1 CSCI 104 Splay Trees Mark Redekopp 2
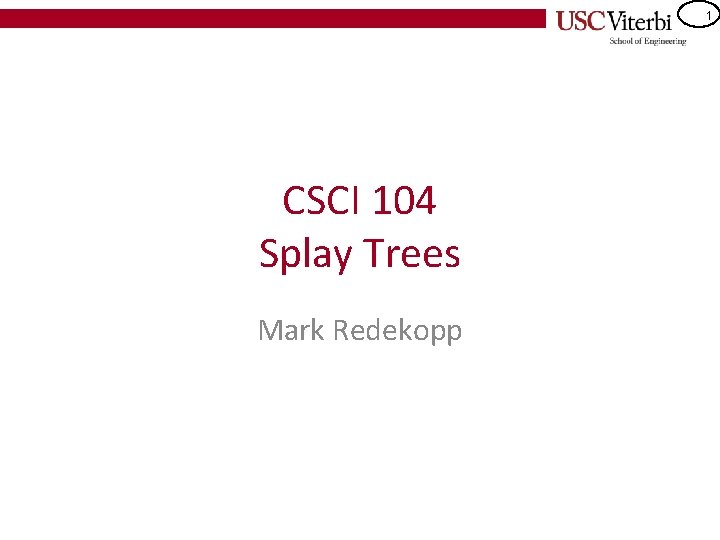
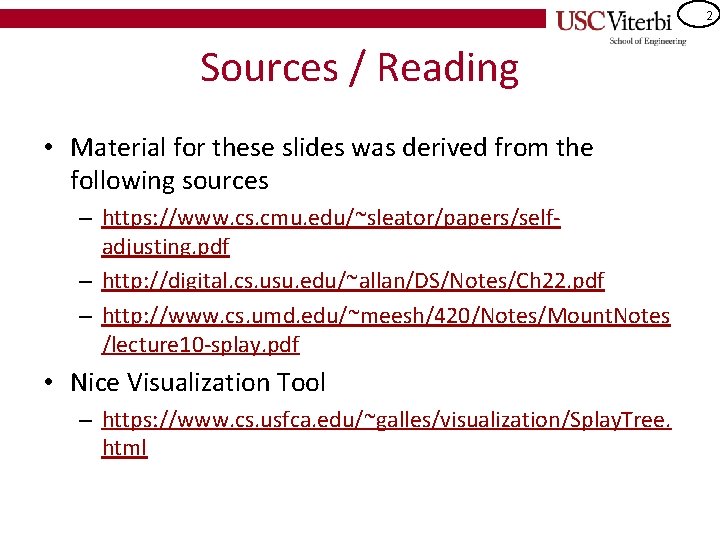
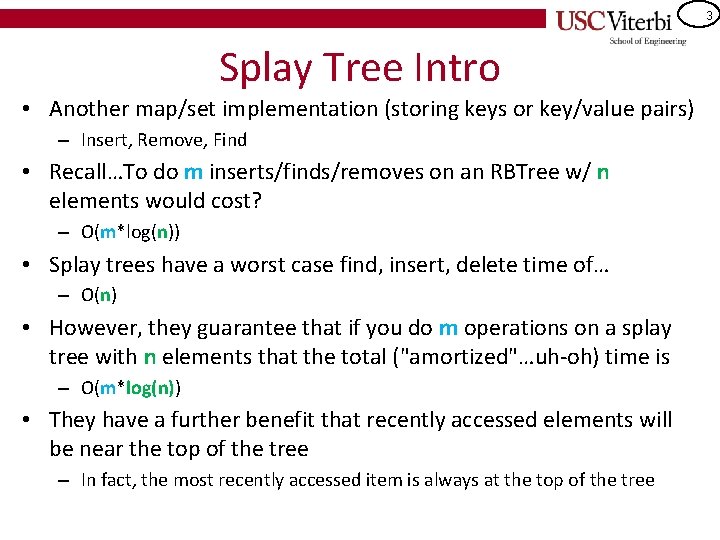
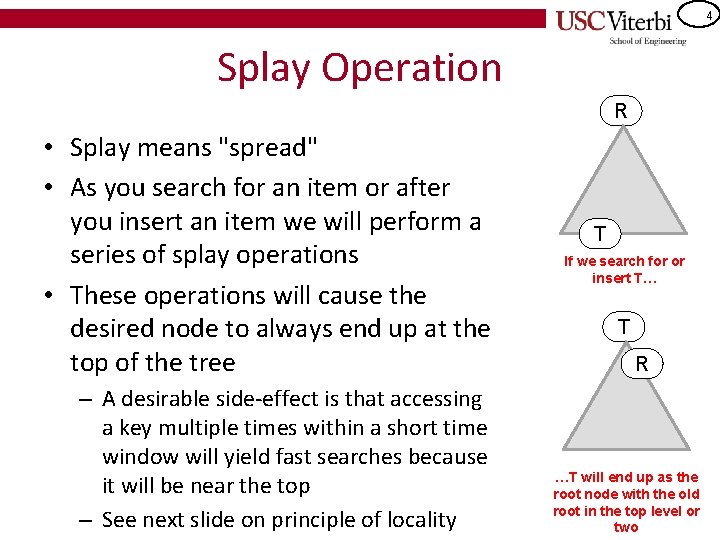
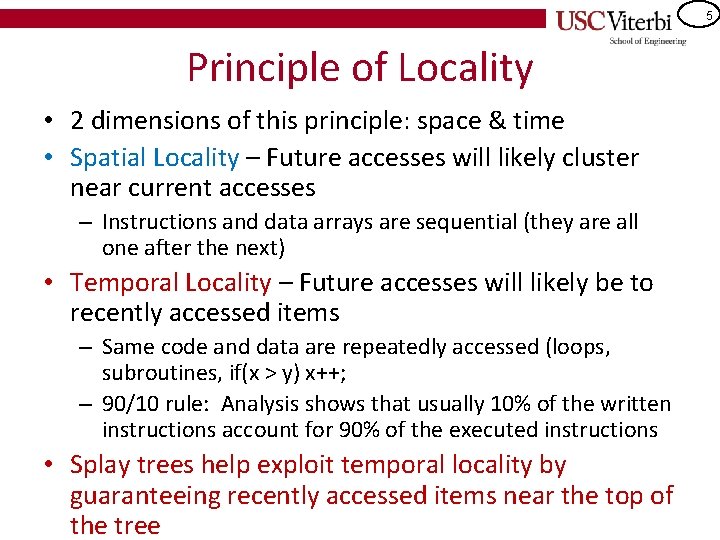
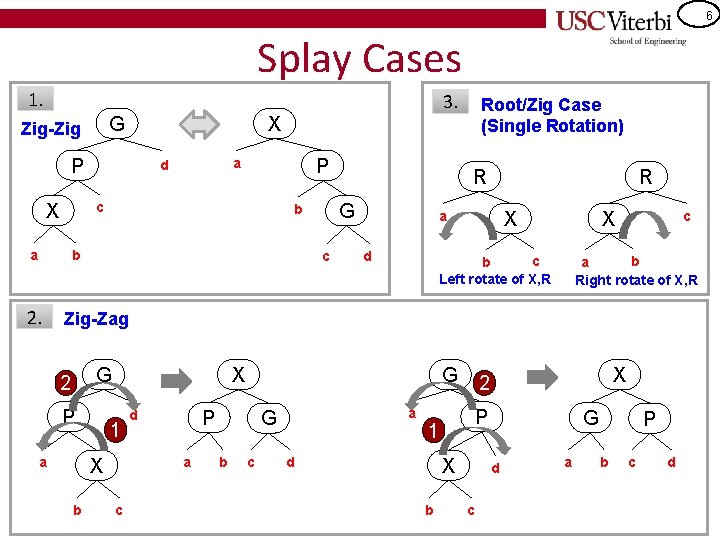
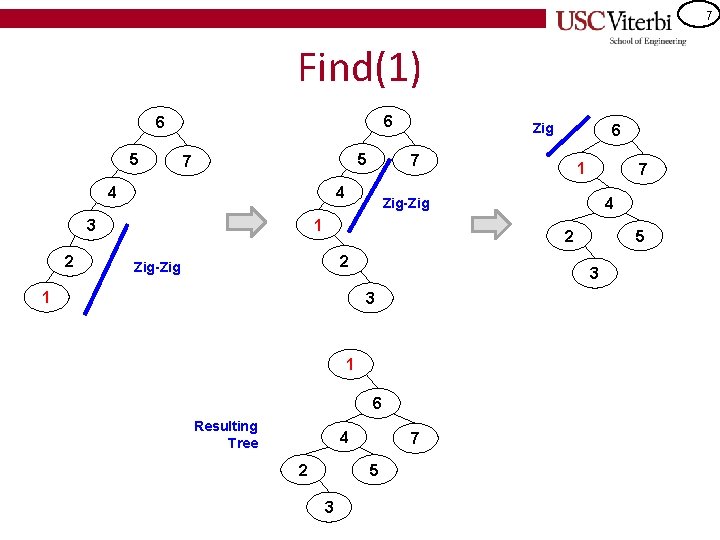
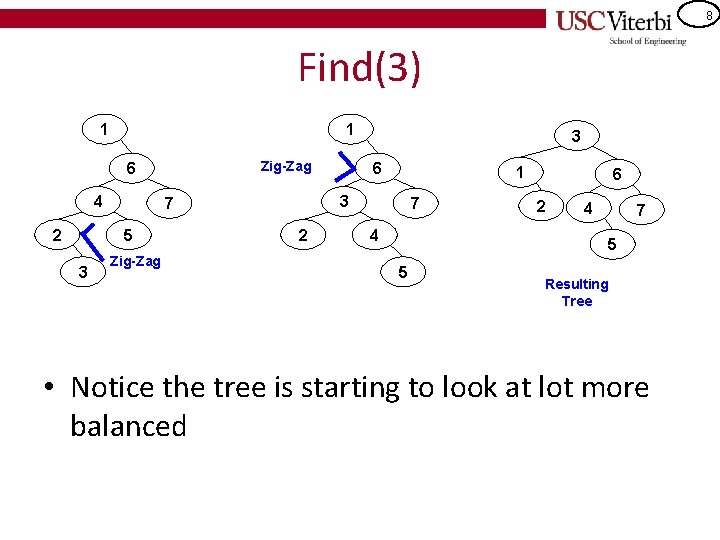
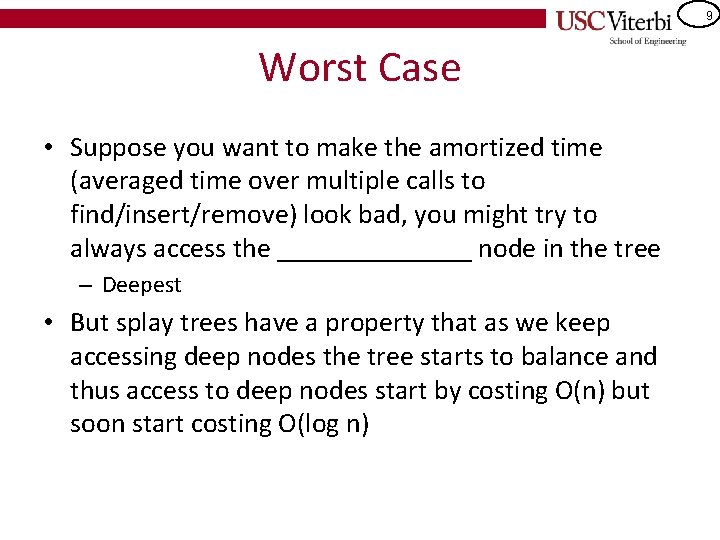
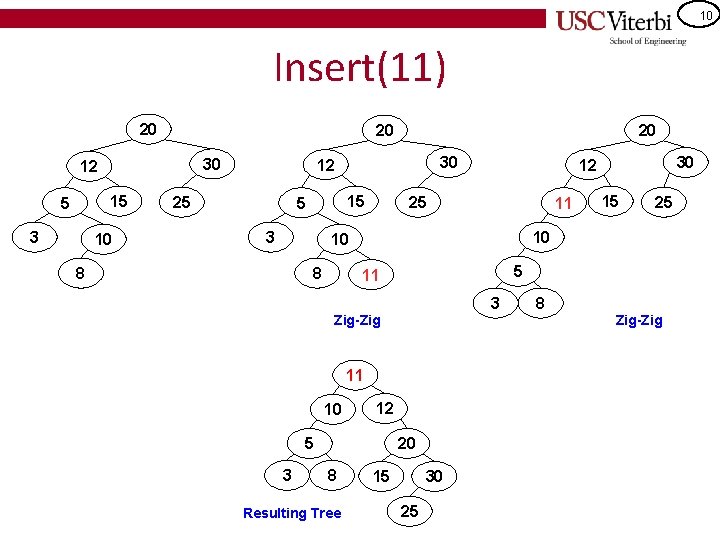
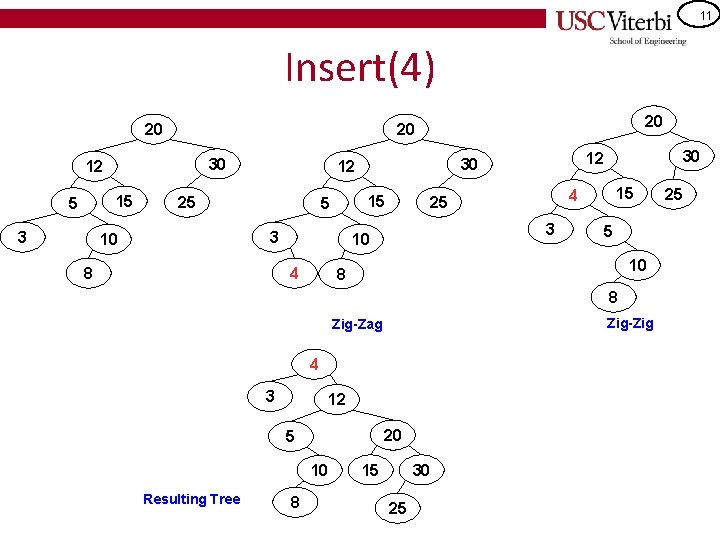
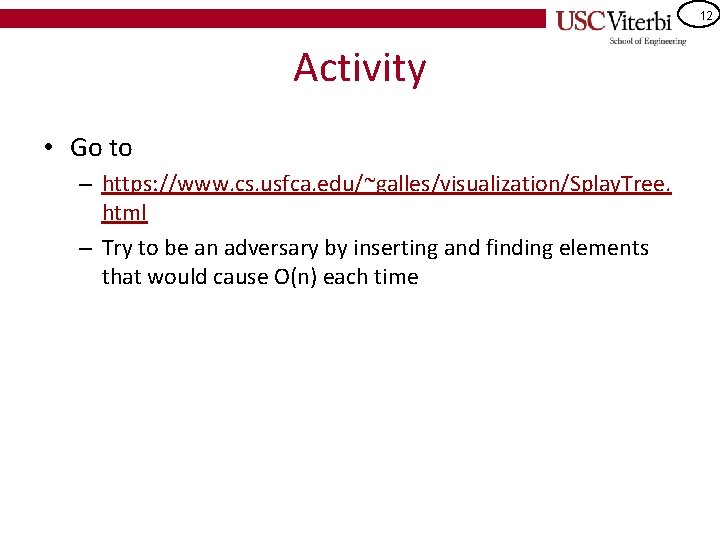
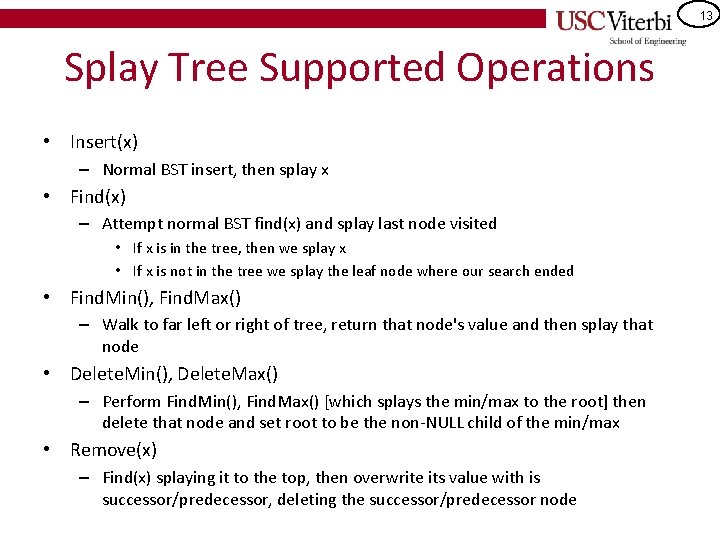
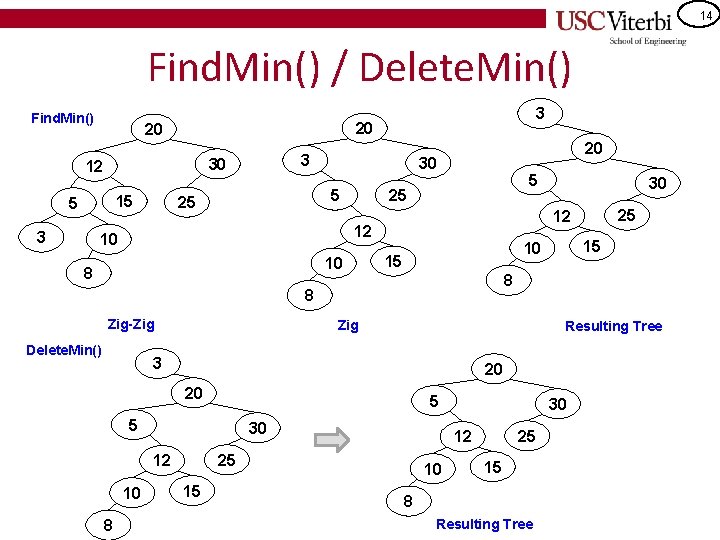
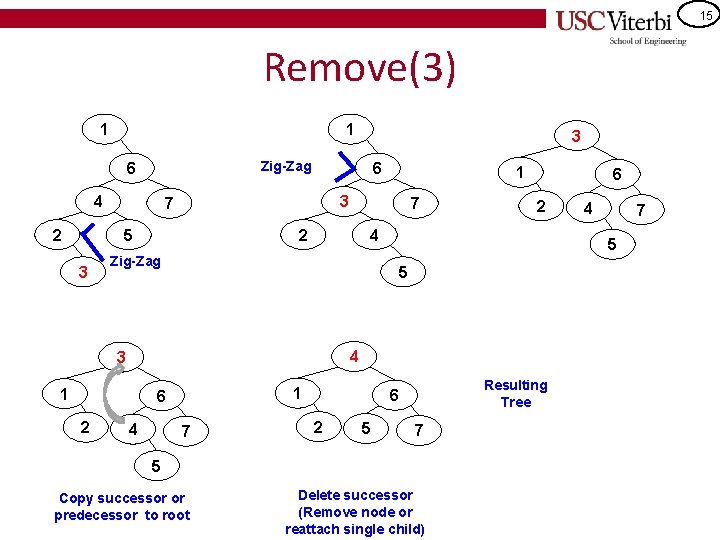
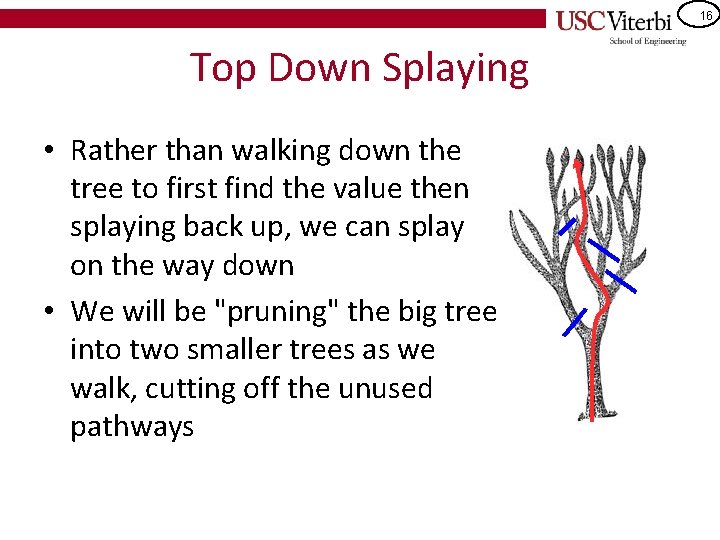
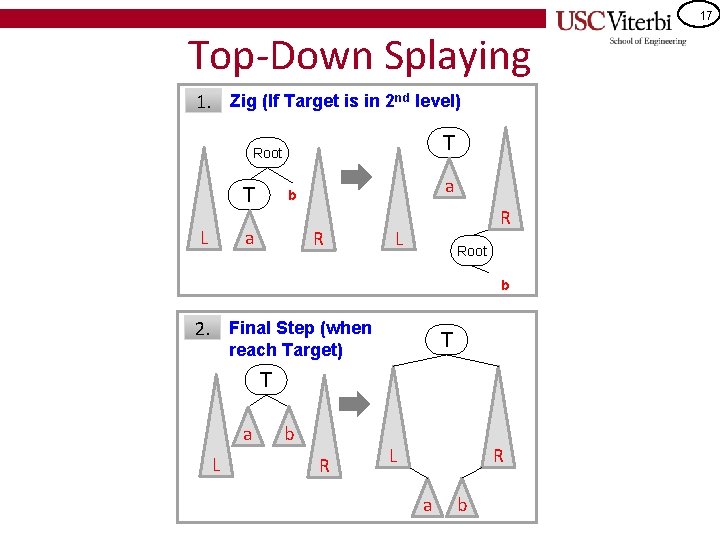
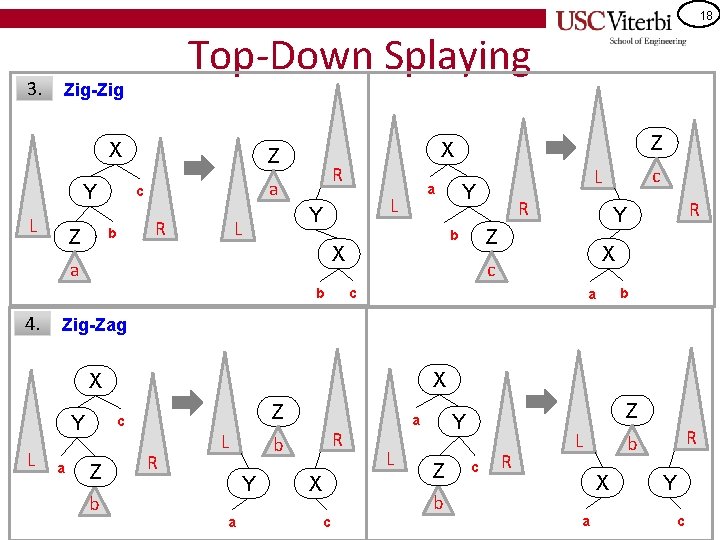
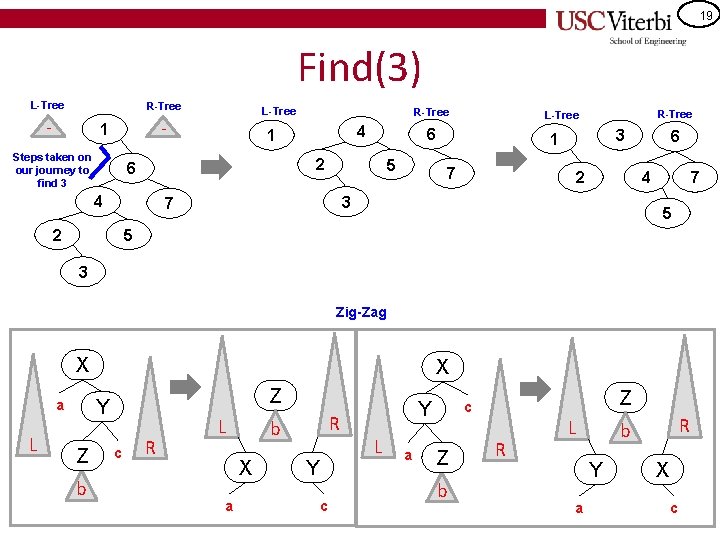
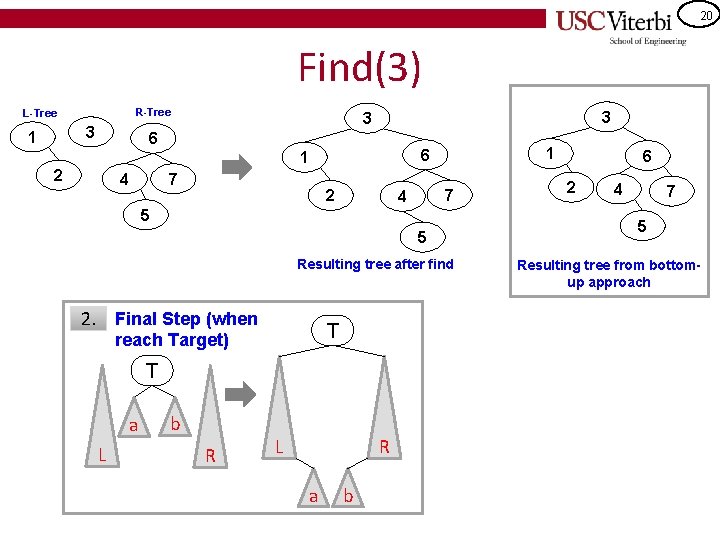
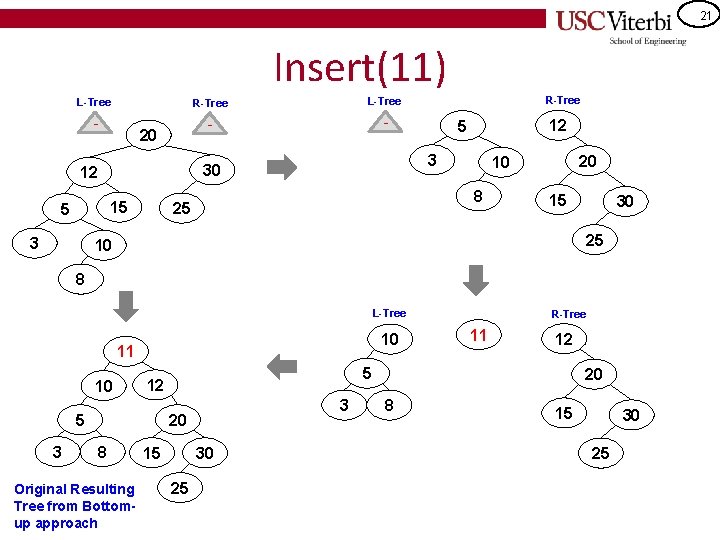
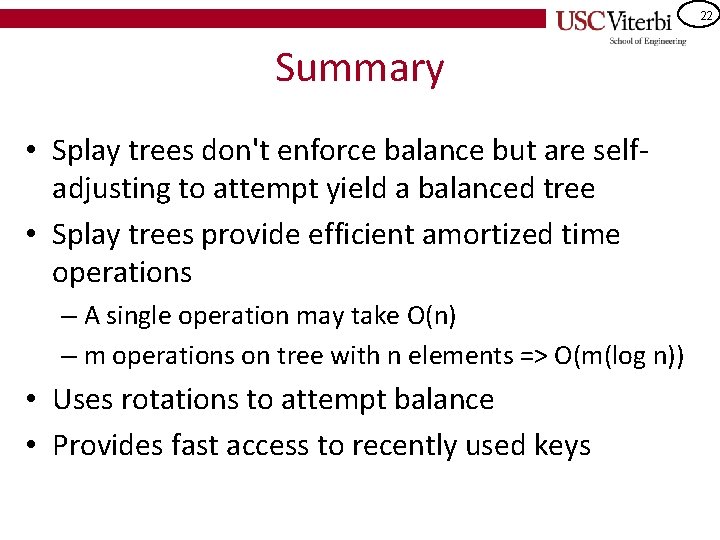
- Slides: 22
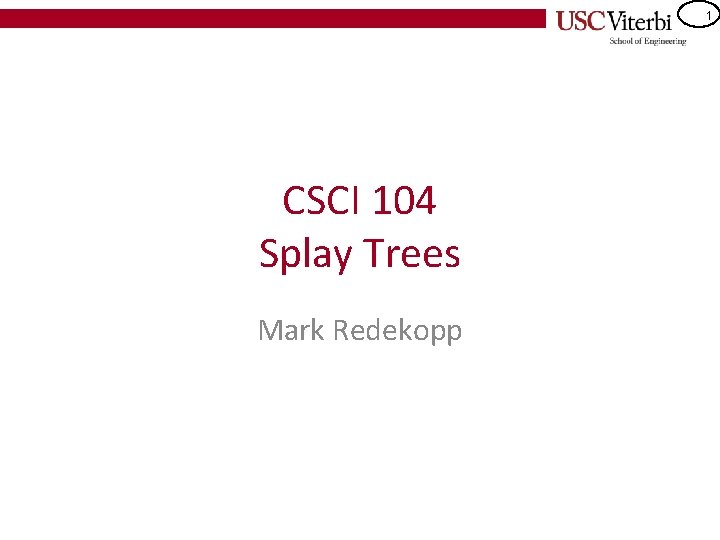
1 CSCI 104 Splay Trees Mark Redekopp
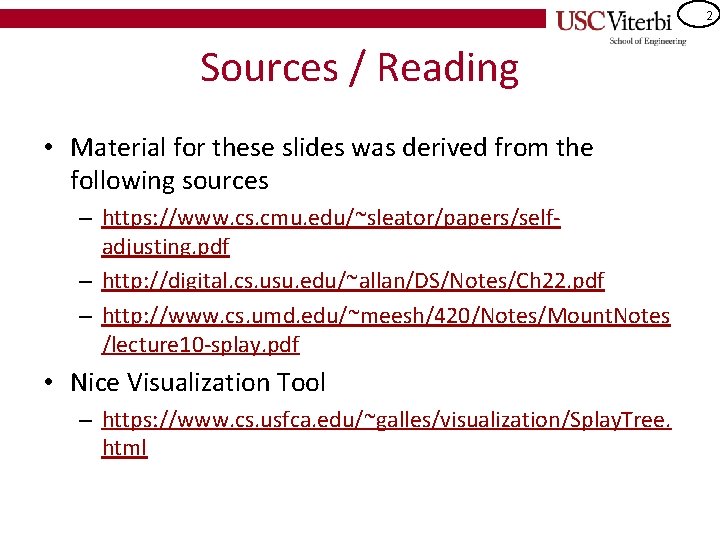
2 Sources / Reading • Material for these slides was derived from the following sources – https: //www. cs. cmu. edu/~sleator/papers/selfadjusting. pdf – http: //digital. cs. usu. edu/~allan/DS/Notes/Ch 22. pdf – http: //www. cs. umd. edu/~meesh/420/Notes/Mount. Notes /lecture 10 -splay. pdf • Nice Visualization Tool – https: //www. cs. usfca. edu/~galles/visualization/Splay. Tree. html
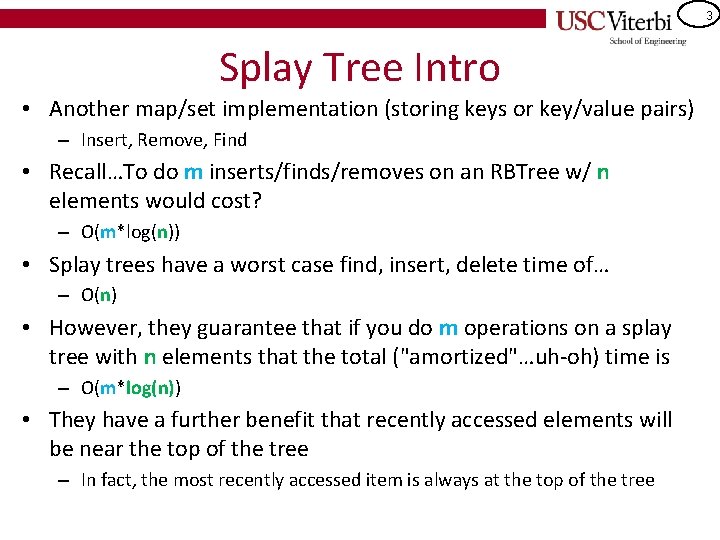
3 Splay Tree Intro • Another map/set implementation (storing keys or key/value pairs) – Insert, Remove, Find • Recall…To do m inserts/finds/removes on an RBTree w/ n elements would cost? – O(m*log(n)) • Splay trees have a worst case find, insert, delete time of… – O(n) • However, they guarantee that if you do m operations on a splay tree with n elements that the total ("amortized"…uh-oh) time is – O(m*log(n)) • They have a further benefit that recently accessed elements will be near the top of the tree – In fact, the most recently accessed item is always at the top of the tree
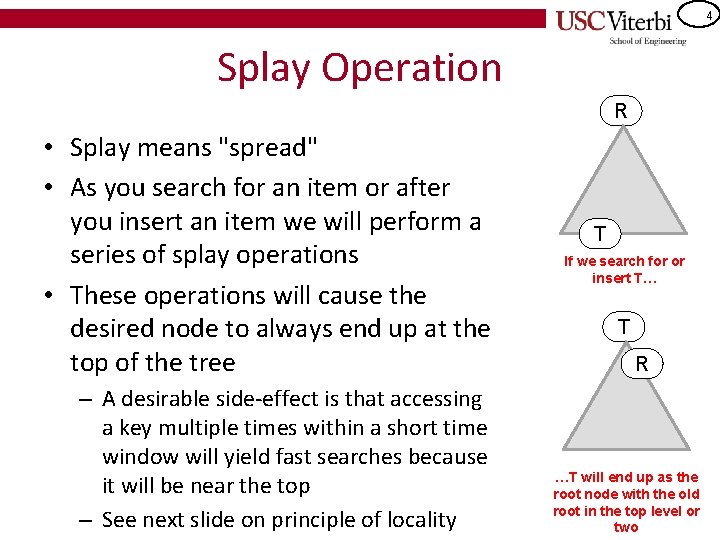
4 Splay Operation R • Splay means "spread" • As you search for an item or after you insert an item we will perform a series of splay operations • These operations will cause the desired node to always end up at the top of the tree – A desirable side-effect is that accessing a key multiple times within a short time window will yield fast searches because it will be near the top – See next slide on principle of locality T If we search for or insert T… T R …T will end up as the root node with the old root in the top level or two
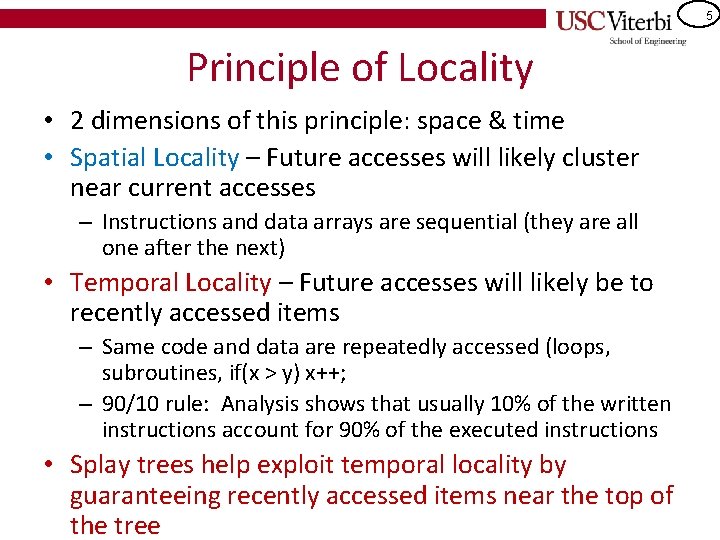
5 Principle of Locality • 2 dimensions of this principle: space & time • Spatial Locality – Future accesses will likely cluster near current accesses – Instructions and data arrays are sequential (they are all one after the next) • Temporal Locality – Future accesses will likely be to recently accessed items – Same code and data are repeatedly accessed (loops, subroutines, if(x > y) x++; – 90/10 rule: Analysis shows that usually 10% of the written instructions account for 90% of the executed instructions • Splay trees help exploit temporal locality by guaranteeing recently accessed items near the top of the tree
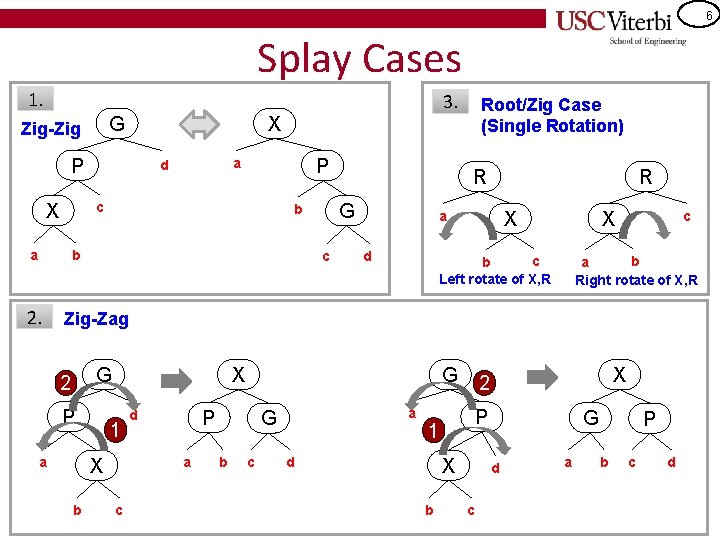
6 Splay Cases 1. G Zig-Zig 3. X P P a d c X a b 2. R G b c Root/Zig Case (Single Rotation) R X a d X c b Left rotate of X, R c b a Right rotate of X, R Zig-Zag G 2 P X 1 b P a X a d c G a G b c P 1 d X b X 2 G d c a P b c d
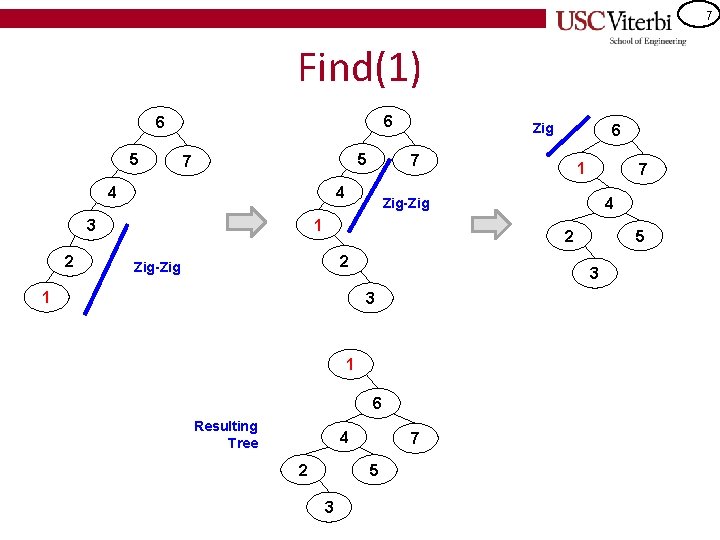
7 Find(1) 6 6 5 5 7 2 1 7 4 Zig-Zig 1 3 6 7 4 4 Zig 2 2 Zig-Zig 1 3 3 1 6 Resulting Tree 4 2 7 5 3 5
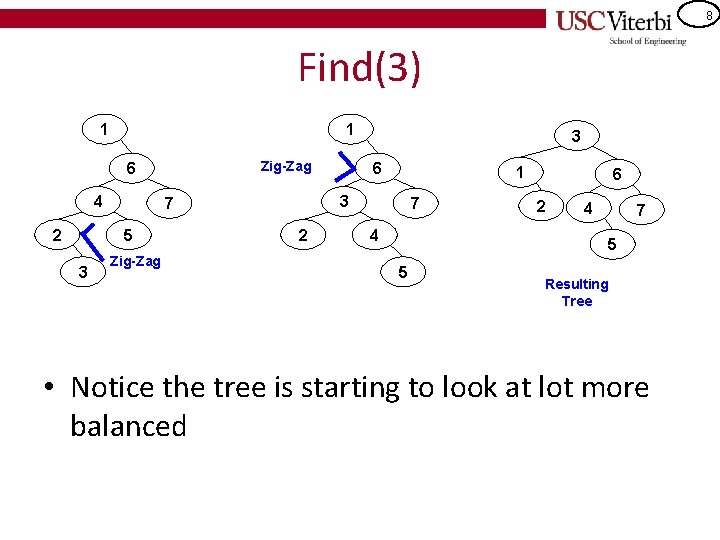
8 Find(3) 1 1 Zig-Zag 6 4 2 3 Zig-Zag 6 1 3 7 5 3 2 7 4 6 2 4 7 5 5 Resulting Tree • Notice the tree is starting to look at lot more balanced
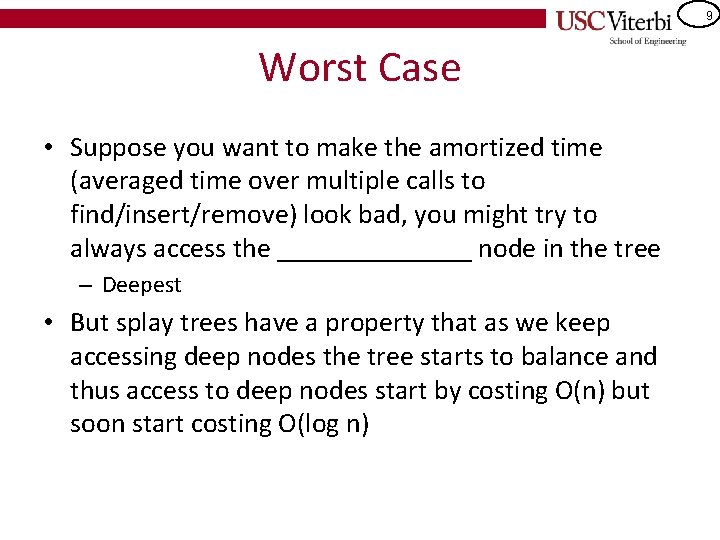
9 Worst Case • Suppose you want to make the amortized time (averaged time over multiple calls to find/insert/remove) look bad, you might try to always access the _______ node in the tree – Deepest • But splay trees have a property that as we keep accessing deep nodes the tree starts to balance and thus access to deep nodes start by costing O(n) but soon start costing O(log n)
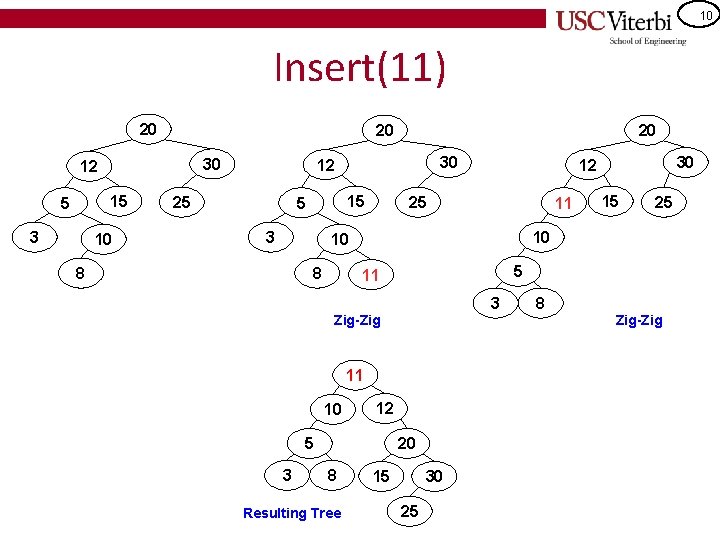
10 Insert(11) 20 20 30 12 15 5 3 10 20 30 12 25 15 5 3 25 11 8 3 Zig-Zig 11 12 5 3 20 8 Resulting Tree 25 5 11 10 15 10 10 8 30 12 15 30 25 8 Zig-Zig
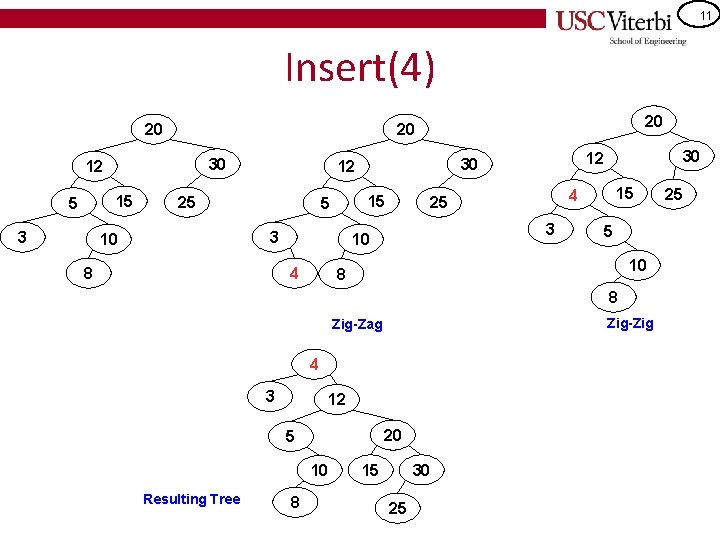
11 Insert(4) 20 30 12 15 5 3 20 20 25 15 5 3 10 8 4 15 4 25 3 10 30 12 5 10 8 8 Zig-Zig Zig-Zag 4 3 12 20 5 10 Resulting Tree 8 15 30 25 25
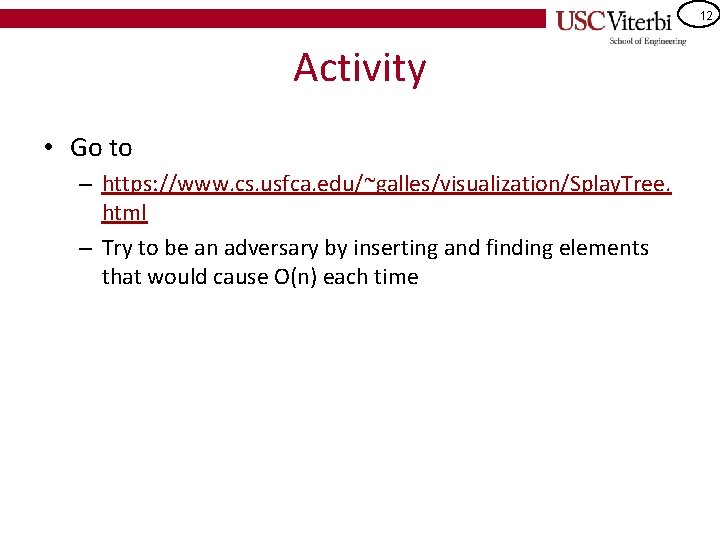
12 Activity • Go to – https: //www. cs. usfca. edu/~galles/visualization/Splay. Tree. html – Try to be an adversary by inserting and finding elements that would cause O(n) each time
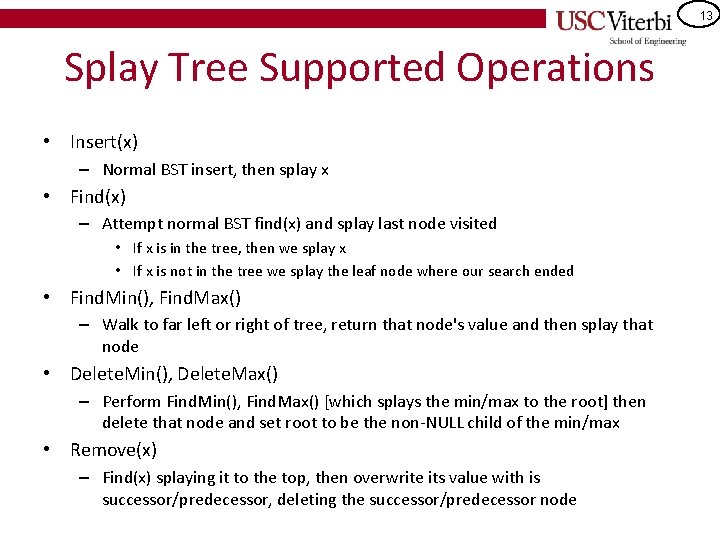
13 Splay Tree Supported Operations • Insert(x) – Normal BST insert, then splay x • Find(x) – Attempt normal BST find(x) and splay last node visited • If x is in the tree, then we splay x • If x is not in the tree we splay the leaf node where our search ended • Find. Min(), Find. Max() – Walk to far left or right of tree, return that node's value and then splay that node • Delete. Min(), Delete. Max() – Perform Find. Min(), Find. Max() [which splays the min/max to the root] then delete that node and set root to be the non-NULL child of the min/max • Remove(x) – Find(x) splaying it to the top, then overwrite its value with is successor/predecessor, deleting the successor/predecessor node
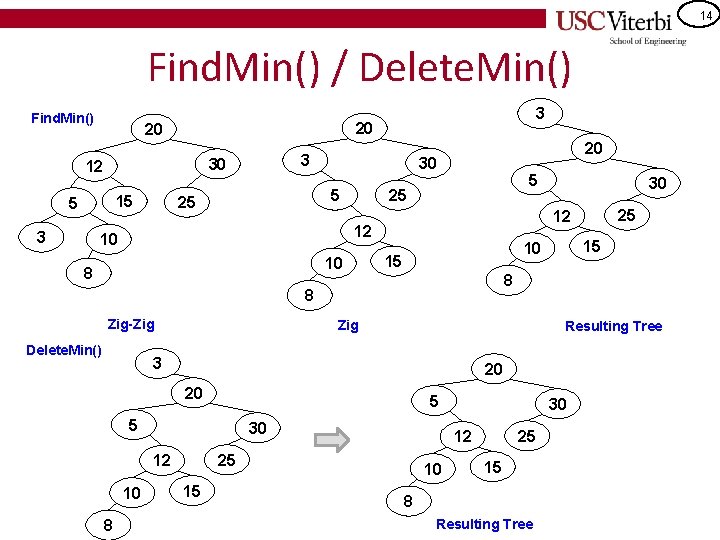
14 Find. Min() / Delete. Min() Find. Min() 20 20 15 3 3 30 12 5 3 30 5 25 20 5 25 10 8 Delete. Min() 15 8 Zig Resulting Tree 3 20 20 5 5 30 8 15 30 25 12 10 15 10 8 Zig-Zig 25 12 12 10 30 10 15 8 Resulting Tree
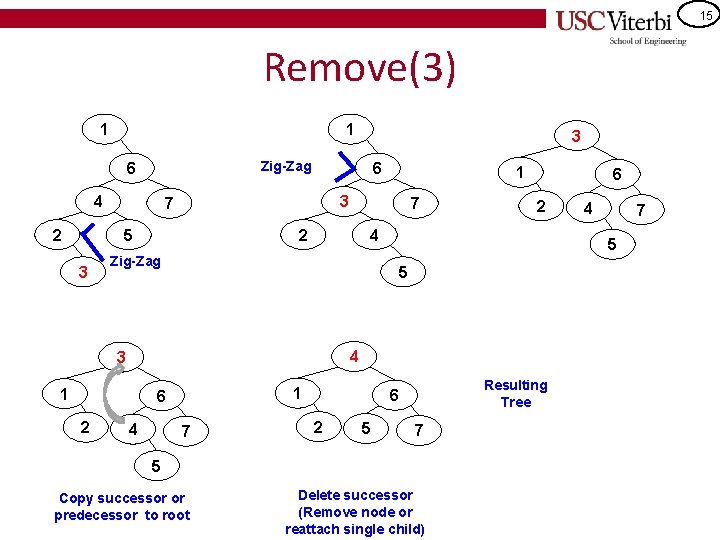
15 Remove(3) 1 1 Zig-Zag 6 4 2 6 7 2 6 2 4 Zig-Zag 4 1 1 6 4 7 Resulting Tree 6 2 5 7 5 Copy successor or predecessor to root 4 7 5 5 3 2 1 3 7 5 3 3 Delete successor (Remove node or reattach single child)
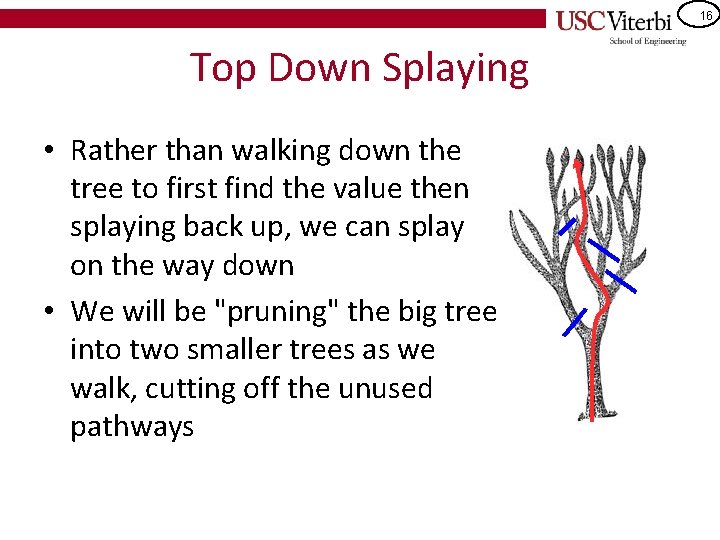
16 Top Down Splaying • Rather than walking down the tree to first find the value then splaying back up, we can splay on the way down • We will be "pruning" the big tree into two smaller trees as we walk, cutting off the unused pathways
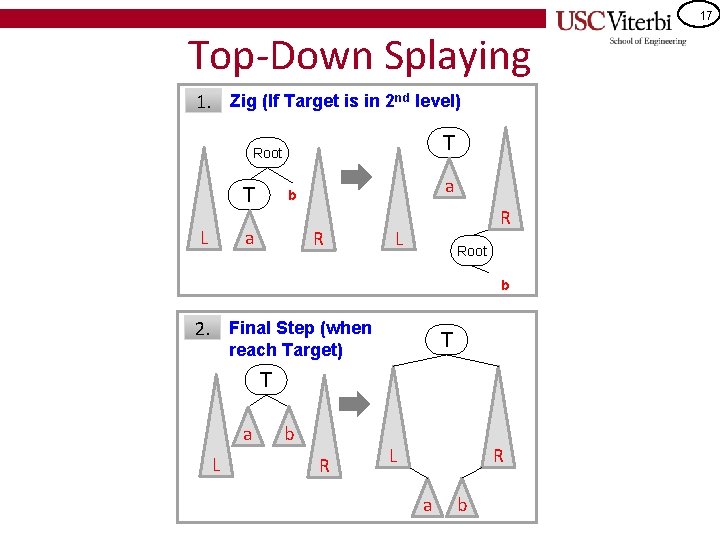
17 Top-Down Splaying 1. Zig (If Target is in 2 nd level) T Root T a L a b R R L Root b 2. Final Step (when T reach Target) T a L b R L R a b
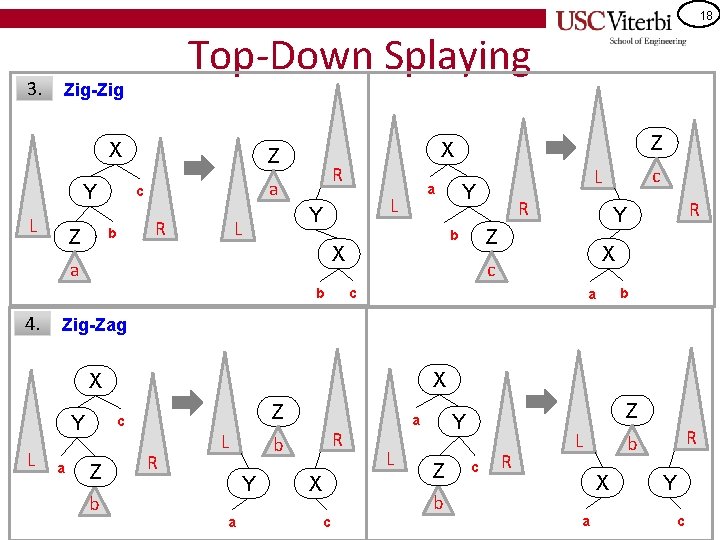
18 3. Top-Down Splaying Zig-Zig X Y L b Z R a c R X a X c a b Zig-Zag X X Y L R Y c b 4. R Z b c L Y a L Y L Z X Z a Z c Z b R L Y a R b X c L Z Y a Z b c R L X a R b Y c
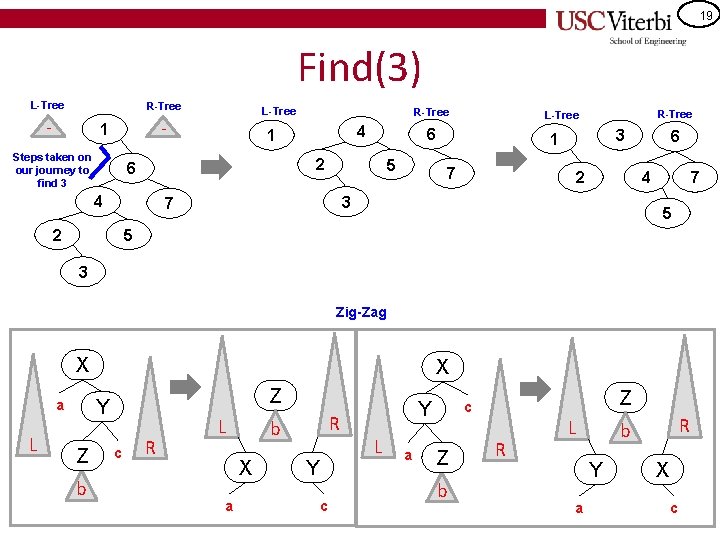
19 Find(3) L-Tree R-Tree - L-Tree - 1 Steps taken on our journey to find 3 R-Tree 4 1 4 5 3 1 7 2 6 4 3 7 2 6 R-Tree L-Tree 7 5 5 3 Zig-Zag X Z Y a L X Z b c R L X a R b Y c Y L a Z c Z b R L Y a R b X c
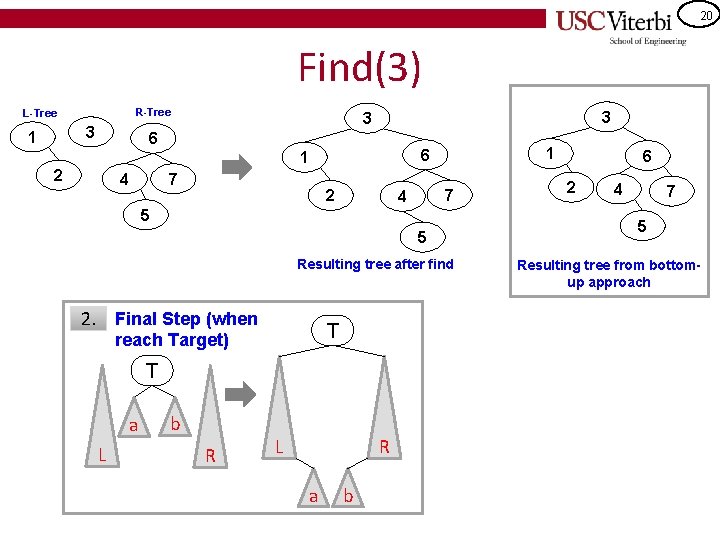
20 Find(3) R-Tree L-Tree 3 1 3 3 6 2 4 7 1 6 1 2 7 4 5 5 Resulting tree after find 2. Final Step (when T reach Target) T a L b R L R a b 6 2 4 7 5 Resulting tree from bottomup approach
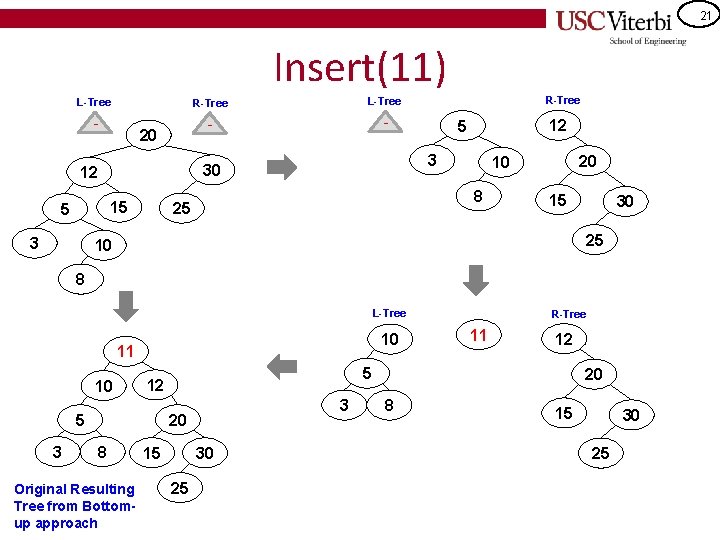
21 Insert(11) L-Tree R-Tree L-Tree - - - 20 15 5 3 12 5 3 30 12 R-Tree 8 25 20 10 15 30 25 10 8 L-Tree 10 11 10 3 3 20 8 Original Resulting Tree from Bottomup approach 11 12 5 R-Tree 15 30 25 20 8 15 30 25
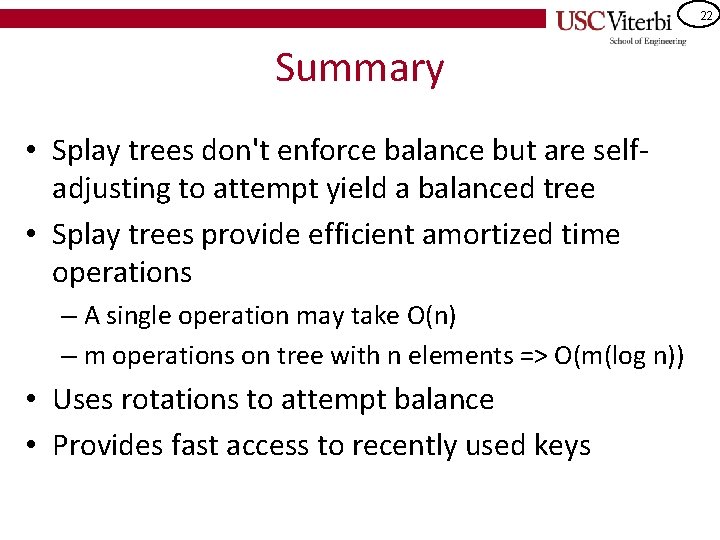
22 Summary • Splay trees don't enforce balance but are selfadjusting to attempt yield a balanced tree • Splay trees provide efficient amortized time operations – A single operation may take O(n) – m operations on tree with n elements => O(m(log n)) • Uses rotations to attempt balance • Provides fast access to recently used keys