1 Chapter 12 C Stream InputOutput Outline 12
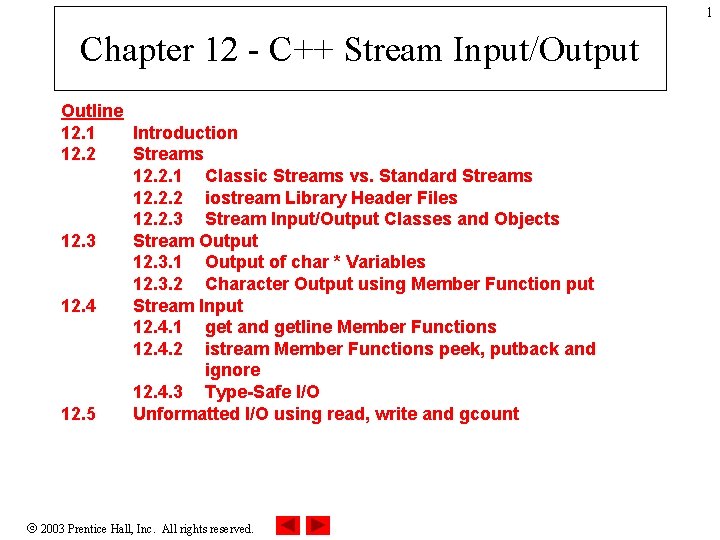
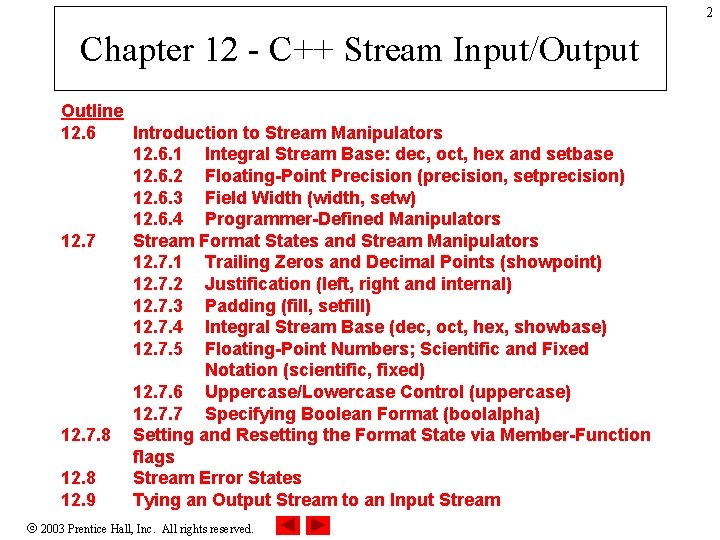
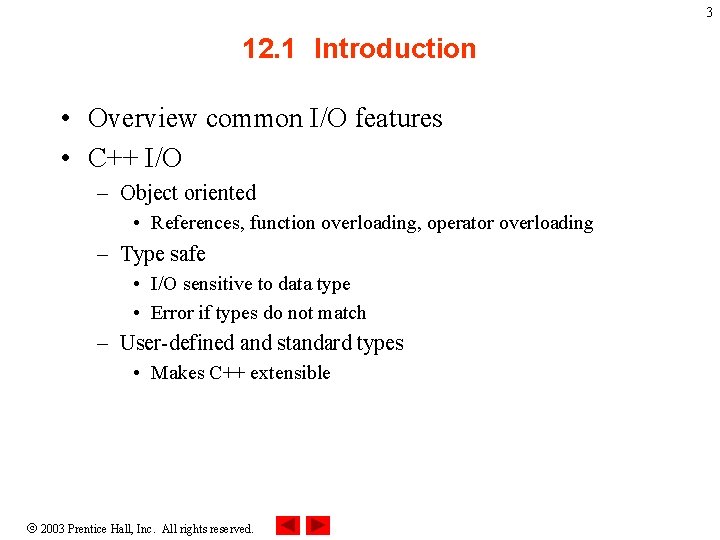
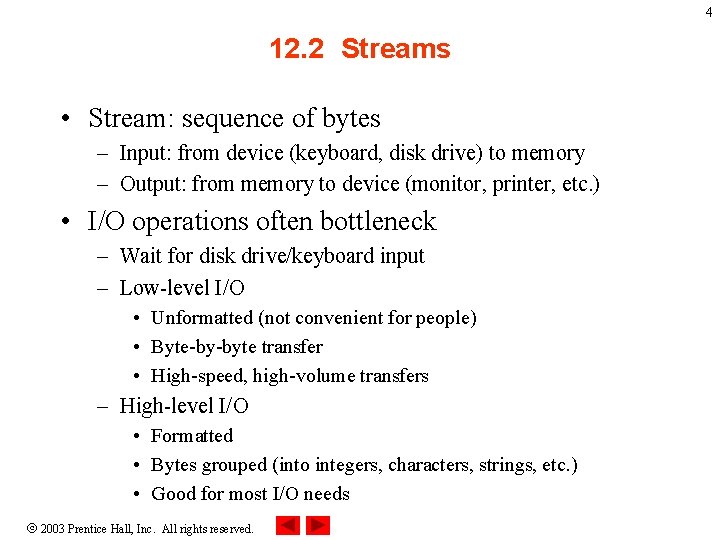
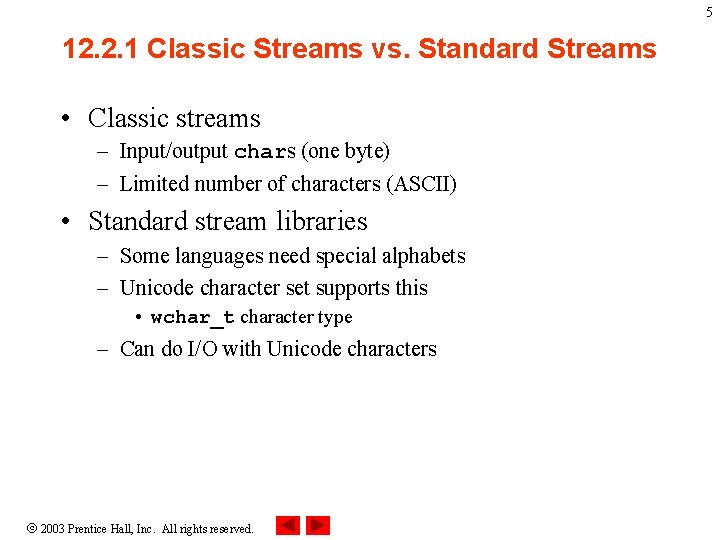
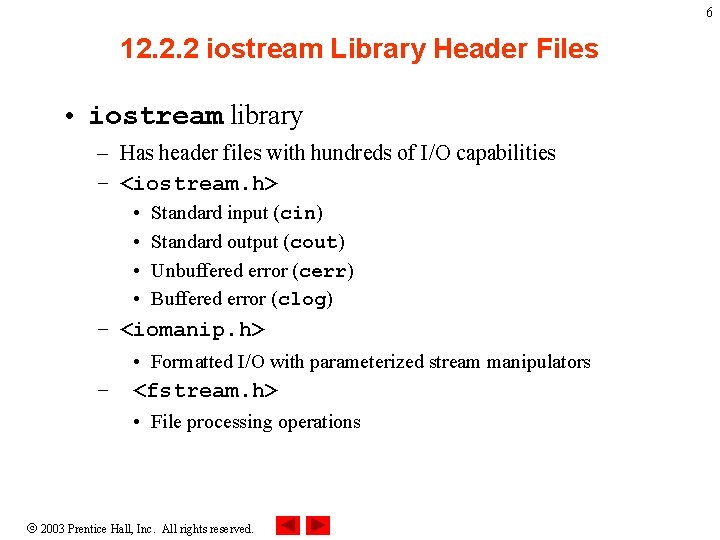
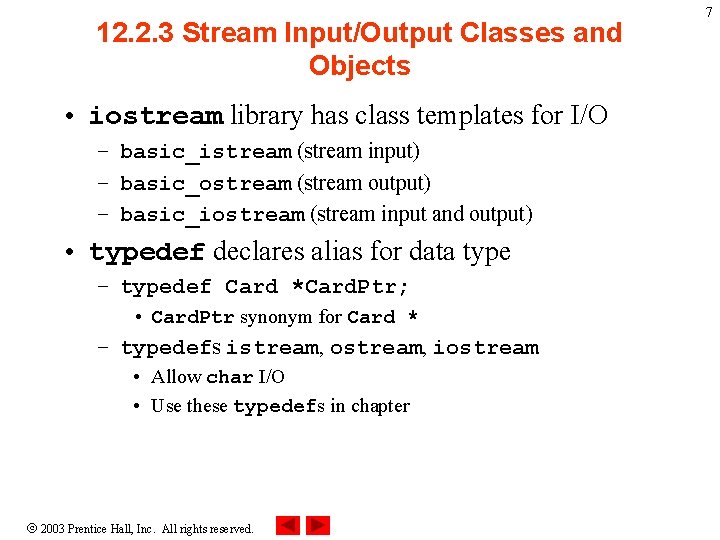
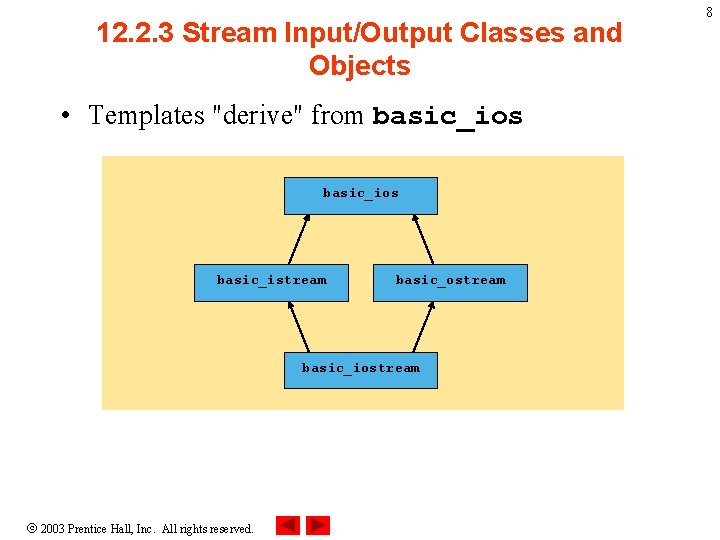
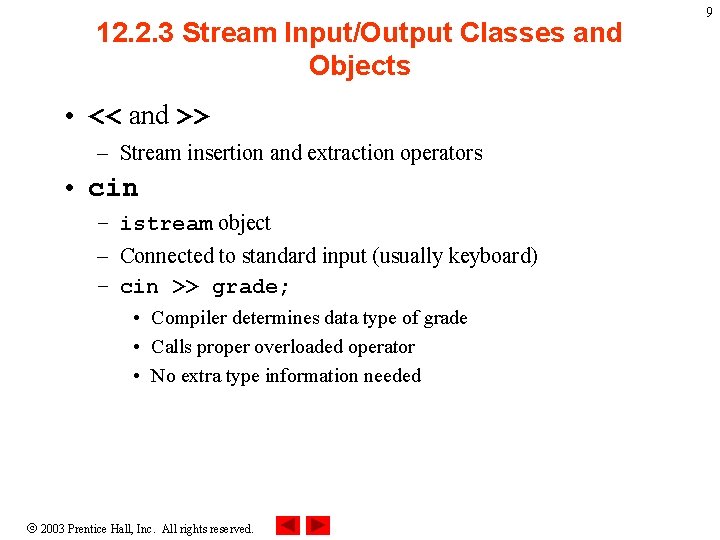
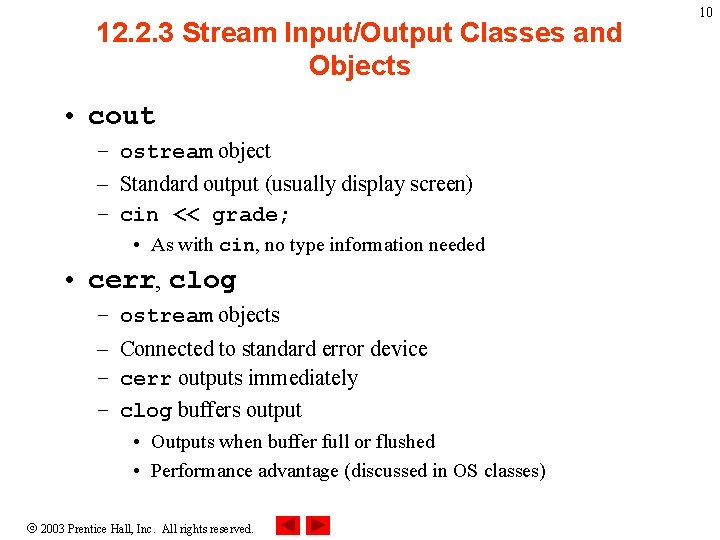
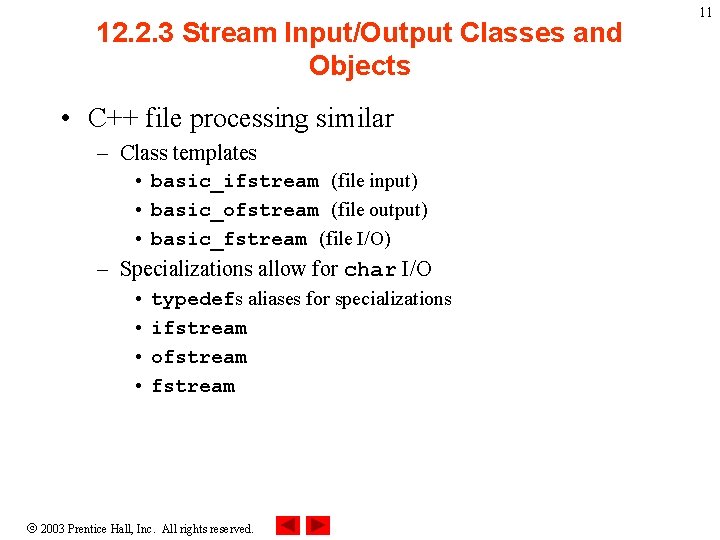
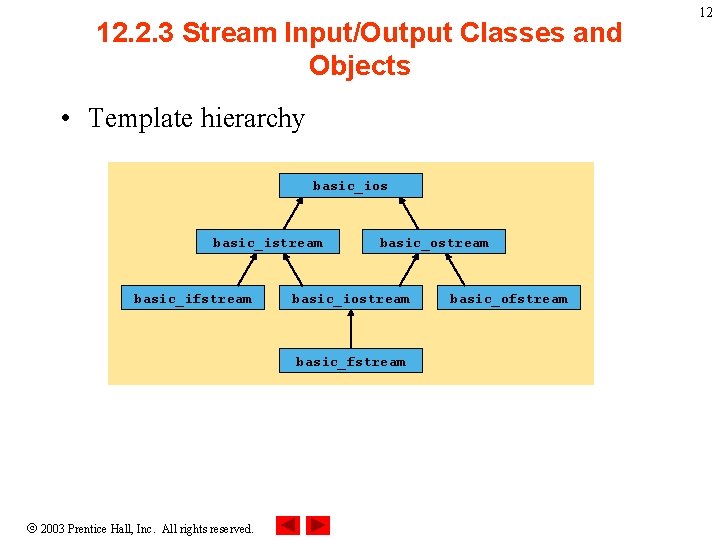
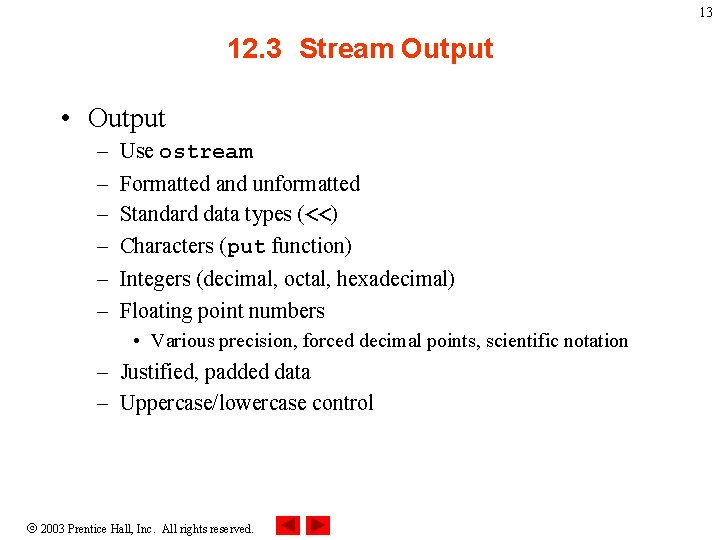
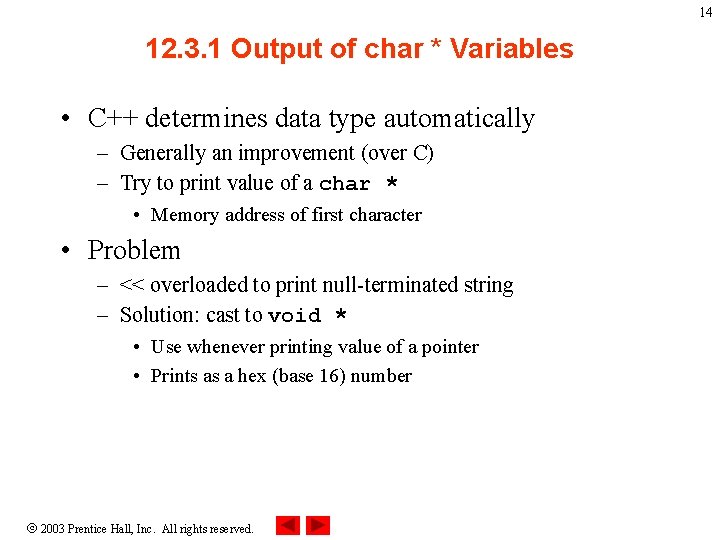
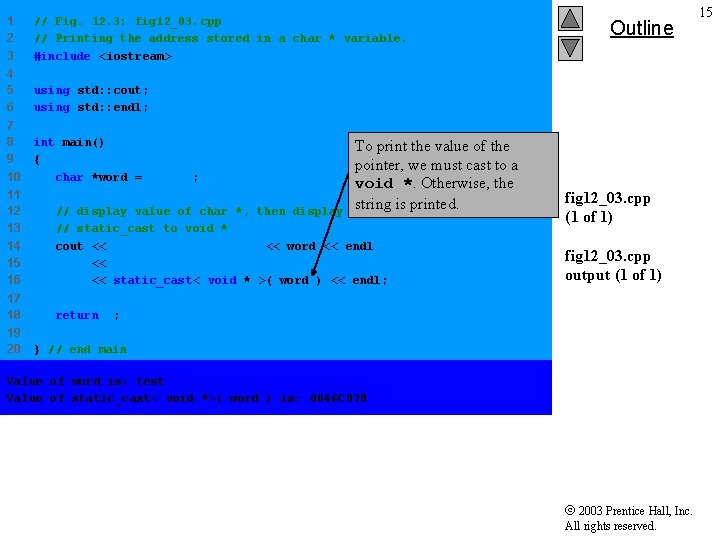
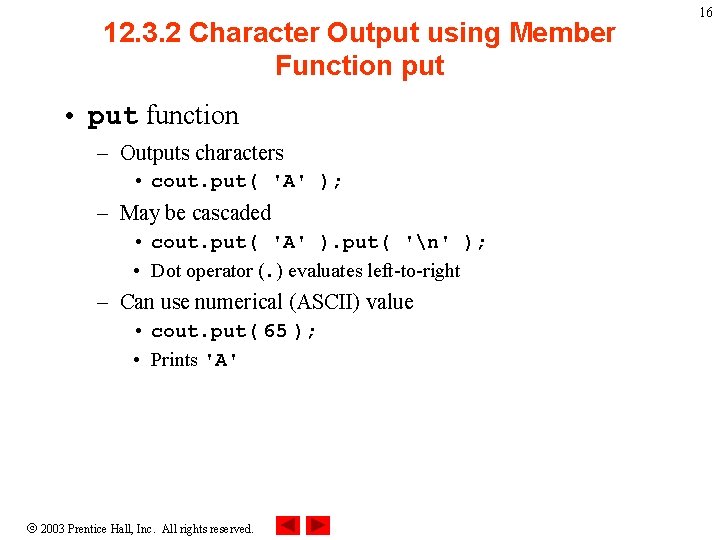
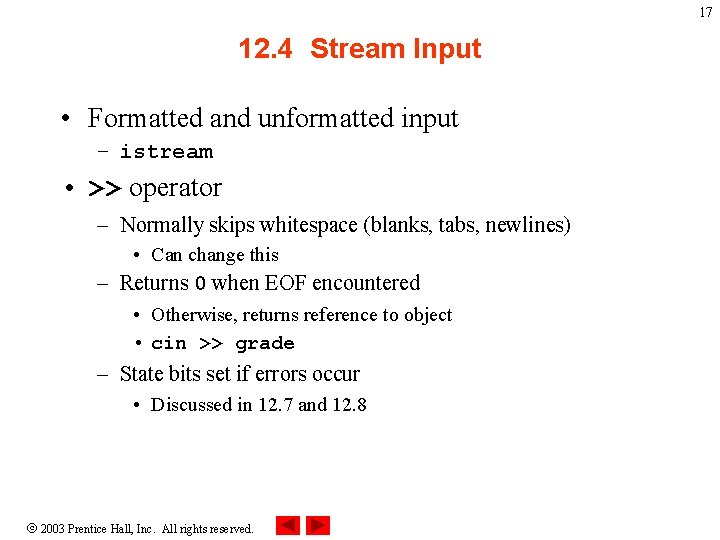
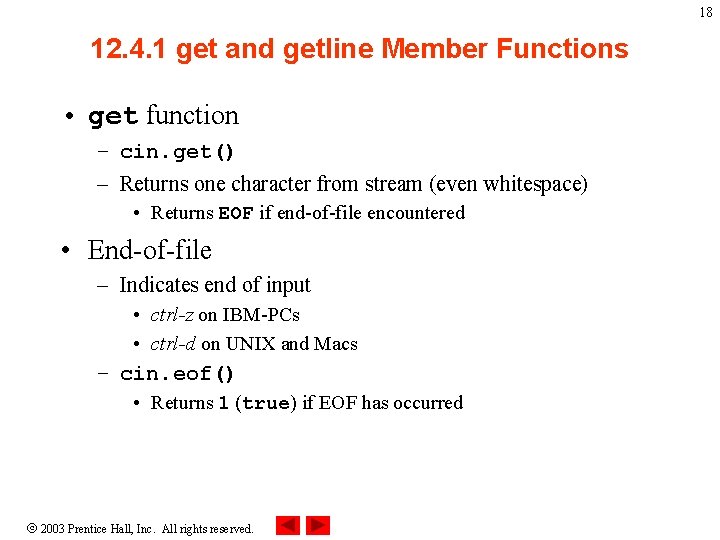
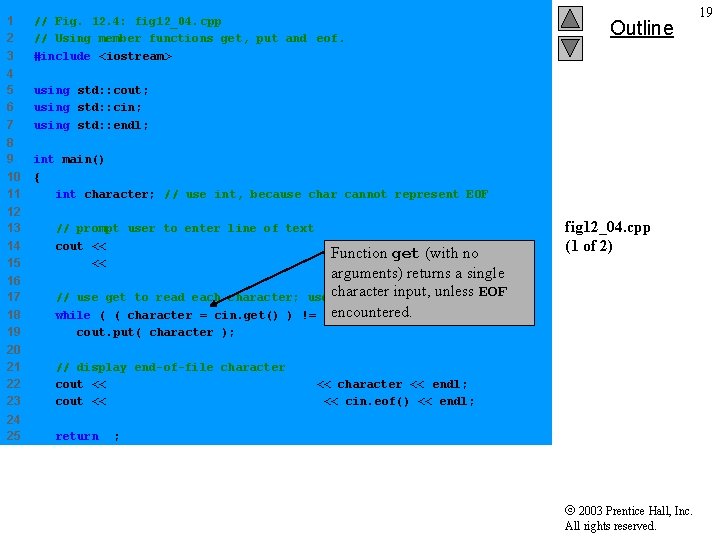
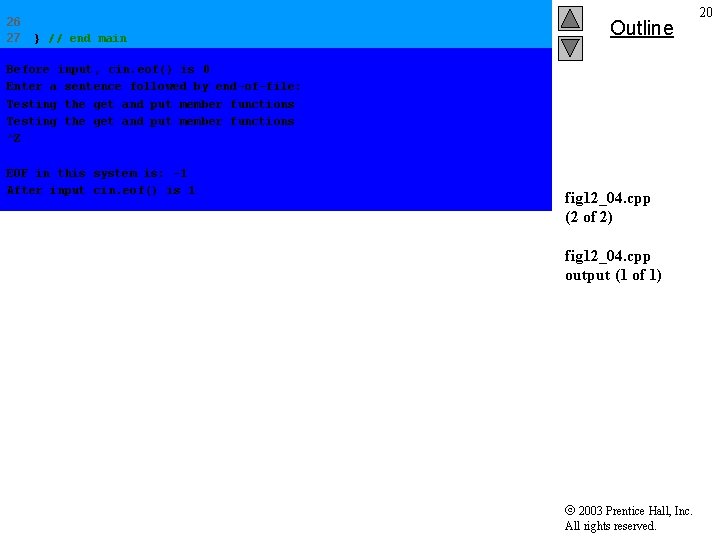
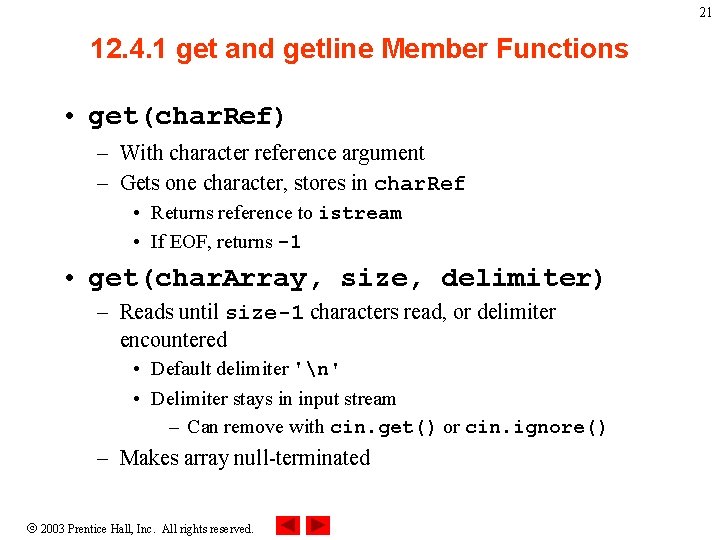
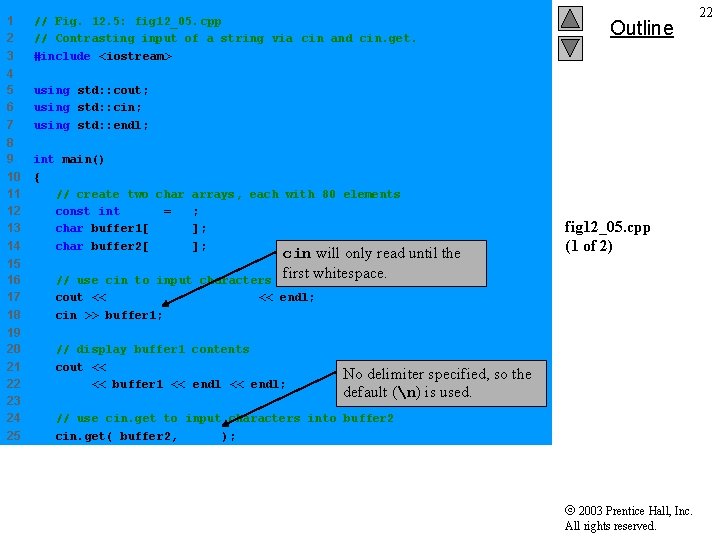
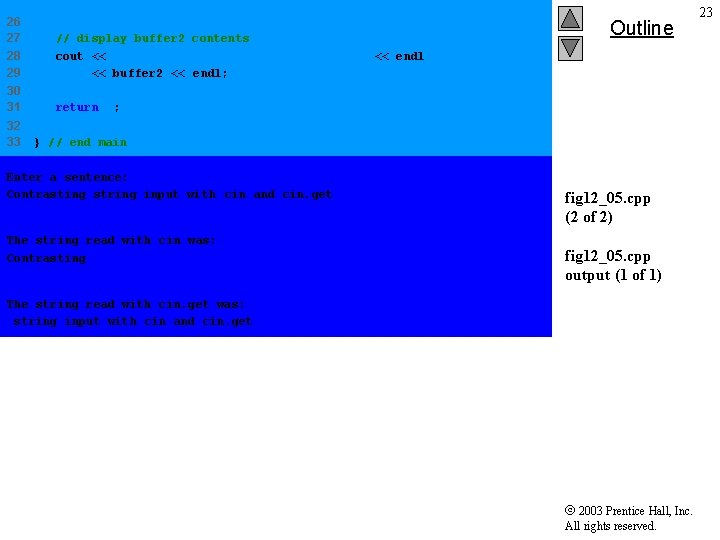
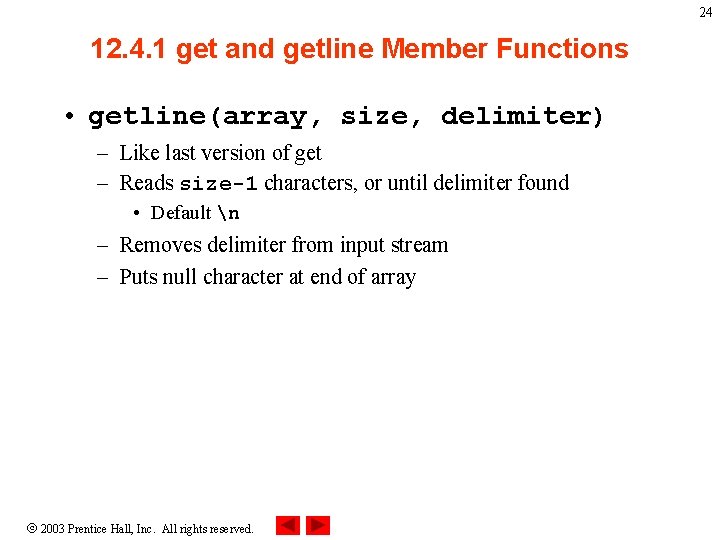
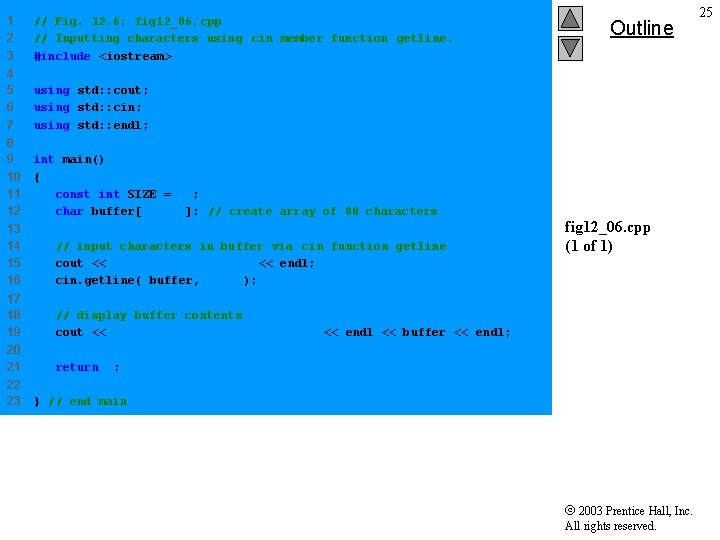
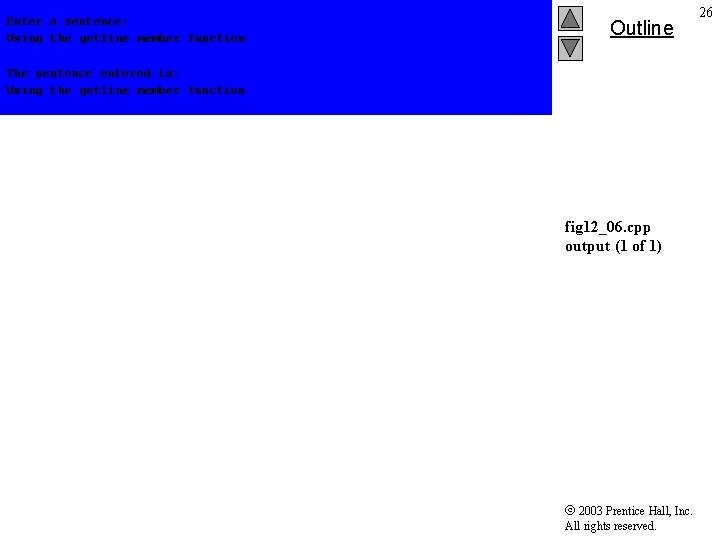
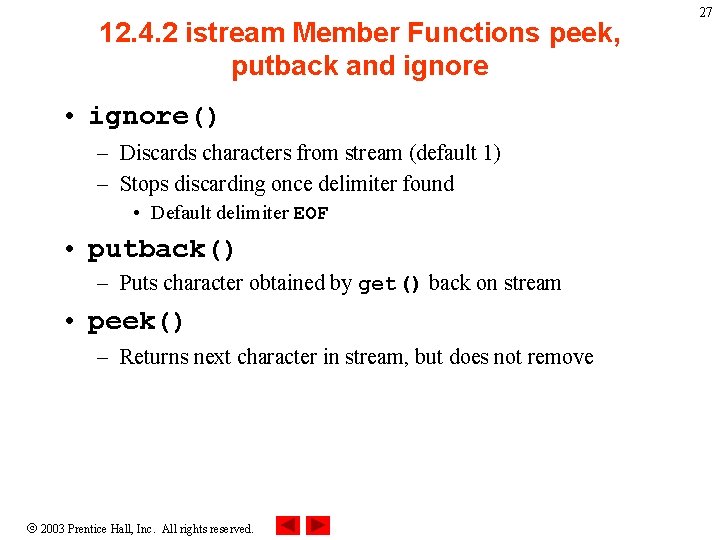
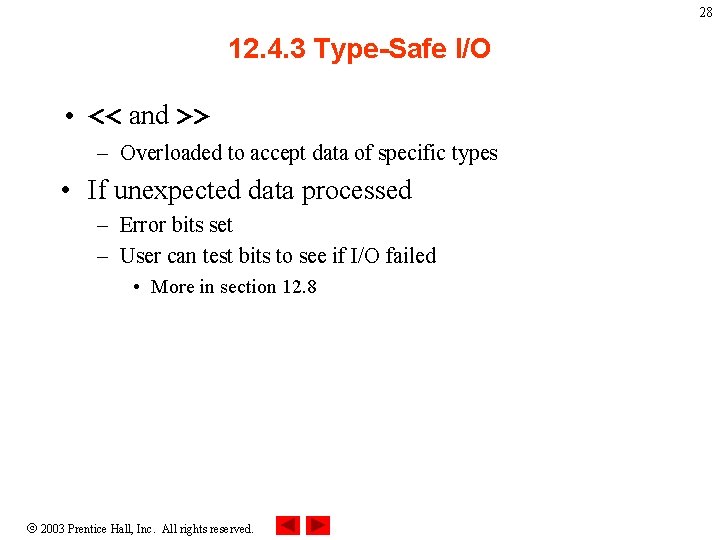
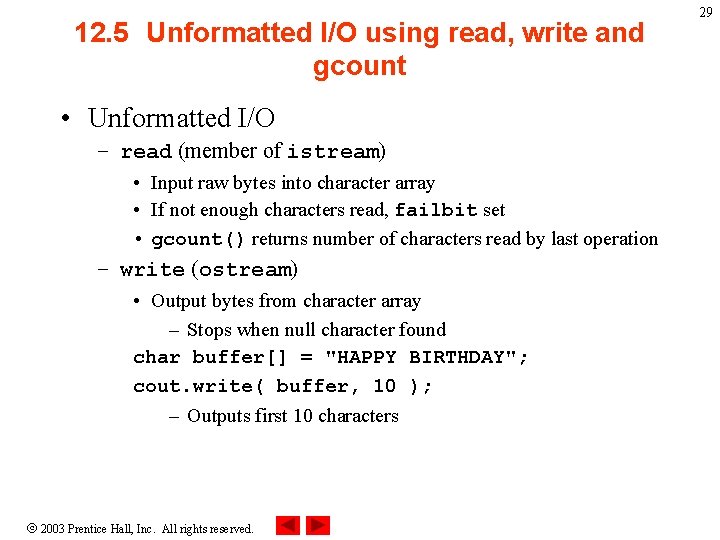
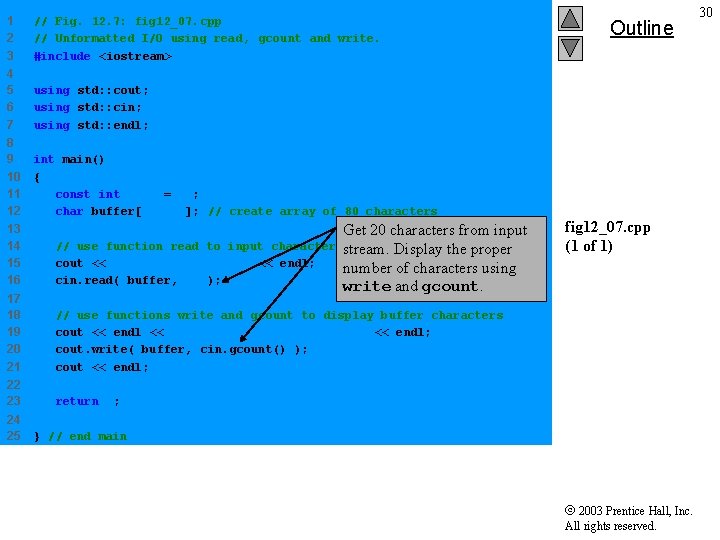
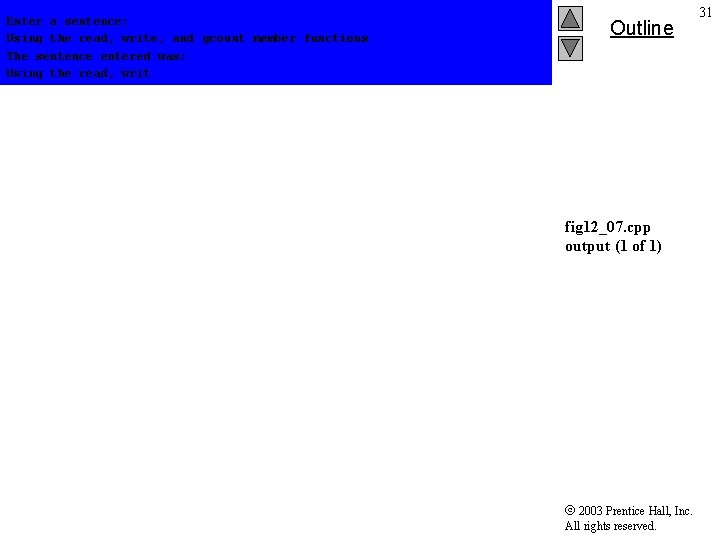
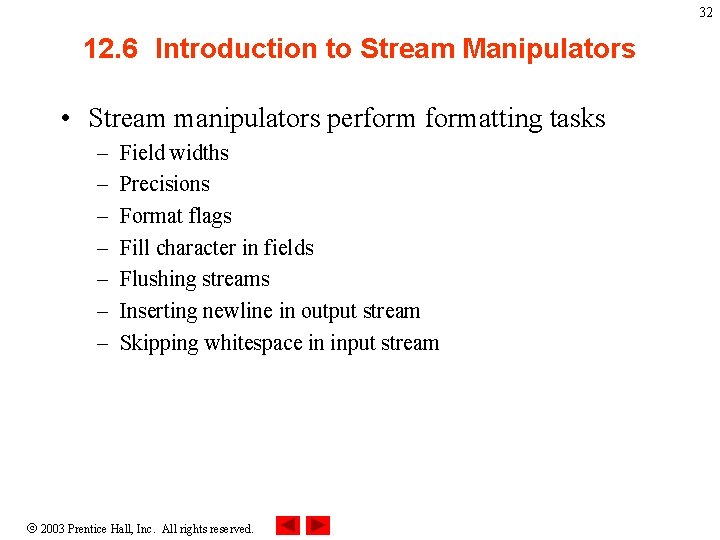
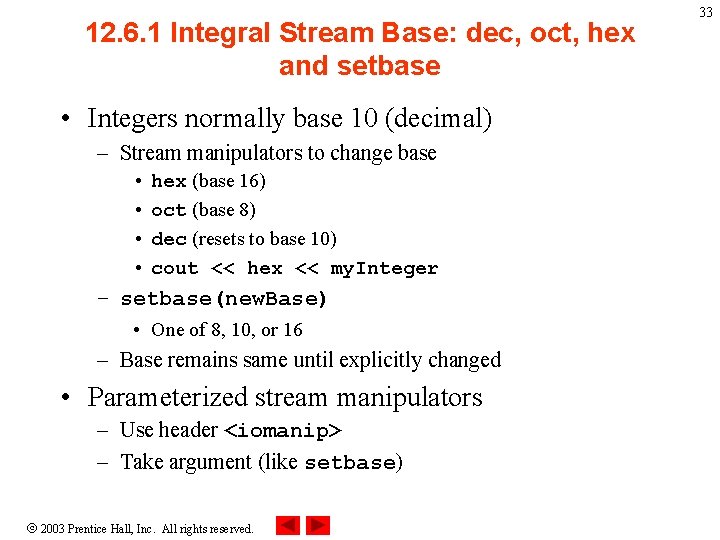
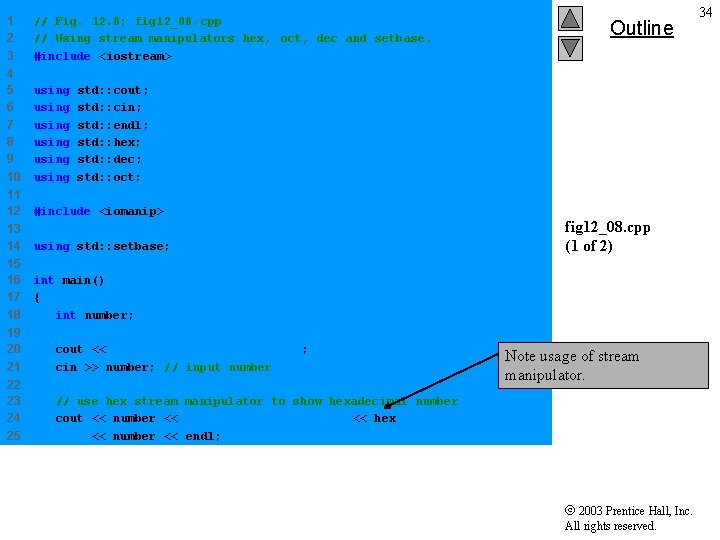
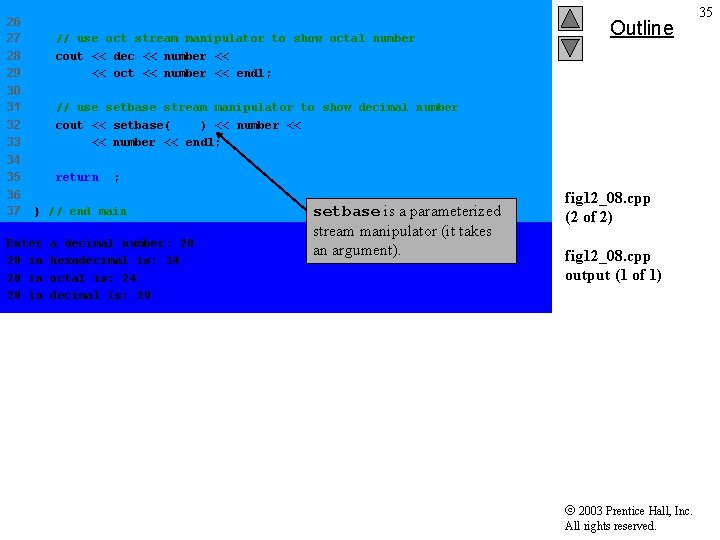
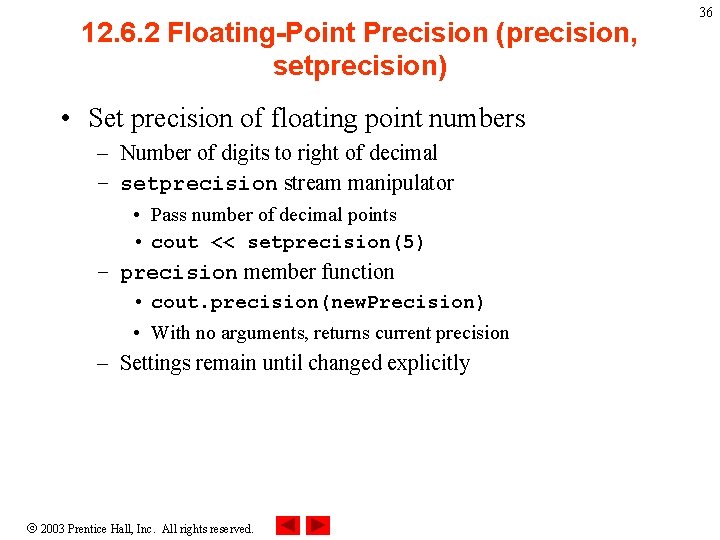
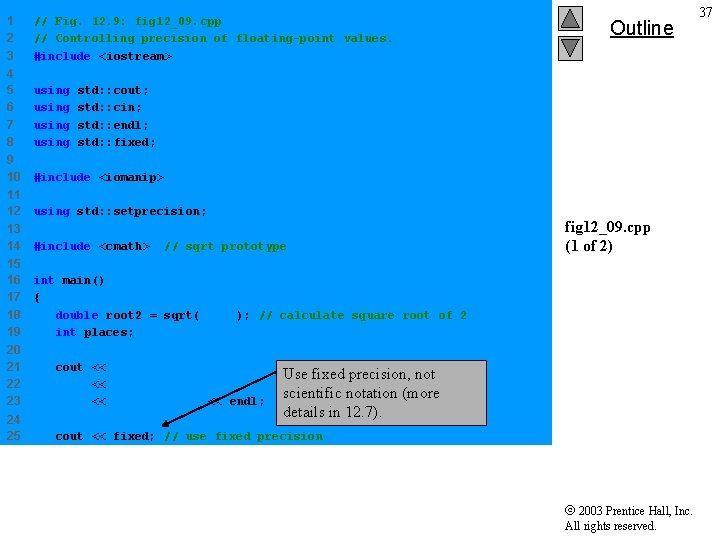
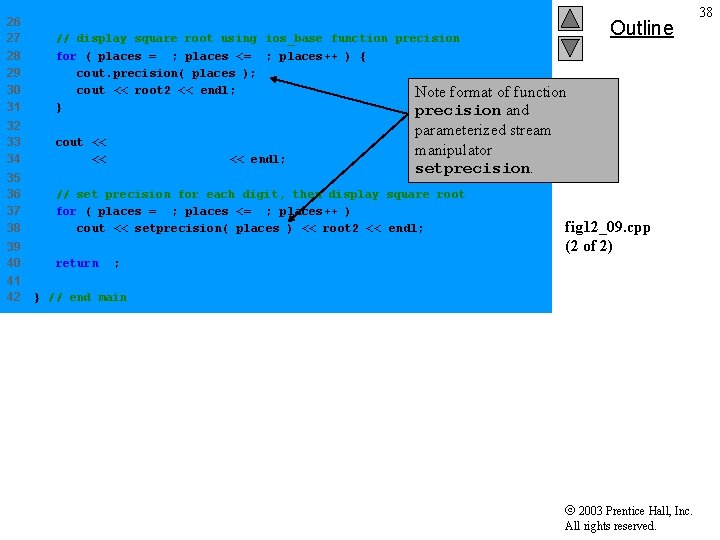
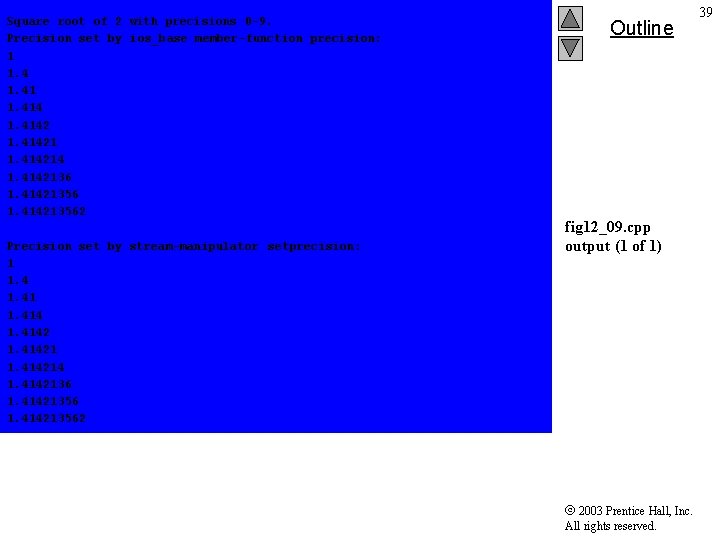
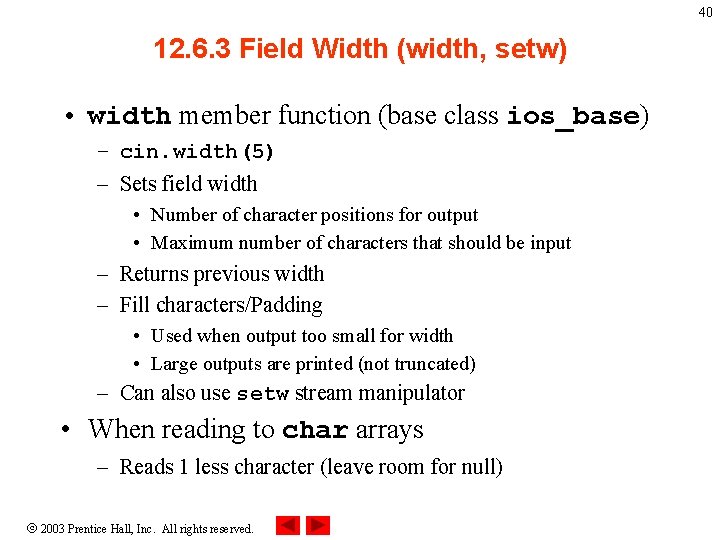
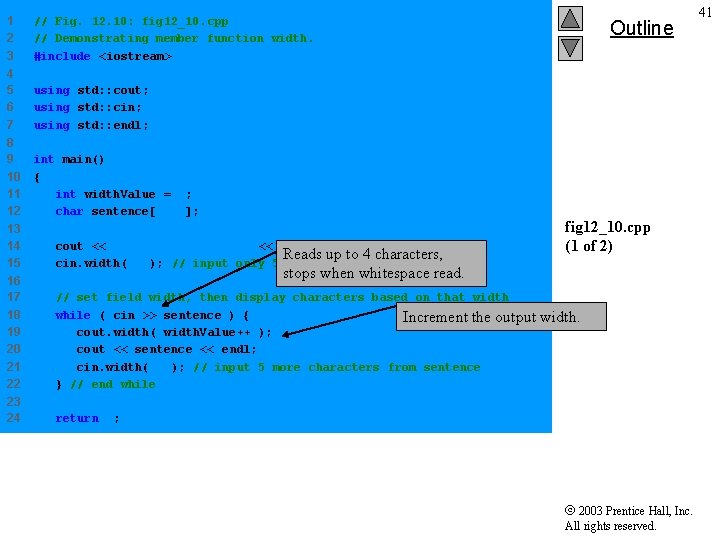
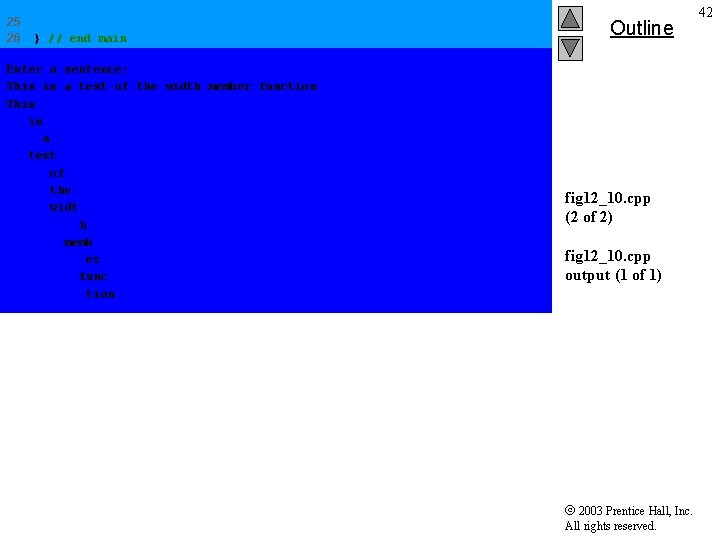
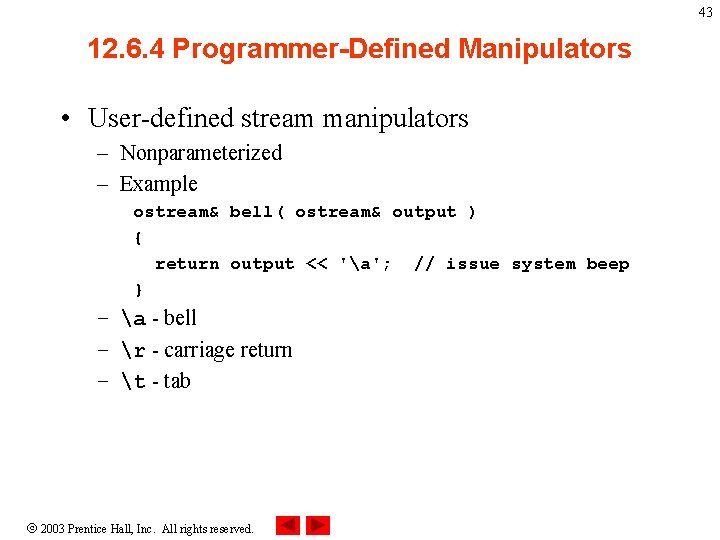
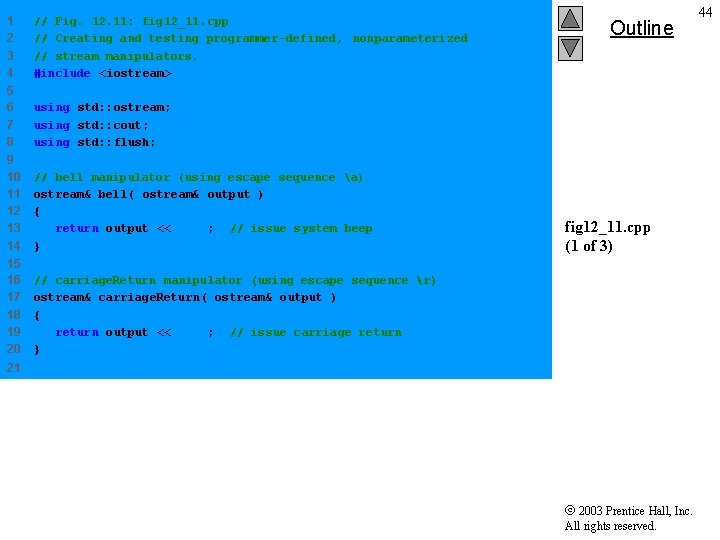
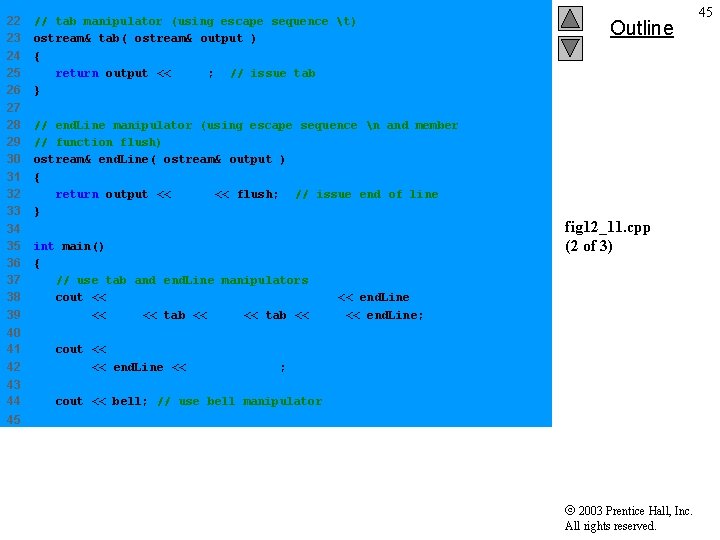
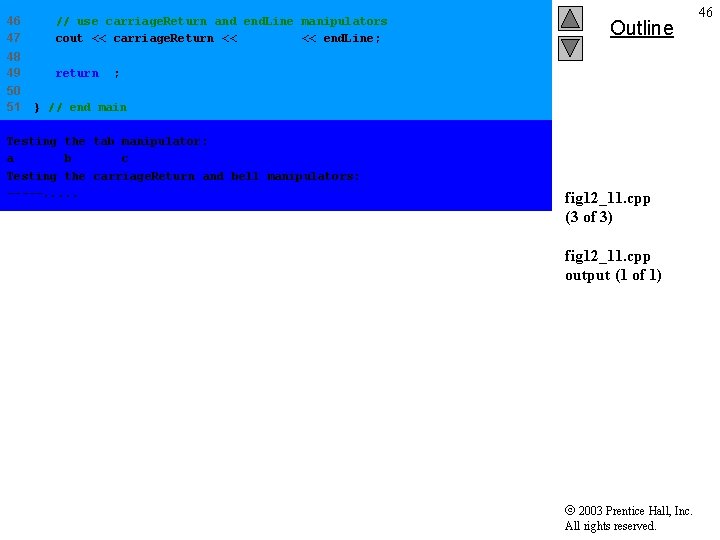
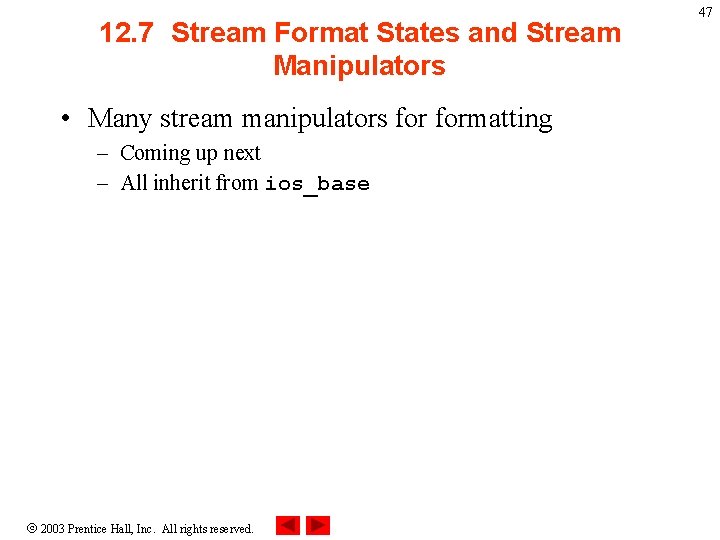
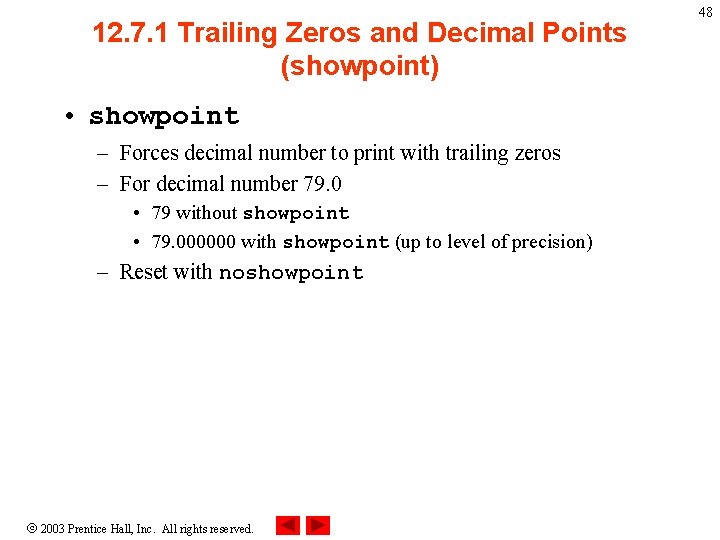
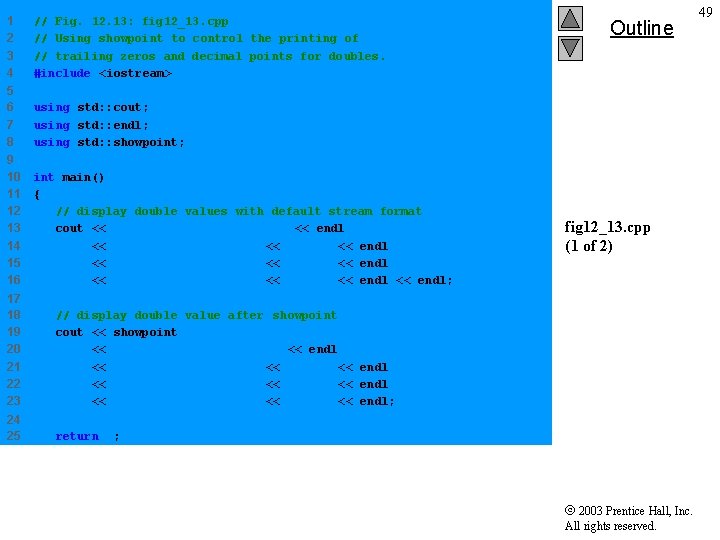
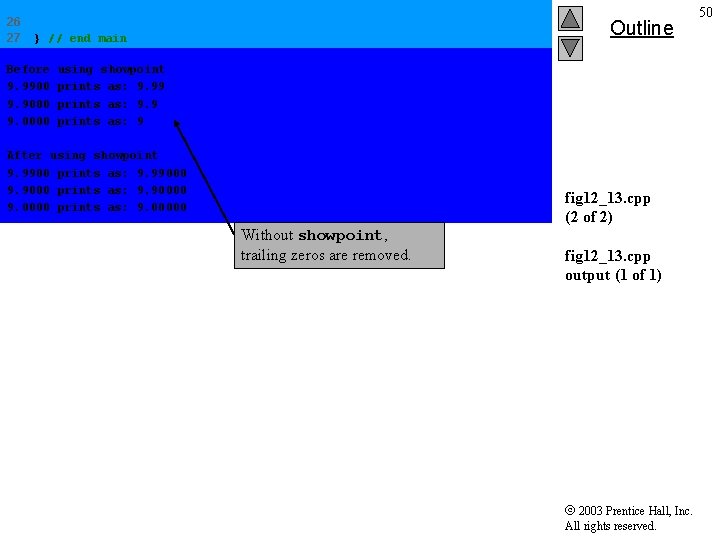
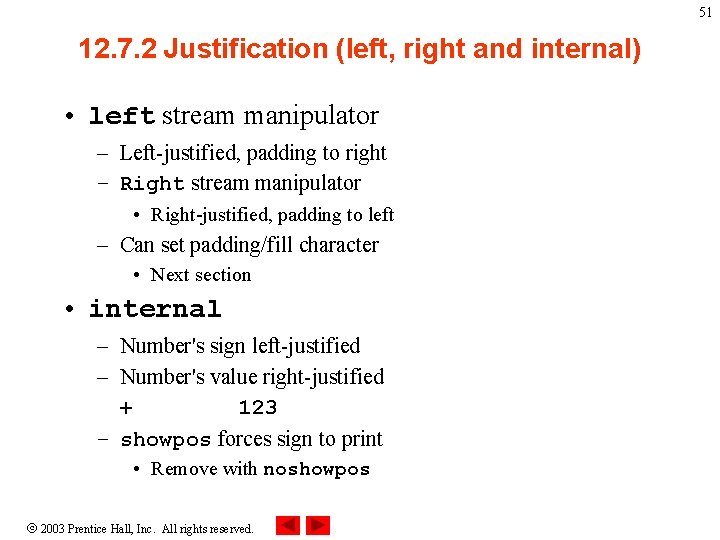
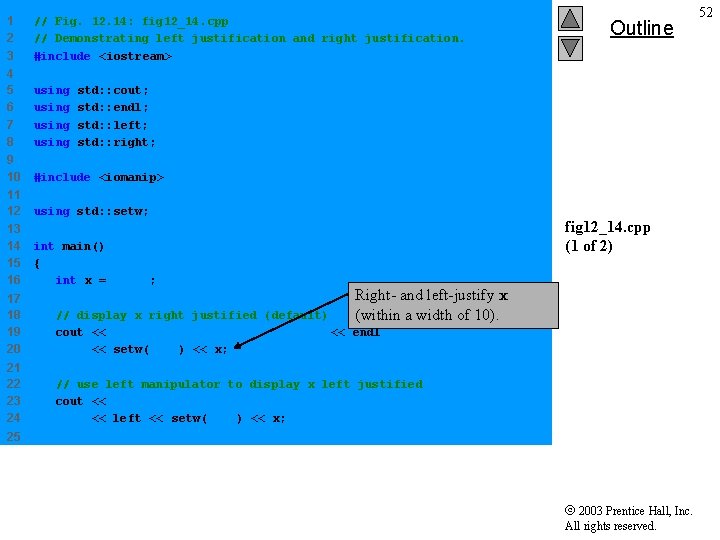
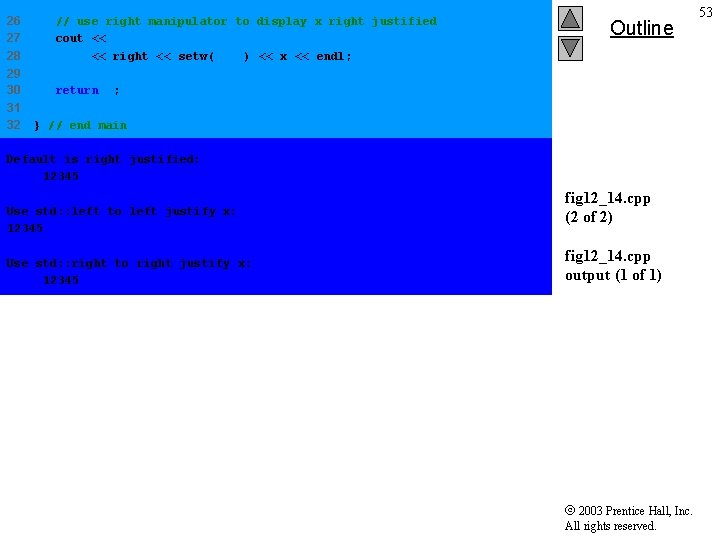
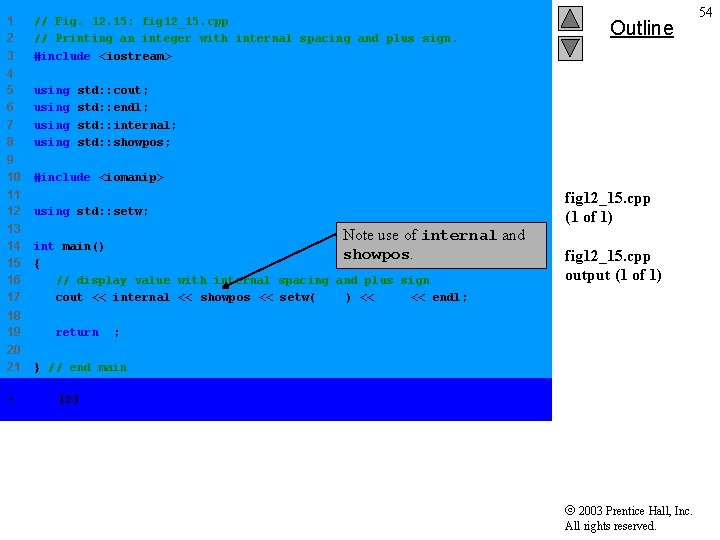
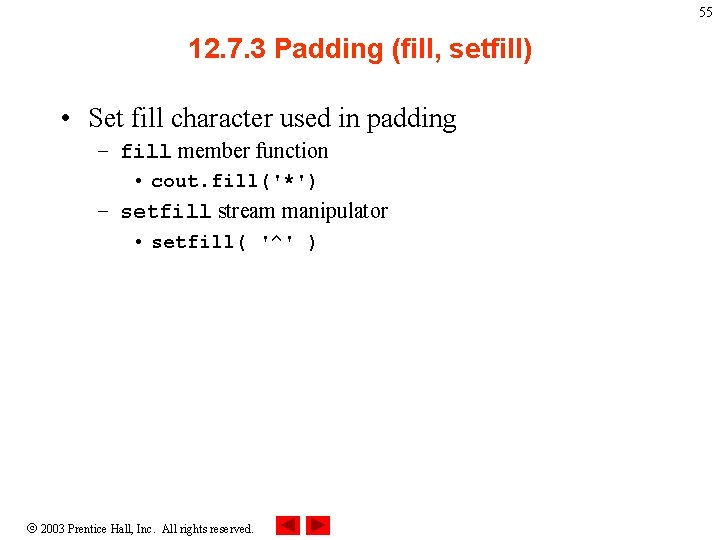
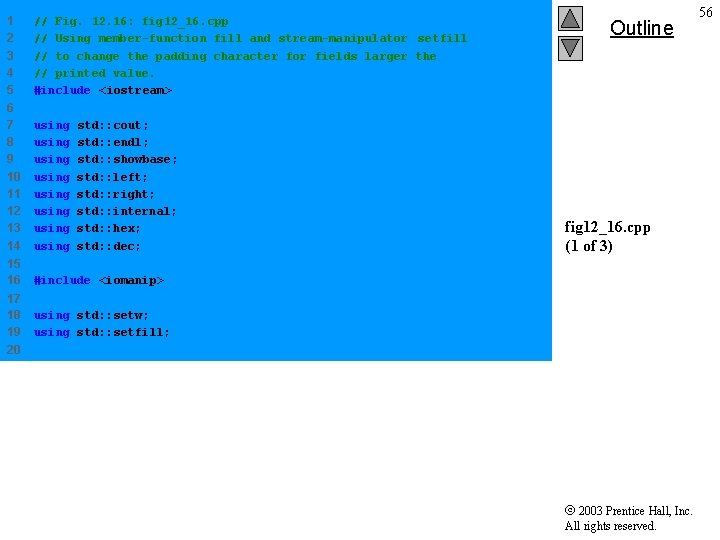
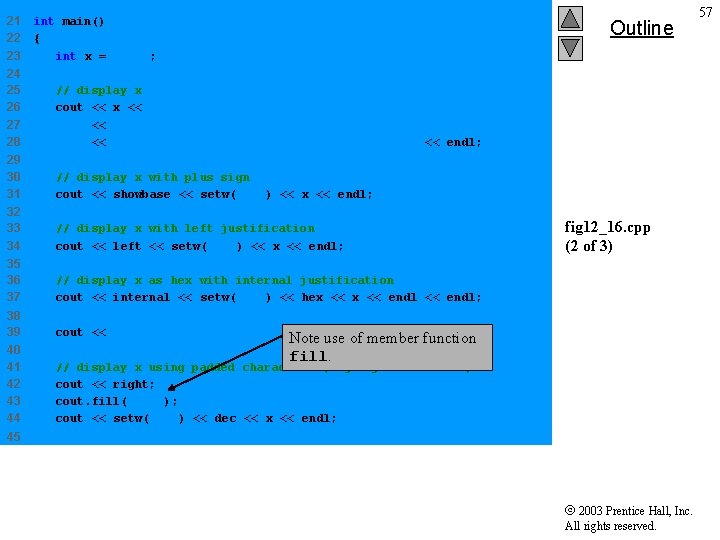
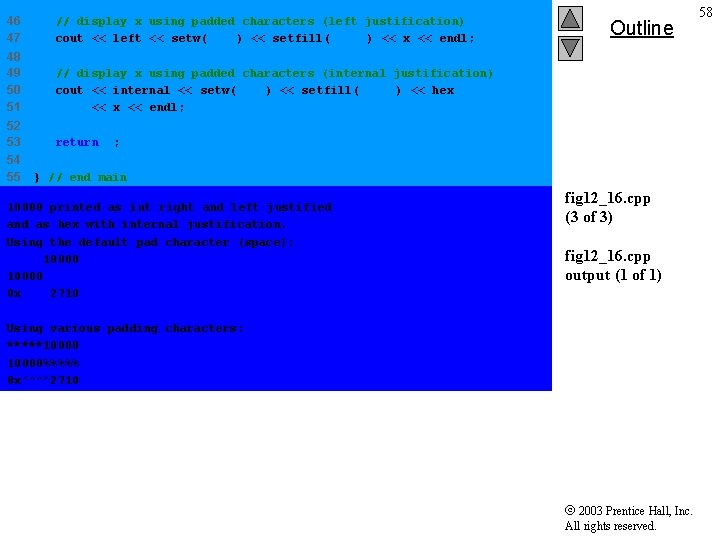
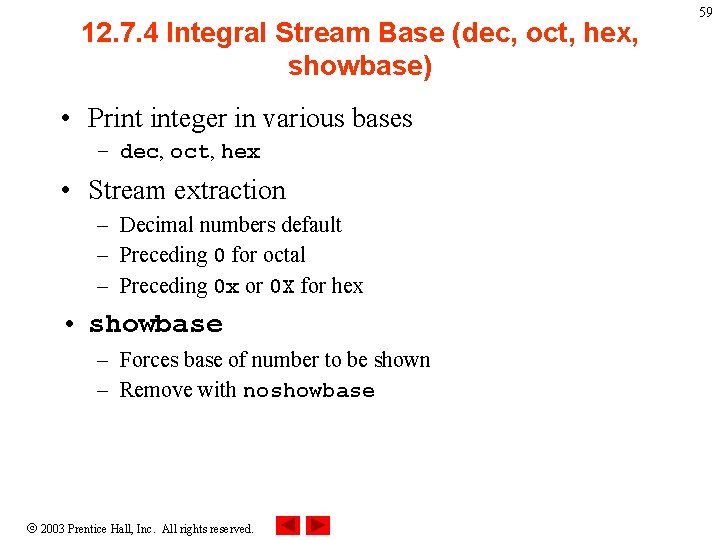
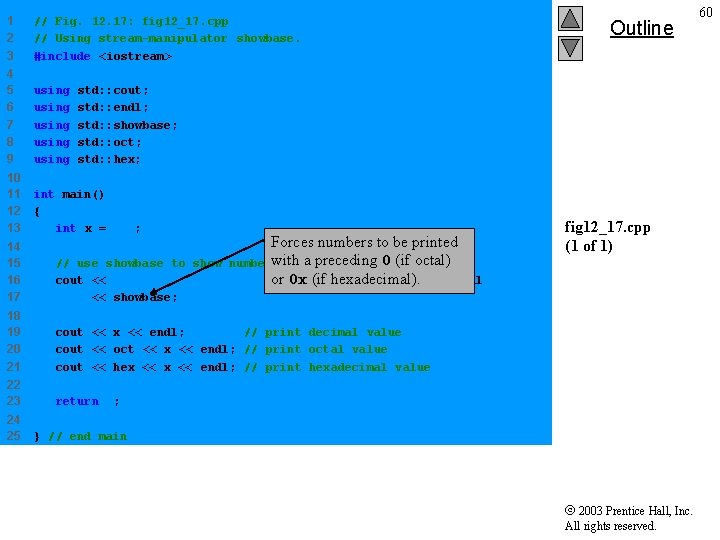
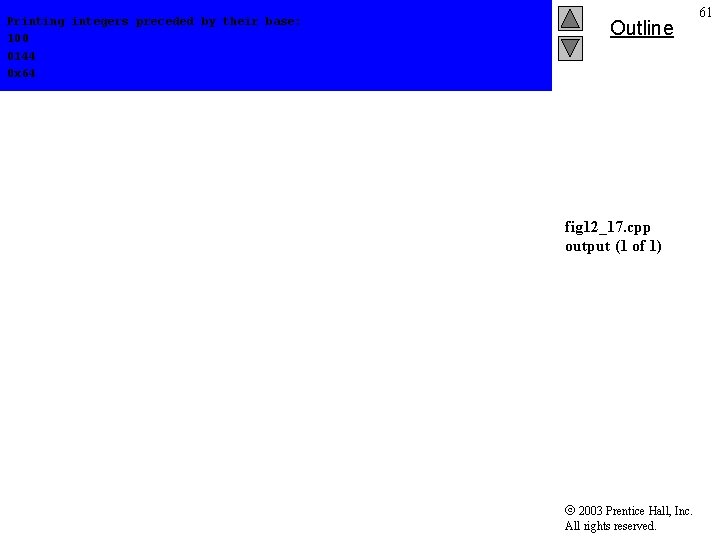
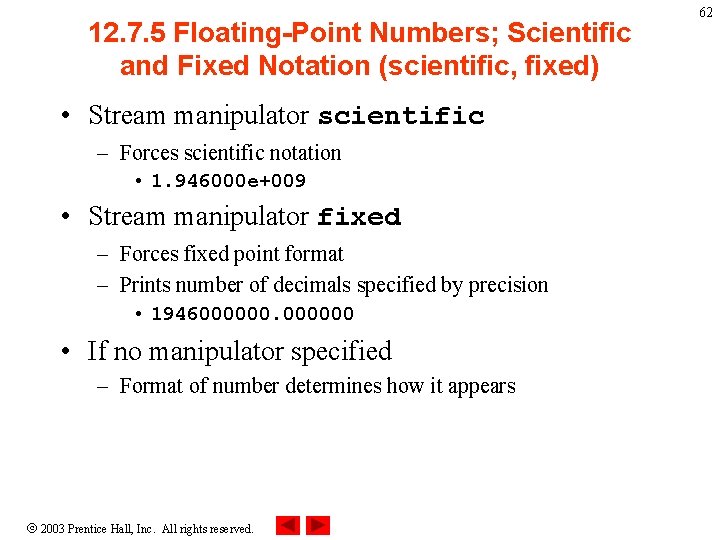
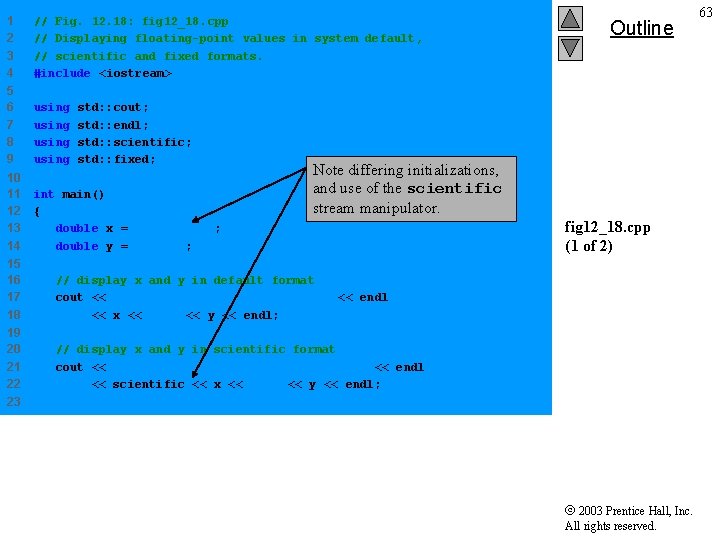
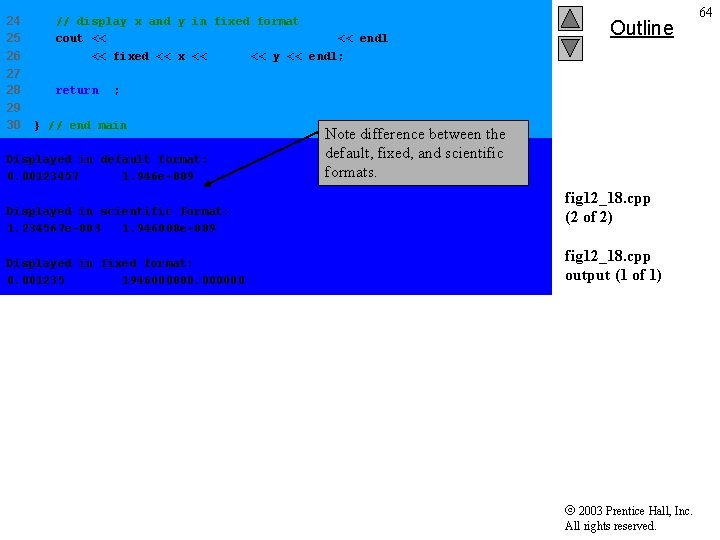
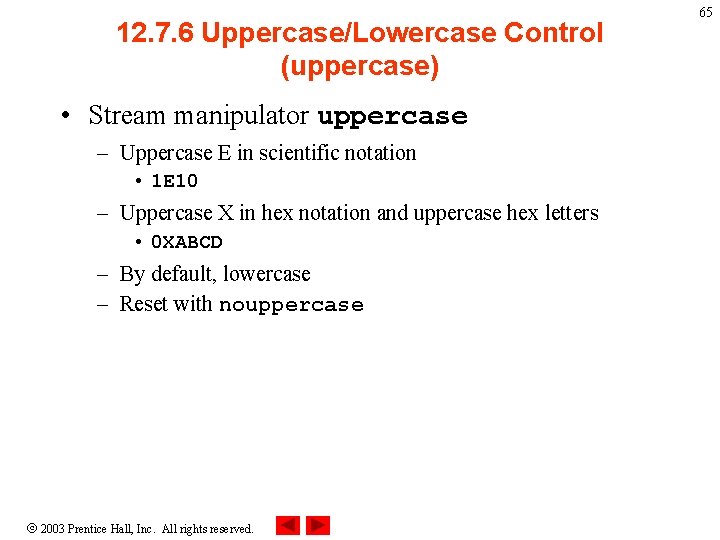
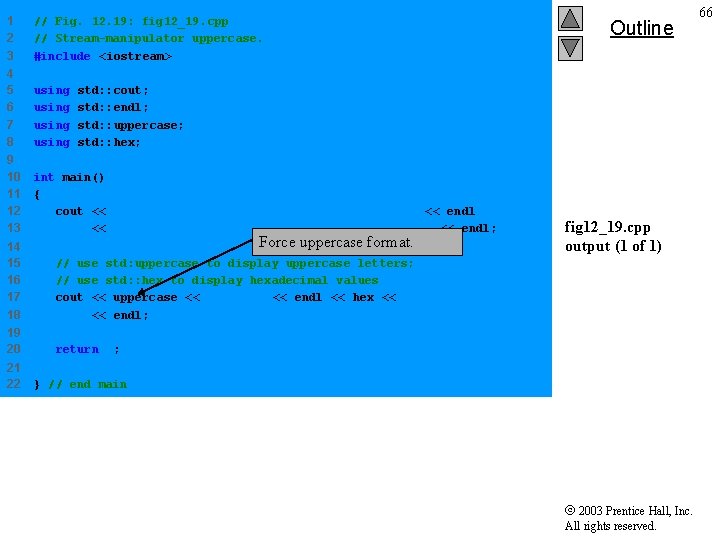
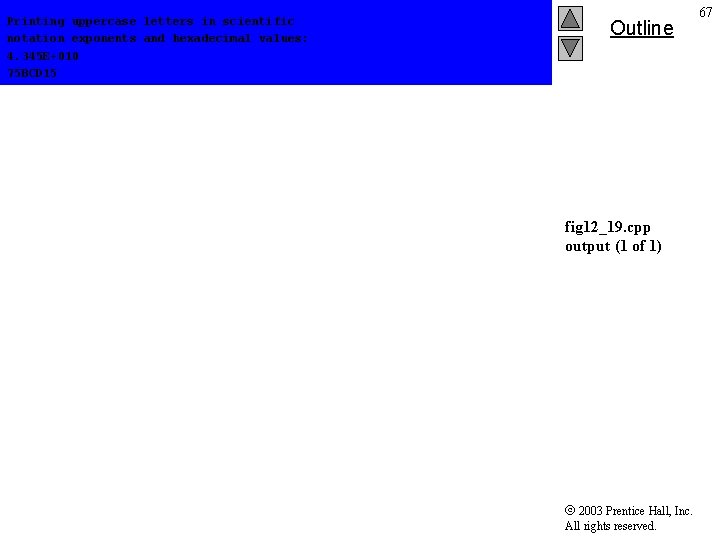
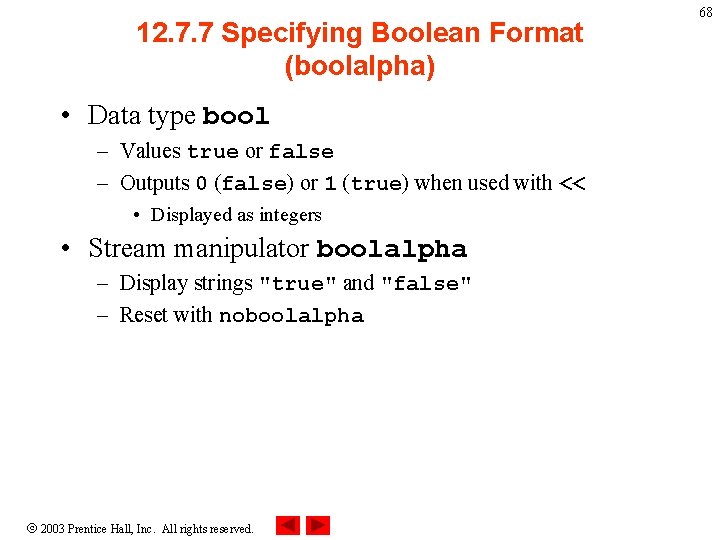
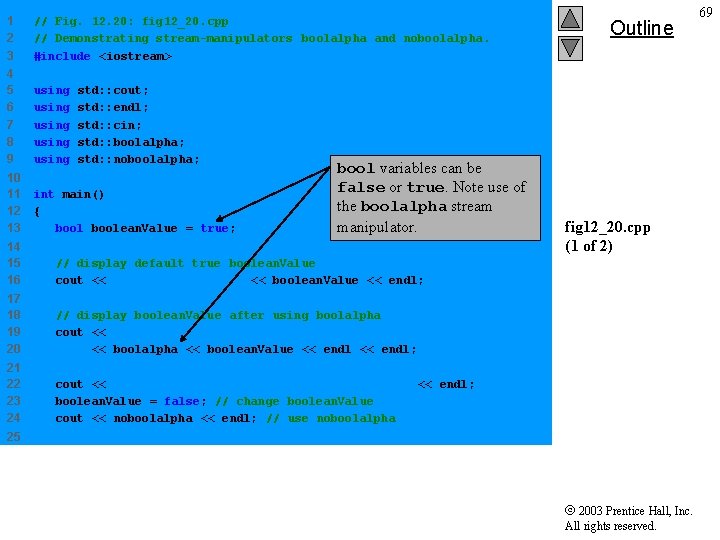
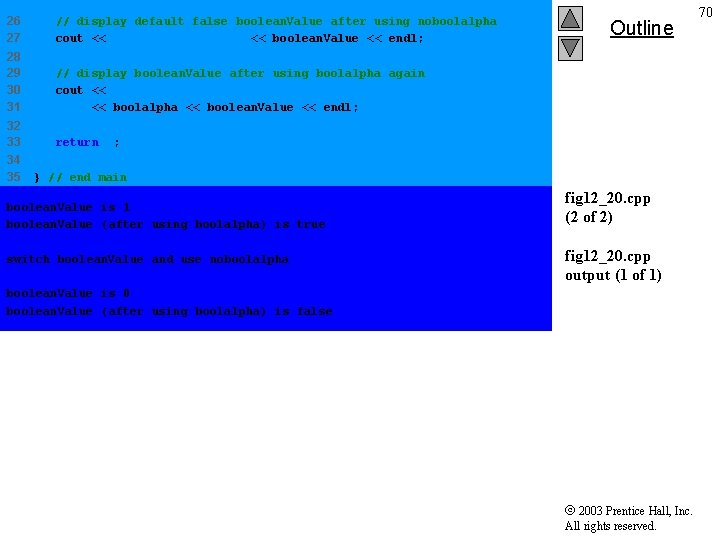
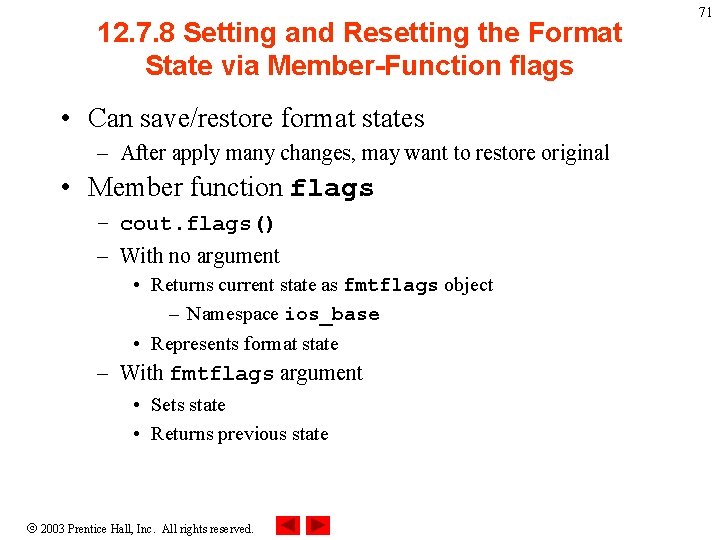
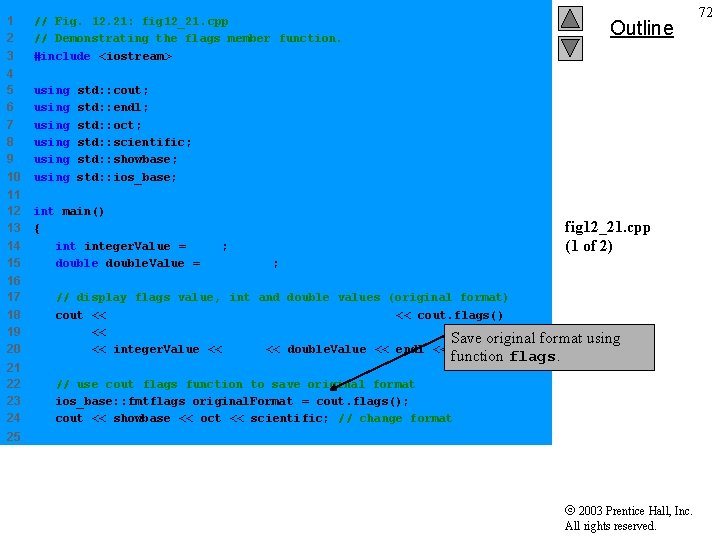
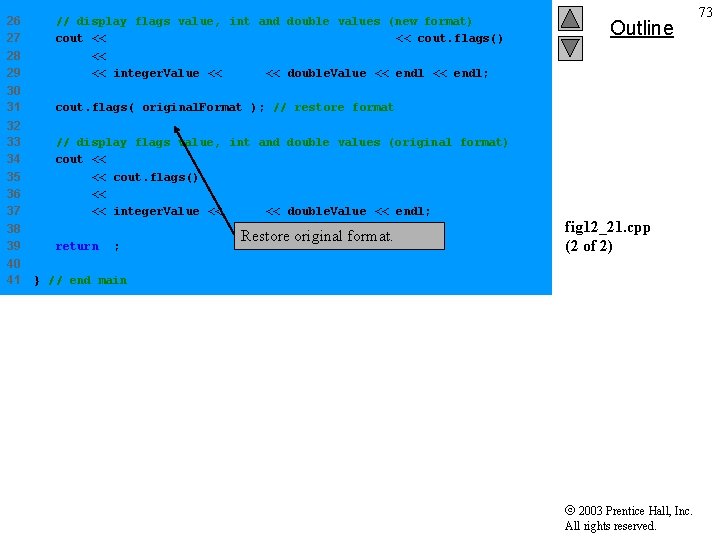
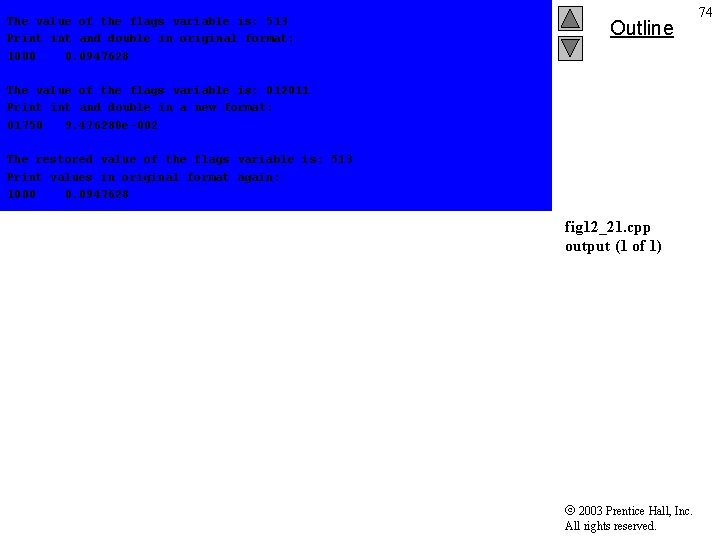
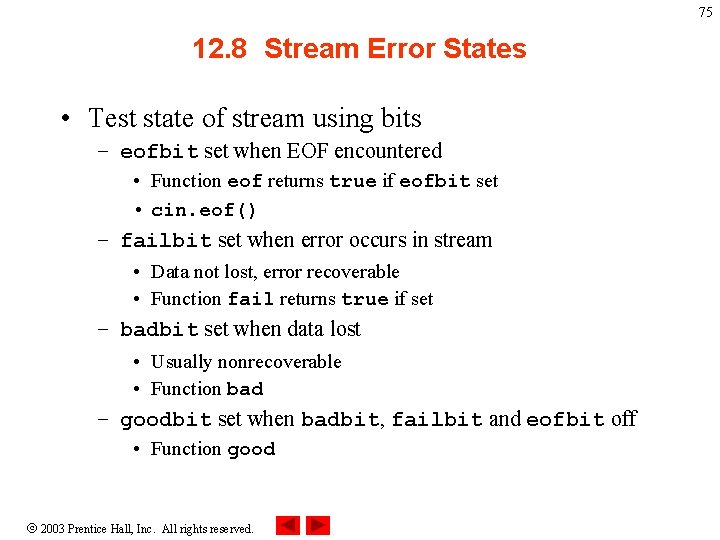
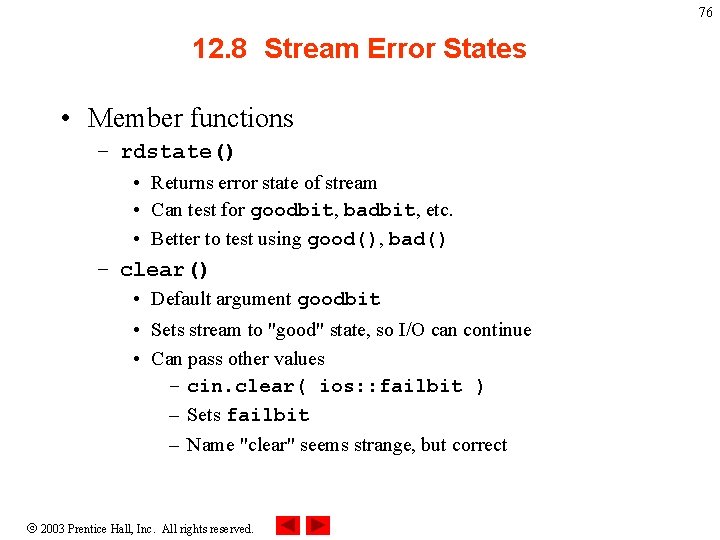
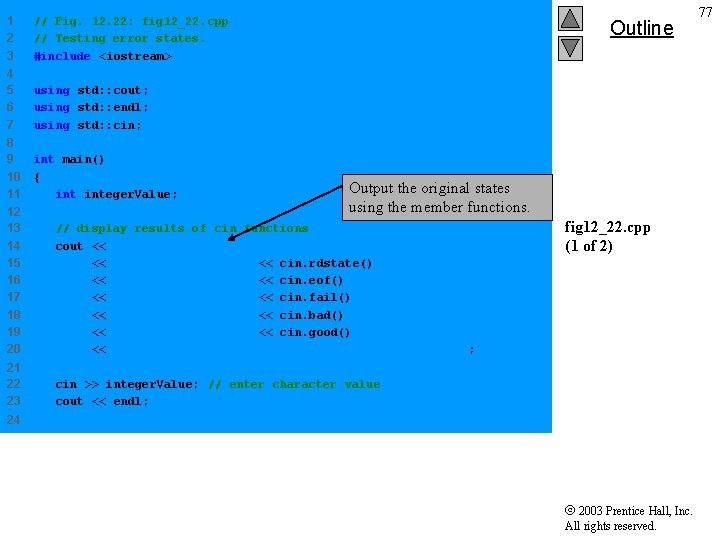
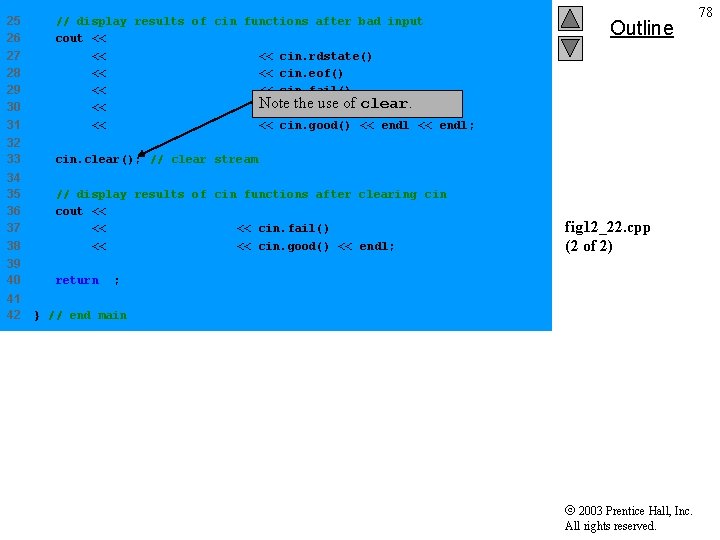
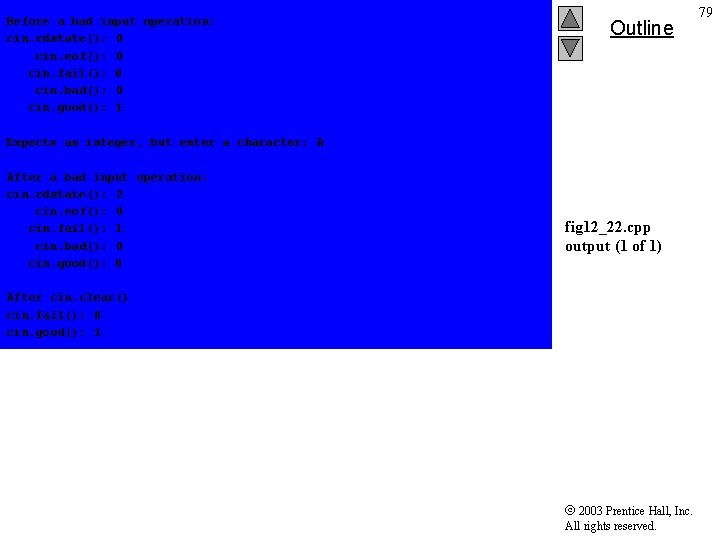
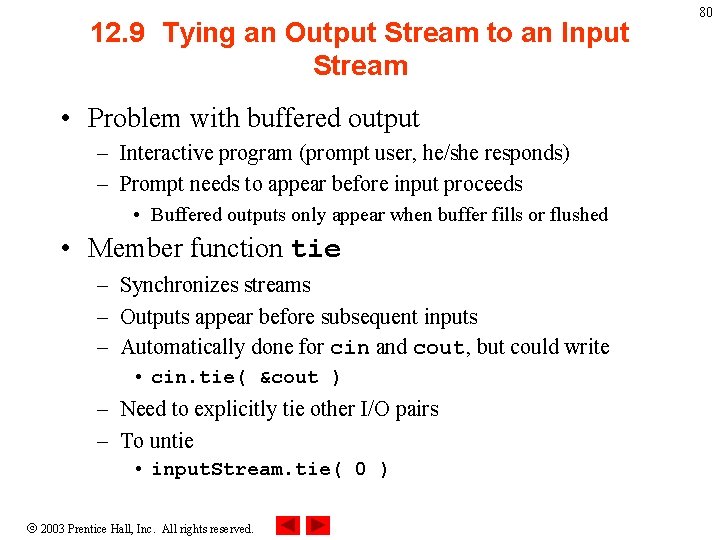
- Slides: 80
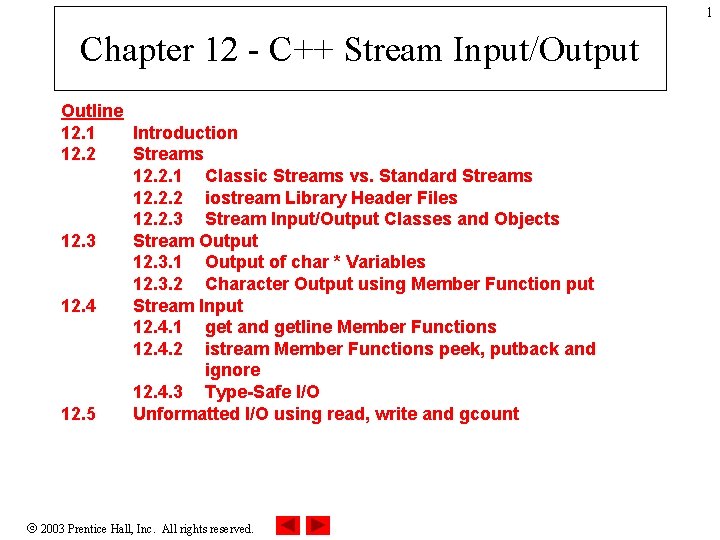
1 Chapter 12 - C++ Stream Input/Output Outline 12. 1 Introduction 12. 2 Streams 12. 2. 1 Classic Streams vs. Standard Streams 12. 2. 2 iostream Library Header Files 12. 2. 3 Stream Input/Output Classes and Objects 12. 3 Stream Output 12. 3. 1 Output of char * Variables 12. 3. 2 Character Output using Member Function put 12. 4 Stream Input 12. 4. 1 get and getline Member Functions 12. 4. 2 istream Member Functions peek, putback and ignore 12. 4. 3 Type-Safe I/O 12. 5 Unformatted I/O using read, write and gcount 2003 Prentice Hall, Inc. All rights reserved.
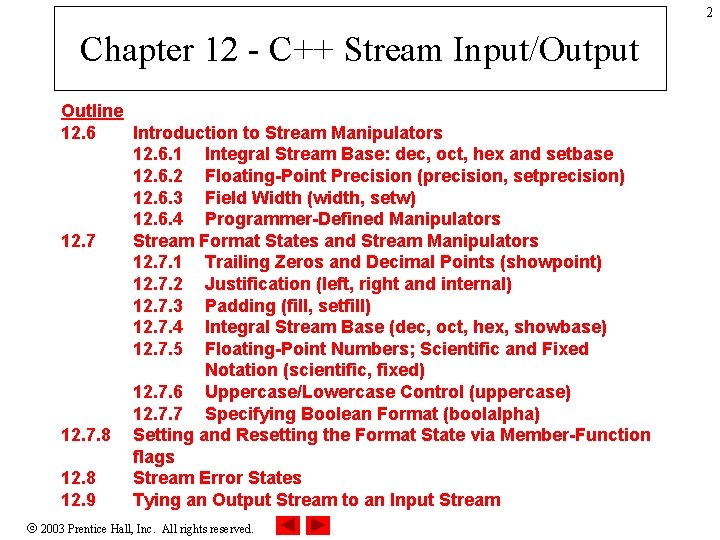
2 Chapter 12 - C++ Stream Input/Output Outline 12. 6 Introduction to Stream Manipulators 12. 6. 1 Integral Stream Base: dec, oct, hex and setbase 12. 6. 2 Floating-Point Precision (precision, setprecision) 12. 6. 3 Field Width (width, setw) 12. 6. 4 Programmer-Defined Manipulators 12. 7 Stream Format States and Stream Manipulators 12. 7. 1 Trailing Zeros and Decimal Points (showpoint) 12. 7. 2 Justification (left, right and internal) 12. 7. 3 Padding (fill, setfill) 12. 7. 4 Integral Stream Base (dec, oct, hex, showbase) 12. 7. 5 Floating-Point Numbers; Scientific and Fixed Notation (scientific, fixed) 12. 7. 6 Uppercase/Lowercase Control (uppercase) 12. 7. 7 Specifying Boolean Format (boolalpha) 12. 7. 8 Setting and Resetting the Format State via Member-Function flags 12. 8 Stream Error States 12. 9 Tying an Output Stream to an Input Stream 2003 Prentice Hall, Inc. All rights reserved.
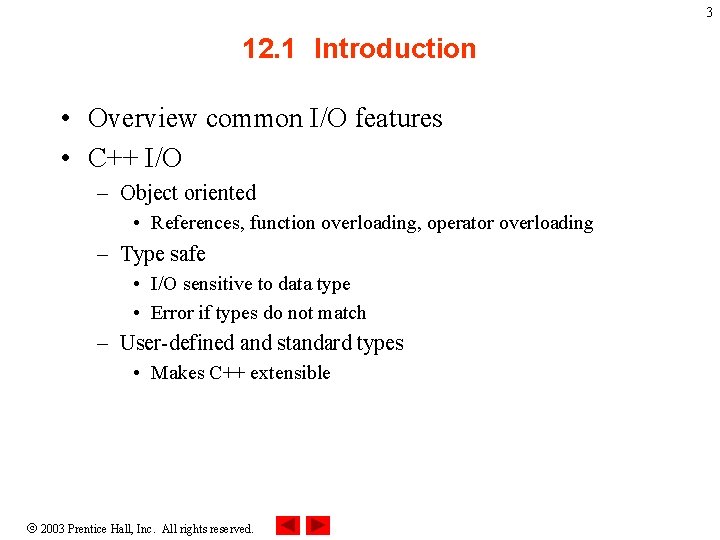
3 12. 1 Introduction • Overview common I/O features • C++ I/O – Object oriented • References, function overloading, operator overloading – Type safe • I/O sensitive to data type • Error if types do not match – User-defined and standard types • Makes C++ extensible 2003 Prentice Hall, Inc. All rights reserved.
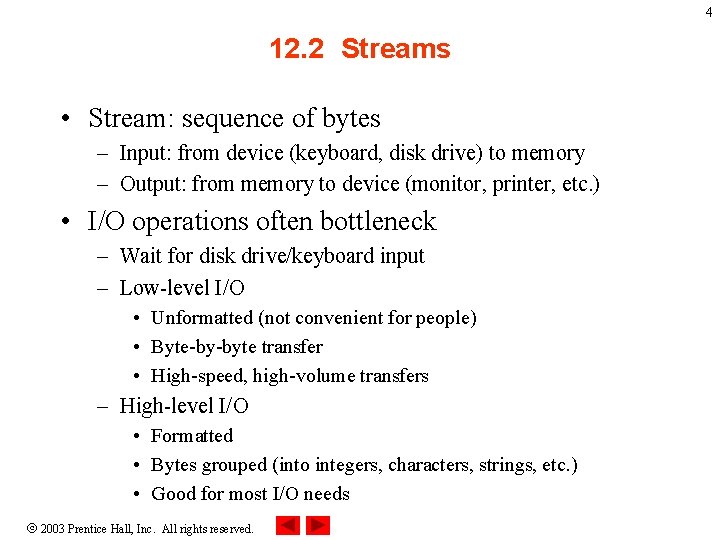
4 12. 2 Streams • Stream: sequence of bytes – Input: from device (keyboard, disk drive) to memory – Output: from memory to device (monitor, printer, etc. ) • I/O operations often bottleneck – Wait for disk drive/keyboard input – Low-level I/O • Unformatted (not convenient for people) • Byte-by-byte transfer • High-speed, high-volume transfers – High-level I/O • Formatted • Bytes grouped (into integers, characters, strings, etc. ) • Good for most I/O needs 2003 Prentice Hall, Inc. All rights reserved.
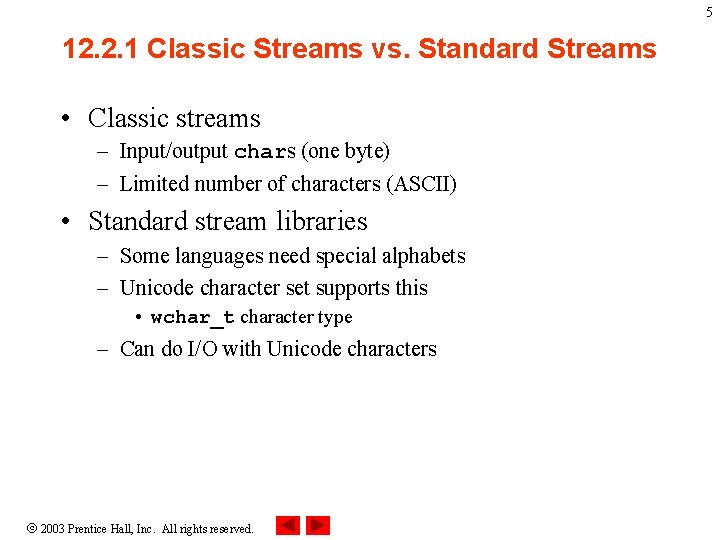
5 12. 2. 1 Classic Streams vs. Standard Streams • Classic streams – Input/output chars (one byte) – Limited number of characters (ASCII) • Standard stream libraries – Some languages need special alphabets – Unicode character set supports this • wchar_t character type – Can do I/O with Unicode characters 2003 Prentice Hall, Inc. All rights reserved.
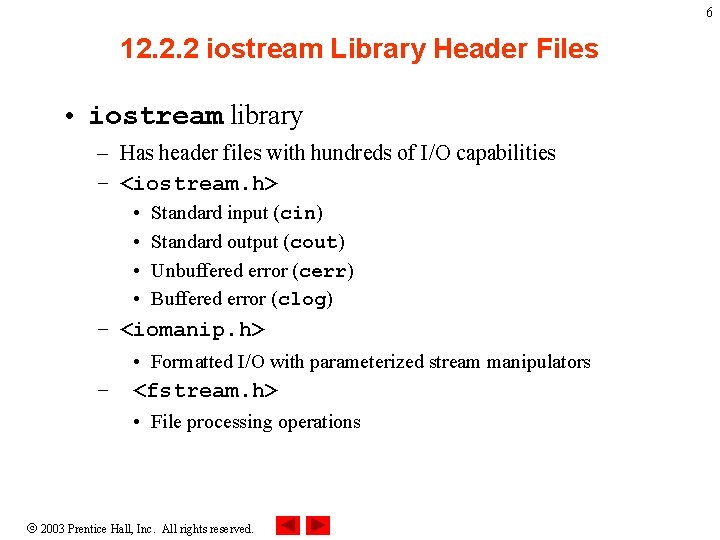
6 12. 2. 2 iostream Library Header Files • iostream library – Has header files with hundreds of I/O capabilities – <iostream. h> • • Standard input (cin) Standard output (cout) Unbuffered error (cerr) Buffered error (clog) – <iomanip. h> • Formatted I/O with parameterized stream manipulators – <fstream. h> • File processing operations 2003 Prentice Hall, Inc. All rights reserved.
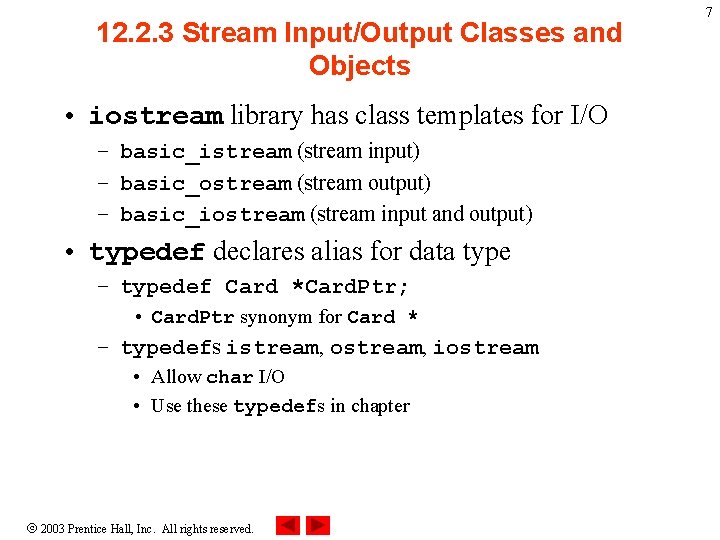
12. 2. 3 Stream Input/Output Classes and Objects • iostream library has class templates for I/O – basic_istream (stream input) – basic_ostream (stream output) – basic_iostream (stream input and output) • typedef declares alias for data type – typedef Card *Card. Ptr; • Card. Ptr synonym for Card * – typedefs istream, ostream, iostream • Allow char I/O • Use these typedefs in chapter 2003 Prentice Hall, Inc. All rights reserved. 7
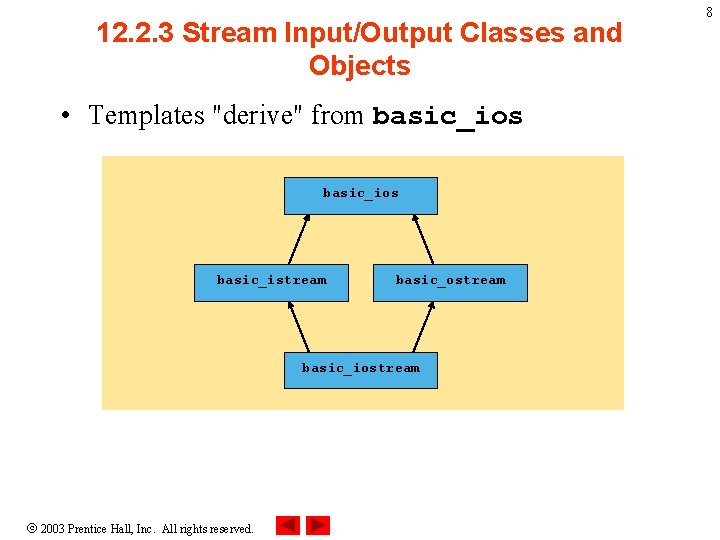
12. 2. 3 Stream Input/Output Classes and Objects • Templates "derive" from basic_ios basic_istream basic_ostream basic_iostream 2003 Prentice Hall, Inc. All rights reserved. 8
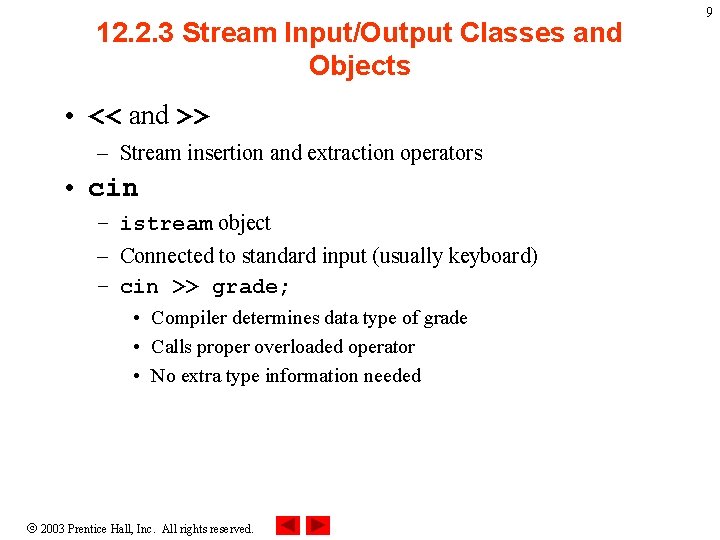
12. 2. 3 Stream Input/Output Classes and Objects • << and >> – Stream insertion and extraction operators • cin – istream object – Connected to standard input (usually keyboard) – cin >> grade; • Compiler determines data type of grade • Calls proper overloaded operator • No extra type information needed 2003 Prentice Hall, Inc. All rights reserved. 9
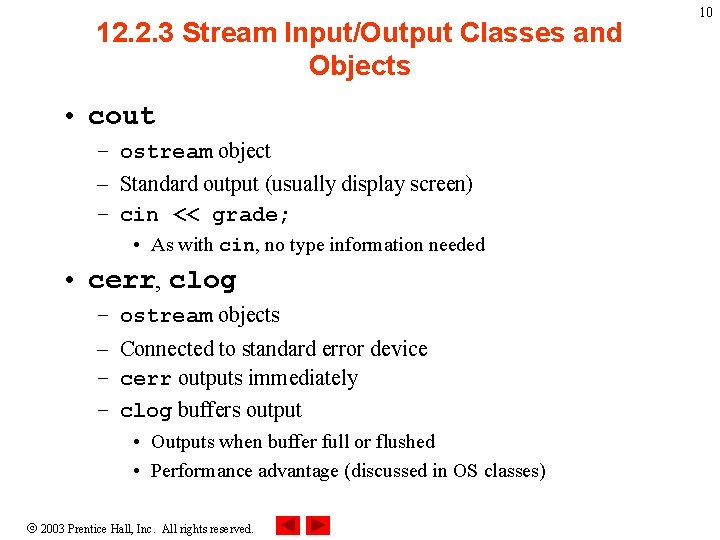
12. 2. 3 Stream Input/Output Classes and Objects • cout – ostream object – Standard output (usually display screen) – cin << grade; • As with cin, no type information needed • cerr, clog – – ostream objects Connected to standard error device cerr outputs immediately clog buffers output • Outputs when buffer full or flushed • Performance advantage (discussed in OS classes) 2003 Prentice Hall, Inc. All rights reserved. 10
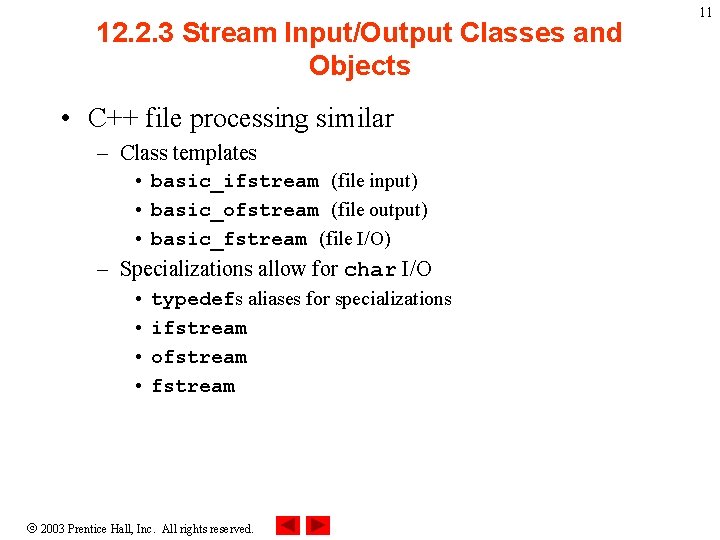
12. 2. 3 Stream Input/Output Classes and Objects • C++ file processing similar – Class templates • basic_ifstream (file input) • basic_ofstream (file output) • basic_fstream (file I/O) – Specializations allow for char I/O • typedefs aliases for specializations • ifstream • ofstream • fstream 2003 Prentice Hall, Inc. All rights reserved. 11
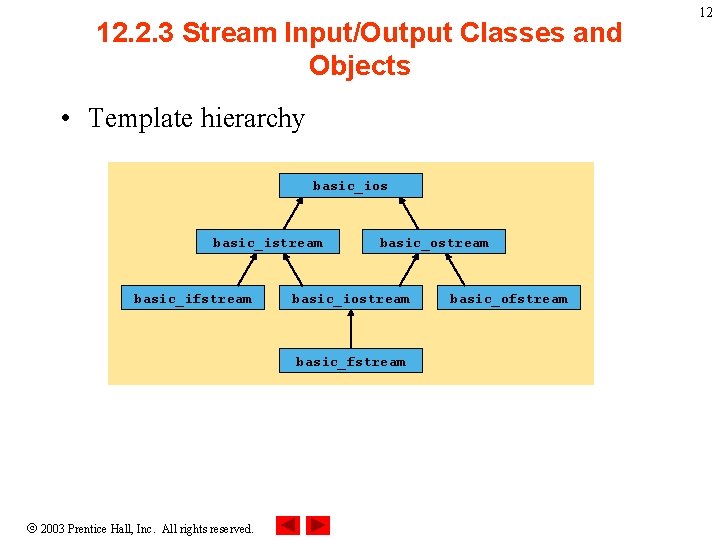
12. 2. 3 Stream Input/Output Classes and Objects • Template hierarchy basic_ios basic_istream basic_ifstream basic_ostream basic_iostream basic_fstream 2003 Prentice Hall, Inc. All rights reserved. basic_ofstream 12
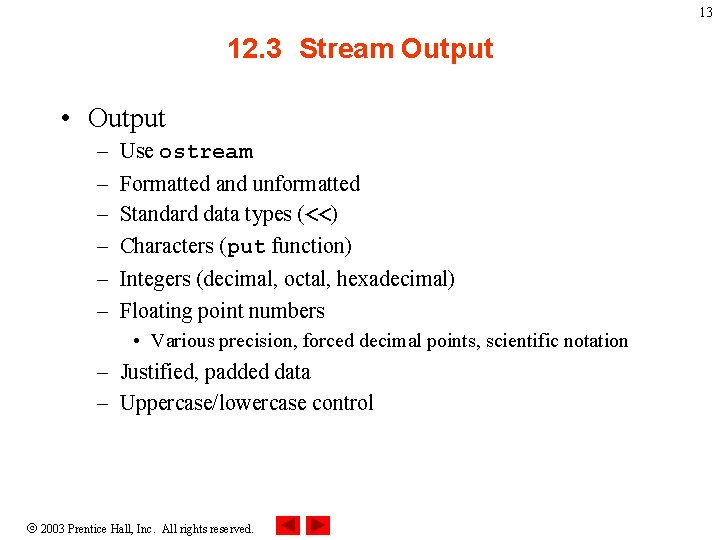
13 12. 3 Stream Output • Output – – – Use ostream Formatted and unformatted Standard data types (<<) Characters (put function) Integers (decimal, octal, hexadecimal) Floating point numbers • Various precision, forced decimal points, scientific notation – Justified, padded data – Uppercase/lowercase control 2003 Prentice Hall, Inc. All rights reserved.
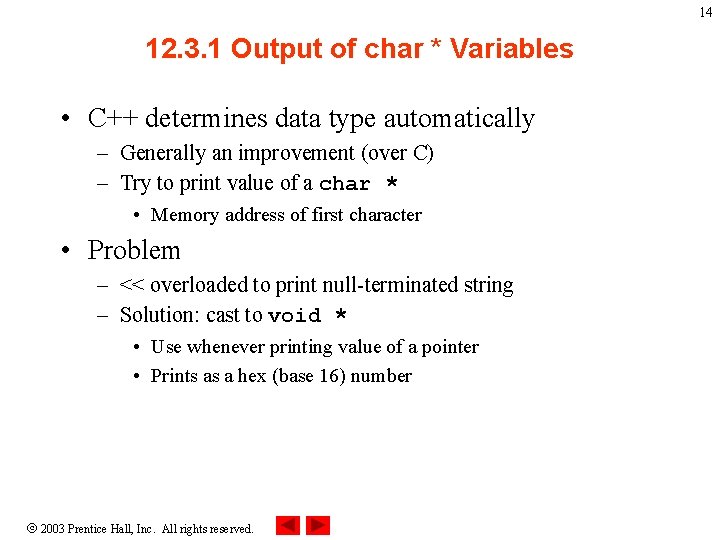
14 12. 3. 1 Output of char * Variables • C++ determines data type automatically – Generally an improvement (over C) – Try to print value of a char * • Memory address of first character • Problem – << overloaded to print null-terminated string – Solution: cast to void * • Use whenever printing value of a pointer • Prints as a hex (base 16) number 2003 Prentice Hall, Inc. All rights reserved.
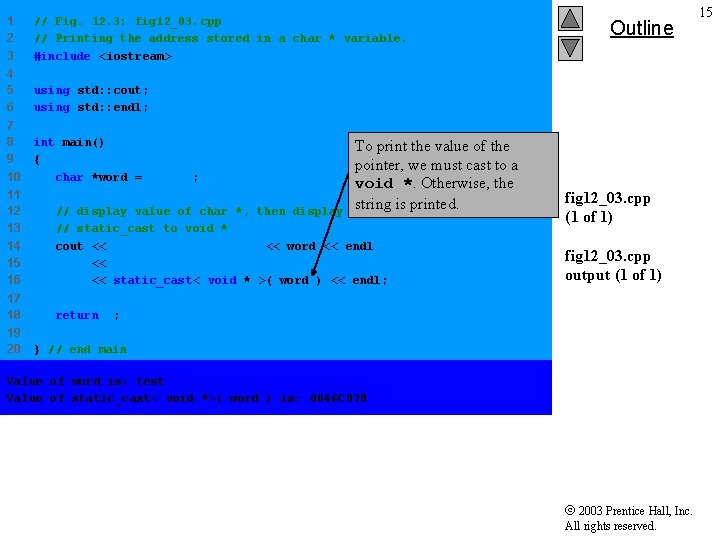
1 2 3 // Fig. 12. 3: fig 12_03. cpp // Printing the address stored in a char * variable. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 int main() { char *word = "test"; To print the value of the pointer, we must cast to a void *. Otherwise, the string is printed. value of char * 11 12 13 14 15 16 // display value of char *, then display // static_cast to void * cout << "Value of word is: " << word << endl << "Value of static_cast< void * >( word ) is: " << static_cast< void * >( word ) << endl; 17 18 return 0; 19 20 Outline fig 12_03. cpp (1 of 1) fig 12_03. cpp output (1 of 1) } // end main Value of word is: test Value of static_cast< void *>( word ) is: 0046 C 070 2003 Prentice Hall, Inc. All rights reserved. 15
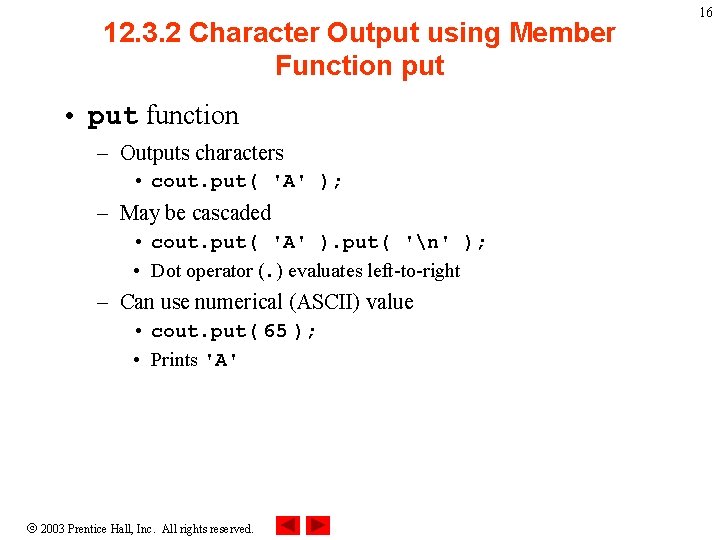
12. 3. 2 Character Output using Member Function put • put function – Outputs characters • cout. put( 'A' ); – May be cascaded • cout. put( 'A' ). put( 'n' ); • Dot operator (. ) evaluates left-to-right – Can use numerical (ASCII) value • cout. put( 65 ); • Prints 'A' 2003 Prentice Hall, Inc. All rights reserved. 16
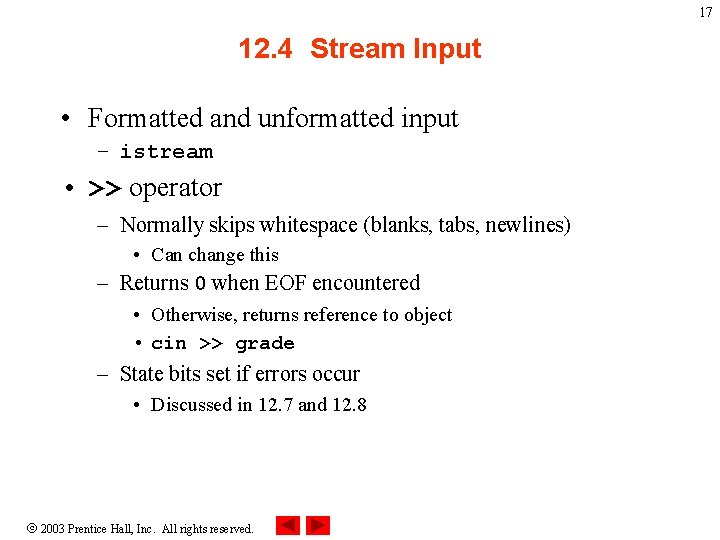
17 12. 4 Stream Input • Formatted and unformatted input – istream • >> operator – Normally skips whitespace (blanks, tabs, newlines) • Can change this – Returns 0 when EOF encountered • Otherwise, returns reference to object • cin >> grade – State bits set if errors occur • Discussed in 12. 7 and 12. 8 2003 Prentice Hall, Inc. All rights reserved.
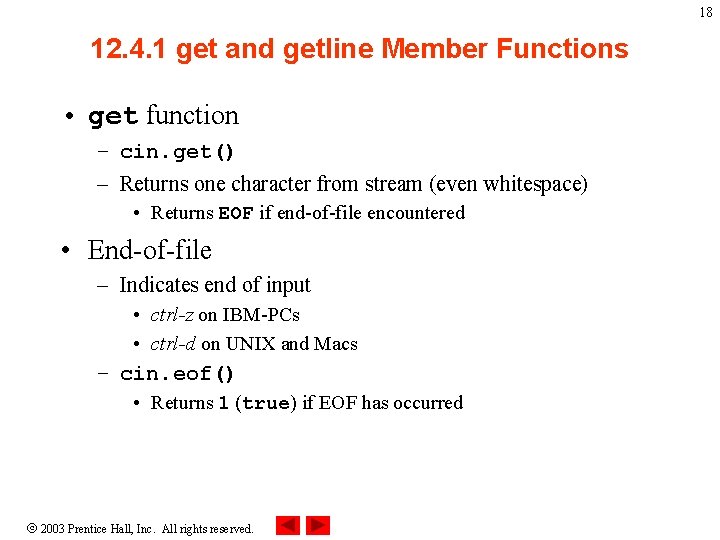
18 12. 4. 1 get and getline Member Functions • get function – cin. get() – Returns one character from stream (even whitespace) • Returns EOF if end-of-file encountered • End-of-file – Indicates end of input • ctrl-z on IBM-PCs • ctrl-d on UNIX and Macs – cin. eof() • Returns 1 (true) if EOF has occurred 2003 Prentice Hall, Inc. All rights reserved.
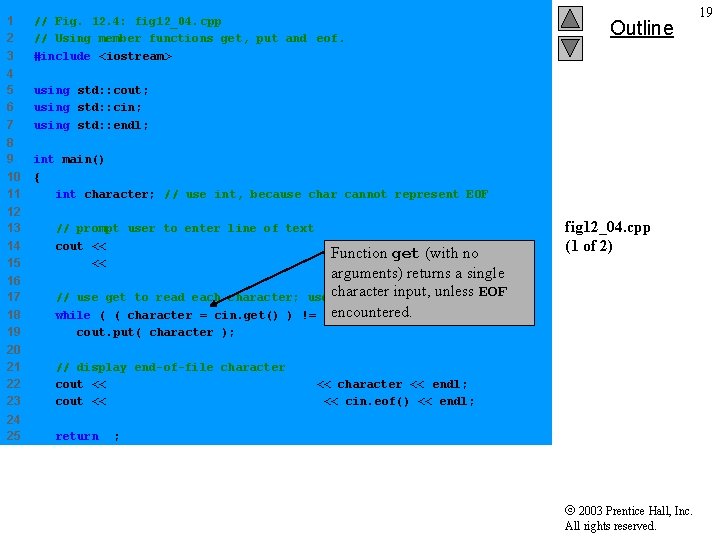
1 2 3 // Fig. 12. 4: fig 12_04. cpp // Using member functions get, put and eof. #include <iostream> 4 5 6 7 using std: : cout; using std: : cin; using std: : endl; 8 9 10 11 int main() { int character; // use int, because char cannot represent EOF 12 13 14 15 // prompt user to enter line of text cout << "Before input, cin. eof() is " << cin. eof() << endl Function get (with no << "Enter a sentence followed by end-of-file: " << endl; 16 17 18 19 Outline fig 12_04. cpp (1 of 2) arguments) returns a single input, unless EOF // use get to read each character; use character put to display it encountered. while ( ( character = cin. get() ) != EOF ) cout. put( character ); 20 21 22 23 // display end-of-file character cout << "n. EOF in this system is: " << character << endl; cout << "After input, cin. eof() is " << cin. eof() << endl; 24 25 return 0; 2003 Prentice Hall, Inc. All rights reserved. 19
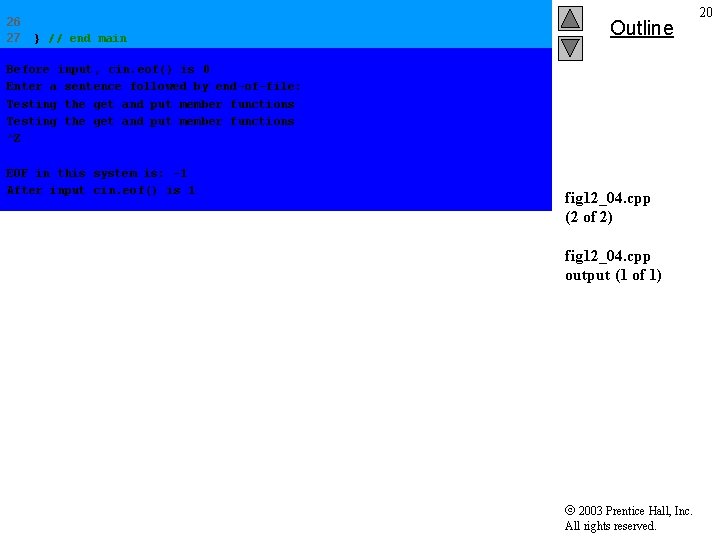
26 27 } // end main Outline Before input, cin. eof() is 0 Enter a sentence followed by end-of-file: Testing the get and put member functions ^Z EOF in this system is: -1 After input cin. eof() is 1 fig 12_04. cpp (2 of 2) fig 12_04. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 20
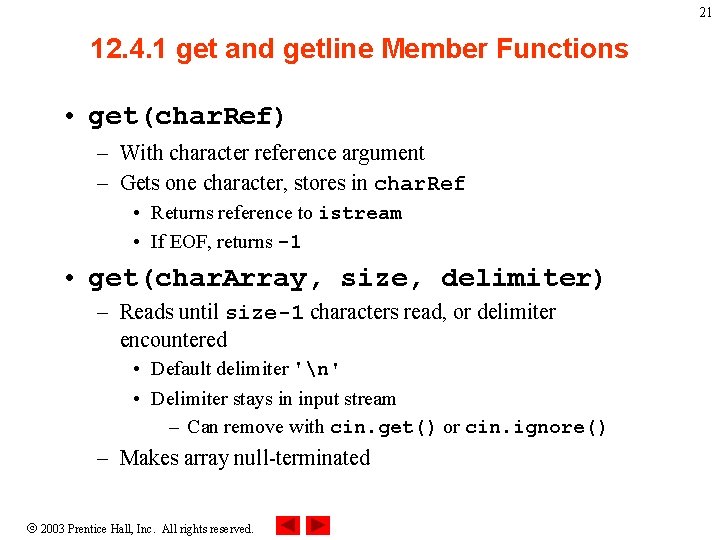
21 12. 4. 1 get and getline Member Functions • get(char. Ref) – With character reference argument – Gets one character, stores in char. Ref • Returns reference to istream • If EOF, returns -1 • get(char. Array, size, delimiter) – Reads until size-1 characters read, or delimiter encountered • Default delimiter 'n' • Delimiter stays in input stream – Can remove with cin. get() or cin. ignore() – Makes array null-terminated 2003 Prentice Hall, Inc. All rights reserved.
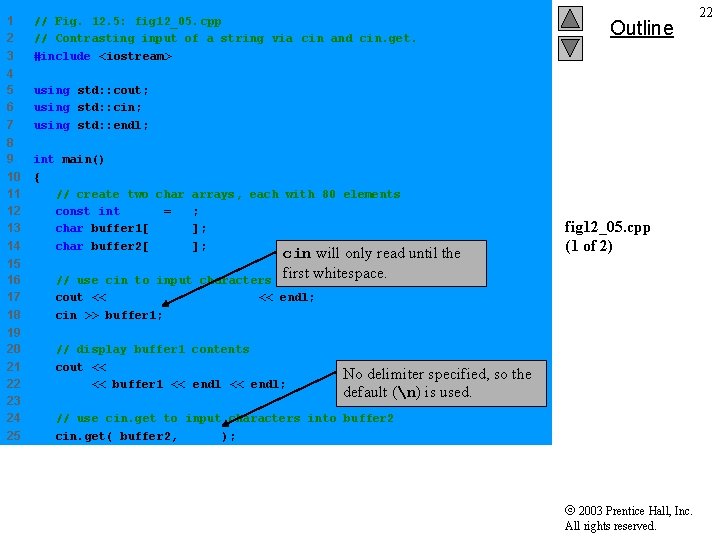
1 2 3 // Fig. 12. 5: fig 12_05. cpp // Contrasting input of a string via cin and cin. get. #include <iostream> 4 5 6 7 using std: : cout; using std: : cin; using std: : endl; 8 9 10 11 12 13 14 int main() { // create two char arrays, each with 80 elements const int SIZE = 80; char buffer 1[ SIZE ]; char buffer 2[ SIZE ]; 15 16 17 18 19 20 21 22 23 24 25 cin will only read until the first whitespace. into buffer 1 Outline fig 12_05. cpp (1 of 2) // use cin to input characters cout << "Enter a sentence: " << endl; cin >> buffer 1; // display buffer 1 contents cout << "n. The string read with cin was: " << endl No delimiter << buffer 1 << endl; specified, so the default (n) is used. // use cin. get to input characters into buffer 2 cin. get( buffer 2, SIZE ); 2003 Prentice Hall, Inc. All rights reserved. 22
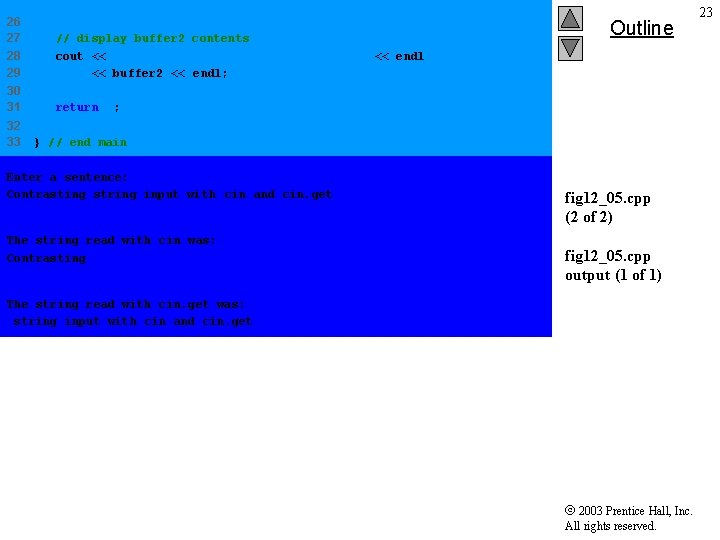
26 27 28 29 // display buffer 2 contents cout << "The string read with cin. get was: " << endl << buffer 2 << endl; 30 31 return 0; 32 33 Outline } // end main Enter a sentence: Contrasting string input with cin and cin. get The string read with cin was: Contrasting fig 12_05. cpp (2 of 2) fig 12_05. cpp output (1 of 1) The string read with cin. get was: string input with cin and cin. get 2003 Prentice Hall, Inc. All rights reserved. 23
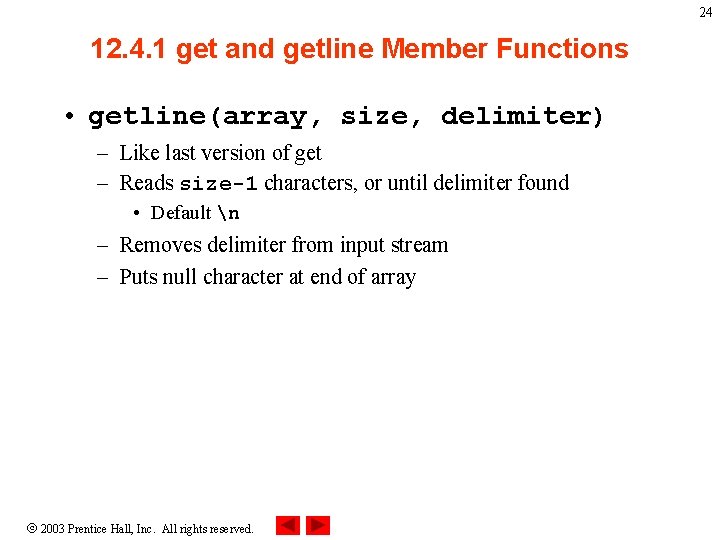
24 12. 4. 1 get and getline Member Functions • getline(array, size, delimiter) – Like last version of get – Reads size-1 characters, or until delimiter found • Default n – Removes delimiter from input stream – Puts null character at end of array 2003 Prentice Hall, Inc. All rights reserved.
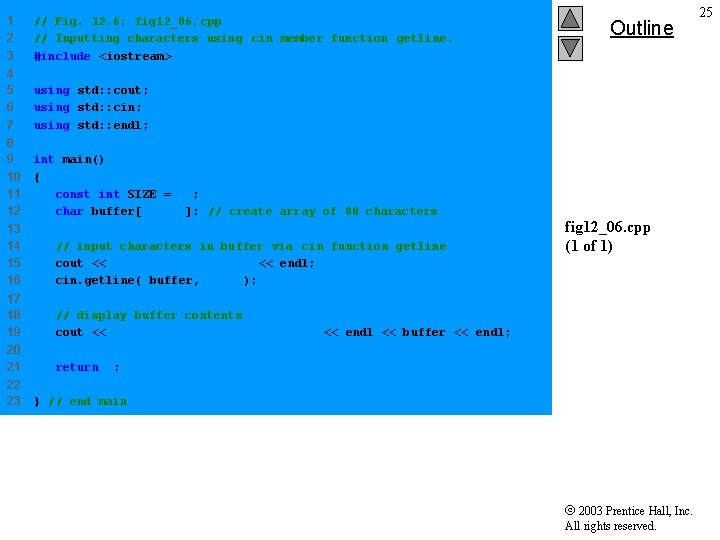
1 2 3 // Fig. 12. 6: fig 12_06. cpp // Inputting characters using cin member function getline. #include <iostream> 4 5 6 7 using std: : cout; using std: : cin; using std: : endl; 8 9 10 11 12 int main() { const int SIZE = 80; char buffer[ SIZE ]; // create array of 80 characters 13 14 15 16 // input characters in buffer via cin function getline cout << "Enter a sentence: " << endl; cin. getline( buffer, SIZE ); 17 18 19 // display buffer contents cout << "n. The sentence entered is: " << endl << buffer << endl; 20 21 return 0; 22 23 Outline fig 12_06. cpp (1 of 1) } // end main 2003 Prentice Hall, Inc. All rights reserved. 25
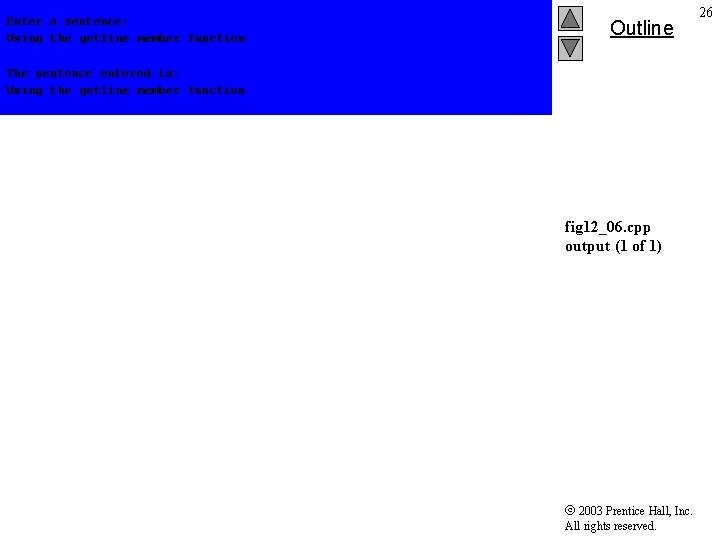
Enter a sentence: Using the getline member function Outline The sentence entered is: Using the getline member function fig 12_06. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 26
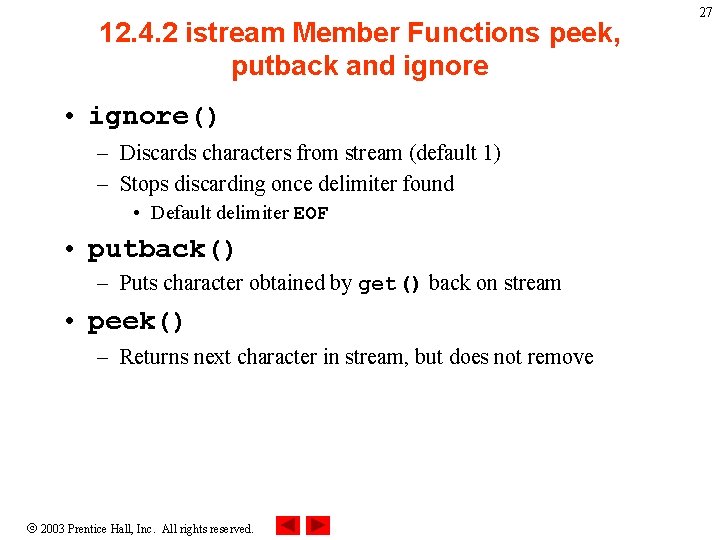
12. 4. 2 istream Member Functions peek, putback and ignore • ignore() – Discards characters from stream (default 1) – Stops discarding once delimiter found • Default delimiter EOF • putback() – Puts character obtained by get() back on stream • peek() – Returns next character in stream, but does not remove 2003 Prentice Hall, Inc. All rights reserved. 27
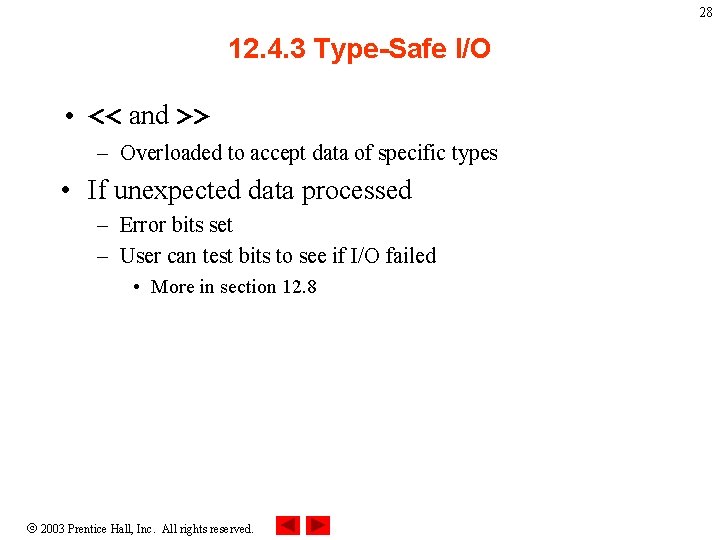
28 12. 4. 3 Type-Safe I/O • << and >> – Overloaded to accept data of specific types • If unexpected data processed – Error bits set – User can test bits to see if I/O failed • More in section 12. 8 2003 Prentice Hall, Inc. All rights reserved.
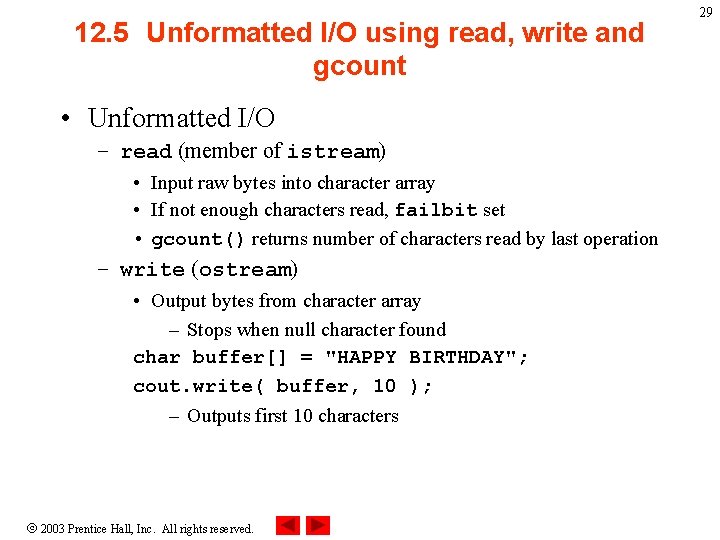
12. 5 Unformatted I/O using read, write and gcount • Unformatted I/O – read (member of istream) • Input raw bytes into character array • If not enough characters read, failbit set • gcount() returns number of characters read by last operation – write (ostream) • Output bytes from character array – Stops when null character found char buffer[] = "HAPPY BIRTHDAY"; cout. write( buffer, 10 ); – Outputs first 10 characters 2003 Prentice Hall, Inc. All rights reserved. 29
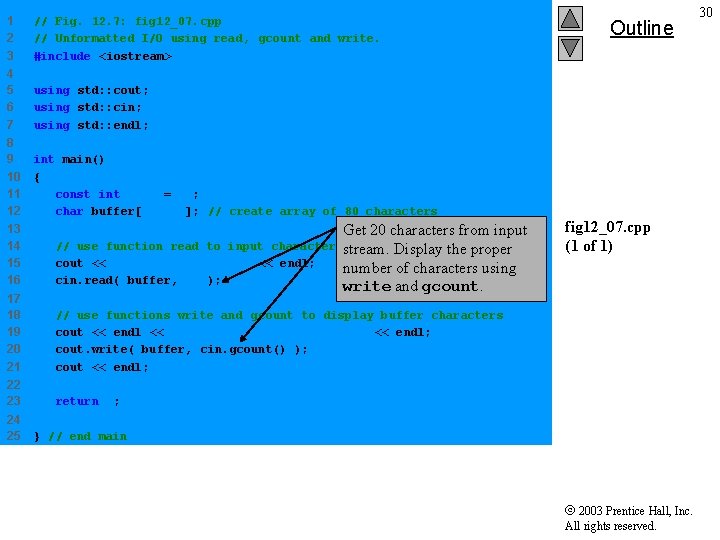
1 2 3 // Fig. 12. 7: fig 12_07. cpp // Unformatted I/O using read, gcount and write. #include <iostream> 4 5 6 7 using std: : cout; using std: : cin; using std: : endl; 8 9 10 11 12 int main() { const int SIZE = 80; char buffer[ SIZE ]; // create array of 80 characters 13 14 15 16 17 18 19 20 21 22 23 24 25 Get 20 characters from input // use function read to input charactersstream. into buffer Display the proper cout << "Enter a sentence: " << endl; number of characters using cin. read( buffer, 20 ); write and gcount. Outline fig 12_07. cpp (1 of 1) // use functions write and gcount to display buffer characters cout << endl << "The sentence entered was: " << endl; cout. write( buffer, cin. gcount() ); cout << endl; return 0; } // end main 2003 Prentice Hall, Inc. All rights reserved. 30
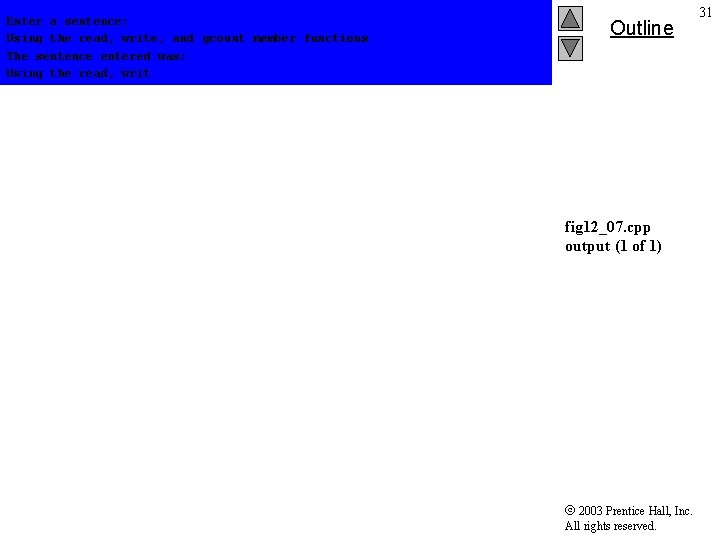
Enter a sentence: Using the read, write, and gcount member functions The sentence entered was: Using the read, writ Outline fig 12_07. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 31
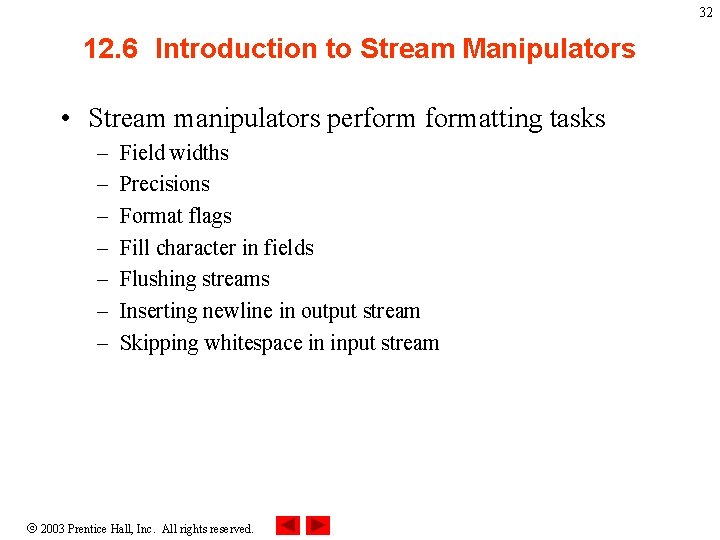
32 12. 6 Introduction to Stream Manipulators • Stream manipulators performatting tasks – – – – Field widths Precisions Format flags Fill character in fields Flushing streams Inserting newline in output stream Skipping whitespace in input stream 2003 Prentice Hall, Inc. All rights reserved.
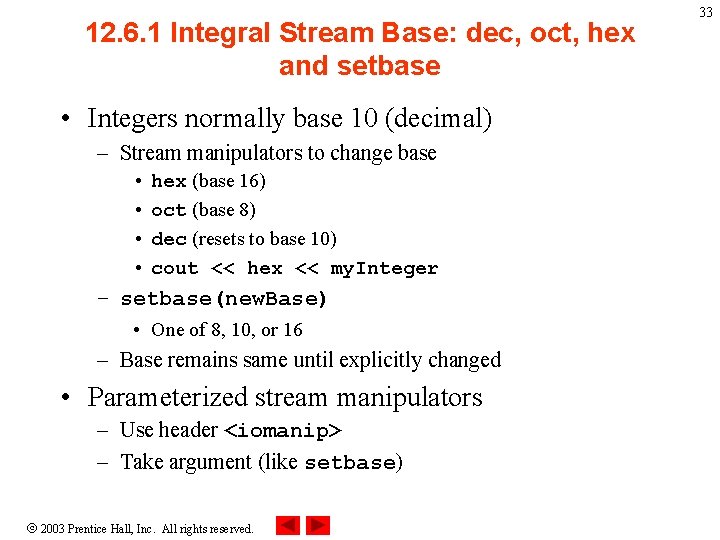
12. 6. 1 Integral Stream Base: dec, oct, hex and setbase • Integers normally base 10 (decimal) – Stream manipulators to change base • hex (base 16) • oct (base 8) • dec (resets to base 10) • cout << hex << my. Integer – setbase(new. Base) • One of 8, 10, or 16 – Base remains same until explicitly changed • Parameterized stream manipulators – Use header <iomanip> – Take argument (like setbase) 2003 Prentice Hall, Inc. All rights reserved. 33
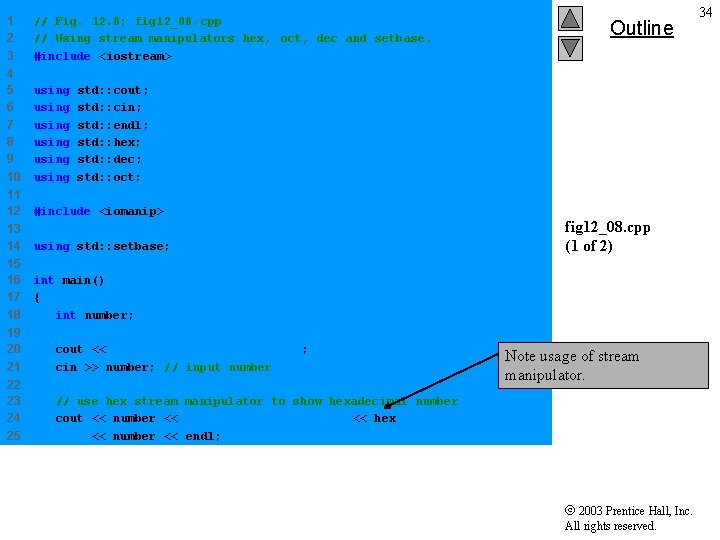
1 2 3 // Fig. 12. 8: fig 12_08. cpp // Using stream manipulators hex, oct, dec and setbase. #include <iostream> 4 5 6 7 8 9 10 using using 11 12 #include <iomanip> Outline std: : cout; std: : cin; std: : endl; std: : hex; std: : dec; std: : oct; 13 14 using std: : setbase; 15 16 17 18 int main() { int number; 19 20 21 cout << "Enter a decimal number: " ; cin >> number; // input number 22 23 24 25 // use hex stream manipulator to show hexadecimal number cout << number << " in hexadecimal is: " << hex << number << endl; fig 12_08. cpp (1 of 2) Note usage of stream manipulator. 2003 Prentice Hall, Inc. All rights reserved. 34
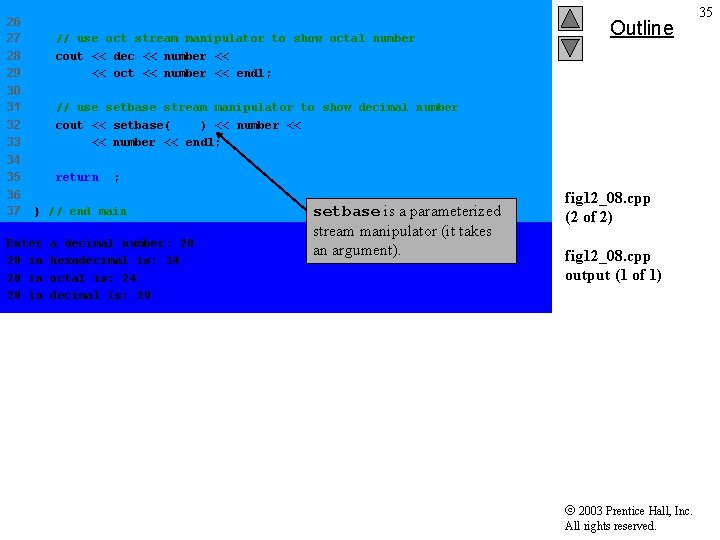
26 27 28 29 // use oct stream manipulator to show octal number cout << dec << number << " in octal is: " << oct << number << endl; 30 31 32 33 // use setbase stream manipulator to show decimal number cout << setbase( 10 ) << number << " in decimal is: " << number << endl; 34 35 return 0; 36 37 } // end main Enter 20 in a decimal number: 20 hexadecimal is: 14 octal is: 24 decimal is: 20 setbase is a parameterized stream manipulator (it takes an argument). Outline fig 12_08. cpp (2 of 2) fig 12_08. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 35
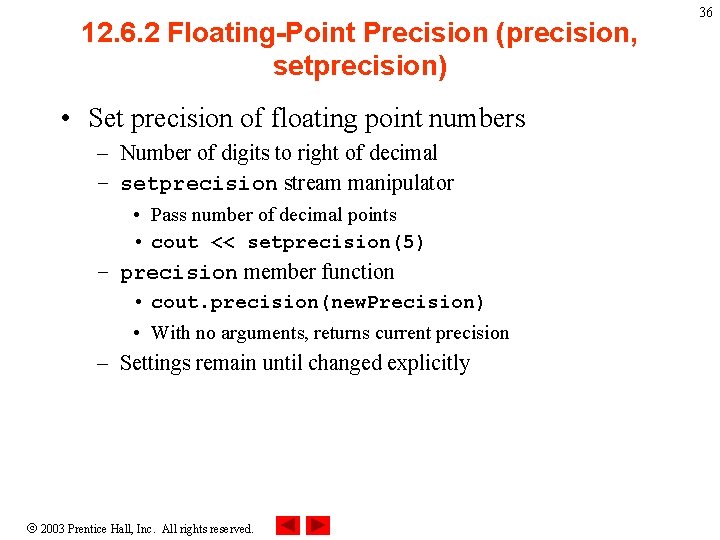
12. 6. 2 Floating-Point Precision (precision, setprecision) • Set precision of floating point numbers – Number of digits to right of decimal – setprecision stream manipulator • Pass number of decimal points • cout << setprecision(5) – precision member function • cout. precision(new. Precision) • With no arguments, returns current precision – Settings remain until changed explicitly 2003 Prentice Hall, Inc. All rights reserved. 36
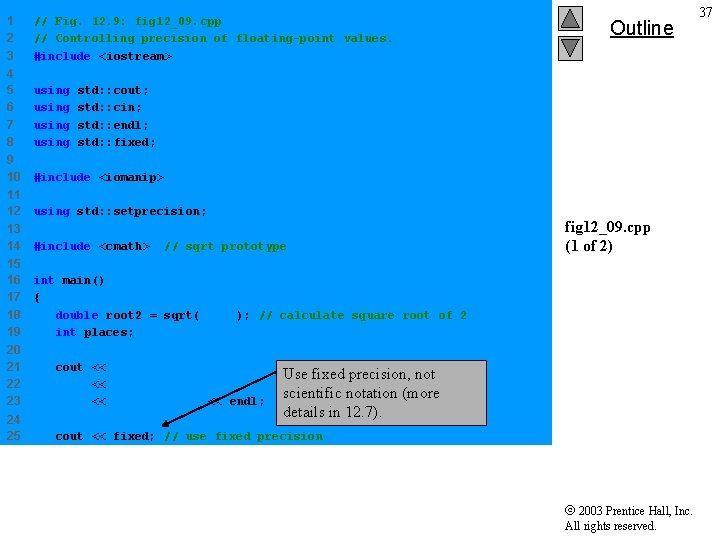
1 2 3 // Fig. 12. 9: fig 12_09. cpp // Controlling precision of floating-point values. #include <iostream> 4 5 6 7 8 using 9 10 #include <iomanip> 11 12 using std: : setprecision; Outline std: : cout; std: : cin; std: : endl; std: : fixed; 13 14 #include <cmath> 15 16 17 18 19 int main() { double root 2 = sqrt( 2. 0 ); // calculate square root of 2 int places; // sqrt prototype 20 21 22 23 cout << "Square root of 2 with precisions 0 -9. " << endl Use fixed precision, not << "Precision set by ios_base member-function " scientific notation (more << "precision: " << endl; 24 25 cout << fixed; // use fixed precision fig 12_09. cpp (1 of 2) details in 12. 7). 2003 Prentice Hall, Inc. All rights reserved. 37
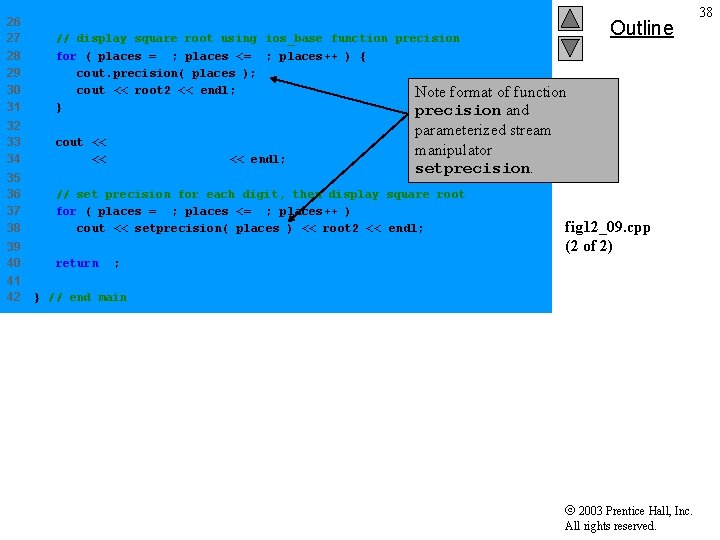
26 27 28 29 30 31 32 33 34 // display square root using ios_base function precision for ( places = 0; places <= 9; places++ ) { cout. precision( places ); cout << root 2 << endl; Note format of function } precision and cout << "n. Precision set by stream-manipulator " << "setprecision: " << endl; parameterized stream manipulator setprecision. 35 36 37 38 // set precision for each digit, then display square root for ( places = 0; places <= 9; places++ ) cout << setprecision( places ) << root 2 << endl; 39 40 return 0; 41 42 Outline fig 12_09. cpp (2 of 2) } // end main 2003 Prentice Hall, Inc. All rights reserved. 38
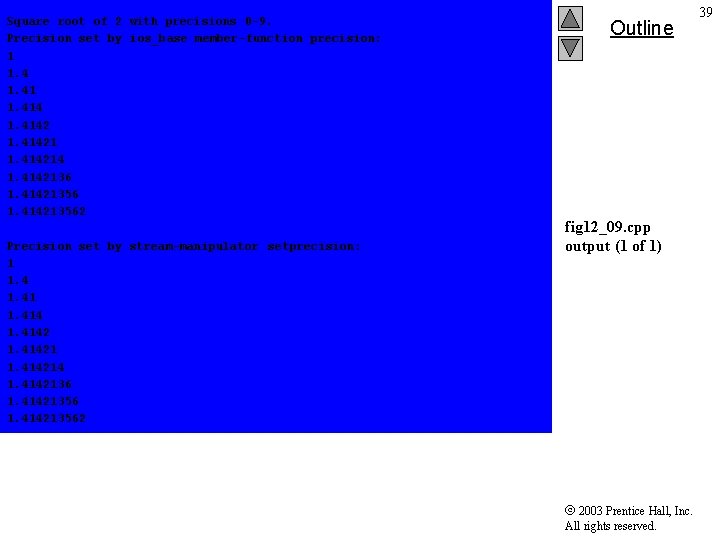
Square root of 2 with precisions 0 -9. Precision set by ios_base member-function precision: 1 1. 4142 1. 414214 1. 4142136 1. 414213562 Precision set by stream-manipulator setprecision: 1 1. 4142 1. 414214 1. 4142136 1. 414213562 Outline fig 12_09. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 39
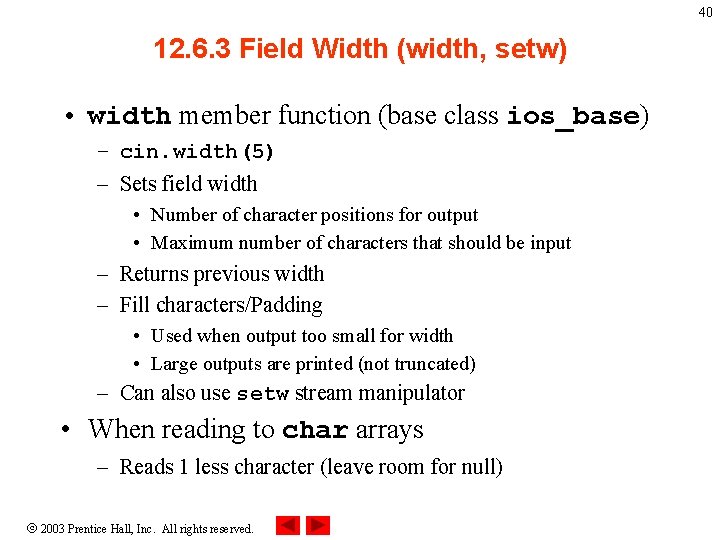
40 12. 6. 3 Field Width (width, setw) • width member function (base class ios_base) – cin. width(5) – Sets field width • Number of character positions for output • Maximum number of characters that should be input – Returns previous width – Fill characters/Padding • Used when output too small for width • Large outputs are printed (not truncated) – Can also use setw stream manipulator • When reading to char arrays – Reads 1 less character (leave room for null) 2003 Prentice Hall, Inc. All rights reserved.
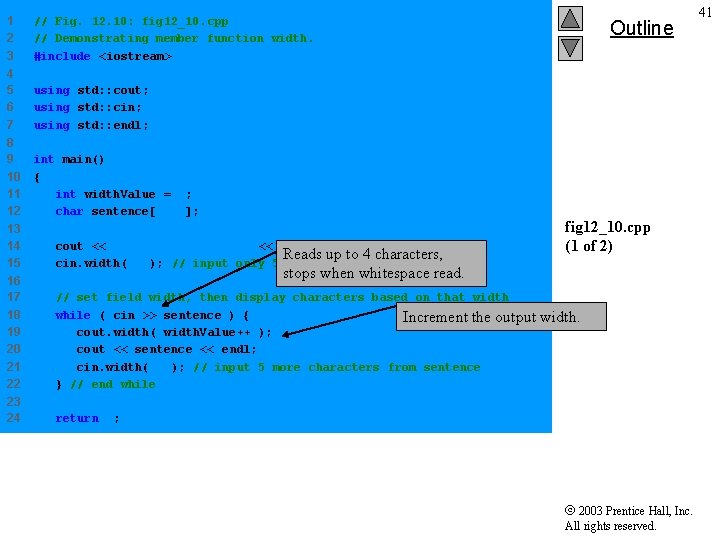
1 2 3 // Fig. 12. 10: fig 12_10. cpp // Demonstrating member function width. #include <iostream> 4 5 6 7 using std: : cout; using std: : cin; using std: : endl; 8 9 10 11 12 int main() { int width. Value = 4; char sentence[ 10 ]; 13 14 15 cout << "Enter a sentence: " << endl; Reads up to 4 characters, cin. width( 5 ); // input only 5 characters from sentence 16 17 18 19 20 21 22 // set field width, then display characters based on that width while ( cin >> sentence ) { Increment the output cout. width( width. Value++ ); cout << sentence << endl; cin. width( 5 ); // input 5 more characters from sentence } // end while 23 24 return 0; Outline fig 12_10. cpp (1 of 2) stops when whitespace read. width. 2003 Prentice Hall, Inc. All rights reserved. 41
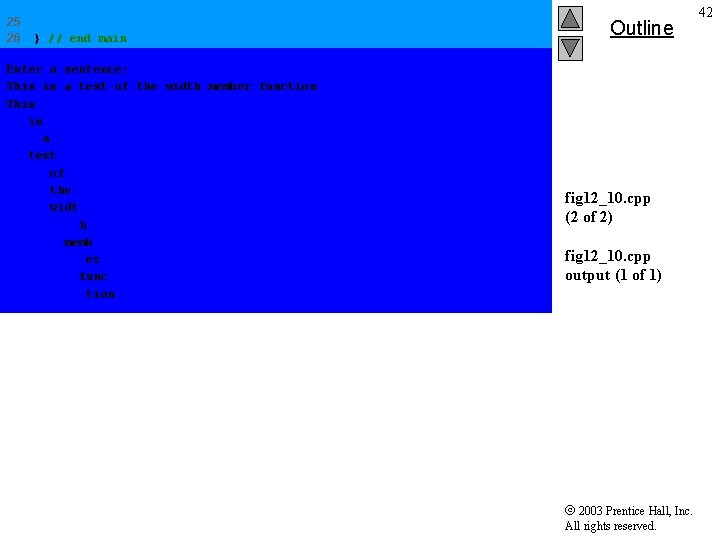
25 26 } // end main Enter a sentence: This is a test of the width member function This is a test of the widt h memb er func tion Outline fig 12_10. cpp (2 of 2) fig 12_10. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 42
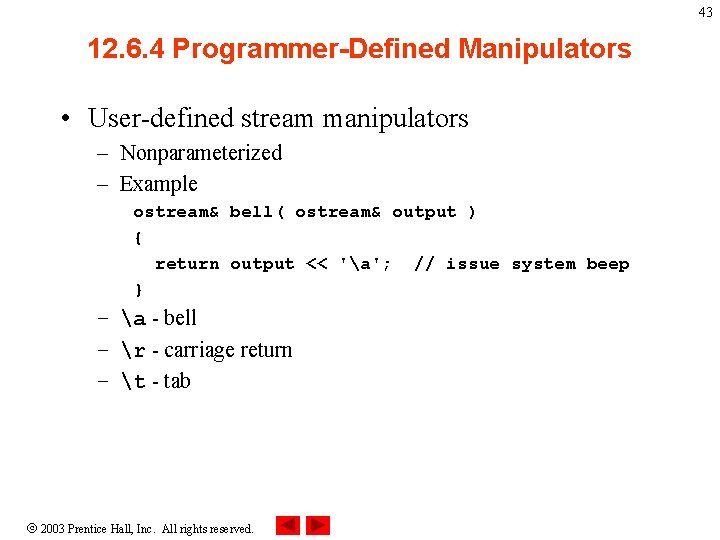
43 12. 6. 4 Programmer-Defined Manipulators • User-defined stream manipulators – Nonparameterized – Example ostream& bell( ostream& output ) { return output << 'a'; // issue system beep } – a - bell – r - carriage return – t - tab 2003 Prentice Hall, Inc. All rights reserved.
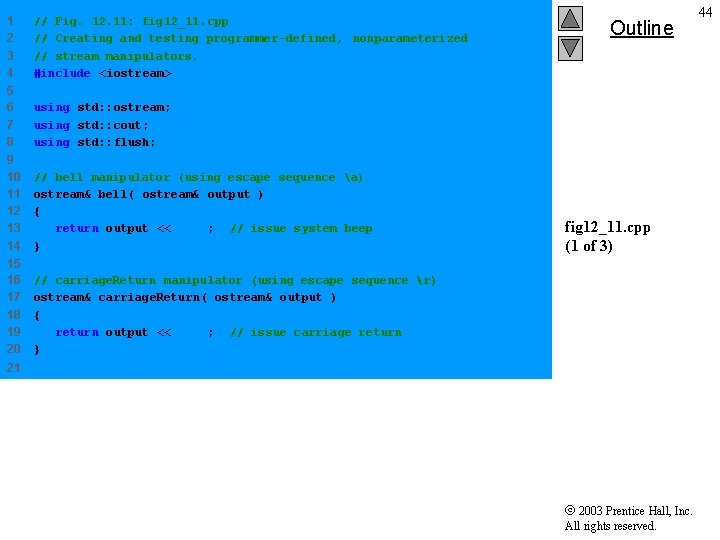
1 2 3 4 // Fig. 12. 11: fig 12_11. cpp // Creating and testing programmer-defined, nonparameterized // stream manipulators. #include <iostream> 5 6 7 8 using std: : ostream; using std: : cout; using std: : flush; 9 10 11 12 13 14 // bell manipulator (using escape sequence a) ostream& bell( ostream& output ) { return output << 'a'; // issue system beep } 15 16 17 18 19 20 // carriage. Return manipulator (using escape sequence r) ostream& carriage. Return( ostream& output ) { return output << 'r'; // issue carriage return } Outline fig 12_11. cpp (1 of 3) 21 2003 Prentice Hall, Inc. All rights reserved. 44
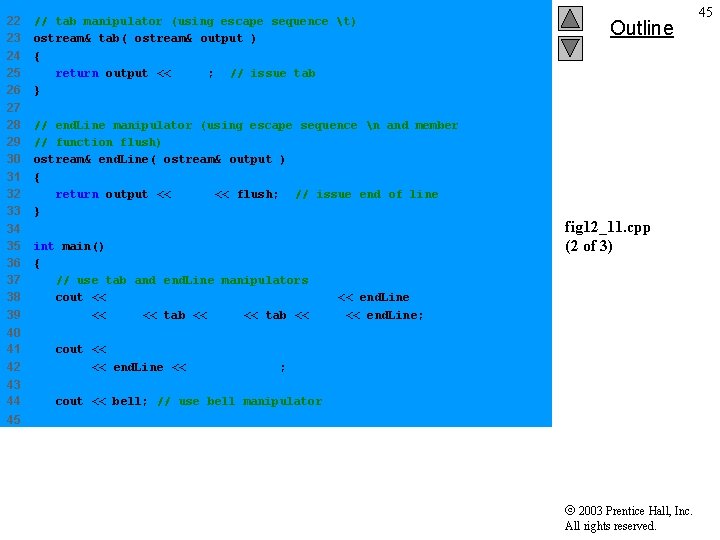
22 23 24 25 26 // tab manipulator (using escape sequence t) ostream& tab( ostream& output ) { return output << 't'; // issue tab } 27 28 29 30 31 32 33 // end. Line manipulator (using escape sequence n and member // function flush) ostream& end. Line( ostream& output ) { return output << 'n' << flush; // issue end of line } 34 35 36 37 38 39 int main() { // use tab and end. Line manipulators cout << "Testing the tab manipulator: " << end. Line << 'a' << tab << 'b' << tab << 'c' << end. Line; 40 41 42 cout << "Testing the carriage. Return and bell manipulators: " << end. Line << ". . "; 43 44 cout << bell; // use bell manipulator Outline fig 12_11. cpp (2 of 3) 45 2003 Prentice Hall, Inc. All rights reserved. 45
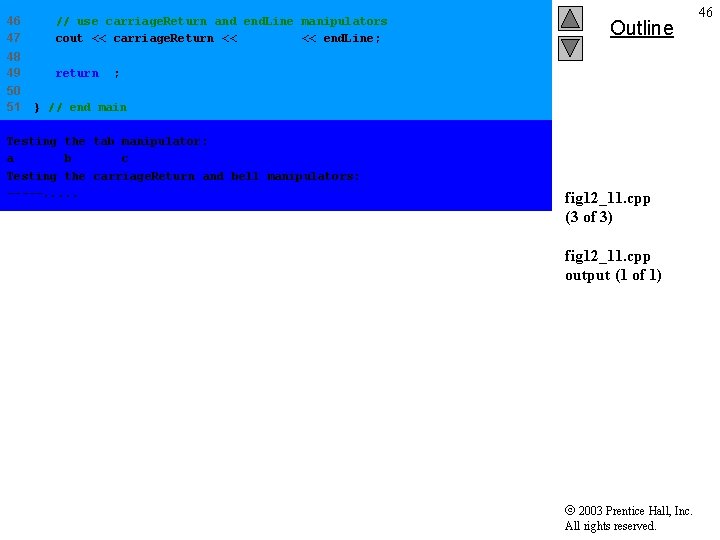
46 47 // use carriage. Return and end. Line manipulators cout << carriage. Return << "-----" << end. Line; 48 49 return 0; 50 51 Outline } // end main Testing the tab manipulator: a b c Testing the carriage. Return and bell manipulators: -----. . . fig 12_11. cpp (3 of 3) fig 12_11. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 46
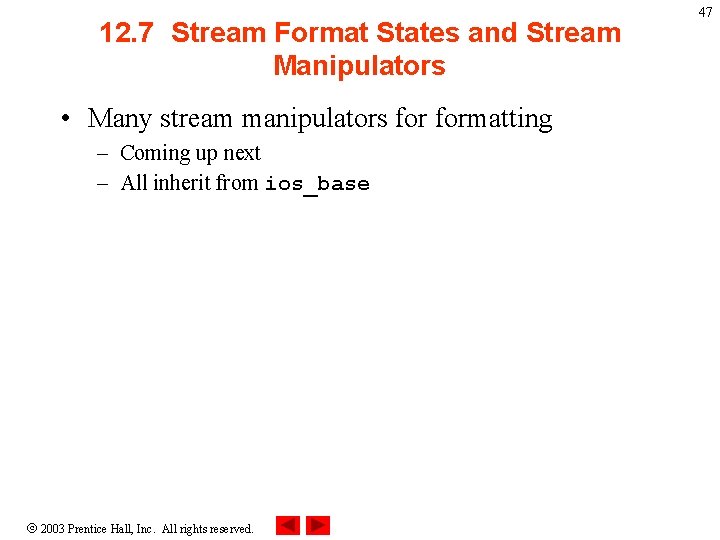
12. 7 Stream Format States and Stream Manipulators • Many stream manipulators formatting – Coming up next – All inherit from ios_base 2003 Prentice Hall, Inc. All rights reserved. 47
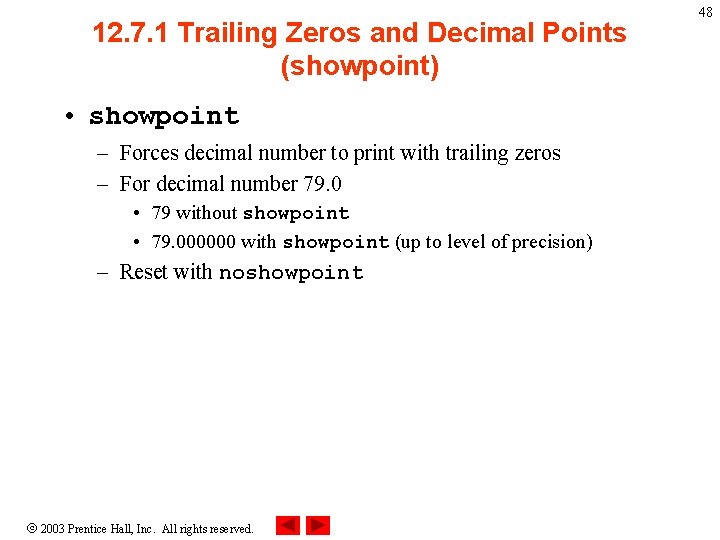
12. 7. 1 Trailing Zeros and Decimal Points (showpoint) • showpoint – Forces decimal number to print with trailing zeros – For decimal number 79. 0 • 79 without showpoint • 79. 000000 with showpoint (up to level of precision) – Reset with noshowpoint 2003 Prentice Hall, Inc. All rights reserved. 48
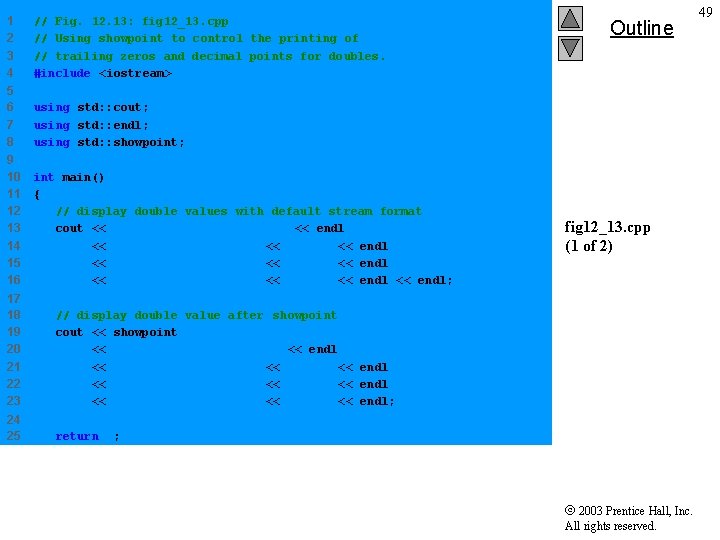
1 2 3 4 // Fig. 12. 13: fig 12_13. cpp // Using showpoint to control the printing of // trailing zeros and decimal points for doubles. #include <iostream> 5 6 7 8 using std: : cout; using std: : endl; using std: : showpoint; 9 10 11 12 13 14 15 16 int main() { // display double values with default stream format cout << "Before using showpoint" << endl << "9. 9900 prints as: " << 9. 9900 << endl << "9. 9000 prints as: " << 9. 9000 << endl << "9. 0000 prints as: " << 9. 0000 << endl; 17 18 19 20 21 22 23 // display double value after showpoint cout << showpoint << "After using showpoint" << endl << "9. 9900 prints as: " << 9. 9900 << endl << "9. 9000 prints as: " << 9. 9000 << endl << "9. 0000 prints as: " << 9. 0000 << endl; 24 25 return 0; Outline fig 12_13. cpp (1 of 2) 2003 Prentice Hall, Inc. All rights reserved. 49
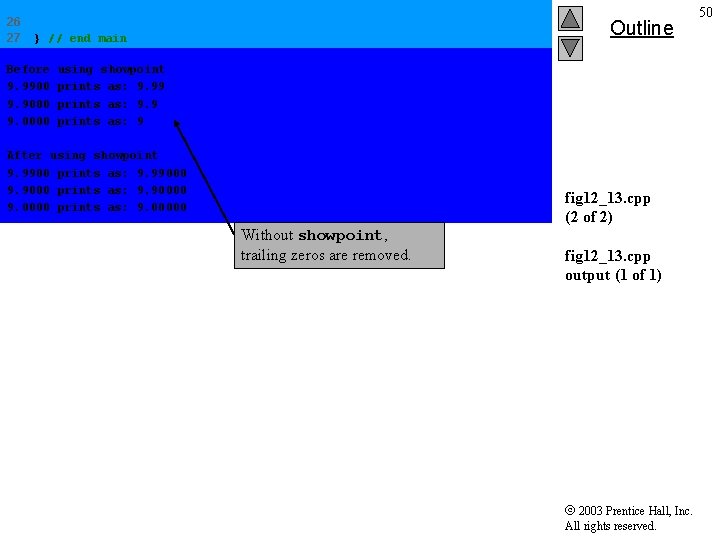
26 27 Outline } // end main Before 9. 9900 9. 9000 9. 0000 using showpoint prints as: 9. 99 prints as: 9 After using showpoint 9. 9900 prints as: 9. 99000 9. 9000 prints as: 9. 90000 9. 0000 prints as: 9. 00000 Without showpoint, trailing zeros are removed. fig 12_13. cpp (2 of 2) fig 12_13. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 50
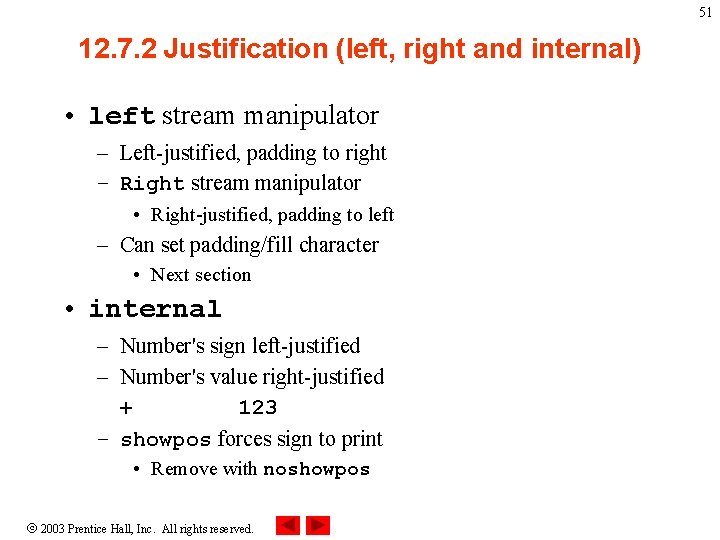
51 12. 7. 2 Justification (left, right and internal) • left stream manipulator – Left-justified, padding to right – Right stream manipulator • Right-justified, padding to left – Can set padding/fill character • Next section • internal – Number's sign left-justified – Number's value right-justified + 123 – showpos forces sign to print • Remove with noshowpos 2003 Prentice Hall, Inc. All rights reserved.
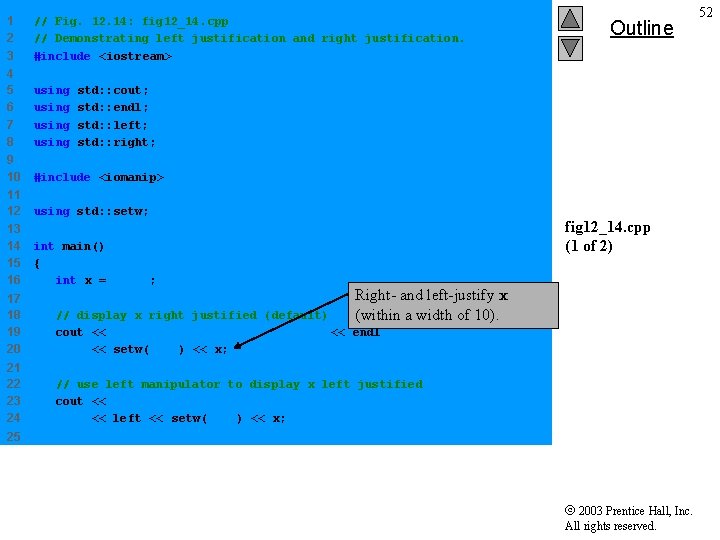
1 2 3 // Fig. 12. 14: fig 12_14. cpp // Demonstrating left justification and right justification. #include <iostream> 4 5 6 7 8 using 9 10 #include <iomanip> 11 12 using std: : setw; 13 14 15 16 Outline std: : cout; std: : endl; std: : left; std: : right; int main() { int x = 12345; fig 12_14. cpp (1 of 2) Right- and left-justify x (within a width of 10). 17 18 19 20 // display x right justified (default) cout << "Default is right justified: " << endl << setw( 10 ) << x; 21 22 23 24 // use left manipulator to display x left justified cout << "nn. Use std: : left to left justify x: n" << left << setw( 10 ) << x; 25 2003 Prentice Hall, Inc. All rights reserved. 52
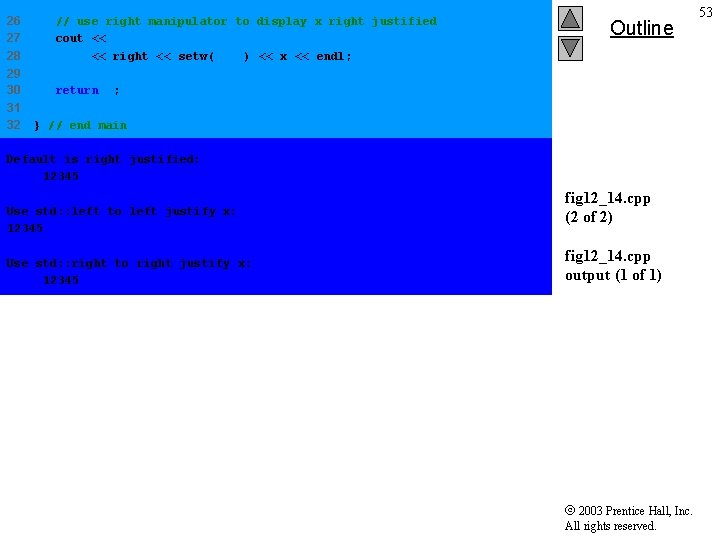
26 27 28 // use right manipulator to display x right justified cout << "nn. Use std: : right to right justify x: n" << right << setw( 10 ) << x << endl; 29 30 return 0; 31 32 Outline } // end main Default is right justified: 12345 Use std: : left to left justify x: 12345 Use std: : right to right justify x: 12345 fig 12_14. cpp (2 of 2) fig 12_14. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 53
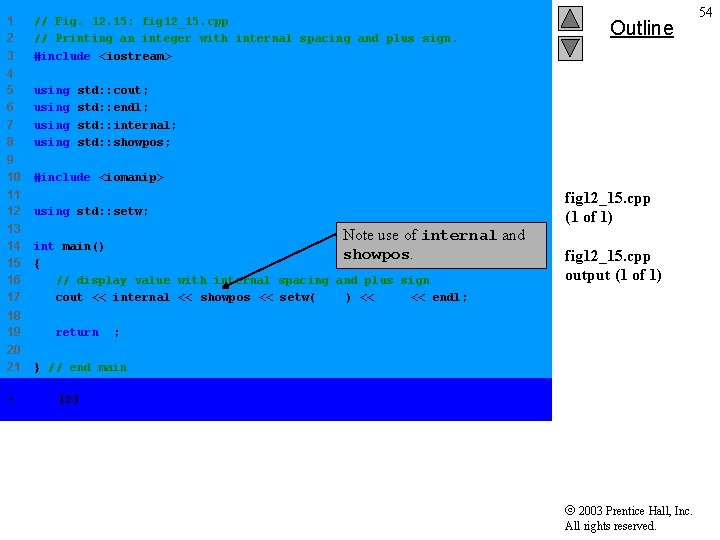
1 2 3 // Fig. 12. 15: fig 12_15. cpp // Printing an integer with internal spacing and plus sign. #include <iostream> 4 5 6 7 8 using 9 10 #include <iomanip> 11 12 using std: : setw; 13 14 15 16 17 int main() showpos. { // display value with internal spacing and plus sign cout << internal << showpos << setw( 10 ) << 123 << endl; 18 19 20 21 + Outline std: : cout; std: : endl; std: : internal; std: : showpos; Note use of internal and fig 12_15. cpp (1 of 1) fig 12_15. cpp output (1 of 1) return 0; } // end main 123 2003 Prentice Hall, Inc. All rights reserved. 54
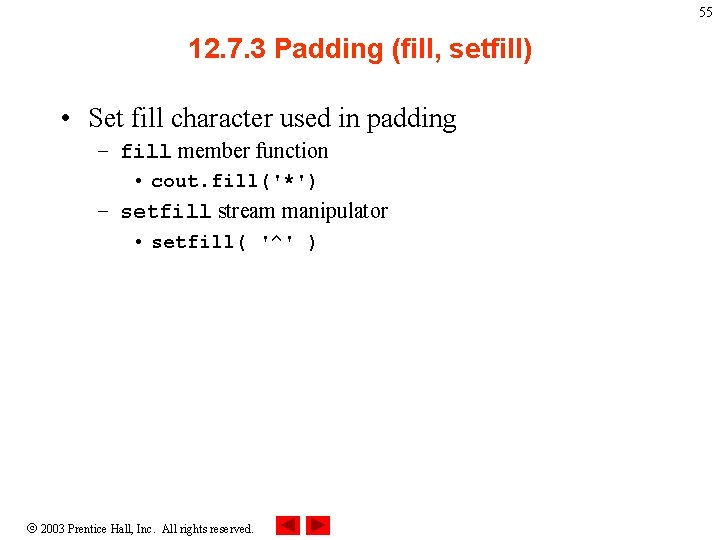
55 12. 7. 3 Padding (fill, setfill) • Set fill character used in padding – fill member function • cout. fill('*') – setfill stream manipulator • setfill( '^' ) 2003 Prentice Hall, Inc. All rights reserved.
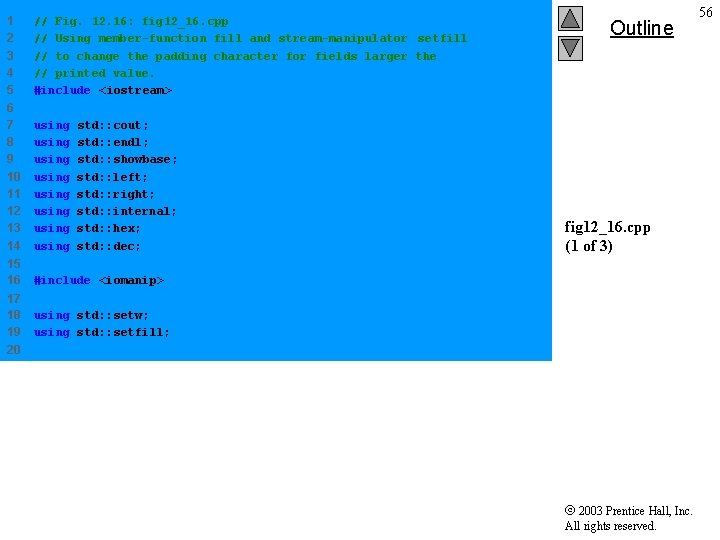
1 2 3 4 5 // Fig. 12. 16: fig 12_16. cpp // Using member-function fill and stream-manipulator setfill // to change the padding character for fields larger the // printed value. #include <iostream> 6 7 8 9 10 11 12 13 14 using using 15 16 #include <iomanip> 17 18 19 using std: : setw; using std: : setfill; std: : cout; std: : endl; std: : showbase; std: : left; std: : right; std: : internal; std: : hex; std: : dec; Outline fig 12_16. cpp (1 of 3) 20 2003 Prentice Hall, Inc. All rights reserved. 56
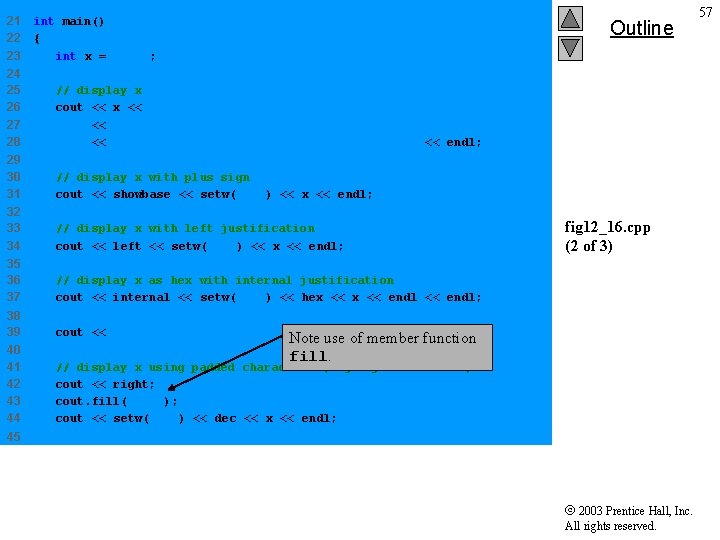
21 22 23 int main() { int x = 10000; Outline 24 25 26 27 28 // display x cout << x << " printed as int right and left justifiedn" << "and as hex with internal justification. n" << "Using the default pad character (space): " << endl; 29 30 31 // display x with plus sign cout << showbase << setw( 10 ) << x << endl; 32 33 34 // display x with left justification cout << left << setw( 10 ) << x << endl; 35 36 37 // display x as hex with internal justification cout << internal << setw( 10 ) << hex << endl; 38 39 cout << "Using various padding characters: " << endl; 40 41 42 43 44 // display x using padded characters (right justification) cout << right; cout. fill( '*' ); cout << setw( 10 ) << dec << x << endl; fig 12_16. cpp (2 of 3) Note use of member function fill. 45 2003 Prentice Hall, Inc. All rights reserved. 57
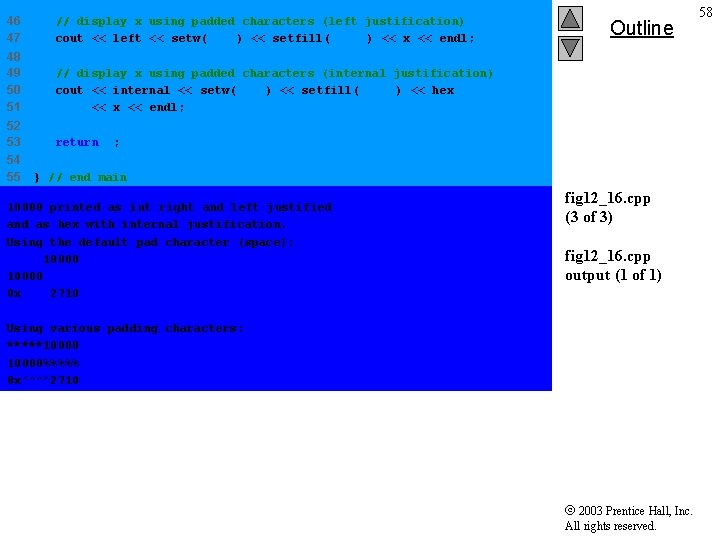
46 47 // display x using padded characters (left justification) cout << left << setw( 10 ) << setfill( '%' ) << x << endl; 48 49 50 51 // display x using padded characters (internal justification) cout << internal << setw( 10 ) << setfill( '^' ) << hex << endl; 52 53 return 0; 54 55 Outline } // end main 10000 printed as int right and left justified and as hex with internal justification. Using the default pad character (space): 10000 0 x 2710 fig 12_16. cpp (3 of 3) fig 12_16. cpp output (1 of 1) Using various padding characters: *****10000%%%%% 0 x^^^^2710 2003 Prentice Hall, Inc. All rights reserved. 58
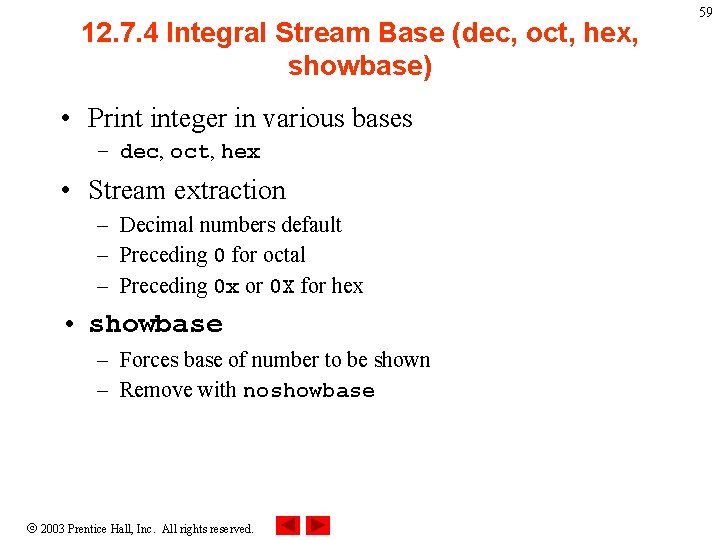
12. 7. 4 Integral Stream Base (dec, oct, hex, showbase) • Print integer in various bases – dec, oct, hex • Stream extraction – Decimal numbers default – Preceding 0 for octal – Preceding 0 x or 0 X for hex • showbase – Forces base of number to be shown – Remove with noshowbase 2003 Prentice Hall, Inc. All rights reserved. 59
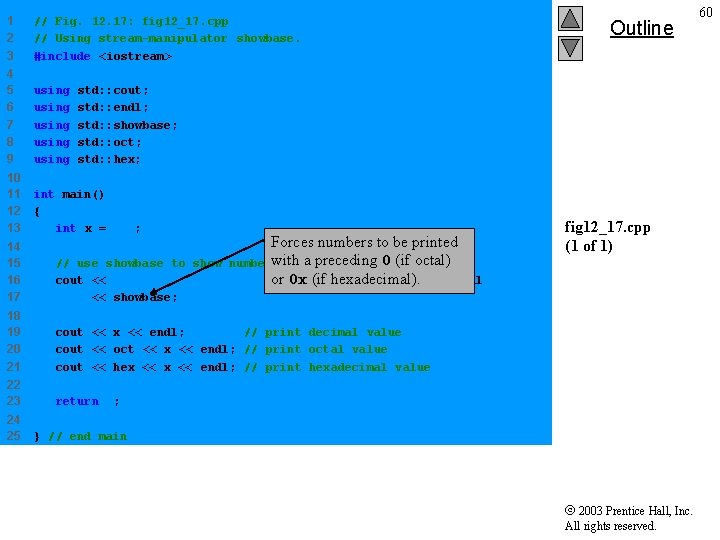
1 2 3 // Fig. 12. 17: fig 12_17. cpp // Using stream-manipulator showbase. #include <iostream> 4 5 6 7 8 9 using using 10 11 12 13 int main() { int x = 100; 14 15 16 17 std: : cout; std: : endl; std: : showbase; std: : oct; std: : hex; Forces numbers to be printed // use showbase to show numberwith basea preceding 0 (if octal) or 0 x (if cout << "Printing integers preceded by hexadecimal). their base: " << endl fig 12_17. cpp (1 of 1) << showbase; 18 19 20 21 cout << x << endl; // print decimal value cout << oct << x << endl; // print octal value cout << hex << endl; // print hexadecimal value 22 23 return 0; 24 25 Outline } // end main 2003 Prentice Hall, Inc. All rights reserved. 60
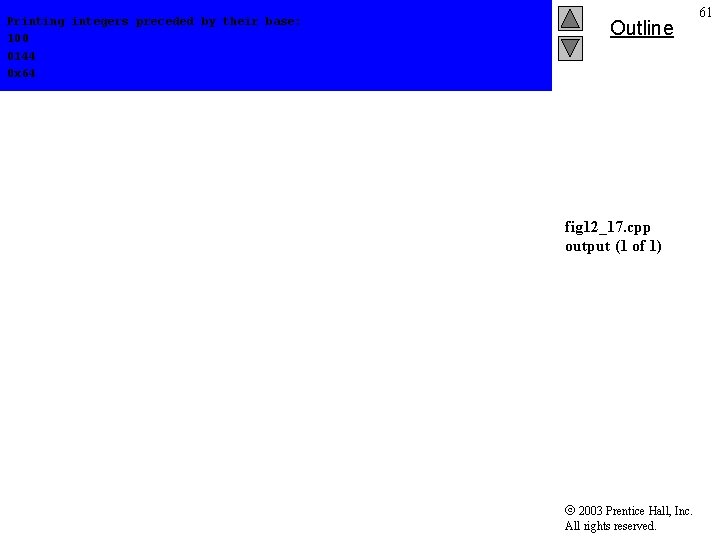
Printing integers preceded by their base: 100 0144 0 x 64 Outline fig 12_17. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 61
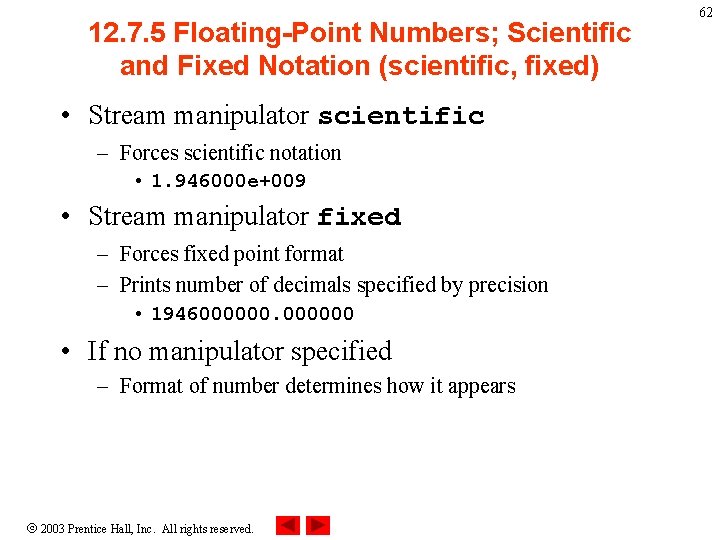
12. 7. 5 Floating-Point Numbers; Scientific and Fixed Notation (scientific, fixed) • Stream manipulator scientific – Forces scientific notation • 1. 946000 e+009 • Stream manipulator fixed – Forces fixed point format – Prints number of decimals specified by precision • 1946000000 • If no manipulator specified – Format of number determines how it appears 2003 Prentice Hall, Inc. All rights reserved. 62
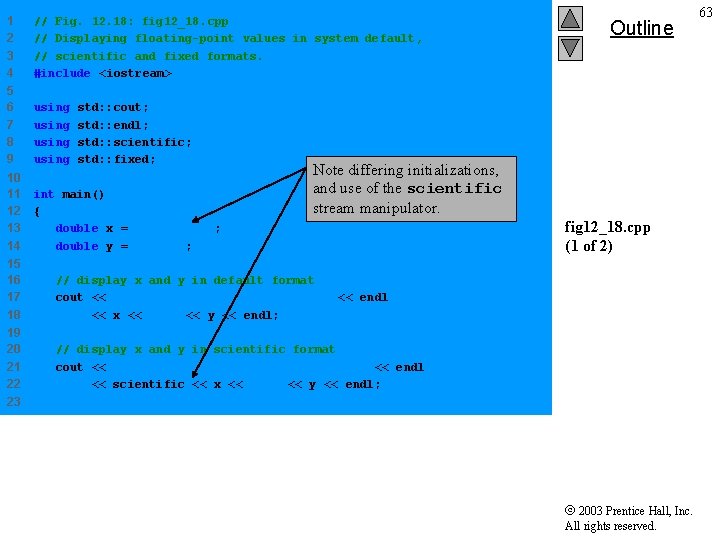
1 2 3 4 // Fig. 12. 18: fig 12_18. cpp // Displaying floating-point values in system default, // scientific and fixed formats. #include <iostream> 5 6 7 8 9 using 10 11 12 13 14 int main() { double x = 0. 001234567; double y = 1. 946 e 9; std: : cout; std: : endl; std: : scientific; std: : fixed; Outline Note differing initializations, and use of the scientific stream manipulator. 15 16 17 18 // display x and y in default format cout << "Displayed in default format: " << endl << x << 't' << y << endl; 19 20 21 22 // display x and y in scientific format cout << "n. Displayed in scientific format: " << endl << scientific << x << 't' << y << endl; fig 12_18. cpp (1 of 2) 23 2003 Prentice Hall, Inc. All rights reserved. 63
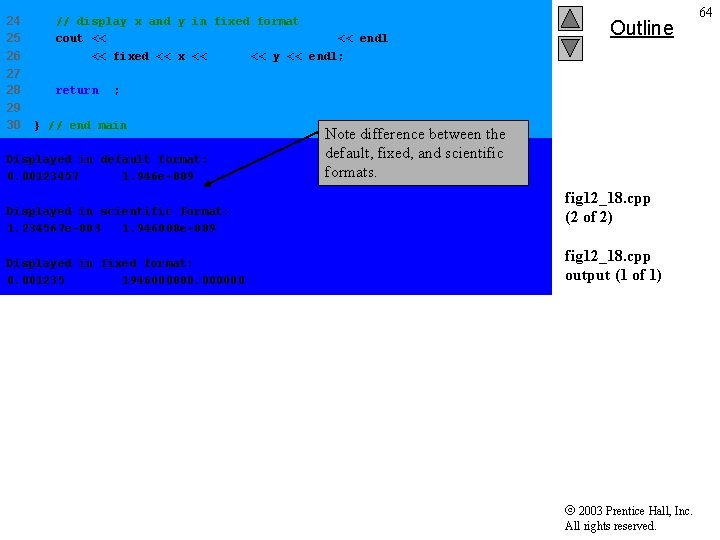
24 25 26 // display x and y in fixed format cout << "n. Displayed in fixed format: " << endl << fixed << x << 't' << y << endl; 27 28 return 0; 29 30 } // end main Displayed in default format: 0. 00123457 1. 946 e+009 Displayed in scientific format: 1. 234567 e-003 1. 946000 e+009 Displayed in fixed format: 0. 001235 1946000000 Outline Note difference between the default, fixed, and scientific formats. fig 12_18. cpp (2 of 2) fig 12_18. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 64
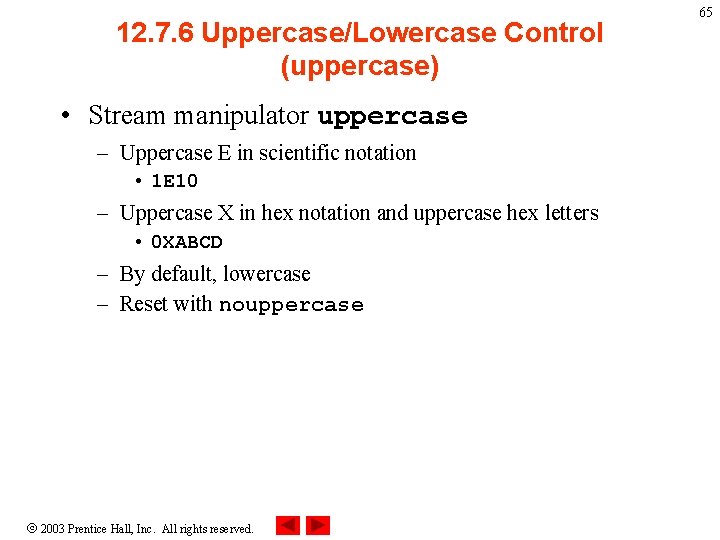
12. 7. 6 Uppercase/Lowercase Control (uppercase) • Stream manipulator uppercase – Uppercase E in scientific notation • 1 E 10 – Uppercase X in hex notation and uppercase hex letters • 0 XABCD – By default, lowercase – Reset with nouppercase 2003 Prentice Hall, Inc. All rights reserved. 65
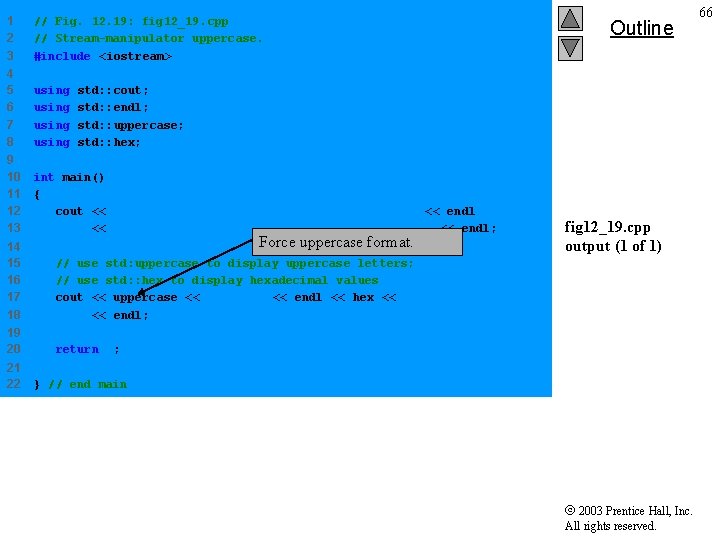
1 2 3 // Fig. 12. 19: fig 12_19. cpp // Stream-manipulator uppercase. #include <iostream> 4 5 6 7 8 using 9 10 11 12 13 int main() { cout << "Printing uppercase letters in scientific" << endl << "notation exponents and hexadecimal values: " << endl; std: : cout; std: : endl; std: : uppercase; std: : hex; Force uppercase format. 14 15 16 17 18 // use std: uppercase to display uppercase letters; // use std: : hex to display hexadecimal values cout << uppercase << 4. 345 e 10 << endl << hex << 123456789 << endl; 19 20 return 0; 21 22 Outline fig 12_19. cpp output (1 of 1) } // end main 2003 Prentice Hall, Inc. All rights reserved. 66
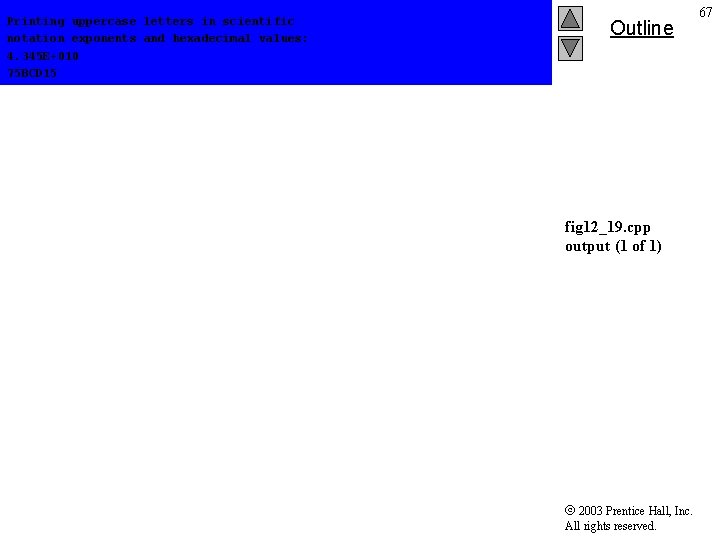
Printing uppercase letters in scientific notation exponents and hexadecimal values: 4. 345 E+010 75 BCD 15 Outline fig 12_19. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 67
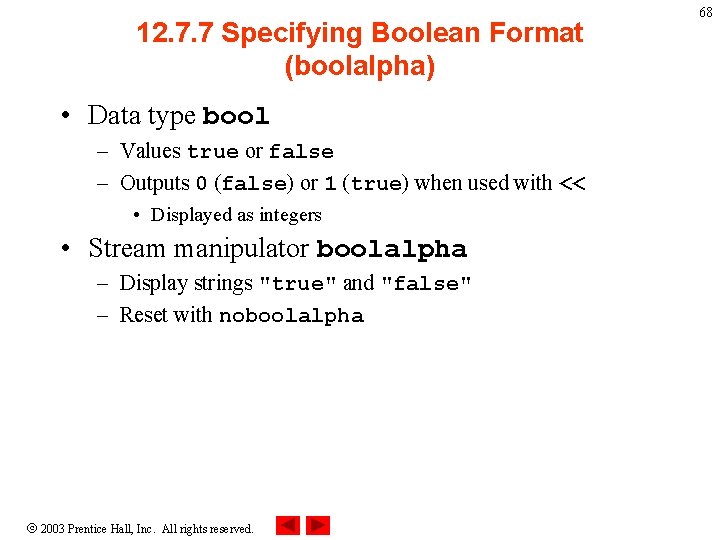
12. 7. 7 Specifying Boolean Format (boolalpha) • Data type bool – Values true or false – Outputs 0 (false) or 1 (true) when used with << • Displayed as integers • Stream manipulator boolalpha – Display strings "true" and "false" – Reset with noboolalpha 2003 Prentice Hall, Inc. All rights reserved. 68
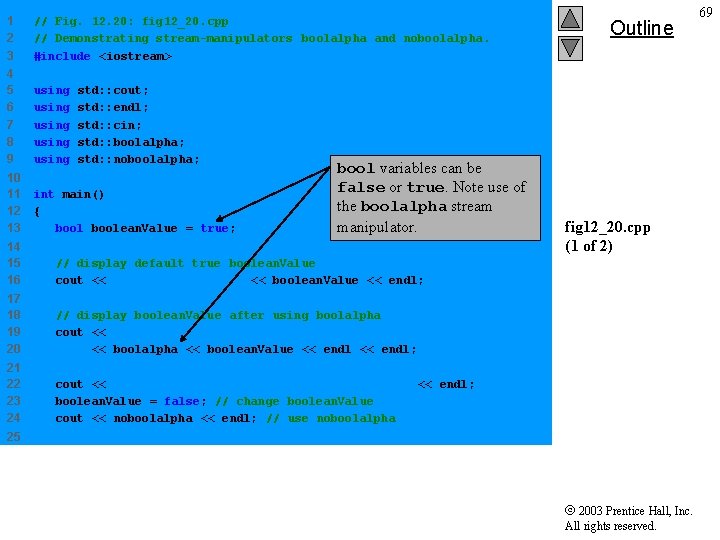
1 2 3 // Fig. 12. 20: fig 12_20. cpp // Demonstrating stream-manipulators boolalpha and noboolalpha. #include <iostream> 4 5 6 7 8 9 using using 10 11 12 13 int main() { boolean. Value = true; std: : cout; std: : endl; std: : cin; std: : boolalpha; std: : noboolalpha; bool variables can be false or true. Note use of the boolalpha stream manipulator. 14 15 16 // display default true boolean. Value cout << "boolean. Value is " << boolean. Value << endl; 17 18 19 20 // display boolean. Value after using boolalpha cout << "boolean. Value (after using boolalpha) is " << boolalpha << boolean. Value << endl; 21 22 23 24 cout << "switch boolean. Value and use noboolalpha" << endl; boolean. Value = false; // change boolean. Value cout << noboolalpha << endl; // use noboolalpha Outline fig 12_20. cpp (1 of 2) 25 2003 Prentice Hall, Inc. All rights reserved. 69
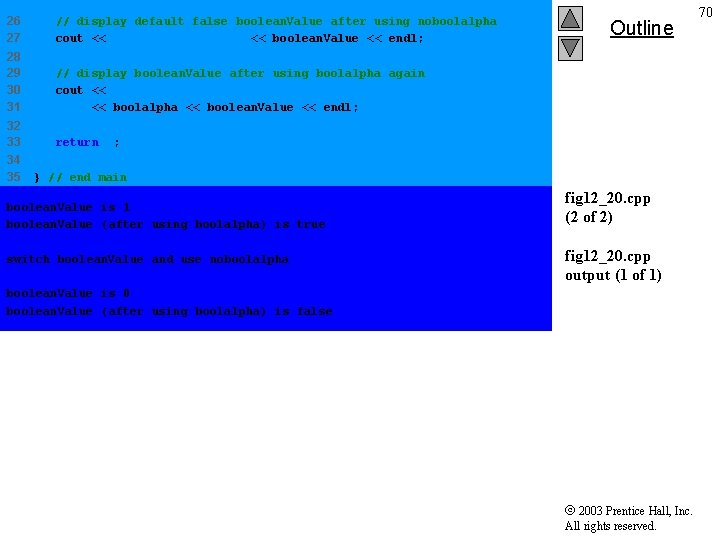
26 27 // display default false boolean. Value after using noboolalpha cout << "boolean. Value is " << boolean. Value << endl; 28 29 30 31 // display boolean. Value after using boolalpha again cout << "boolean. Value (after using boolalpha) is " << boolalpha << boolean. Value << endl; 32 33 return 0; 34 35 Outline } // end main boolean. Value is 1 boolean. Value (after using boolalpha) is true switch boolean. Value and use noboolalpha fig 12_20. cpp (2 of 2) fig 12_20. cpp output (1 of 1) boolean. Value is 0 boolean. Value (after using boolalpha) is false 2003 Prentice Hall, Inc. All rights reserved. 70
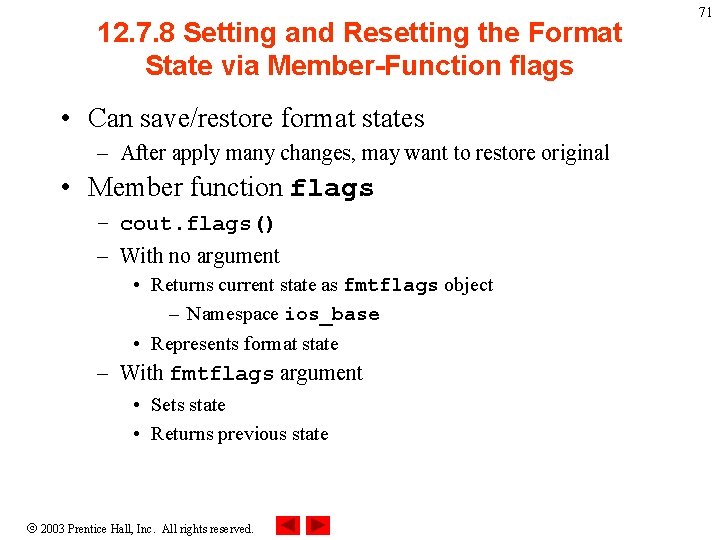
12. 7. 8 Setting and Resetting the Format State via Member-Function flags • Can save/restore format states – After apply many changes, may want to restore original • Member function flags – cout. flags() – With no argument • Returns current state as fmtflags object – Namespace ios_base • Represents format state – With fmtflags argument • Sets state • Returns previous state 2003 Prentice Hall, Inc. All rights reserved. 71
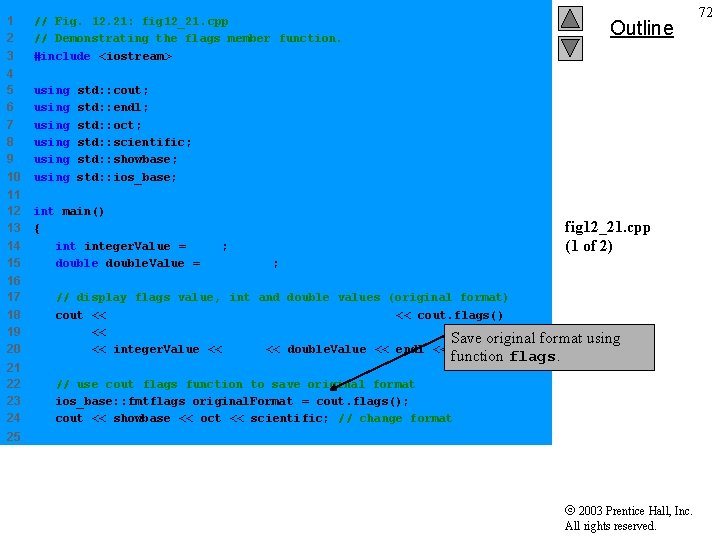
1 2 3 // Fig. 12. 21: fig 12_21. cpp // Demonstrating the flags member function. #include <iostream> 4 5 6 7 8 9 10 using using 11 12 13 14 15 int main() { integer. Value = 1000; double. Value = 0. 0947628; 16 17 18 19 20 // display flags value, int and cout << "The value of the flags << "n. Print and double << integer. Value << 't' << 21 22 23 24 // use cout flags function to save original format ios_base: : fmtflags original. Format = cout. flags(); cout << showbase << oct << scientific; // change format Outline std: : cout; std: : endl; std: : oct; std: : scientific; std: : showbase; std: : ios_base; fig 12_21. cpp (1 of 2) double values (original format) variable is: " << cout. flags() in original format: n" Save original double. Value << endl; format using function flags. 25 2003 Prentice Hall, Inc. All rights reserved. 72
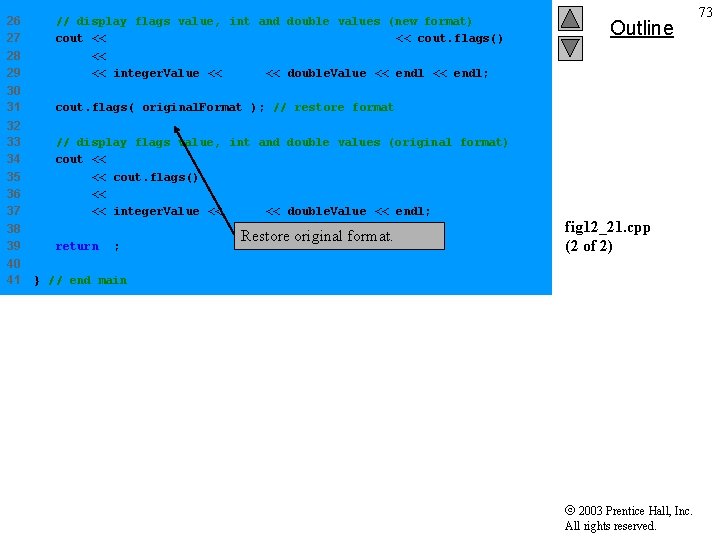
26 27 28 29 // display flags value, int and cout << "The value of the flags << "n. Print and double << integer. Value << 't' << 30 31 cout. flags( original. Format ); // restore format 32 33 34 35 36 37 // display flags value, int and double values (original format) cout << "The restored value of the flags variable is: " << cout. flags() << "n. Print values in original format again: n" << integer. Value << 't' << double. Value << endl; 38 39 40 41 return 0; double values (new format) variable is: " << cout. flags() in a new format: n" double. Value << endl; Restore original format. Outline fig 12_21. cpp (2 of 2) } // end main 2003 Prentice Hall, Inc. All rights reserved. 73
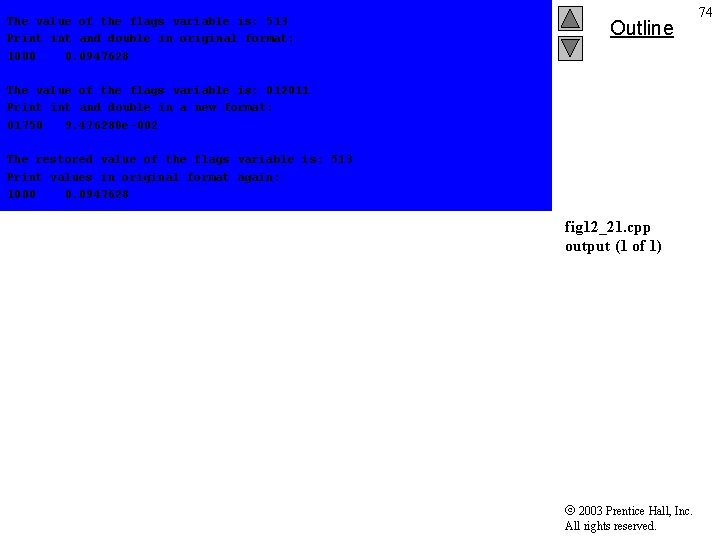
The value of the flags variable is: 513 Print and double in original format: 1000 0. 0947628 Outline The value of the flags variable is: 012011 Print and double in a new format: 01750 9. 476280 e-002 The restored value of the flags variable is: 513 Print values in original format again: 1000 0. 0947628 fig 12_21. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 74
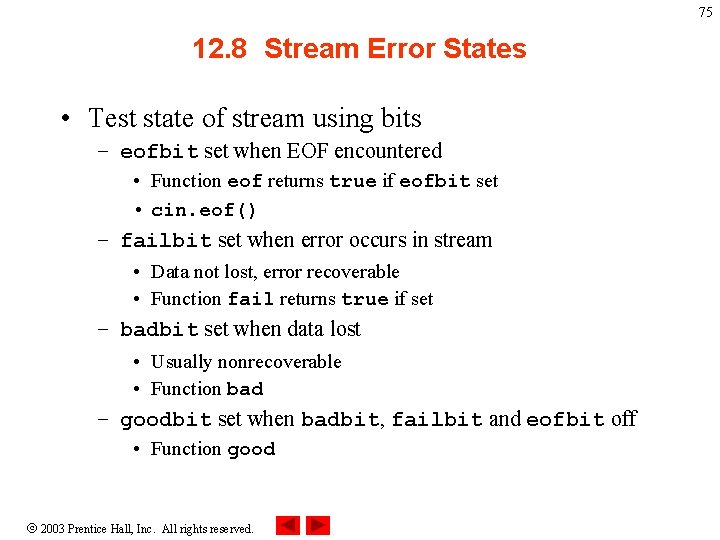
75 12. 8 Stream Error States • Test state of stream using bits – eofbit set when EOF encountered • Function eof returns true if eofbit set • cin. eof() – failbit set when error occurs in stream • Data not lost, error recoverable • Function fail returns true if set – badbit set when data lost • Usually nonrecoverable • Function bad – goodbit set when badbit, failbit and eofbit off • Function good 2003 Prentice Hall, Inc. All rights reserved.
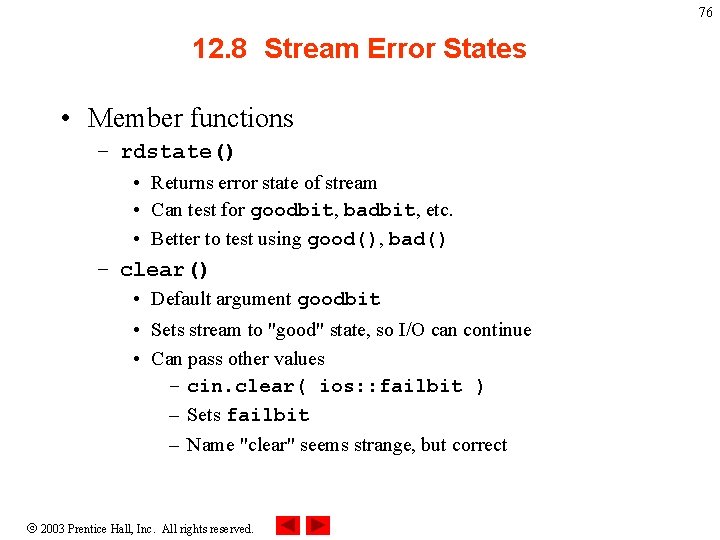
76 12. 8 Stream Error States • Member functions – rdstate() • Returns error state of stream • Can test for goodbit, badbit, etc. • Better to test using good(), bad() – clear() • Default argument goodbit • Sets stream to "good" state, so I/O can continue • Can pass other values – cin. clear( ios: : failbit ) – Sets failbit – Name "clear" seems strange, but correct 2003 Prentice Hall, Inc. All rights reserved.
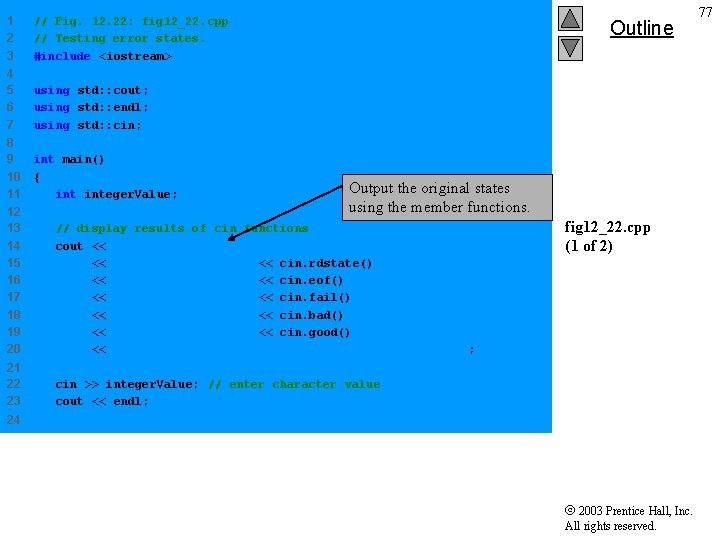
1 2 3 // Fig. 12. 22: fig 12_22. cpp // Testing error states. #include <iostream> 4 5 6 7 using std: : cout; using std: : endl; using std: : cin; 8 9 10 11 int main() { integer. Value; Outline Output the original states using the member functions. 12 13 14 15 16 17 18 19 20 // display results of cin functions cout << "Before a bad input operation: " << "ncin. rdstate(): " << cin. rdstate() << "n cin. eof(): " << cin. eof() << "n cin. fail(): " << cin. fail() << "n cin. bad(): " << cin. bad() << "n cin. good(): " << cin. good() << "nn. Expects an integer, but enter a character: " ; 21 22 23 cin >> integer. Value; // enter character value cout << endl; fig 12_22. cpp (1 of 2) 24 2003 Prentice Hall, Inc. All rights reserved. 77
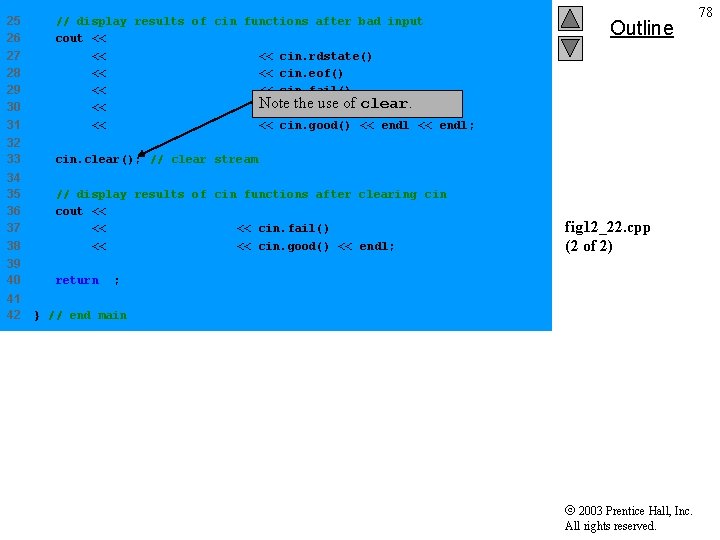
25 26 27 28 29 30 31 // display results of cin functions after bad input cout << "After a bad input operation: " << "ncin. rdstate(): " << cin. rdstate() << "n cin. eof(): " << cin. eof() << "n cin. fail(): " << cin. fail() Note the use of clear. << "n cin. bad(): " << cin. bad() << "n cin. good(): " << cin. good() << endl; 32 33 cin. clear(); // clear stream 34 35 36 37 38 // display results of cin functions after clearing cin cout << "After cin. clear()" << "ncin. fail(): " << cin. fail() << "ncin. good(): " << cin. good() << endl; 39 40 return 0; 41 42 Outline fig 12_22. cpp (2 of 2) } // end main 2003 Prentice Hall, Inc. All rights reserved. 78
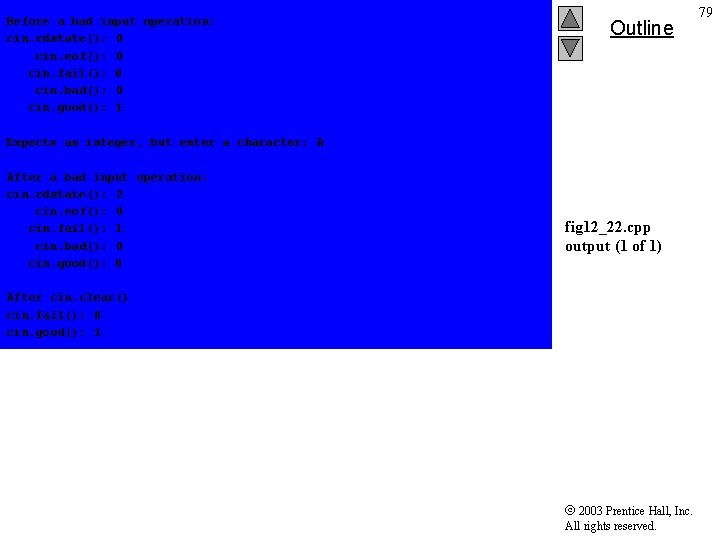
Before a bad input operation: cin. rdstate(): 0 cin. eof(): 0 cin. fail(): 0 cin. bad(): 0 cin. good(): 1 Outline Expects an integer, but enter a character: A After a bad input operation: cin. rdstate(): 2 cin. eof(): 0 cin. fail(): 1 cin. bad(): 0 cin. good(): 0 fig 12_22. cpp output (1 of 1) After cin. clear() cin. fail(): 0 cin. good(): 1 2003 Prentice Hall, Inc. All rights reserved. 79
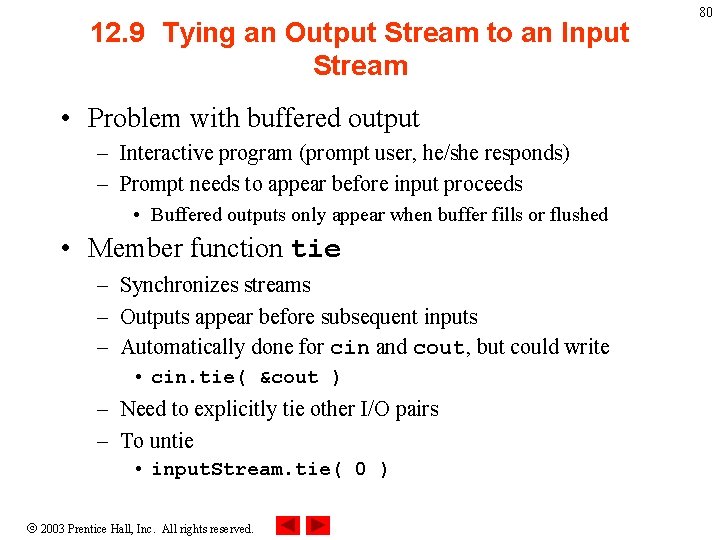
12. 9 Tying an Output Stream to an Input Stream • Problem with buffered output – Interactive program (prompt user, he/she responds) – Prompt needs to appear before input proceeds • Buffered outputs only appear when buffer fills or flushed • Member function tie – Synchronizes streams – Outputs appear before subsequent inputs – Automatically done for cin and cout, but could write • cin. tie( &cout ) – Need to explicitly tie other I/O pairs – To untie • input. Stream. tie( 0 ) 2003 Prentice Hall, Inc. All rights reserved. 80
Inputoutput devices
Differentiate byte stream and character stream
Topic sentence sandwich
Chapter 11 section 2 stream and river deposits answer key
What's labeling theory
Romans outline by chapter
Write an introduction
Give me liberty ch 27
Methodology chapter outline
Chapter 38 a world without borders outline
Vbscript
Hunger games chapter 27 questions and answers
Chapter 31 societies at crossroads outline
Ap world history chapter 28 outline
Chapter 2 learning goals outline sociology answers
Chapter 1 outline
Chapter 1 outline
Chapter 30
Chapter 2 outline
Sermon outlines on ananias and sapphira
Government spending multiplier
24 chapter outline
Apush chapter 16 conquering a continent outline
一生愛你
Strahler stream order
Trunk stream example
What is the stream of consciousness technique
Value stream mapping karen martin
Idm healthcare
Stream classes in c++
The gift of knowing you lyrics
Base level river
Molly ivors
Stream is a sequence of
Stream adalah
Stream continuum concept
Block cipher vs stream cipher example
Where can i stream blade
Aspen recycle stream not converging
Labrador ve gulf stream akıntıları
Recycle bypass and purge definition
Hair cowlick definition
Stream of praise praise the lord, o my soul lyrics
Counting distinct elements in a stream in big data
Bloom filter for stream data mining
Toyota value stream mapping
Value stream mapping healthcare examples
Pond ecosystem food chain examples
Stream is a sequence of
Planet e stream
Stream of consciousness technique
Stream incision
Fire streams
What is metaphor
Rotary control valve firefighting
Stream load
Stream load
Ultimate base level of a stream
Data stream java
Dbms vs dsms
Data stream characteristics for continuous media
Microsoft stream warwick
Acme stamping value stream mapping
Stream-oriented
Stream anatomy
Setfill
Fire piercing nozzle
The randomaccessfile class treats a file as a stream of
Base level is
Stream order characteristics
Stream order characteristics
Ciri-ciri stream cipher
Stream of consciousness literature
Nord stream 1 vs 2
Flink stateful stream processing
Government statistical service graduate scheme
Current state map
Vnf event stream
Vnf event stream
Who coined stream of consciousness
Virgo stellar stream