1 STRINGS CONCATENATION Concatenating two strings means joining
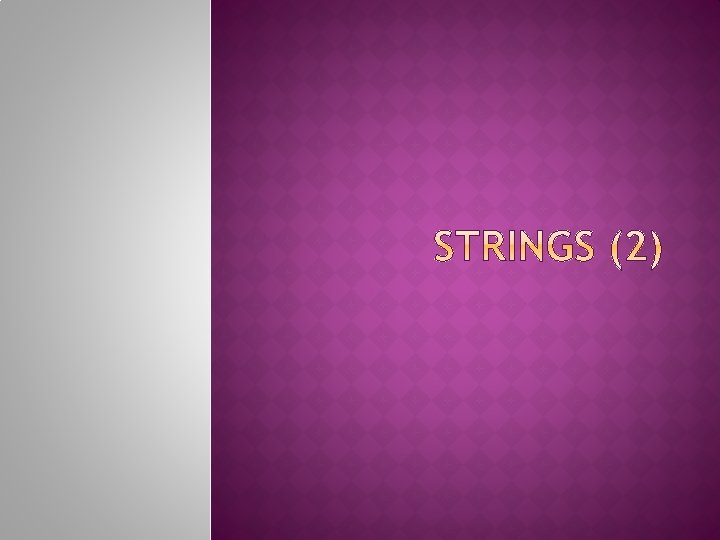
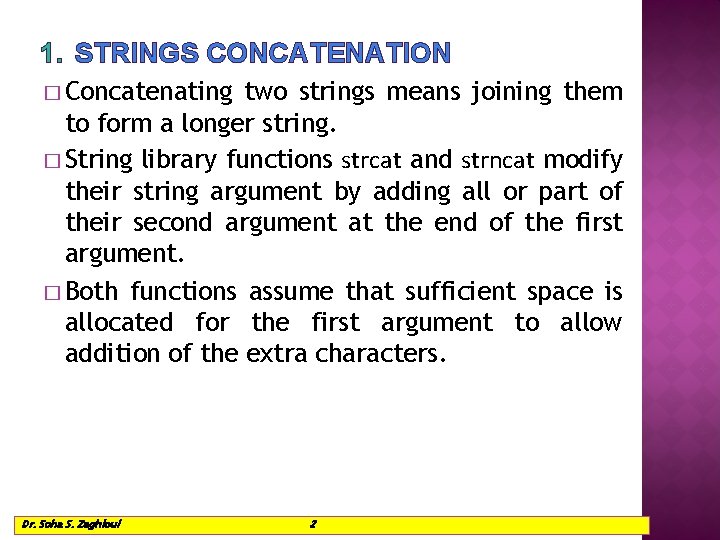
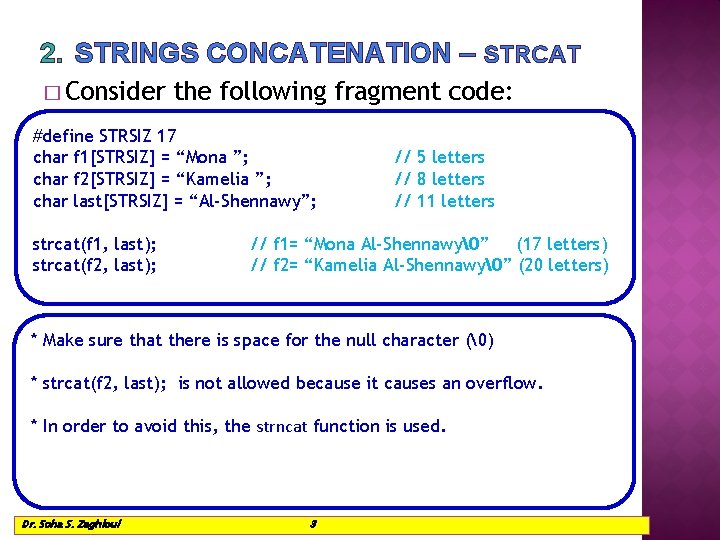
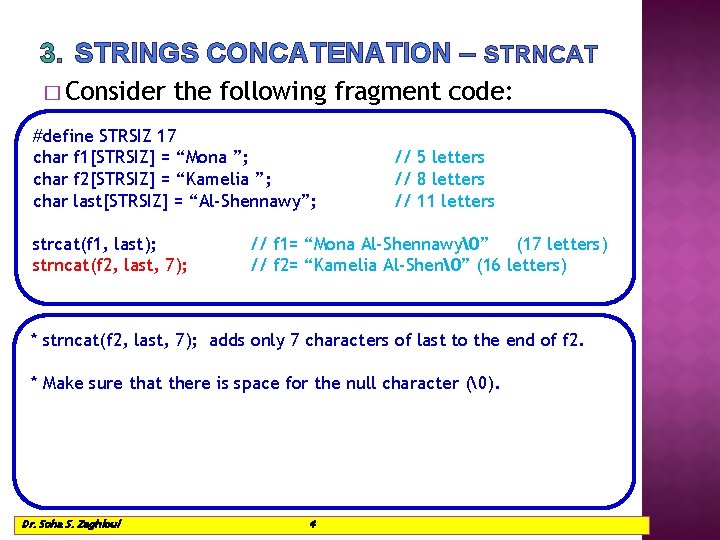
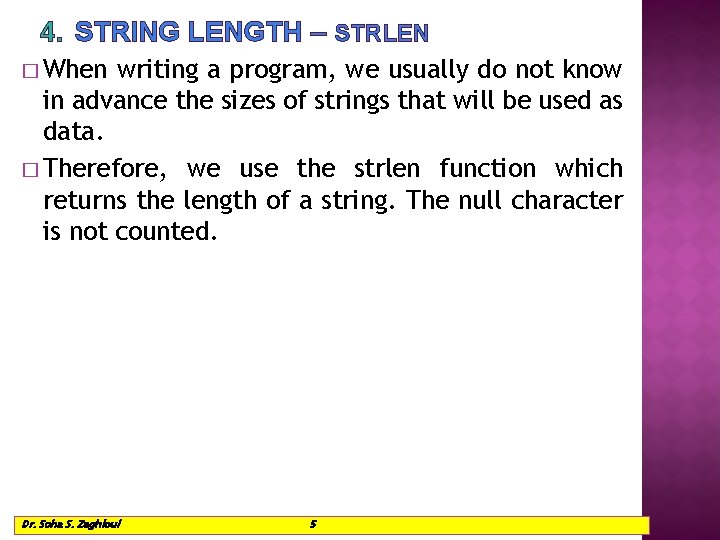
![5. STRING LENGTH – STRLEN – EXAMPLE #define STRSIZ 17 char f 1[STRSIZ] = 5. STRING LENGTH – STRLEN – EXAMPLE #define STRSIZ 17 char f 1[STRSIZ] =](https://slidetodoc.com/presentation_image/4e84adb24ff6b1a1e4929d1d0120d97e/image-6.jpg)
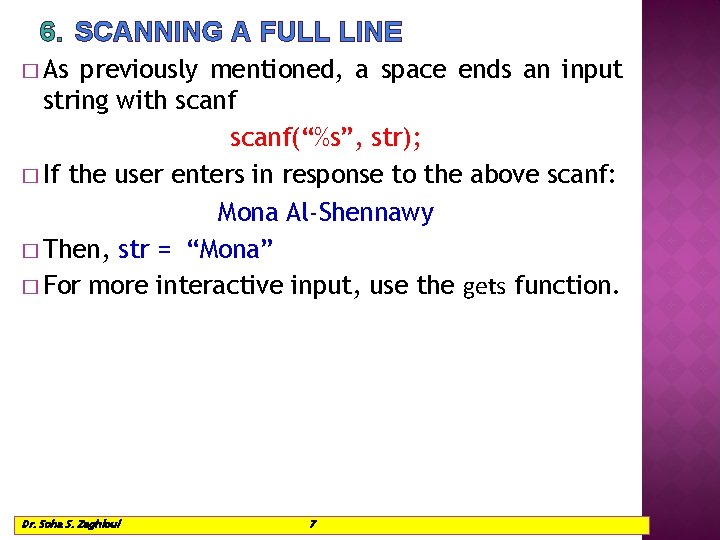
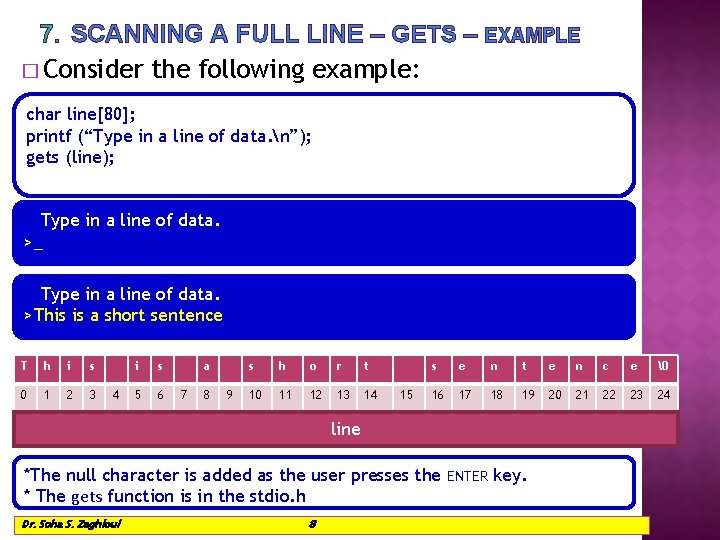
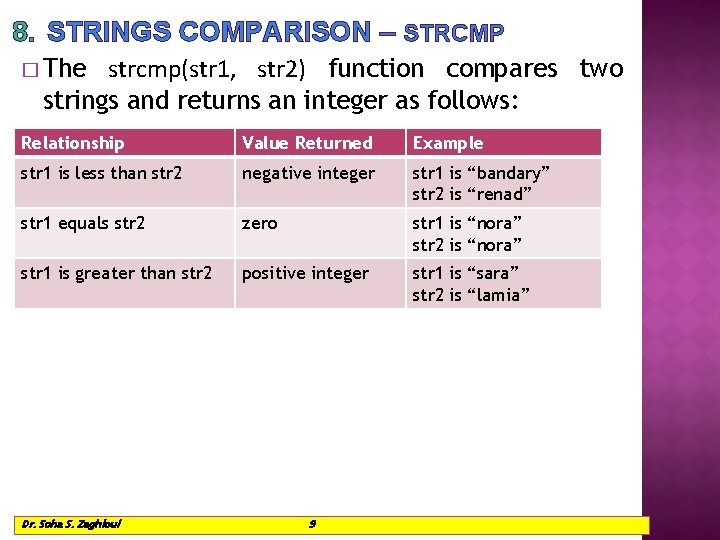
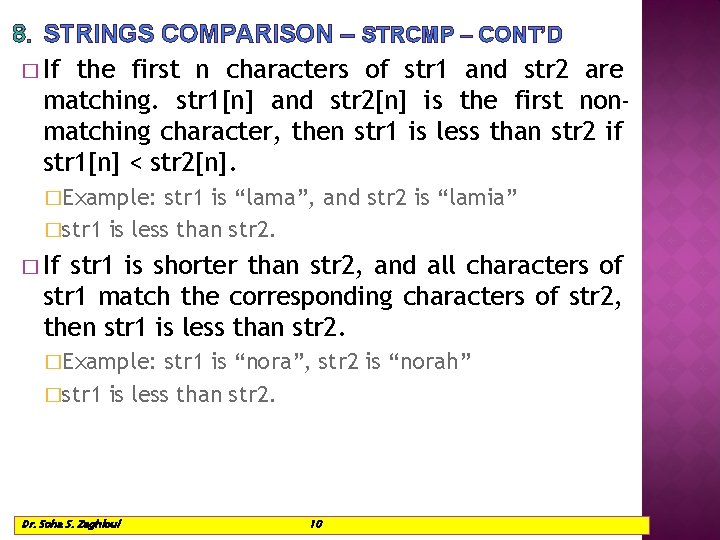
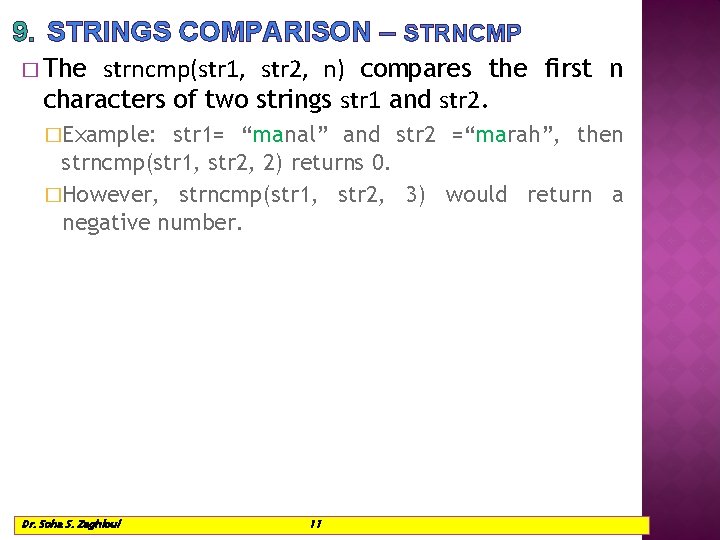
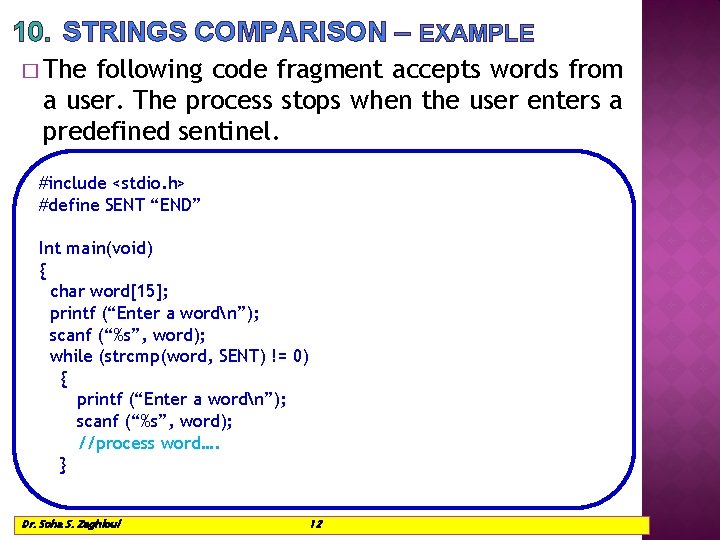
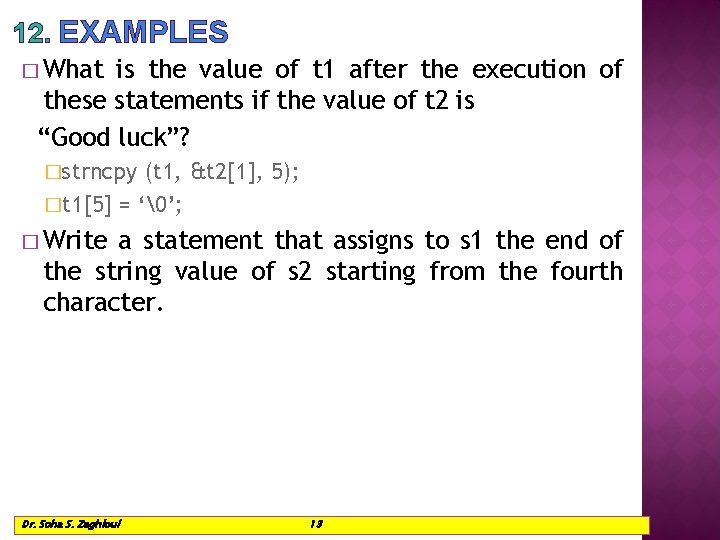
![13. SELF-CHECK EXERCISES � Given the following declarations: �char s 5[5], s 10[10], s 13. SELF-CHECK EXERCISES � Given the following declarations: �char s 5[5], s 10[10], s](https://slidetodoc.com/presentation_image/4e84adb24ff6b1a1e4929d1d0120d97e/image-14.jpg)
- Slides: 14
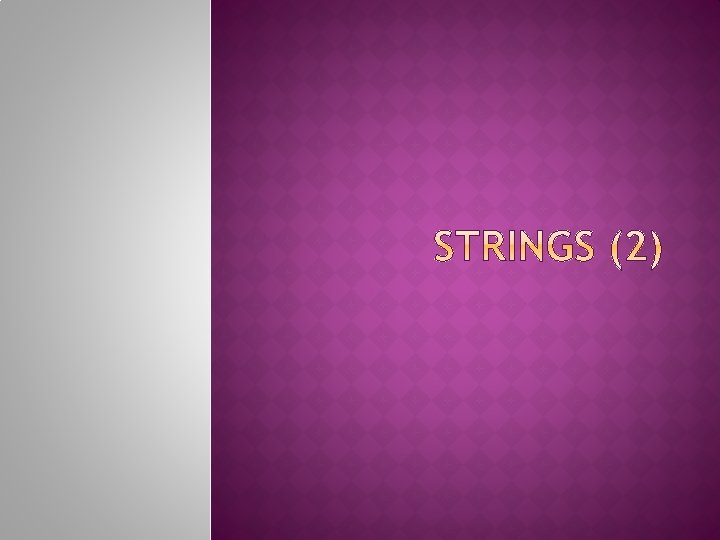
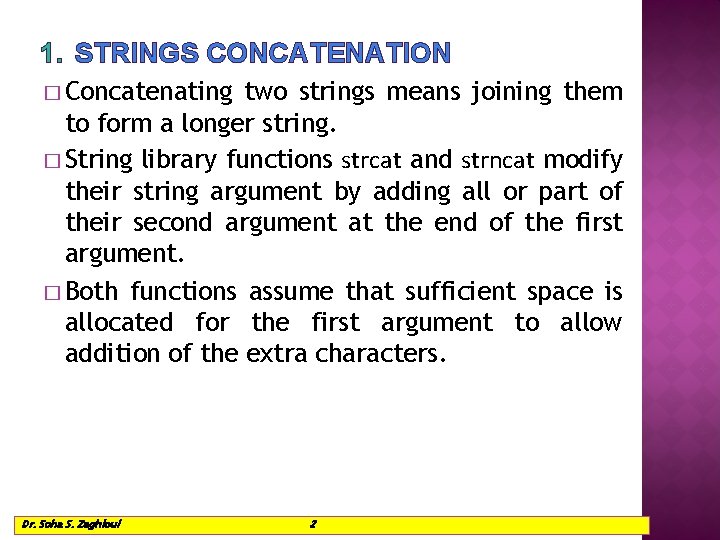
1. STRINGS CONCATENATION � Concatenating two strings means joining them to form a longer string. � String library functions strcat and strncat modify their string argument by adding all or part of their second argument at the end of the first argument. � Both functions assume that sufficient space is allocated for the first argument to allow addition of the extra characters. Dr. Soha S. Zaghloul 2
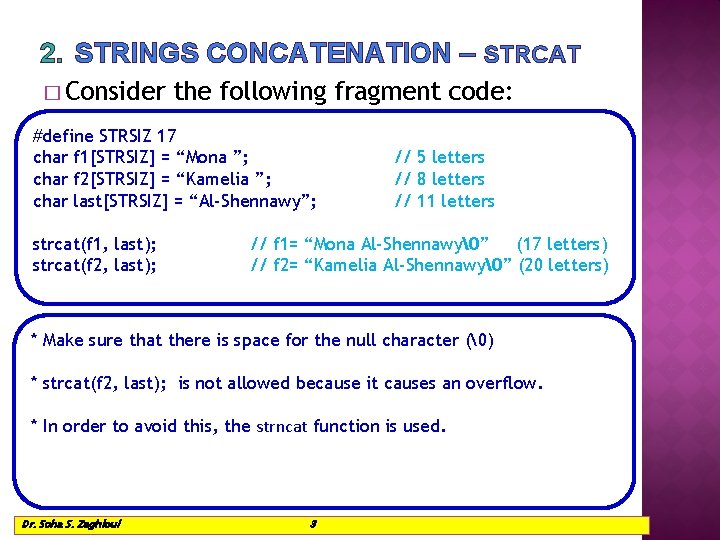
2. STRINGS CONCATENATION – STRCAT � Consider the following fragment code: #define STRSIZ 17 char f 1[STRSIZ] = “Mona ”; char f 2[STRSIZ] = “Kamelia ”; char last[STRSIZ] = “Al-Shennawy”; strcat(f 1, last); strcat(f 2, last); // 5 letters // 8 letters // 11 letters // f 1= “Mona Al-Shennawy ” (17 letters) // f 2= “Kamelia Al-Shennawy ” (20 letters) * Make sure that there is space for the null character ( ) * strcat(f 2, last); is not allowed because it causes an overflow. * In order to avoid this, the strncat function is used. Dr. Soha S. Zaghloul 3
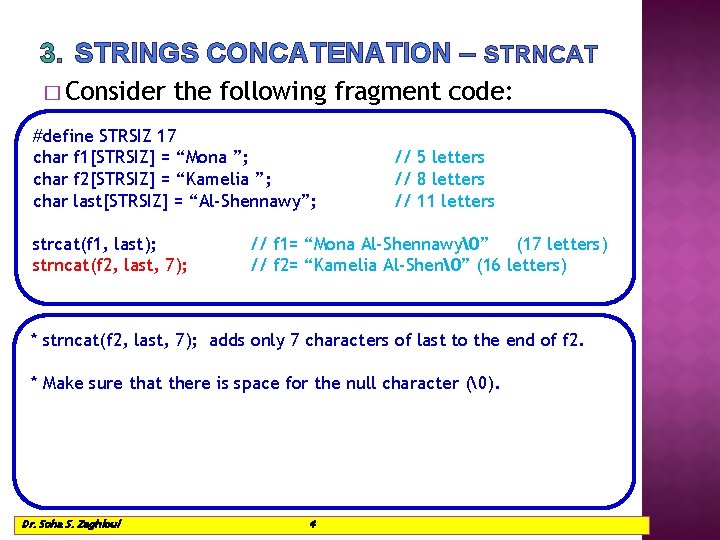
3. STRINGS CONCATENATION – STRNCAT � Consider the following fragment code: #define STRSIZ 17 char f 1[STRSIZ] = “Mona ”; char f 2[STRSIZ] = “Kamelia ”; char last[STRSIZ] = “Al-Shennawy”; strcat(f 1, last); strncat(f 2, last, 7); // 5 letters // 8 letters // 11 letters // f 1= “Mona Al-Shennawy ” (17 letters) // f 2= “Kamelia Al-Shen ” (16 letters) * strncat(f 2, last, 7); adds only 7 characters of last to the end of f 2. * Make sure that there is space for the null character ( ). Dr. Soha S. Zaghloul 4
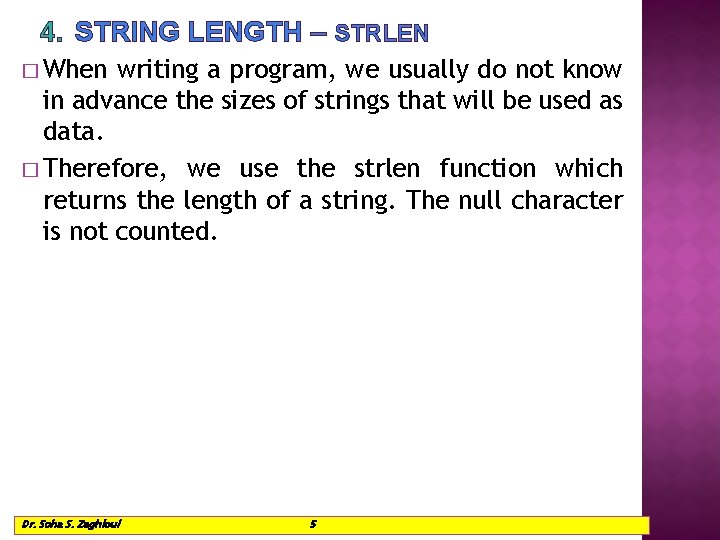
4. STRING LENGTH – STRLEN � When writing a program, we usually do not know in advance the sizes of strings that will be used as data. � Therefore, we use the strlen function which returns the length of a string. The null character is not counted. Dr. Soha S. Zaghloul 5
![5 STRING LENGTH STRLEN EXAMPLE define STRSIZ 17 char f 1STRSIZ 5. STRING LENGTH – STRLEN – EXAMPLE #define STRSIZ 17 char f 1[STRSIZ] =](https://slidetodoc.com/presentation_image/4e84adb24ff6b1a1e4929d1d0120d97e/image-6.jpg)
5. STRING LENGTH – STRLEN – EXAMPLE #define STRSIZ 17 char f 1[STRSIZ] = “Mona ”; char f 2[STRSIZ] = “Kamelia ”; char last[STRSIZ] = “Al-Shennawy”; // 5 letters // 8 letters // 11 letters if (strlen(f 1) + strlen(last) < STRSIZ) strcat (f 1, last); // ‘ ’ is automatically placed by strcat else { strncat(f 1, last, STRSIZ – strlen(f 1) – 1); f 1[STRSIZ – 1] = ‘ ’; // add the null character to the end of f 1 } Dr. Soha S. Zaghloul 6
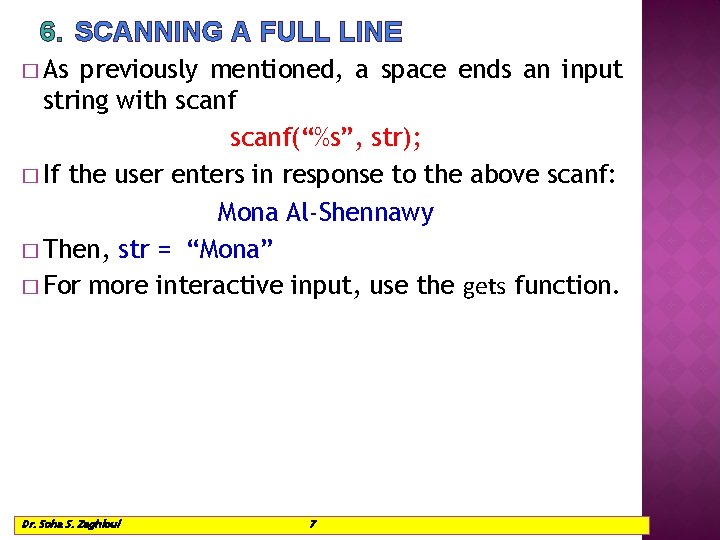
6. SCANNING A FULL LINE � As previously mentioned, a space ends an input string with scanf(“%s”, str); � If the user enters in response to the above scanf: Mona Al-Shennawy � Then, str = “Mona” � For more interactive input, use the gets function. Dr. Soha S. Zaghloul 7
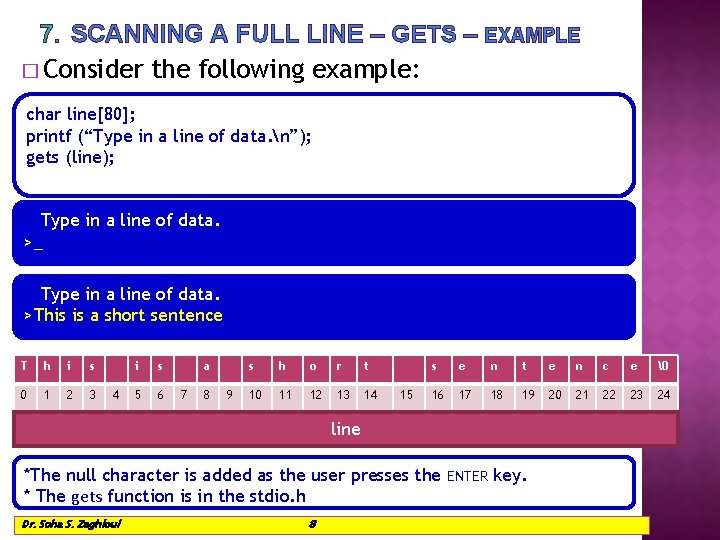
7. SCANNING A FULL LINE – GETS – EXAMPLE � Consider the following example: char line[80]; printf (“Type in a line of data. n”); gets (line); Type in a line of data. >_ Type in a line of data. >This is a short sentence T h i s 0 1 2 3 4 i s 5 6 a 7 8 9 s h o r t 10 11 12 13 14 15 s e n t e n c e 16 17 18 19 20 21 22 23 24 line *The null character is added as the user presses the ENTER key. * The gets function is in the stdio. h Dr. Soha S. Zaghloul 8
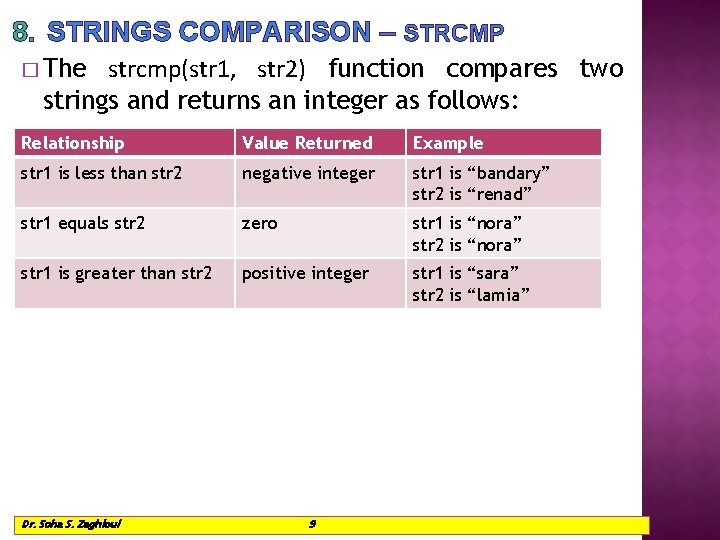
8. STRINGS COMPARISON – STRCMP strcmp(str 1, str 2) function compares two strings and returns an integer as follows: � The Relationship Value Returned Example str 1 is less than str 2 negative integer str 1 is “bandary” str 2 is “renad” str 1 equals str 2 zero str 1 is “nora” str 2 is “nora” str 1 is greater than str 2 positive integer str 1 is “sara” str 2 is “lamia” Dr. Soha S. Zaghloul 9
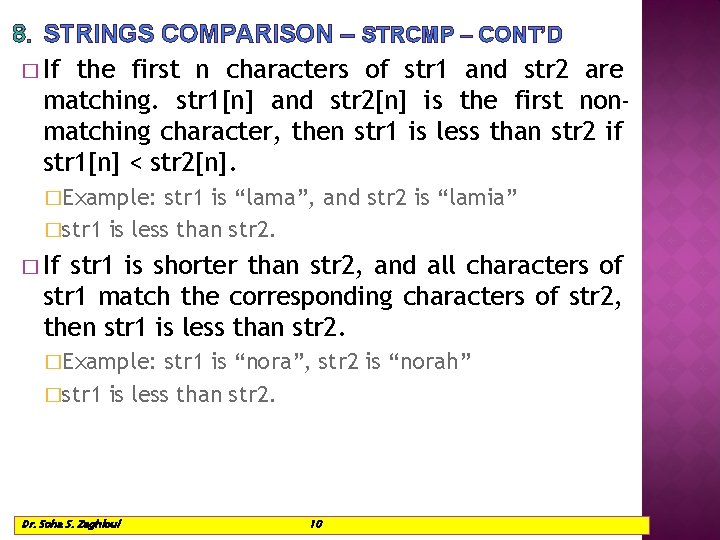
8. STRINGS COMPARISON – STRCMP – CONT’D � If the first n characters of str 1 and str 2 are matching. str 1[n] and str 2[n] is the first nonmatching character, then str 1 is less than str 2 if str 1[n] < str 2[n]. �Example: str 1 is “lama”, and str 2 is “lamia” �str 1 is less than str 2. � If str 1 is shorter than str 2, and all characters of str 1 match the corresponding characters of str 2, then str 1 is less than str 2. �Example: str 1 is “nora”, str 2 is “norah” �str 1 is less than str 2. Dr. Soha S. Zaghloul 10
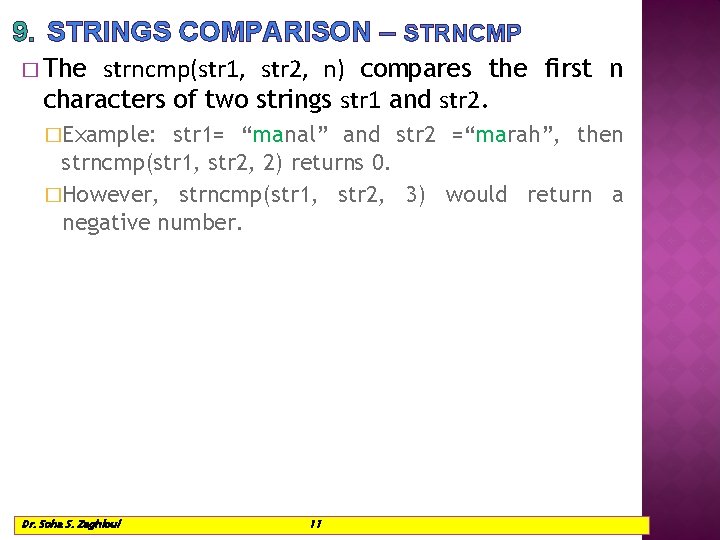
9. STRINGS COMPARISON – STRNCMP strncmp(str 1, str 2, n) compares the first n characters of two strings str 1 and str 2. � The �Example: str 1= “manal” and str 2 =“marah”, then strncmp(str 1, str 2, 2) returns 0. �However, strncmp(str 1, str 2, 3) would return a negative number. Dr. Soha S. Zaghloul 11
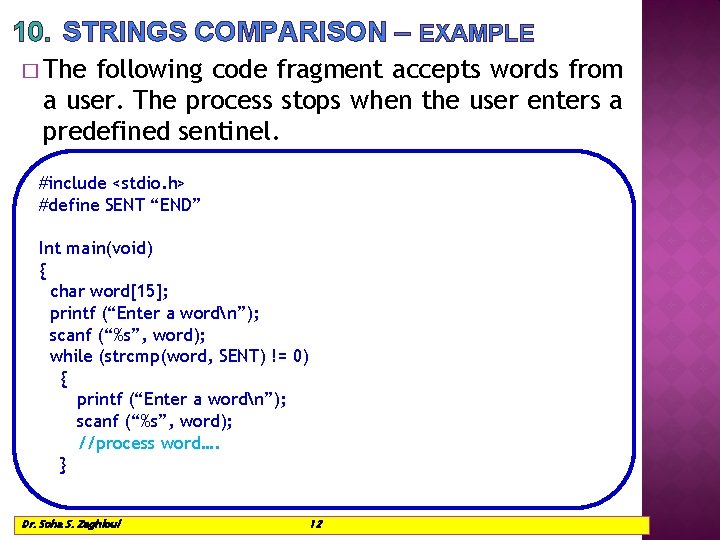
10. STRINGS COMPARISON – EXAMPLE � The following code fragment accepts words from a user. The process stops when the user enters a predefined sentinel. #include <stdio. h> #define SENT “END” Int main(void) { char word[15]; printf (“Enter a wordn”); scanf (“%s”, word); while (strcmp(word, SENT) != 0) { printf (“Enter a wordn”); scanf (“%s”, word); //process word…. } Dr. Soha S. Zaghloul 12
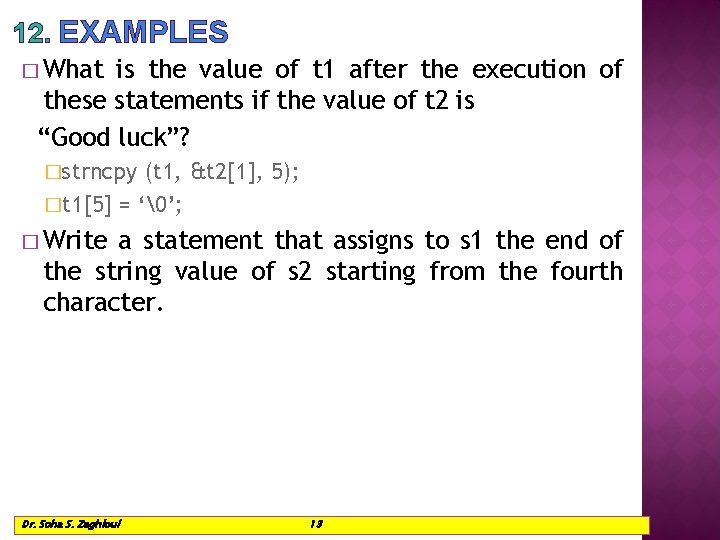
12. EXAMPLES � What is the value of t 1 after the execution of these statements if the value of t 2 is “Good luck”? �strncpy (t 1, &t 2[1], 5); �t 1[5] = ‘ ’; � Write a statement that assigns to s 1 the end of the string value of s 2 starting from the fourth character. Dr. Soha S. Zaghloul 13
![13 SELFCHECK EXERCISES Given the following declarations char s 55 s 1010 s 13. SELF-CHECK EXERCISES � Given the following declarations: �char s 5[5], s 10[10], s](https://slidetodoc.com/presentation_image/4e84adb24ff6b1a1e4929d1d0120d97e/image-14.jpg)
13. SELF-CHECK EXERCISES � Given the following declarations: �char s 5[5], s 10[10], s 20[20]; �char aday[7] = “sunday”; �char another[9] = “saturday”; � What is the output of the following: �strncopy(s 5, another, 4); s 5[4] = ‘ ’; �strcpy (s 10, &aday[3]); �strlen (another); �strcpy (s 20, aday); strcat(s 20, another); Dr. Soha S. Zaghloul 14