11 Map ADTs Map concepts Map applications A
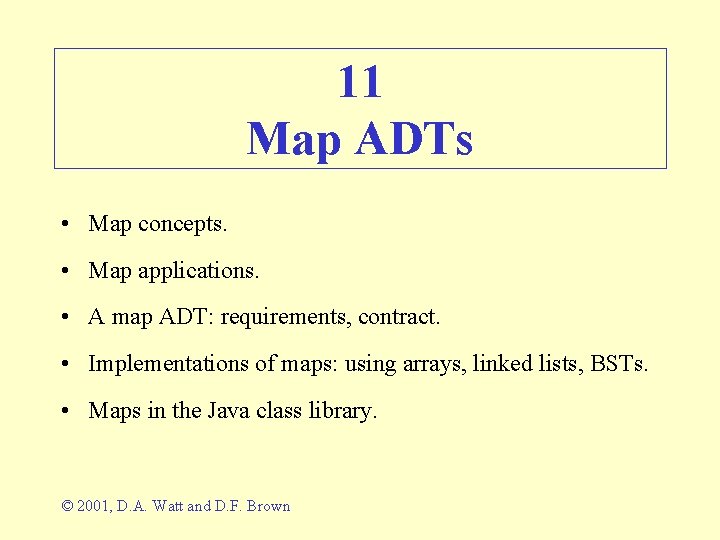
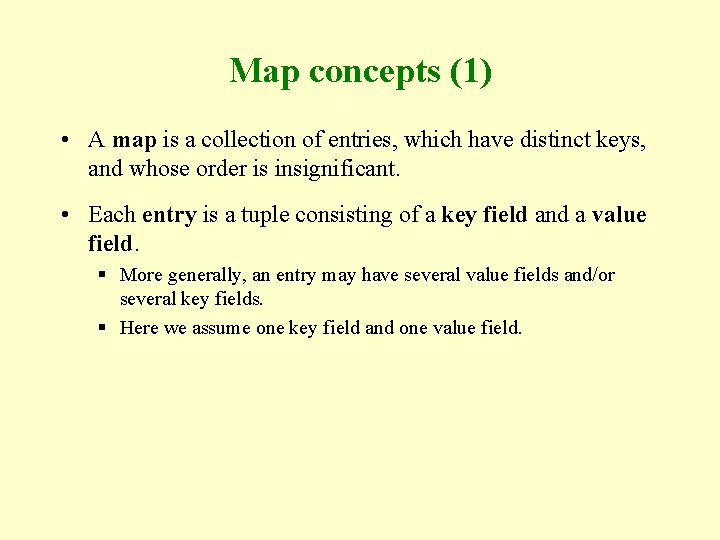
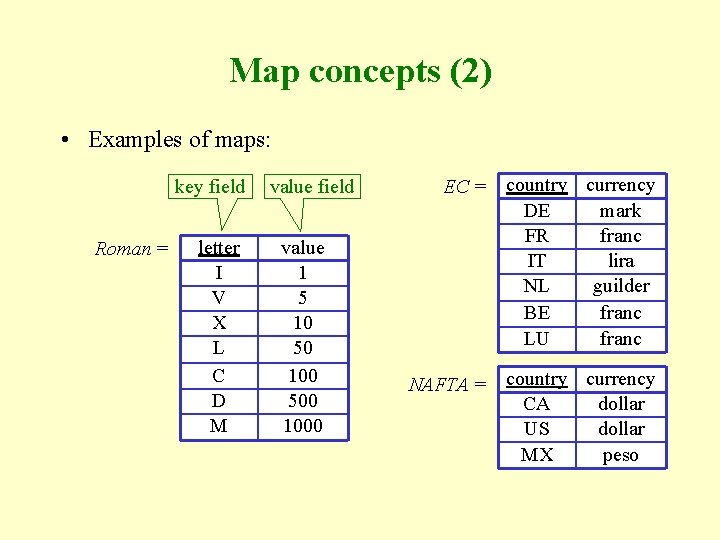
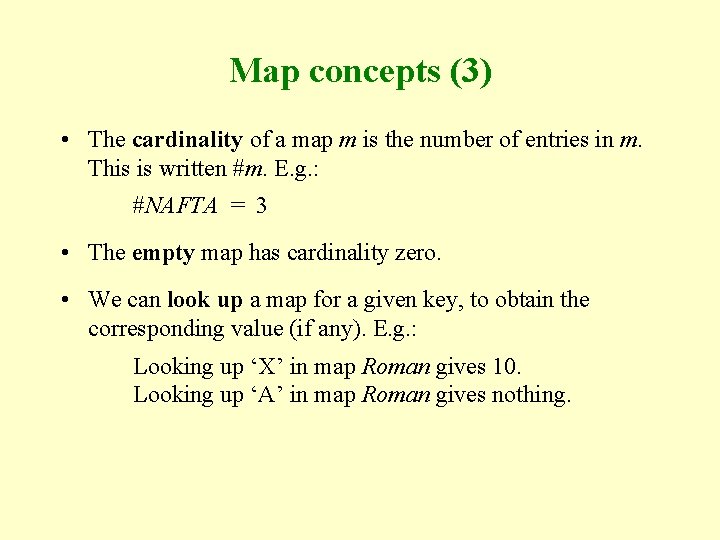
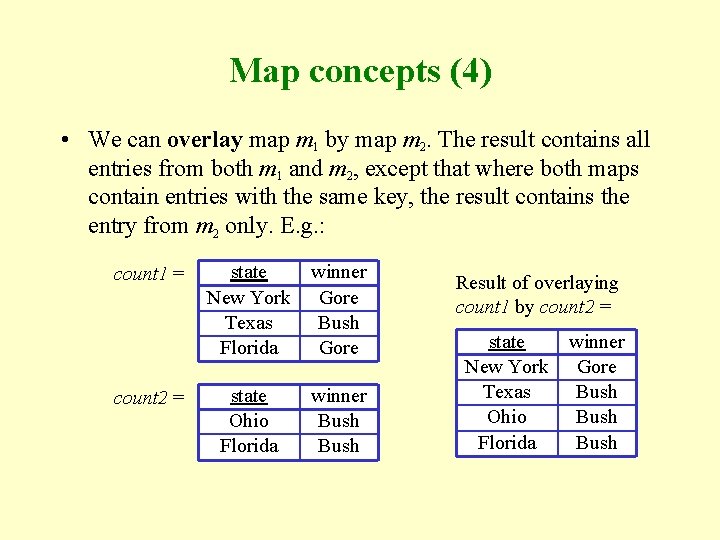
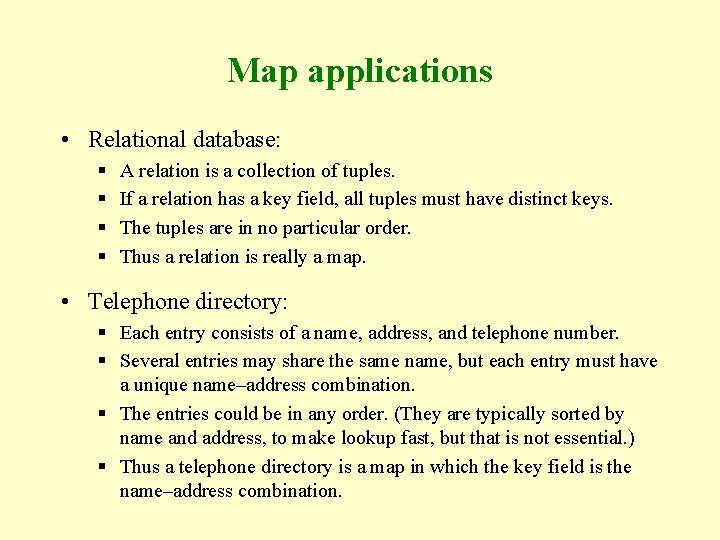
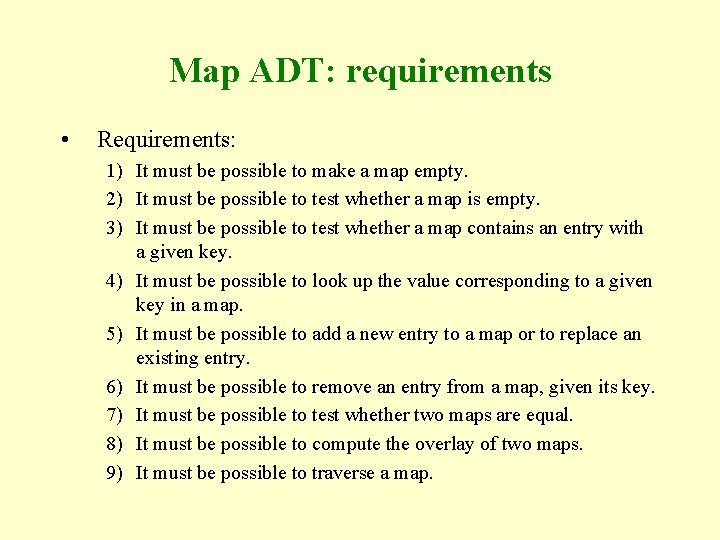
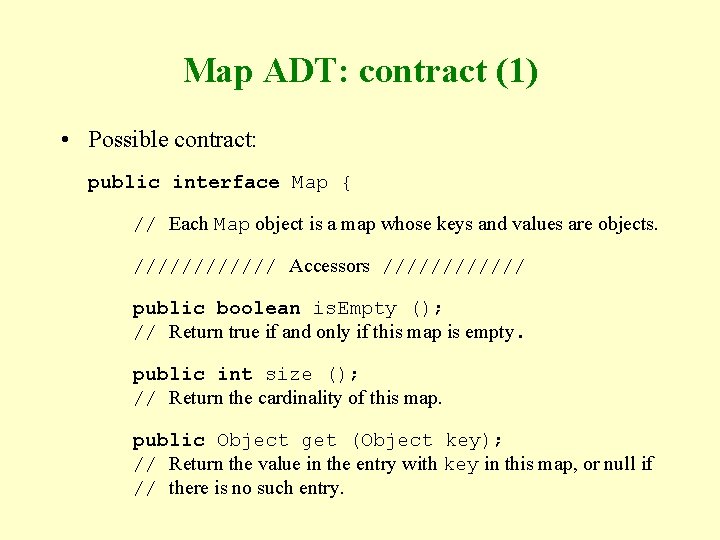
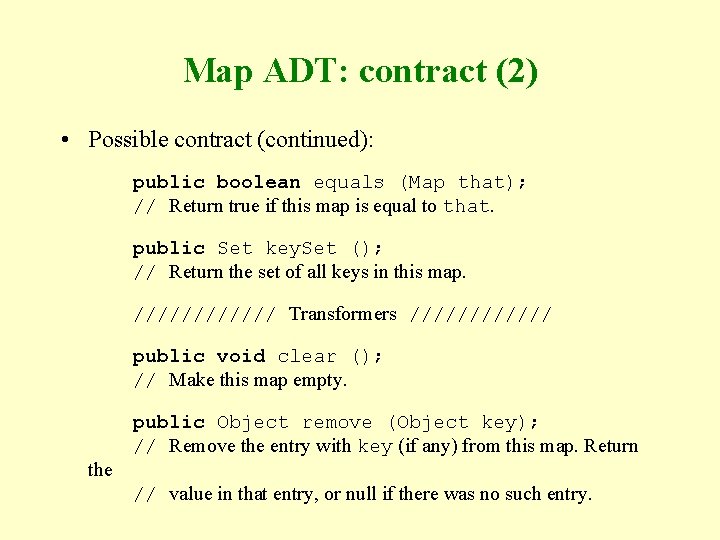
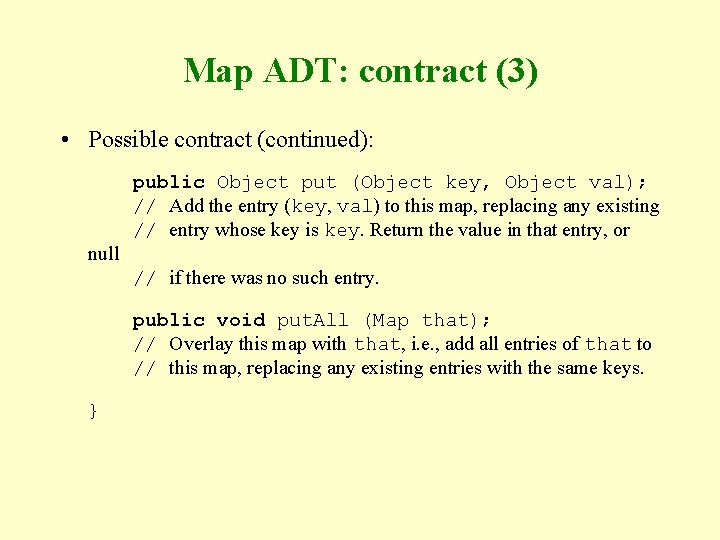
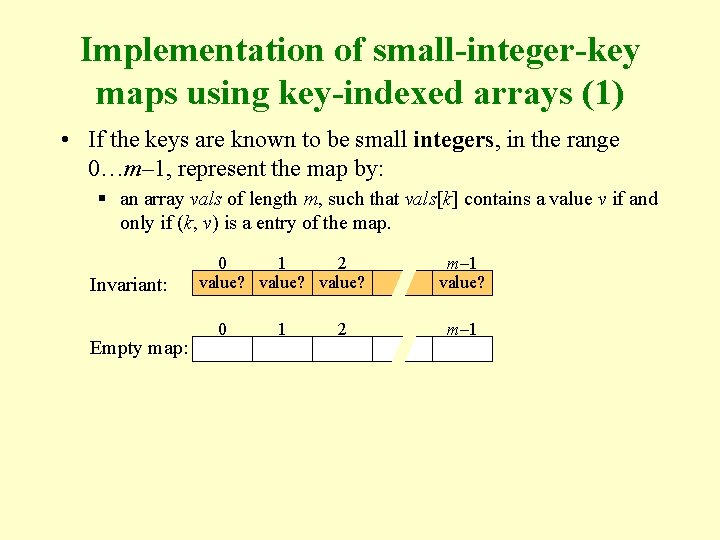
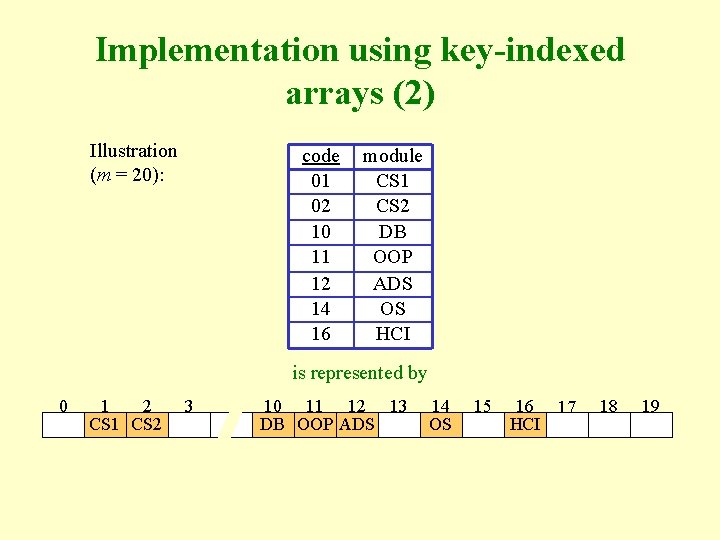
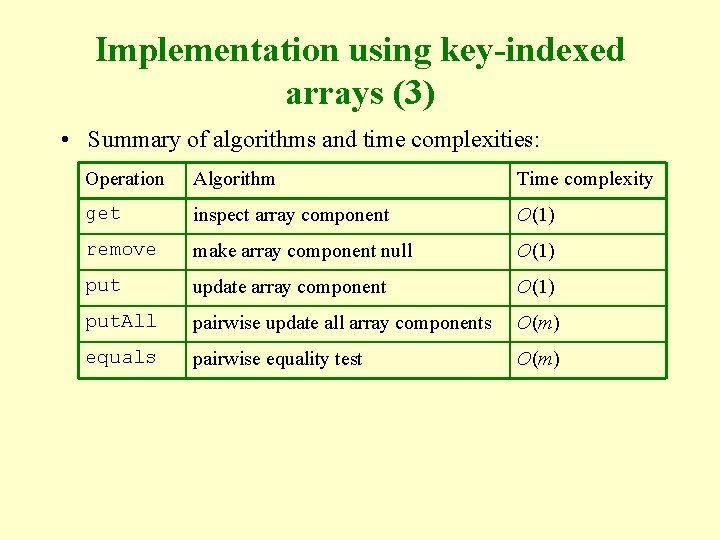
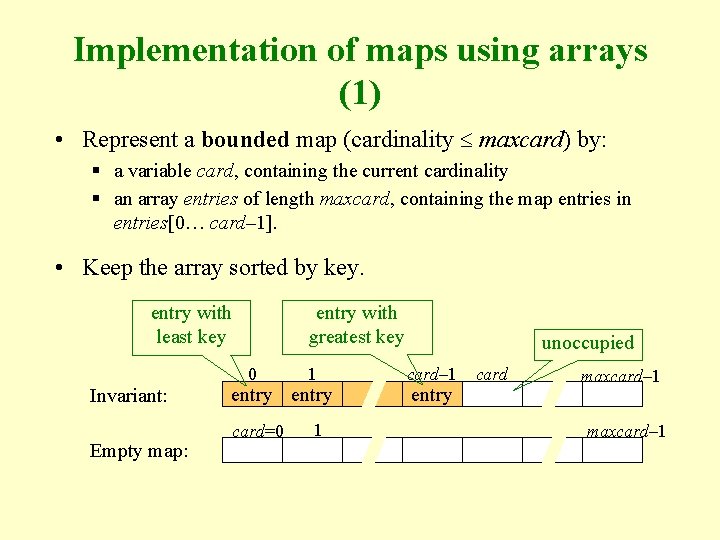
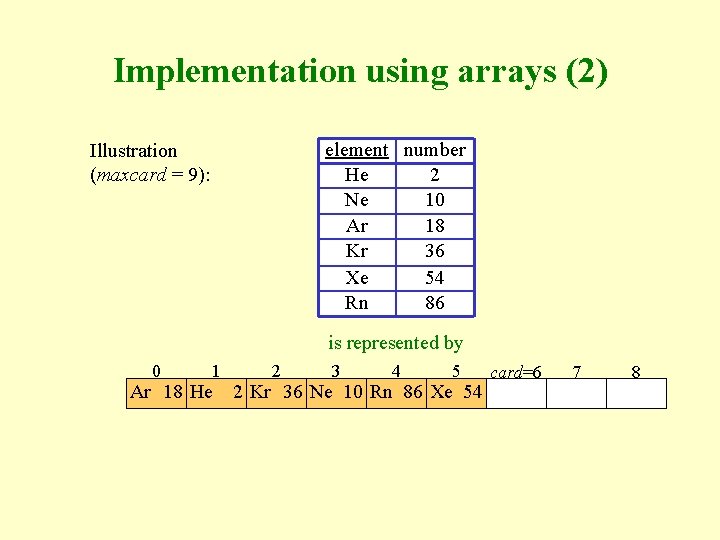
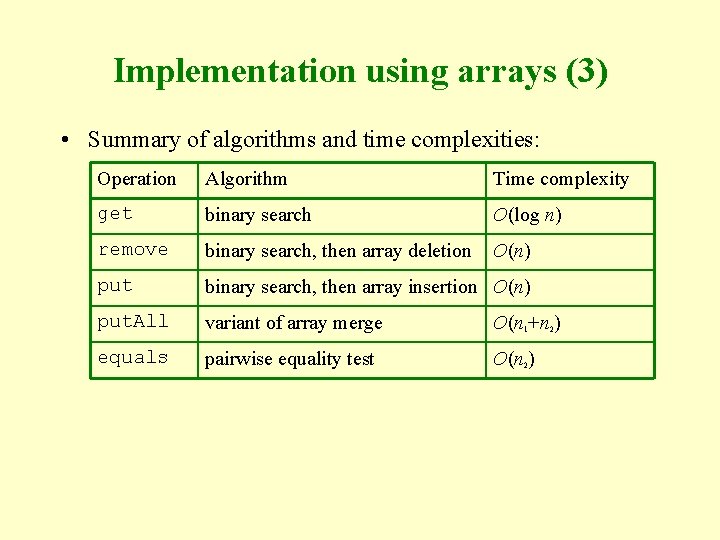
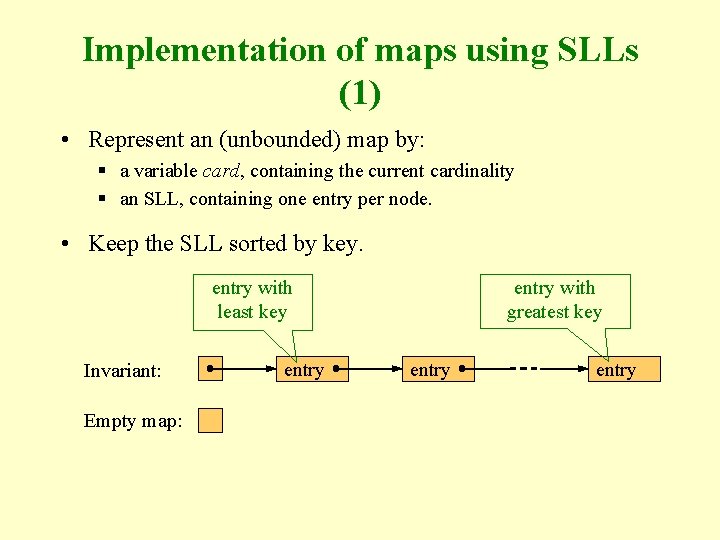
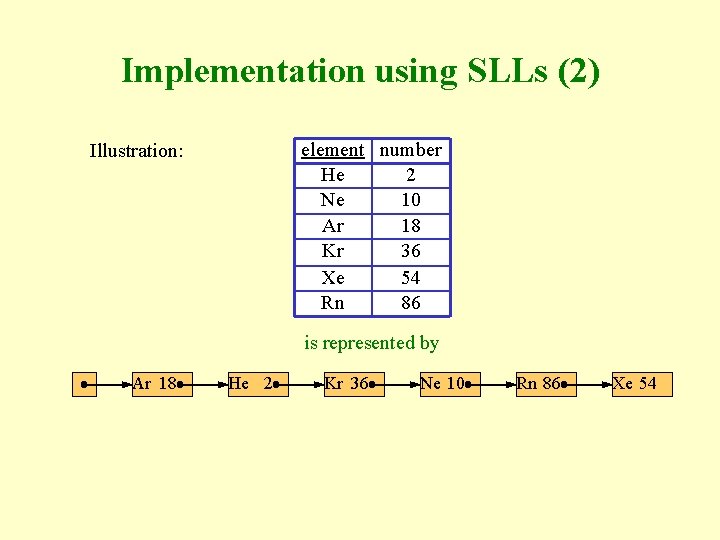
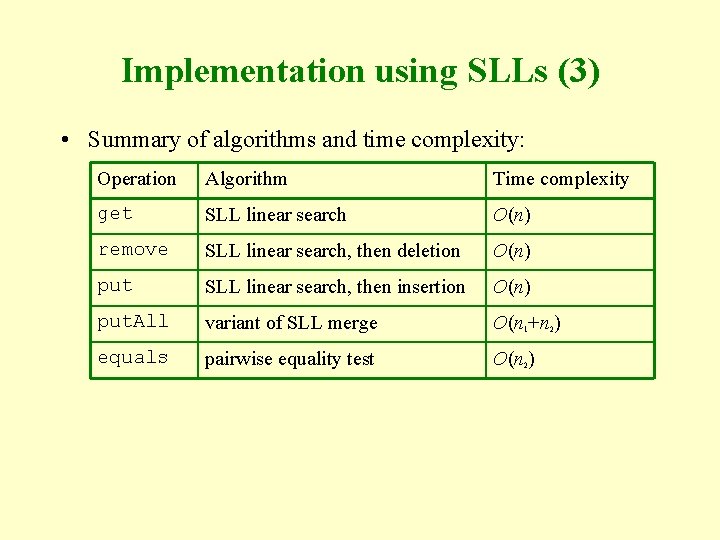
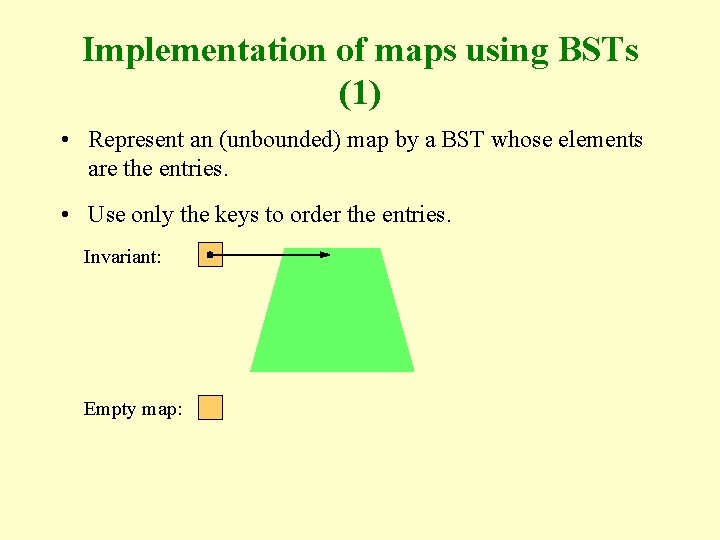
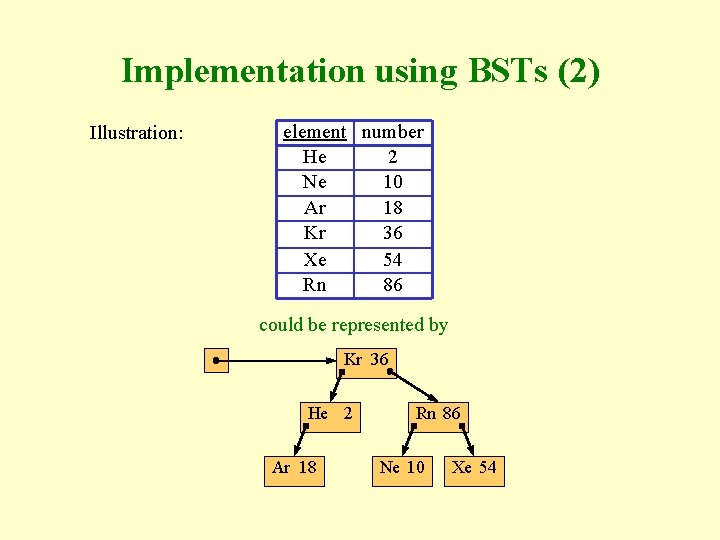
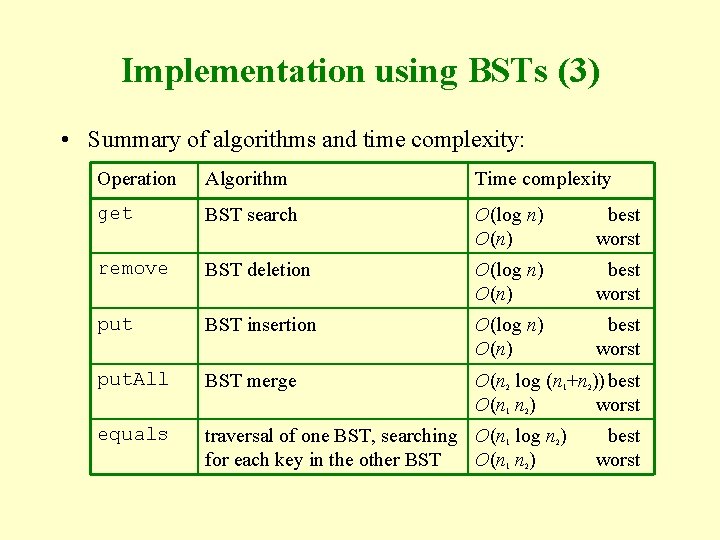
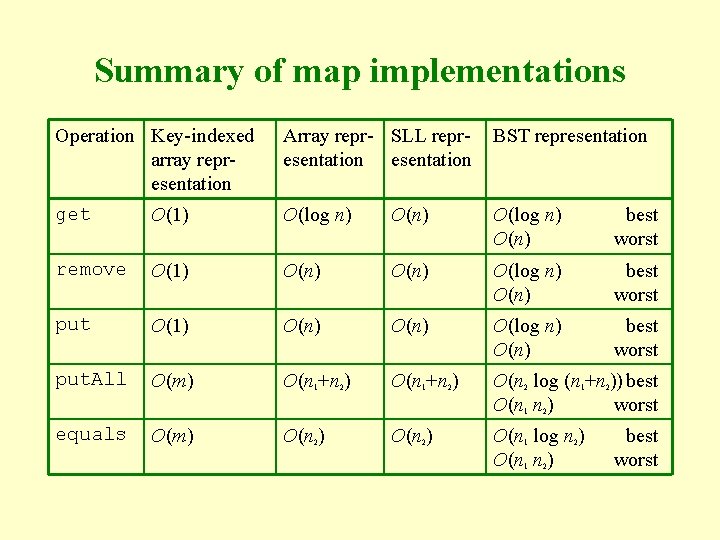
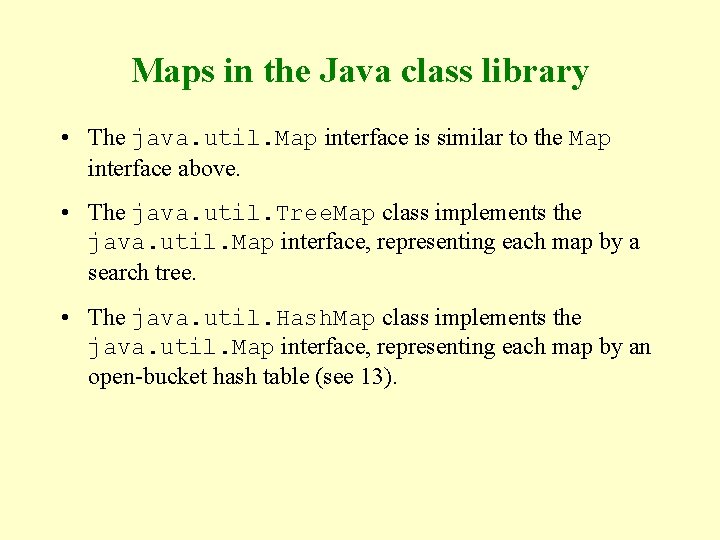
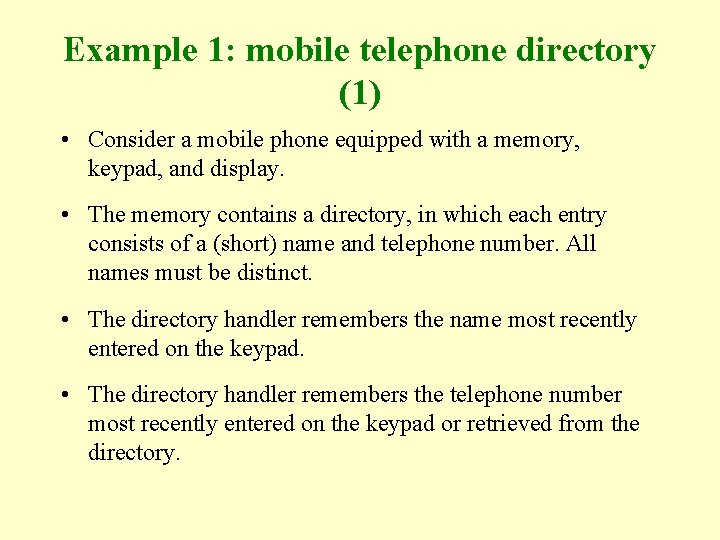
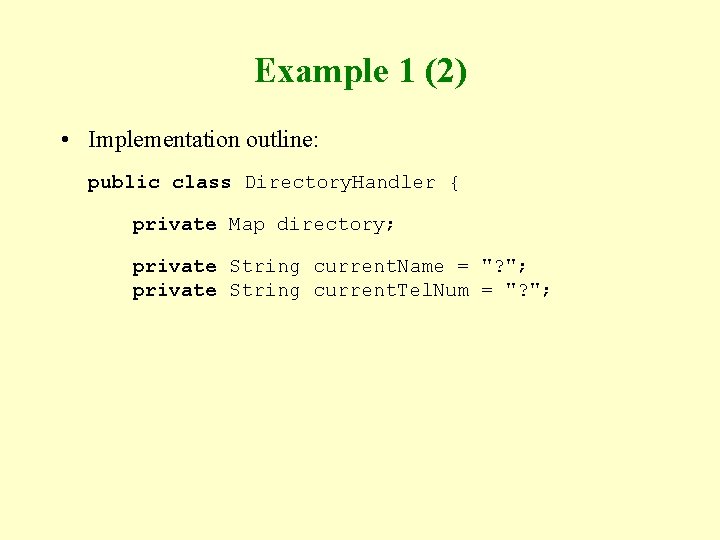
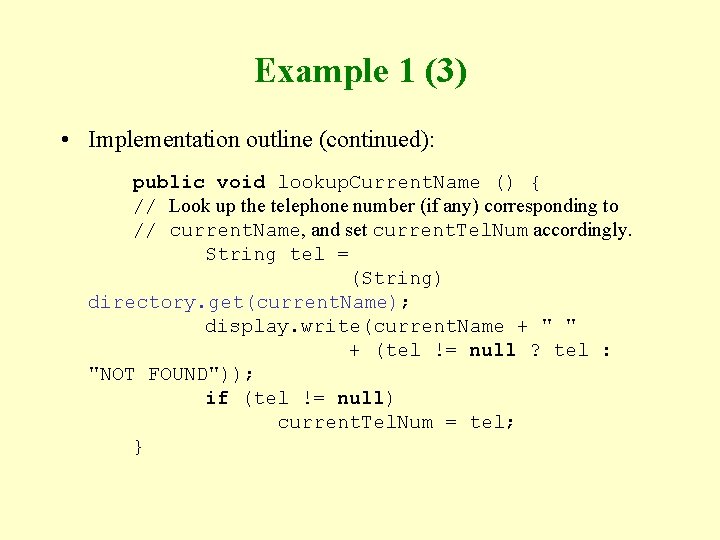
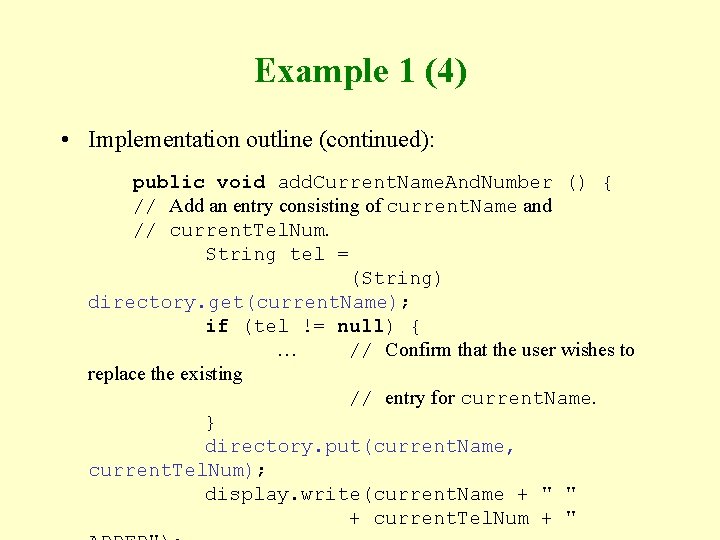
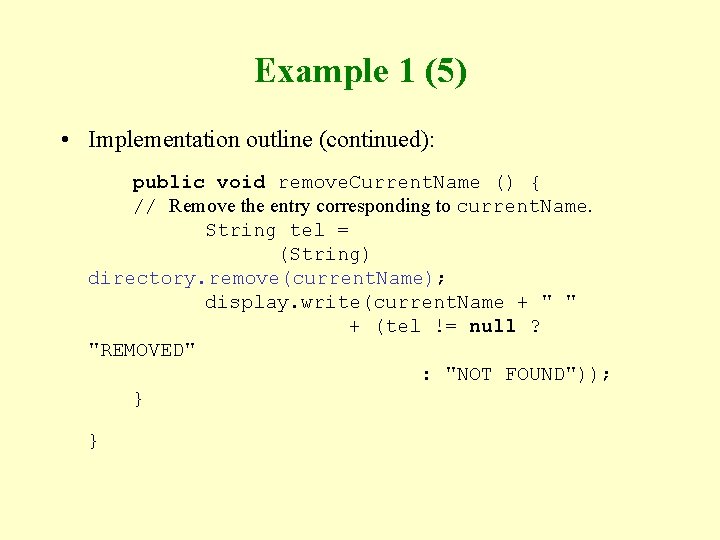
- Slides: 29
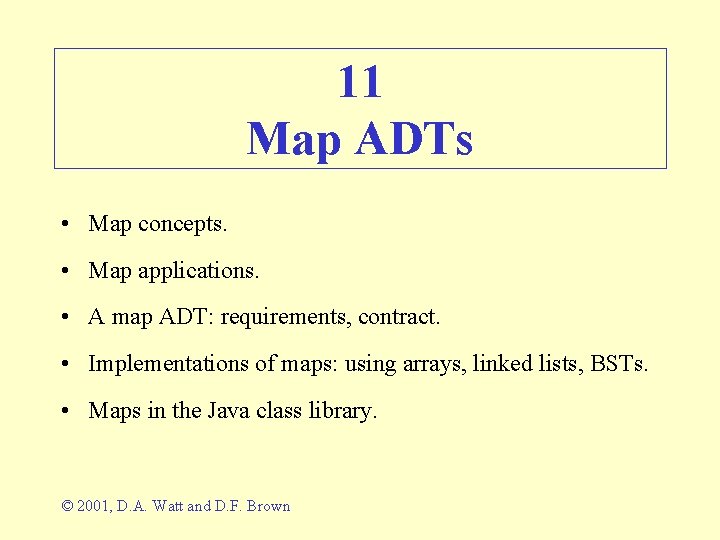
11 Map ADTs • Map concepts. • Map applications. • A map ADT: requirements, contract. • Implementations of maps: using arrays, linked lists, BSTs. • Maps in the Java class library. © 2001, D. A. Watt and D. F. Brown
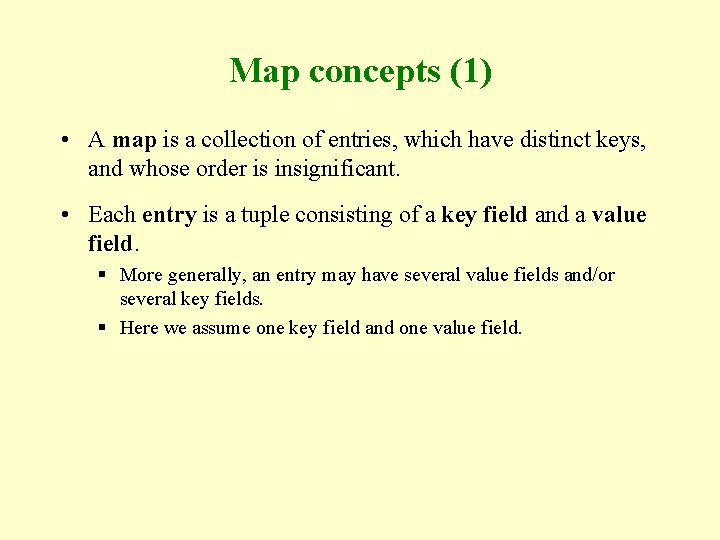
Map concepts (1) • A map is a collection of entries, which have distinct keys, and whose order is insignificant. • Each entry is a tuple consisting of a key field and a value field. § More generally, an entry may have several value fields and/or several key fields. § Here we assume one key field and one value field.
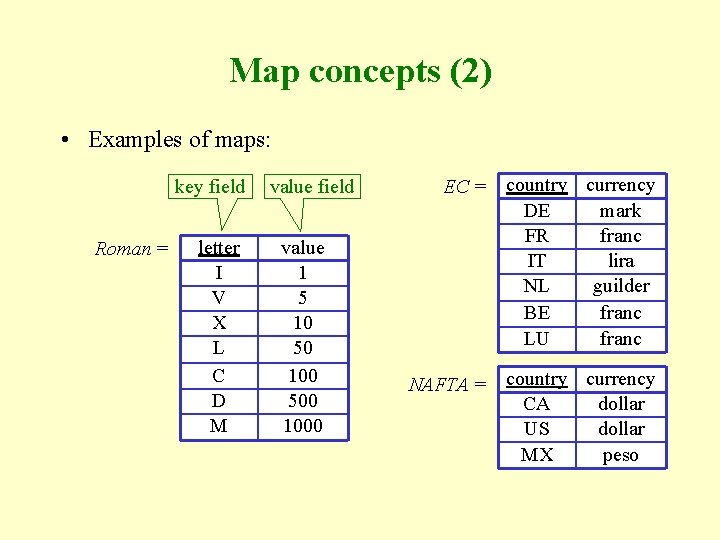
Map concepts (2) • Examples of maps: key field Roman = letter I V X L C D M value field value 1 5 10 50 100 500 1000 EC = country currency DE mark FR franc IT lira NL guilder BE franc LU franc NAFTA = country currency CA dollar US dollar MX peso
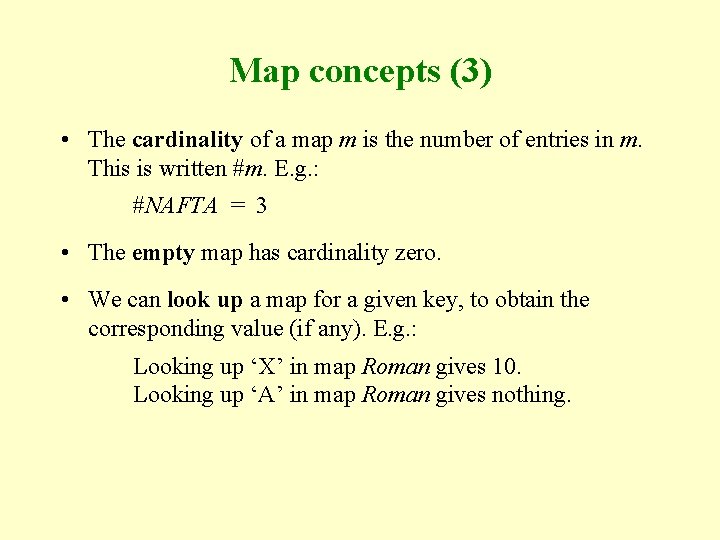
Map concepts (3) • The cardinality of a map m is the number of entries in m. This is written #m. E. g. : #NAFTA = 3 • The empty map has cardinality zero. • We can look up a map for a given key, to obtain the corresponding value (if any). E. g. : Looking up ‘X’ in map Roman gives 10. Looking up ‘A’ in map Roman gives nothing.
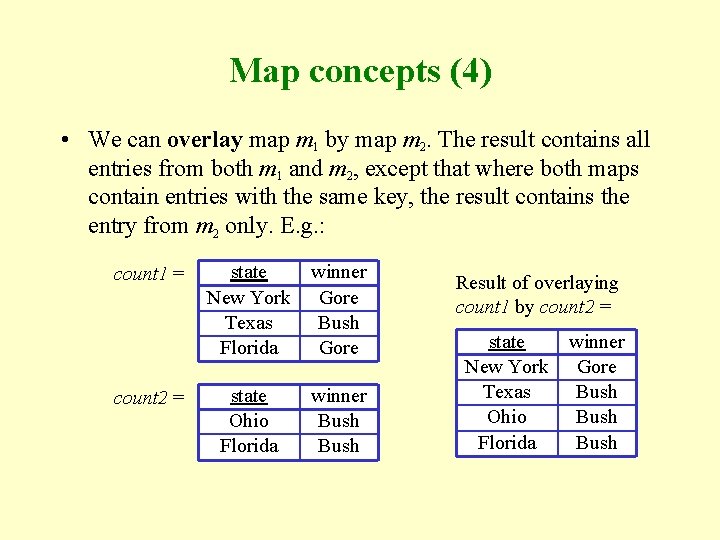
Map concepts (4) • We can overlay map m 1 by map m 2. The result contains all entries from both m 1 and m 2, except that where both maps contain entries with the same key, the result contains the entry from m 2 only. E. g. : count 1 = count 2 = state New York Texas Florida winner Gore Bush Gore state Ohio Florida winner Bush Result of overlaying count 1 by count 2 = state New York Texas Ohio Florida winner Gore Bush
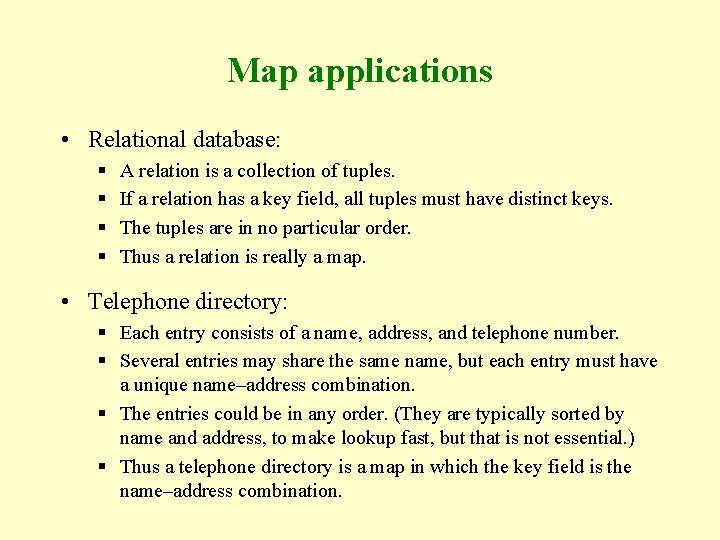
Map applications • Relational database: § § A relation is a collection of tuples. If a relation has a key field, all tuples must have distinct keys. The tuples are in no particular order. Thus a relation is really a map. • Telephone directory: § Each entry consists of a name, address, and telephone number. § Several entries may share the same name, but each entry must have a unique name–address combination. § The entries could be in any order. (They are typically sorted by name and address, to make lookup fast, but that is not essential. ) § Thus a telephone directory is a map in which the key field is the name–address combination.
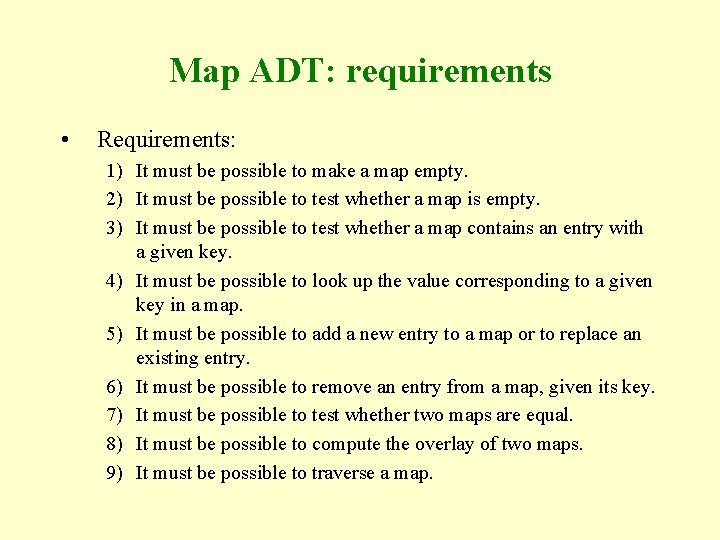
Map ADT: requirements • Requirements: 1) It must be possible to make a map empty. 2) It must be possible to test whether a map is empty. 3) It must be possible to test whether a map contains an entry with a given key. 4) It must be possible to look up the value corresponding to a given key in a map. 5) It must be possible to add a new entry to a map or to replace an existing entry. 6) It must be possible to remove an entry from a map, given its key. 7) It must be possible to test whether two maps are equal. 8) It must be possible to compute the overlay of two maps. 9) It must be possible to traverse a map.
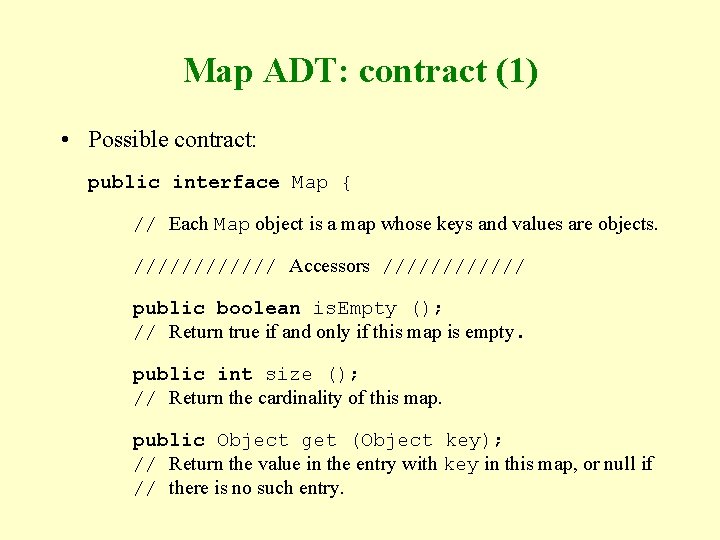
Map ADT: contract (1) • Possible contract: public interface Map { // Each Map object is a map whose keys and values are objects. ////// Accessors ////// public boolean is. Empty (); // Return true if and only if this map is empty. public int size (); // Return the cardinality of this map. public Object get (Object key); // Return the value in the entry with key in this map, or null if // there is no such entry.
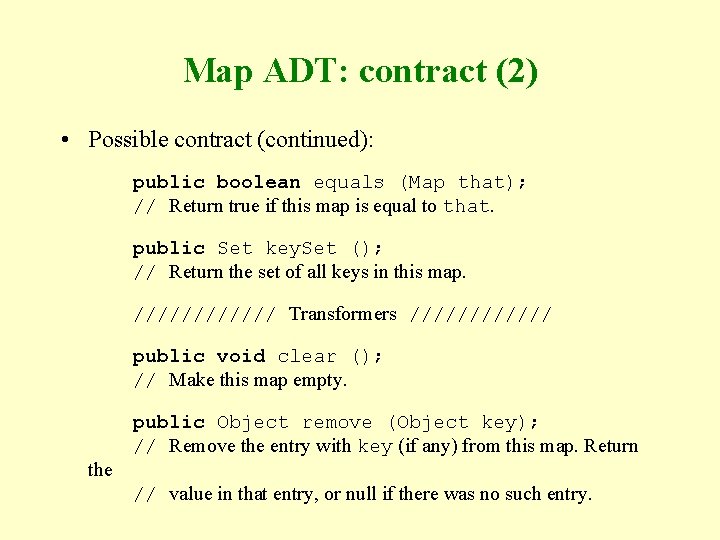
Map ADT: contract (2) • Possible contract (continued): public boolean equals (Map that); // Return true if this map is equal to that. public Set key. Set (); // Return the set of all keys in this map. ////// Transformers ////// public void clear (); // Make this map empty. public Object remove (Object key); // Remove the entry with key (if any) from this map. Return the // value in that entry, or null if there was no such entry.
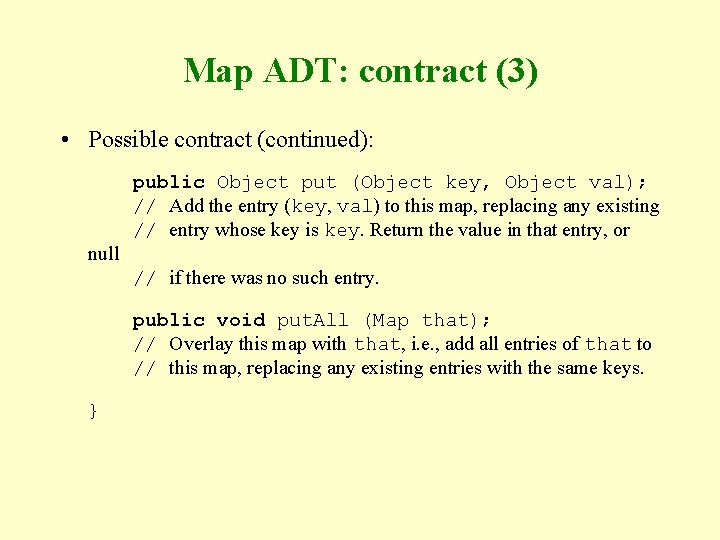
Map ADT: contract (3) • Possible contract (continued): public Object put (Object key, Object val); // Add the entry (key, val) to this map, replacing any existing // entry whose key is key. Return the value in that entry, or null // if there was no such entry. public void put. All (Map that); // Overlay this map with that, i. e. , add all entries of that to // this map, replacing any existing entries with the same keys. }
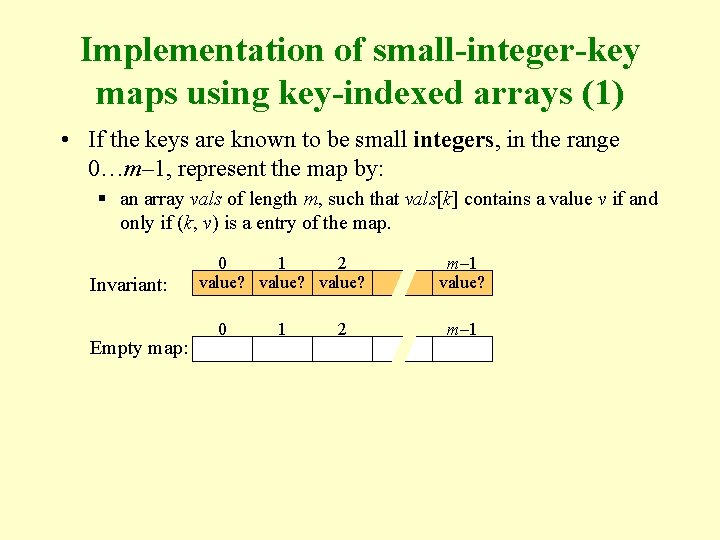
Implementation of small-integer-key maps using key-indexed arrays (1) • If the keys are known to be small integers, in the range 0…m– 1, represent the map by: § an array vals of length m, such that vals[k] contains a value v if and only if (k, v) is a entry of the map. Invariant: Empty map: 0 1 2 value? 0 1 2 m– 1 value? m– 1
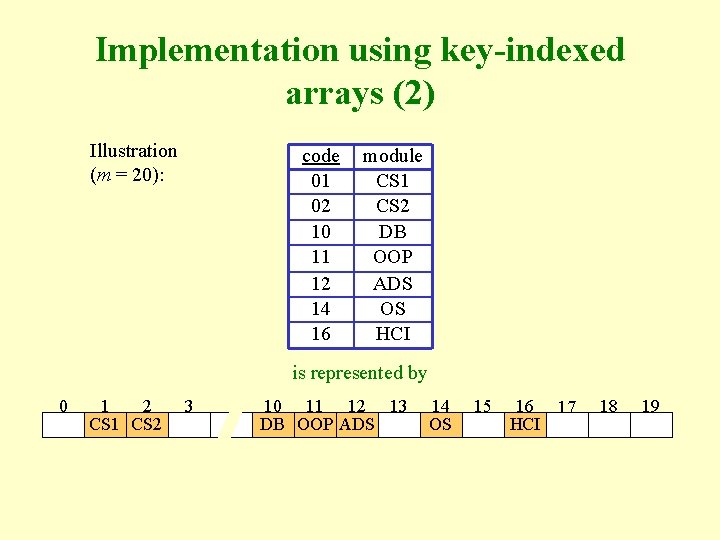
Implementation using key-indexed arrays (2) Illustration (m = 20): code 01 02 10 11 12 14 16 module CS 1 CS 2 DB OOP ADS OS HCI is represented by 0 1 2 CS 1 CS 2 3 10 11 12 13 DB OOP ADS 14 OS 15 16 17 HCI 18 19
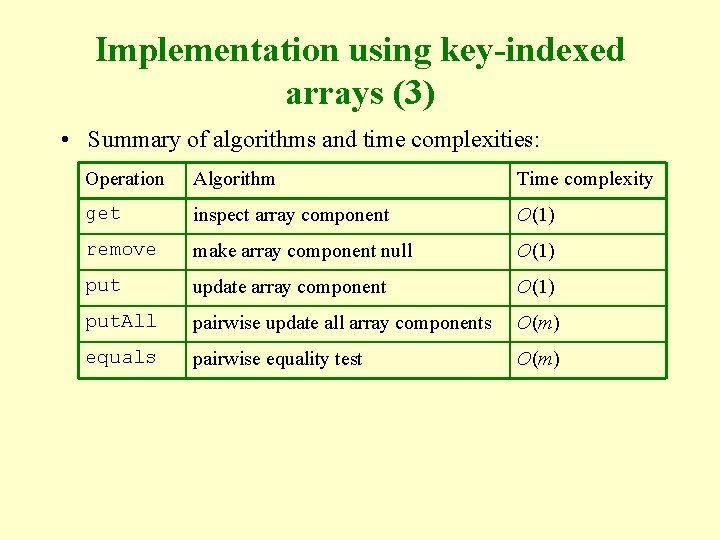
Implementation using key-indexed arrays (3) • Summary of algorithms and time complexities: Operation Algorithm Time complexity get inspect array component O(1) remove make array component null O(1) put update array component O(1) put. All pairwise update all array components O(m) equals pairwise equality test O(m)
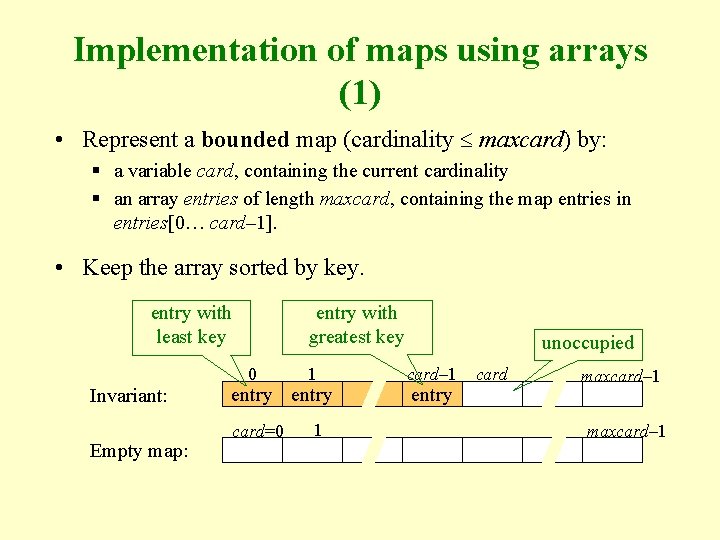
Implementation of maps using arrays (1) • Represent a bounded map (cardinality maxcard) by: § a variable card, containing the current cardinality § an array entries of length maxcard, containing the map entries in entries[0… card– 1]. • Keep the array sorted by key. entry with least key Invariant: Empty map: entry with greatest key 0 1 entry card=0 1 unoccupied card– 1 entry card maxcard– 1
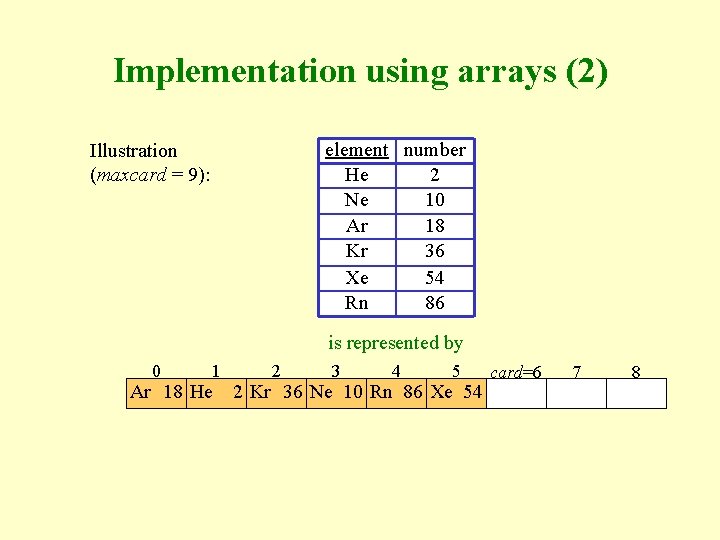
Implementation using arrays (2) element number He 2 Ne 10 Ar 18 Kr 36 Xe 54 Rn 86 Illustration (maxcard = 9): is represented by 0 1 2 3 4 5 Ar 18 He 2 Kr 36 Ne 10 Rn 86 Xe 54 card=6 7 8
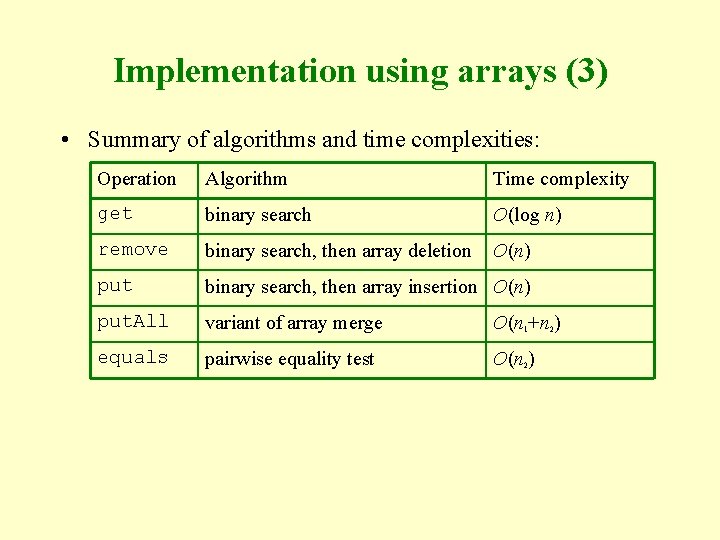
Implementation using arrays (3) • Summary of algorithms and time complexities: Operation Algorithm Time complexity get binary search O(log n) remove binary search, then array deletion O(n) put binary search, then array insertion O(n) put. All variant of array merge O(n +n ) equals pairwise equality test O(n ) 1 2 2
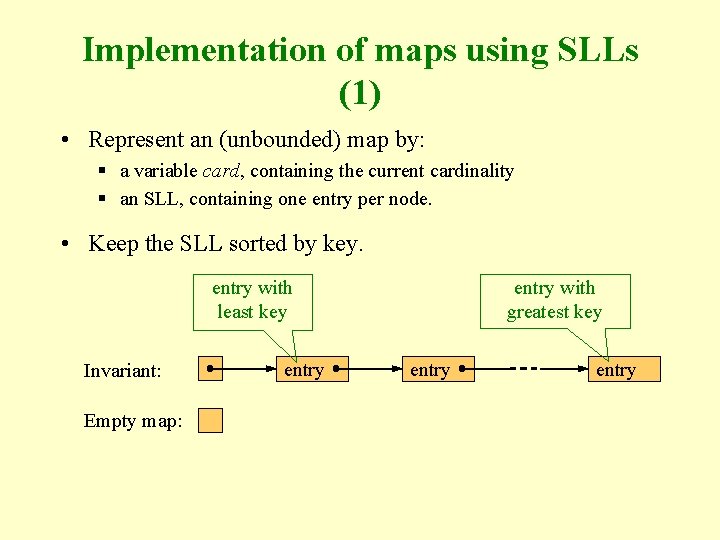
Implementation of maps using SLLs (1) • Represent an (unbounded) map by: § a variable card, containing the current cardinality § an SLL, containing one entry per node. • Keep the SLL sorted by key. entry with least key Invariant: Empty map: entry with greatest key entry
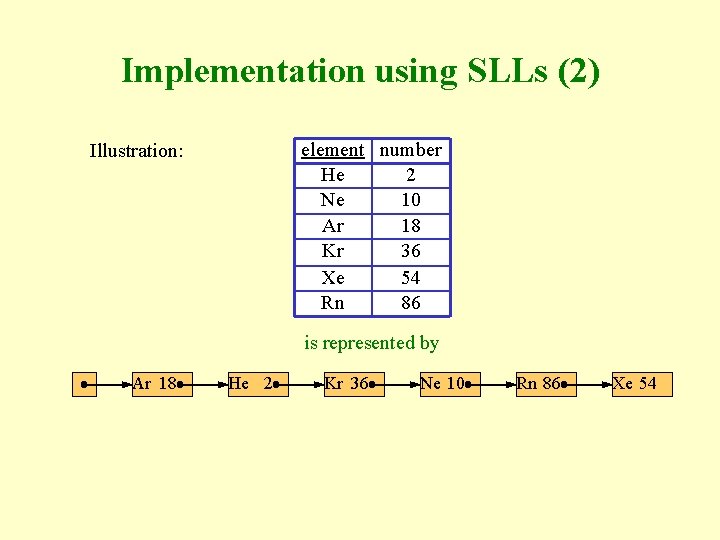
Implementation using SLLs (2) element number He 2 Ne 10 Ar 18 Kr 36 Xe 54 Rn 86 Illustration: is represented by Ar 18 He 2 Kr 36 Ne 10 Rn 86 Xe 54
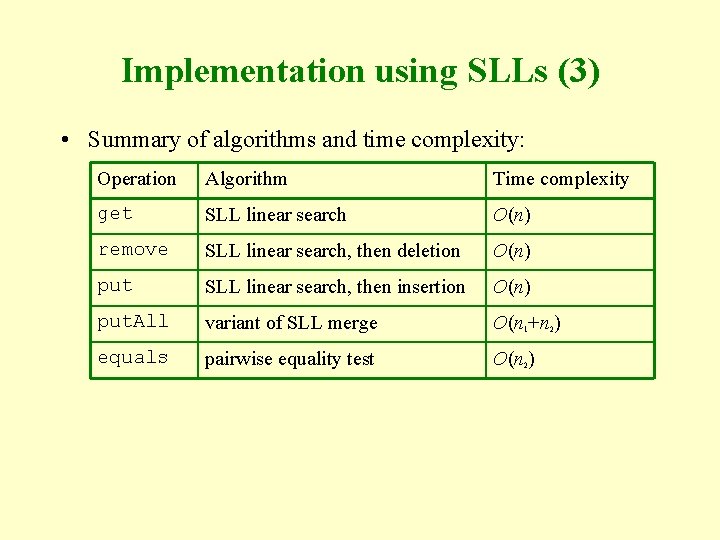
Implementation using SLLs (3) • Summary of algorithms and time complexity: Operation Algorithm Time complexity get SLL linear search O(n) remove SLL linear search, then deletion O(n) put SLL linear search, then insertion O(n) put. All variant of SLL merge O(n +n ) equals pairwise equality test O(n ) 1 2 2
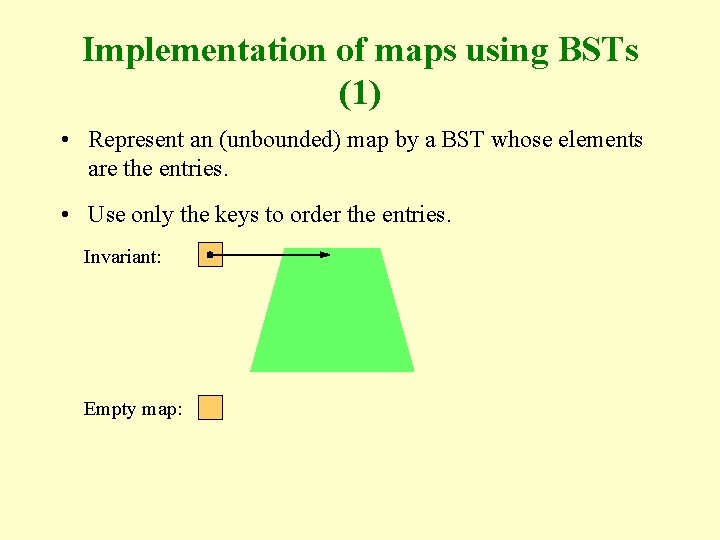
Implementation of maps using BSTs (1) • Represent an (unbounded) map by a BST whose elements are the entries. • Use only the keys to order the entries. Invariant: Empty map:
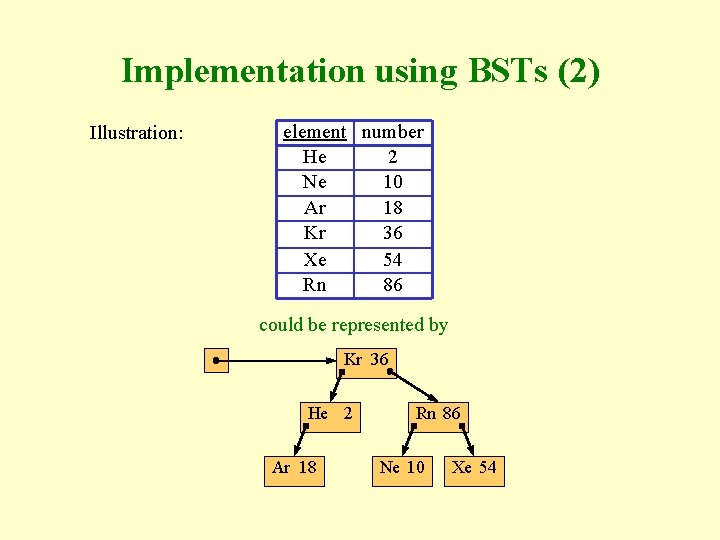
Implementation using BSTs (2) Illustration: element number He 2 Ne 10 Ar 18 Kr 36 Xe 54 Rn 86 could be represented by Kr 36 He 2 Ar 18 Rn 86 Ne 10 Xe 54
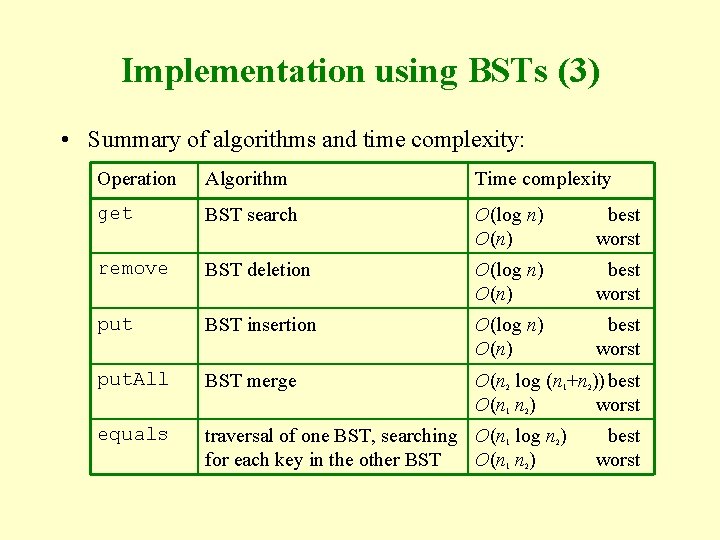
Implementation using BSTs (3) • Summary of algorithms and time complexity: Operation Algorithm Time complexity get BST search O(log n) O(n) best worst remove BST deletion O(log n) O(n) best worst put BST insertion O(log n) O(n) best worst put. All BST merge O(n log (n +n )) best O(n n ) worst 2 1 equals 1 2 traversal of one BST, searching O(n log n ) for each key in the other BST O(n n ) 1 1 2 2 2 best worst
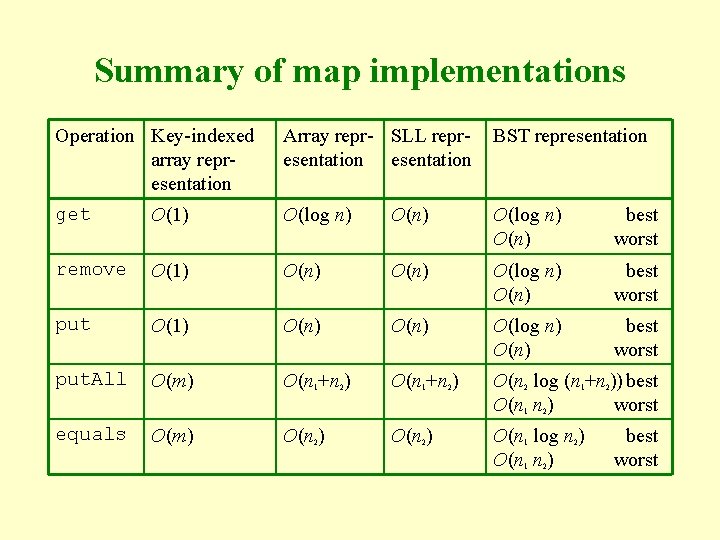
Summary of map implementations Operation Key-indexed array representation get O(1) Array repr- SLL representation BST representation O(log n) O(n) best worst remove O(1) O(n) O(log n) O(n) best worst put. All O(m) O(n +n ) 1 2 O(n log (n +n )) best O(n n ) worst 2 1 equals O(m) O(n ) 2 1 2 O(n log n ) O(n n ) 1 1 2 2 2 best worst
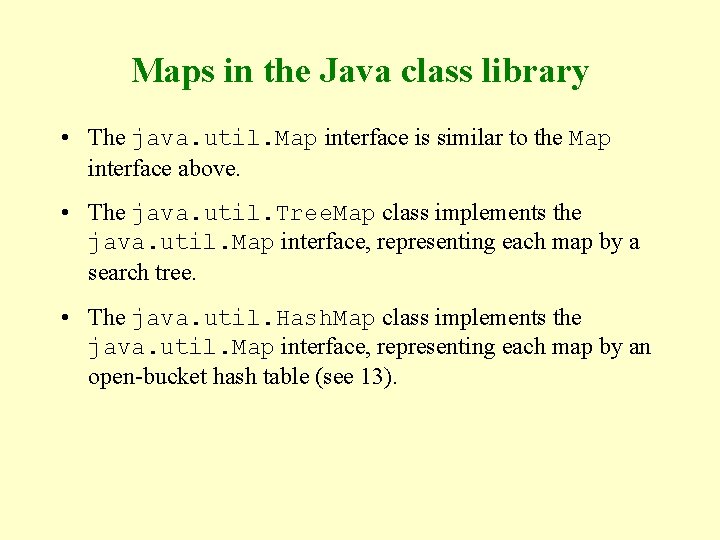
Maps in the Java class library • The java. util. Map interface is similar to the Map interface above. • The java. util. Tree. Map class implements the java. util. Map interface, representing each map by a search tree. • The java. util. Hash. Map class implements the java. util. Map interface, representing each map by an open-bucket hash table (see 13).
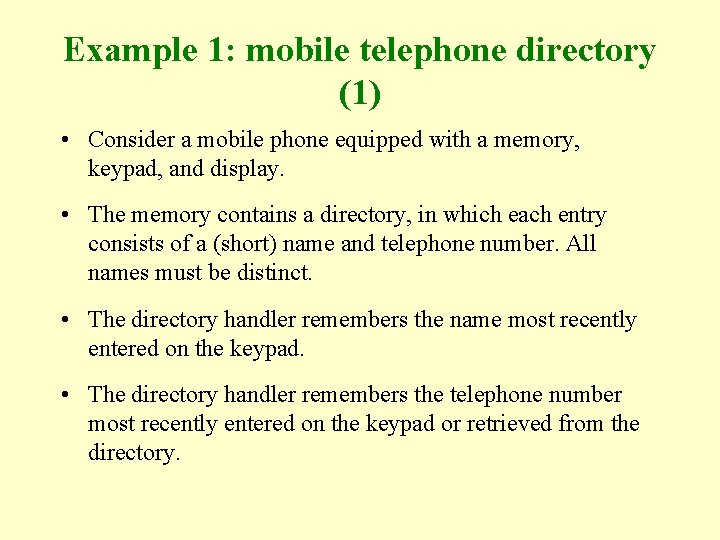
Example 1: mobile telephone directory (1) • Consider a mobile phone equipped with a memory, keypad, and display. • The memory contains a directory, in which each entry consists of a (short) name and telephone number. All names must be distinct. • The directory handler remembers the name most recently entered on the keypad. • The directory handler remembers the telephone number most recently entered on the keypad or retrieved from the directory.
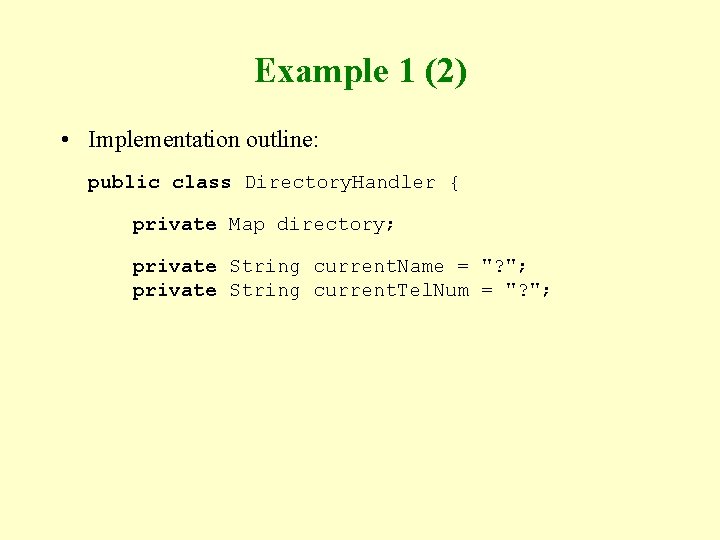
Example 1 (2) • Implementation outline: public class Directory. Handler { private Map directory; private String current. Name = "? "; private String current. Tel. Num = "? ";
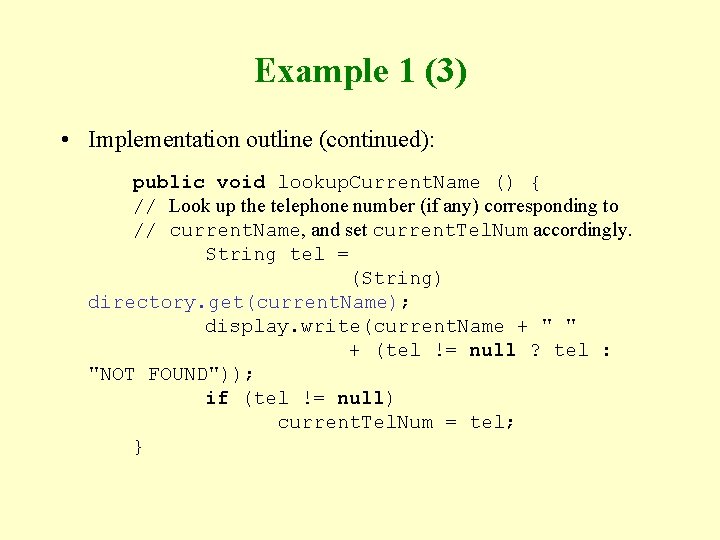
Example 1 (3) • Implementation outline (continued): public void lookup. Current. Name () { // Look up the telephone number (if any) corresponding to // current. Name, and set current. Tel. Num accordingly. String tel = (String) directory. get(current. Name); display. write(current. Name + " " + (tel != null ? tel : "NOT FOUND")); if (tel != null) current. Tel. Num = tel; }
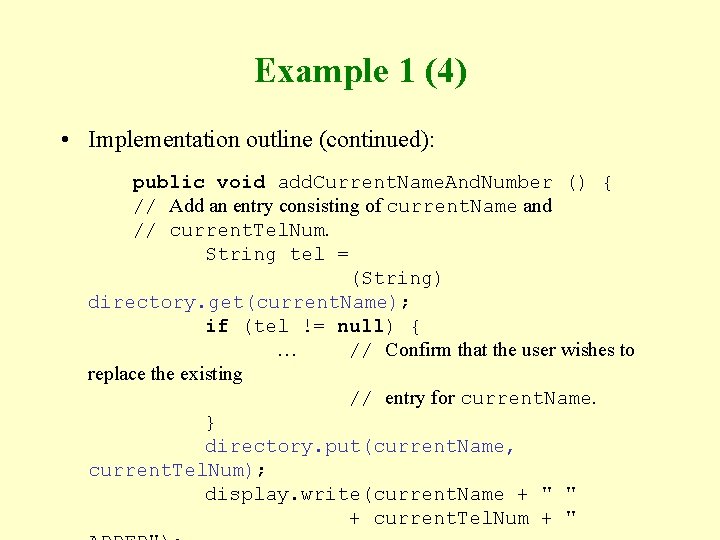
Example 1 (4) • Implementation outline (continued): public void add. Current. Name. And. Number () { // Add an entry consisting of current. Name and // current. Tel. Num. String tel = (String) directory. get(current. Name); if (tel != null) { … // Confirm that the user wishes to replace the existing // entry for current. Name. } directory. put(current. Name, current. Tel. Num); display. write(current. Name + " " + current. Tel. Num + "
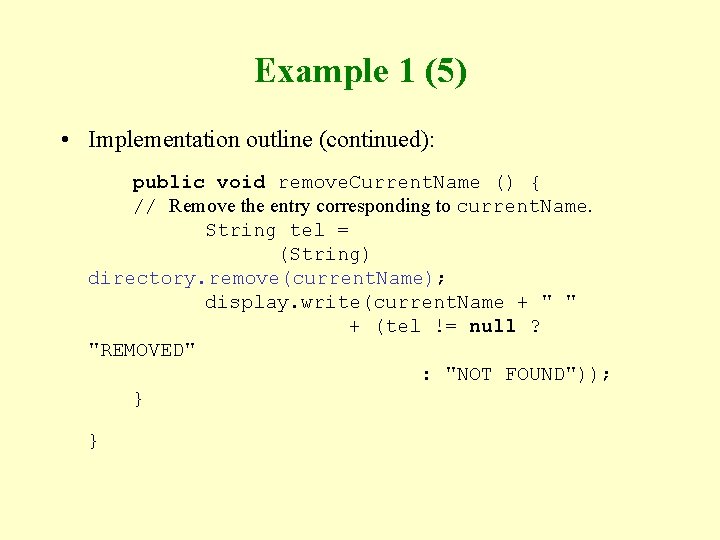
Example 1 (5) • Implementation outline (continued): public void remove. Current. Name () { // Remove the entry corresponding to current. Name. String tel = (String) directory. remove(current. Name); display. write(current. Name + " " + (tel != null ? "REMOVED" : "NOT FOUND")); } }