11 Functions and Modules Mark Dixon SOFT 131
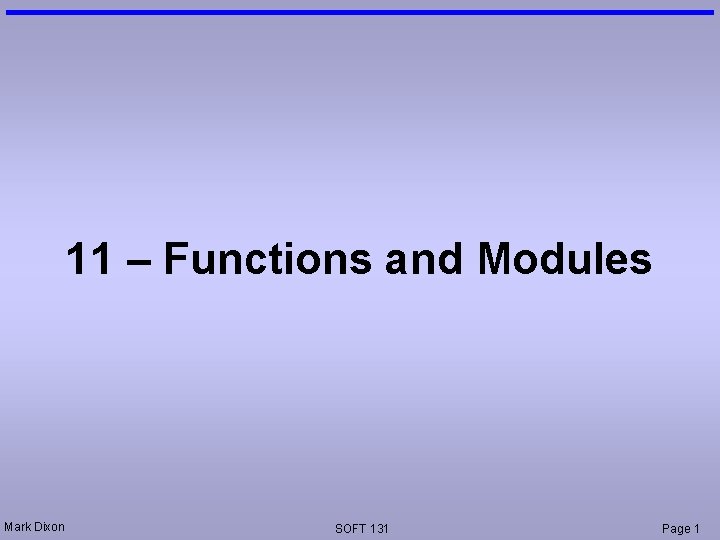
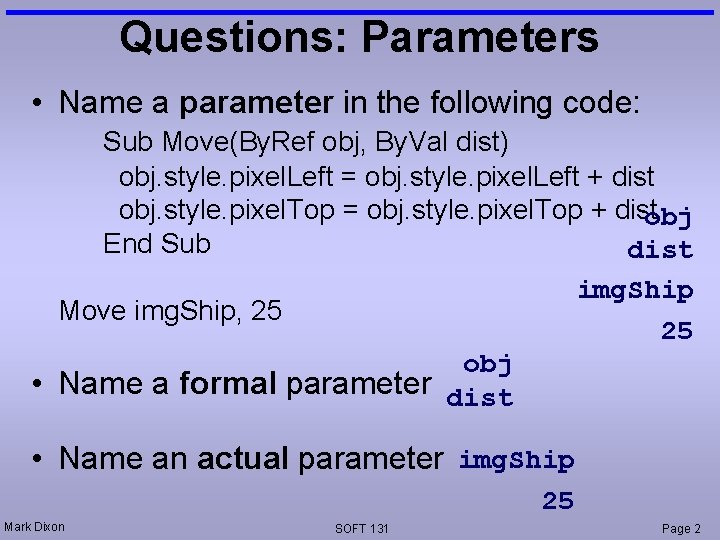
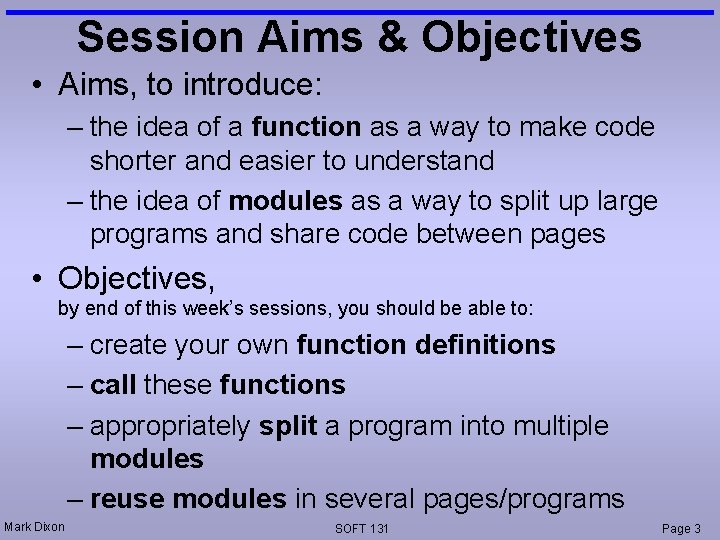
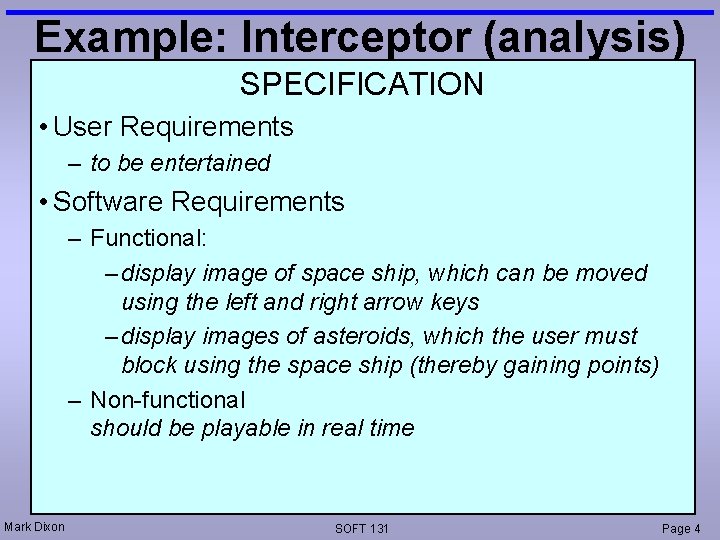
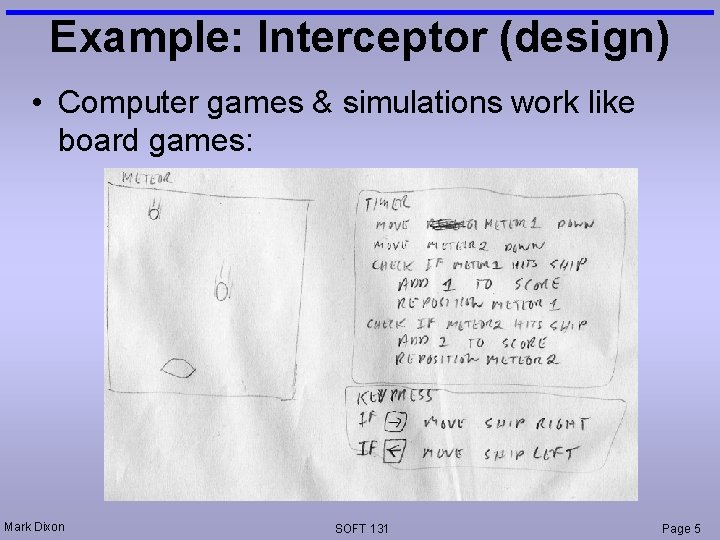
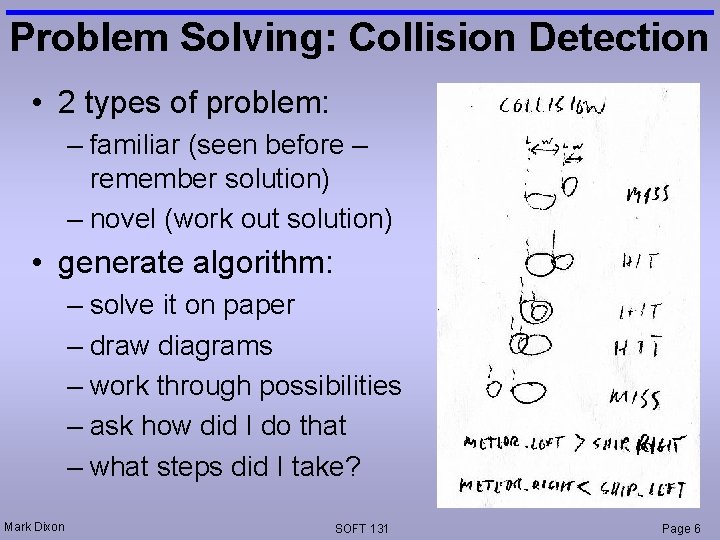
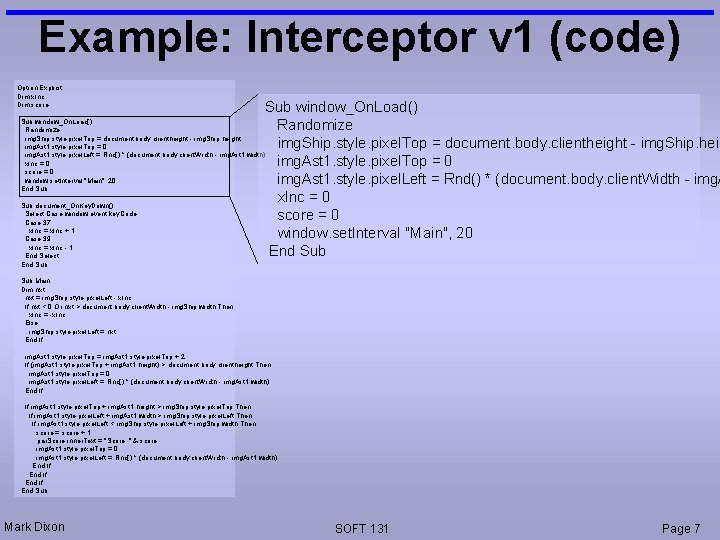
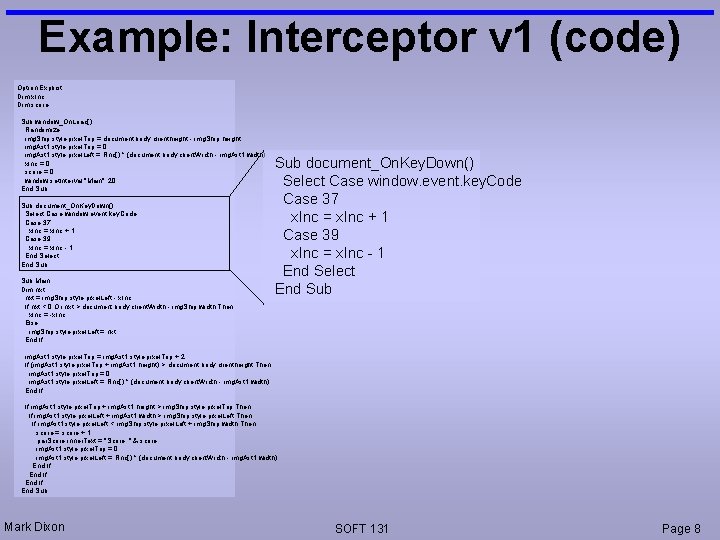
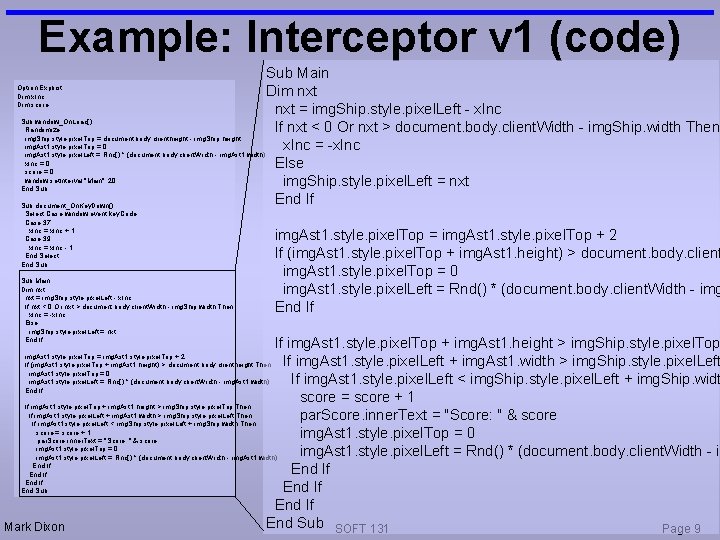
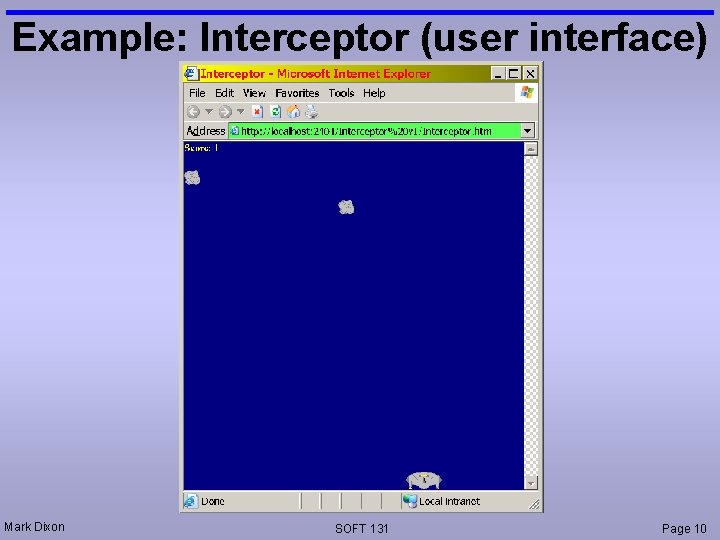
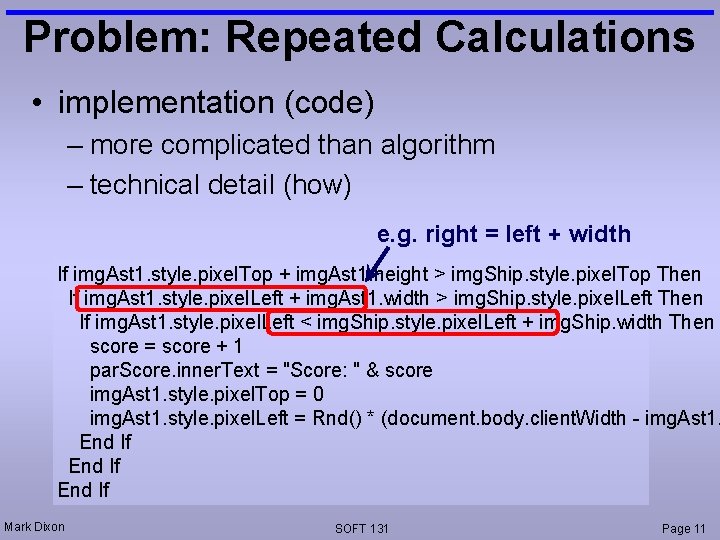
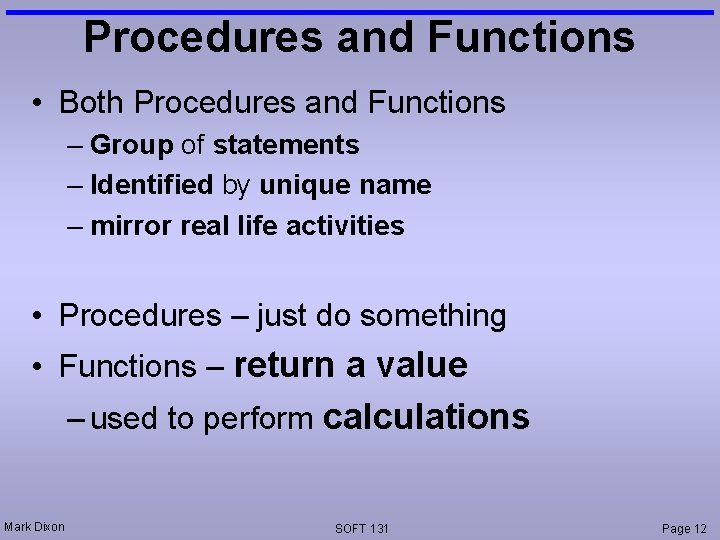
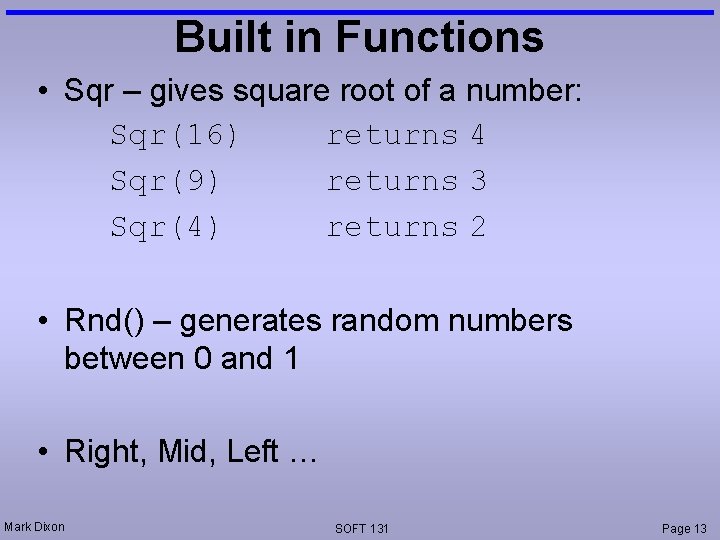
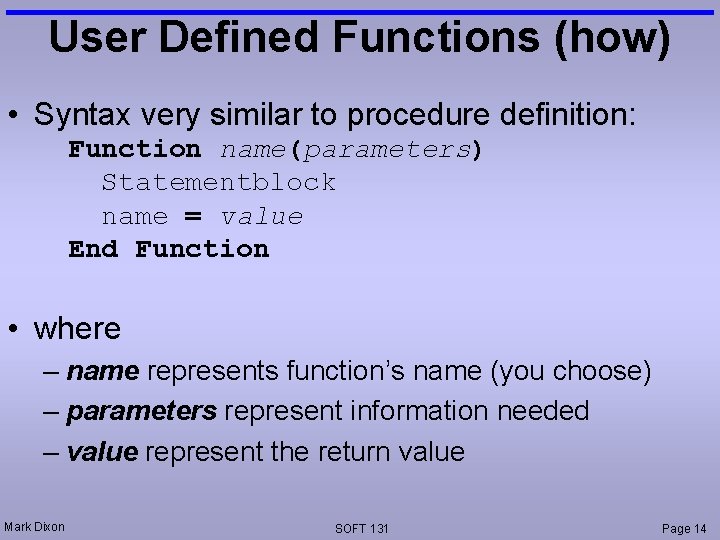
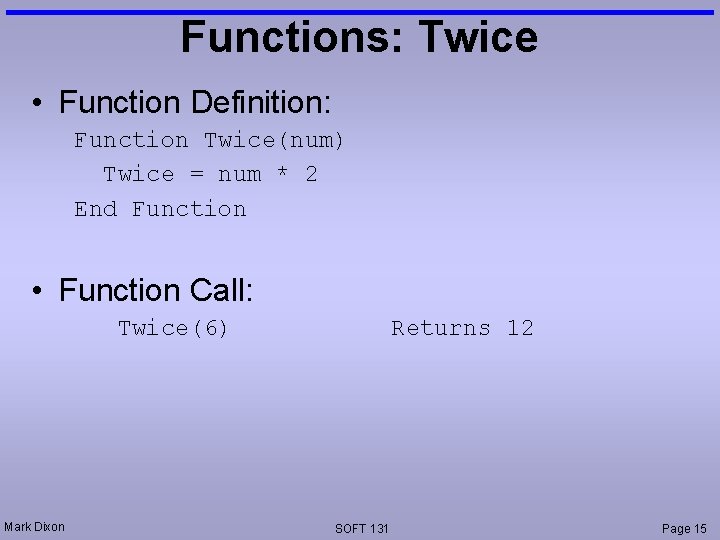
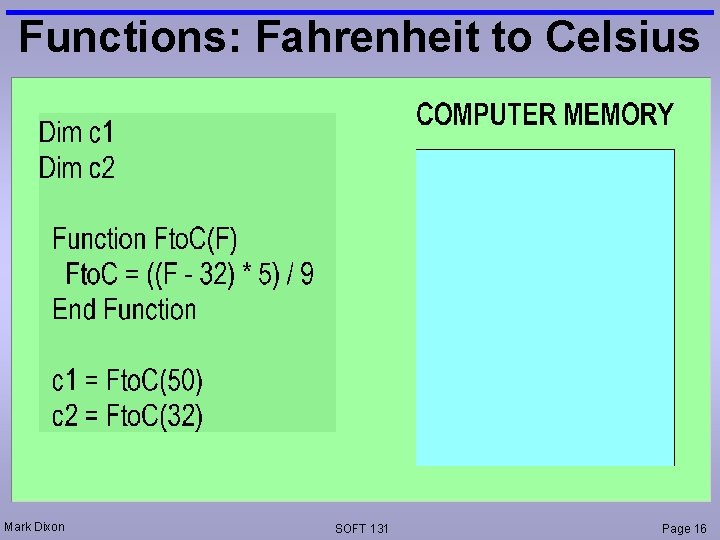
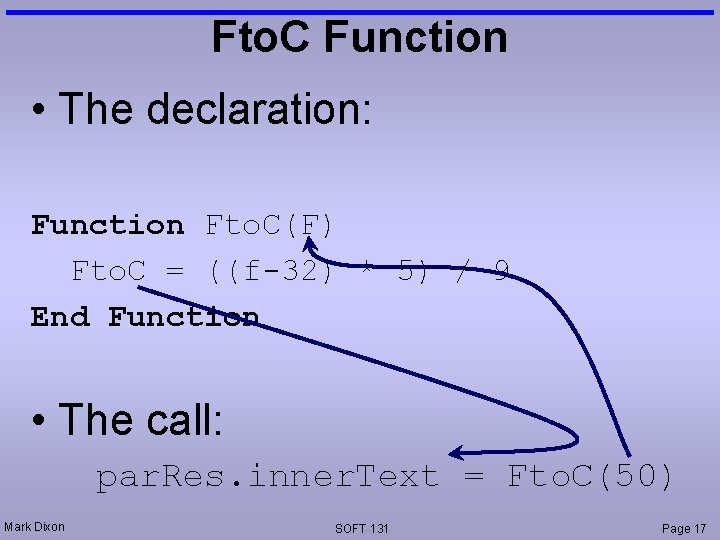
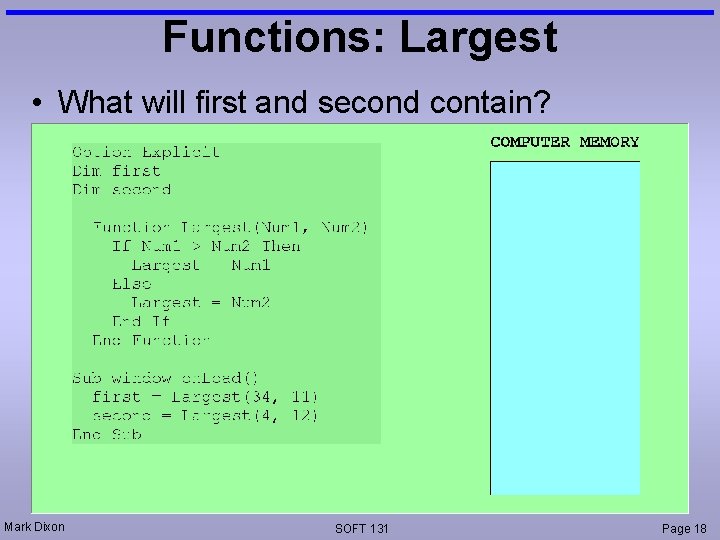
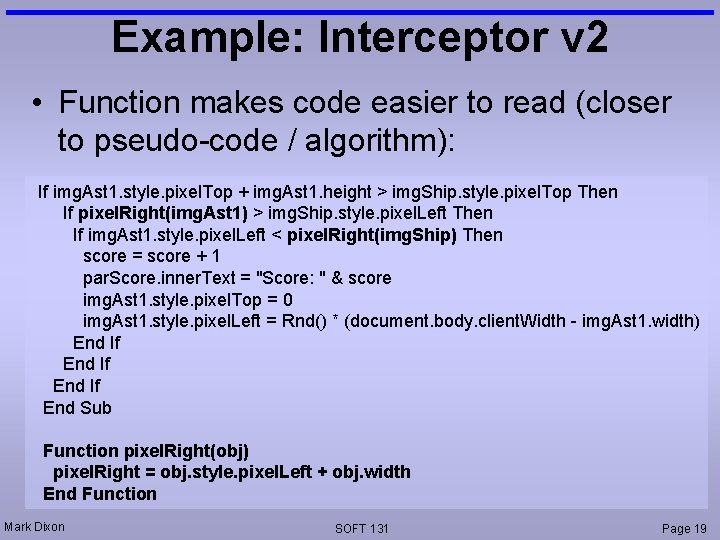
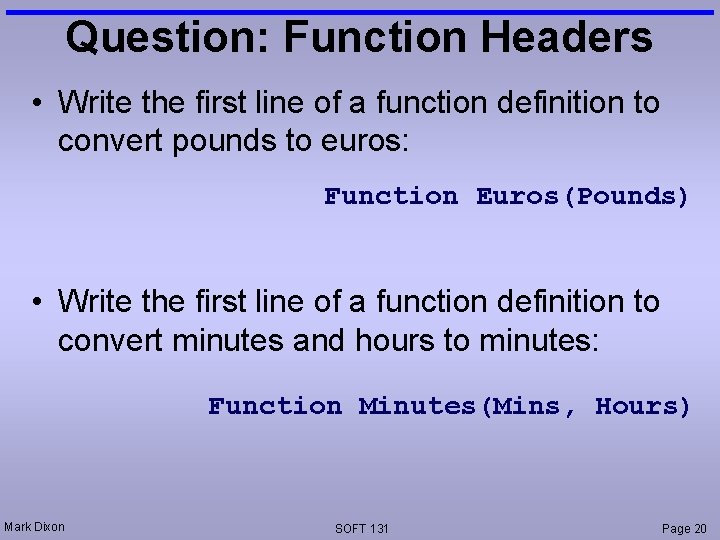
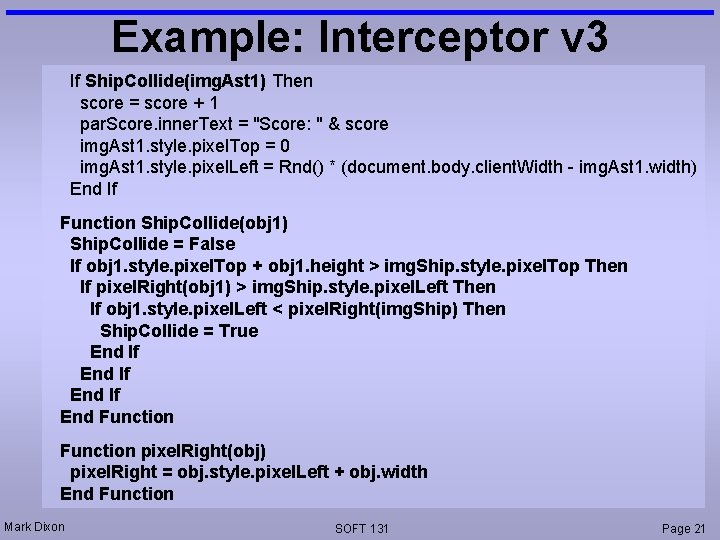
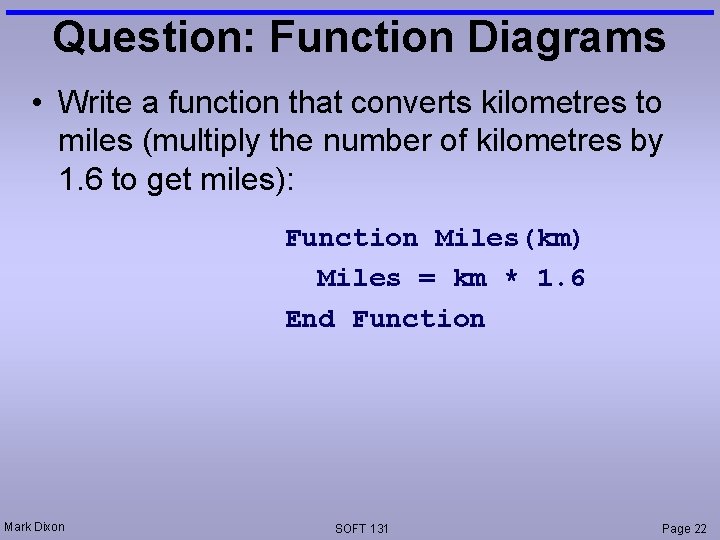
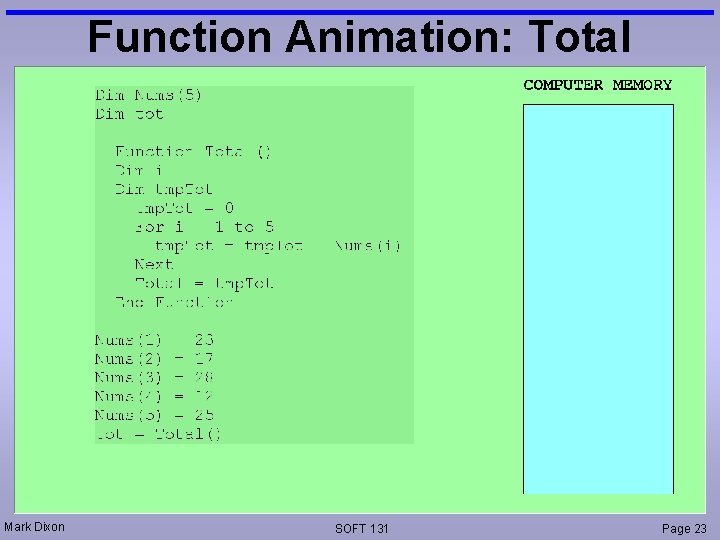
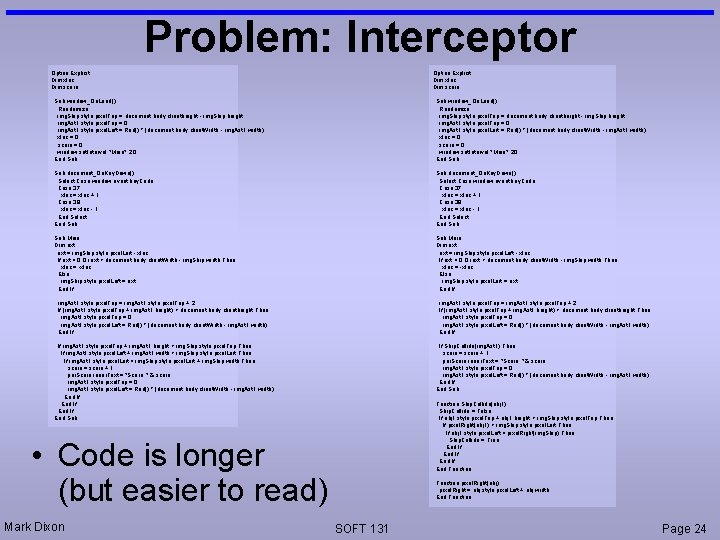
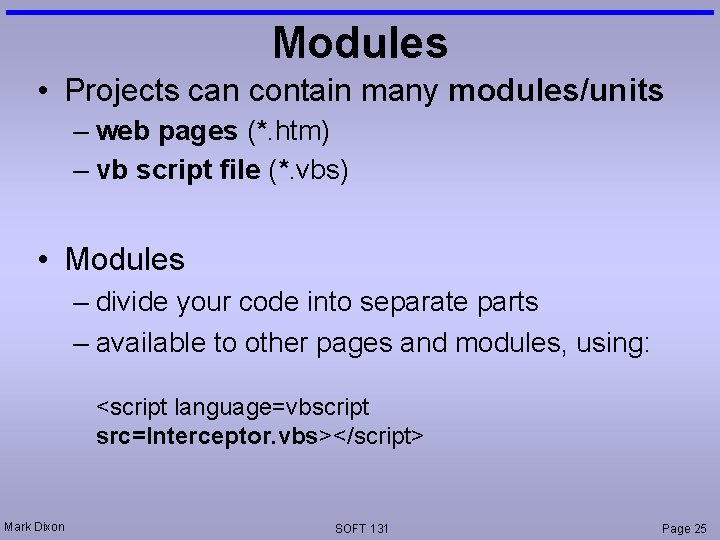
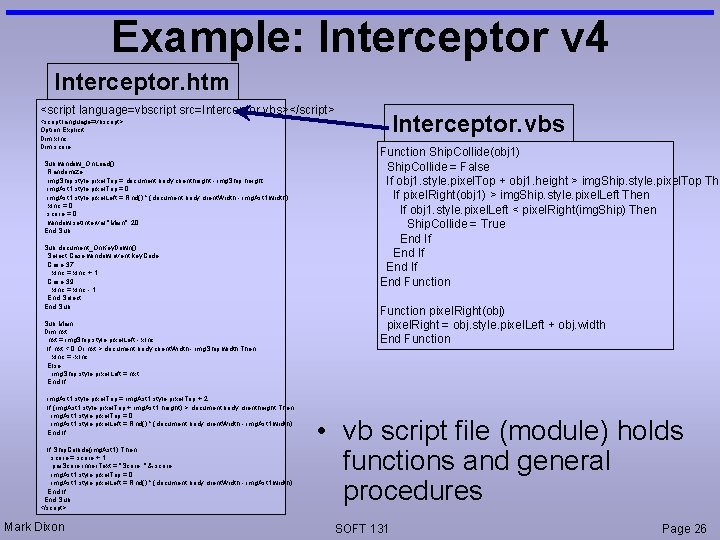
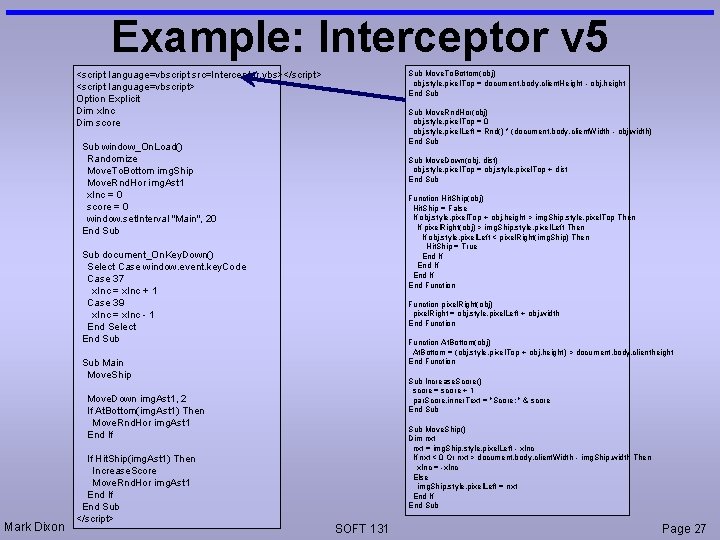
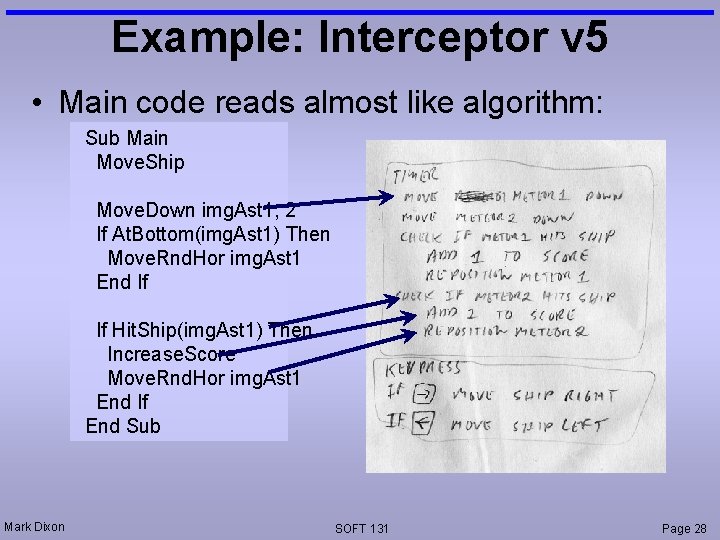
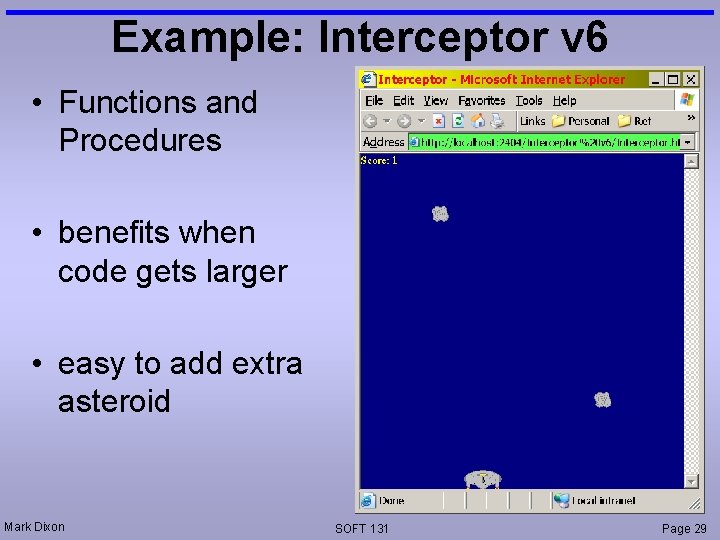
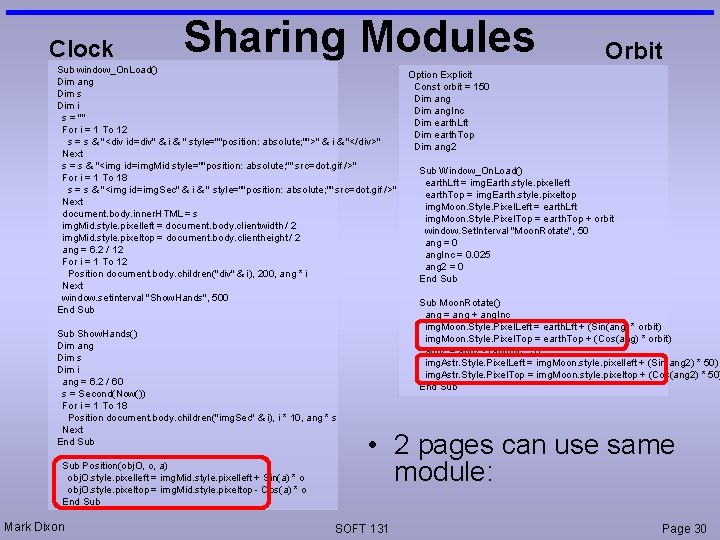
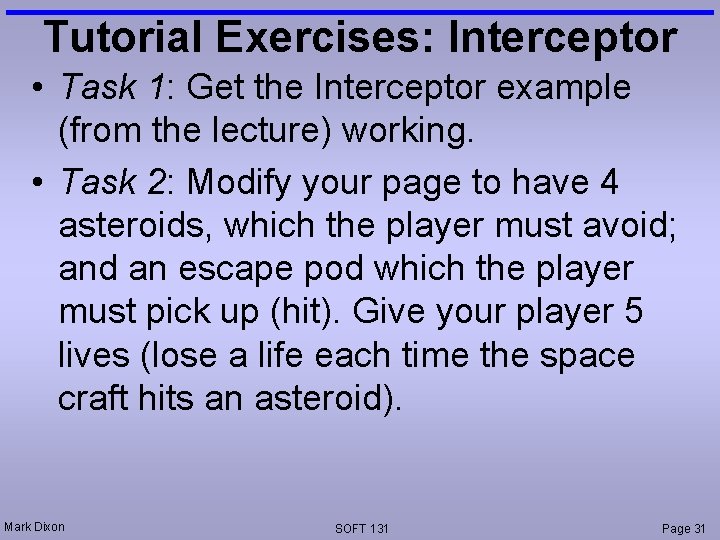
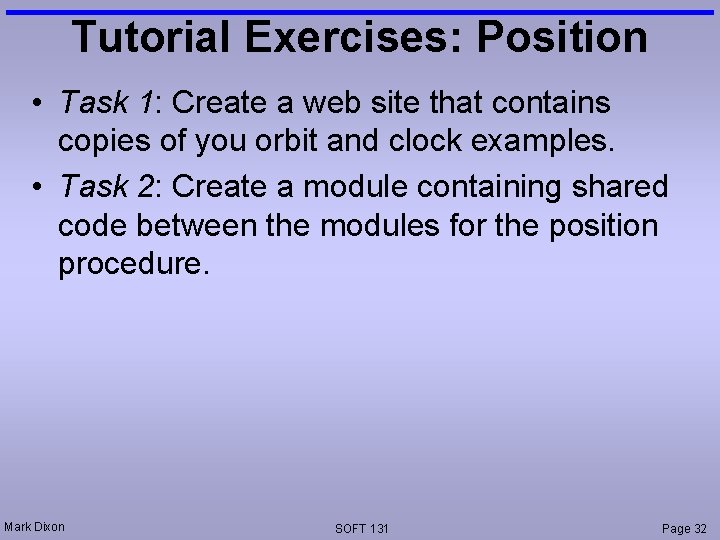
- Slides: 32
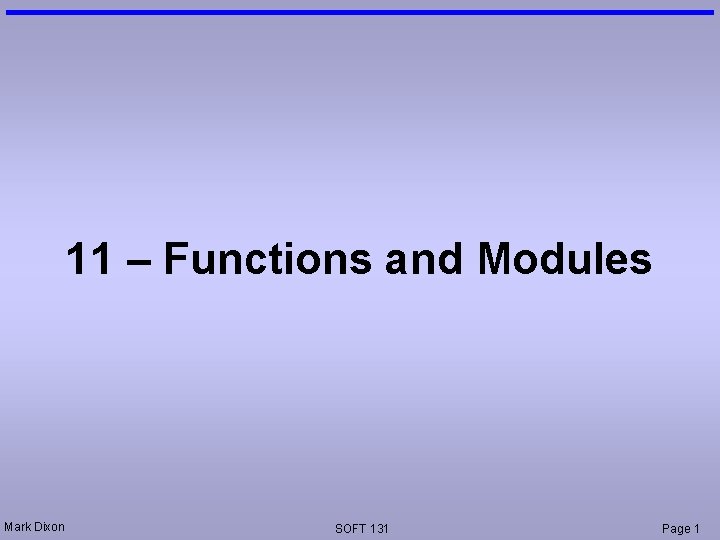
11 – Functions and Modules Mark Dixon SOFT 131 Page 1
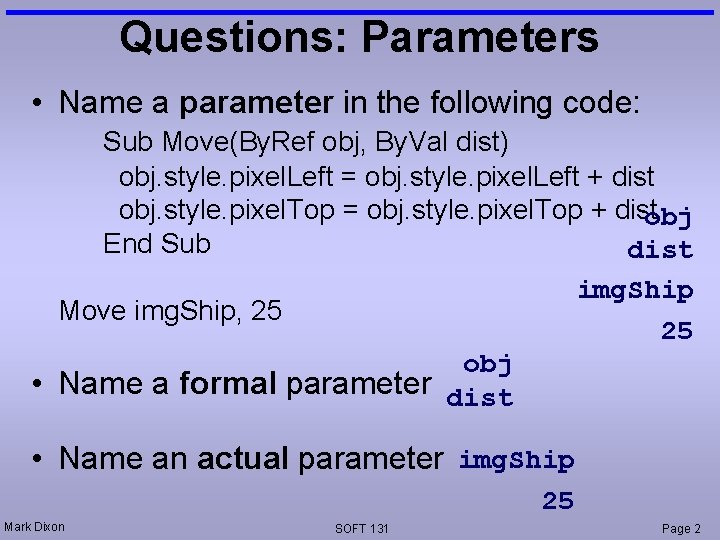
Questions: Parameters • Name a parameter in the following code: Sub Move(By. Ref obj, By. Val dist) obj. style. pixel. Left = obj. style. pixel. Left + dist obj. style. pixel. Top = obj. style. pixel. Top + dist obj End Sub dist img. Ship Move img. Ship, 25 25 obj • Name a formal parameter dist • Name an actual parameter img. Ship 25 Mark Dixon SOFT 131 Page 2
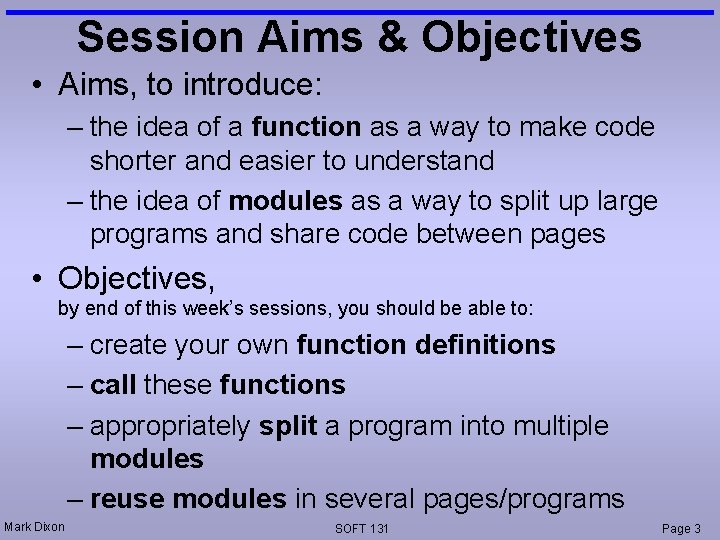
Session Aims & Objectives • Aims, to introduce: – the idea of a function as a way to make code shorter and easier to understand – the idea of modules as a way to split up large programs and share code between pages • Objectives, by end of this week’s sessions, you should be able to: – create your own function definitions – call these functions – appropriately split a program into multiple modules – reuse modules in several pages/programs Mark Dixon SOFT 131 Page 3
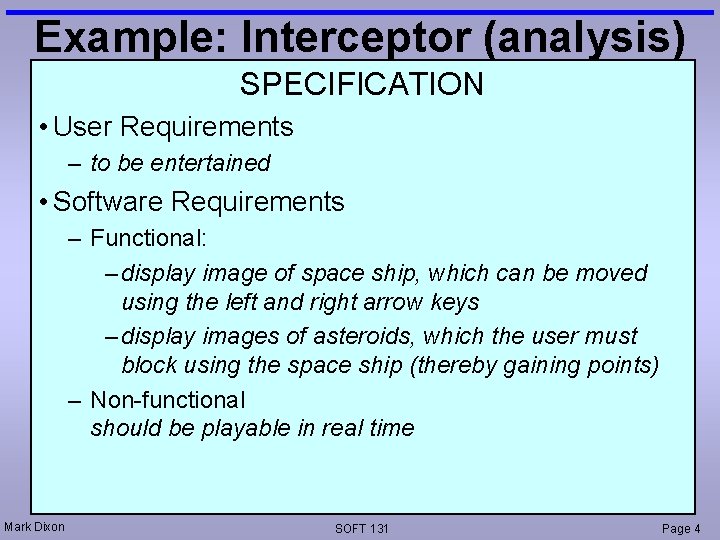
Example: Interceptor (analysis) SPECIFICATION • User Requirements – to be entertained • Software Requirements – Functional: – display image of space ship, which can be moved using the left and right arrow keys – display images of asteroids, which the user must block using the space ship (thereby gaining points) – Non-functional should be playable in real time Mark Dixon SOFT 131 Page 4
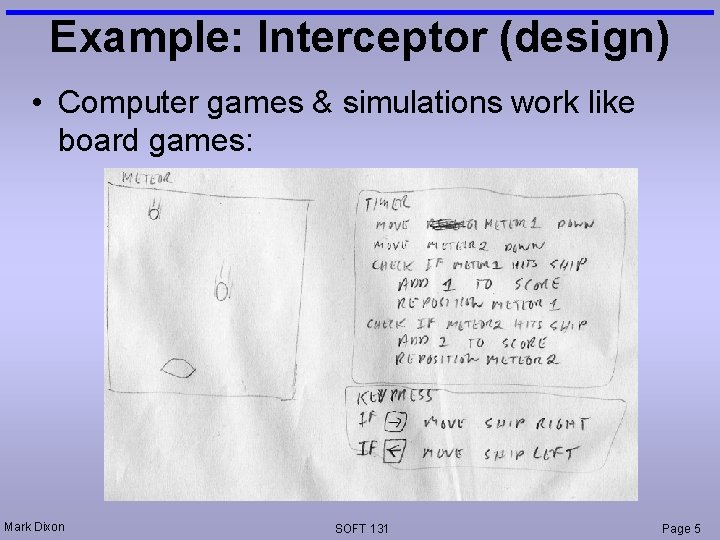
Example: Interceptor (design) • Computer games & simulations work like board games: Mark Dixon SOFT 131 Page 5
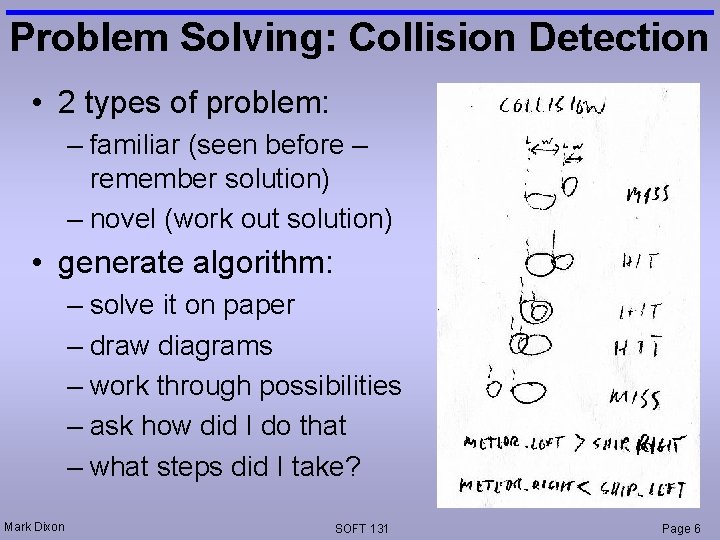
Problem Solving: Collision Detection • 2 types of problem: – familiar (seen before – remember solution) – novel (work out solution) • generate algorithm: – solve it on paper – draw diagrams – work through possibilities – ask how did I do that – what steps did I take? Mark Dixon SOFT 131 Page 6
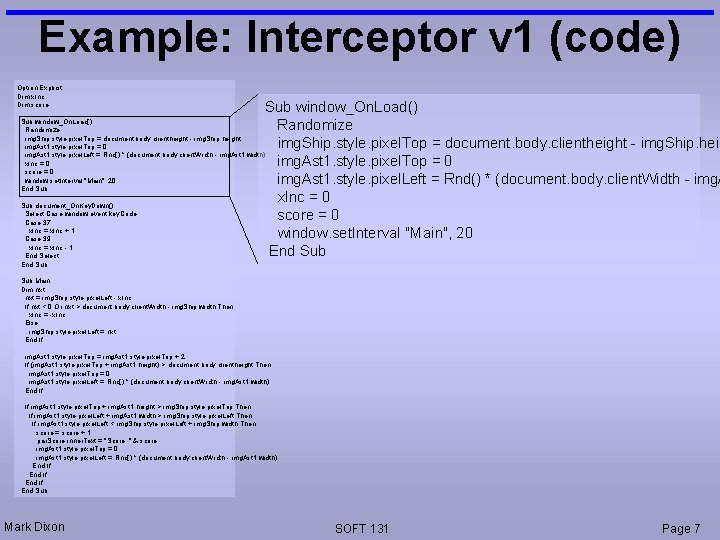
Example: Interceptor v 1 (code) Option Explicit Dim x. Inc Dim score Sub window_On. Load() Randomize img. Ship. style. pixel. Top = document. body. clientheight - img. Ship. heig img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. A x. Inc = 0 score = 0 window. set. Interval "Main", 20 End Sub window_On. Load() Randomize img. Ship. style. pixel. Top = document. body. clientheight - img. Ship. height img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) x. Inc = 0 score = 0 window. set. Interval "Main", 20 End Sub document_On. Key. Down() Select Case window. event. key. Code Case 37 x. Inc = x. Inc + 1 Case 39 x. Inc = x. Inc - 1 End Select End Sub Main Dim nxt = img. Ship. style. pixel. Left - x. Inc If nxt < 0 Or nxt > document. body. client. Width - img. Ship. width Then x. Inc = -x. Inc Else img. Ship. style. pixel. Left = nxt End If img. Ast 1. style. pixel. Top = img. Ast 1. style. pixel. Top + 2 If (img. Ast 1. style. pixel. Top + img. Ast 1. height) > document. body. clientheight Then img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If If img. Ast 1. style. pixel. Top + img. Ast 1. height > img. Ship. style. pixel. Top Then If img. Ast 1. style. pixel. Left + img. Ast 1. width > img. Ship. style. pixel. Left Then If img. Ast 1. style. pixel. Left < img. Ship. style. pixel. Left + img. Ship. width Then score = score + 1 par. Score. inner. Text = "Score: " & score img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If End Sub Mark Dixon SOFT 131 Page 7
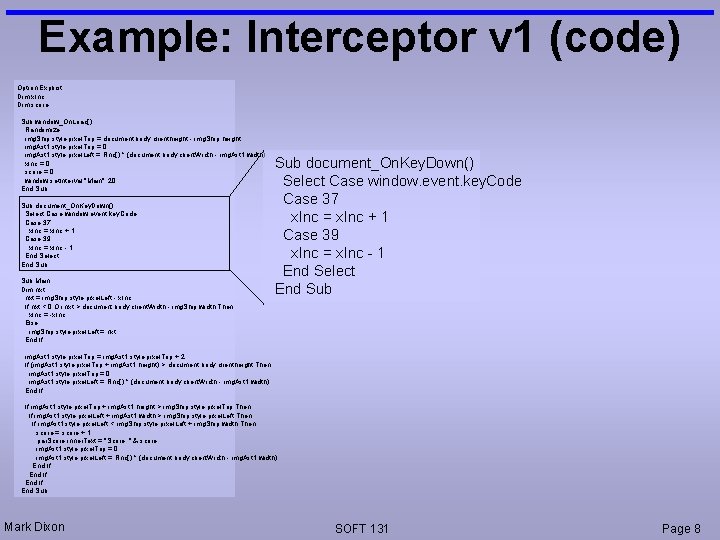
Example: Interceptor v 1 (code) Option Explicit Dim x. Inc Dim score Sub window_On. Load() Randomize img. Ship. style. pixel. Top = document. body. clientheight - img. Ship. height img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) x. Inc = 0 score = 0 window. set. Interval "Main", 20 End Sub document_On. Key. Down() Select Case window. event. key. Code Case 37 x. Inc = x. Inc + 1 Case 39 x. Inc = x. Inc - 1 End Select End Sub Main Dim nxt = img. Ship. style. pixel. Left - x. Inc If nxt < 0 Or nxt > document. body. client. Width - img. Ship. width Then x. Inc = -x. Inc Else img. Ship. style. pixel. Left = nxt End If Sub document_On. Key. Down() Select Case window. event. key. Code Case 37 x. Inc = x. Inc + 1 Case 39 x. Inc = x. Inc - 1 End Select End Sub img. Ast 1. style. pixel. Top = img. Ast 1. style. pixel. Top + 2 If (img. Ast 1. style. pixel. Top + img. Ast 1. height) > document. body. clientheight Then img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If If img. Ast 1. style. pixel. Top + img. Ast 1. height > img. Ship. style. pixel. Top Then If img. Ast 1. style. pixel. Left + img. Ast 1. width > img. Ship. style. pixel. Left Then If img. Ast 1. style. pixel. Left < img. Ship. style. pixel. Left + img. Ship. width Then score = score + 1 par. Score. inner. Text = "Score: " & score img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If End Sub Mark Dixon SOFT 131 Page 8
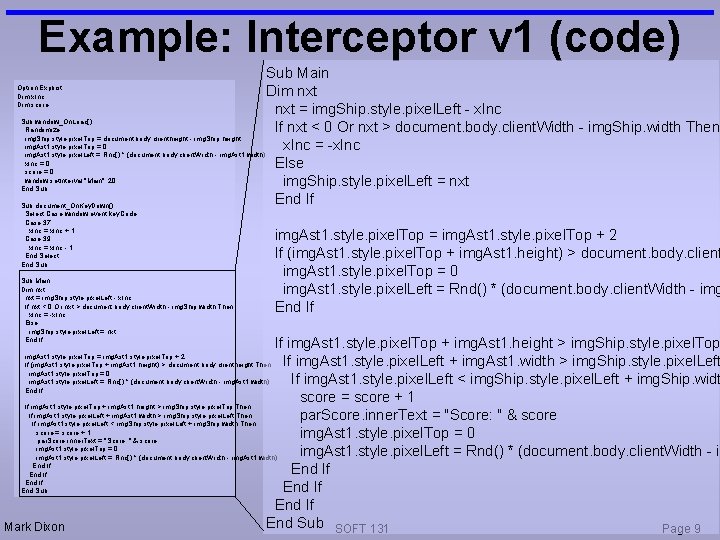
Example: Interceptor v 1 (code) Sub Main Dim nxt = img. Ship. style. pixel. Left - x. Inc Sub window_On. Load() If nxt < 0 Or nxt > document. body. client. Width - img. Ship. width Then Randomize img. Ship. style. pixel. Top = document. body. clientheight - img. Ship. height img. Ast 1. style. pixel. Top = 0 x. Inc = -x. Inc img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) x. Inc = 0 Else score = 0 window. set. Interval "Main", 20 img. Ship. style. pixel. Left = nxt End Sub End If Sub document_On. Key. Down() Option Explicit Dim x. Inc Dim score Select Case window. event. key. Code Case 37 x. Inc = x. Inc + 1 Case 39 x. Inc = x. Inc - 1 End Select End Sub Main Dim nxt = img. Ship. style. pixel. Left - x. Inc If nxt < 0 Or nxt > document. body. client. Width - img. Ship. width Then x. Inc = -x. Inc Else img. Ship. style. pixel. Left = nxt End If img. Ast 1. style. pixel. Top = img. Ast 1. style. pixel. Top + 2 If (img. Ast 1. style. pixel. Top + img. Ast 1. height) > document. body. client img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img End If If img. Ast 1. style. pixel. Top + img. Ast 1. height > img. Ship. style. pixel. Top If img. Ast 1. style. pixel. Left + img. Ast 1. width > img. Ship. style. pixel. Left If img. Ast 1. style. pixel. Left < img. Ship. style. pixel. Left + img. Ship. widt score = score + 1 If img. Ast 1. style. pixel. Top + img. Ast 1. height > img. Ship. style. pixel. Top Then If img. Ast 1. style. pixel. Left + img. Ast 1. width > img. Ship. style. pixel. Left Then par. Score. inner. Text = "Score: " & score If img. Ast 1. style. pixel. Left < img. Ship. style. pixel. Left + img. Ship. width Then score = score + 1 img. Ast 1. style. pixel. Top = 0 par. Score. inner. Text = "Score: " & score img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If End If End Sub SOFT 131 Mark Dixon Page 9 img. Ast 1. style. pixel. Top = img. Ast 1. style. pixel. Top + 2 If (img. Ast 1. style. pixel. Top + img. Ast 1. height) > document. body. clientheight Then img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If
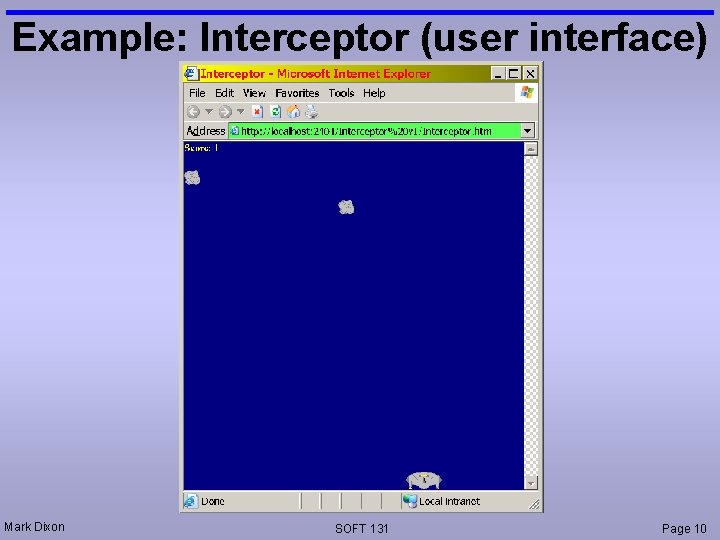
Example: Interceptor (user interface) Mark Dixon SOFT 131 Page 10
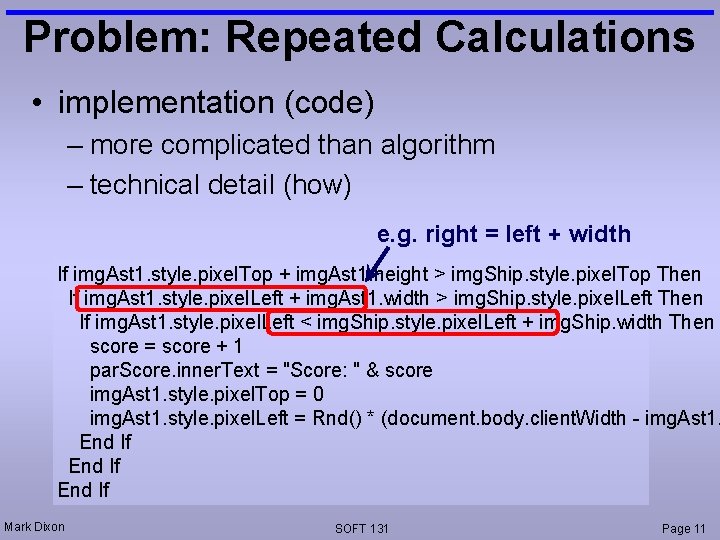
Problem: Repeated Calculations • implementation (code) – more complicated than algorithm – technical detail (how) e. g. right = left + width If img. Ast 1. style. pixel. Top + img. Ast 1. height > img. Ship. style. pixel. Top Then If img. Ast 1. style. pixel. Left + img. Ast 1. width > img. Ship. style. pixel. Left Then If img. Ast 1. style. pixel. Left < img. Ship. style. pixel. Left + img. Ship. width Then score = score + 1 par. Score. inner. Text = "Score: " & score img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. End If Mark Dixon SOFT 131 Page 11
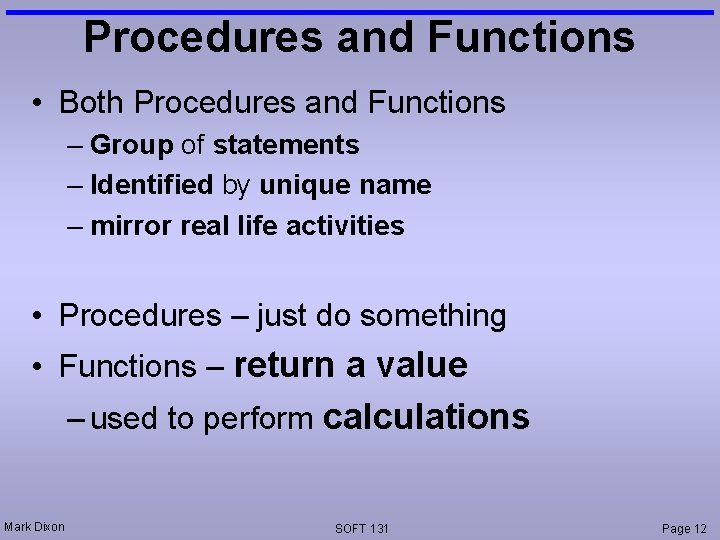
Procedures and Functions • Both Procedures and Functions – Group of statements – Identified by unique name – mirror real life activities • Procedures – just do something • Functions – return a value – used to perform calculations Mark Dixon SOFT 131 Page 12
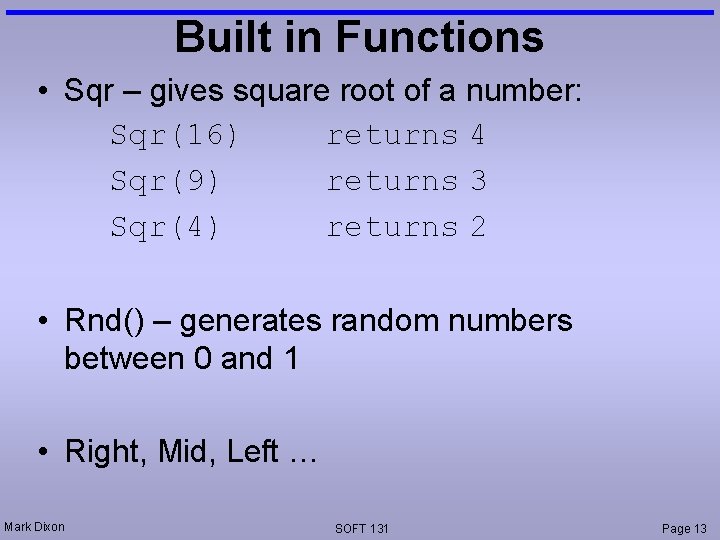
Built in Functions • Sqr – gives square root of a number: Sqr(16) returns 4 Sqr(9) returns 3 Sqr(4) returns 2 • Rnd() – generates random numbers between 0 and 1 • Right, Mid, Left … Mark Dixon SOFT 131 Page 13
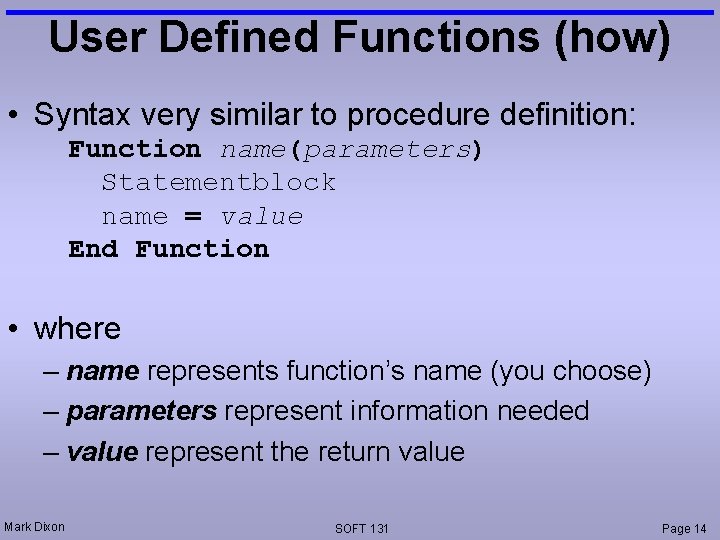
User Defined Functions (how) • Syntax very similar to procedure definition: Function name(parameters) Statementblock name = value End Function • where – name represents function’s name (you choose) – parameters represent information needed – value represent the return value Mark Dixon SOFT 131 Page 14
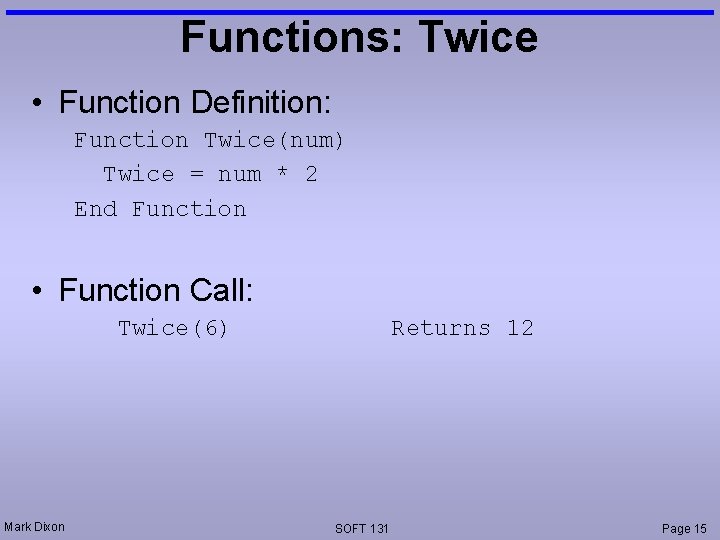
Functions: Twice • Function Definition: Function Twice(num) Twice = num * 2 End Function • Function Call: Twice(6) Mark Dixon Returns 12 SOFT 131 Page 15
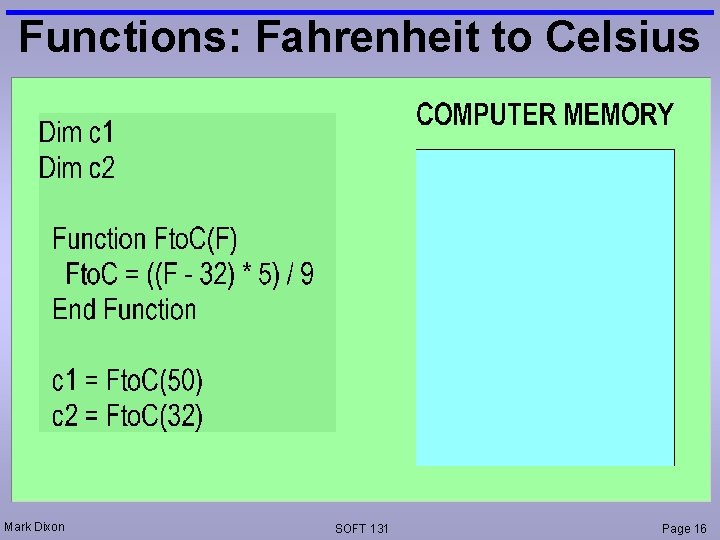
Functions: Fahrenheit to Celsius Mark Dixon SOFT 131 Page 16
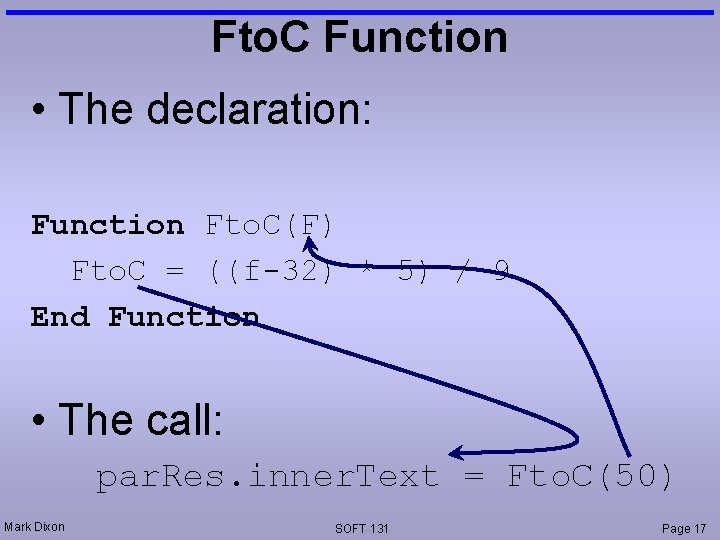
Fto. C Function • The declaration: Function Fto. C(F) Fto. C = ((f-32) * 5) / 9 End Function • The call: par. Res. inner. Text = Fto. C(50) Mark Dixon SOFT 131 Page 17
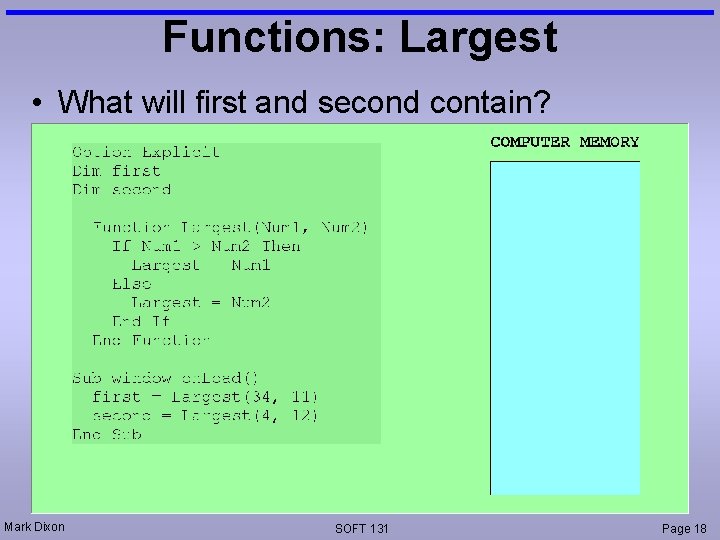
Functions: Largest • What will first and second contain? Mark Dixon SOFT 131 Page 18
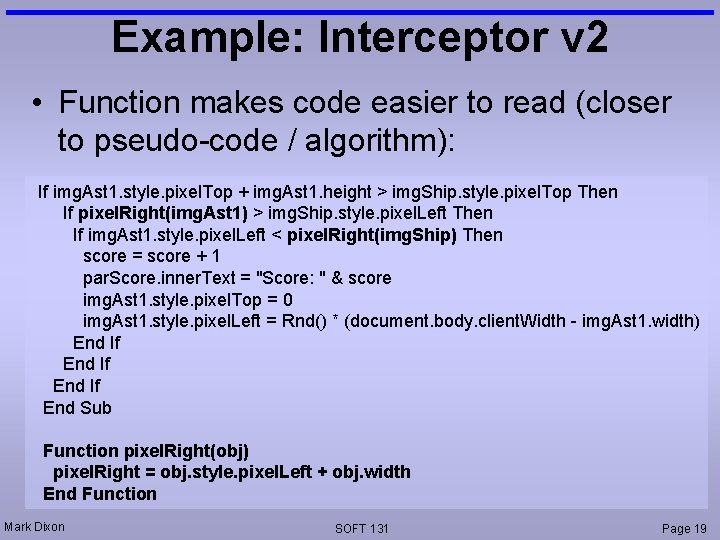
Example: Interceptor v 2 • Function makes code easier to read (closer to pseudo-code / algorithm): If img. Ast 1. style. pixel. Top + img. Ast 1. height > img. Ship. style. pixel. Top Then If pixel. Right(img. Ast 1) > img. Ship. style. pixel. Left Then If img. Ast 1. style. pixel. Left < pixel. Right(img. Ship) Then score = score + 1 par. Score. inner. Text = "Score: " & score img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If End Sub Function pixel. Right(obj) pixel. Right = obj. style. pixel. Left + obj. width End Function Mark Dixon SOFT 131 Page 19
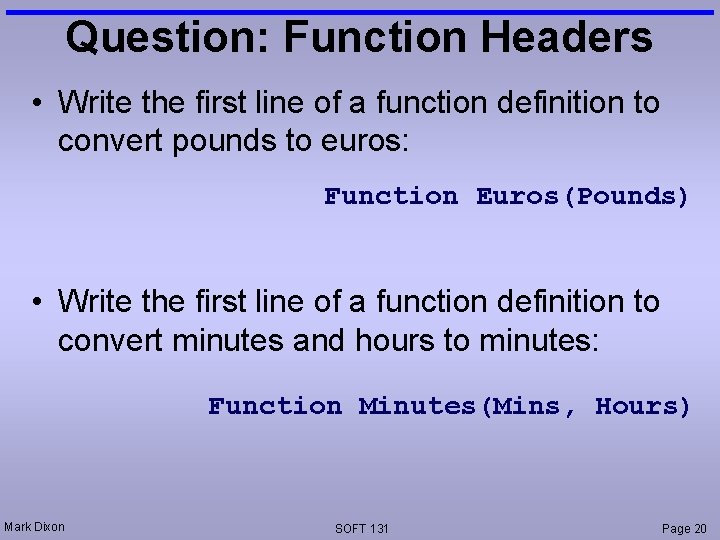
Question: Function Headers • Write the first line of a function definition to convert pounds to euros: Function Euros(Pounds) • Write the first line of a function definition to convert minutes and hours to minutes: Function Minutes(Mins, Hours) Mark Dixon SOFT 131 Page 20
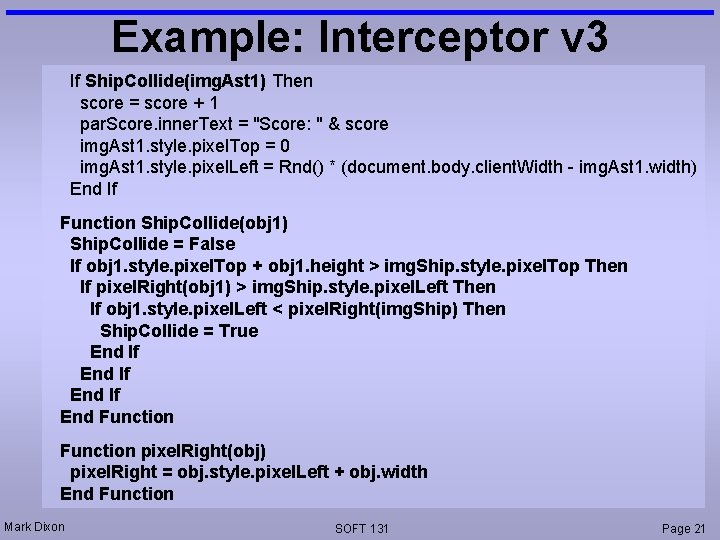
Example: Interceptor v 3 If Ship. Collide(img. Ast 1) Then score = score + 1 par. Score. inner. Text = "Score: " & score img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If Function Ship. Collide(obj 1) Ship. Collide = False If obj 1. style. pixel. Top + obj 1. height > img. Ship. style. pixel. Top Then If pixel. Right(obj 1) > img. Ship. style. pixel. Left Then If obj 1. style. pixel. Left < pixel. Right(img. Ship) Then Ship. Collide = True End If End Function pixel. Right(obj) pixel. Right = obj. style. pixel. Left + obj. width End Function Mark Dixon SOFT 131 Page 21
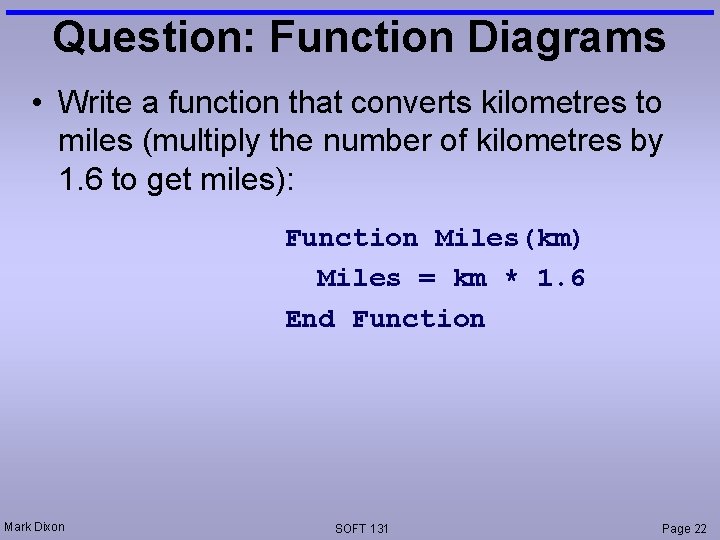
Question: Function Diagrams • Write a function that converts kilometres to miles (multiply the number of kilometres by 1. 6 to get miles): Function Miles(km) Miles = km * 1. 6 End Function Mark Dixon SOFT 131 Page 22
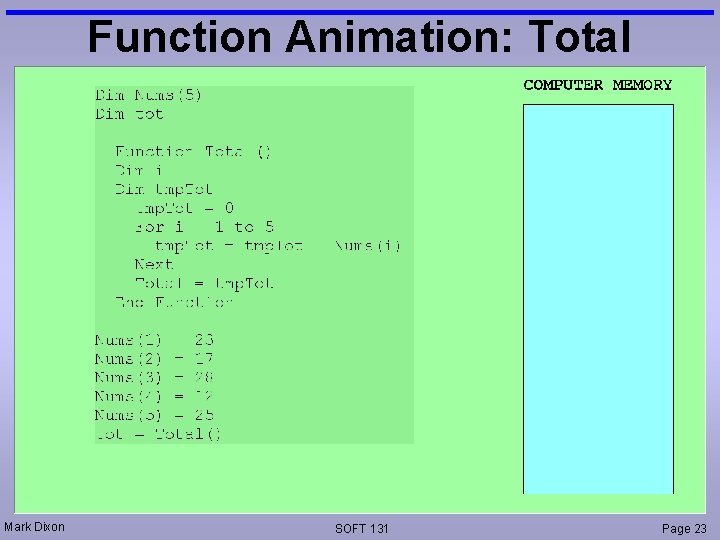
Function Animation: Total Mark Dixon SOFT 131 Page 23
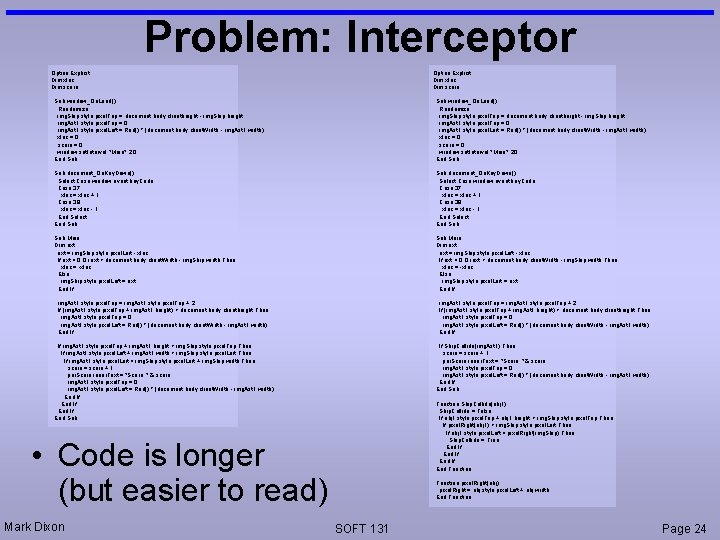
Problem: Interceptor Option Explicit Dim x. Inc Dim score Sub window_On. Load() Randomize img. Ship. style. pixel. Top = document. body. clientheight - img. Ship. height img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) x. Inc = 0 score = 0 window. set. Interval "Main", 20 End Sub Sub document_On. Key. Down() Select Case window. event. key. Code Case 37 x. Inc = x. Inc + 1 Case 39 x. Inc = x. Inc - 1 End Select End Sub Sub Main Dim nxt nxt = img. Ship. style. pixel. Left - x. Inc If nxt < 0 Or nxt > document. body. client. Width - img. Ship. width Then x. Inc = -x. Inc Else img. Ship. style. pixel. Left = nxt End If img. Ast 1. style. pixel. Top = img. Ast 1. style. pixel. Top + 2 If (img. Ast 1. style. pixel. Top + img. Ast 1. height) > document. body. clientheight Then img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If If img. Ast 1. style. pixel. Top + img. Ast 1. height > img. Ship. style. pixel. Top Then If img. Ast 1. style. pixel. Left + img. Ast 1. width > img. Ship. style. pixel. Left Then If img. Ast 1. style. pixel. Left < img. Ship. style. pixel. Left + img. Ship. width Then score = score + 1 par. Score. inner. Text = "Score: " & score img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If End Sub If Ship. Collide(img. Ast 1) Then score = score + 1 par. Score. inner. Text = "Score: " & score img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If End Sub Function Ship. Collide(obj 1) Ship. Collide = False If obj 1. style. pixel. Top + obj 1. height > img. Ship. style. pixel. Top Then If pixel. Right(obj 1) > img. Ship. style. pixel. Left Then If obj 1. style. pixel. Left < pixel. Right(img. Ship) Then Ship. Collide = True End If End Function • Code is longer (but easier to read) Mark Dixon Function pixel. Right(obj) pixel. Right = obj. style. pixel. Left + obj. width End Function SOFT 131 Page 24
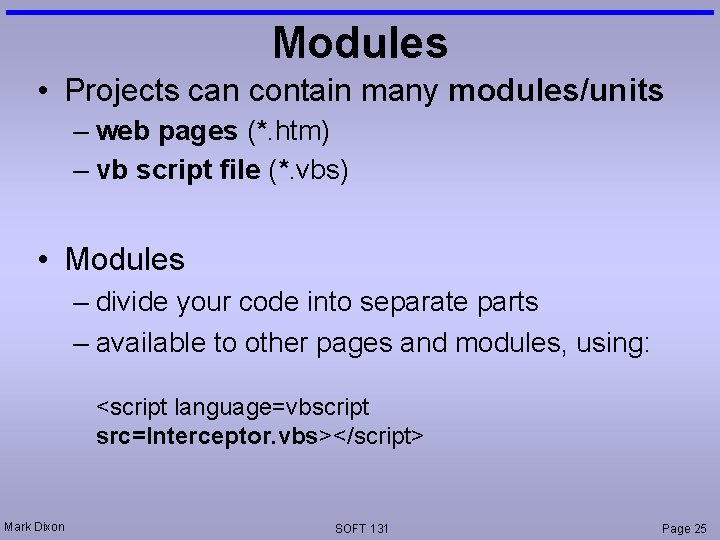
Modules • Projects can contain many modules/units – web pages (*. htm) – vb script file (*. vbs) • Modules – divide your code into separate parts – available to other pages and modules, using: <script language=vbscript src=Interceptor. vbs></script> Mark Dixon SOFT 131 Page 25
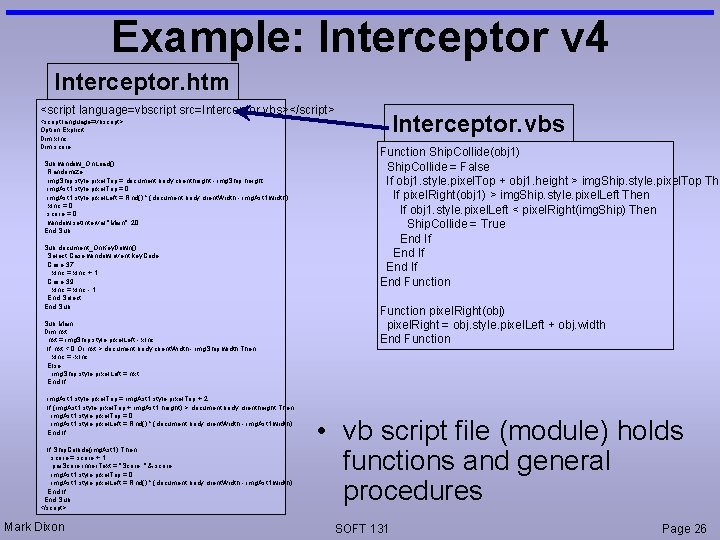
Example: Interceptor v 4 Interceptor. htm <script language=vbscript src=Interceptor. vbs></script> <script language=vbscript> Option Explicit Dim x. Inc Dim score Sub window_On. Load() Randomize img. Ship. style. pixel. Top = document. body. clientheight - img. Ship. height img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) x. Inc = 0 score = 0 window. set. Interval "Main", 20 End Sub document_On. Key. Down() Select Case window. event. key. Code Case 37 x. Inc = x. Inc + 1 Case 39 x. Inc = x. Inc - 1 End Select End Sub Main Dim nxt = img. Ship. style. pixel. Left - x. Inc If nxt < 0 Or nxt > document. body. client. Width - img. Ship. width Then x. Inc = -x. Inc Else img. Ship. style. pixel. Left = nxt End If img. Ast 1. style. pixel. Top = img. Ast 1. style. pixel. Top + 2 If (img. Ast 1. style. pixel. Top + img. Ast 1. height) > document. body. clientheight Then img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If If Ship. Collide(img. Ast 1) Then score = score + 1 par. Score. inner. Text = "Score: " & score img. Ast 1. style. pixel. Top = 0 img. Ast 1. style. pixel. Left = Rnd() * (document. body. client. Width - img. Ast 1. width) End If End Sub </script> Mark Dixon Interceptor. vbs Function Ship. Collide(obj 1) Ship. Collide = False If obj 1. style. pixel. Top + obj 1. height > img. Ship. style. pixel. Top The If pixel. Right(obj 1) > img. Ship. style. pixel. Left Then If obj 1. style. pixel. Left < pixel. Right(img. Ship) Then Ship. Collide = True End If End Function pixel. Right(obj) pixel. Right = obj. style. pixel. Left + obj. width End Function • vb script file (module) holds functions and general procedures SOFT 131 Page 26
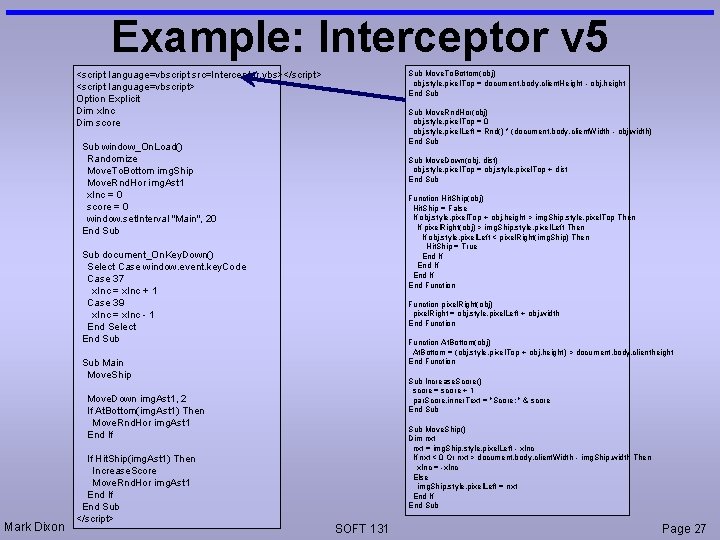
Example: Interceptor v 5 Sub Move. To. Bottom(obj) obj. style. pixel. Top = document. body. client. Height - obj. height End Sub <script language=vbscript src=Interceptor. vbs></script> <script language=vbscript> Option Explicit Dim x. Inc Dim score Sub Move. Rnd. Hor(obj) obj. style. pixel. Top = 0 obj. style. pixel. Left = Rnd() * (document. body. client. Width - obj. width) End Sub window_On. Load() Randomize Move. To. Bottom img. Ship Move. Rnd. Hor img. Ast 1 x. Inc = 0 score = 0 window. set. Interval "Main", 20 End Sub Move. Down(obj, dist) obj. style. pixel. Top = obj. style. pixel. Top + dist End Sub Function Hit. Ship(obj) Hit. Ship = False If obj. style. pixel. Top + obj. height > img. Ship. style. pixel. Top Then If pixel. Right(obj) > img. Ship. style. pixel. Left Then If obj. style. pixel. Left < pixel. Right(img. Ship) Then Hit. Ship = True End If End Function Sub document_On. Key. Down() Select Case window. event. key. Code Case 37 x. Inc = x. Inc + 1 Case 39 x. Inc = x. Inc - 1 End Select End Sub Function pixel. Right(obj) pixel. Right = obj. style. pixel. Left + obj. width End Function At. Bottom(obj) At. Bottom = (obj. style. pixel. Top + obj. height) > document. body. clientheight End Function Sub Main Move. Ship Sub Increase. Score() score = score + 1 par. Score. inner. Text = "Score: " & score End Sub Move. Down img. Ast 1, 2 If At. Bottom(img. Ast 1) Then Move. Rnd. Hor img. Ast 1 End If Mark Dixon If Hit. Ship(img. Ast 1) Then Increase. Score Move. Rnd. Hor img. Ast 1 End If End Sub </script> Sub Move. Ship() Dim nxt = img. Ship. style. pixel. Left - x. Inc If nxt < 0 Or nxt > document. body. client. Width - img. Ship. width Then x. Inc = -x. Inc Else img. Ship. style. pixel. Left = nxt End If End Sub SOFT 131 Page 27
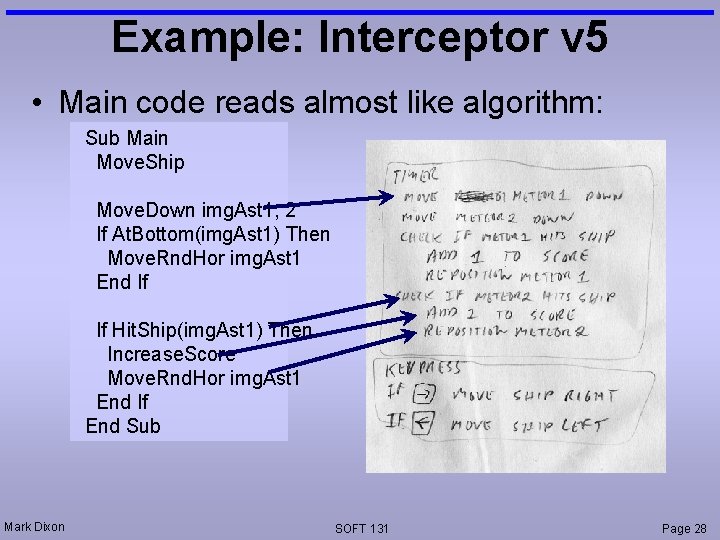
Example: Interceptor v 5 • Main code reads almost like algorithm: Sub Main Move. Ship Move. Down img. Ast 1, 2 If At. Bottom(img. Ast 1) Then Move. Rnd. Hor img. Ast 1 End If If Hit. Ship(img. Ast 1) Then Increase. Score Move. Rnd. Hor img. Ast 1 End If End Sub Mark Dixon SOFT 131 Page 28
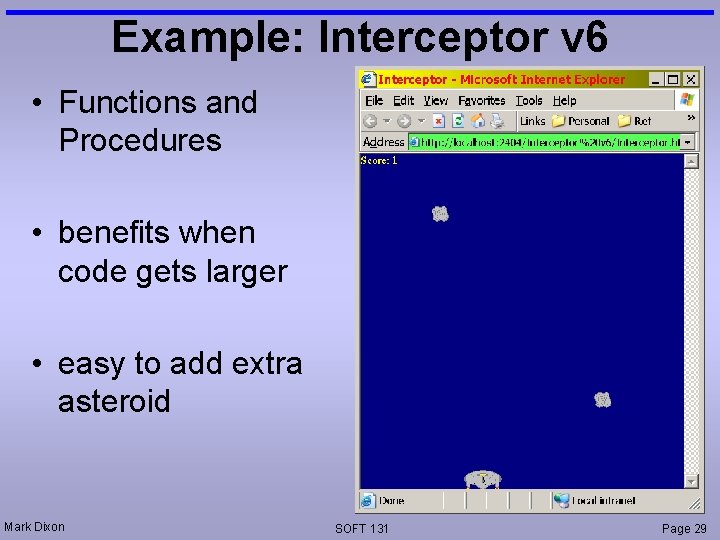
Example: Interceptor v 6 • Functions and Procedures • benefits when code gets larger • easy to add extra asteroid Mark Dixon SOFT 131 Page 29
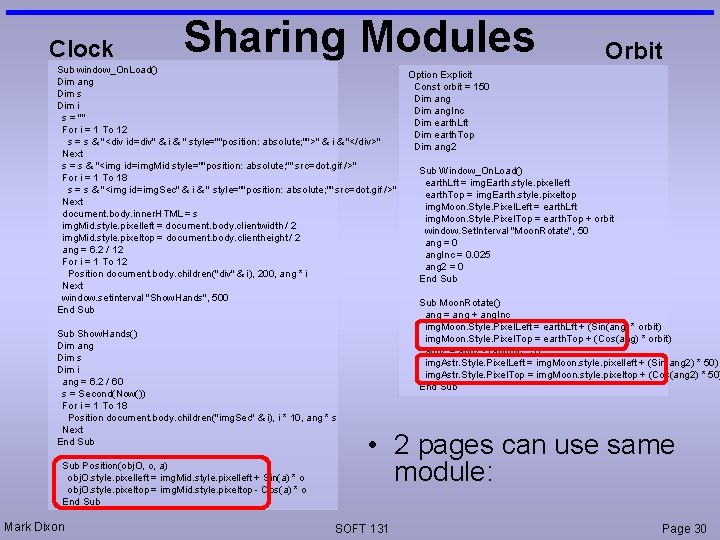
Clock Sharing Modules Sub window_On. Load() Dim ang Dim s Dim i s = "" For i = 1 To 12 s = s & "<div id=div" & i & " style=""position: absolute; "">" & i & "</div>" Next s = s & "<img id=img. Mid style=""position: absolute; "" src=dot. gif />" For i = 1 To 18 s = s & "<img id=img. Sec" & i & " style=""position: absolute; "" src=dot. gif />" Next document. body. inner. HTML = s img. Mid. style. pixelleft = document. body. clientwidth / 2 img. Mid. style. pixeltop = document. body. clientheight / 2 ang = 6. 2 / 12 For i = 1 To 12 Position document. body. children("div" & i), 200, ang * i Next window. setinterval "Show. Hands", 500 End Sub Show. Hands() Dim ang Dim s Dim i ang = 6. 2 / 60 s = Second(Now()) For i = 1 To 18 Position document. body. children("img. Sec" & i), i * 10, ang * s Next End Sub Position(obj. O, o, a) obj. O. style. pixelleft = img. Mid. style. pixelleft + Sin(a) * o obj. O. style. pixeltop = img. Mid. style. pixeltop - Cos(a) * o End Sub Mark Dixon Orbit Option Explicit Const orbit = 150 Dim ang. Inc Dim earth. Lft Dim earth. Top Dim ang 2 Sub Window_On. Load() earth. Lft = img. Earth. style. pixelleft earth. Top = img. Earth. style. pixeltop img. Moon. Style. Pixel. Left = earth. Lft img. Moon. Style. Pixel. Top = earth. Top + orbit window. Set. Interval "Moon. Rotate", 50 ang = 0 ang. Inc = 0. 025 ang 2 = 0 End Sub Moon. Rotate() ang = ang + ang. Inc img. Moon. Style. Pixel. Left = earth. Lft + (Sin(ang) * orbit) img. Moon. Style. Pixel. Top = earth. Top + (Cos(ang) * orbit) ang 2 = ang 2 - (ang. Inc * 3) img. Astr. Style. Pixel. Left = img. Moon. style. pixelleft + (Sin(ang 2) * 50) img. Astr. Style. Pixel. Top = img. Moon. style. pixeltop + (Cos(ang 2) * 50) End Sub • 2 pages can use same module: SOFT 131 Page 30
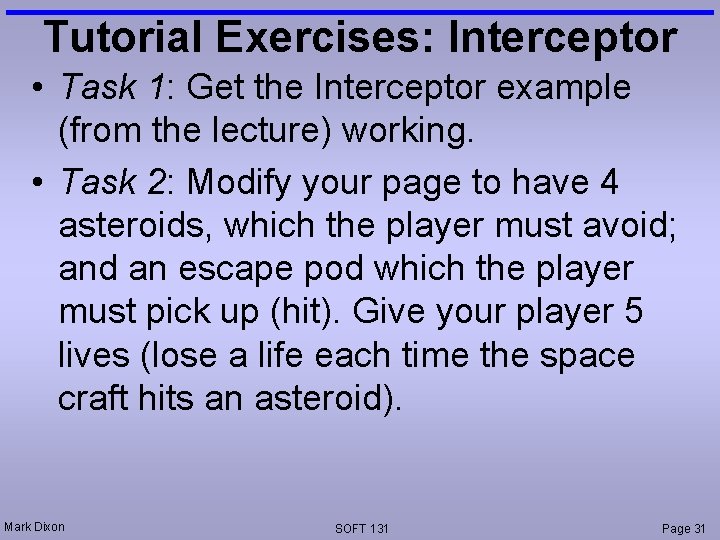
Tutorial Exercises: Interceptor • Task 1: Get the Interceptor example (from the lecture) working. • Task 2: Modify your page to have 4 asteroids, which the player must avoid; and an escape pod which the player must pick up (hit). Give your player 5 lives (lose a life each time the space craft hits an asteroid). Mark Dixon SOFT 131 Page 31
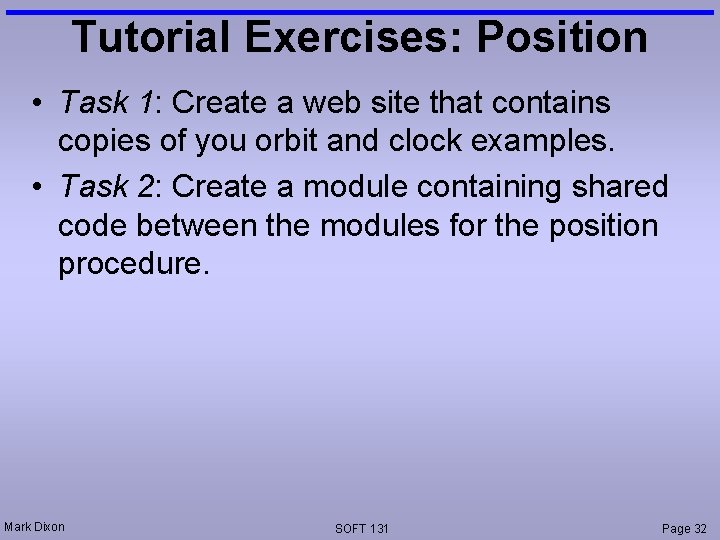
Tutorial Exercises: Position • Task 1: Create a web site that contains copies of you orbit and clock examples. • Task 2: Create a module containing shared code between the modules for the position procedure. Mark Dixon SOFT 131 Page 32