1 String Char Methods For the char value
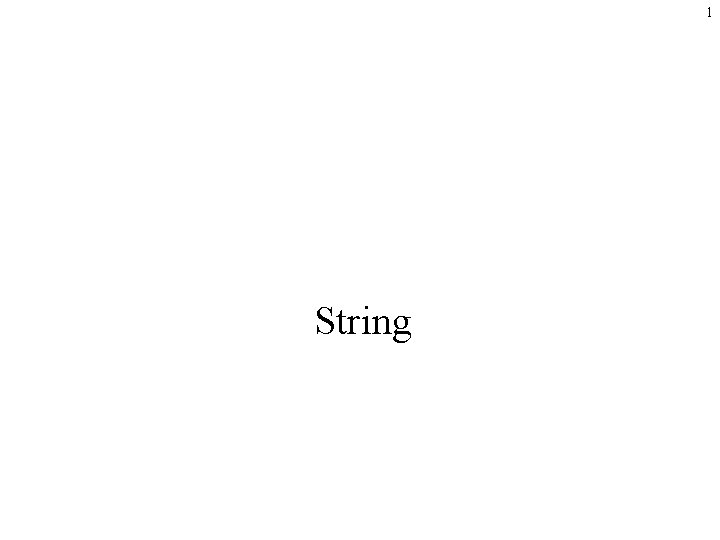
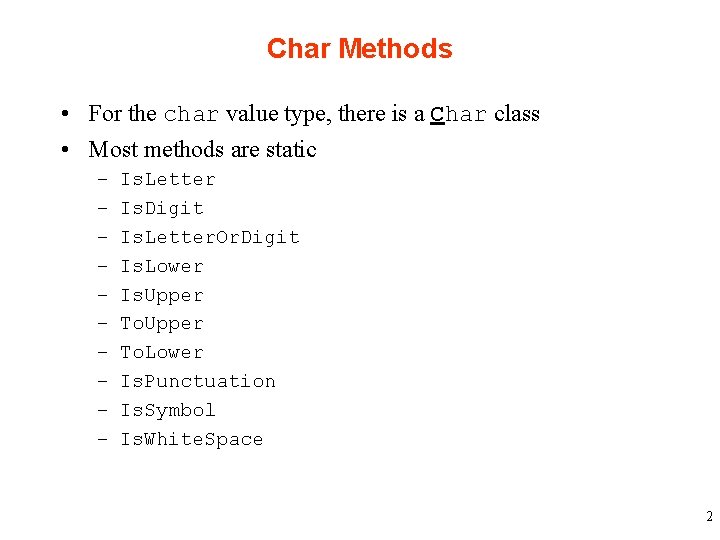
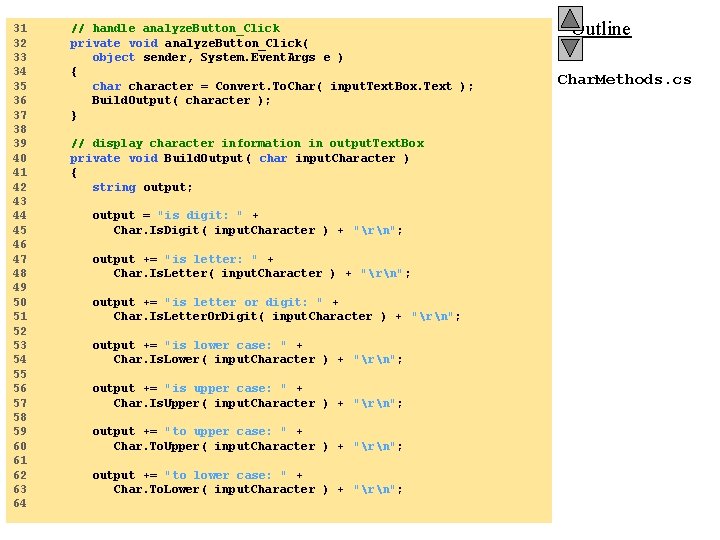
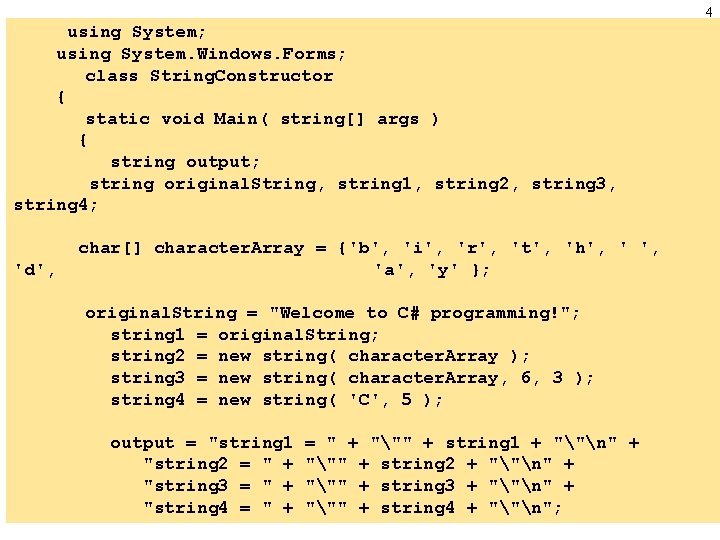
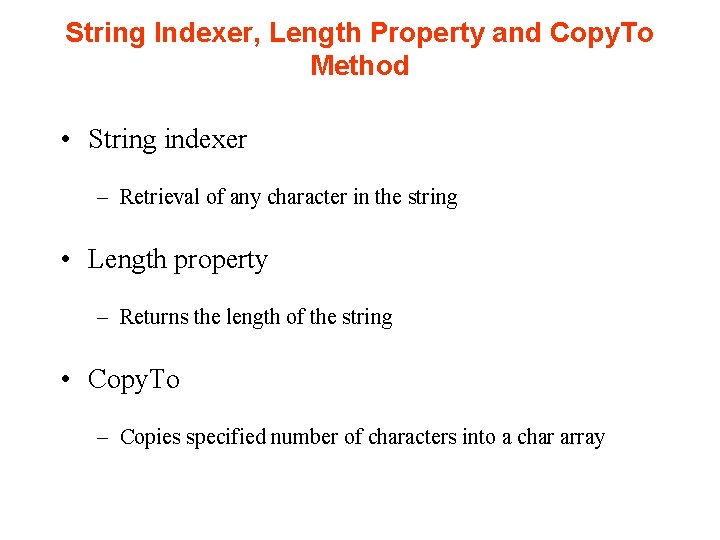
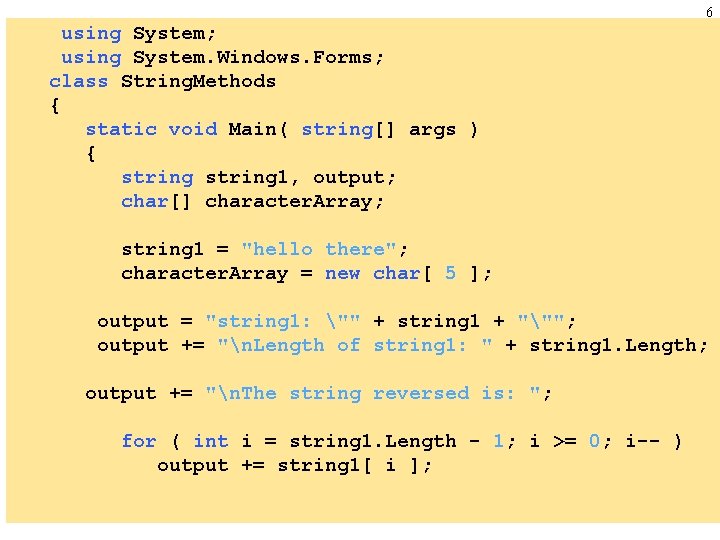
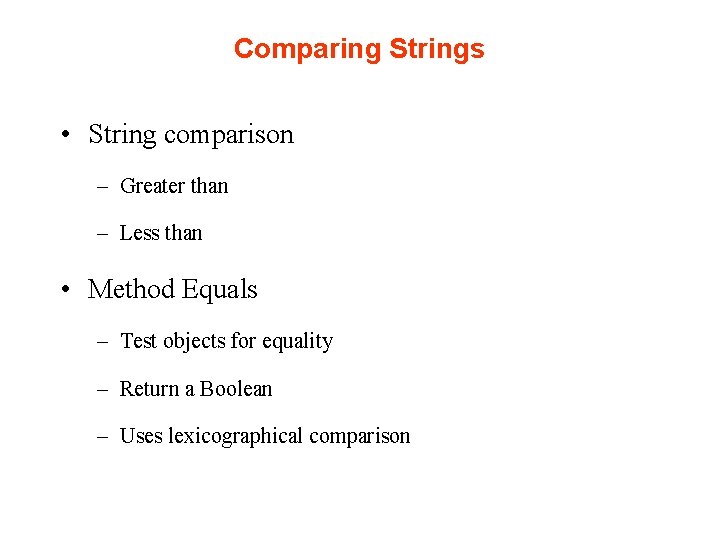
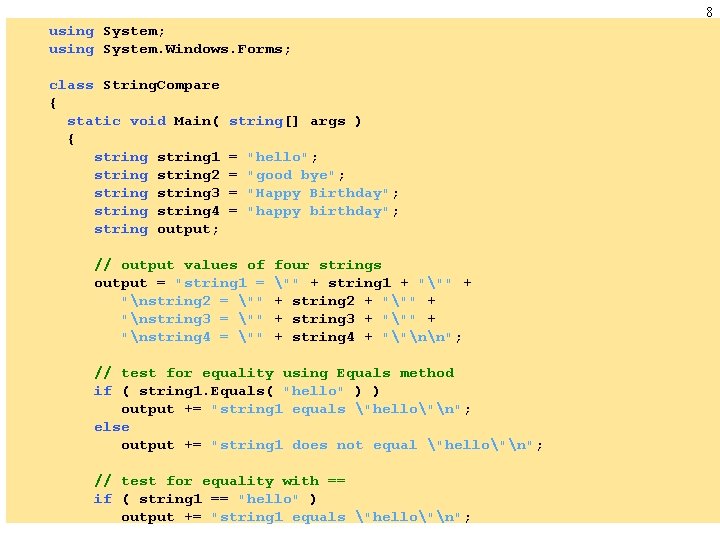
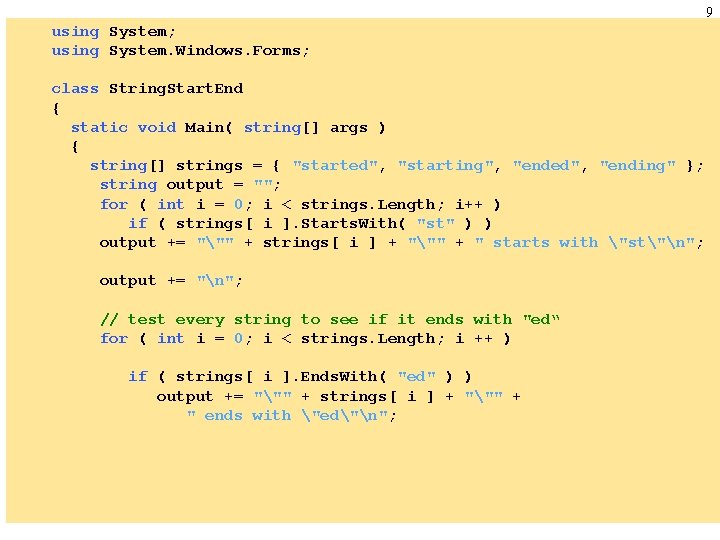
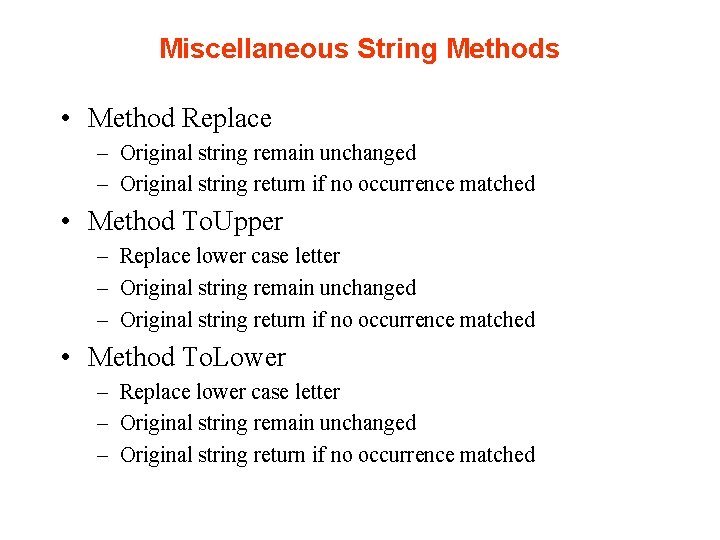
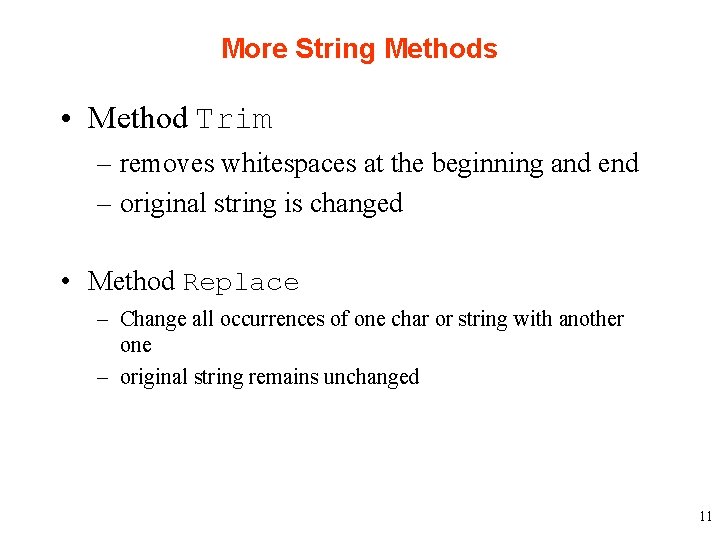
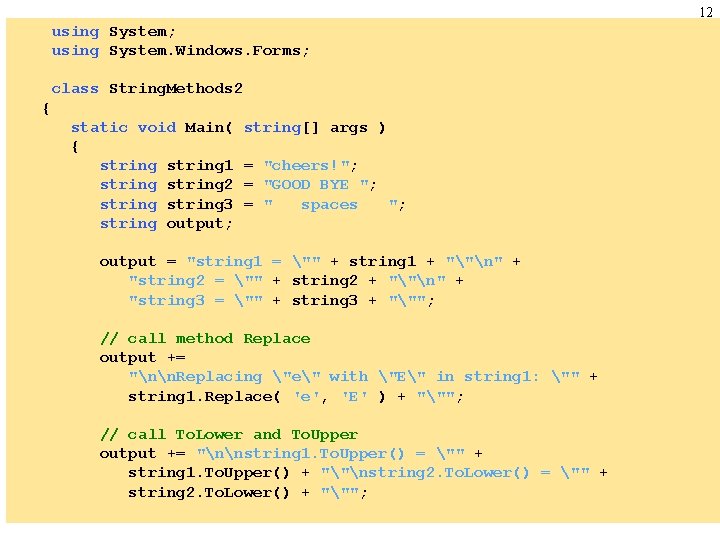
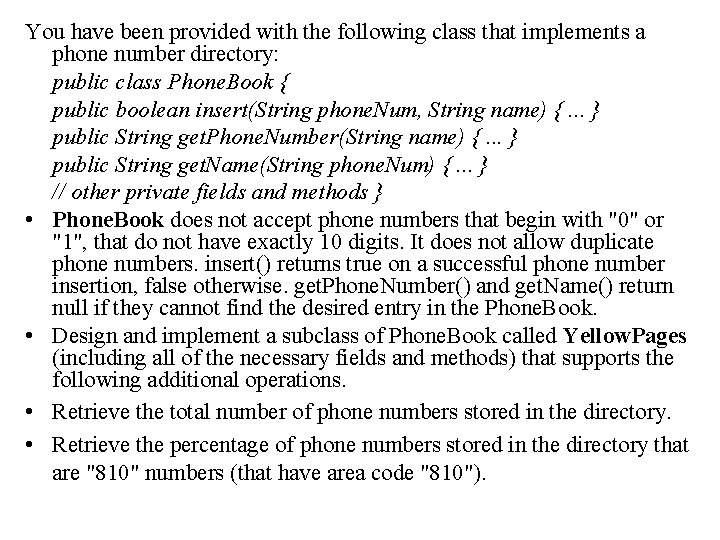
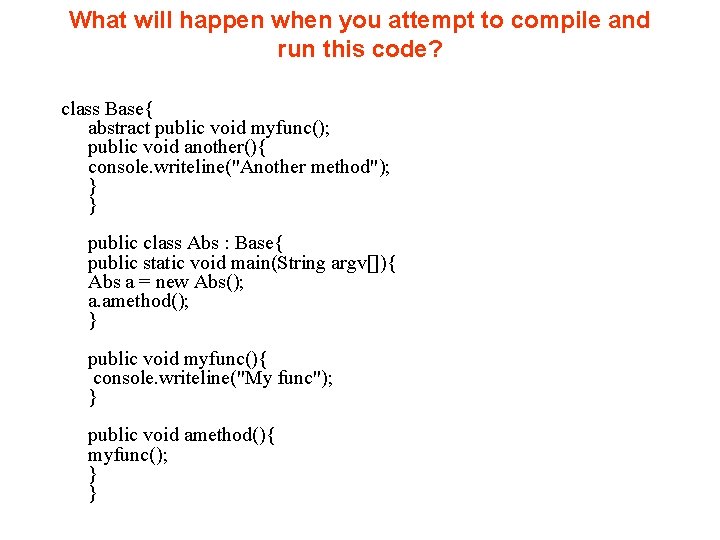
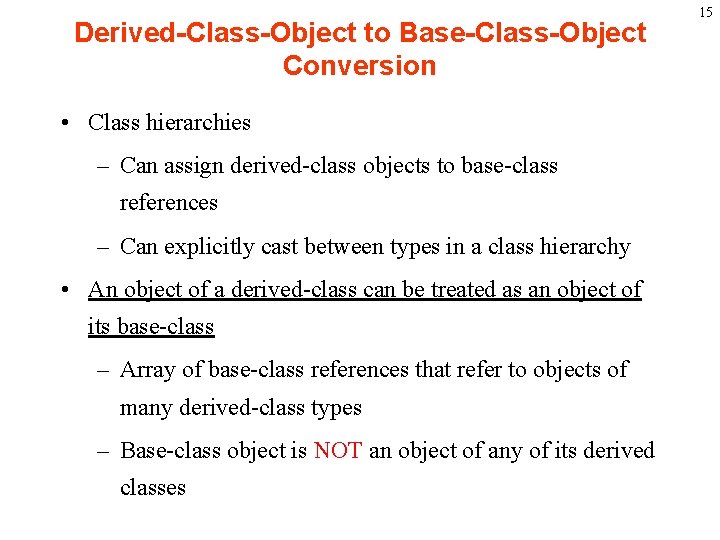
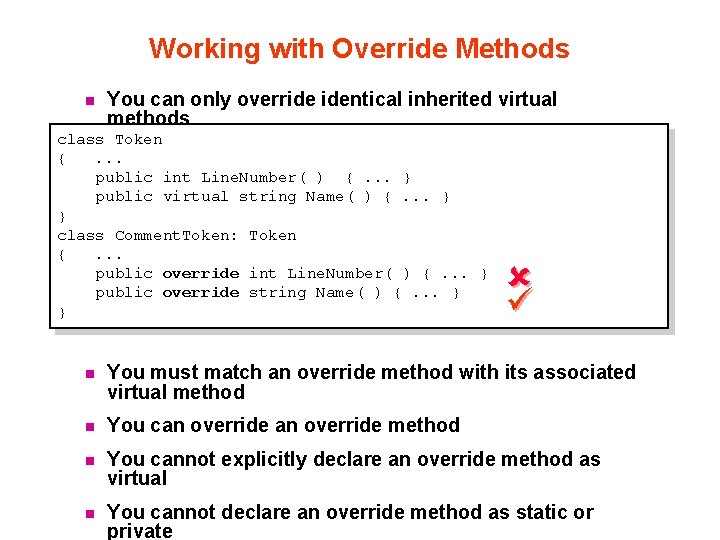
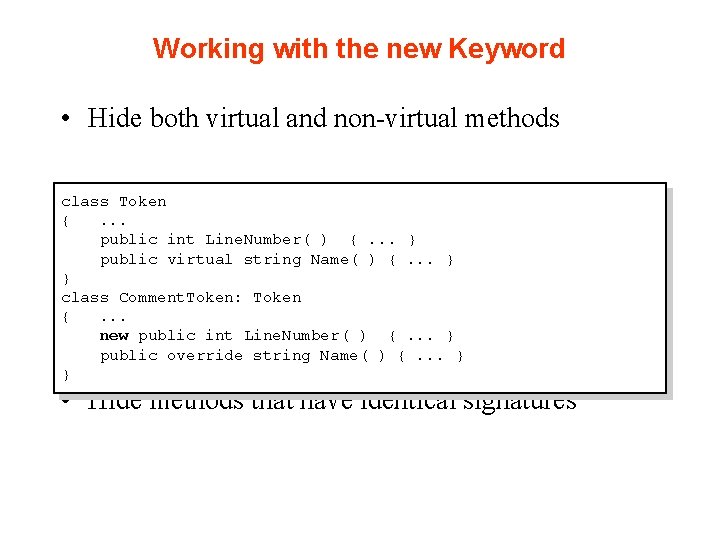
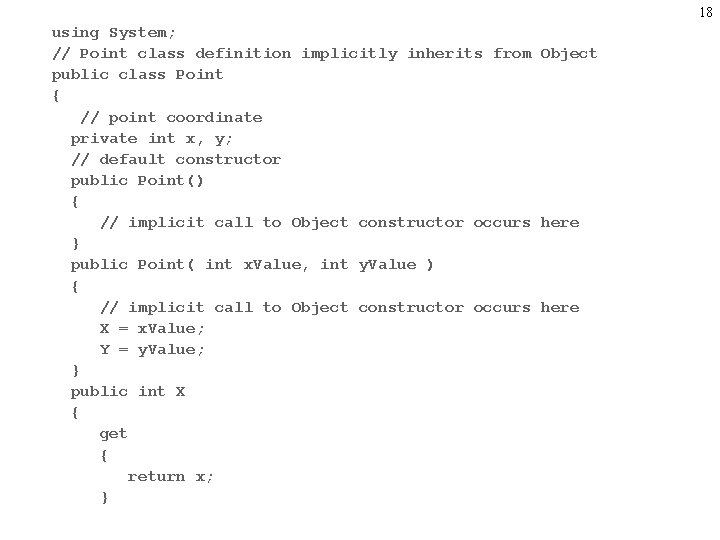
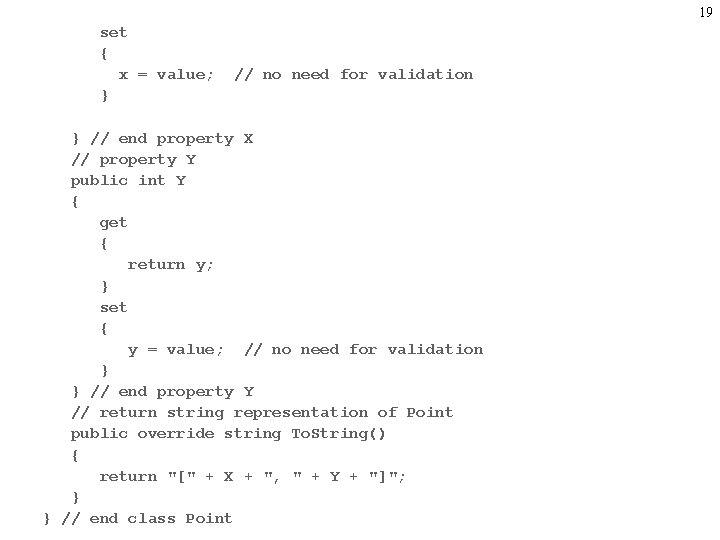
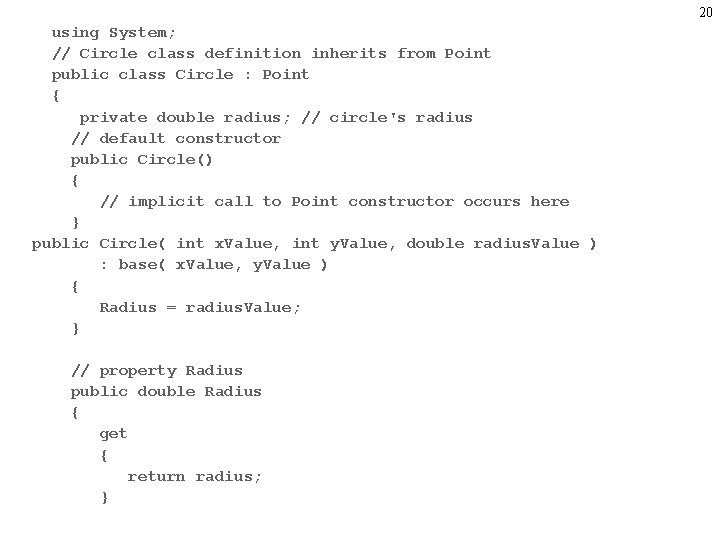
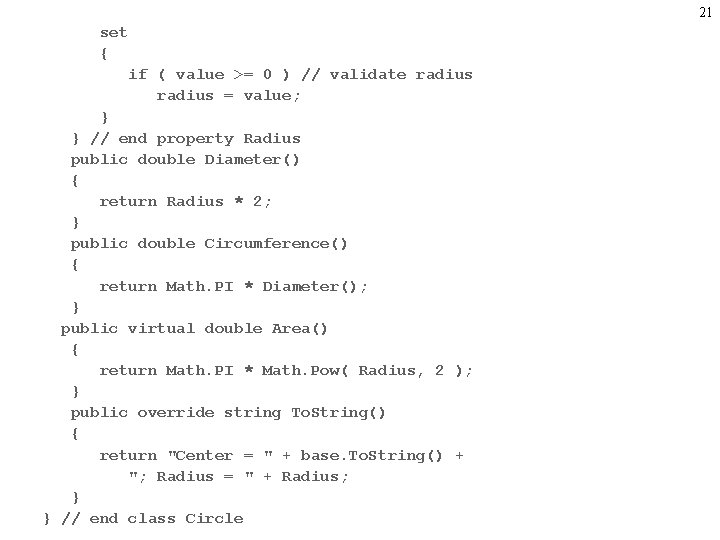
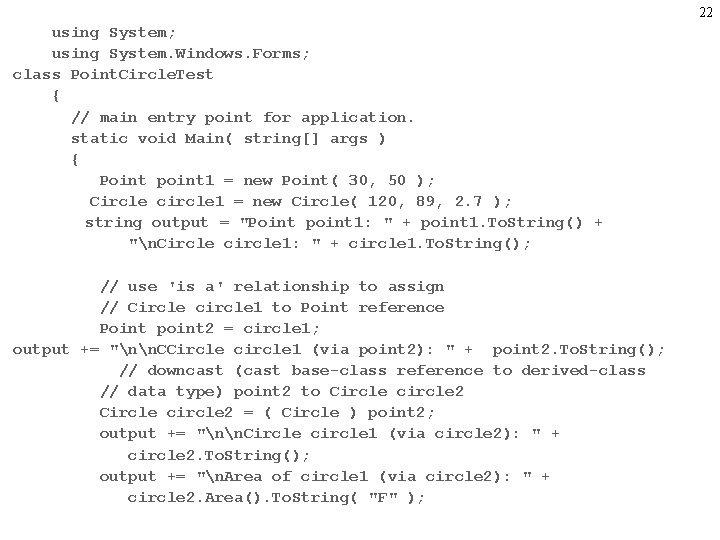
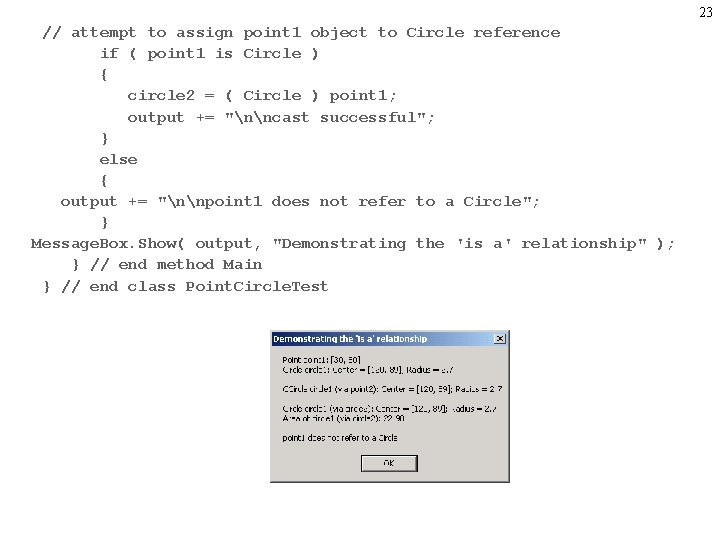
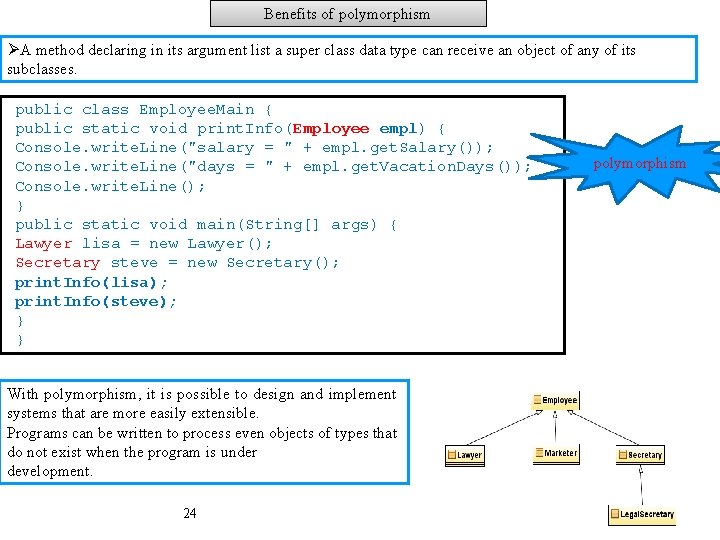
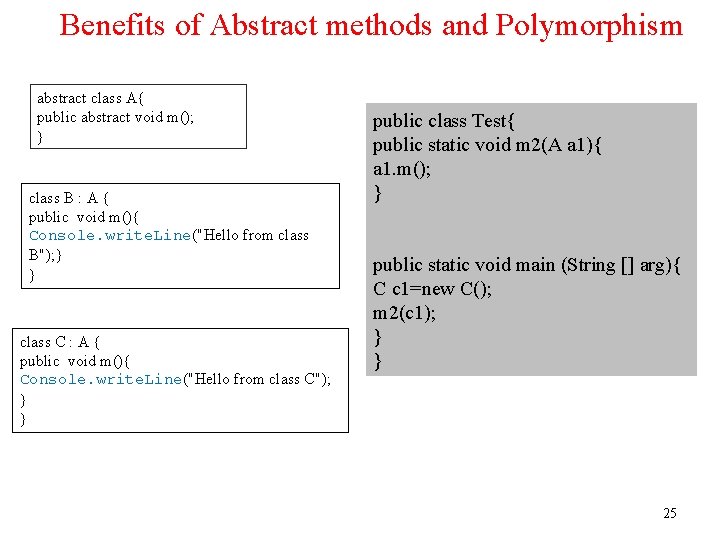
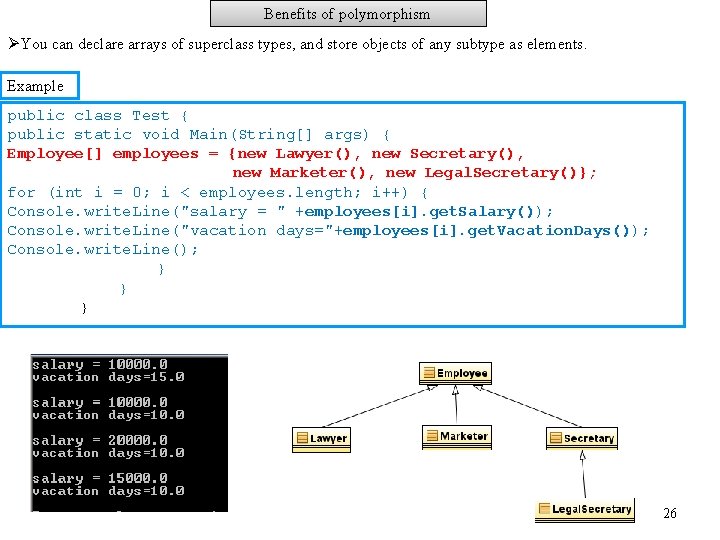
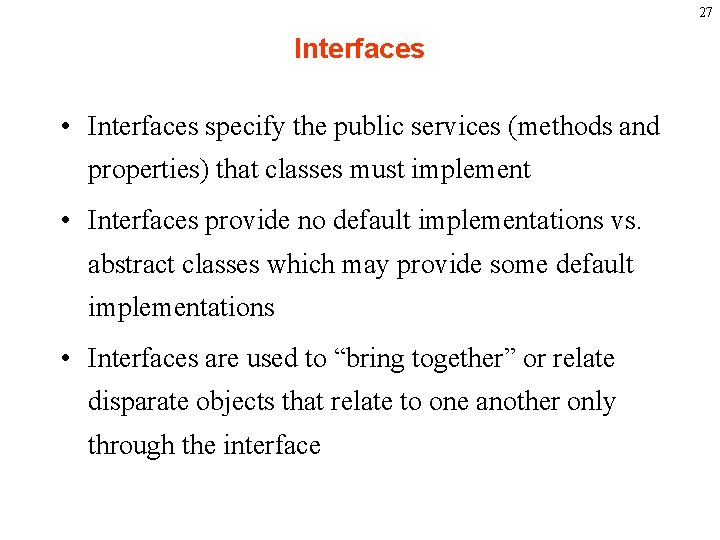
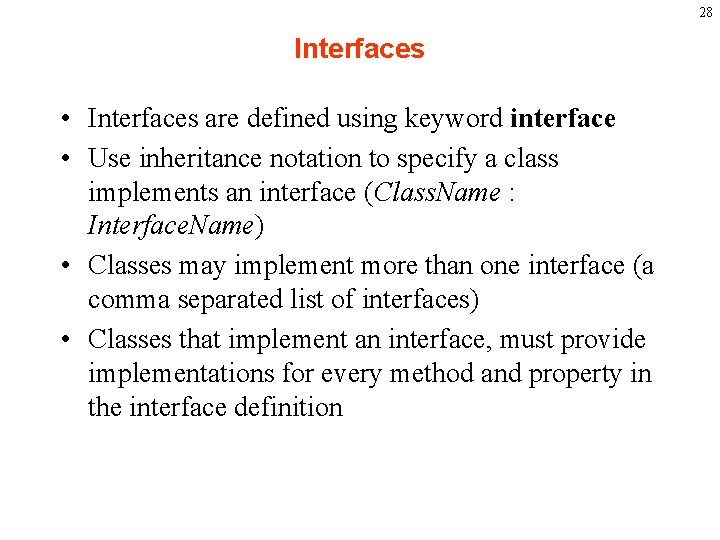
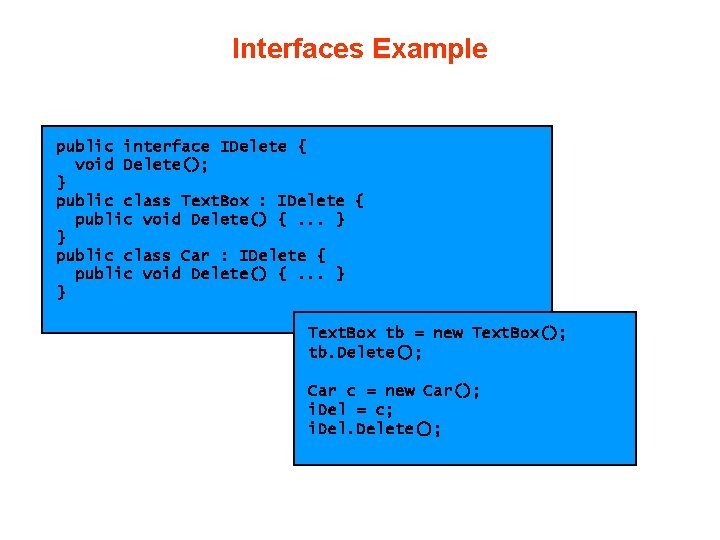
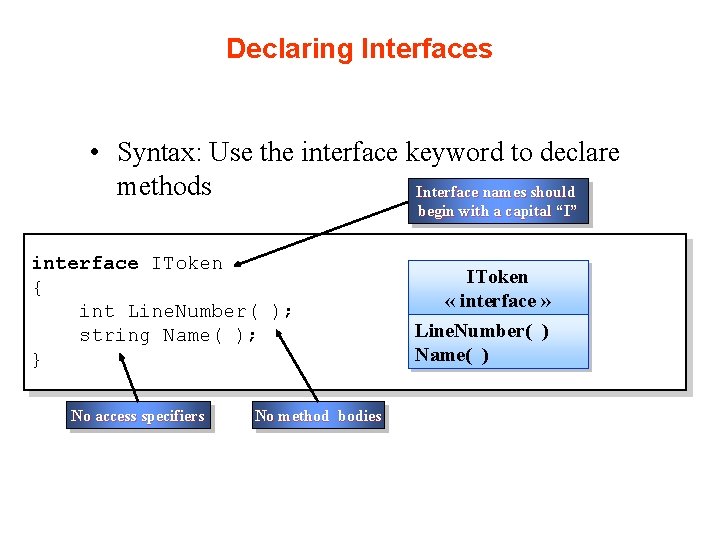
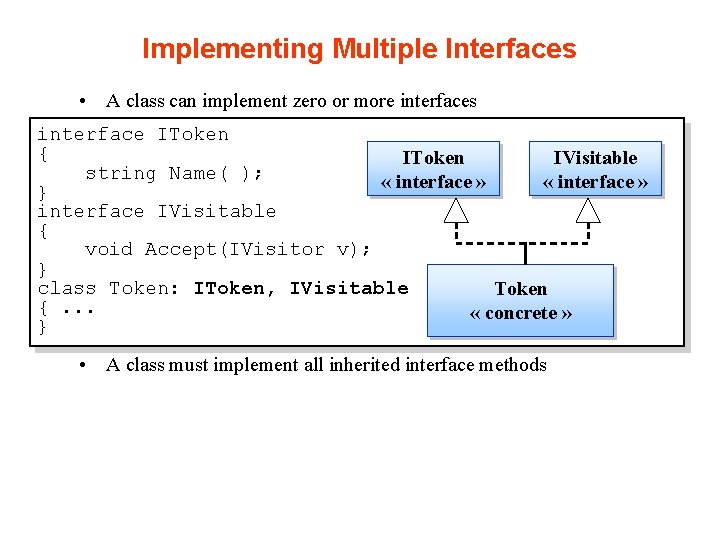
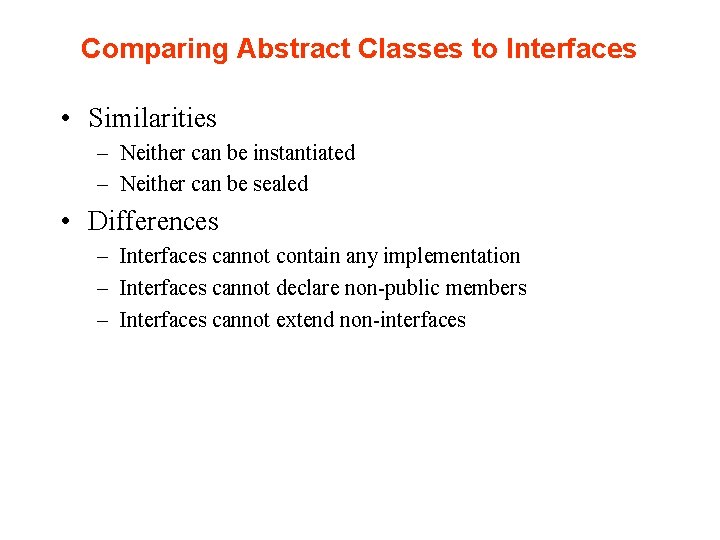
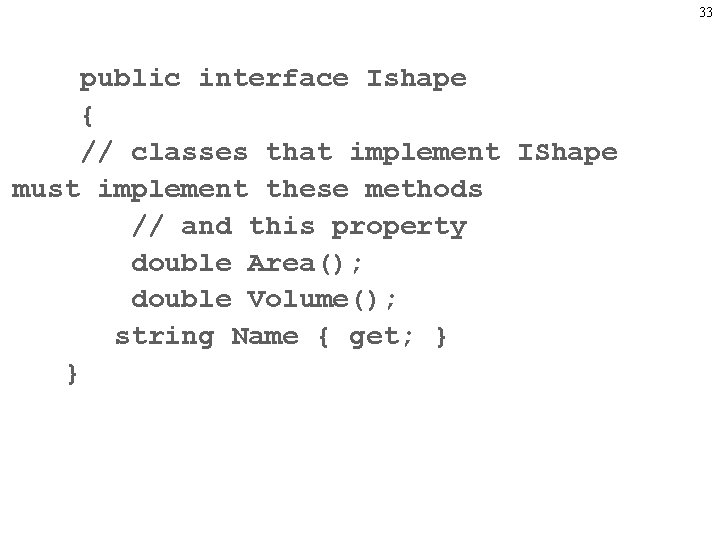
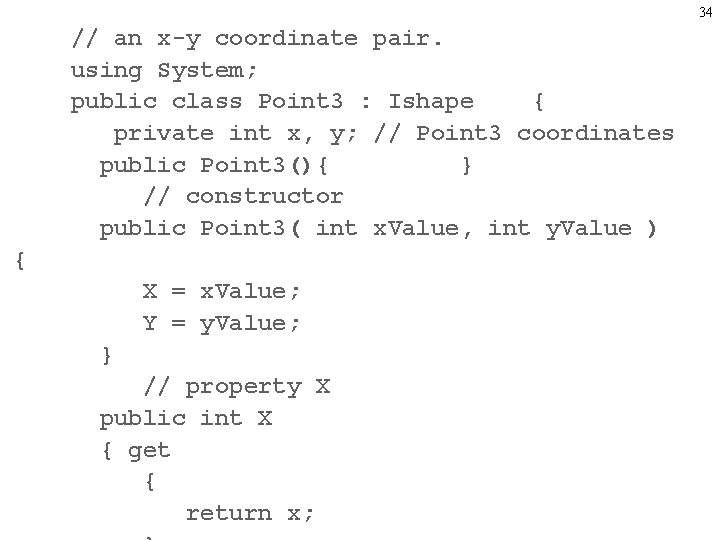
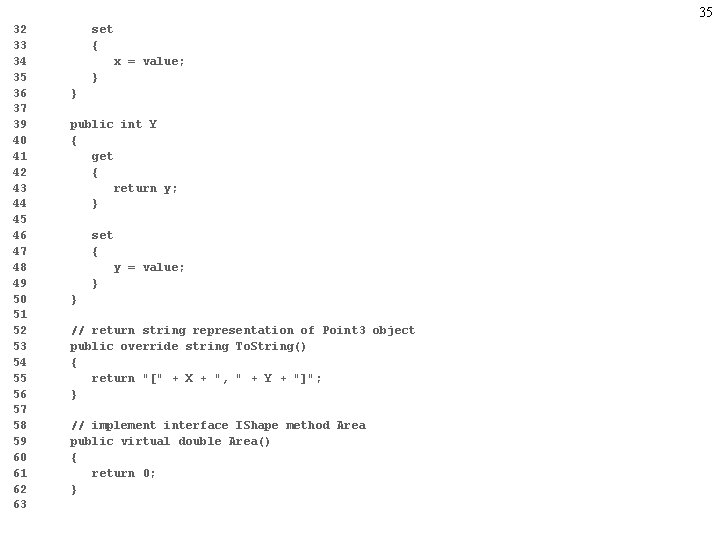
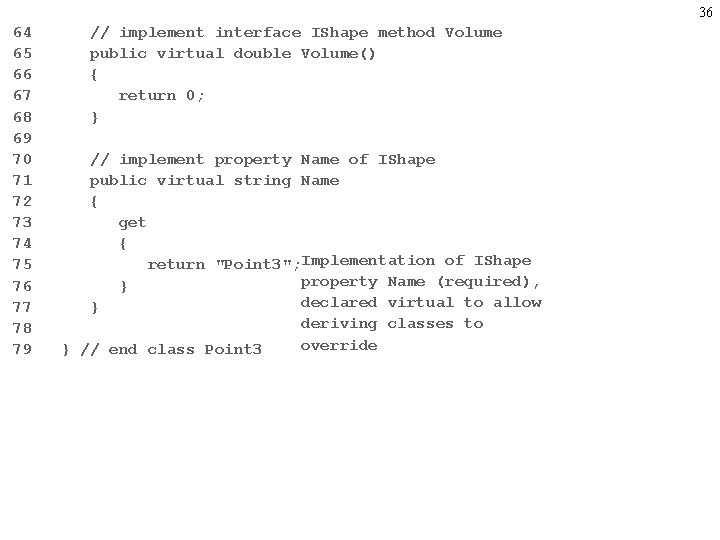
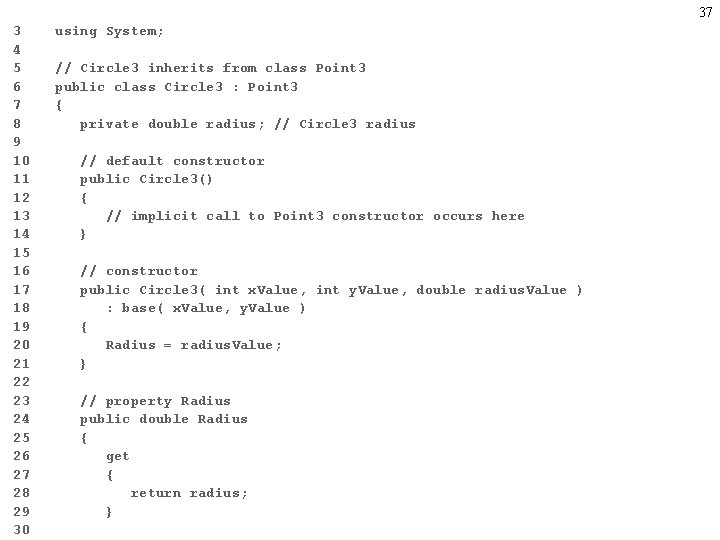
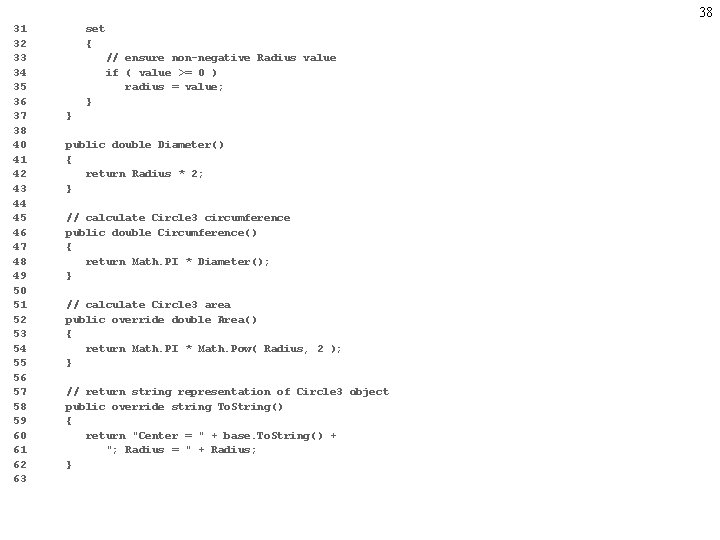
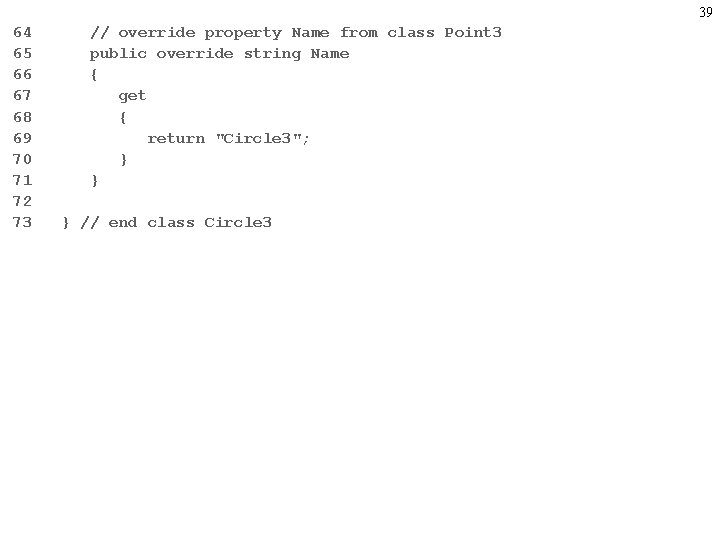
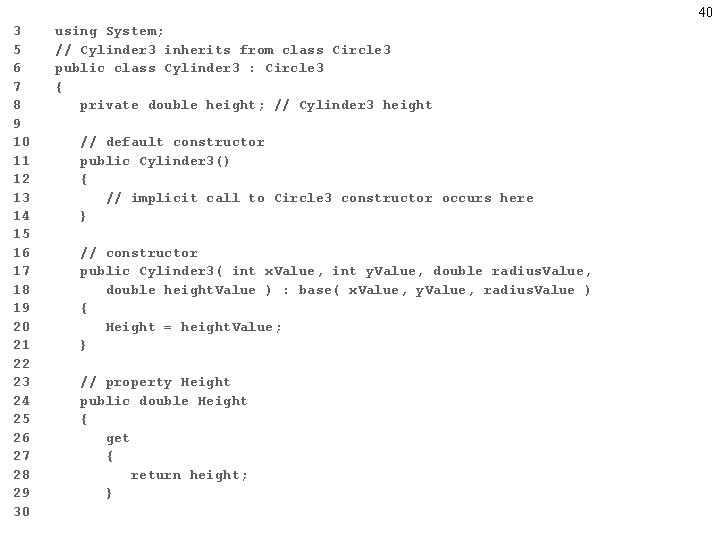
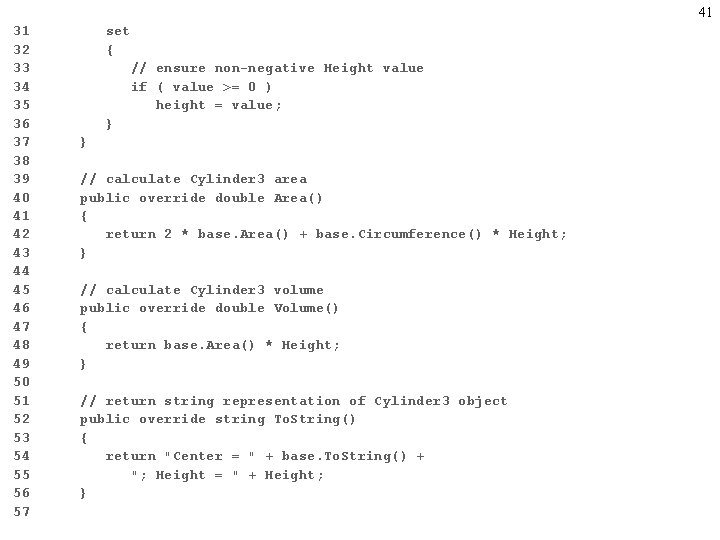
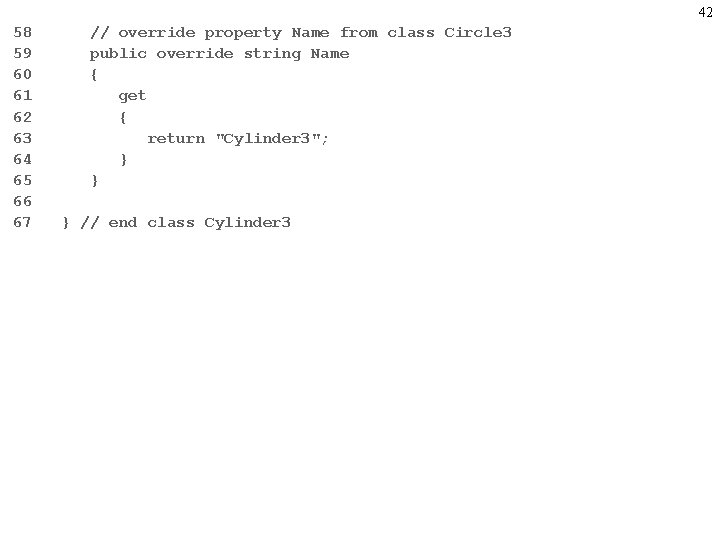
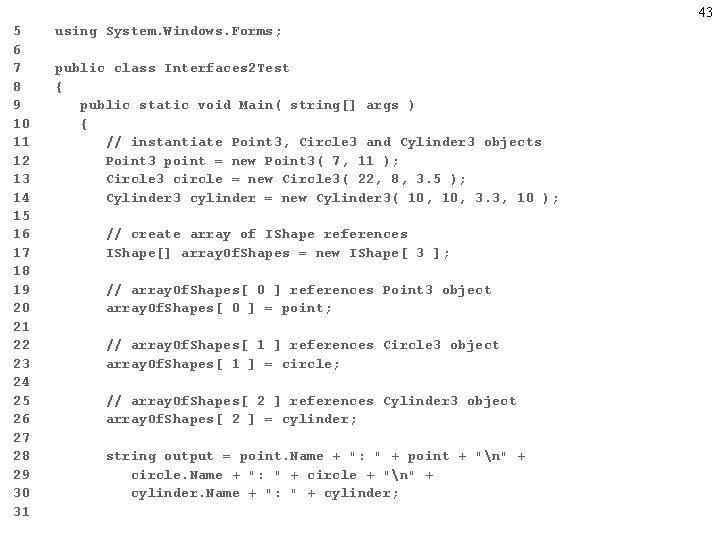
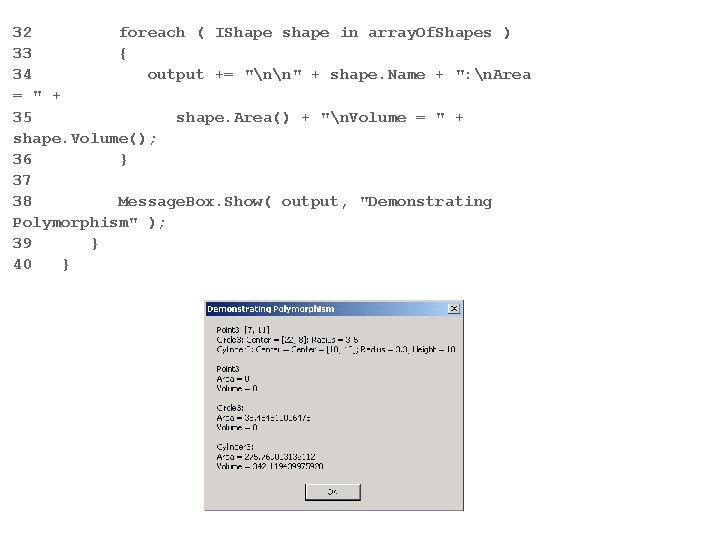
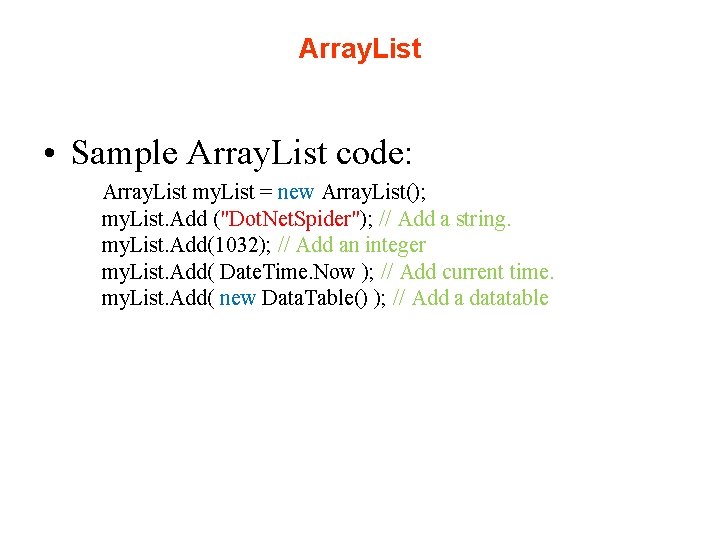
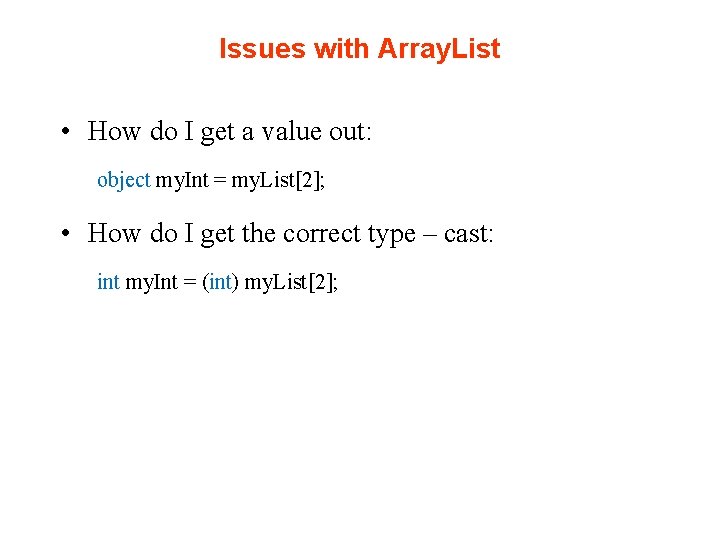
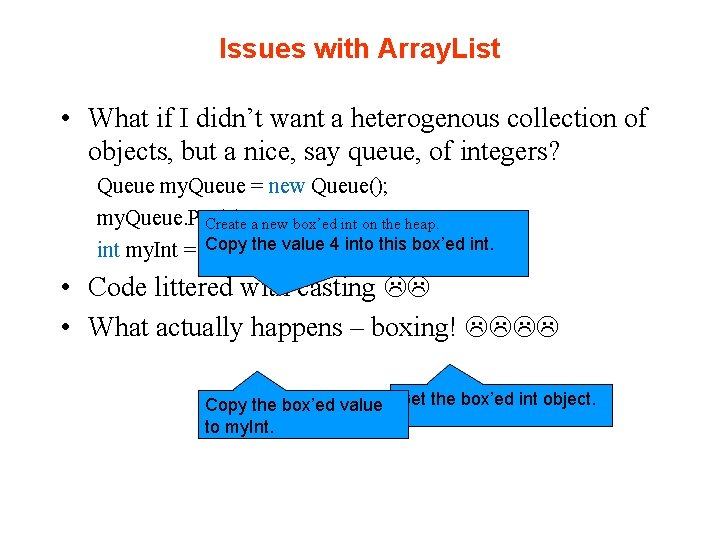
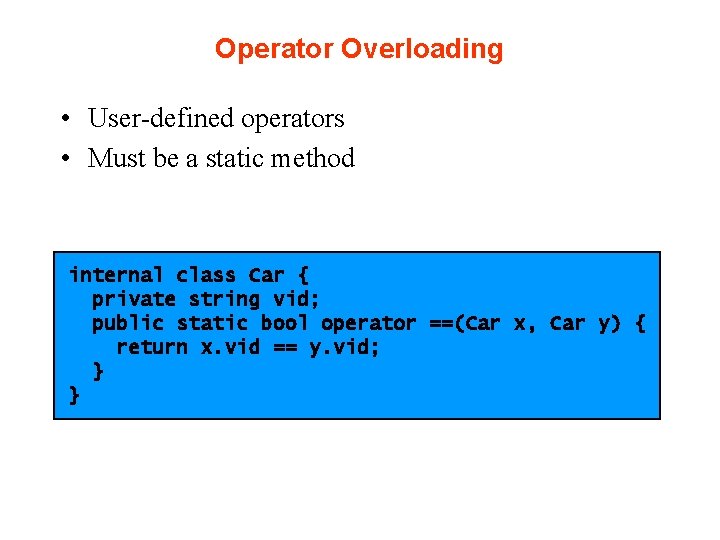
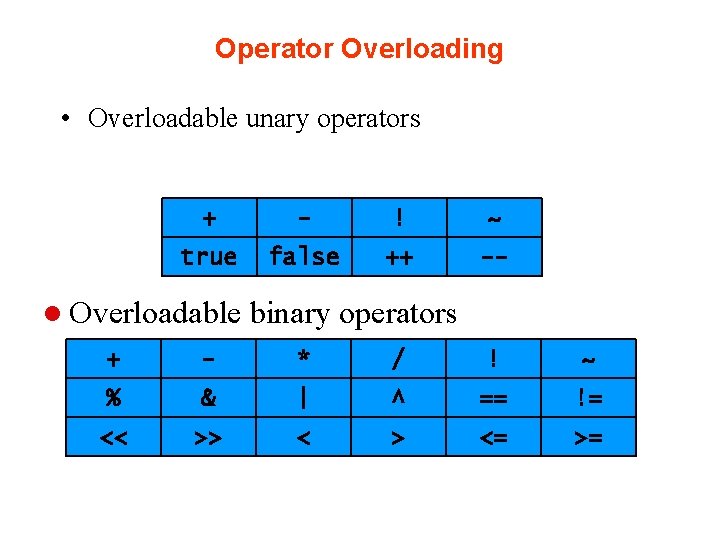
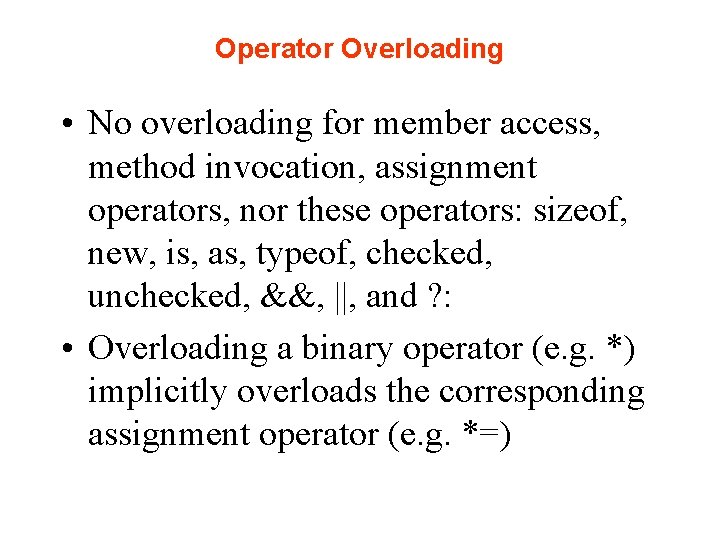
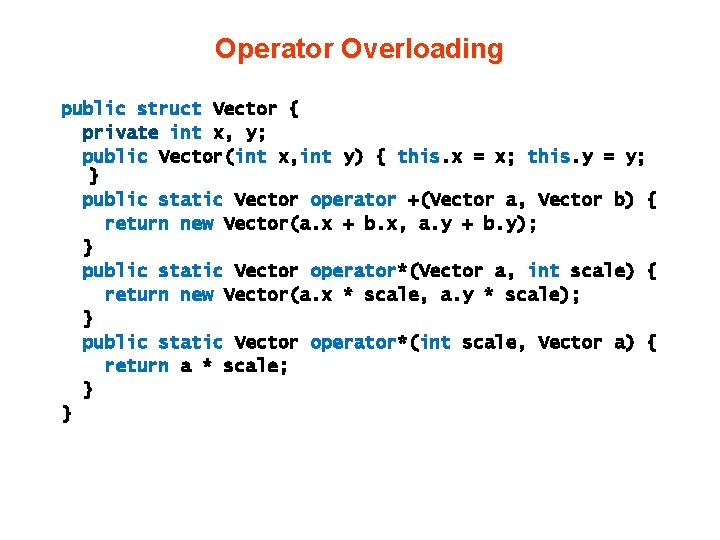
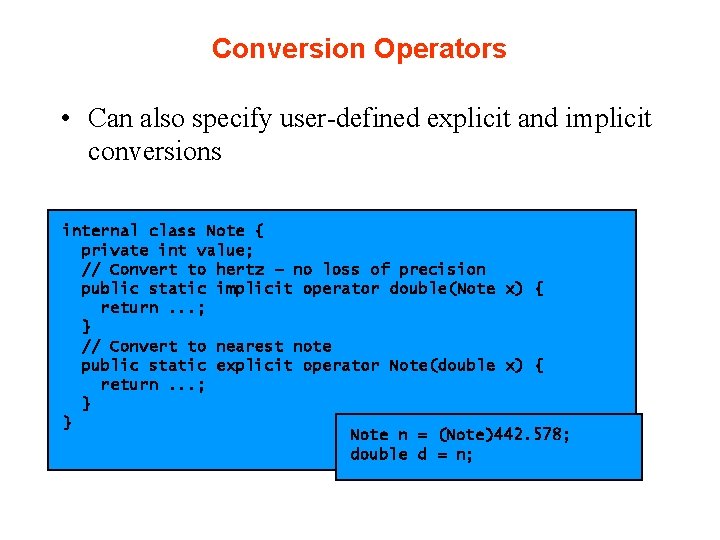
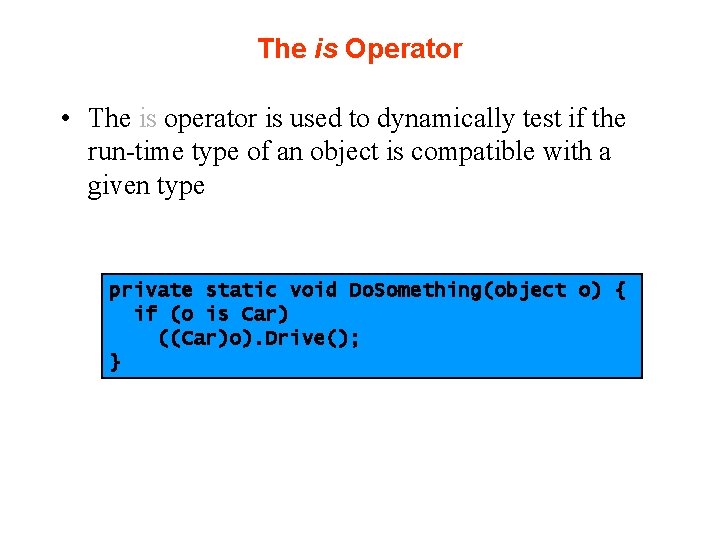
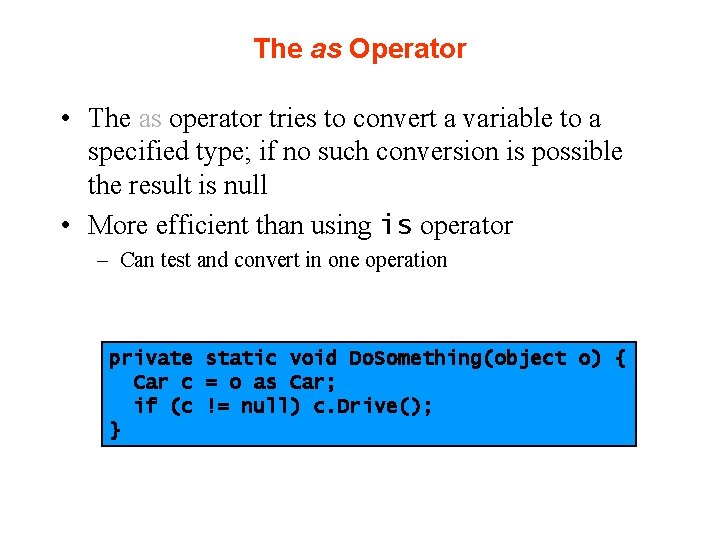
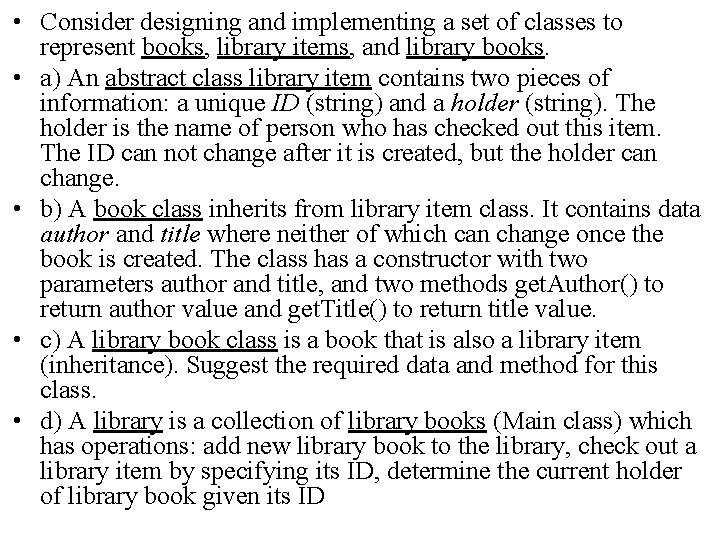
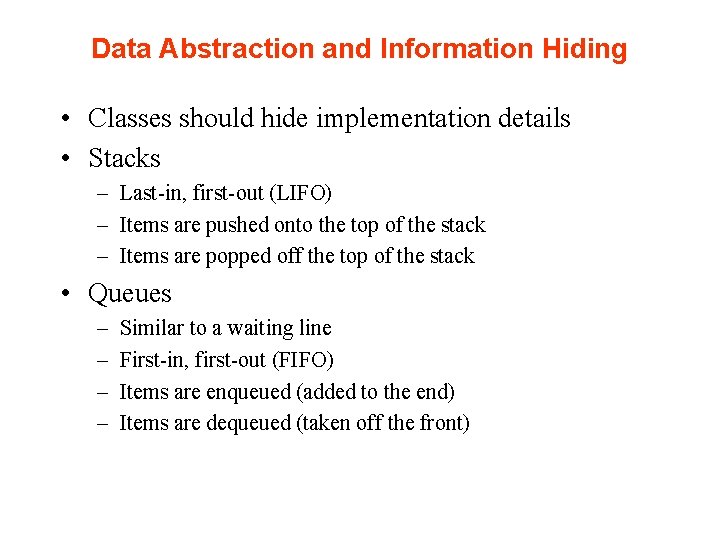
- Slides: 56
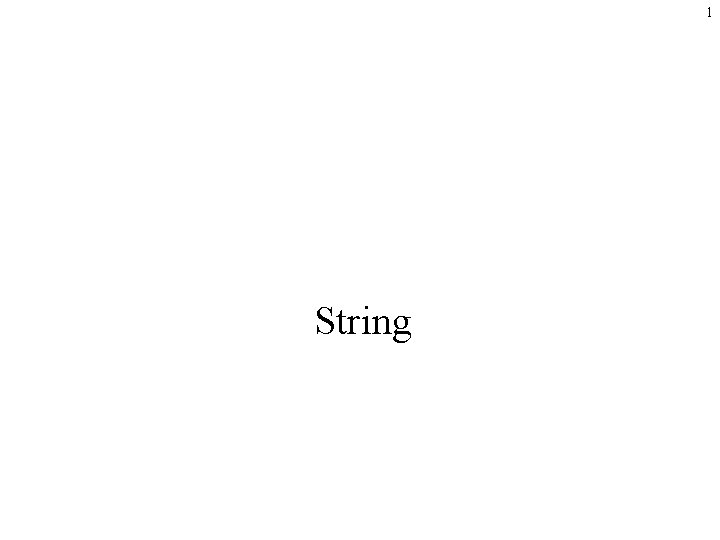
1 String
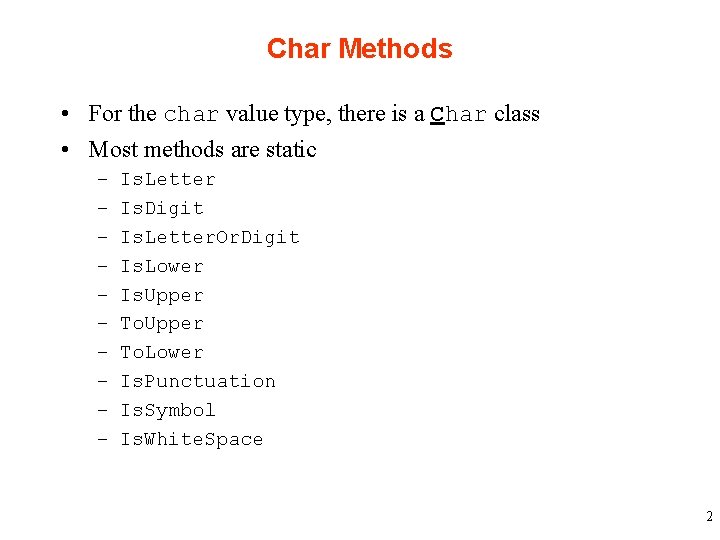
Char Methods • For the char value type, there is a Char class • Most methods are static – – – – – Is. Letter Is. Digit Is. Letter. Or. Digit Is. Lower Is. Upper To. Lower Is. Punctuation Is. Symbol Is. White. Space 2
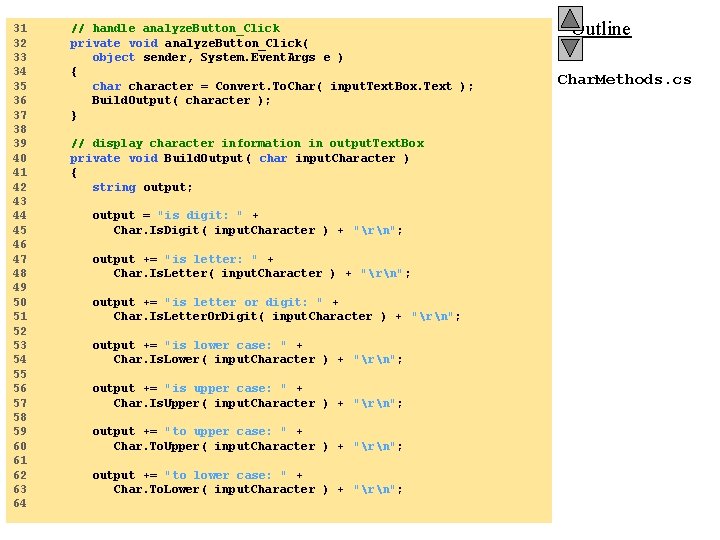
31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 // handle analyze. Button_Click private void analyze. Button_Click( object sender, System. Event. Args e ) { character = Convert. To. Char( input. Text. Box. Text ); Build. Output( character ); } // display character information in output. Text. Box private void Build. Output( char input. Character ) { string output; output = "is digit: " + Char. Is. Digit( input. Character ) + "rn"; output += "is letter: " + Char. Is. Letter( input. Character ) + "rn"; output += "is letter or digit: " + Char. Is. Letter. Or. Digit( input. Character ) + "rn"; output += "is lower case: " + Char. Is. Lower( input. Character ) + "rn"; output += "is upper case: " + Char. Is. Upper( input. Character ) + "rn"; output += "to upper case: " + Char. To. Upper( input. Character ) + "rn"; output += "to lower case: " + Char. To. Lower( input. Character ) + "rn"; Outline Char. Methods. cs
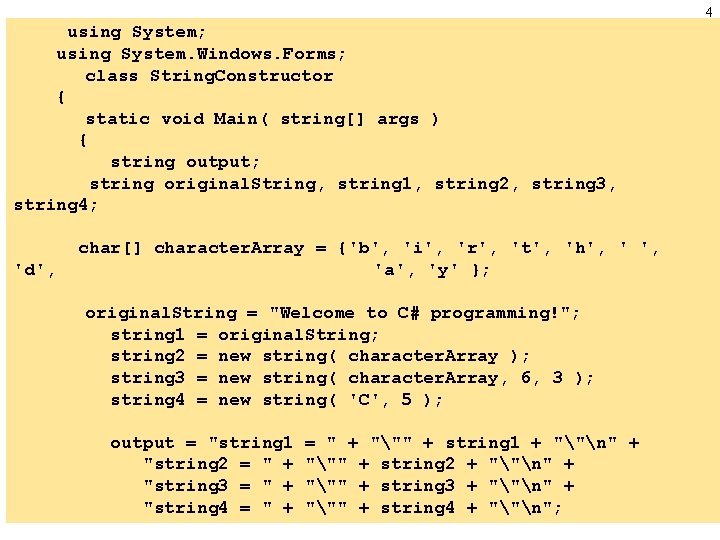
4 using System; using System. Windows. Forms; class String. Constructor { static void Main( string[] args ) { string output; string original. String, string 1, string 2, string 3, string 4; 'd', char[] character. Array = {'b', 'i', 'r', 't', 'h', 'a', 'y' }; String. Construct or. cs original. String = "Welcome to C# programming!"; string 1 = original. String; string 2 = new string( character. Array ); string 3 = new string( character. Array, 6, 3 ); string 4 = new string( 'C', 5 ); output = "string 1 "string 2 = " + "string 3 = " + "string 4 = " + """ + string 1 + ""n" + """ + string 2 + ""n" + """ + string 3 + ""n" + """ + string 4 + ""n";
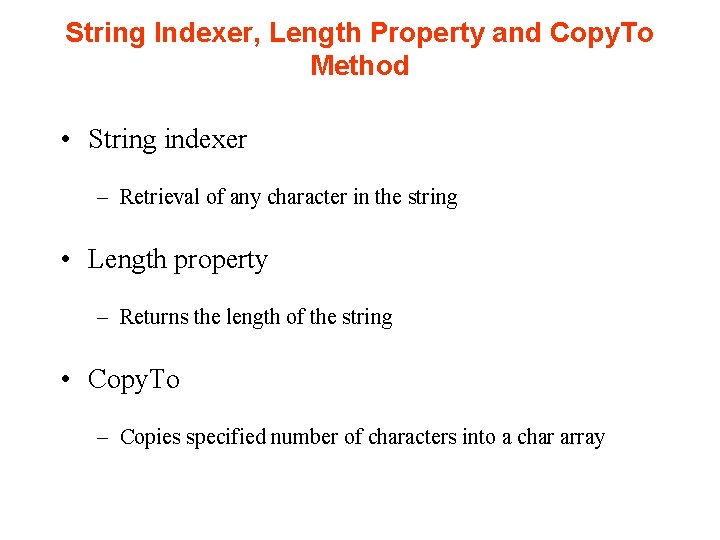
String Indexer, Length Property and Copy. To Method • String indexer – Retrieval of any character in the string • Length property – Returns the length of the string • Copy. To – Copies specified number of characters into a char array
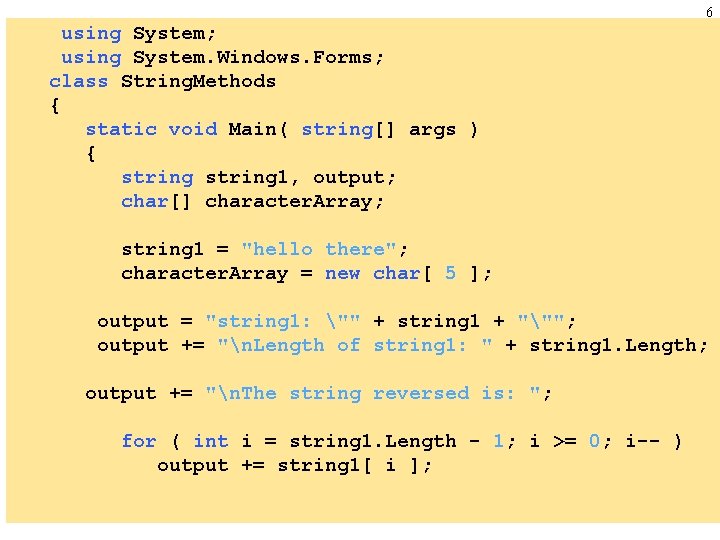
6 using System; using System. Windows. Forms; class String. Methods { static void Main( string[] args ) { string 1, output; char[] character. Array; string 1 = "hello there"; character. Array = new char[ 5 ]; String. Methods. c s output = "string 1: "" + string 1 + """; output += "n. Length of string 1: " + string 1. Length; output += "n. The string reversed is: "; for ( int i = string 1. Length - 1; i >= 0; i-- ) output += string 1[ i ];
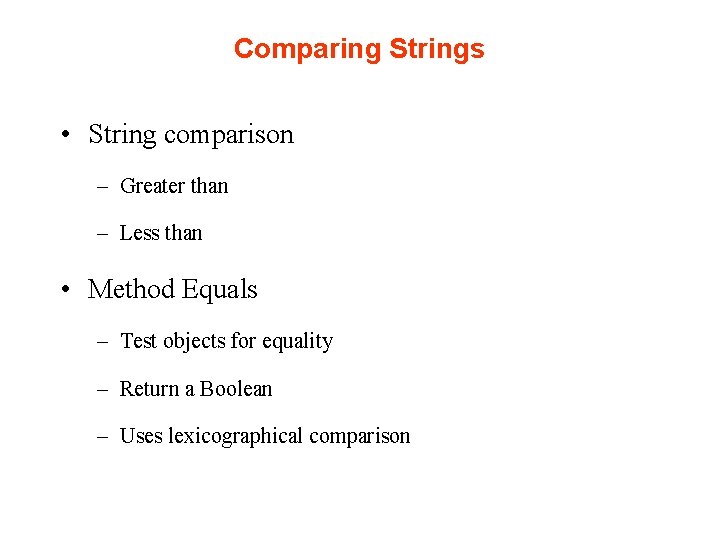
Comparing Strings • String comparison – Greater than – Less than • Method Equals – Test objects for equality – Return a Boolean – Uses lexicographical comparison
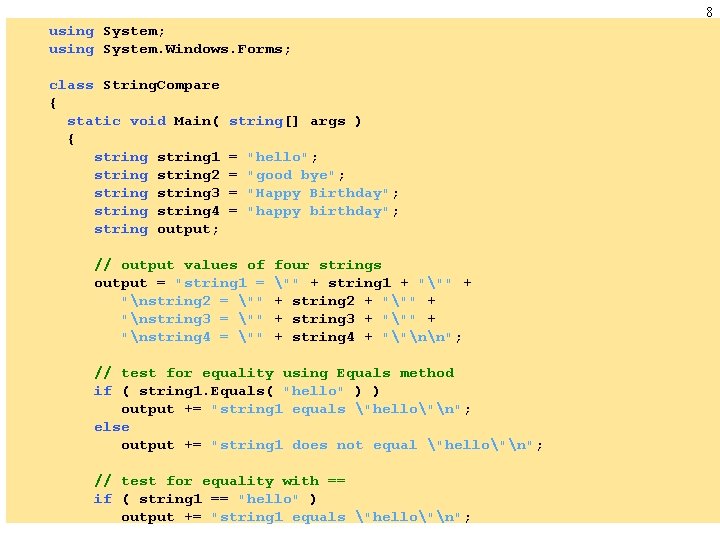
8 using System; using System. Windows. Forms; class String. Compare { static void Main( { string 1 string 2 string 3 string 4 string output; string[] args ) = = "hello"; "good bye"; "Happy Birthday"; "happy birthday"; // output values of output = "string 1 = "nstring 2 = "" "nstring 3 = "" "nstring 4 = "" four strings "" + string 1 + """ + + string 2 + """ + + string 3 + """ + + string 4 + ""nn"; // test for equality using Equals method if ( string 1. Equals( "hello" ) ) output += "string 1 equals "hello"n"; else output += "string 1 does not equal "hello"n"; // test for equality with == if ( string 1 == "hello" ) output += "string 1 equals "hello"n"; String. Compare. c s
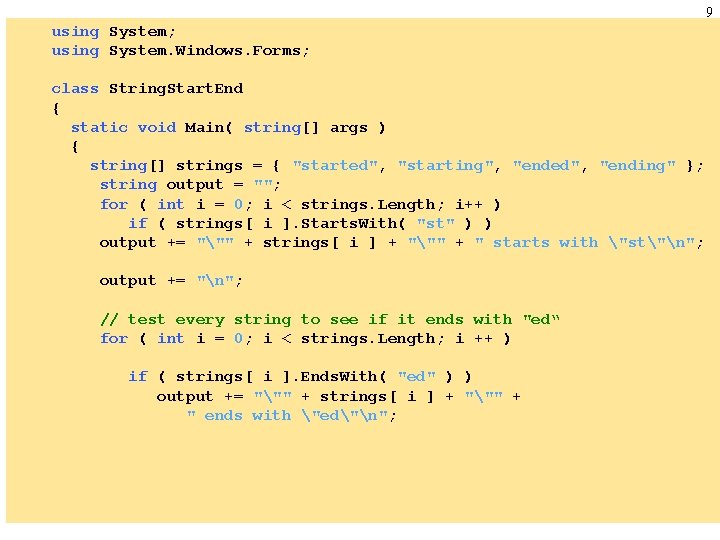
9 using System; using System. Windows. Forms; class String. Start. End { static void Main( string[] args ) { string[] strings = { "started", "starting", "ended", "ending" }; string output = ""; for ( int i = 0; i < strings. Length; i++ ) if ( strings[ i ]. Starts. With( "st" ) ) output += """ + strings[ i ] + """ + " starts with "st"n"; String. Start. End. output += "n"; cs // test every string to see if it ends with "ed“ for ( int i = 0; i < strings. Length; i ++ ) if ( strings[ i ]. Ends. With( "ed" ) ) output += """ + strings[ i ] + """ + " ends with "ed"n";
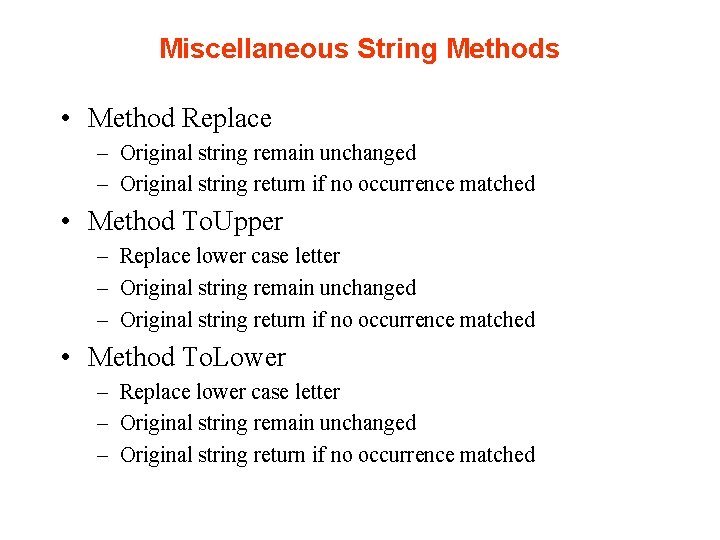
Miscellaneous String Methods • Method Replace – Original string remain unchanged – Original string return if no occurrence matched • Method To. Upper – Replace lower case letter – Original string remain unchanged – Original string return if no occurrence matched • Method To. Lower – Replace lower case letter – Original string remain unchanged – Original string return if no occurrence matched
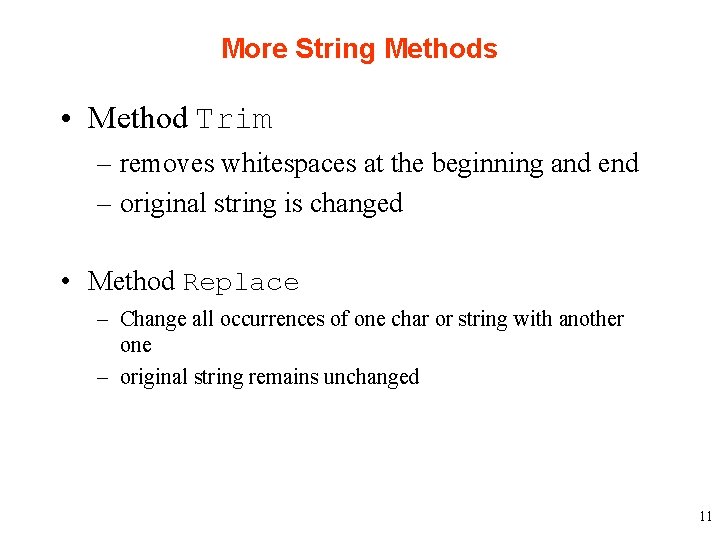
More String Methods • Method Trim – removes whitespaces at the beginning and end – original string is changed • Method Replace – Change all occurrences of one char or string with another one – original string remains unchanged 11
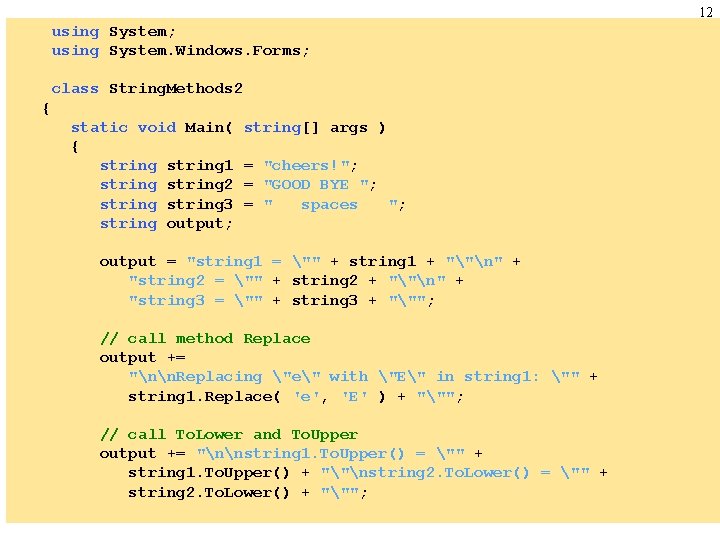
12 using System; using System. Windows. Forms; class String. Methods 2 { static void Main( { string 1 string 2 string 3 string output; string[] args ) = "cheers!"; = "GOOD BYE "; = " spaces "; output = "string 1 = "" + string 1 + ""n" + "string 2 = "" + string 2 + ""n" + "string 3 = "" + string 3 + """; String. Miscellan eous 2. cs // call method Replace output += "nn. Replacing "e" with "E" in string 1: "" + string 1. Replace( 'e', 'E' ) + """; // call To. Lower and To. Upper output += "nnstring 1. To. Upper() = "" + string 1. To. Upper() + ""nstring 2. To. Lower() = "" + string 2. To. Lower() + """;
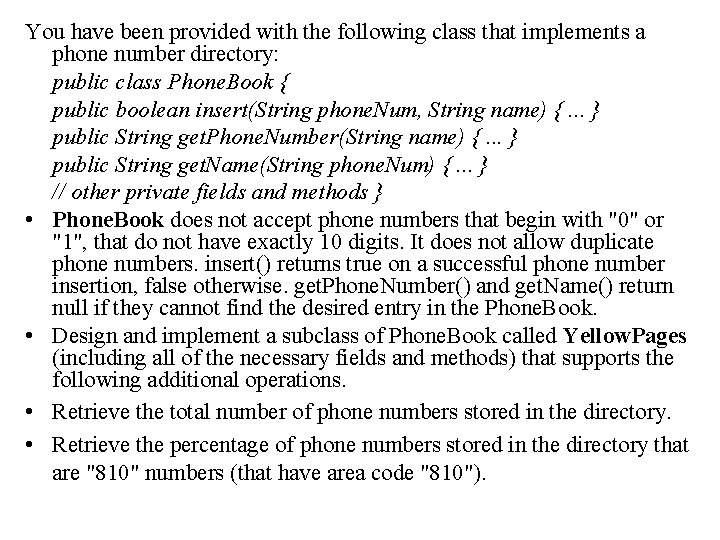
You have been provided with the following class that implements a phone number directory: public class Phone. Book { public boolean insert(String phone. Num, String name) {. . . } public String get. Phone. Number(String name) {. . . } public String get. Name(String phone. Num) {. . . } // other private fields and methods } • Phone. Book does not accept phone numbers that begin with "0" or "1", that do not have exactly 10 digits. It does not allow duplicate phone numbers. insert() returns true on a successful phone number insertion, false otherwise. get. Phone. Number() and get. Name() return null if they cannot find the desired entry in the Phone. Book. • Design and implement a subclass of Phone. Book called Yellow. Pages (including all of the necessary fields and methods) that supports the following additional operations. • Retrieve the total number of phone numbers stored in the directory. • Retrieve the percentage of phone numbers stored in the directory that are "810" numbers (that have area code "810").
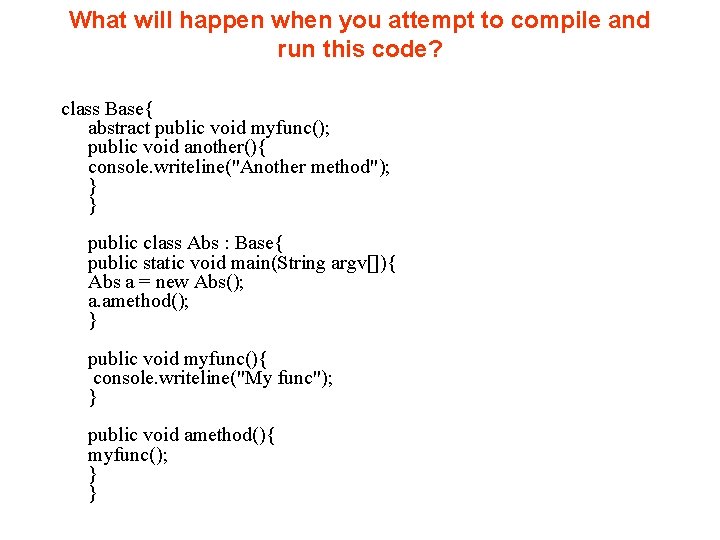
What will happen when you attempt to compile and run this code? class Base{ abstract public void myfunc(); public void another(){ console. writeline("Another method"); } } public class Abs : Base{ public static void main(String argv[]){ Abs a = new Abs(); a. amethod(); } public void myfunc(){ console. writeline("My func"); } public void amethod(){ myfunc(); } }
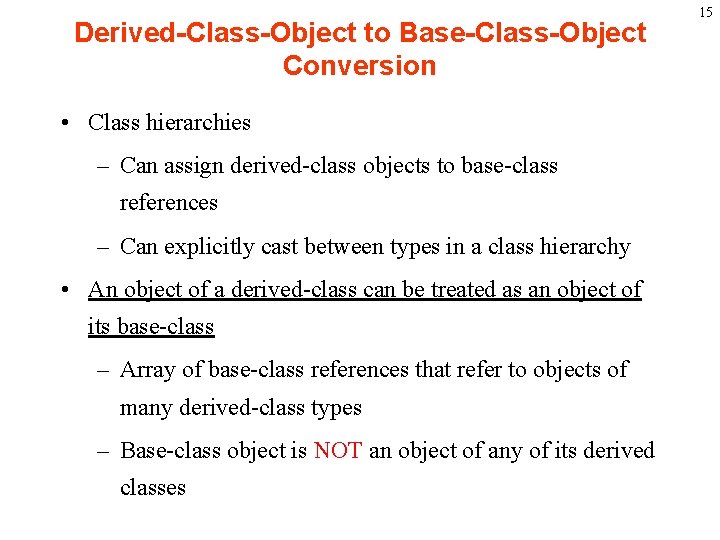
Derived-Class-Object to Base-Class-Object Conversion • Class hierarchies – Can assign derived-class objects to base-class references – Can explicitly cast between types in a class hierarchy • An object of a derived-class can be treated as an object of its base-class – Array of base-class references that refer to objects of many derived-class types – Base-class object is NOT an object of any of its derived classes 15
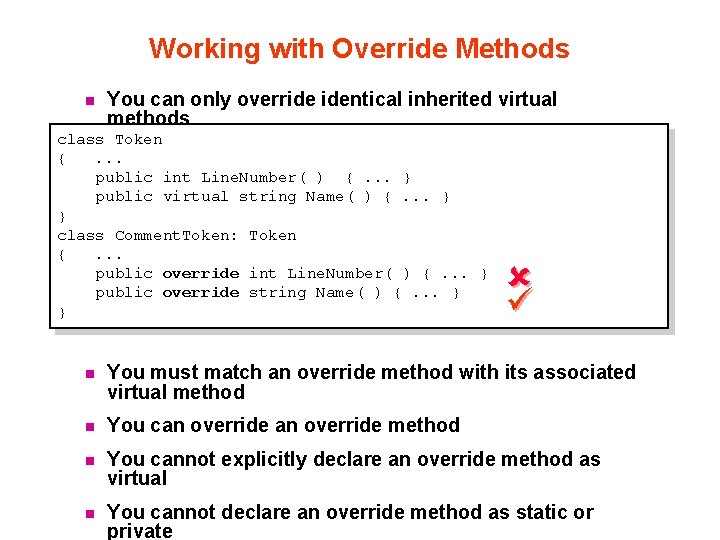
Working with Override Methods n You can only override identical inherited virtual methods class Token {. . . public int Line. Number( ) {. . . } public virtual string Name( ) {. . . } } class Comment. Token: Token {. . . public override int Line. Number( ) {. . . } public override string Name( ) {. . . } } û n You must match an override method with its associated virtual method n You can override method n You cannot explicitly declare an override method as virtual n You cannot declare an override method as static or private
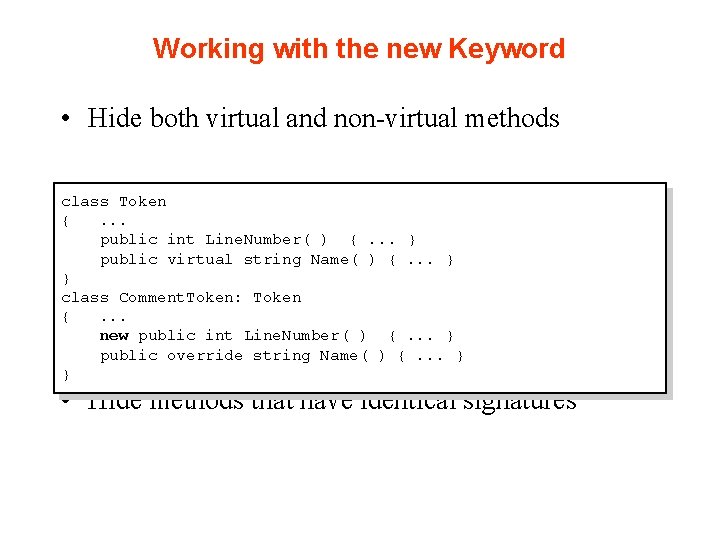
Working with the new Keyword • Hide both virtual and non-virtual methods class Token {. . . public int Line. Number( ) {. . . } public virtual string Name( ) {. . . } } class Comment. Token: Token {. . . new public int Line. Number( ) {. . . } public override string Name( ) {. . . } } • Resolve name clashes in code • Hide methods that have identical signatures
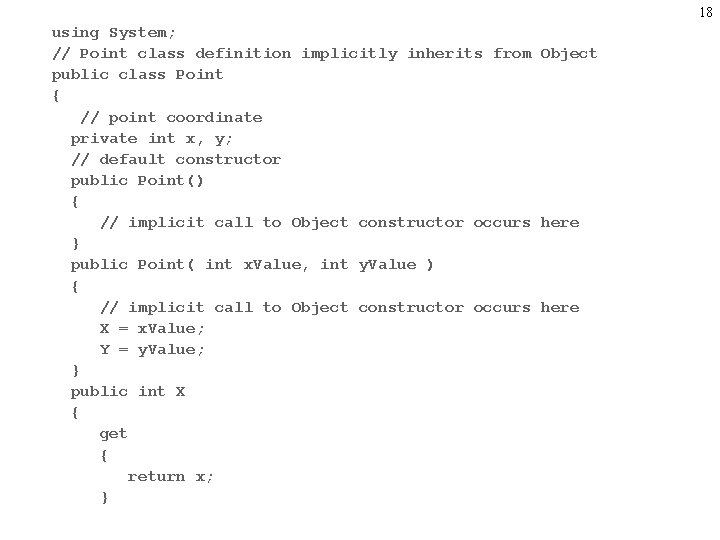
18 using System; // Point class definition implicitly inherits from Object public class Point { // point coordinate private int x, y; // default constructor public Point() { // implicit call to Object constructor occurs here } public Point( int x. Value, int y. Value ) { // implicit call to Object constructor occurs here X = x. Value; Y = y. Value; } public int X { get { return x; }
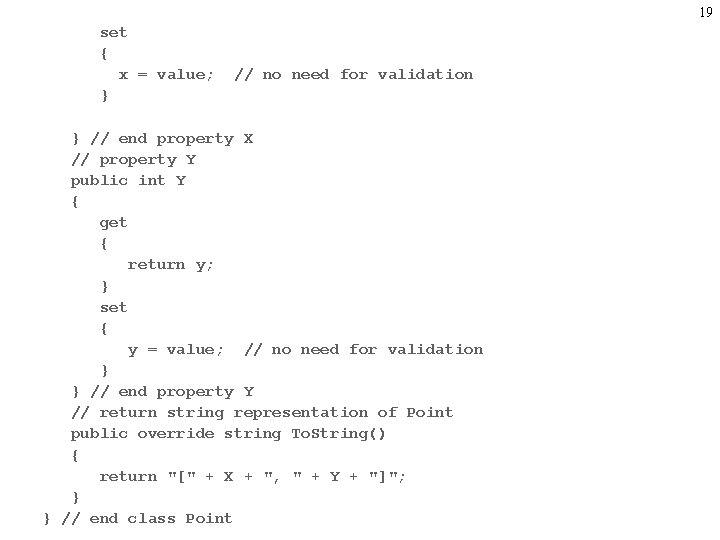
19 set { x = value; } // no need for validation } // end property X // property Y public int Y { get { return y; } set { y = value; // no need for validation } } // end property Y // return string representation of Point public override string To. String() { return "[" + X + ", " + Y + "]"; } } // end class Point
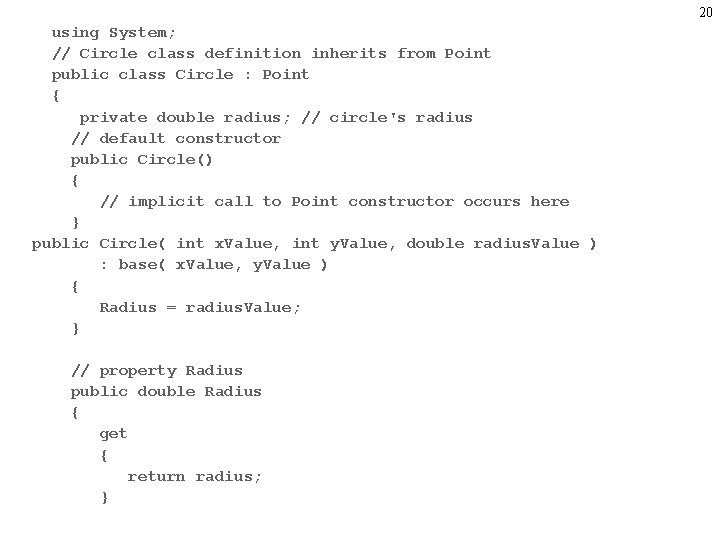
20 using System; // Circle class definition inherits from Point public class Circle : Point { private double radius; // circle's radius // default constructor public Circle() { // implicit call to Point constructor occurs here } public Circle( int x. Value, int y. Value, double radius. Value ) : base( x. Value, y. Value ) { Radius = radius. Value; } // property Radius public double Radius { get { return radius; }
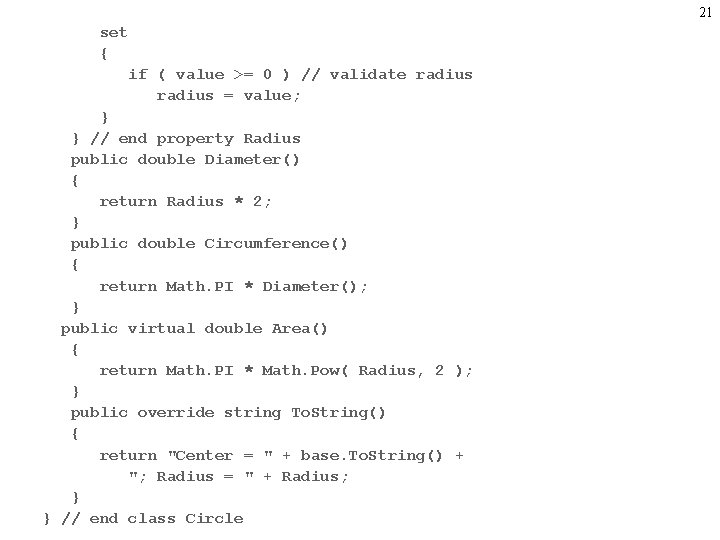
21 set { if ( value >= 0 ) // validate radius = value; } } // end property Radius public double Diameter() { return Radius * 2; } public double Circumference() { return Math. PI * Diameter(); } public virtual double Area() { return Math. PI * Math. Pow( Radius, 2 ); } public override string To. String() { return "Center = " + base. To. String() + "; Radius = " + Radius; } } // end class Circle
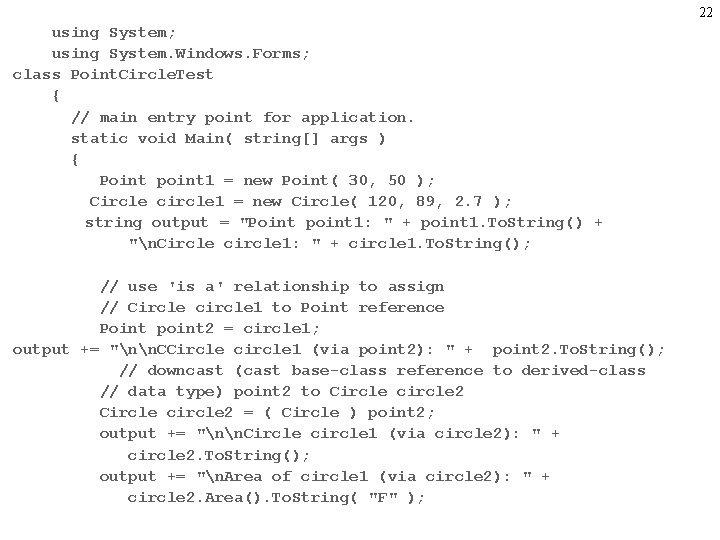
22 using System; using System. Windows. Forms; class Point. Circle. Test { // main entry point for application. static void Main( string[] args ) { Point point 1 = new Point( 30, 50 ); Circle circle 1 = new Circle( 120, 89, 2. 7 ); string output = "Point point 1: " + point 1. To. String() + "n. Circle circle 1: " + circle 1. To. String(); // use 'is a' relationship to assign // Circle circle 1 to Point reference Point point 2 = circle 1; output += "nn. CCircle circle 1 (via point 2): " + point 2. To. String(); // downcast (cast base-class reference to derived-class // data type) point 2 to Circle circle 2 = ( Circle ) point 2; output += "nn. Circle circle 1 (via circle 2): " + circle 2. To. String(); output += "n. Area of circle 1 (via circle 2): " + circle 2. Area(). To. String( "F" );
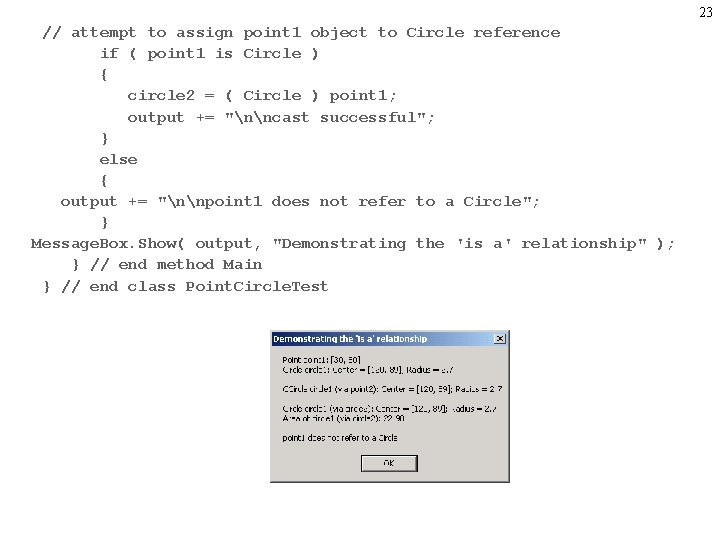
23 // attempt to assign point 1 object to Circle reference if ( point 1 is Circle ) { circle 2 = ( Circle ) point 1; output += "nncast successful"; } else { output += "nnpoint 1 does not refer to a Circle"; } Message. Box. Show( output, "Demonstrating the 'is a' relationship" ); } // end method Main } // end class Point. Circle. Test
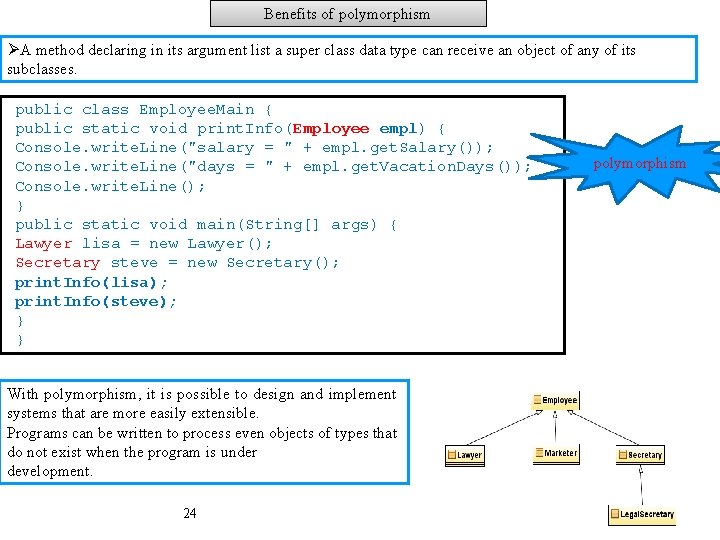
Benefits of polymorphism ØA method declaring in its argument list a super class data type can receive an object of any of its subclasses. public class Employee. Main { public static void print. Info(Employee empl) { Console. write. Line("salary = " + empl. get. Salary()); Console. write. Line("days = " + empl. get. Vacation. Days()); Console. write. Line(); } public static void main(String[] args) { Lawyer lisa = new Lawyer(); Secretary steve = new Secretary(); print. Info(lisa); print. Info(steve); } } With polymorphism, it is possible to design and implement systems that are more easily extensible. Programs can be written to process even objects of types that do not exist when the program is under development. 24 polymorphism
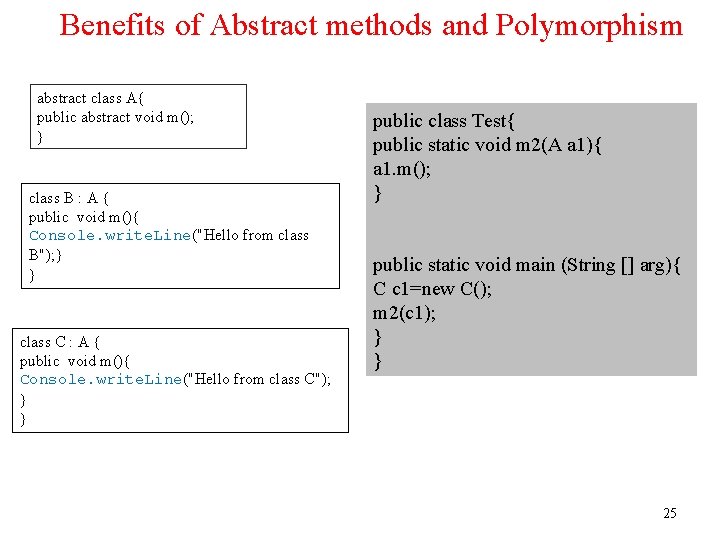
Benefits of Abstract methods and Polymorphism abstract class A{ public abstract void m(); } class B : A { public void m(){ Console. write. Line("Hello from class B"); } } class C : A { public void m(){ Console. write. Line("Hello from class C"); } } public class Test{ public static void m 2(A a 1){ a 1. m(); } public static void main (String [] arg){ C c 1=new C(); m 2(c 1); } } 25
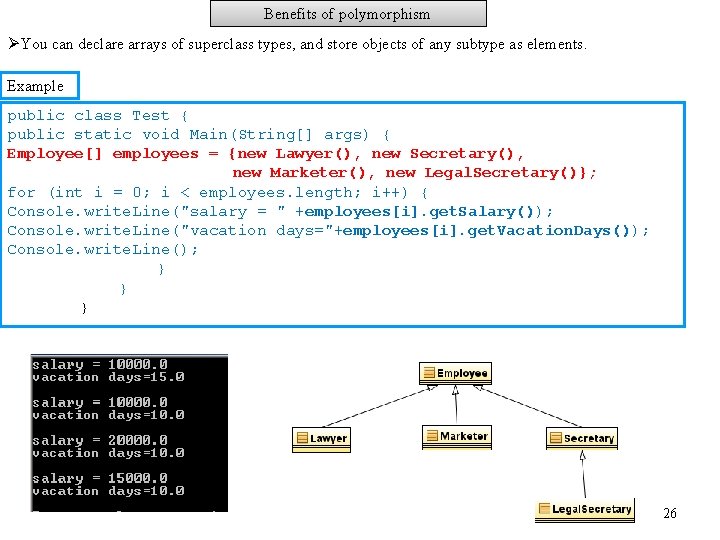
Benefits of polymorphism ØYou can declare arrays of superclass types, and store objects of any subtype as elements. Example public class Test { public static void Main(String[] args) { Employee[] employees = {new Lawyer(), new Secretary(), new Marketer(), new Legal. Secretary()}; for (int i = 0; i < employees. length; i++) { Console. write. Line("salary = " +employees[i]. get. Salary()); Console. write. Line("vacation days="+employees[i]. get. Vacation. Days()); Console. write. Line(); } } } 26
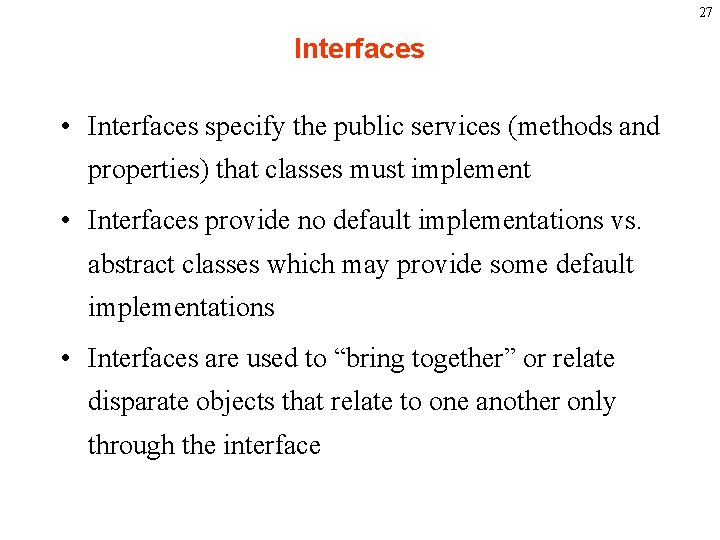
27 Interfaces • Interfaces specify the public services (methods and properties) that classes must implement • Interfaces provide no default implementations vs. abstract classes which may provide some default implementations • Interfaces are used to “bring together” or relate disparate objects that relate to one another only through the interface
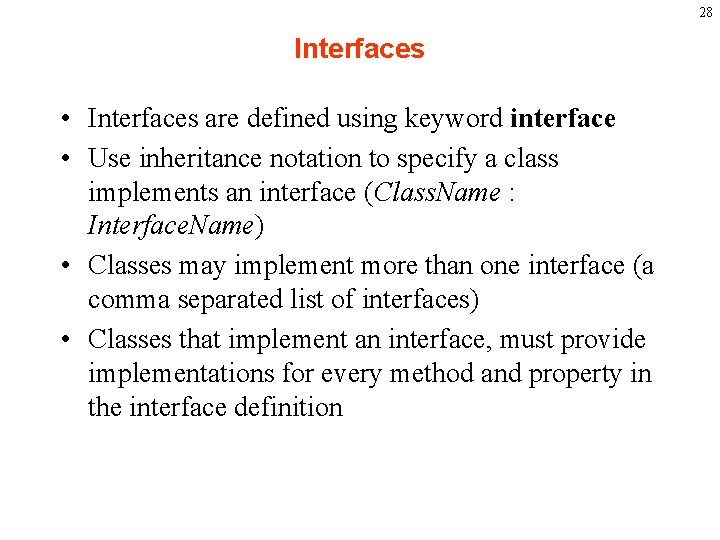
28 Interfaces • Interfaces are defined using keyword interface • Use inheritance notation to specify a class implements an interface (Class. Name : Interface. Name) • Classes may implement more than one interface (a comma separated list of interfaces) • Classes that implement an interface, must provide implementations for every method and property in the interface definition
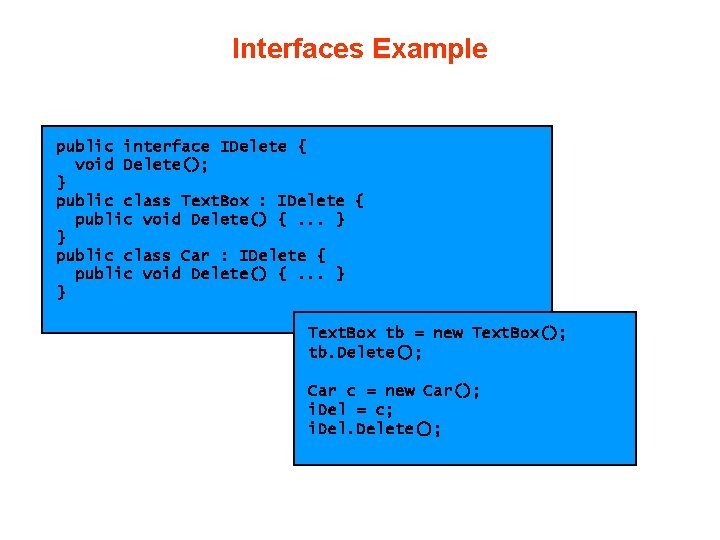
Interfaces Example public interface IDelete { void Delete(); } public class Text. Box : IDelete { public void Delete() {. . . } } public class Car : IDelete { public void Delete() {. . . } } Text. Box tb = new Text. Box(); tb. Delete(); Car c = new Car(); i. Del = c; i. Delete();
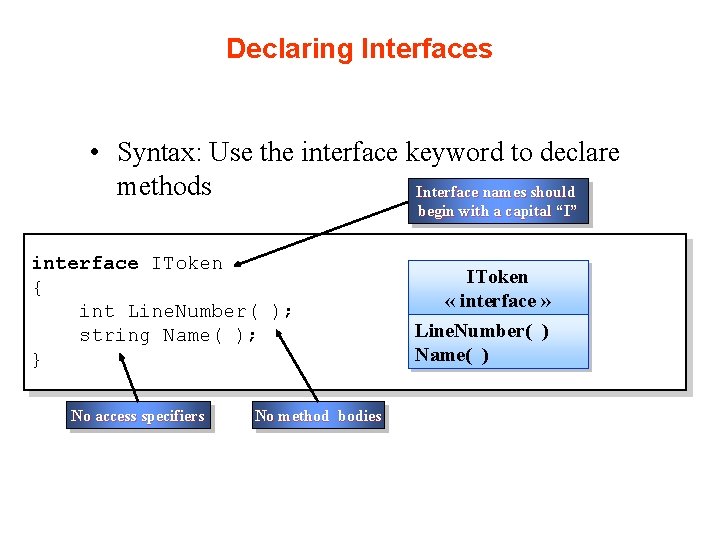
Declaring Interfaces • Syntax: Use the interface keyword to declare methods Interface names should begin with a capital “I” interface IToken { int Line. Number( ); string Name( ); } No access specifiers No method bodies IToken « interface » Line. Number( ) Name( )
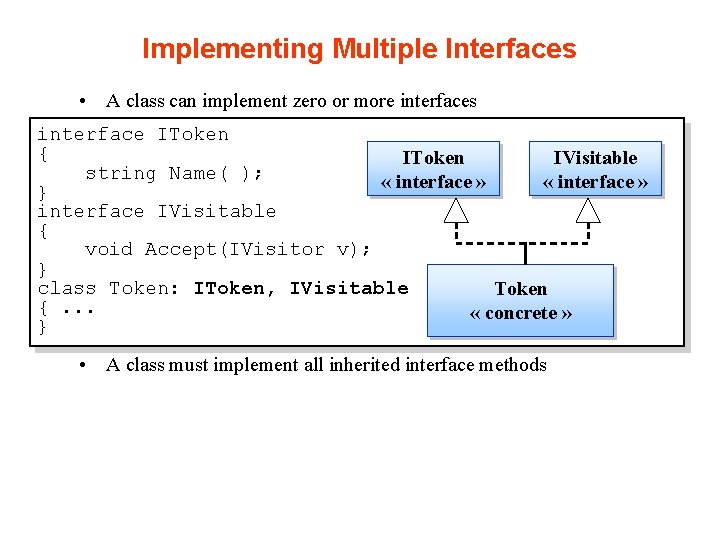
Implementing Multiple Interfaces • A class can implement zero or more interfaces interface IToken { IToken IVisitable string Name( ); « interface » } interface IVisitable { void Accept(IVisitor v); } class Token: IToken, • An interface can extend. IVisitable zero or more interfaces Token {. . . « concrete » • A class can be more accessible than its base interfaces } • An interface cannot be more accessible than its base interfaces • A class must implement all inherited interface methods
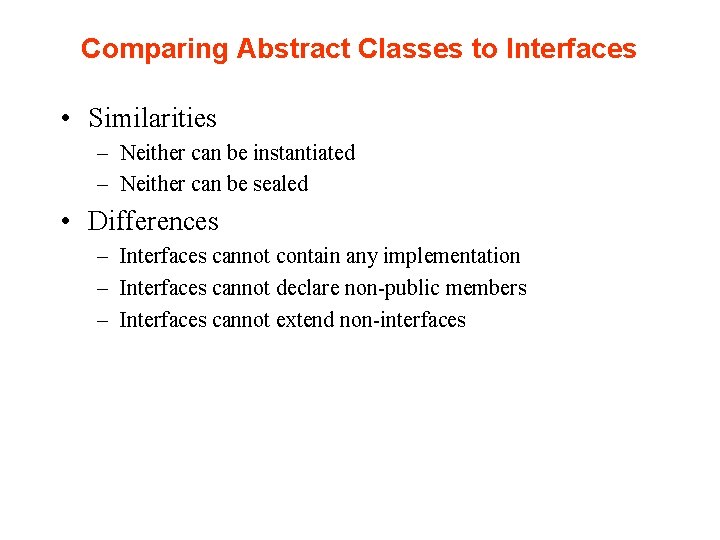
Comparing Abstract Classes to Interfaces • Similarities – Neither can be instantiated – Neither can be sealed • Differences – Interfaces cannot contain any implementation – Interfaces cannot declare non-public members – Interfaces cannot extend non-interfaces
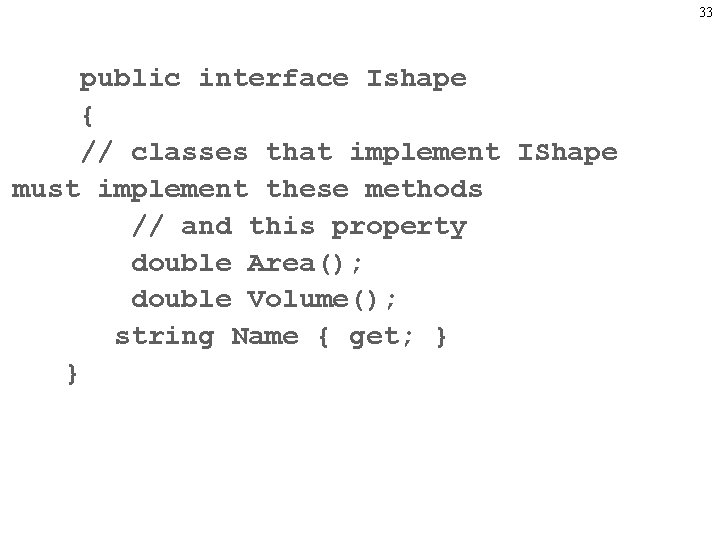
33 public interface Ishape { // classes that implement IShape must implement these methods // and this property double Area(); double Volume(); string Name { get; } }
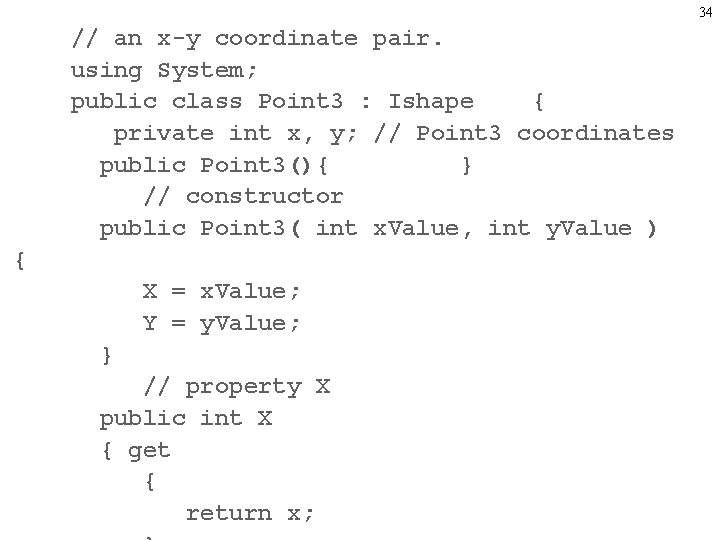
34 // an x-y coordinate pair. using System; public class Point 3 : Ishape { private int x, y; // Point 3 coordinates public Point 3(){ } // constructor public Point 3( int x. Value, int y. Value ) { X = x. Value; Y = y. Value; } // property X public int X { get { return x;
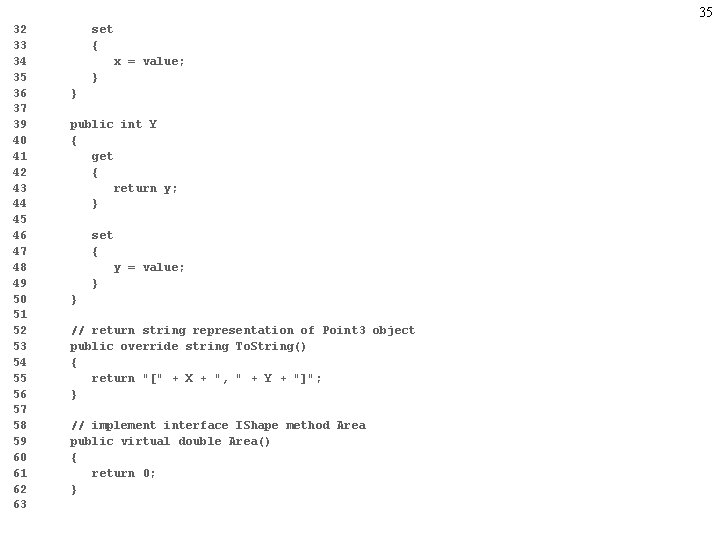
35 32 33 34 35 36 37 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 set { x = value; } } public int Y { get { return y; } set { y = value; } } // return string representation of Point 3 object public override string To. String() { return "[" + X + ", " + Y + "]"; } // implement interface IShape method Area public virtual double Area() { return 0; }
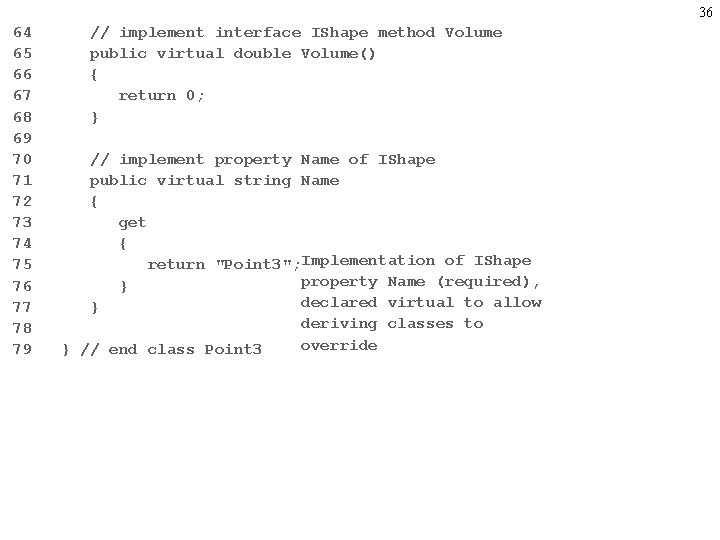
36 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 // implement interface IShape method Volume public virtual double Volume() { return 0; } // implement property Name of IShape public virtual string Name { get { return "Point 3"; Implementation of IShape property Name (required), } declared virtual to allow } deriving classes to override } // end class Point 3
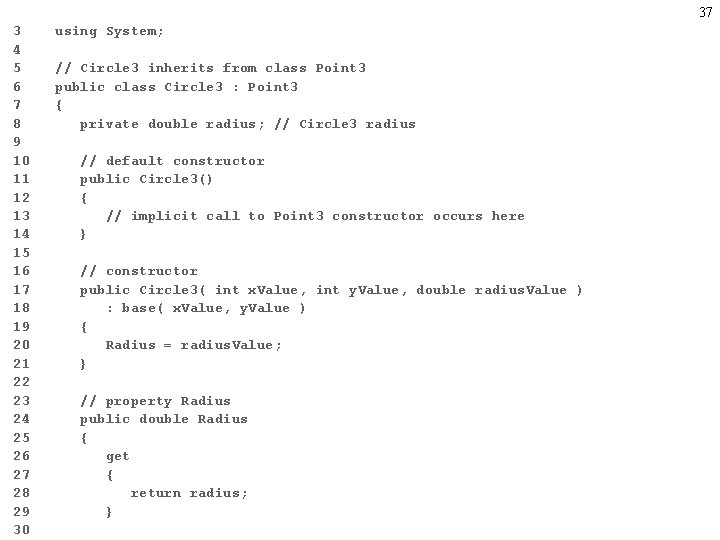
37 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 using System; // Circle 3 inherits from class Point 3 public class Circle 3 : Point 3 { private double radius; // Circle 3 radius // default constructor public Circle 3() { // implicit call to Point 3 constructor occurs here } // constructor public Circle 3( int x. Value, int y. Value, double radius. Value ) : base( x. Value, y. Value ) { Radius = radius. Value; } // property Radius public double Radius { get { return radius; }
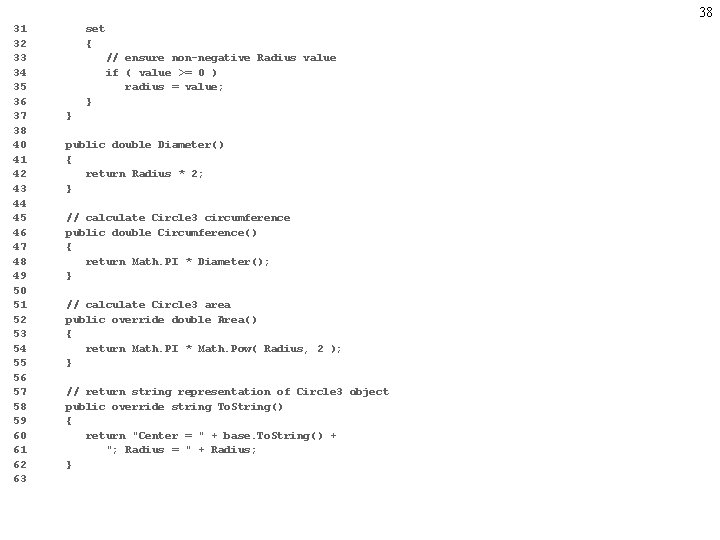
38 31 32 33 34 35 36 37 38 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 set { // ensure non-negative Radius value if ( value >= 0 ) radius = value; } } public double Diameter() { return Radius * 2; } // calculate Circle 3 circumference public double Circumference() { return Math. PI * Diameter(); } // calculate Circle 3 area public override double Area() { return Math. PI * Math. Pow( Radius, 2 ); } // return string representation of Circle 3 object public override string To. String() { return "Center = " + base. To. String() + "; Radius = " + Radius; }
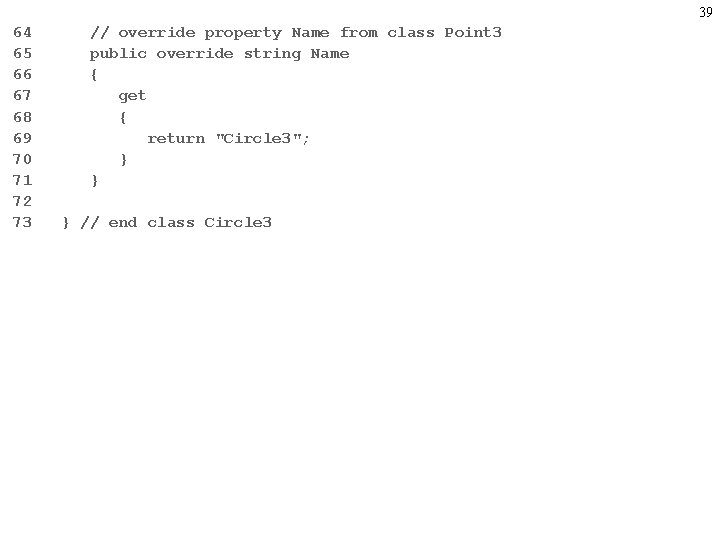
39 64 65 66 67 68 69 70 71 72 73 // override property Name from class Point 3 public override string Name { get { return "Circle 3"; } } } // end class Circle 3
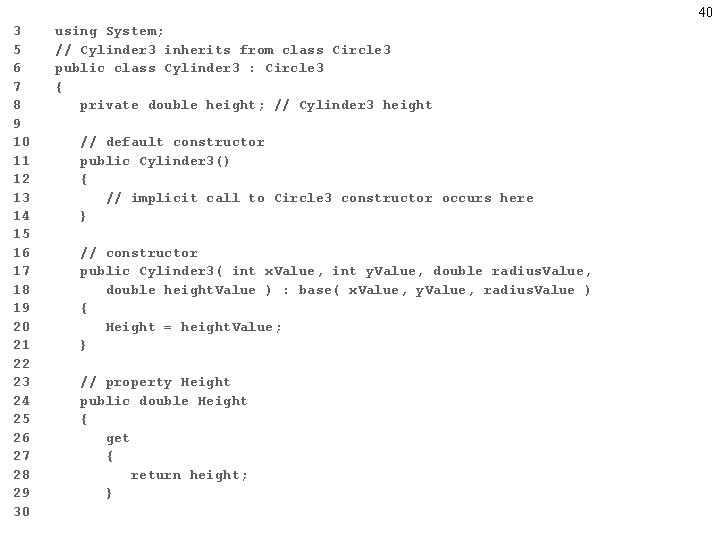
40 3 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 using System; // Cylinder 3 inherits from class Circle 3 public class Cylinder 3 : Circle 3 { private double height; // Cylinder 3 height // default constructor public Cylinder 3() { // implicit call to Circle 3 constructor occurs here } // constructor public Cylinder 3( int x. Value, int y. Value, double radius. Value, double height. Value ) : base( x. Value, y. Value, radius. Value ) { Height = height. Value; } // property Height public double Height { get { return height; }
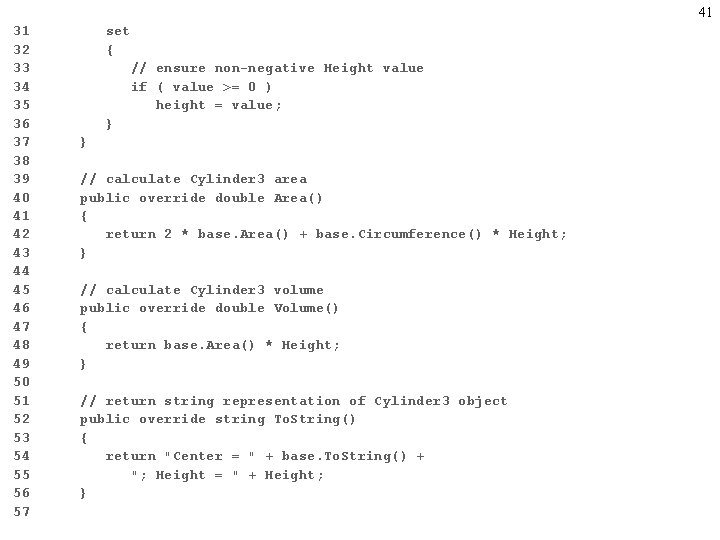
41 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 set { // ensure non-negative Height value if ( value >= 0 ) height = value; } } // calculate Cylinder 3 area public override double Area() { return 2 * base. Area() + base. Circumference() * Height; } // calculate Cylinder 3 volume public override double Volume() { return base. Area() * Height; } // return string representation of Cylinder 3 object public override string To. String() { return "Center = " + base. To. String() + "; Height = " + Height; }
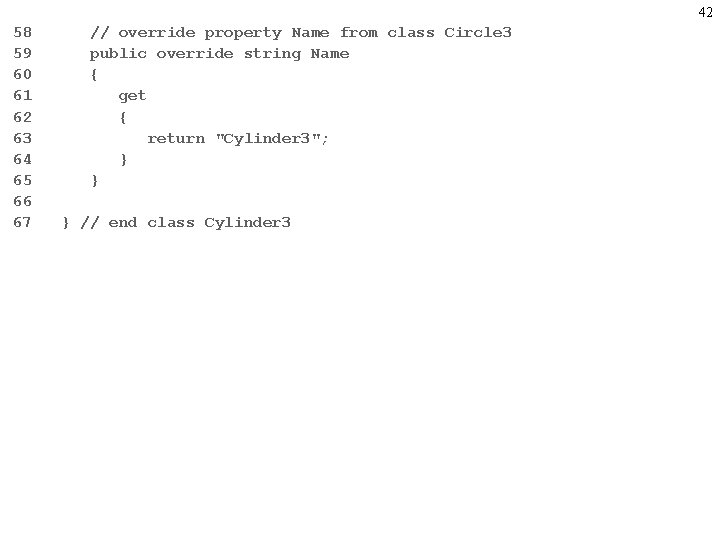
42 58 59 60 61 62 63 64 65 66 67 // override property Name from class Circle 3 public override string Name { get { return "Cylinder 3"; } } } // end class Cylinder 3
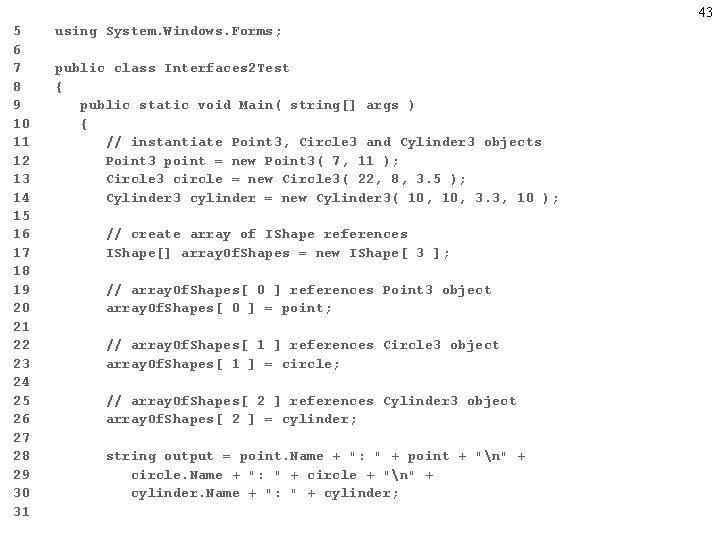
43 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 using System. Windows. Forms; public class Interfaces 2 Test { public static void Main( string[] args ) { // instantiate Point 3, Circle 3 and Cylinder 3 objects Point 3 point = new Point 3( 7, 11 ); Circle 3 circle = new Circle 3( 22, 8, 3. 5 ); Cylinder 3 cylinder = new Cylinder 3( 10, 3. 3, 10 ); // create array of IShape references IShape[] array. Of. Shapes = new IShape[ 3 ]; // array. Of. Shapes[ 0 ] references Point 3 object array. Of. Shapes[ 0 ] = point; // array. Of. Shapes[ 1 ] references Circle 3 object array. Of. Shapes[ 1 ] = circle; // array. Of. Shapes[ 2 ] references Cylinder 3 object array. Of. Shapes[ 2 ] = cylinder; string output = point. Name + ": " + point + "n" + circle. Name + ": " + circle + "n" + cylinder. Name + ": " + cylinder;
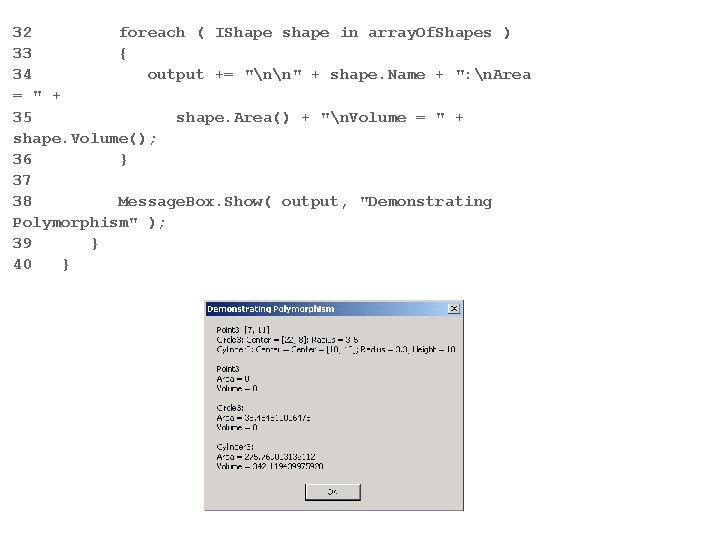
32 foreach ( IShape shape in array. Of. Shapes ) 33 { 34 output += "nn" + shape. Name + ": n. Area = " + 35 shape. Area() + "n. Volume = " + shape. Volume(); 36 } 37 38 Message. Box. Show( output, "Demonstrating Polymorphism" ); 39 } 40 }
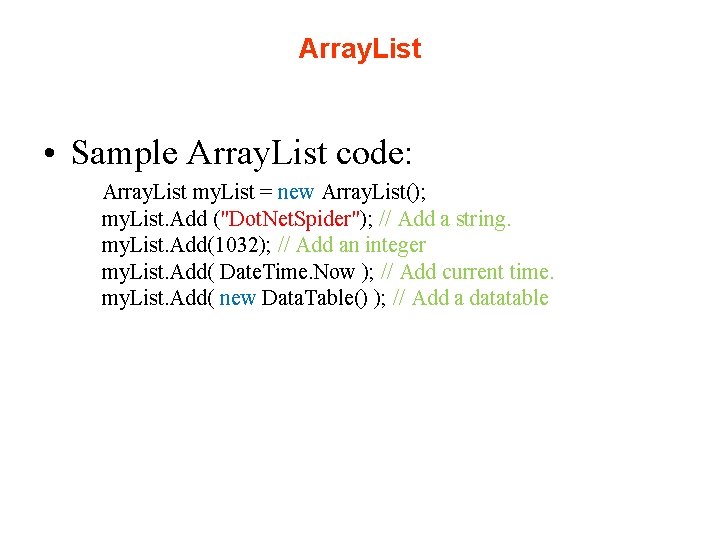
Array. List • Sample Array. List code: Array. List my. List = new Array. List(); my. List. Add ("Dot. Net. Spider"); // Add a string. my. List. Add(1032); // Add an integer my. List. Add( Date. Time. Now ); // Add current time. my. List. Add( new Data. Table() ); // Add a datatable
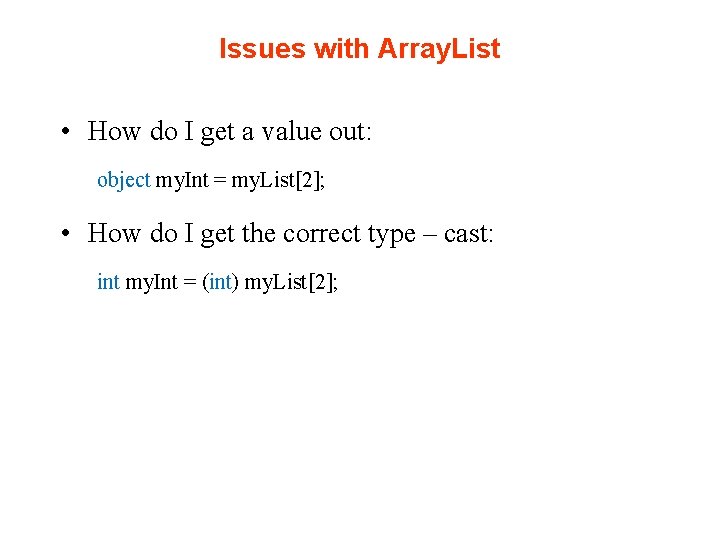
Issues with Array. List • How do I get a value out: object my. Int = my. List[2]; • How do I get the correct type – cast: int my. Int = (int) my. List[2];
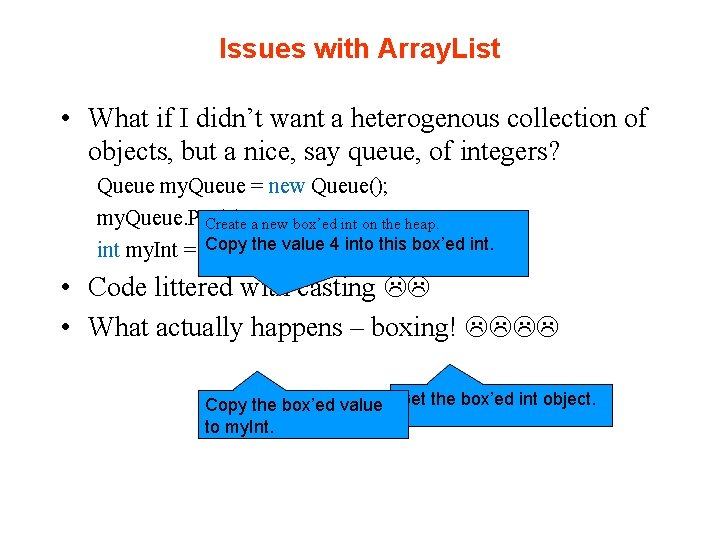
Issues with Array. List • What if I didn’t want a heterogenous collection of objects, but a nice, say queue, of integers? Queue my. Queue = new Queue(); my. Queue. Put(4); Create a new box’ed int on the heap. Copymy. Queue. Get(); the value 4 into this box’ed int my. Int = (int) • Code littered with casting • What actually happens – boxing! Copy the box’ed value Get the box’ed int object. to my. Int.
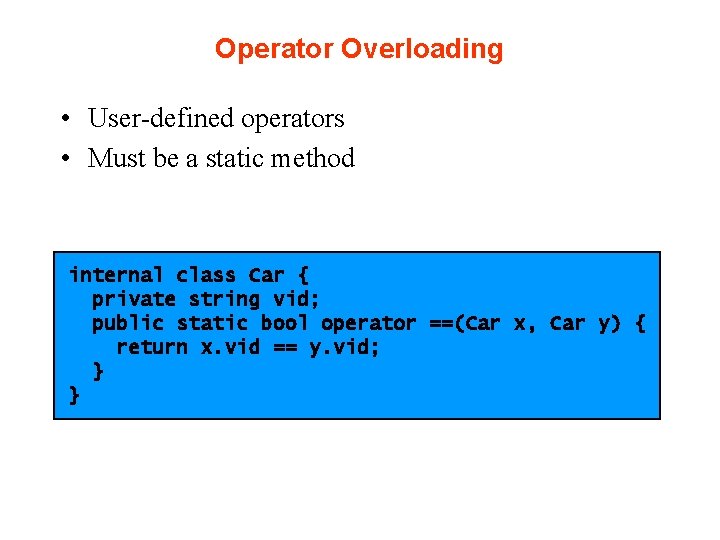
Operator Overloading • User-defined operators • Must be a static method internal class Car { private string vid; public static bool operator ==(Car x, Car y) { return x. vid == y. vid; } }
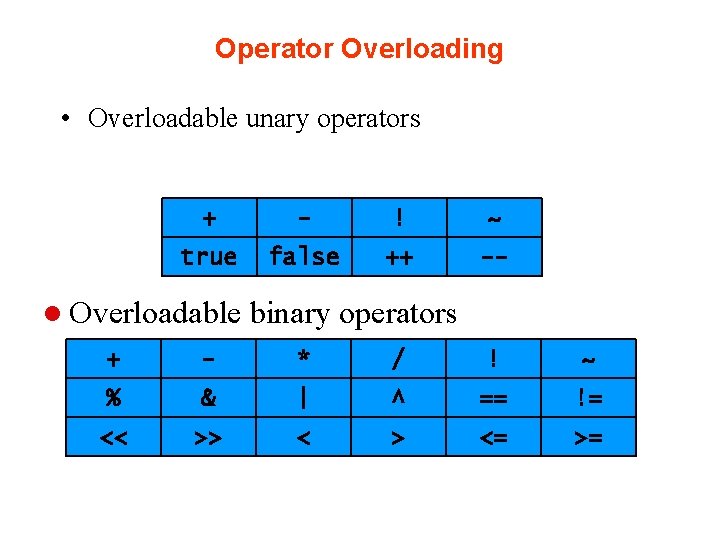
Operator Overloading • Overloadable unary operators + - ! ~ true false ++ -- l Overloadable binary operators + - * / ! ~ % & | ^ == != << >> < > <= >=
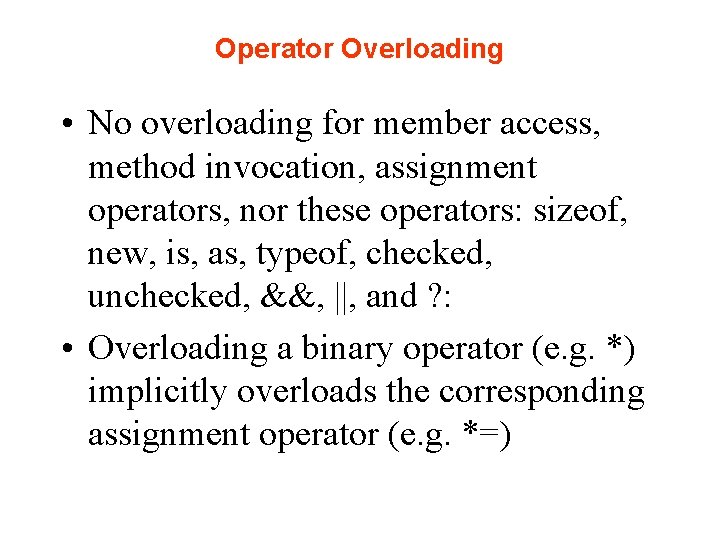
Operator Overloading • No overloading for member access, method invocation, assignment operators, nor these operators: sizeof, new, is, as, typeof, checked, unchecked, &&, ||, and ? : • Overloading a binary operator (e. g. *) implicitly overloads the corresponding assignment operator (e. g. *=)
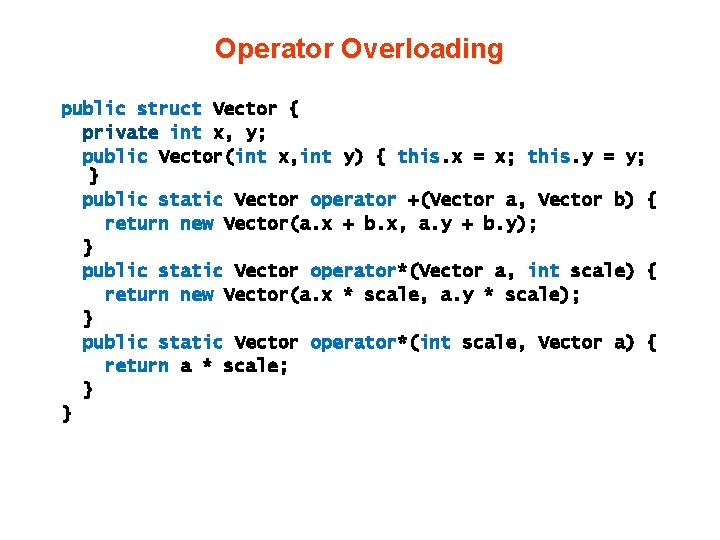
Operator Overloading public struct Vector { private int x, y; public Vector(int x, int y) { this. x = x; this. y = y; } public static Vector operator +(Vector a, Vector b) { return new Vector(a. x + b. x, a. y + b. y); } public static Vector operator*(Vector a, int scale) { return new Vector(a. x * scale, a. y * scale); } public static Vector operator*(int scale, Vector a) { return a * scale; } }
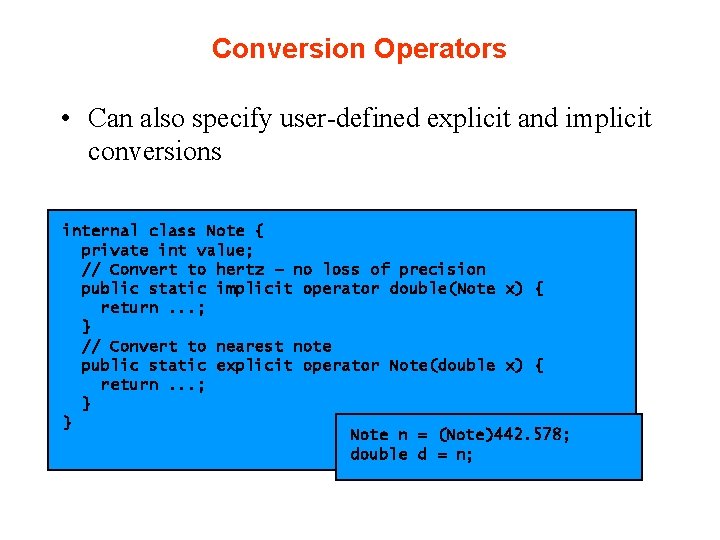
Conversion Operators • Can also specify user-defined explicit and implicit conversions internal class Note { private int value; // Convert to hertz – no loss of precision public static implicit operator double(Note x) { return. . . ; } // Convert to nearest note public static explicit operator Note(double x) { return. . . ; } } Note n = (Note)442. 578; double d = n;
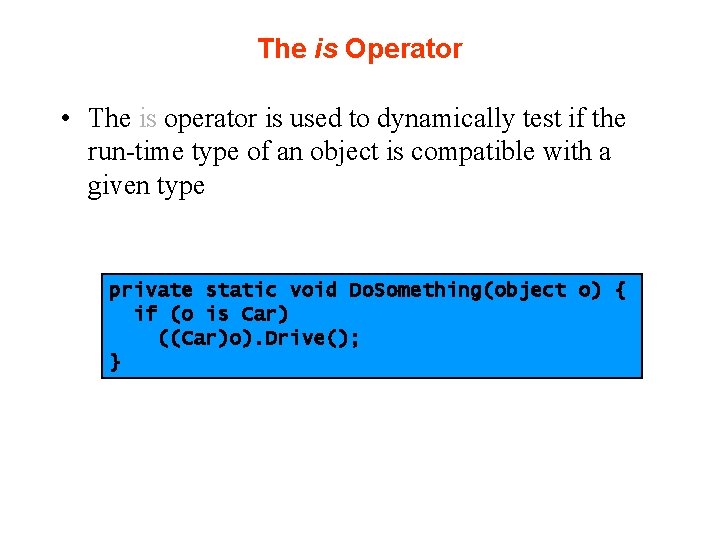
The is Operator • The is operator is used to dynamically test if the run-time type of an object is compatible with a given type private static void Do. Something(object o) { if (o is Car) ((Car)o). Drive(); }
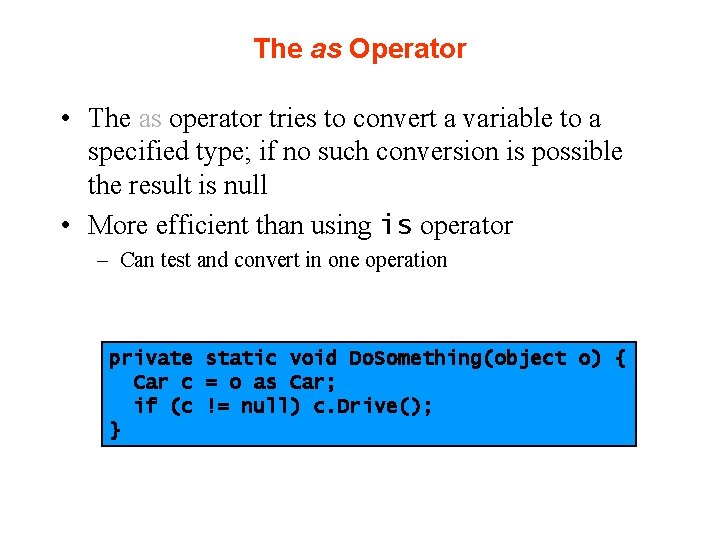
The as Operator • The as operator tries to convert a variable to a specified type; if no such conversion is possible the result is null • More efficient than using is operator – Can test and convert in one operation private static void Do. Something(object o) { Car c = o as Car; if (c != null) c. Drive(); }
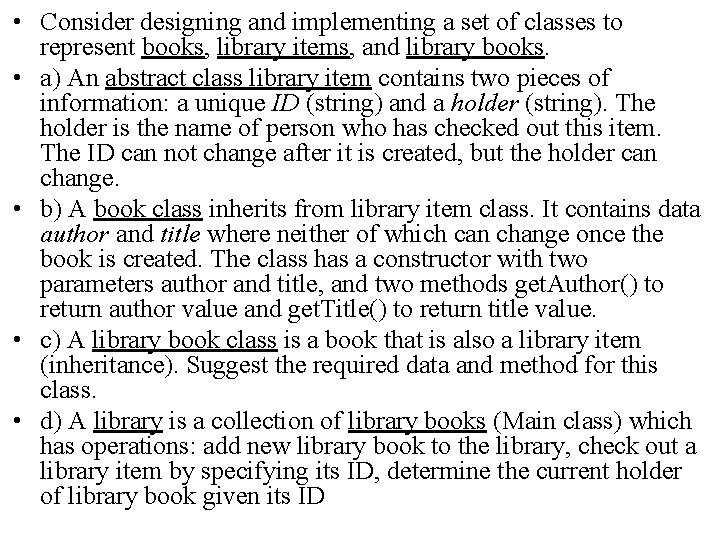
• Consider designing and implementing a set of classes to represent books, library items, and library books. • a) An abstract class library item contains two pieces of information: a unique ID (string) and a holder (string). The holder is the name of person who has checked out this item. The ID can not change after it is created, but the holder can change. • b) A book class inherits from library item class. It contains data author and title where neither of which can change once the book is created. The class has a constructor with two parameters author and title, and two methods get. Author() to return author value and get. Title() to return title value. • c) A library book class is a book that is also a library item (inheritance). Suggest the required data and method for this class. • d) A library is a collection of library books (Main class) which has operations: add new library book to the library, check out a library item by specifying its ID, determine the current holder of library book given its ID
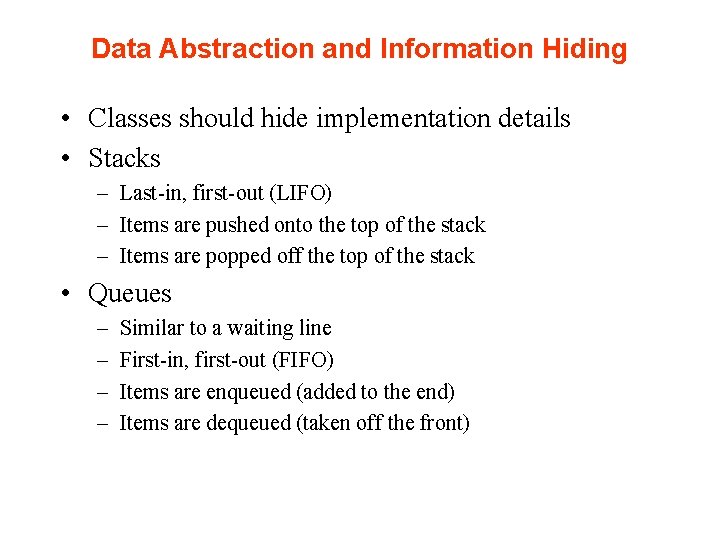
Data Abstraction and Information Hiding • Classes should hide implementation details • Stacks – Last-in, first-out (LIFO) – Items are pushed onto the top of the stack – Items are popped off the top of the stack • Queues – – Similar to a waiting line First-in, first-out (FIFO) Items are enqueued (added to the end) Items are dequeued (taken off the front)