1 n Point Rect Rect h include Point
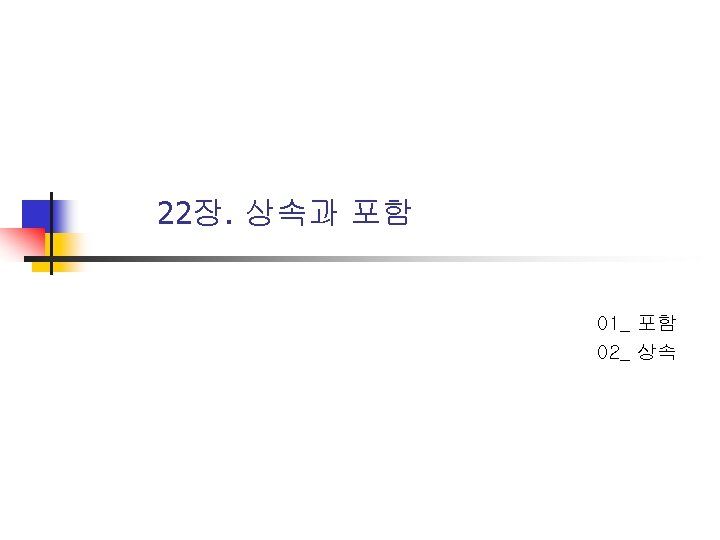
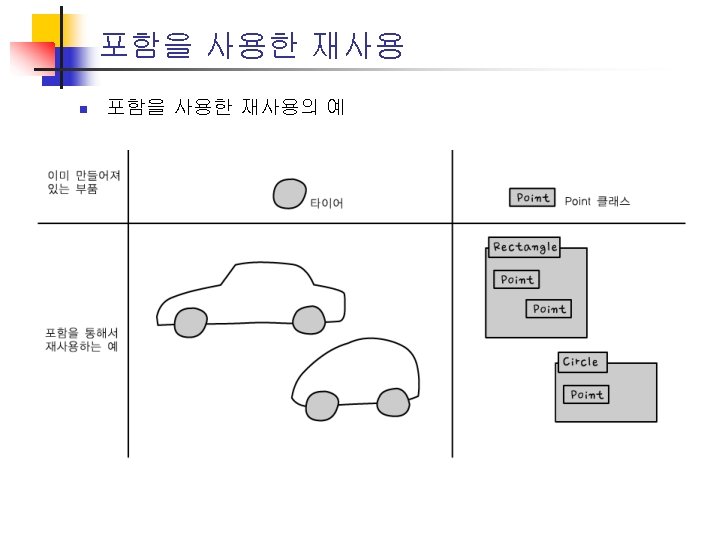
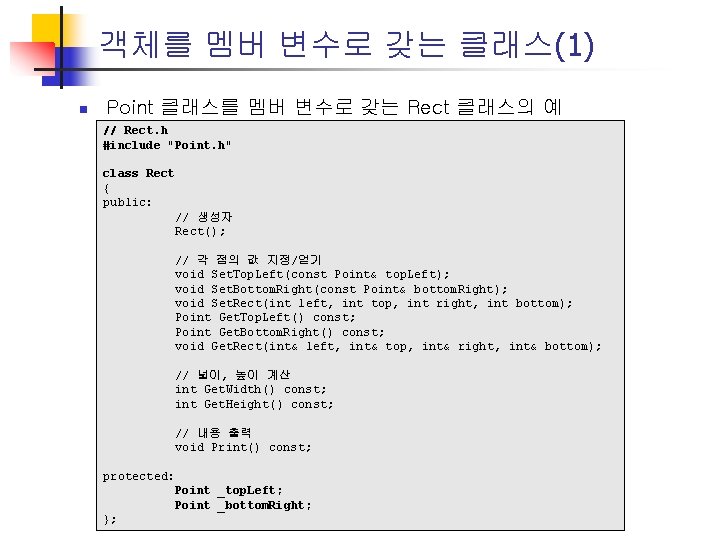
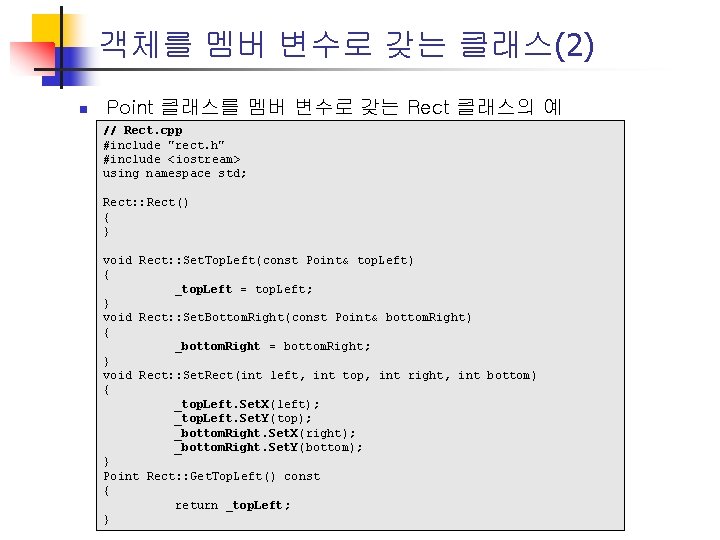
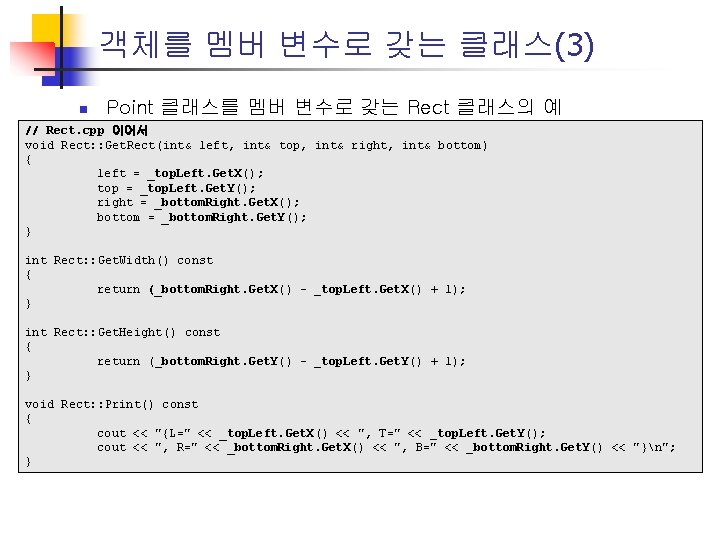
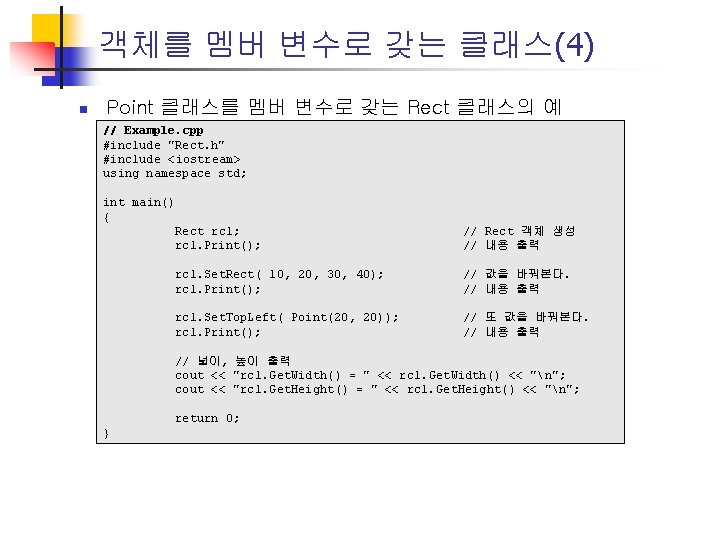
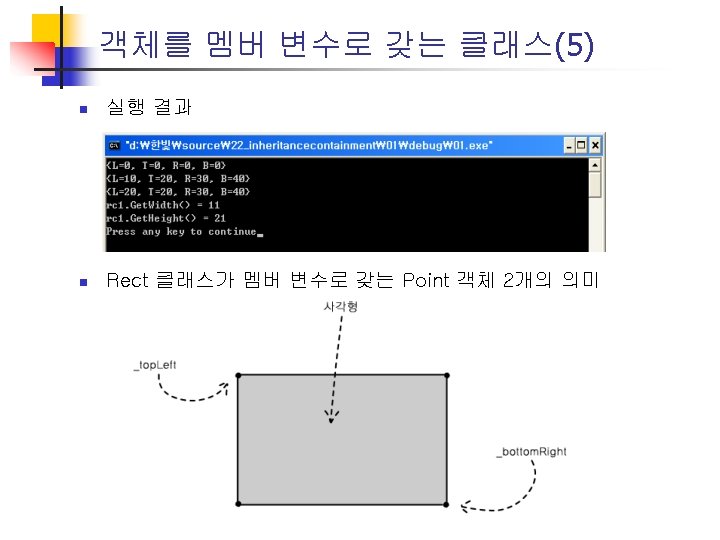
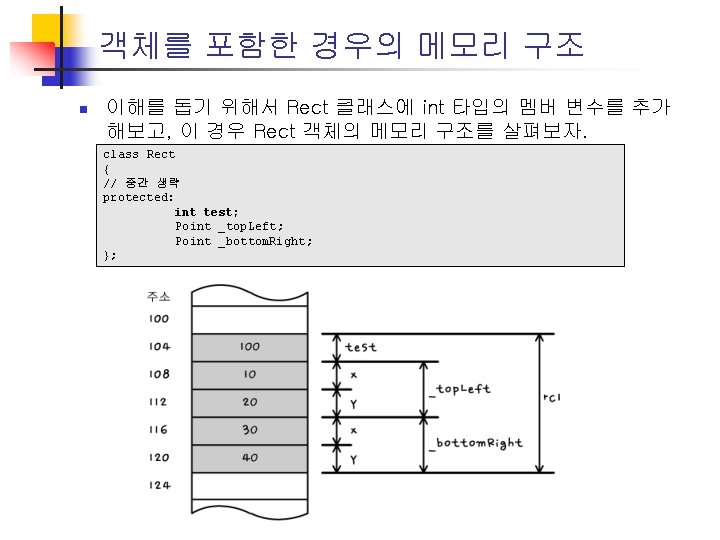
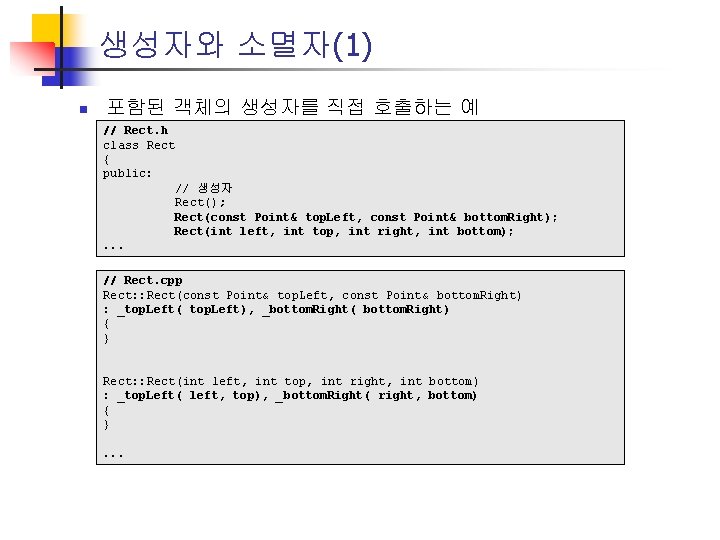
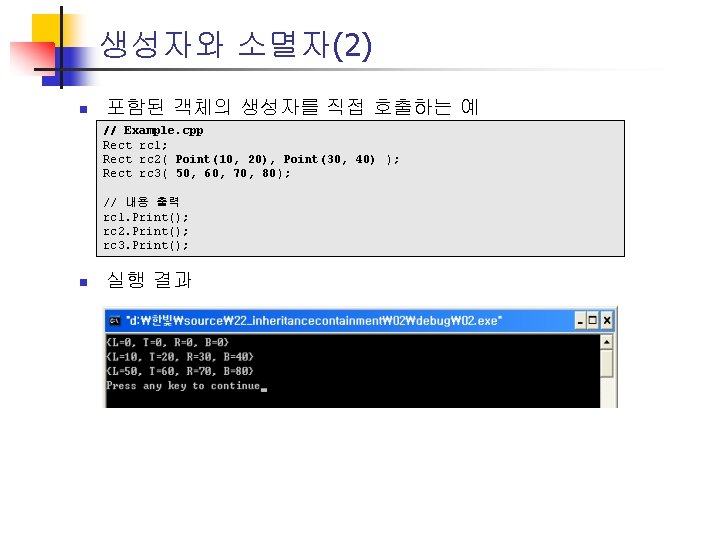
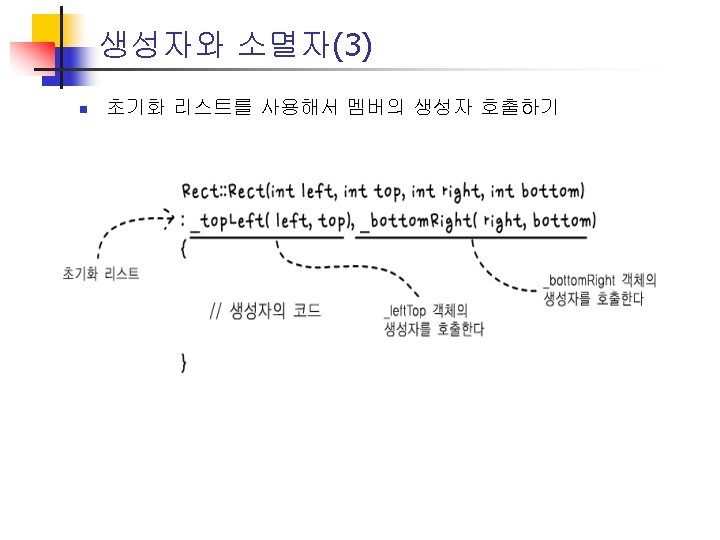
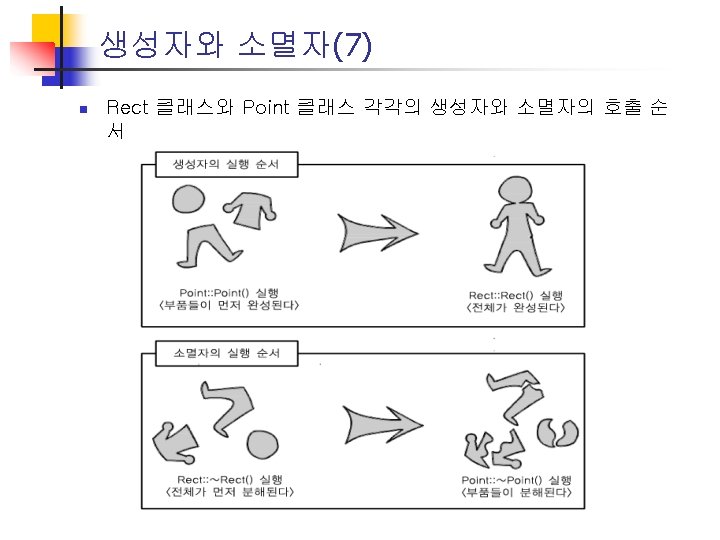
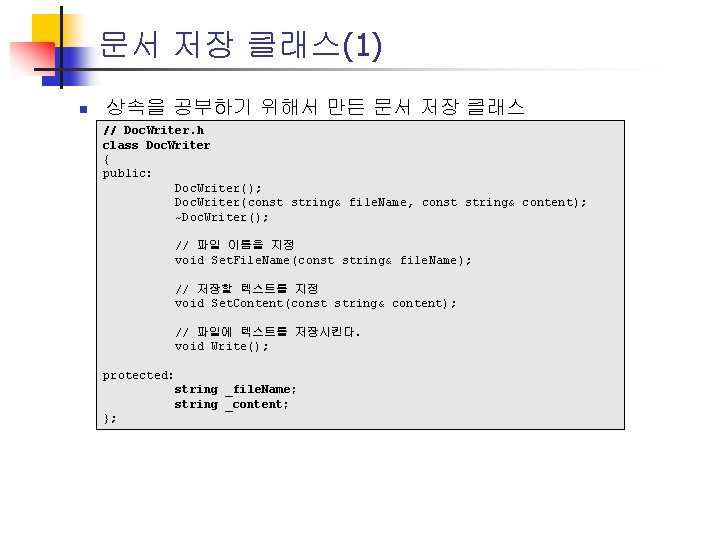
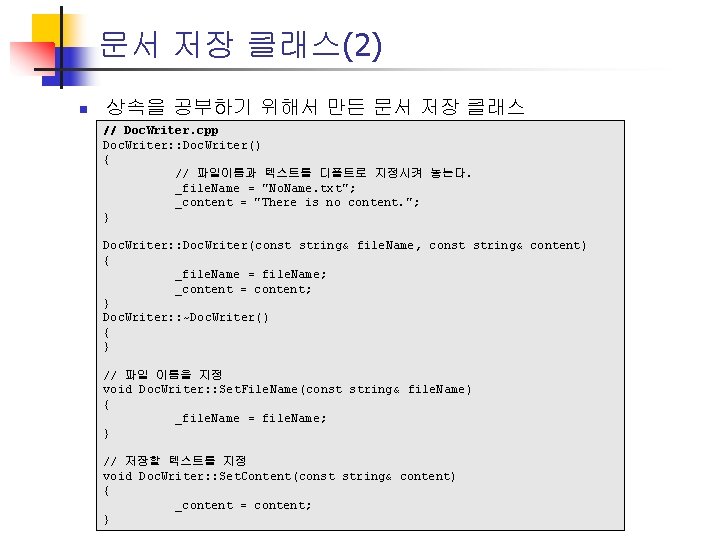
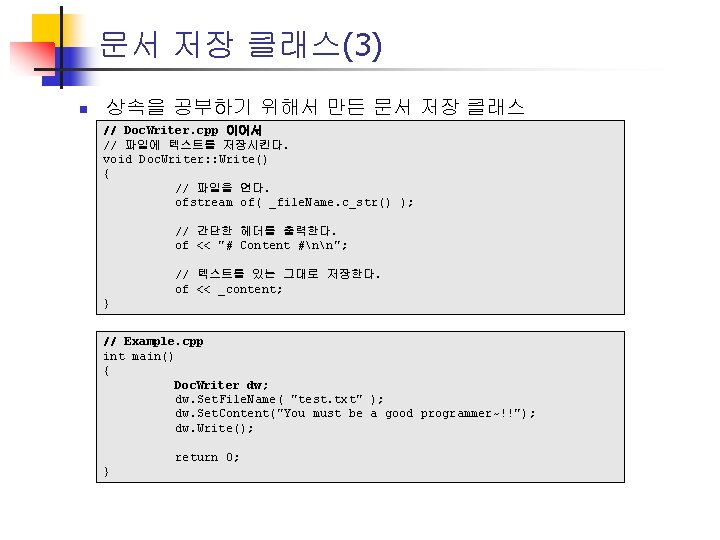
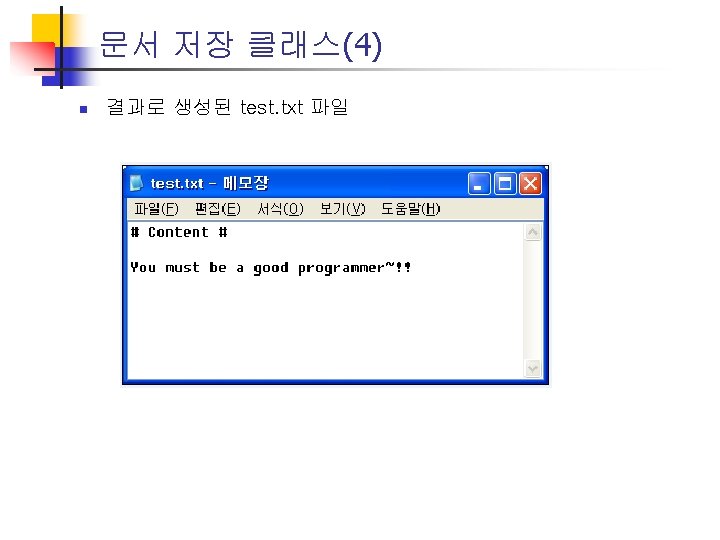
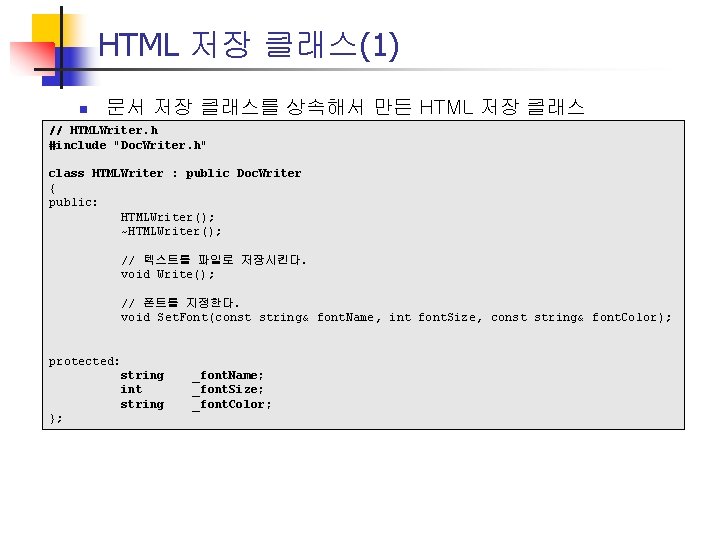
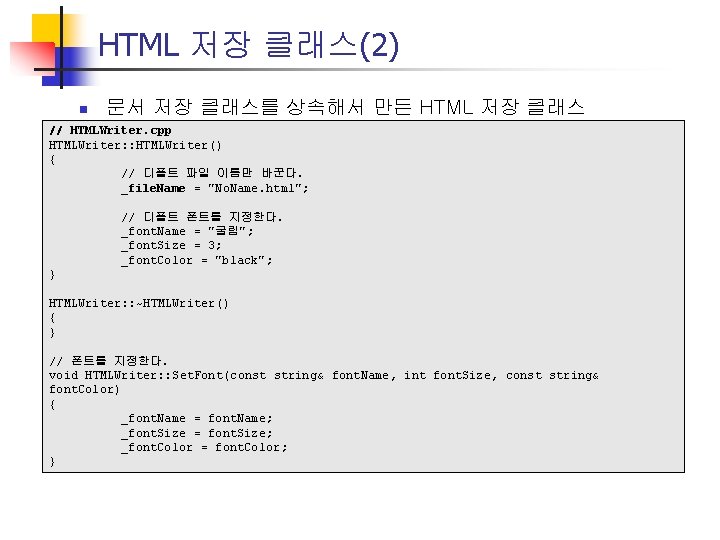
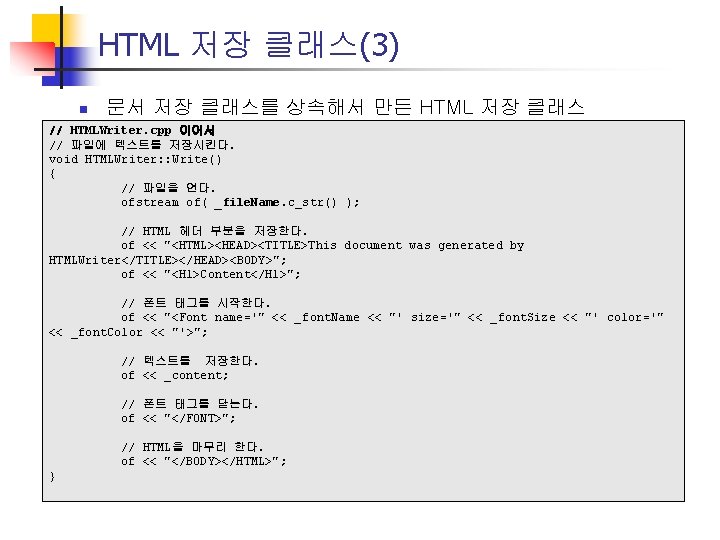
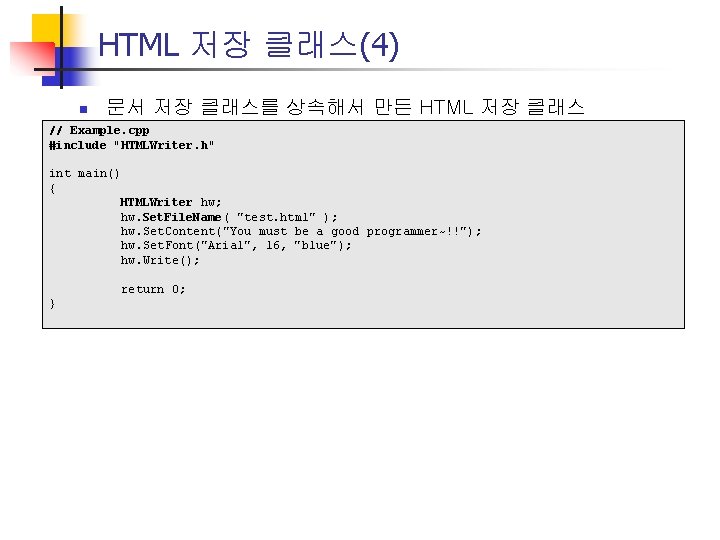
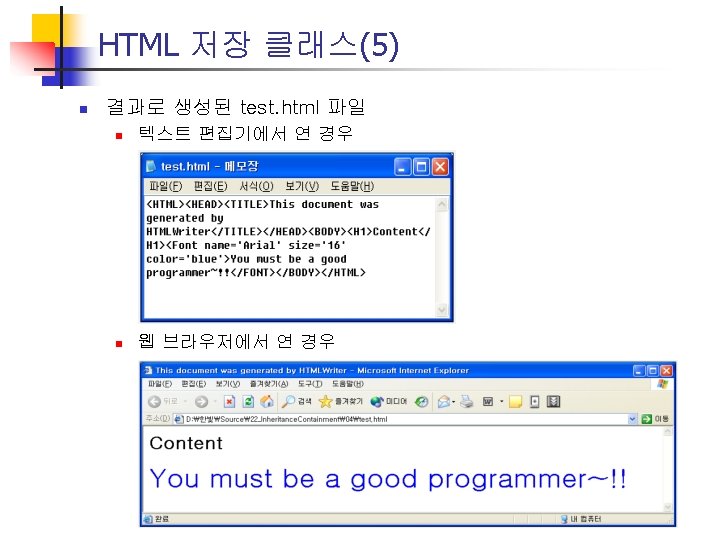
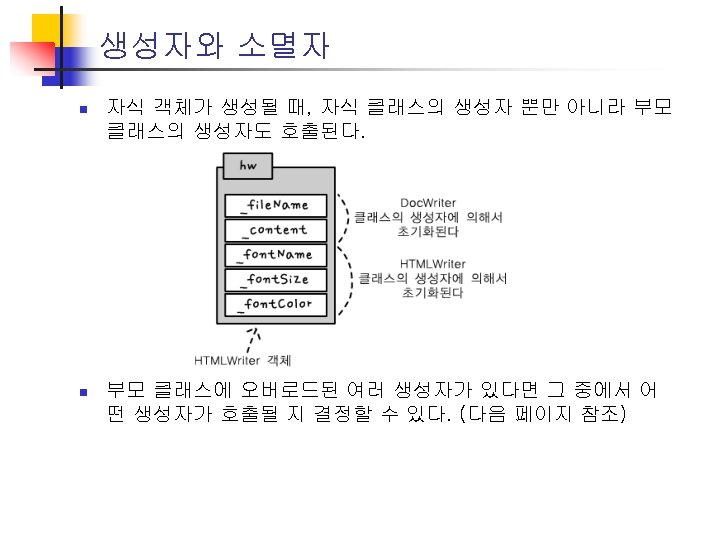
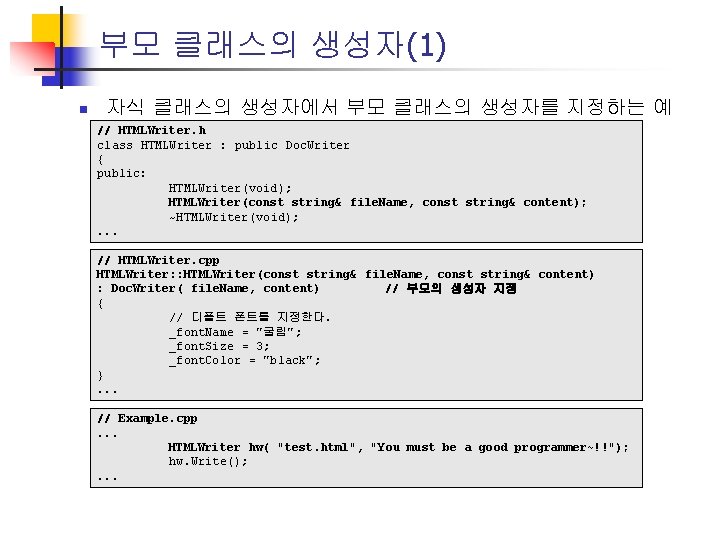
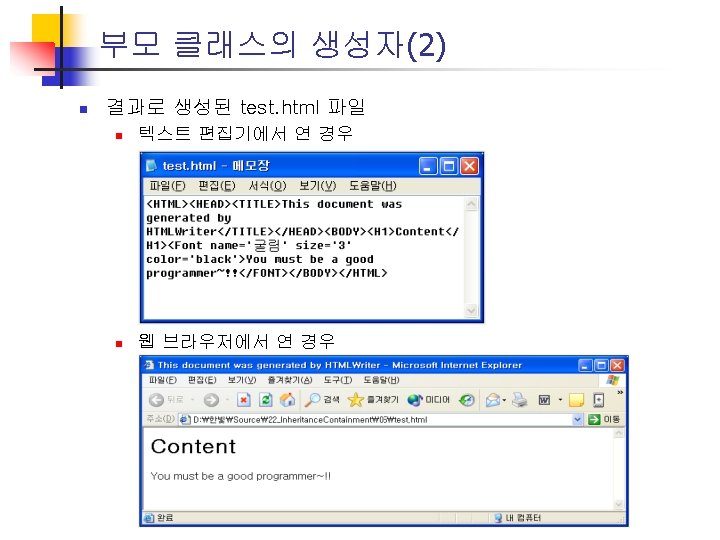
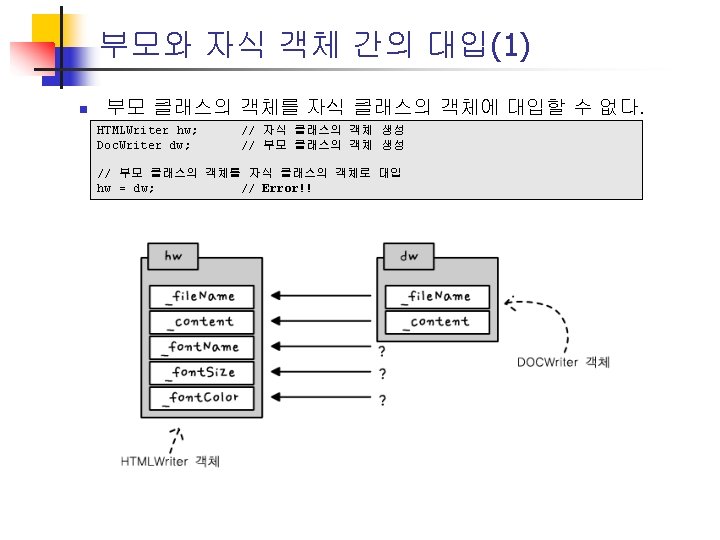
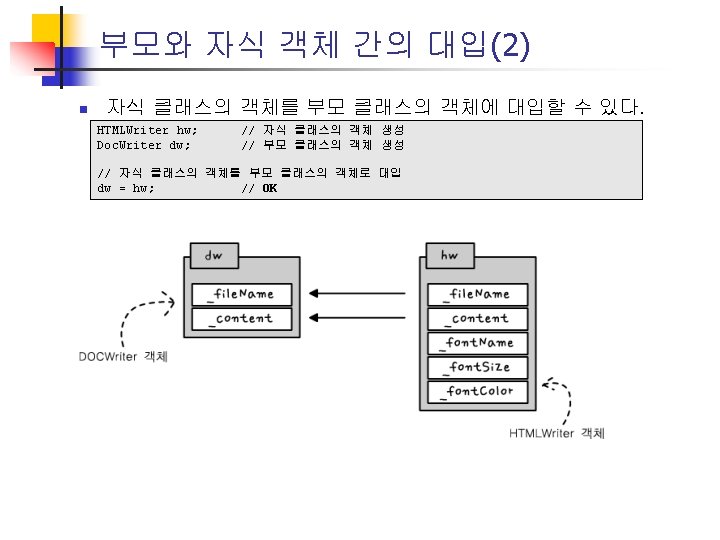
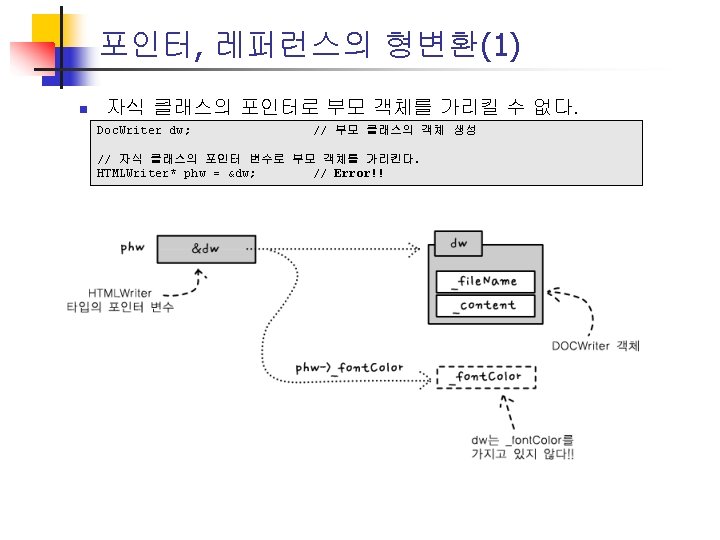
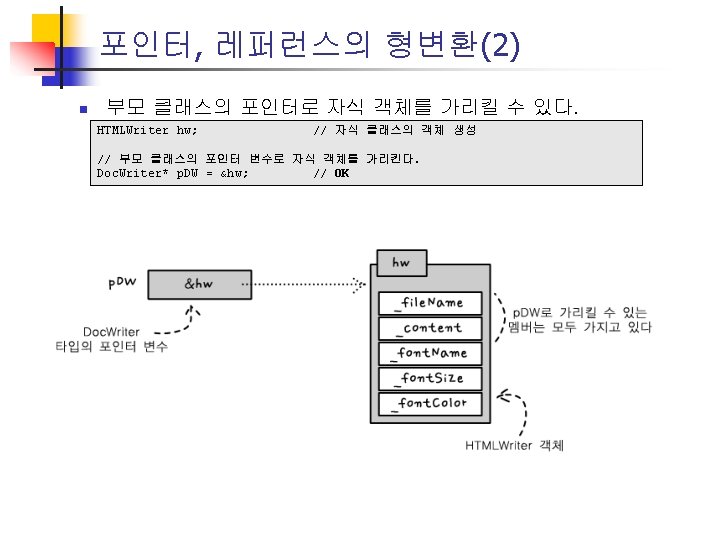
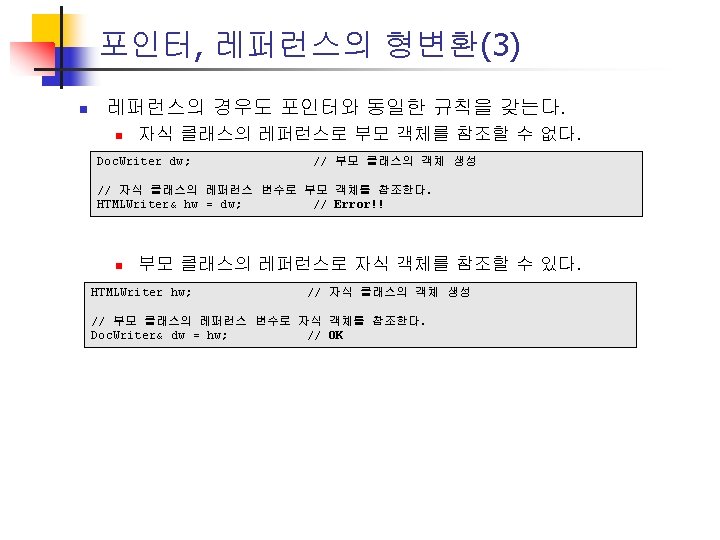
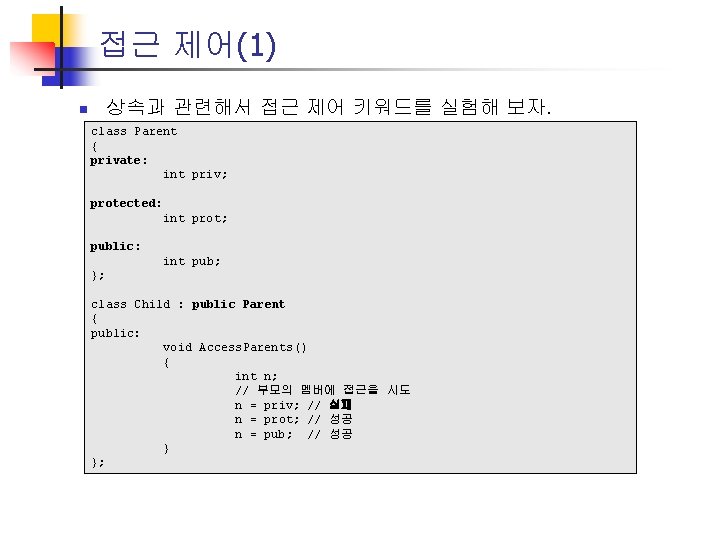
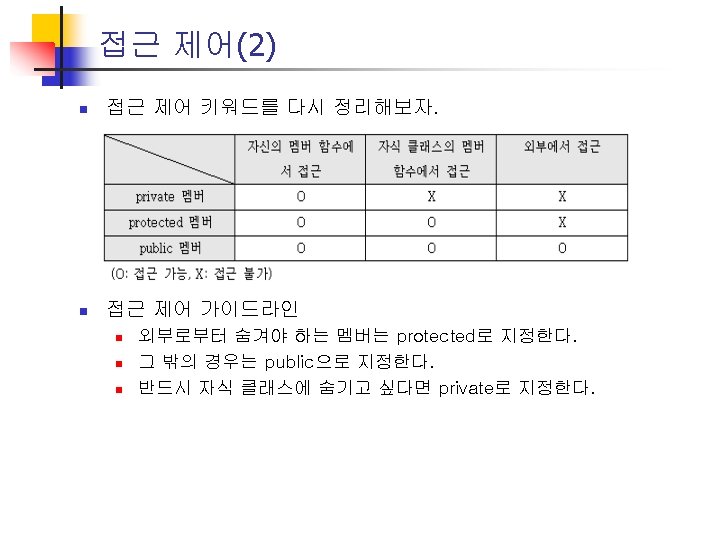
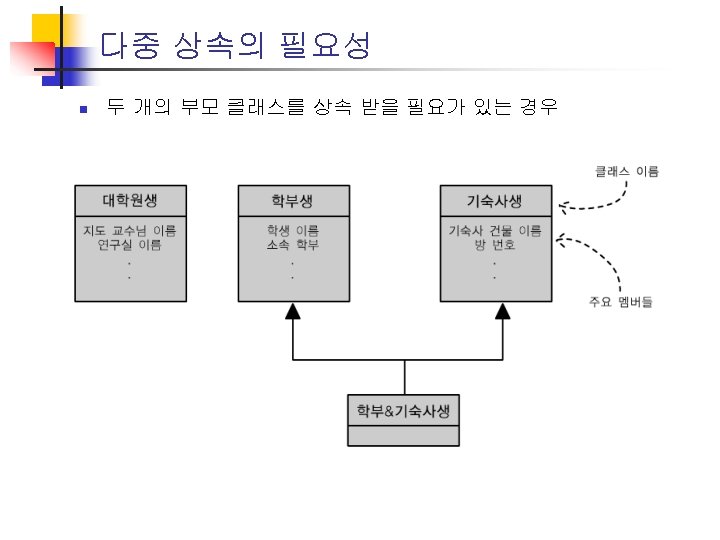
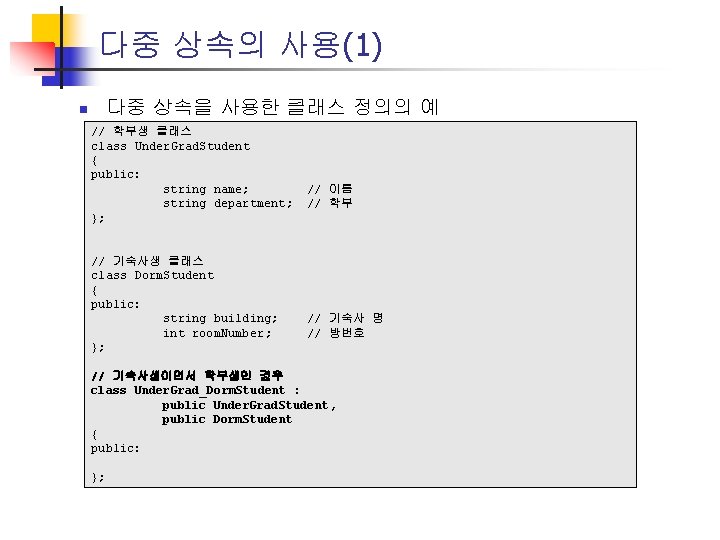
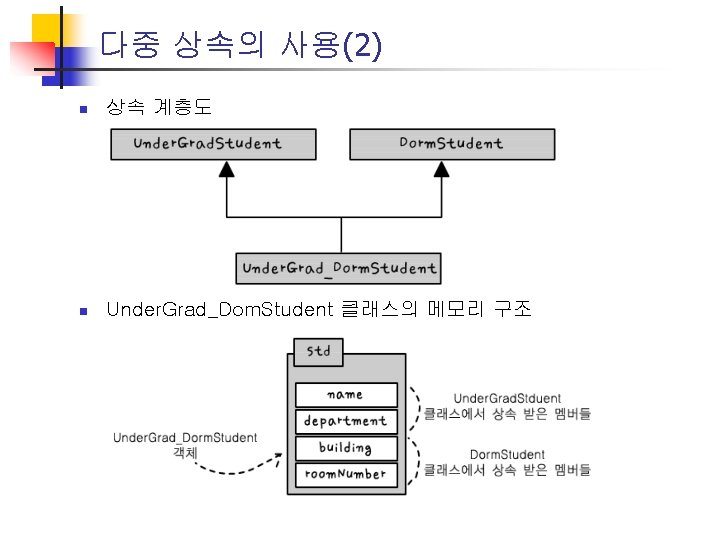
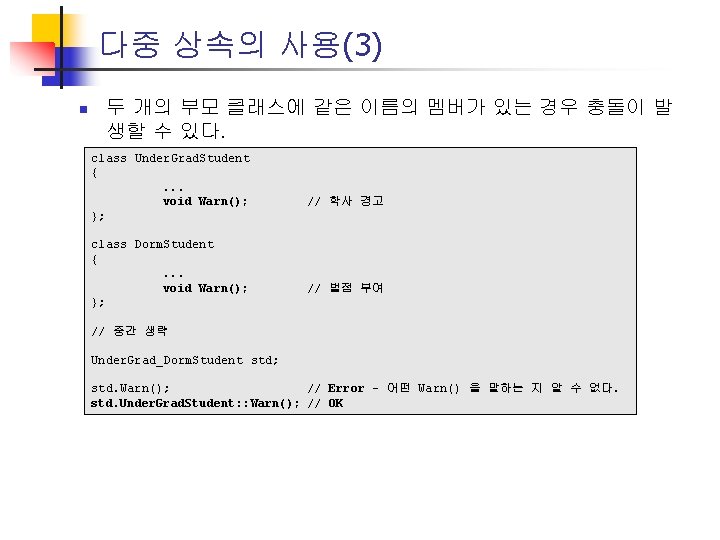
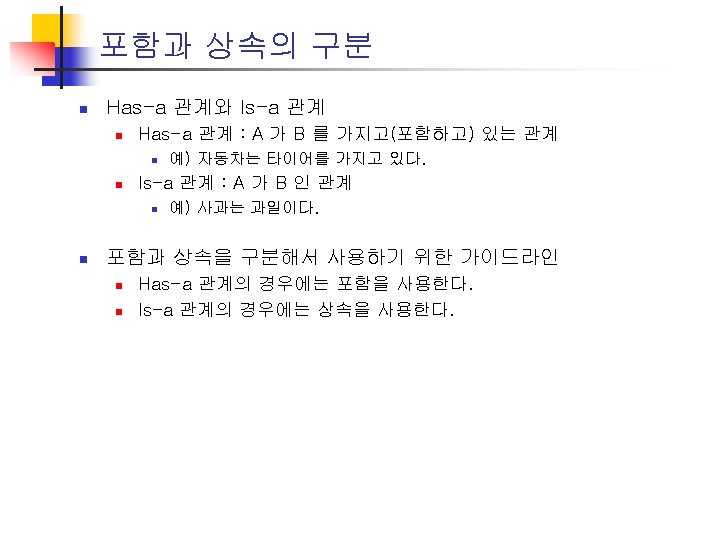
- Slides: 36
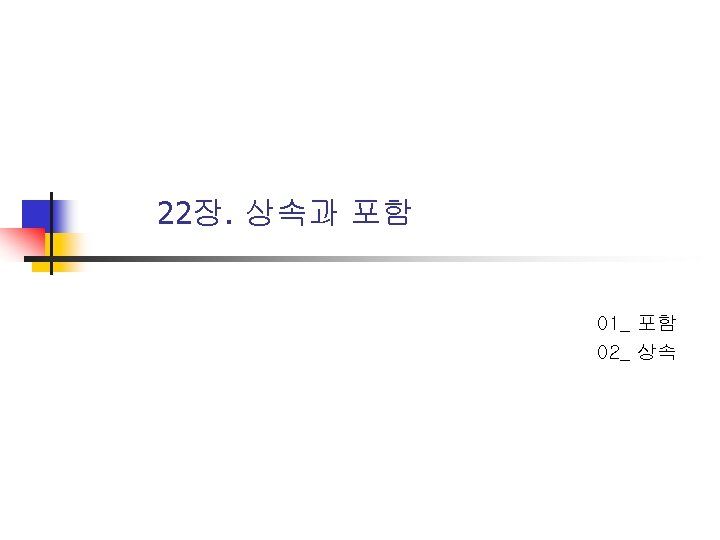
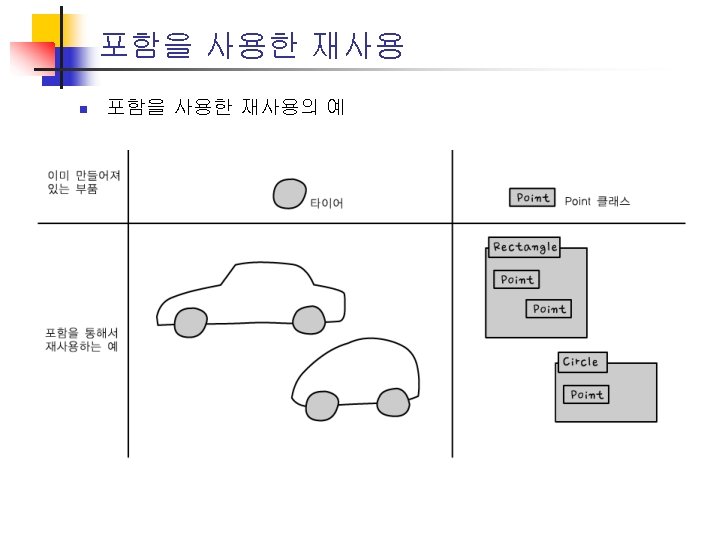
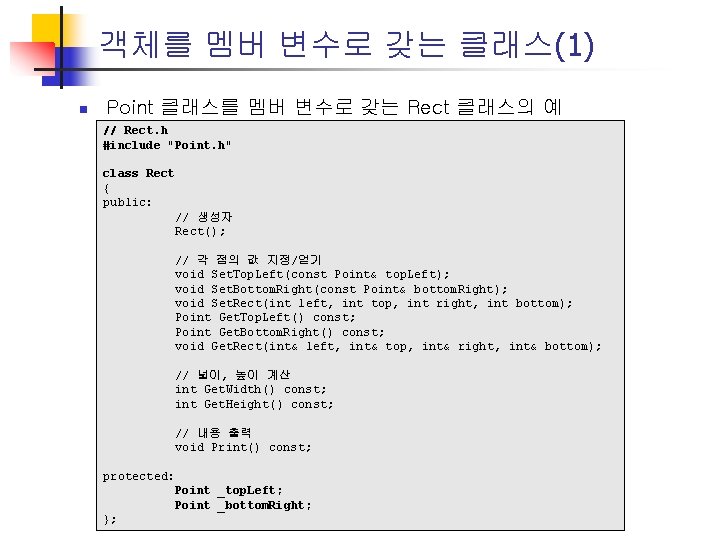
객체를 멤버 변수로 갖는 클래스(1) n Point 클래스를 멤버 변수로 갖는 Rect 클래스의 예 // Rect. h #include "Point. h" class Rect { public: // 생성자 Rect(); // 각 점의 값 지정/얻기 void Set. Top. Left(const Point& top. Left); void Set. Bottom. Right(const Point& bottom. Right); void Set. Rect(int left, int top, int right, int bottom); Point Get. Top. Left() const; Point Get. Bottom. Right() const; void Get. Rect(int& left, int& top, int& right, int& bottom); // 넓이, 높이 계산 int Get. Width() const; int Get. Height() const; // 내용 출력 void Print() const; protected: Point _top. Left; Point _bottom. Right; };
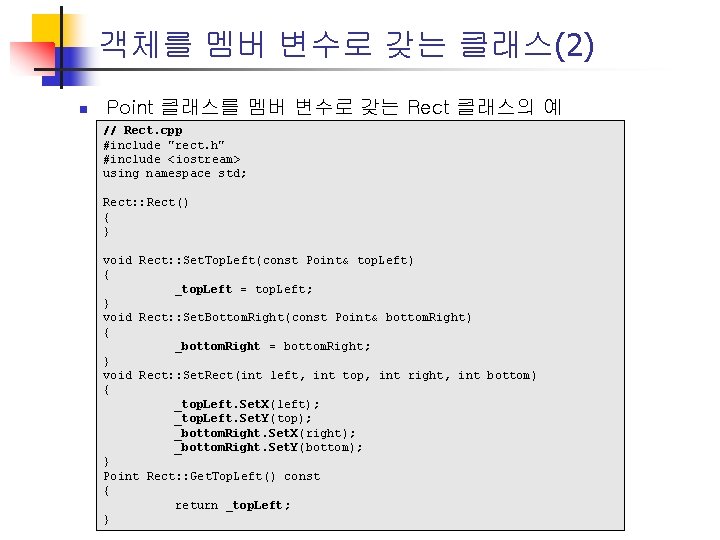
객체를 멤버 변수로 갖는 클래스(2) n Point 클래스를 멤버 변수로 갖는 Rect 클래스의 예 // Rect. cpp #include "rect. h" #include <iostream> using namespace std; Rect: : Rect() { } void Rect: : Set. Top. Left(const Point& top. Left) { _top. Left = top. Left; } void Rect: : Set. Bottom. Right(const Point& bottom. Right) { _bottom. Right = bottom. Right; } void Rect: : Set. Rect(int left, int top, int right, int bottom) { _top. Left. Set. X(left); _top. Left. Set. Y(top); _bottom. Right. Set. X(right); _bottom. Right. Set. Y(bottom); } Point Rect: : Get. Top. Left() const { return _top. Left; }
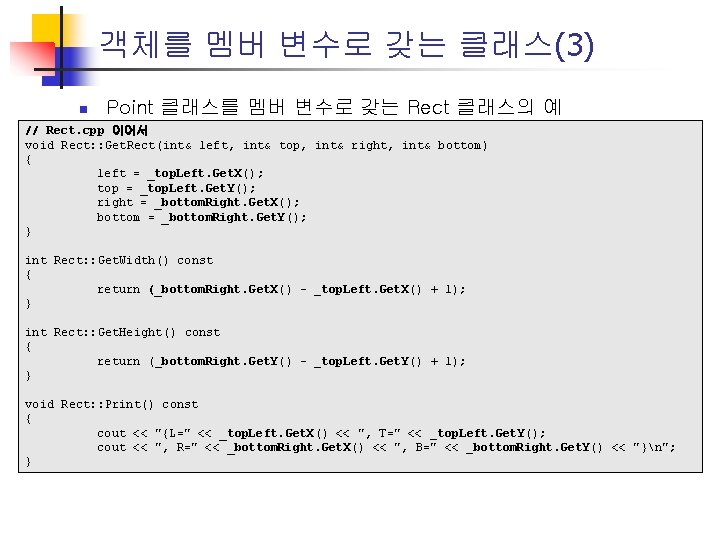
객체를 멤버 변수로 갖는 클래스(3) n Point 클래스를 멤버 변수로 갖는 Rect 클래스의 예 // Rect. cpp 이어서 void Rect: : Get. Rect(int& left, int& top, int& right, int& bottom) { left = _top. Left. Get. X(); top = _top. Left. Get. Y(); right = _bottom. Right. Get. X(); bottom = _bottom. Right. Get. Y(); } int Rect: : Get. Width() const { return (_bottom. Right. Get. X() - _top. Left. Get. X() + 1); } int Rect: : Get. Height() const { return (_bottom. Right. Get. Y() - _top. Left. Get. Y() + 1); } void Rect: : Print() const { cout << "{L=" << _top. Left. Get. X() << ", T=" << _top. Left. Get. Y(); cout << ", R=" << _bottom. Right. Get. X() << ", B=" << _bottom. Right. Get. Y() << "}n"; }
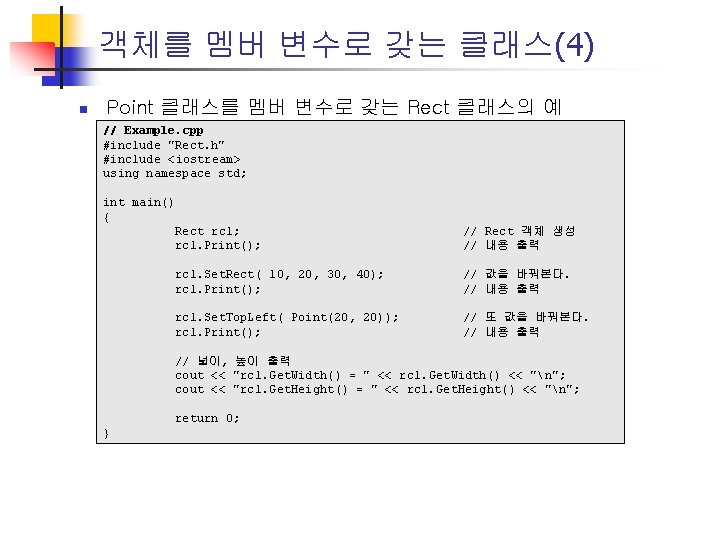
객체를 멤버 변수로 갖는 클래스(4) n Point 클래스를 멤버 변수로 갖는 Rect 클래스의 예 // Example. cpp #include "Rect. h" #include <iostream> using namespace std; int main() { Rect rc 1; rc 1. Print(); // Rect 객체 생성 // 내용 출력 rc 1. Set. Rect( 10, 20, 30, 40); rc 1. Print(); // 값을 바꿔본다. // 내용 출력 rc 1. Set. Top. Left( Point(20, 20)); rc 1. Print(); // 또 값을 바꿔본다. // 내용 출력 // 넓이, 높이 출력 cout << "rc 1. Get. Width() = " << rc 1. Get. Width() << "n"; cout << "rc 1. Get. Height() = " << rc 1. Get. Height() << "n"; return 0; }
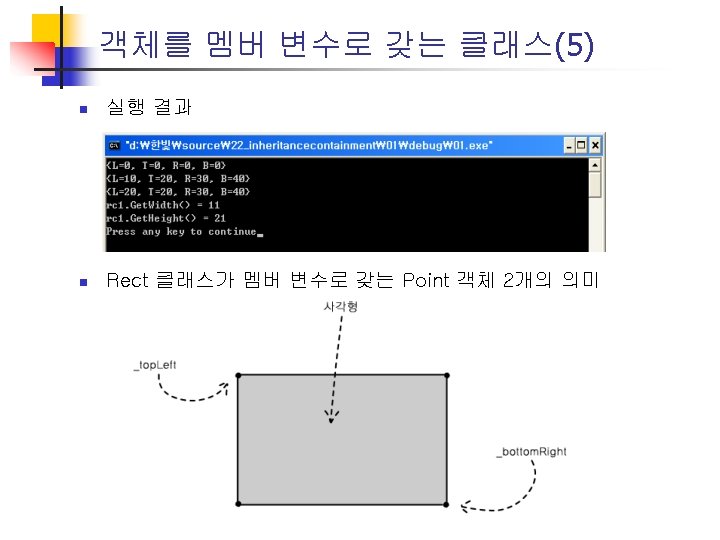
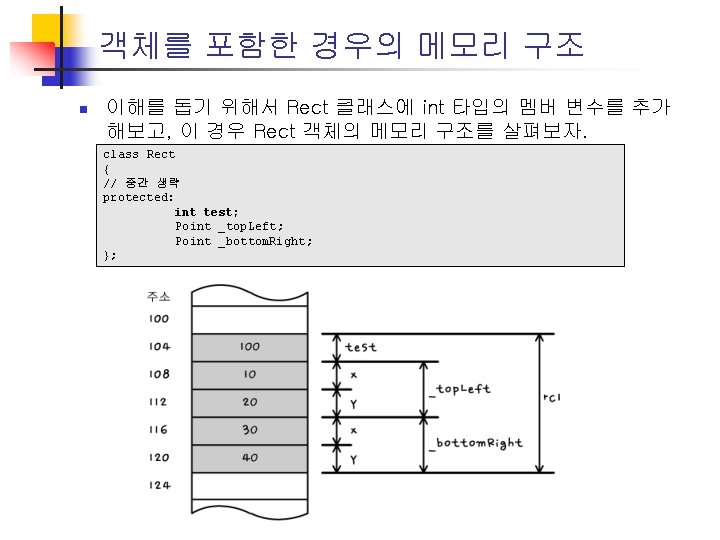
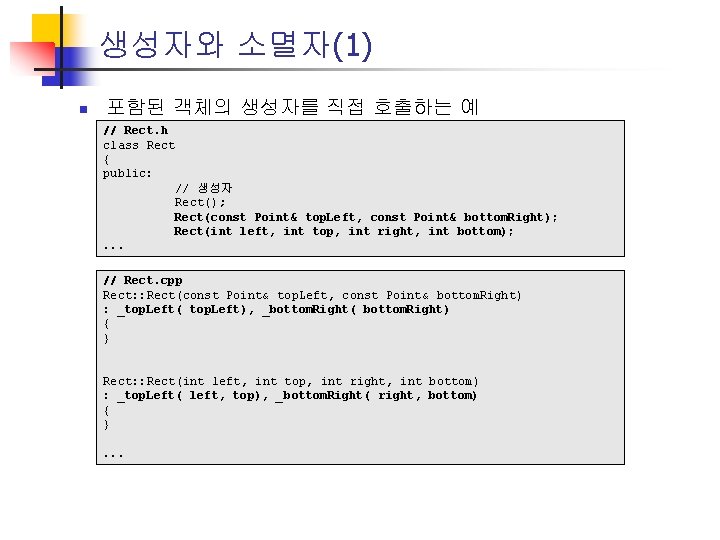
생성자와 소멸자(1) n 포함된 객체의 생성자를 직접 호출하는 예 // Rect. h class Rect { public: // 생성자 Rect(); Rect(const Point& top. Left, const Point& bottom. Right); Rect(int left, int top, int right, int bottom); . . . // Rect. cpp Rect: : Rect(const Point& top. Left, const Point& bottom. Right) : _top. Left( top. Left), _bottom. Right( bottom. Right) { } Rect: : Rect(int left, int top, int right, int bottom) : _top. Left( left, top), _bottom. Right( right, bottom) { }. . .
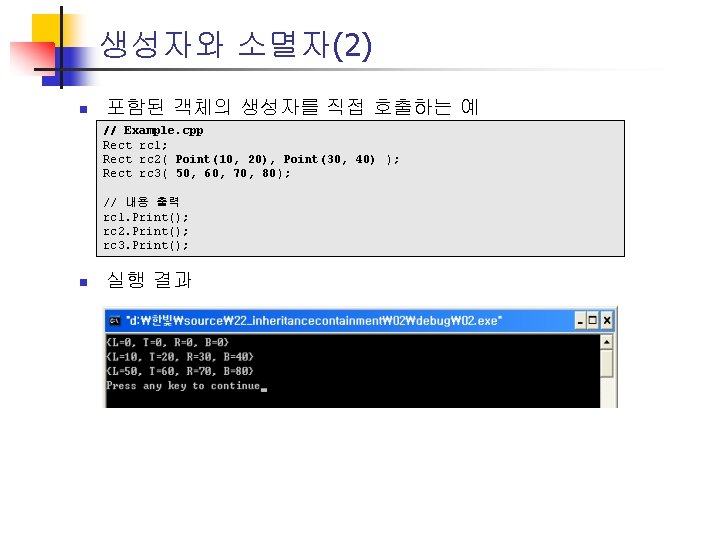
생성자와 소멸자(2) n 포함된 객체의 생성자를 직접 호출하는 예 // Example. cpp Rect rc 1; Rect rc 2( Point(10, 20), Point(30, 40) ); Rect rc 3( 50, 60, 70, 80); // 내용 출력 rc 1. Print(); rc 2. Print(); rc 3. Print(); n 실행 결과 [22 -5]
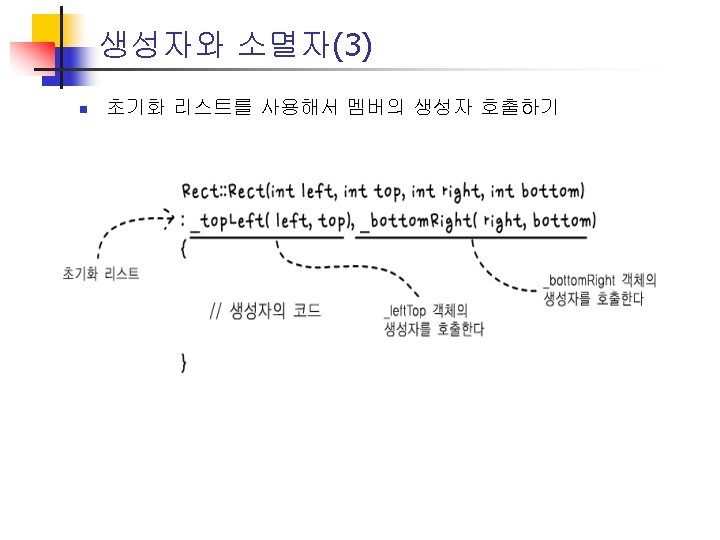
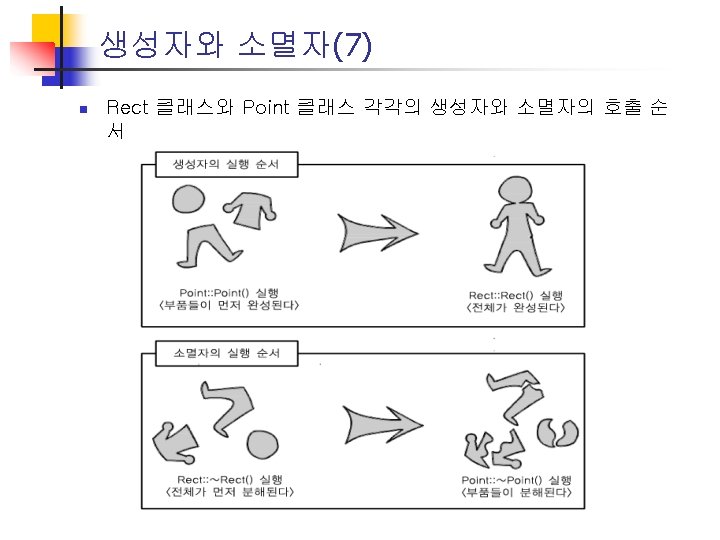
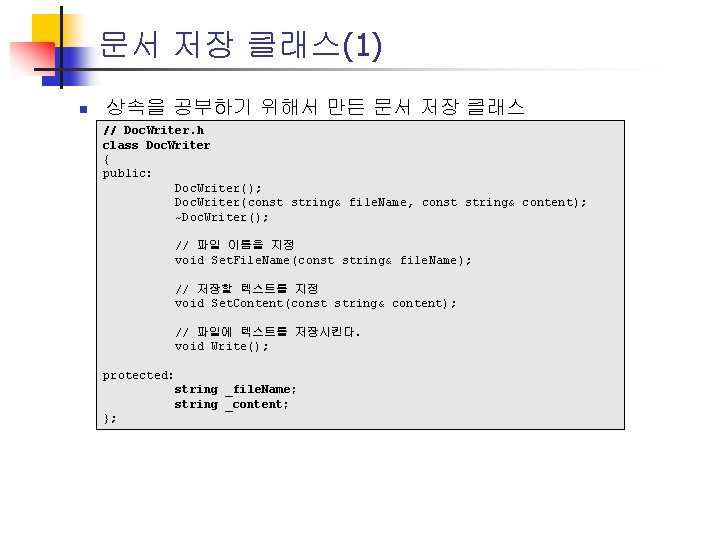
문서 저장 클래스(1) n 상속을 공부하기 위해서 만든 문서 저장 클래스 // Doc. Writer. h class Doc. Writer { public: Doc. Writer(); Doc. Writer(const string& file. Name, const string& content); ~Doc. Writer(); // 파일 이름을 지정 void Set. File. Name(const string& file. Name); // 저장할 텍스트를 지정 void Set. Content(const string& content); // 파일에 텍스트를 저장시킨다. void Write(); protected: string _file. Name; string _content; };
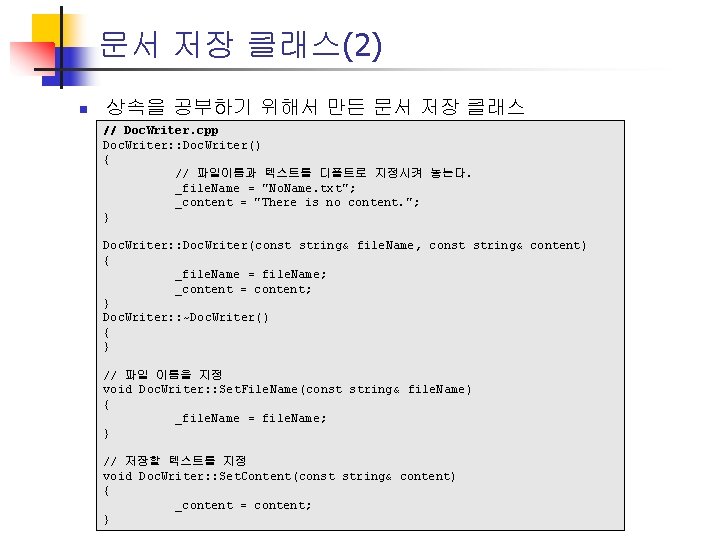
문서 저장 클래스(2) n 상속을 공부하기 위해서 만든 문서 저장 클래스 // Doc. Writer. cpp Doc. Writer: : Doc. Writer() { // 파일이름과 텍스트를 디폴트로 지정시켜 놓는다. _file. Name = "No. Name. txt"; _content = "There is no content. "; } Doc. Writer: : Doc. Writer(const string& file. Name, const string& content) { _file. Name = file. Name; _content = content; } Doc. Writer: : ~Doc. Writer() { } // 파일 이름을 지정 void Doc. Writer: : Set. File. Name(const string& file. Name) { _file. Name = file. Name; } // 저장할 텍스트를 지정 void Doc. Writer: : Set. Content(const string& content) { _content = content; }
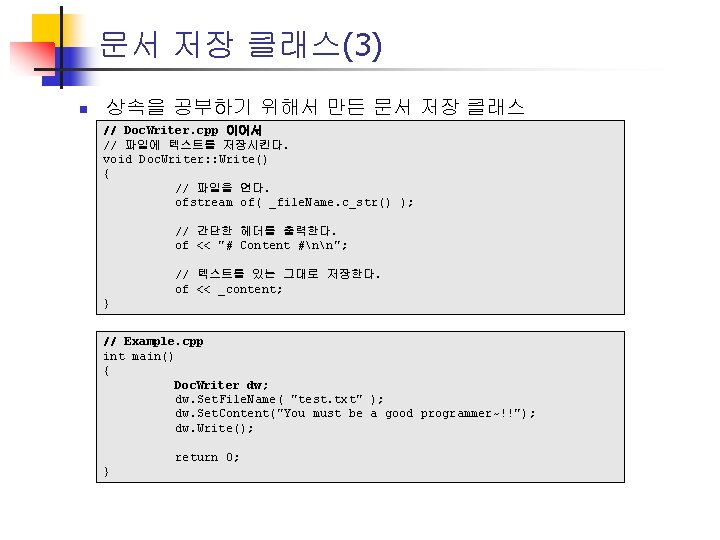
문서 저장 클래스(3) n 상속을 공부하기 위해서 만든 문서 저장 클래스 // Doc. Writer. cpp 이어서 // 파일에 텍스트를 저장시킨다. void Doc. Writer: : Write() { // 파일을 연다. ofstream of( _file. Name. c_str() ); // 간단한 헤더를 출력한다. of << "# Content #nn"; // 텍스트를 있는 그대로 저장한다. of << _content; } // Example. cpp int main() { Doc. Writer dw; dw. Set. File. Name( "test. txt" ); dw. Set. Content("You must be a good programmer~!!"); dw. Write(); return 0; }
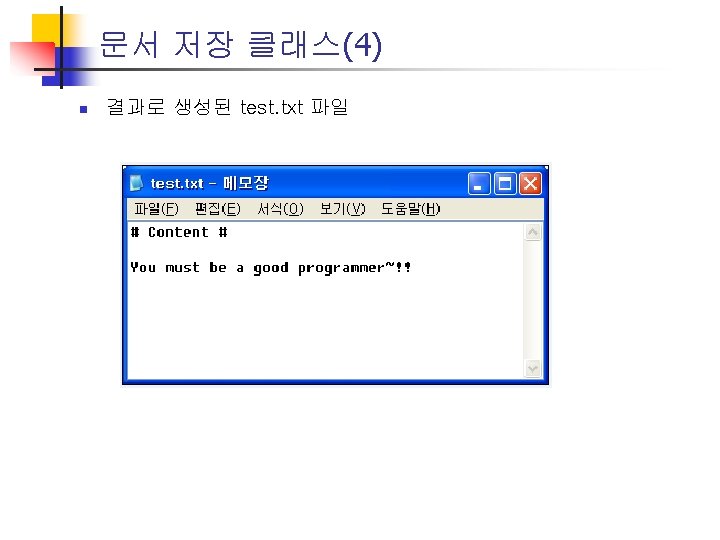
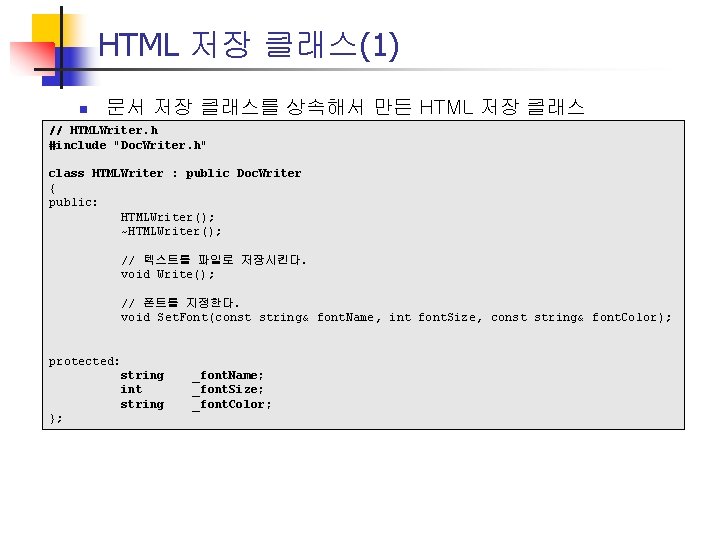
HTML 저장 클래스(1) n 문서 저장 클래스를 상속해서 만든 HTML 저장 클래스 // HTMLWriter. h #include "Doc. Writer. h" class HTMLWriter : public Doc. Writer { public: HTMLWriter(); ~HTMLWriter(); // 텍스트를 파일로 저장시킨다. void Write(); // 폰트를 지정한다. void Set. Font(const string& font. Name, int font. Size, const string& font. Color); protected: string int string }; _font. Name; _font. Size; _font. Color;
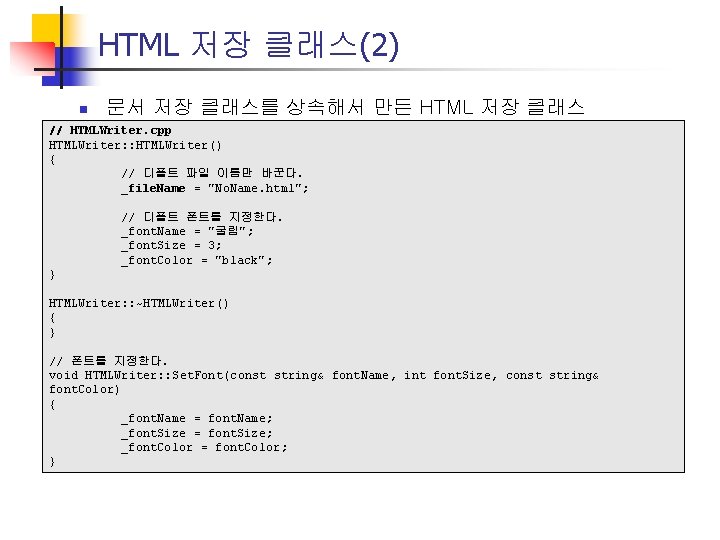
HTML 저장 클래스(2) n 문서 저장 클래스를 상속해서 만든 HTML 저장 클래스 // HTMLWriter. cpp HTMLWriter: : HTMLWriter() { // 디폴트 파일 이름만 바꾼다. _file. Name = "No. Name. html"; // 디폴트 폰트를 지정한다. _font. Name = "굴림"; _font. Size = 3; _font. Color = "black"; } HTMLWriter: : ~HTMLWriter() { } // 폰트를 지정한다. void HTMLWriter: : Set. Font(const string& font. Name, int font. Size, const string& font. Color) { _font. Name = font. Name; _font. Size = font. Size; _font. Color = font. Color; }
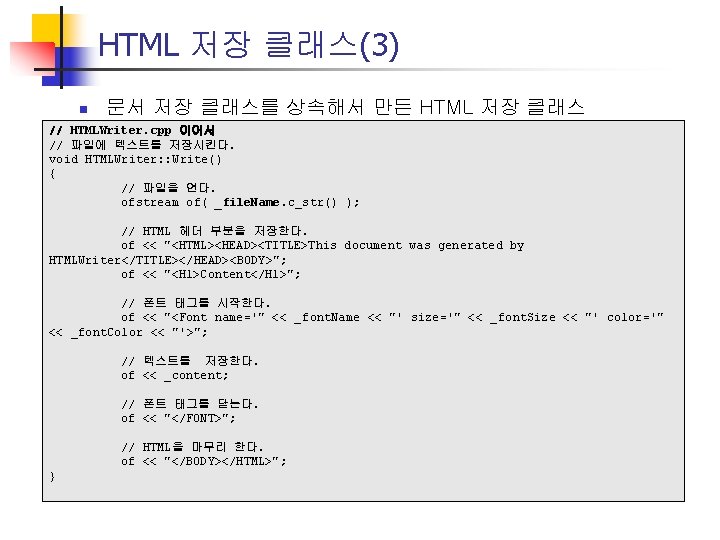
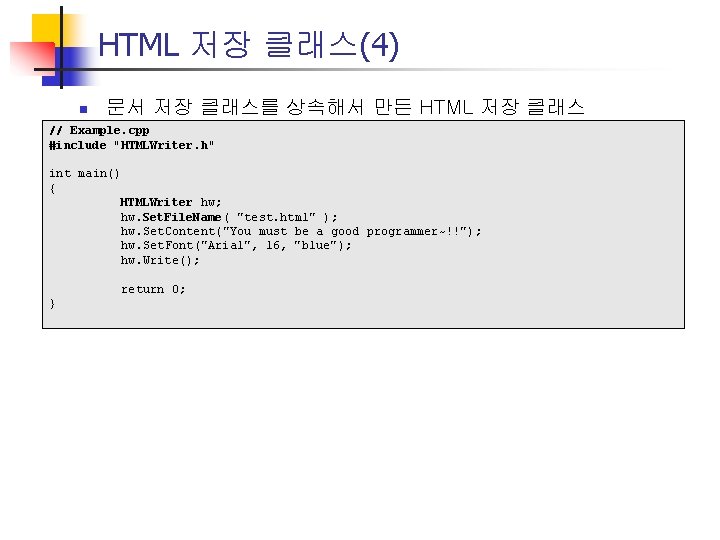
HTML 저장 클래스(4) n 문서 저장 클래스를 상속해서 만든 HTML 저장 클래스 // Example. cpp #include "HTMLWriter. h" int main() { HTMLWriter hw; hw. Set. File. Name( "test. html" ); hw. Set. Content("You must be a good programmer~!!"); hw. Set. Font("Arial", 16, "blue"); hw. Write(); return 0; }
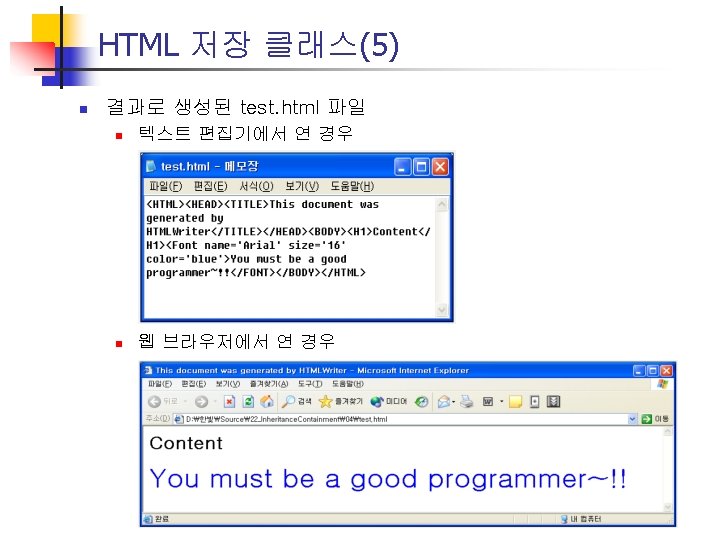
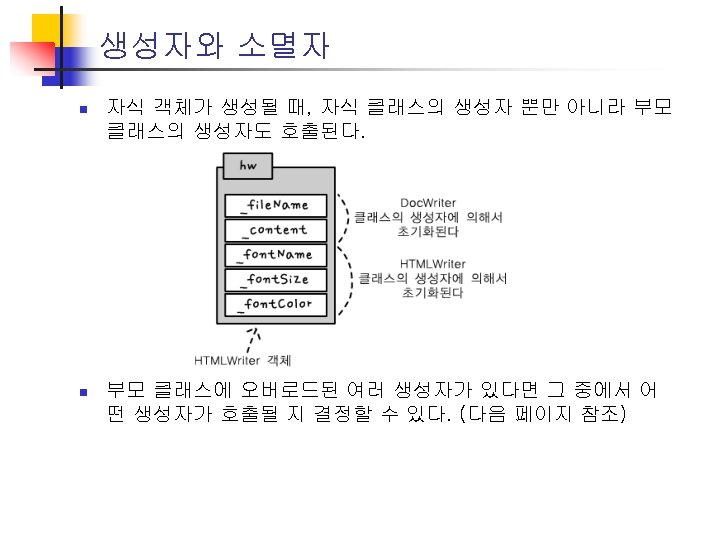
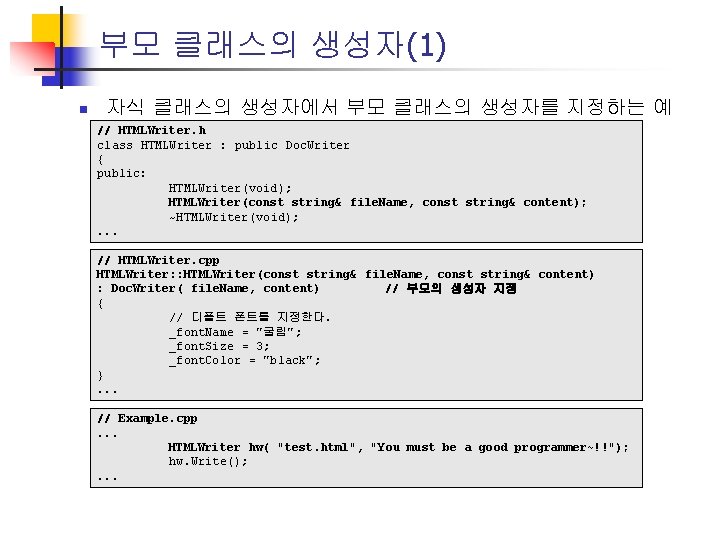
부모 클래스의 생성자(1) n 자식 클래스의 생성자에서 부모 클래스의 생성자를 지정하는 예 // HTMLWriter. h class HTMLWriter : public Doc. Writer { public: HTMLWriter(void); HTMLWriter(const string& file. Name, const string& content); ~HTMLWriter(void); . . . // HTMLWriter. cpp HTMLWriter: : HTMLWriter(const string& file. Name, const string& content) : Doc. Writer( file. Name, content) // 부모의 생성자 지정 { // 디폴트 폰트를 지정한다. _font. Name = "굴림"; _font. Size = 3; _font. Color = "black"; }. . . // Example. cpp. . . HTMLWriter hw( "test. html", "You must be a good programmer~!!"); hw. Write(); . . .
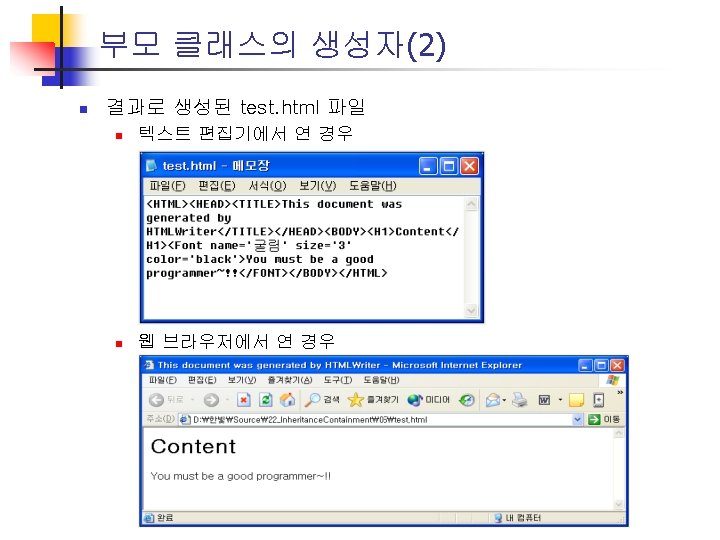
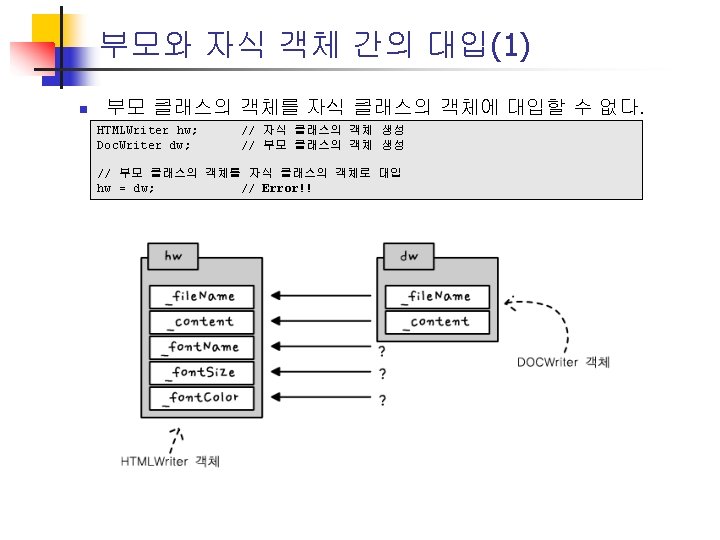
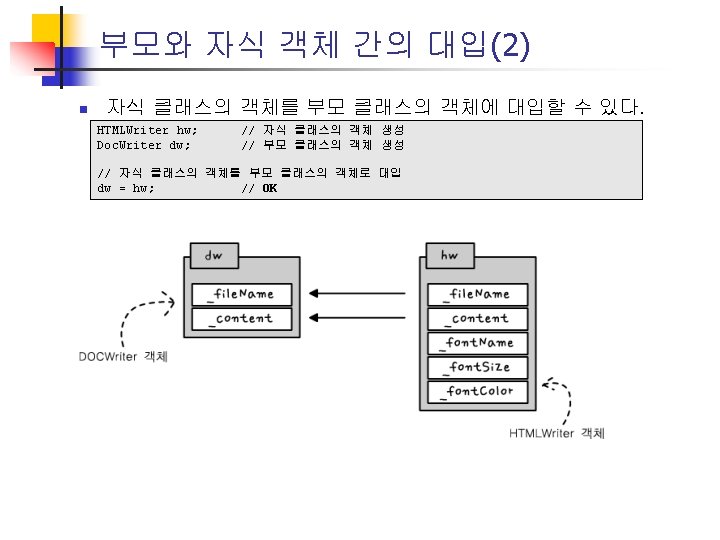
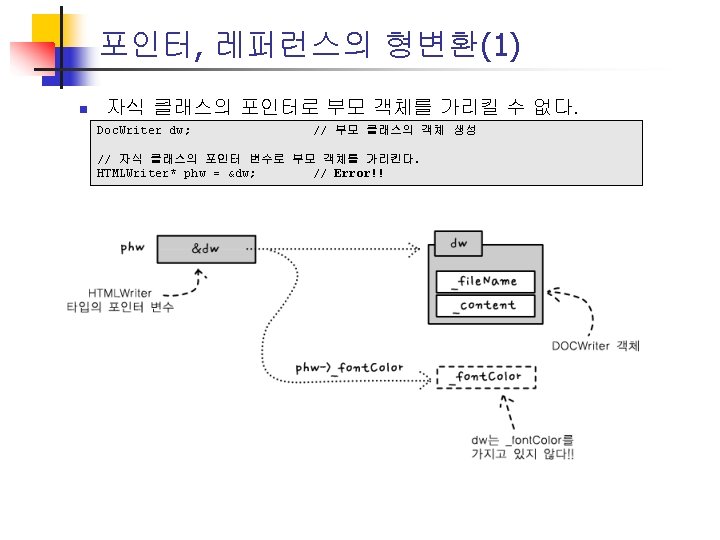
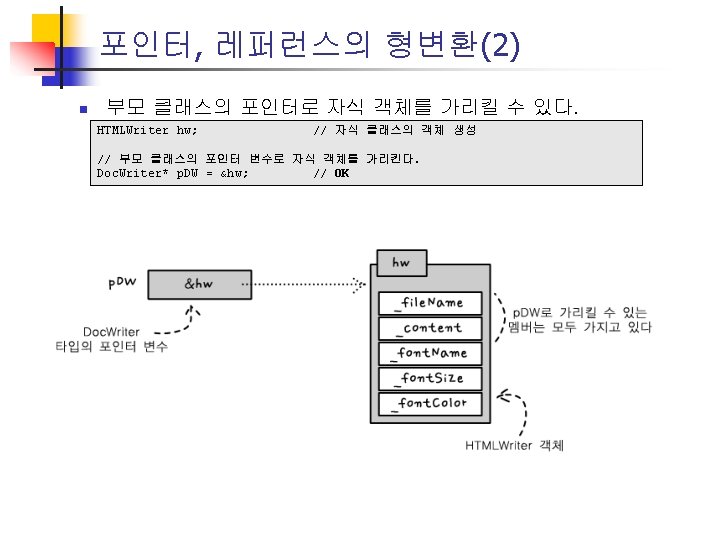
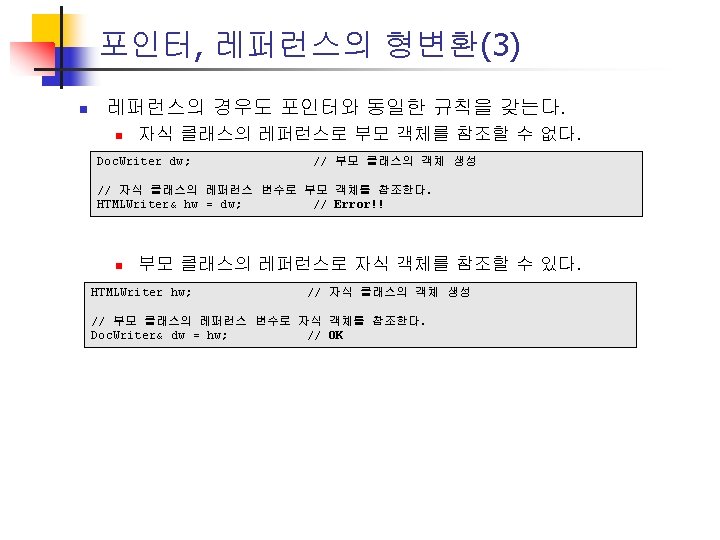
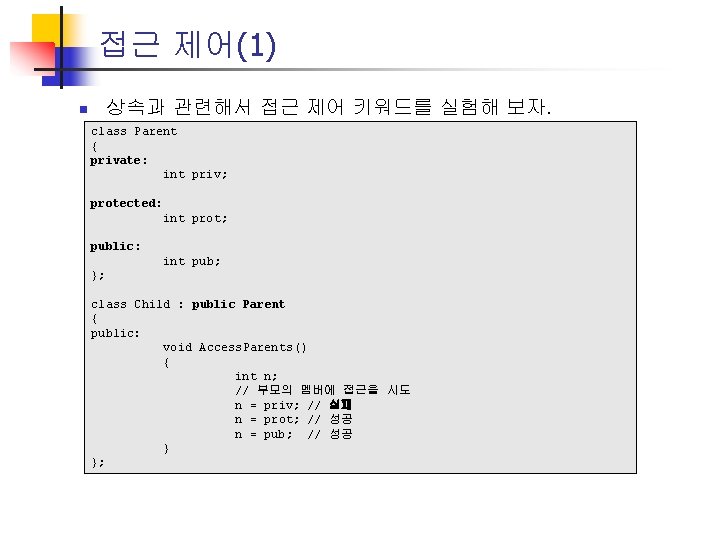
접근 제어(1) 상속과 관련해서 접근 제어 키워드를 실험해 보자. n class Parent { private: int priv; protected: int prot; public: int pub; }; class Child : public Parent { public: void Access. Parents() { int n; // 부모의 멤버에 접근을 시도 n = priv; // 실패 n = prot; // 성공 n = pub; // 성공 } };
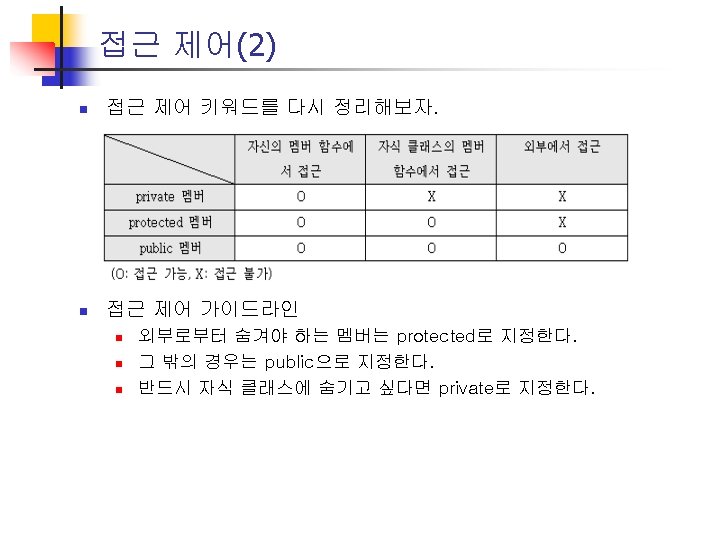
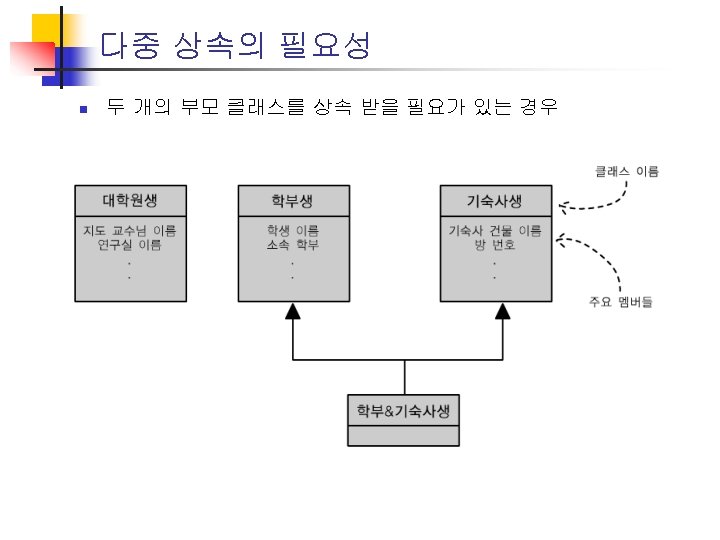
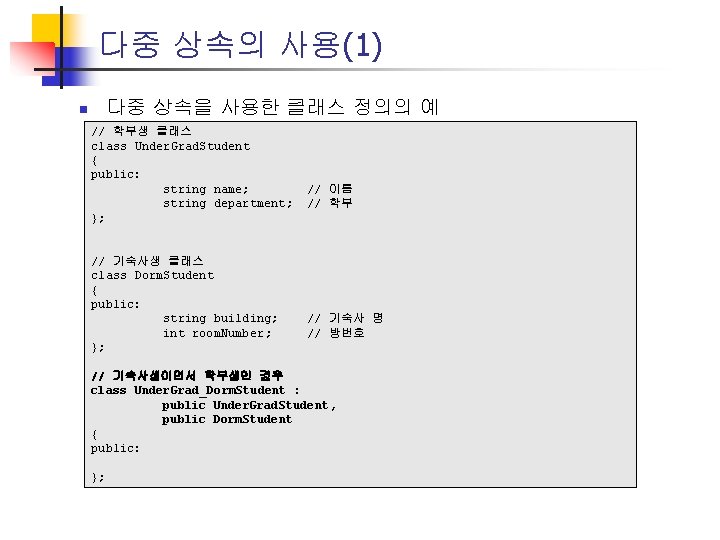
다중 상속의 사용(1) 다중 상속을 사용한 클래스 정의의 예 n // 학부생 클래스 class Under. Grad. Student { public: string name; string department; }; // 기숙사생 클래스 class Dorm. Student { public: string building; int room. Number; }; // 이름 // 학부 // 기숙사 명 // 방번호 // 기숙사생이면서 학부생인 경우 class Under. Grad_Dorm. Student : public Under. Grad. Student, public Dorm. Student { public: };
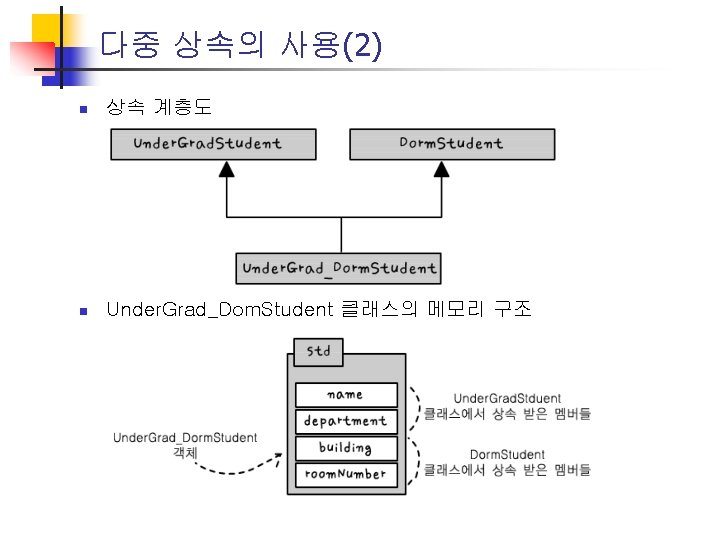
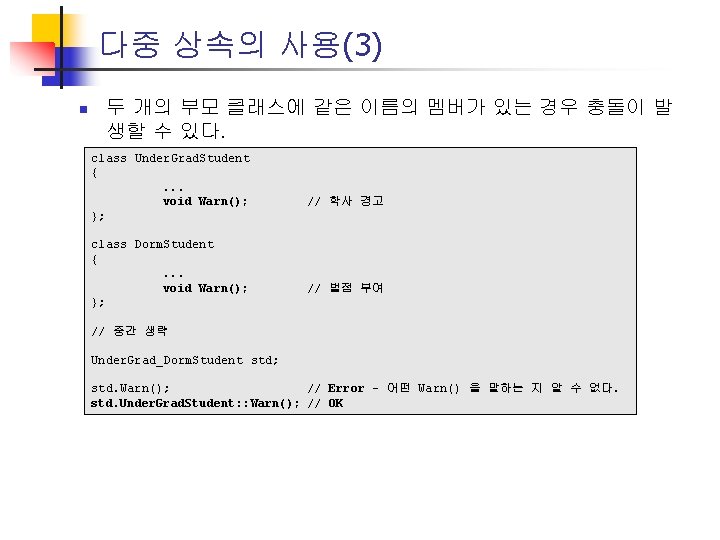
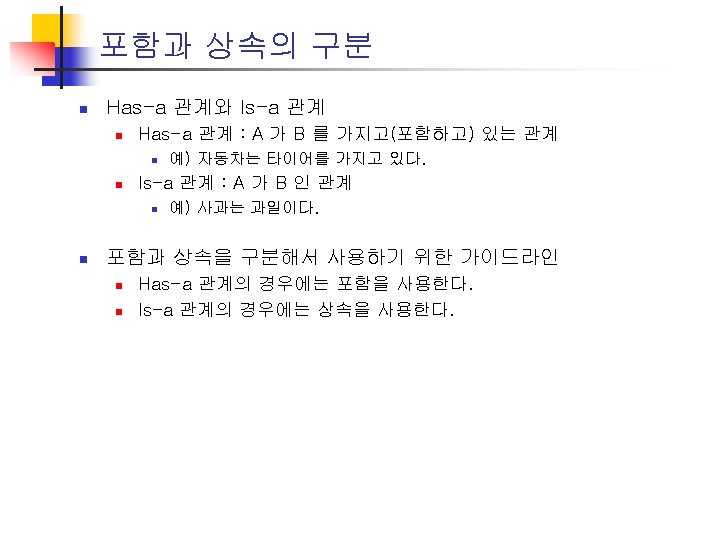