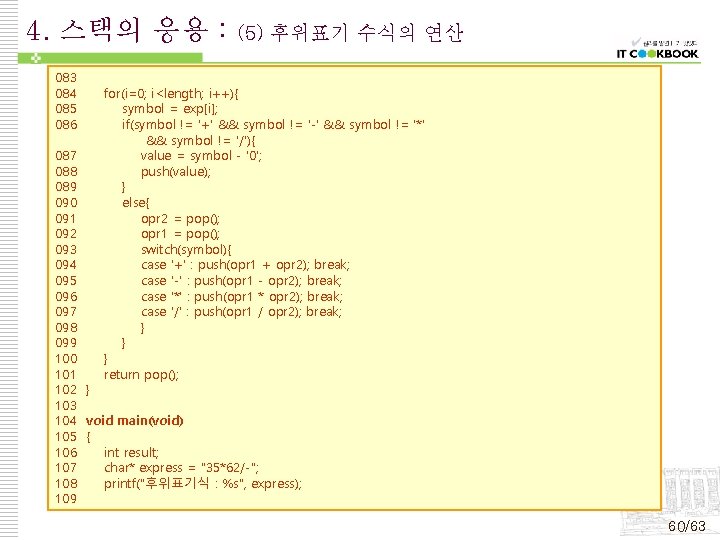
4. 스택의 응용 : 083 084 085 086 (5) 후위표기 수식의 연산 for(i=0; i<length; i++){ symbol = exp[i]; if(symbol != '+' && symbol != '-' && symbol != '*' && symbol != '/'){ value = symbol - '0'; push(value); } else{ opr 2 = pop(); opr 1 = pop(); switch(symbol){ case '+' : push(opr 1 + opr 2); break; case '-' : push(opr 1 - opr 2); break; case '*' : push(opr 1 * opr 2); break; case '/' : push(opr 1 / opr 2); break; } } } return pop(); 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 } 103 104 void main(void) 105 { 106 int result; 107 char* express = "35*62/-"; 108 printf("후위표기식 : %s", express); 109 60/63
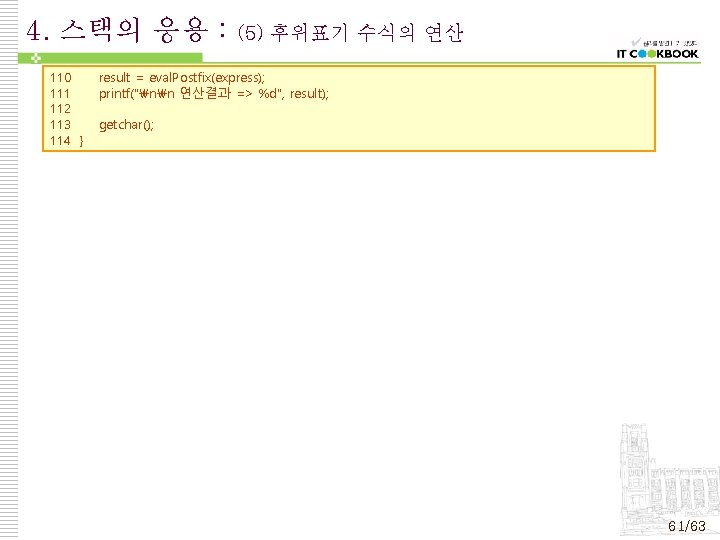
4. 스택의 응용 : 110 111 112 113 114 } (5) 후위표기 수식의 연산 result = eval. Postfix(express); printf("nn 연산결과 => %d", result); getchar(); 61/63
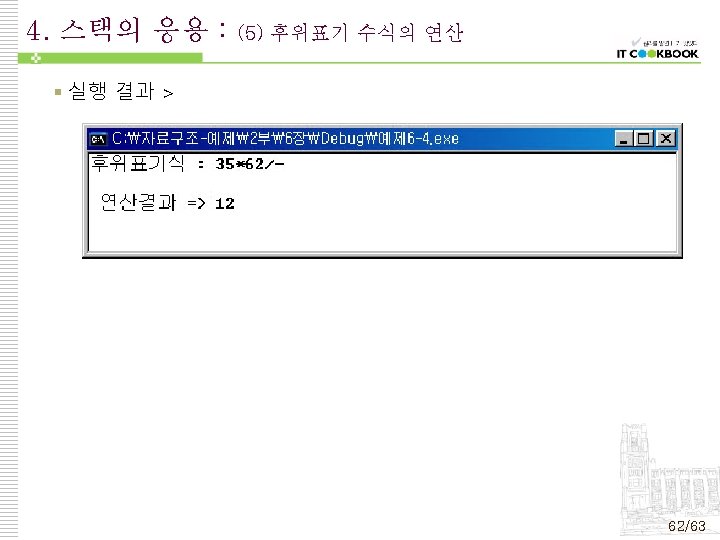
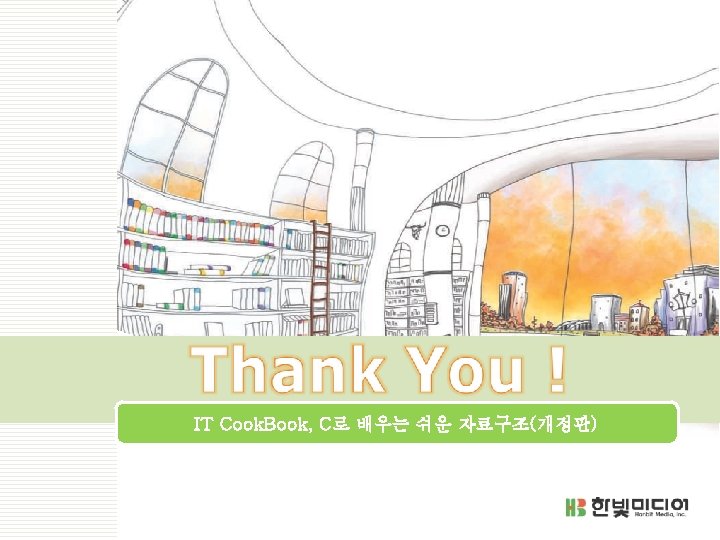
What is stack adt
What is it
Stack adt
Metaphor adalah
Stack smashing
Stack pointer definition
Diketahui suatu stack dengan max_stack = 6
Signal element vs data element
Distinguish between a signal element and a data element.
Adt 1 in case of casual vacancy
Adt in java
Binary tree adt
Disjoint set adt
Android development tools (adt)
Node list adt
Hospital adt system
Adt stands for c++
Taillist
Queue adt
Adt in java
Adt
Adt formula
Adt heap
List adt java
Mean
Adt bag
List adt
Tree adt
"adt" lawsuit or barber or hack or sued
Array based implementation of list adt
(p^ q)^( p^q) is a
Array adt
Adt android download
Dynamic equivalence problem
Dictionary adt
Graph adt
Adt operator meaning
Adt in java
Sdsu adt
List adt
Adt plugin eclipse
Avl tree haskell
Graph abstract
Abstract data type
Adt informatik
Polynomial addition using linked list in data structure
Lbs
Sequence adt
Dictionary adt
Binary tree adt
Table adt
Array adt
Cimss adt
Adt pecahan
An adt's operations are known as its ______.
Graph adt
Adt security training
Adt graph
Polynomial addition using linked list in python
Collection (abstract data type)
Data abstraction and problem solving with java
Adaptable priority queues
Adt functional programming
Binary tree adt