1 9 Classes A Deeper Look Part 1
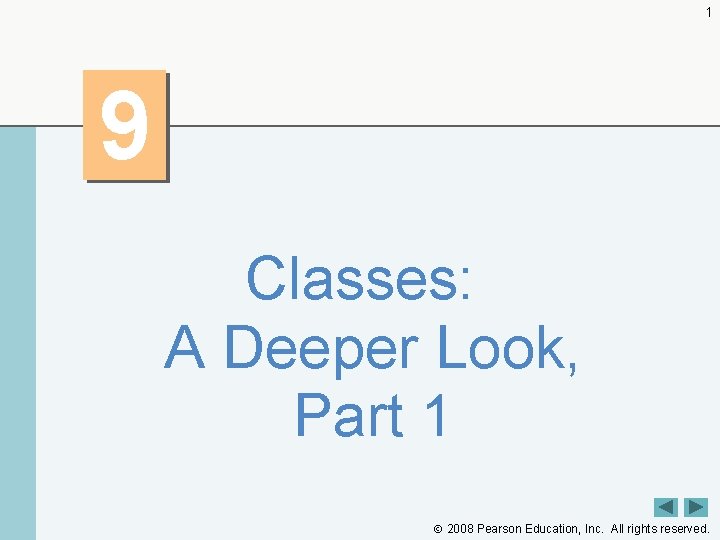
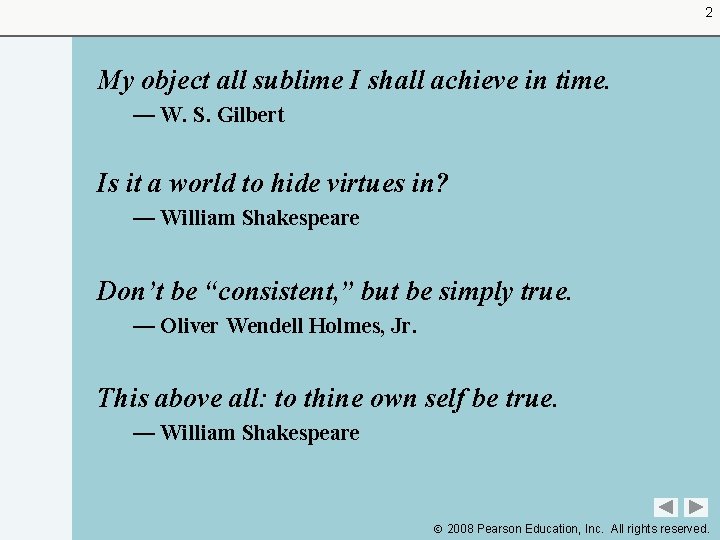
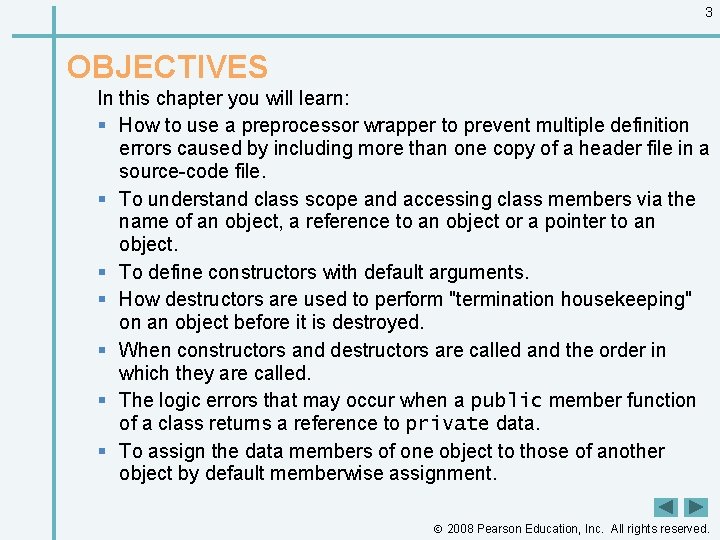
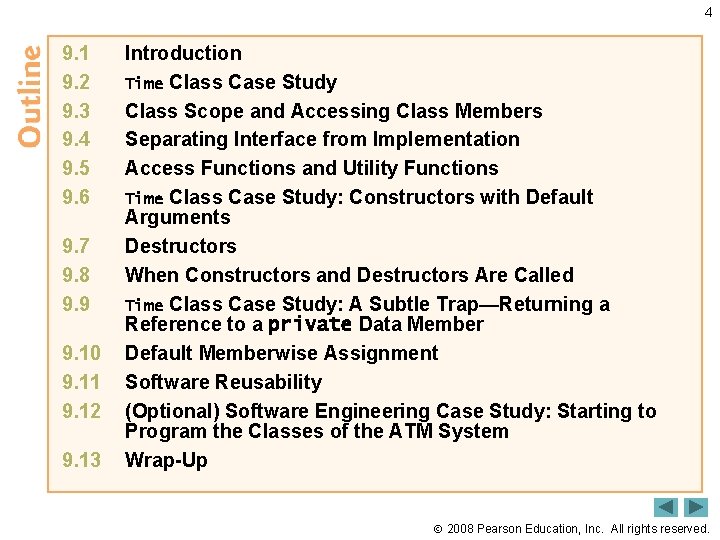
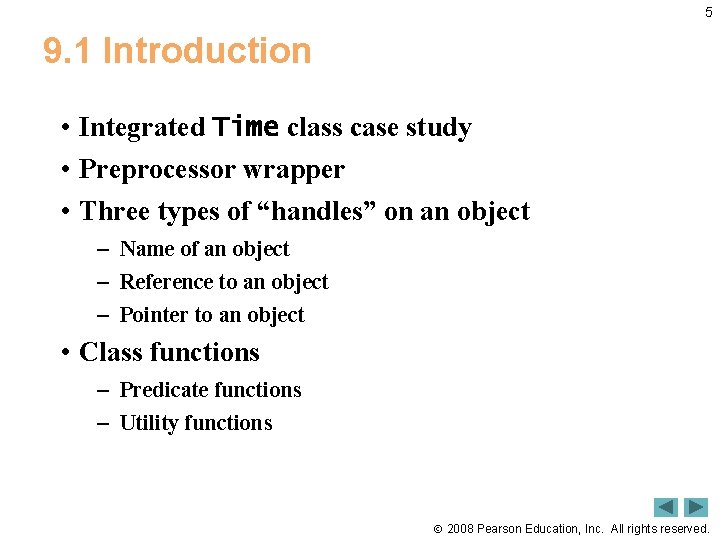
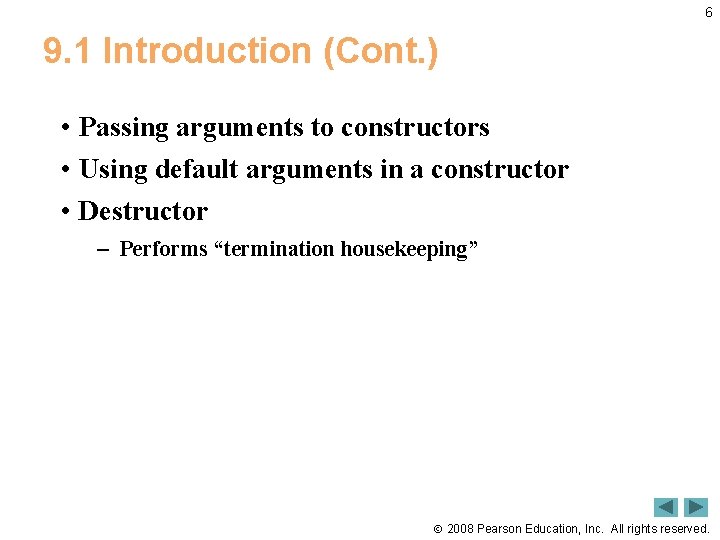
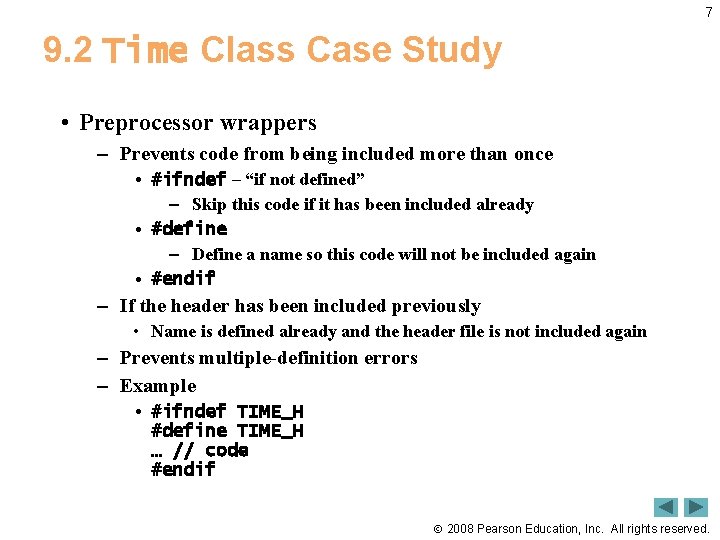
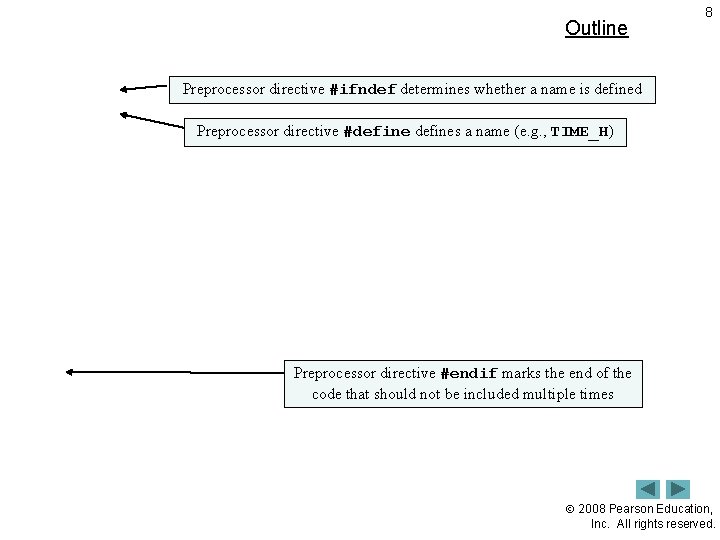
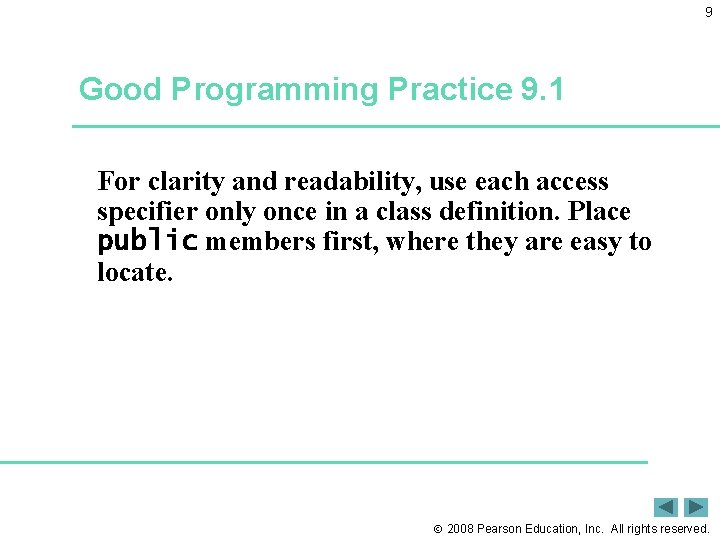
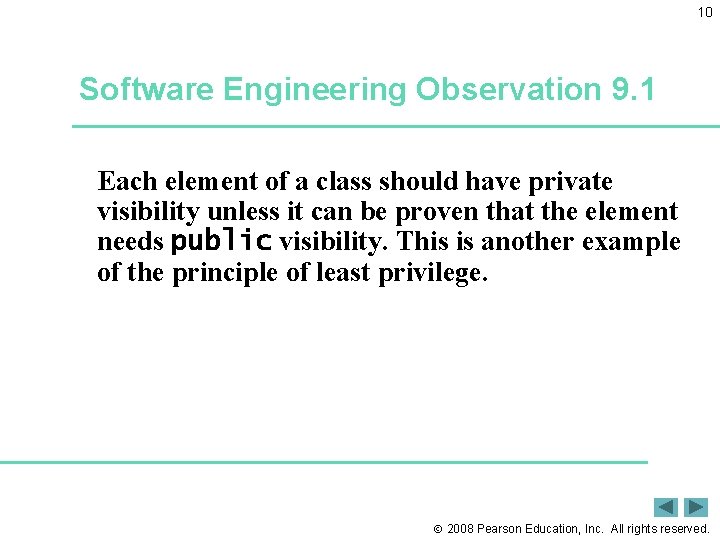
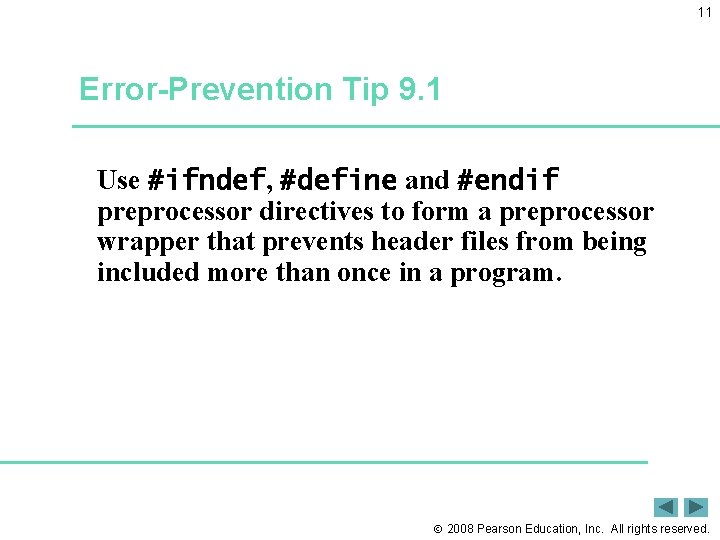
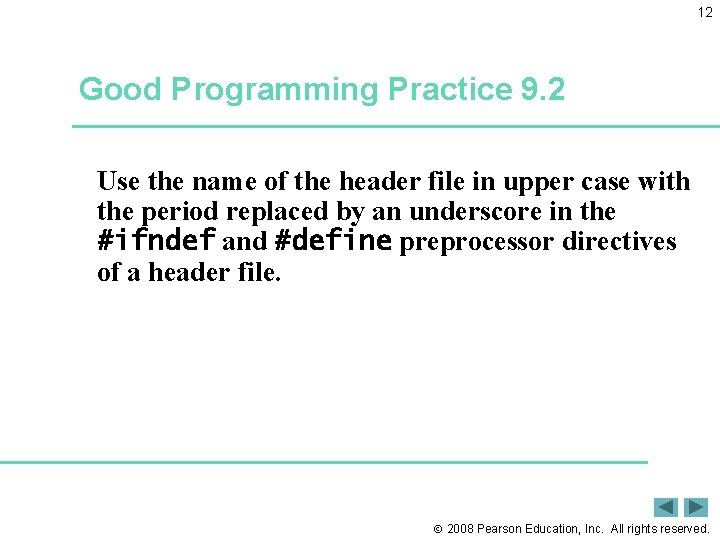
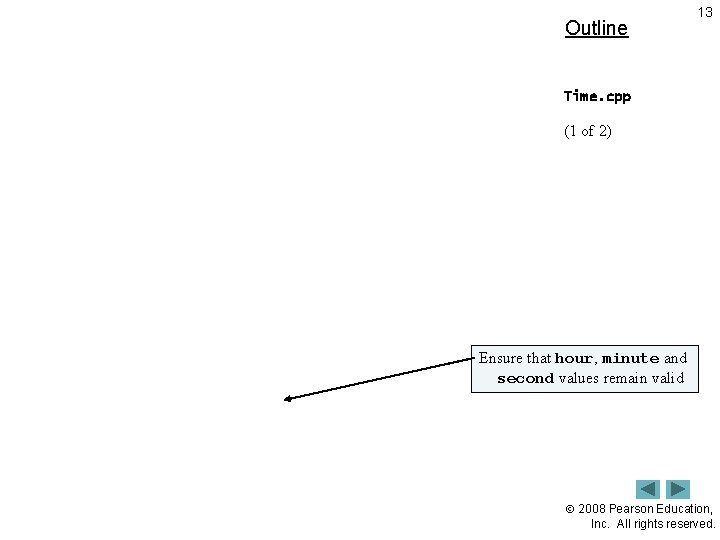
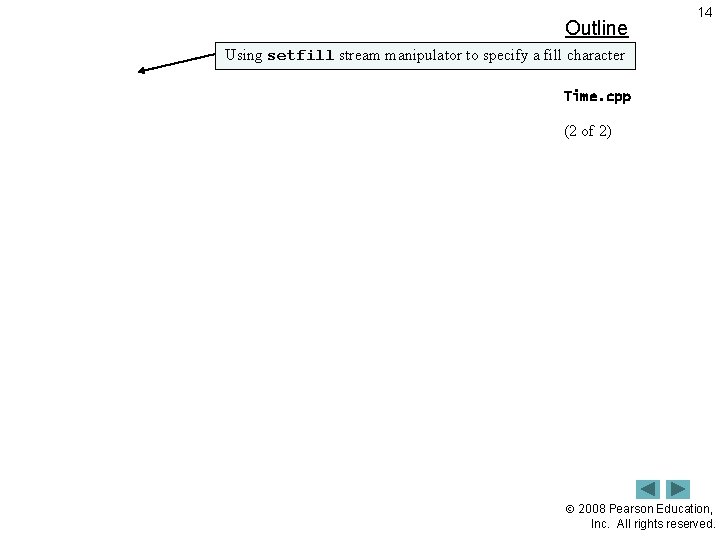
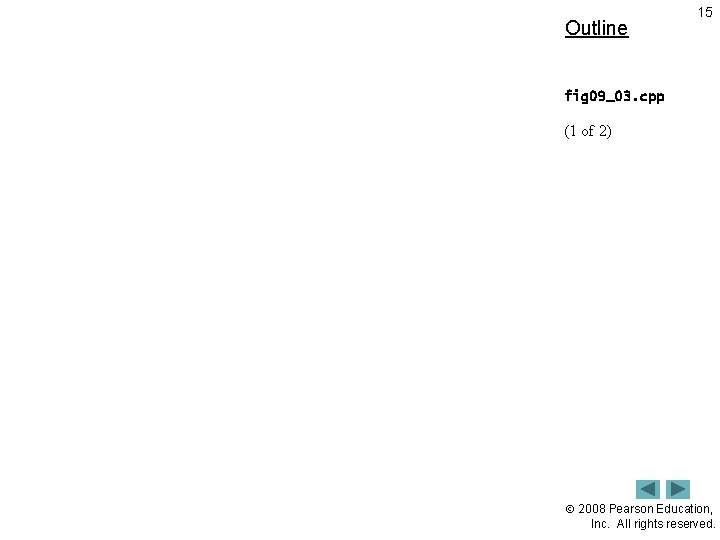
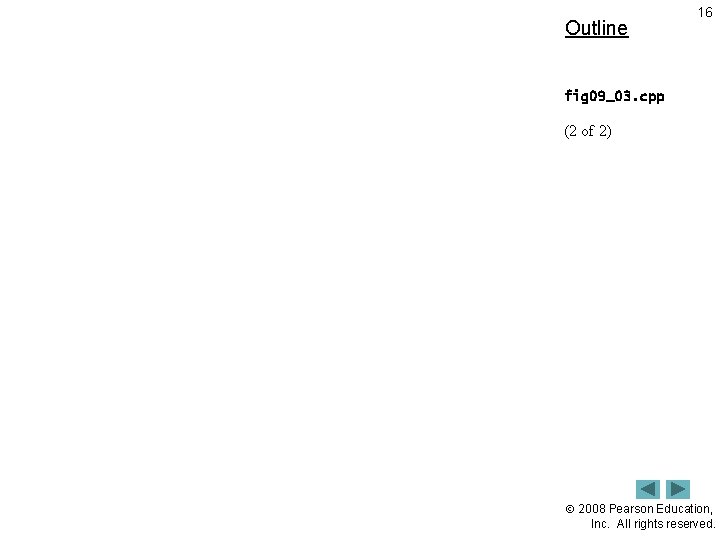
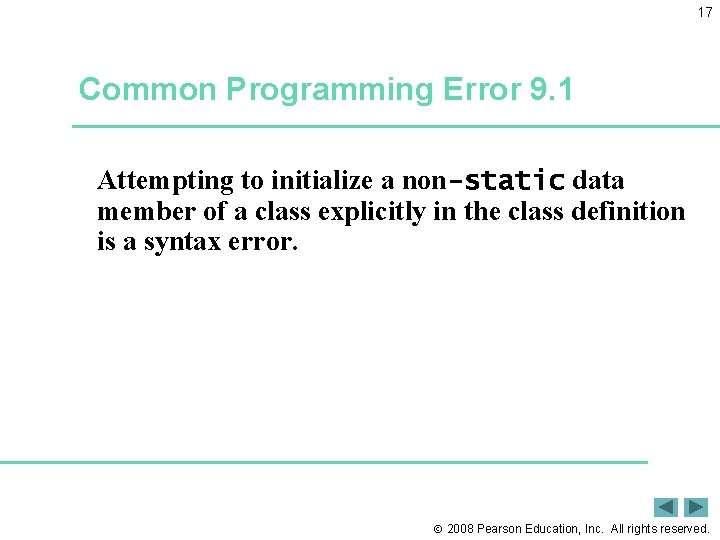
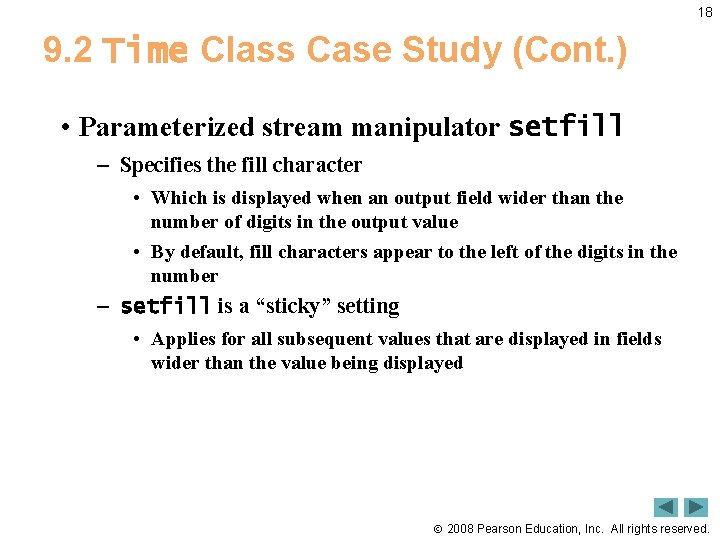
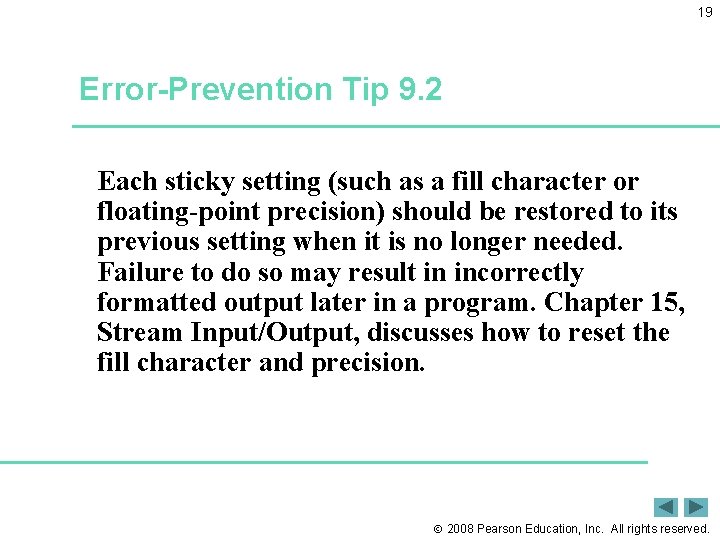
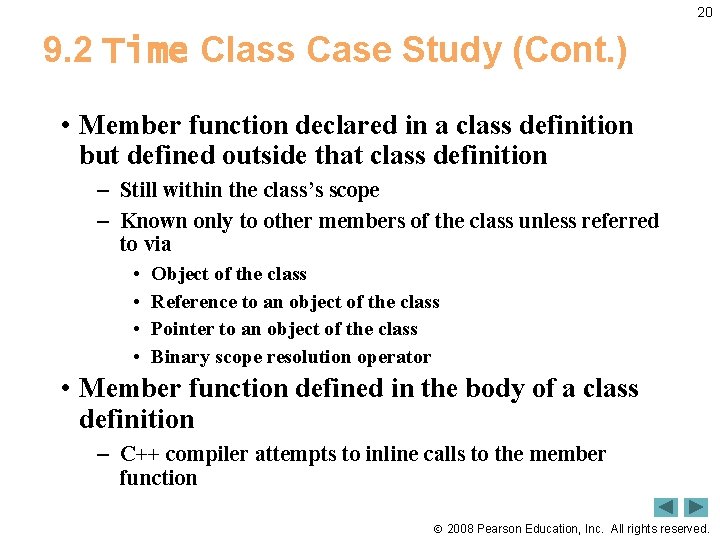
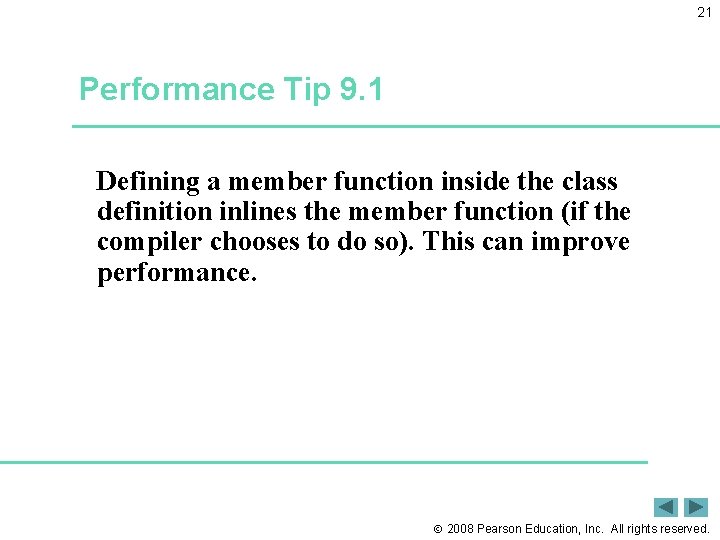
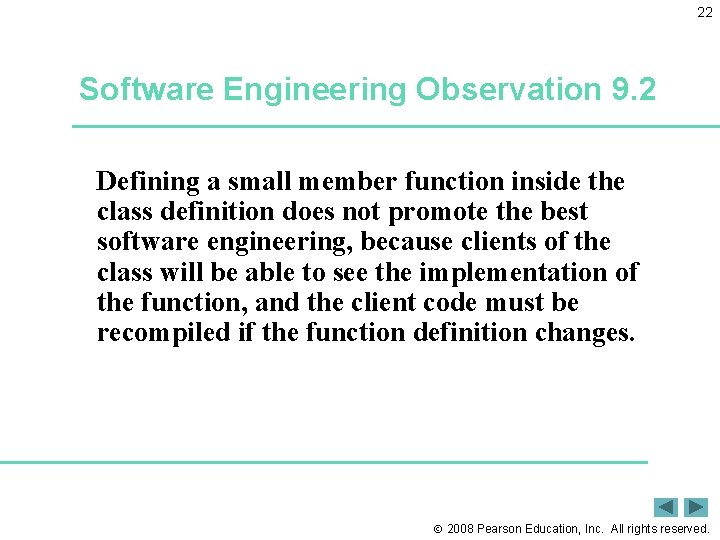
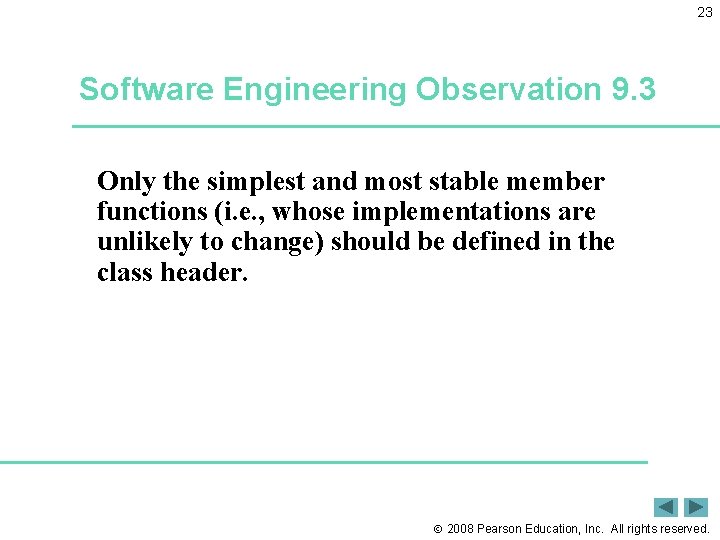
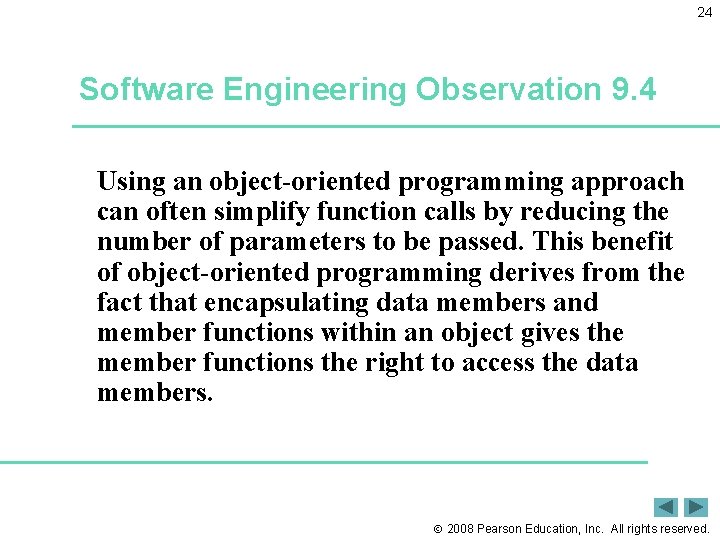
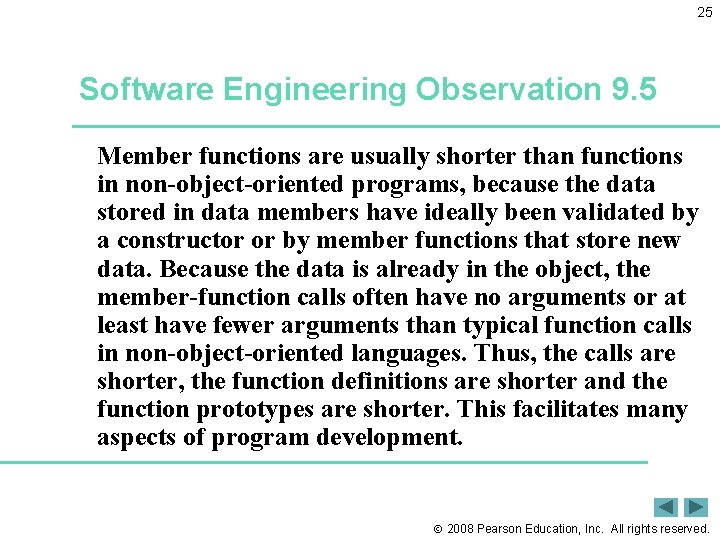
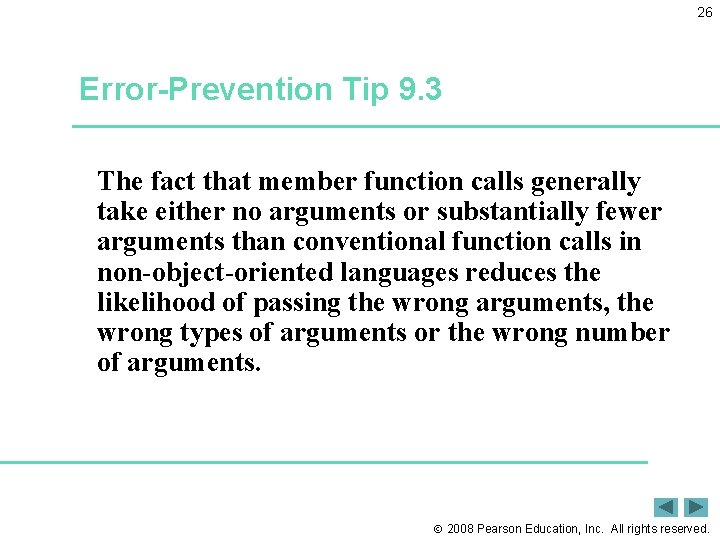
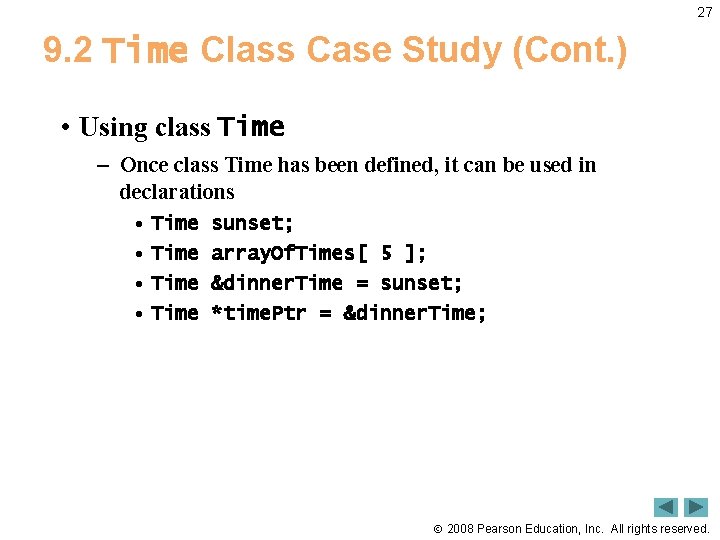
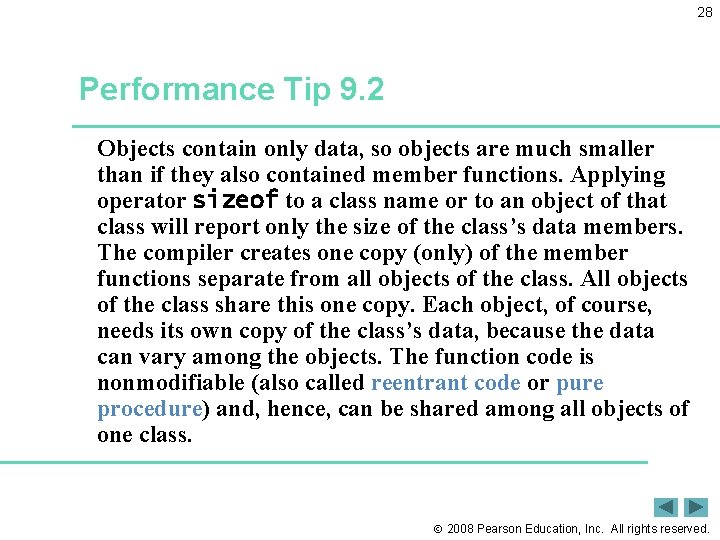
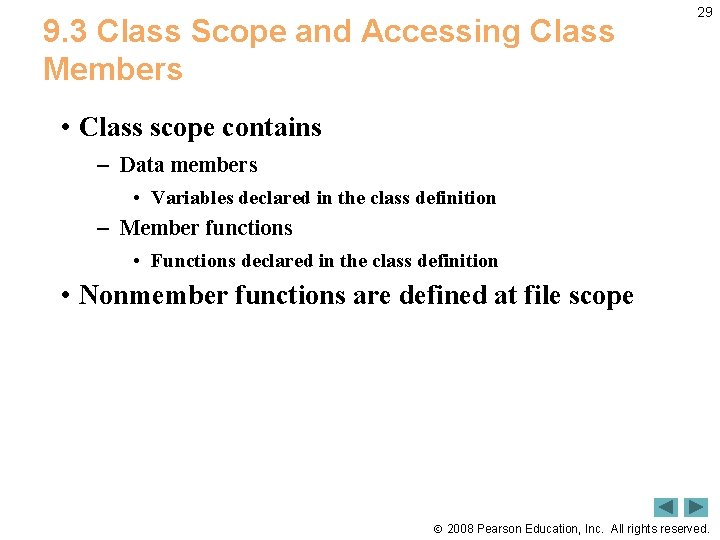
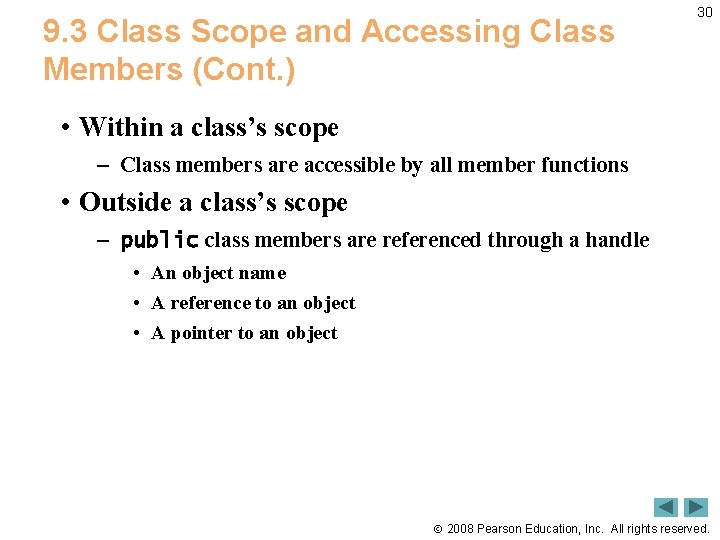
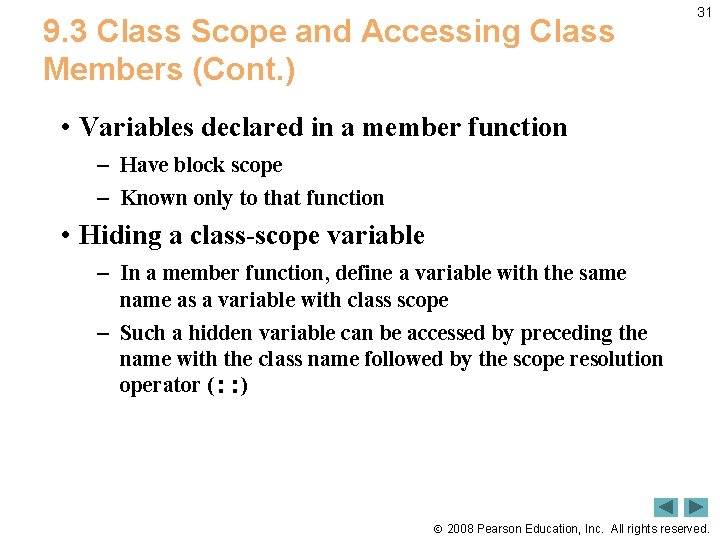
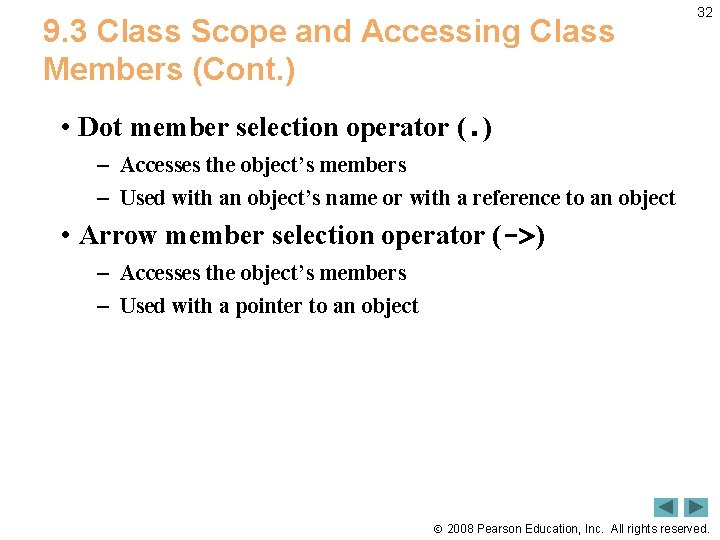
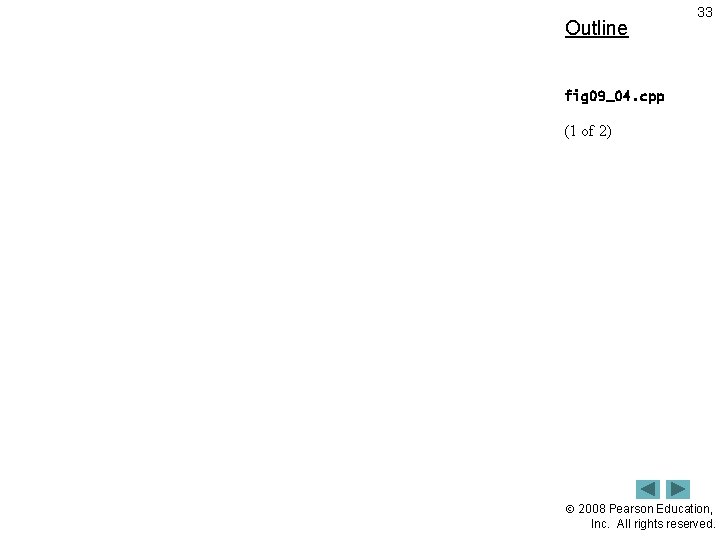
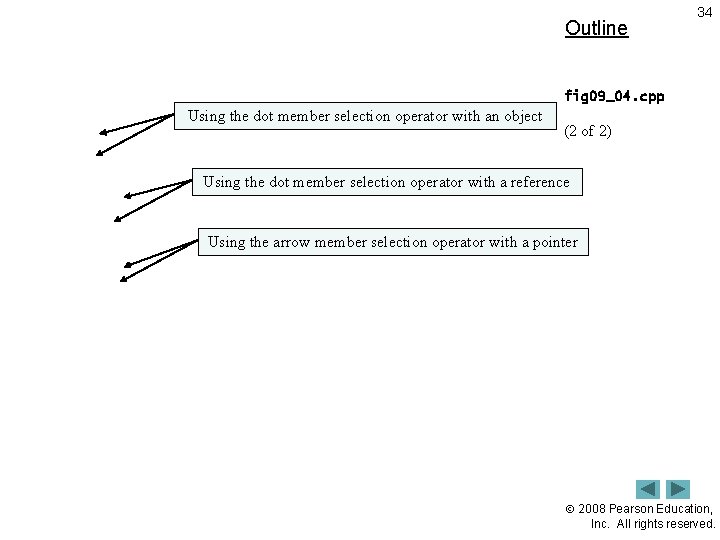
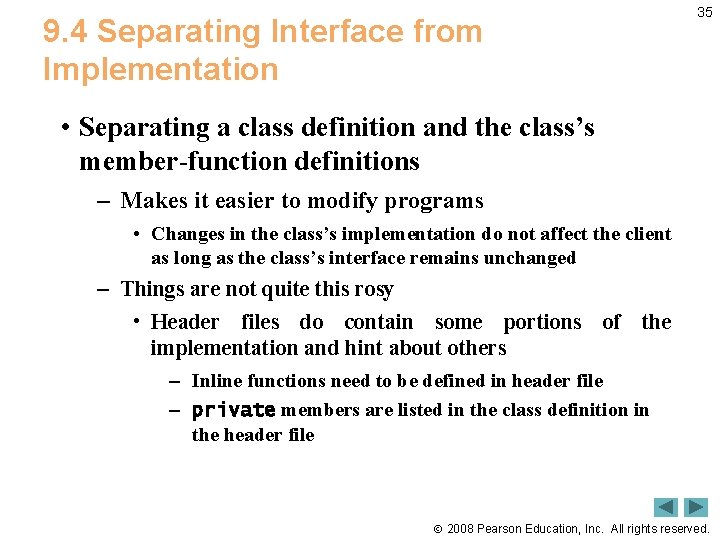
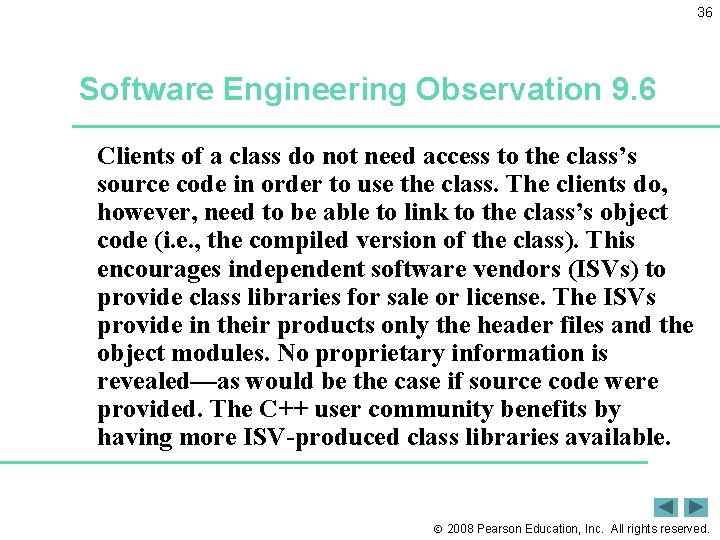
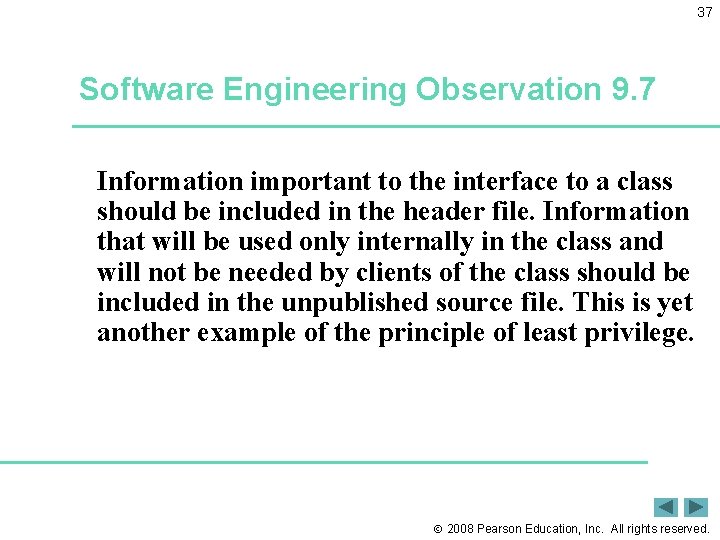
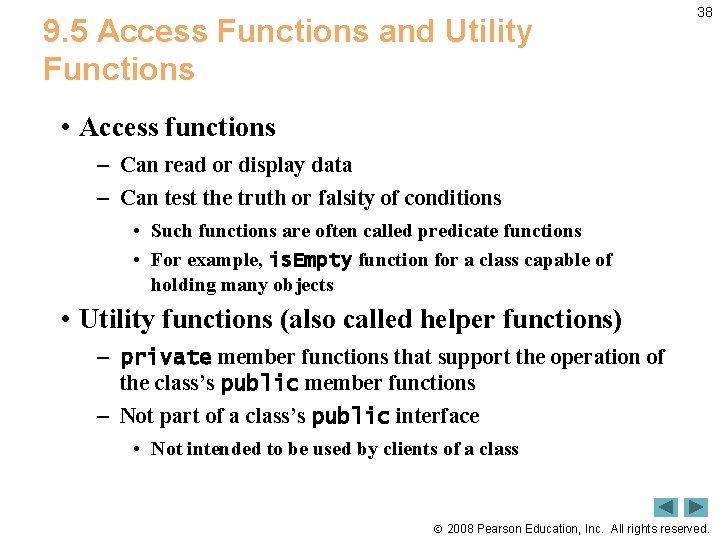
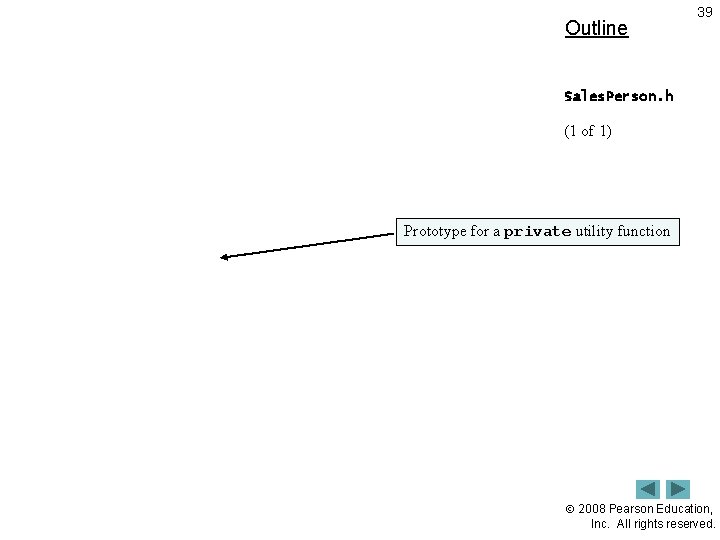
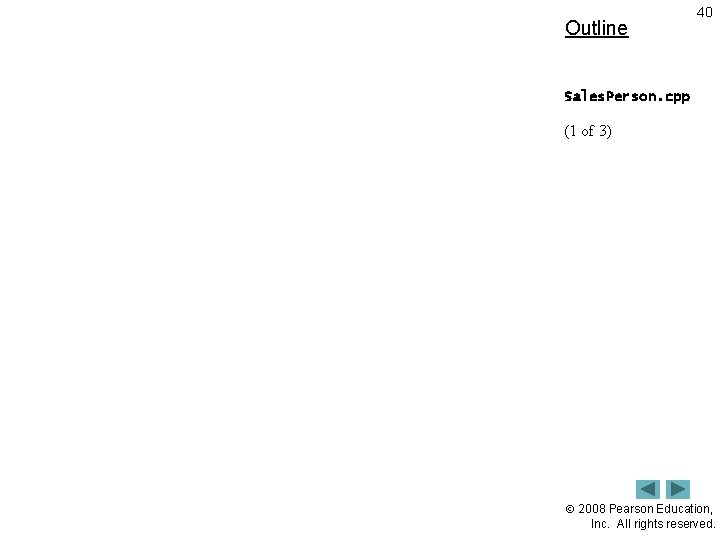
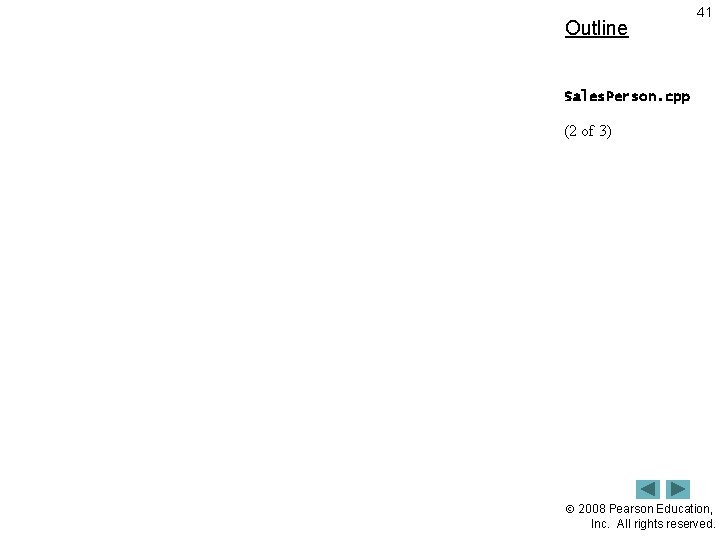
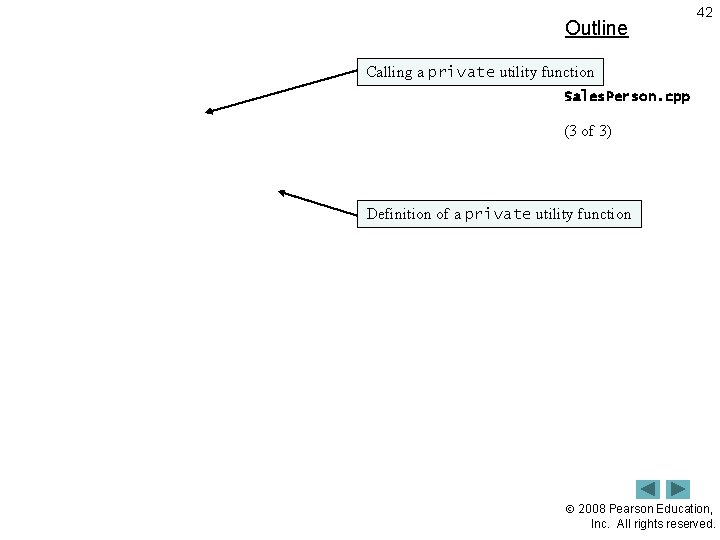
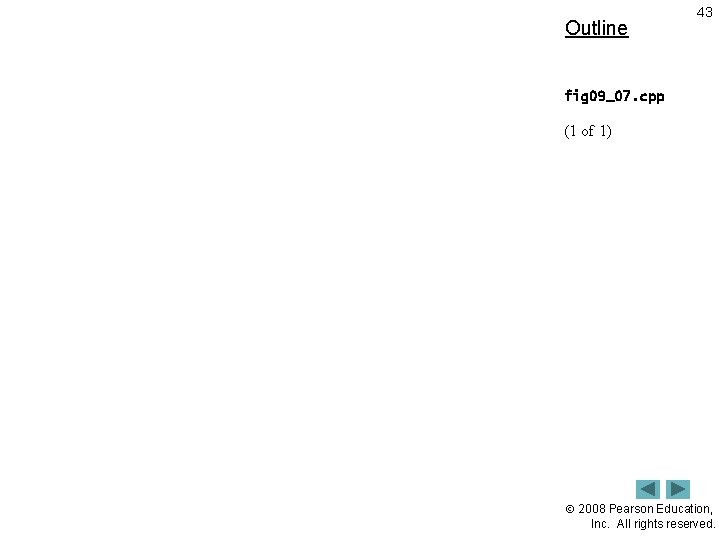
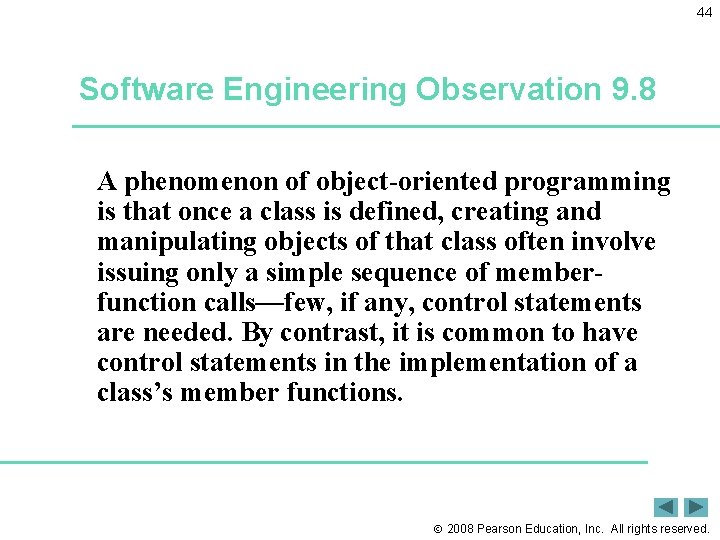
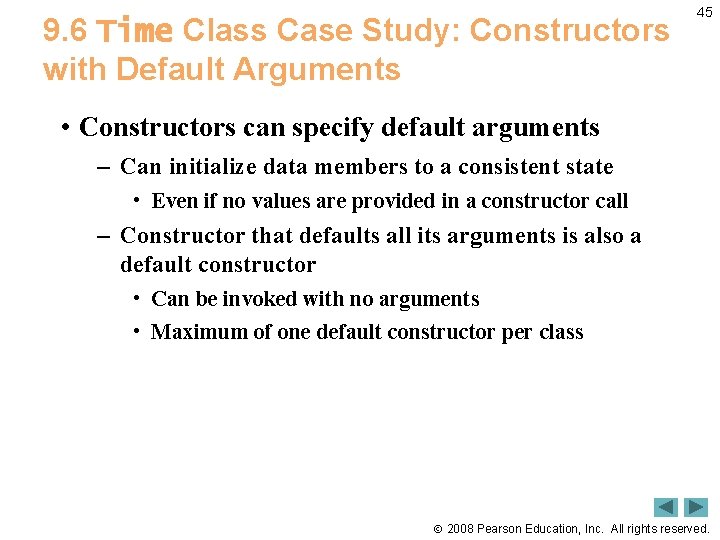
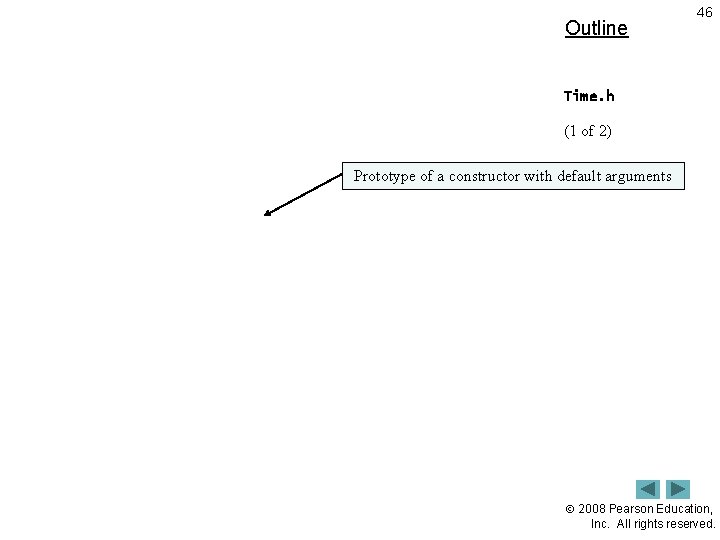
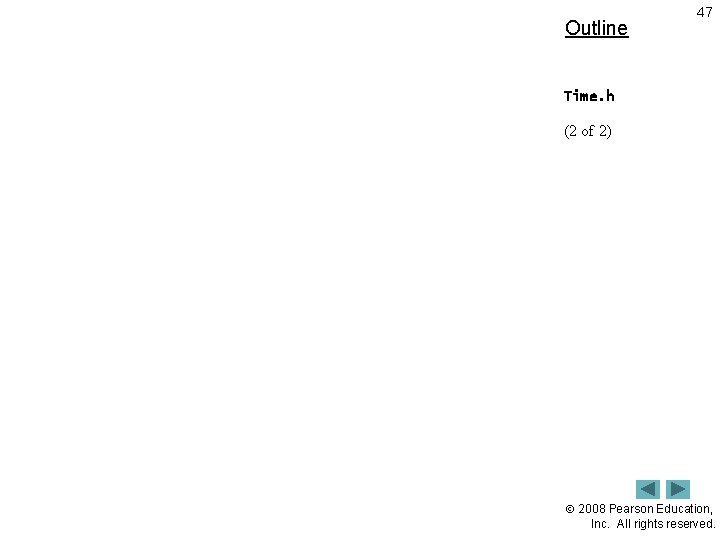
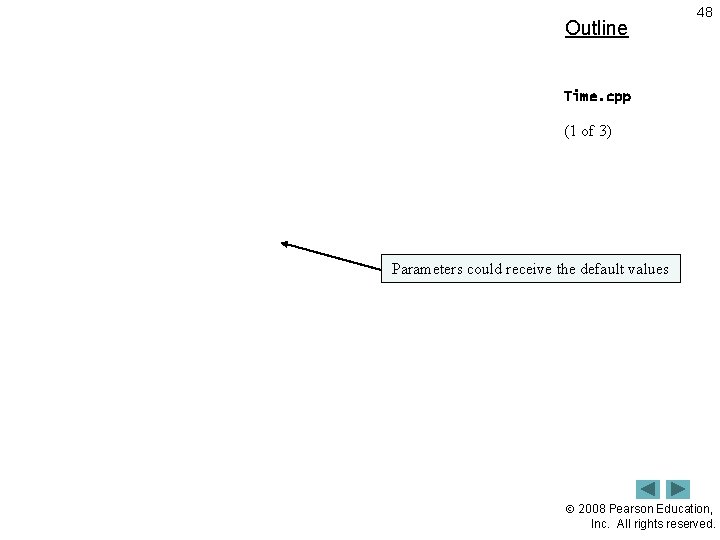
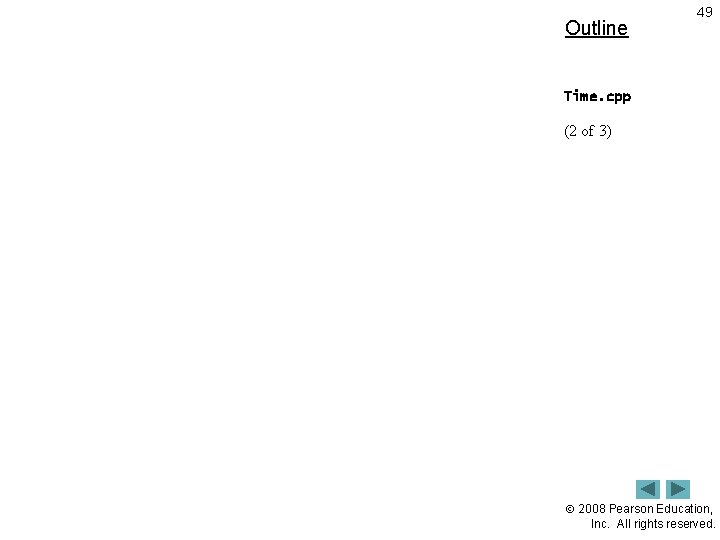
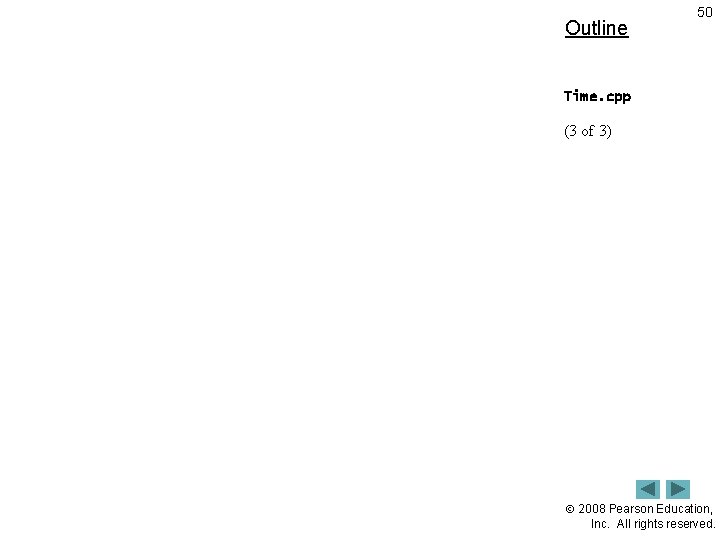
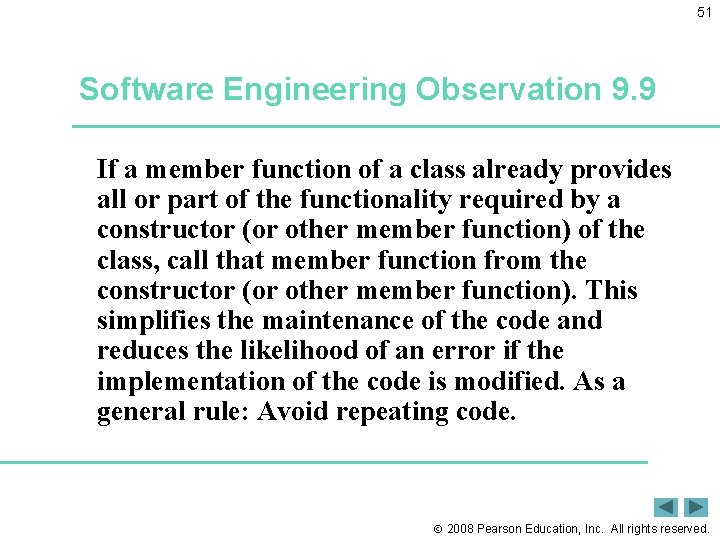
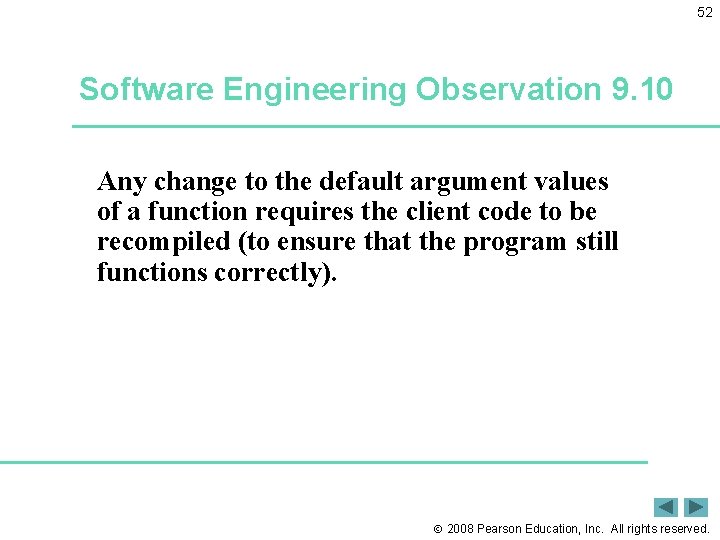
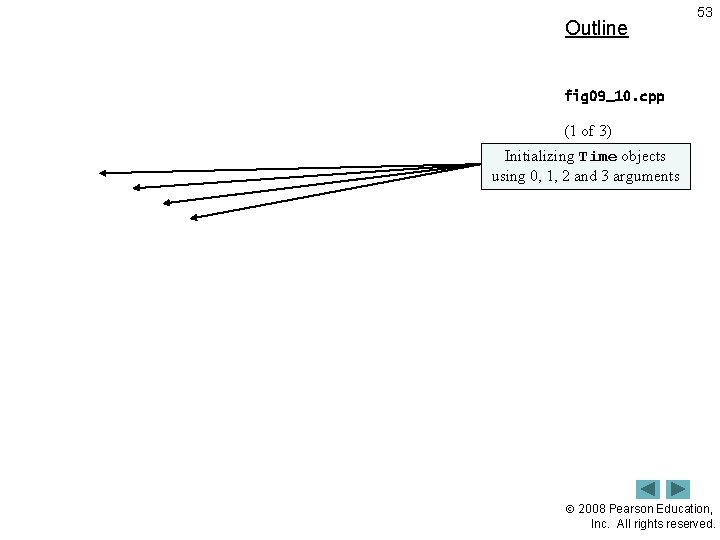
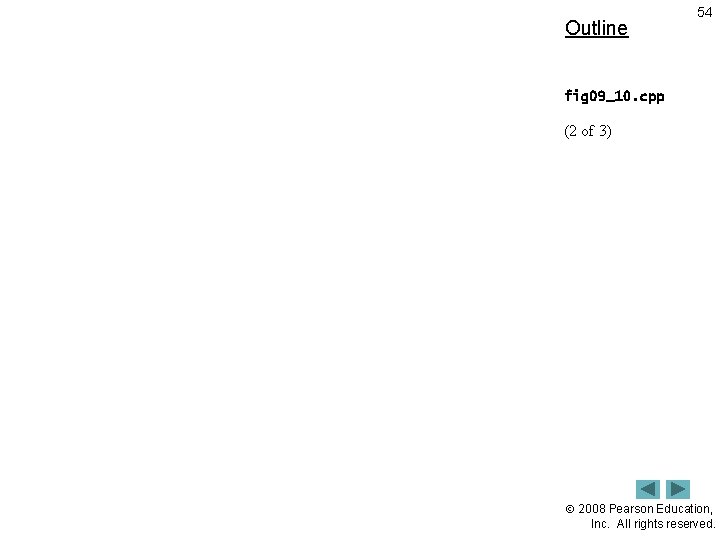
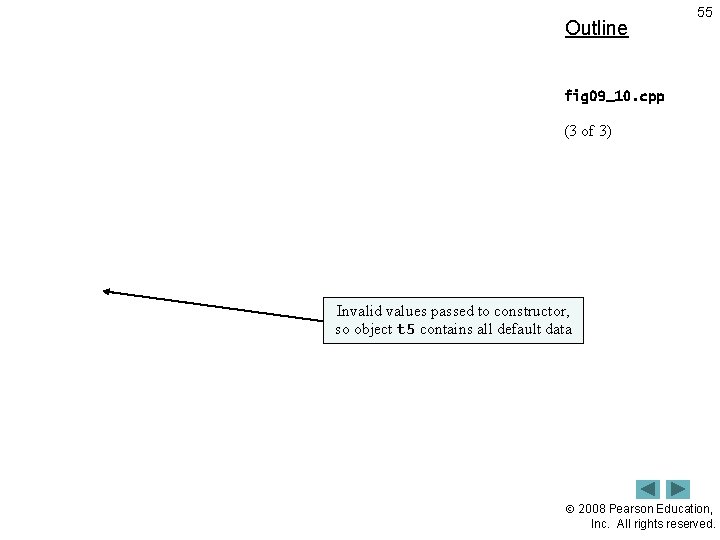
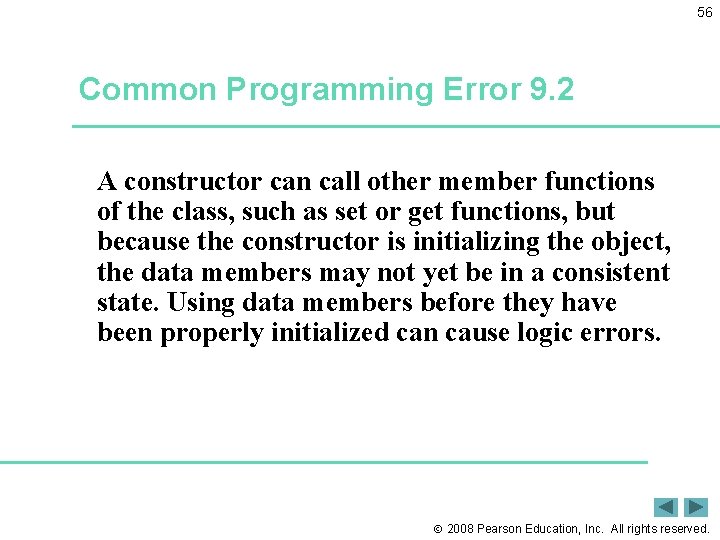
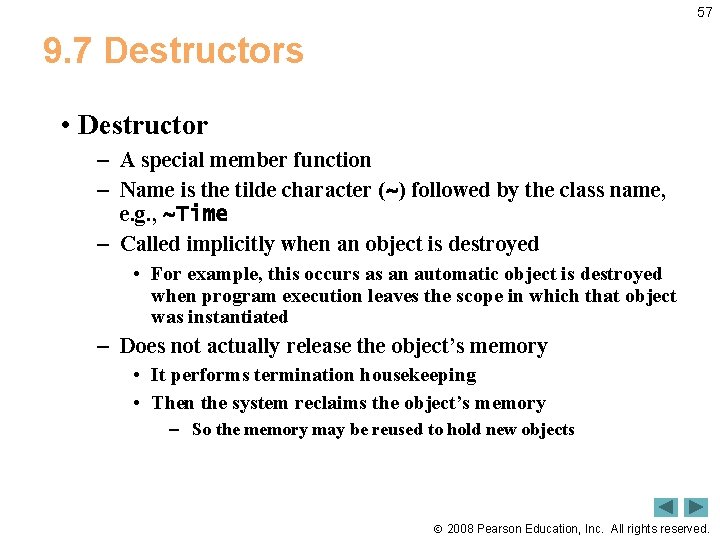
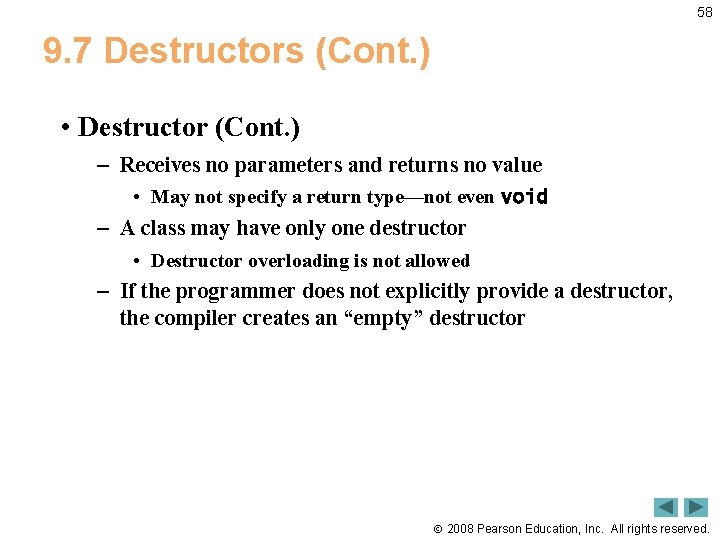
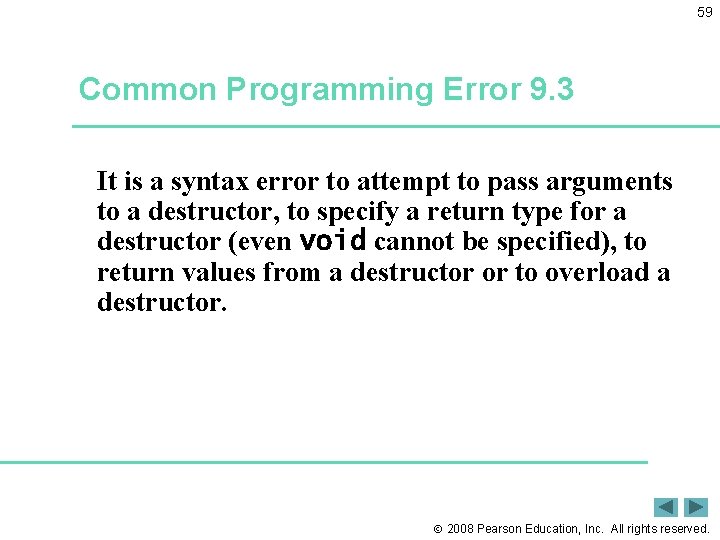
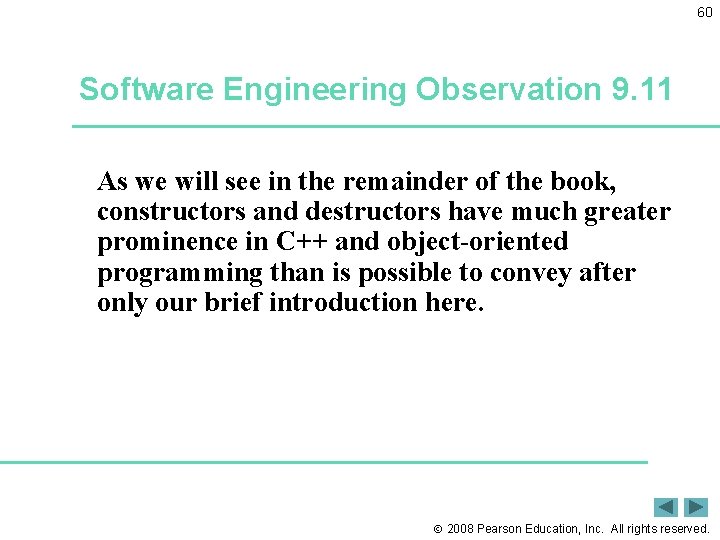
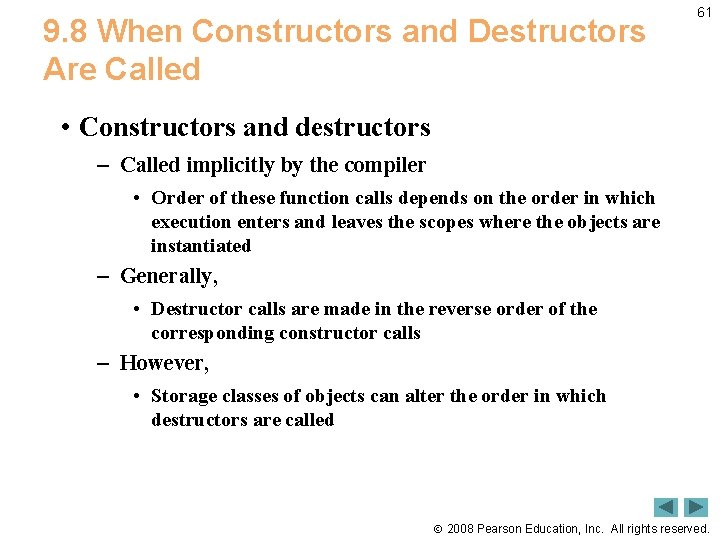
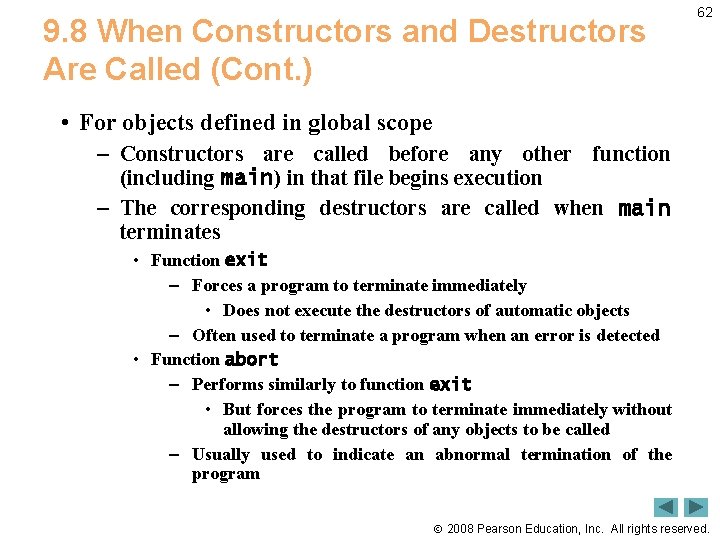
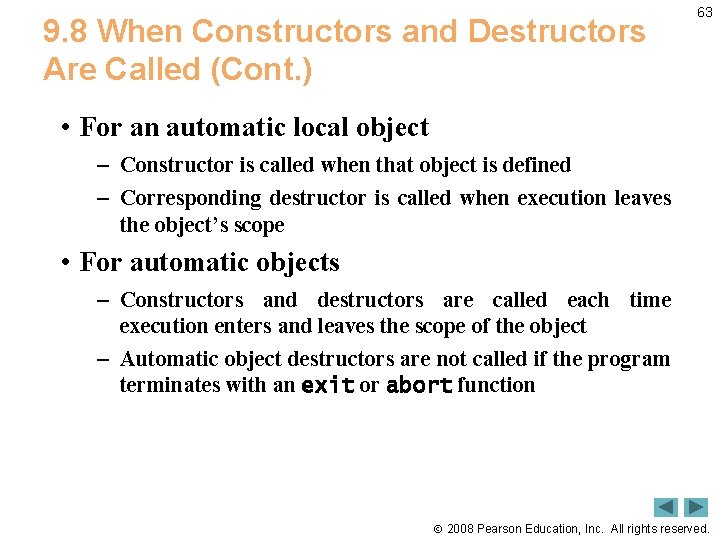
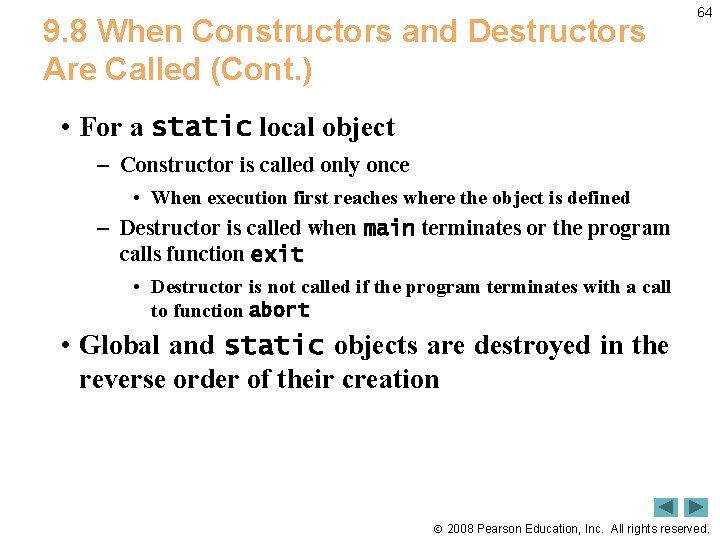
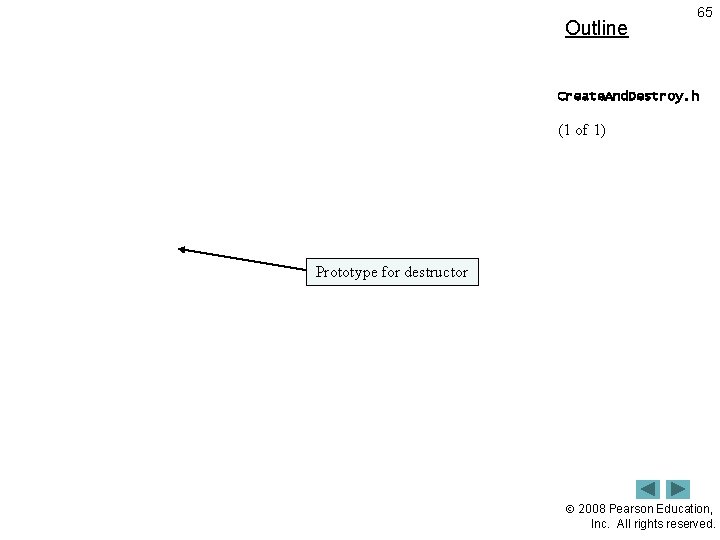
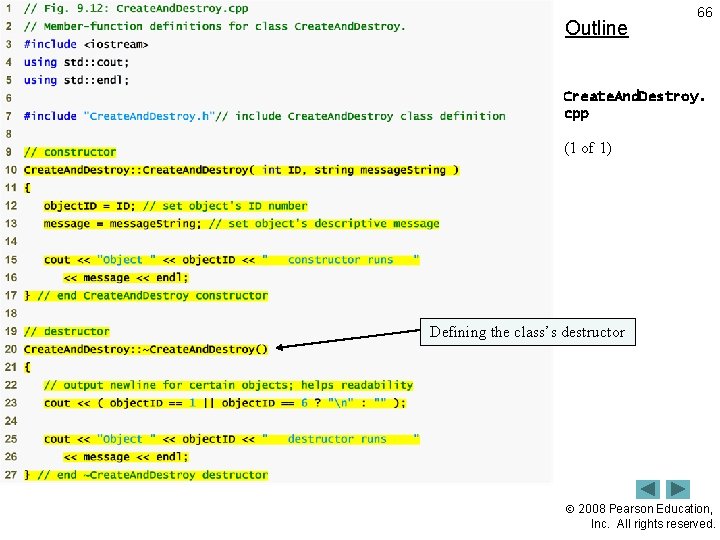
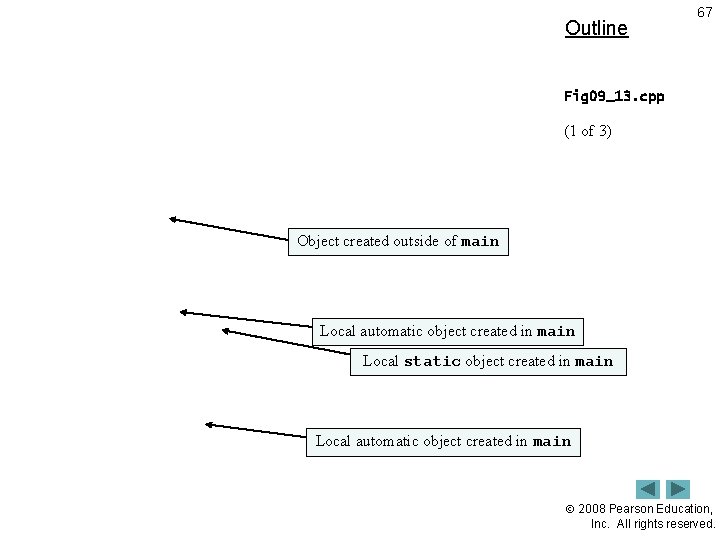
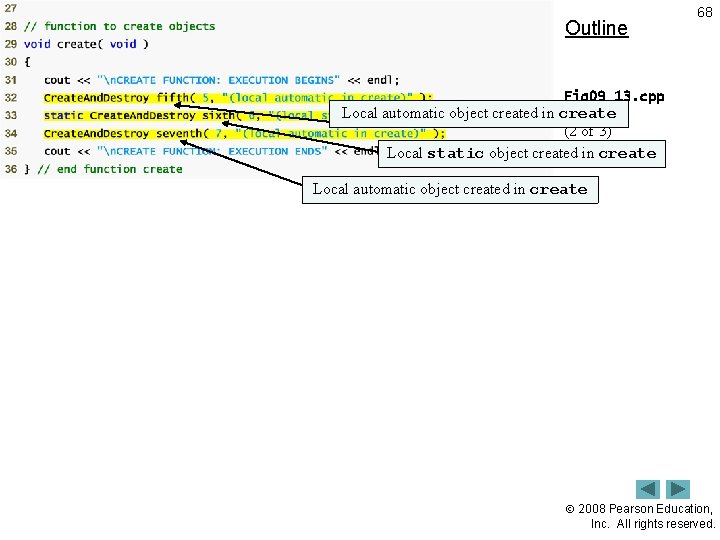
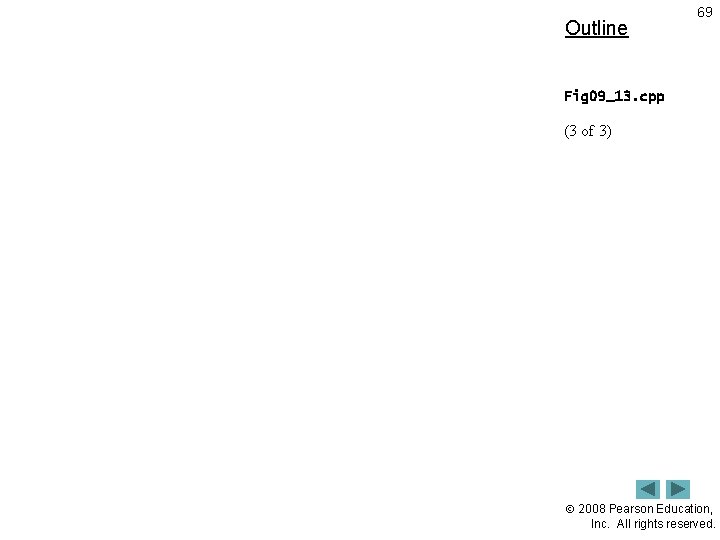
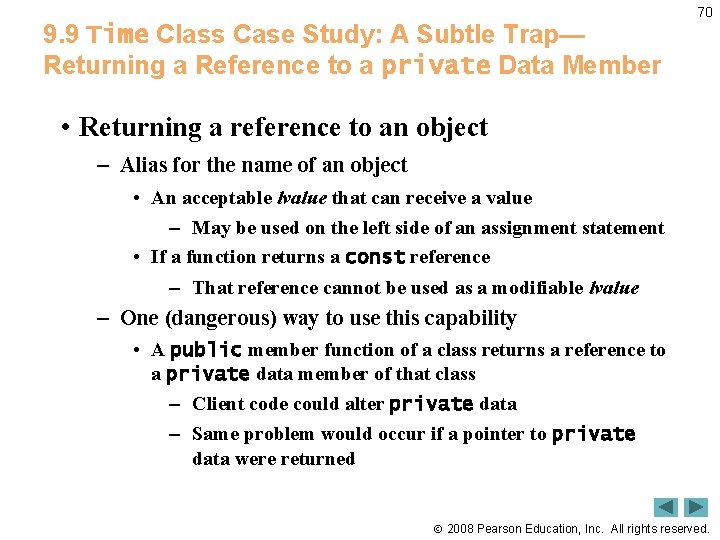
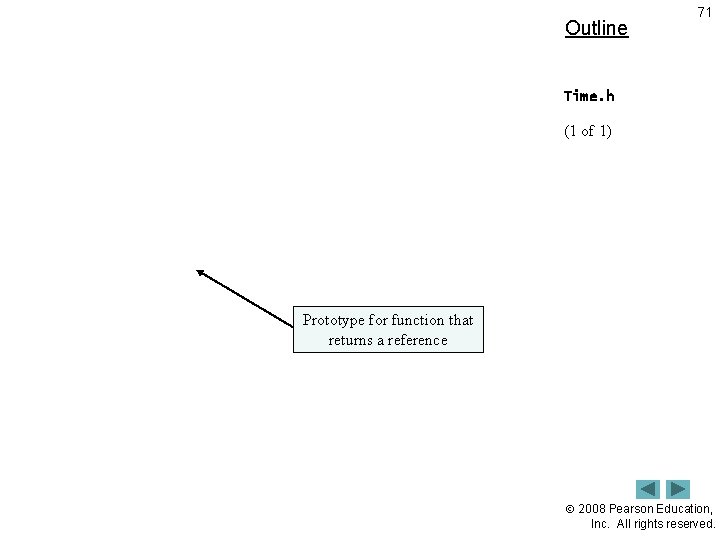
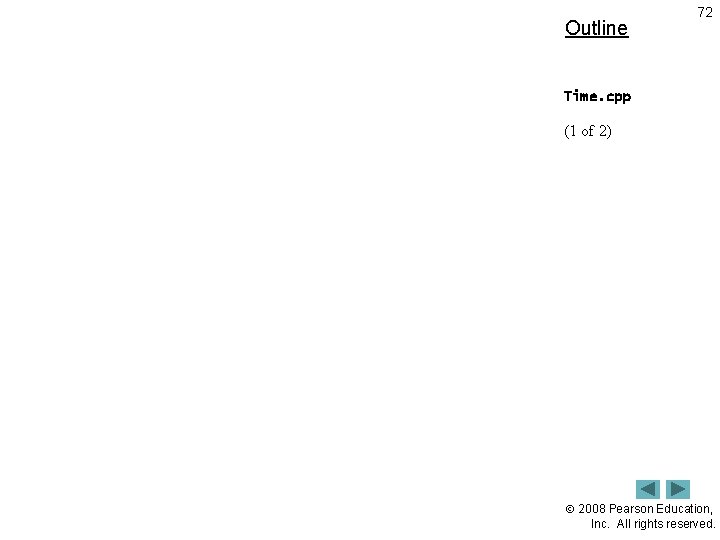
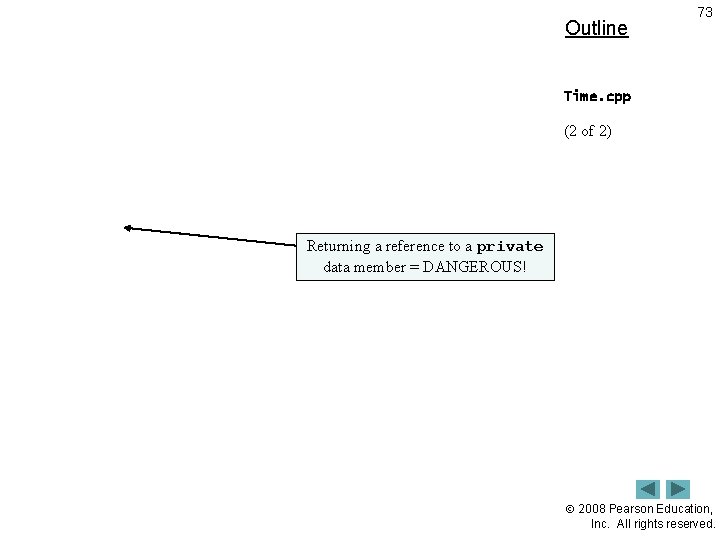
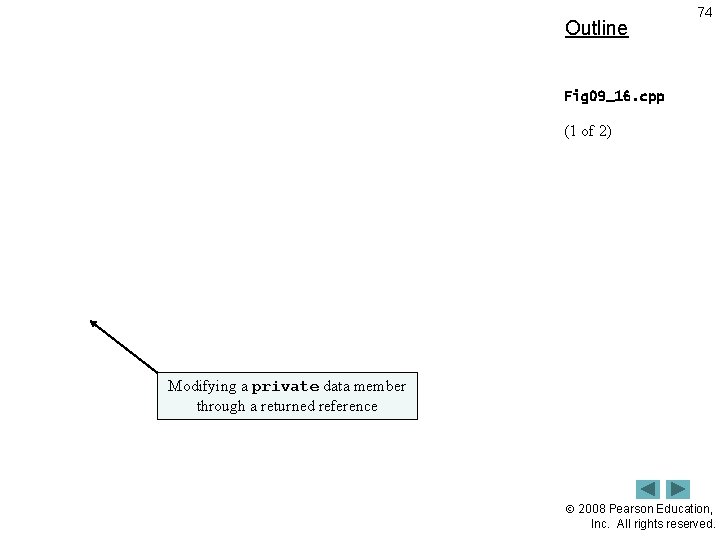
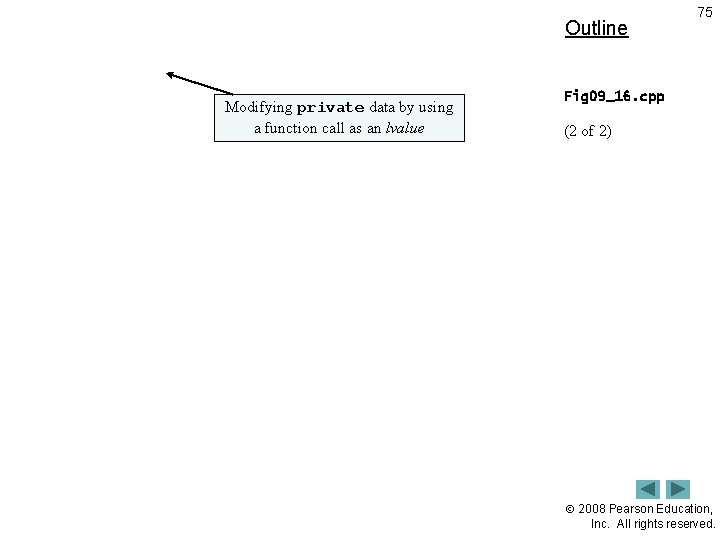
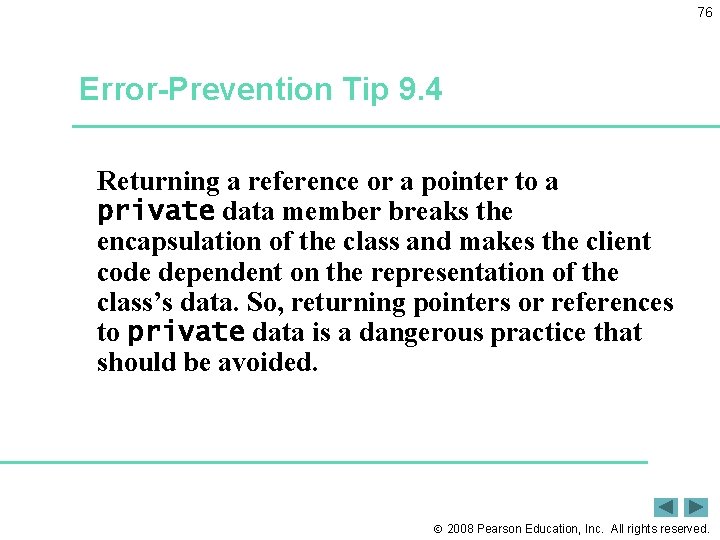
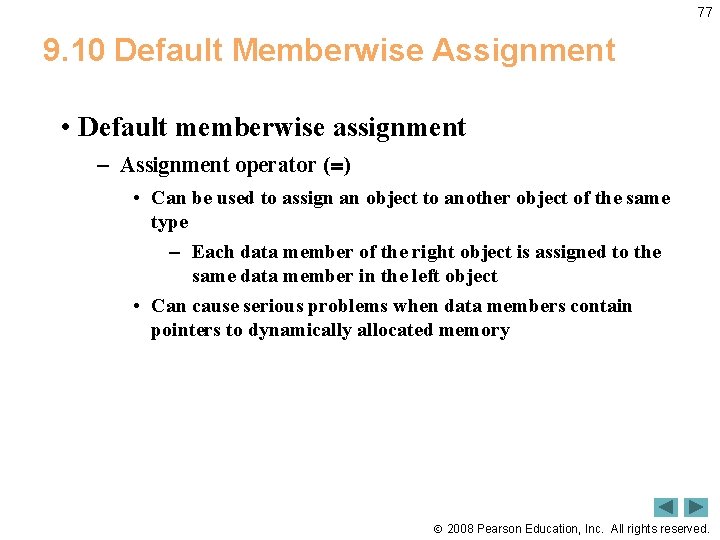
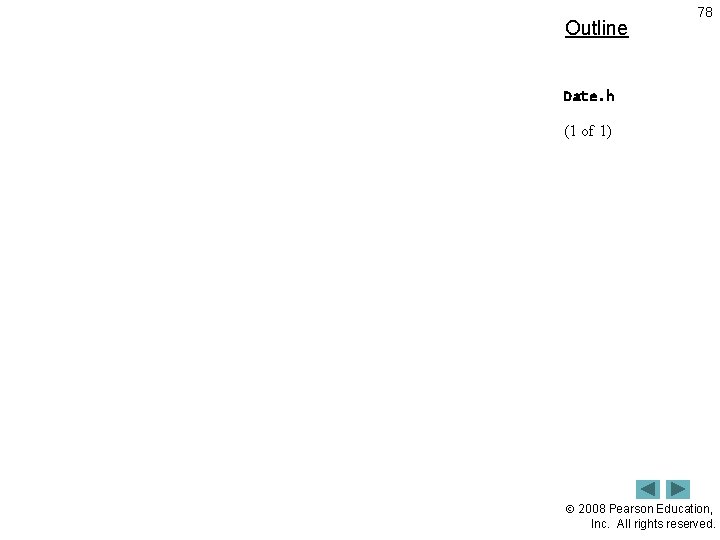
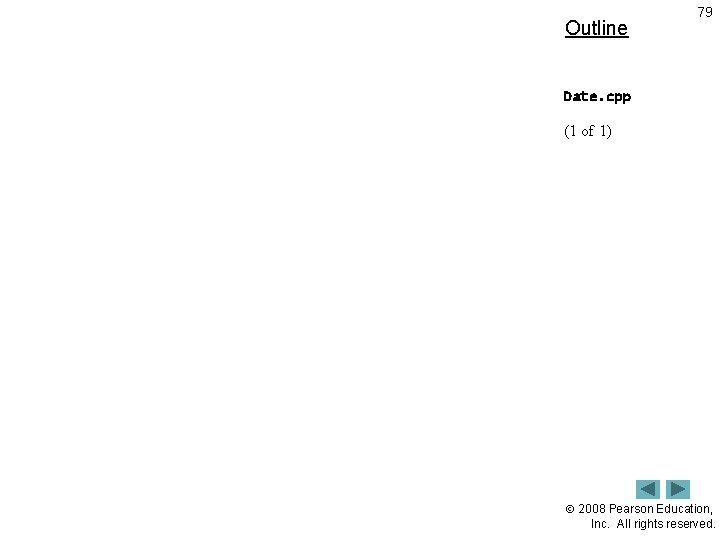
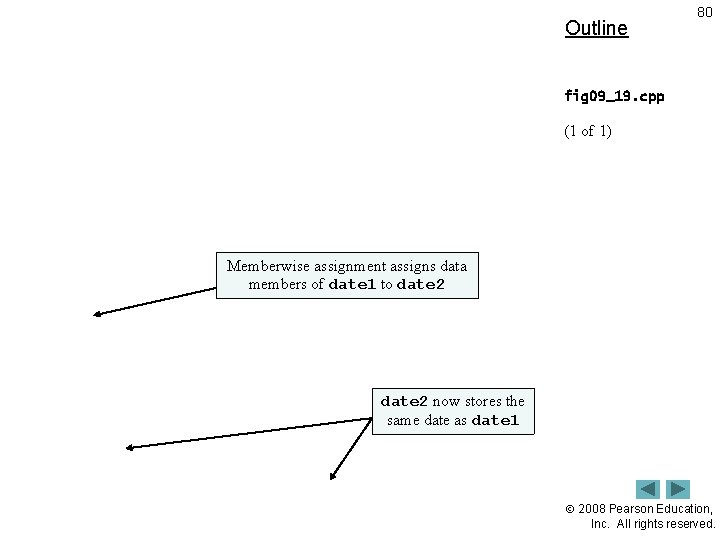
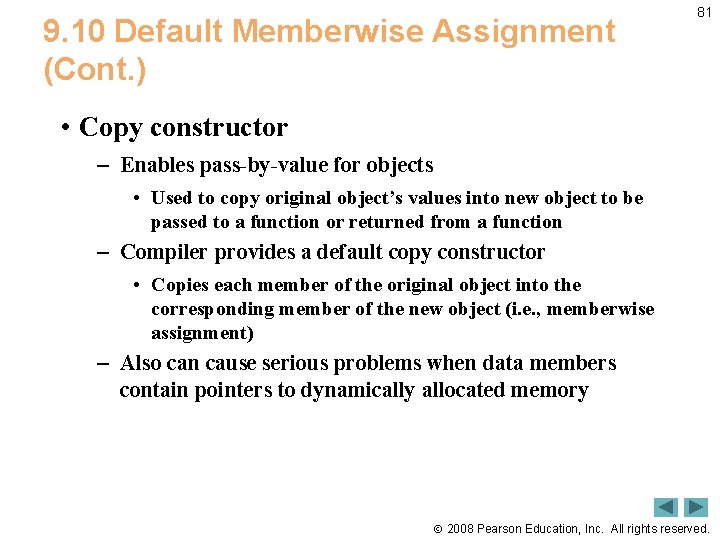
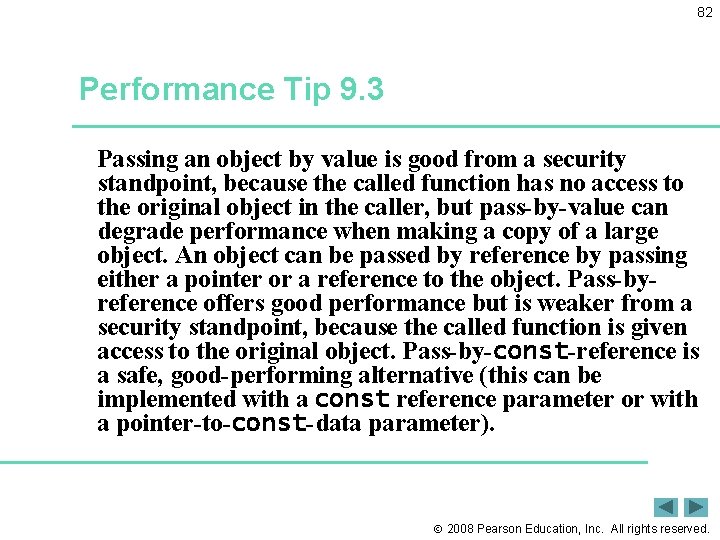
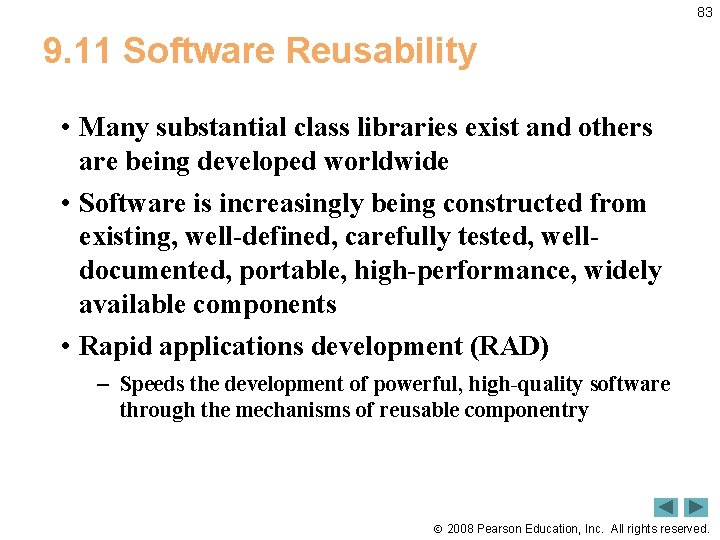
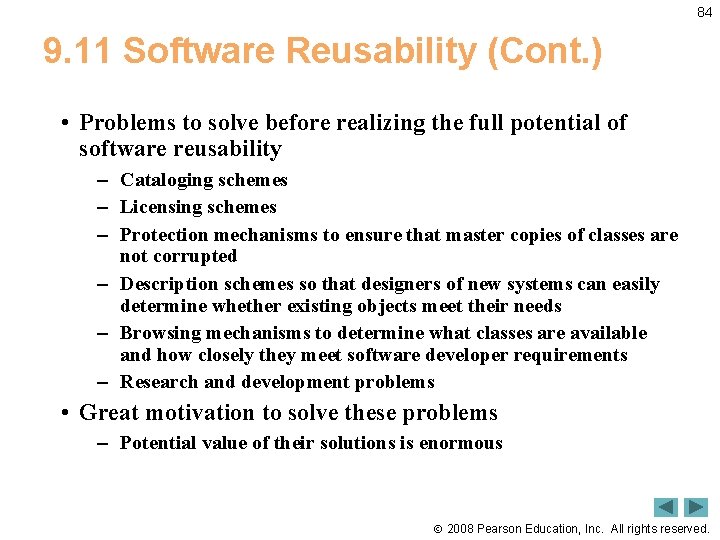
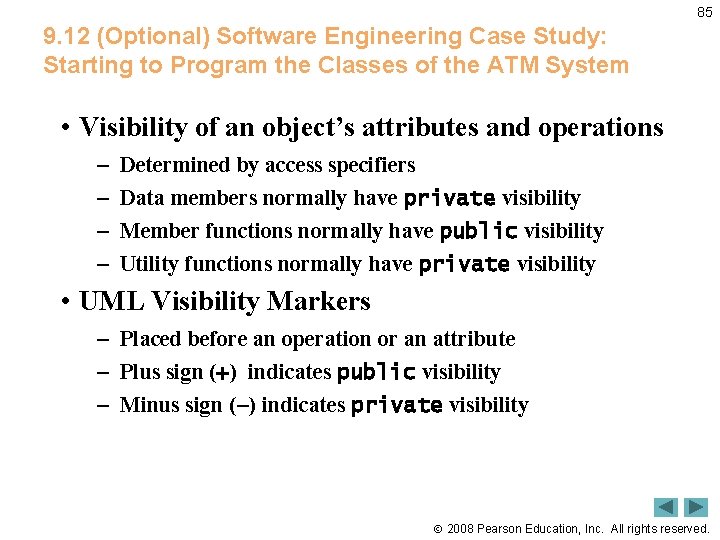
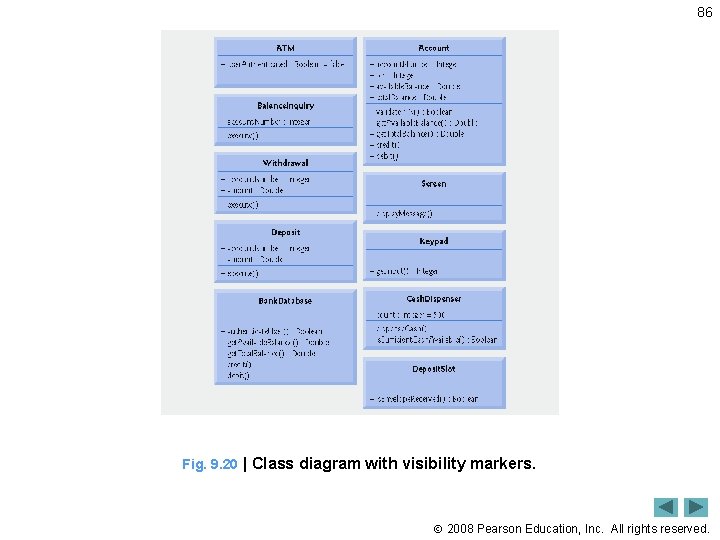
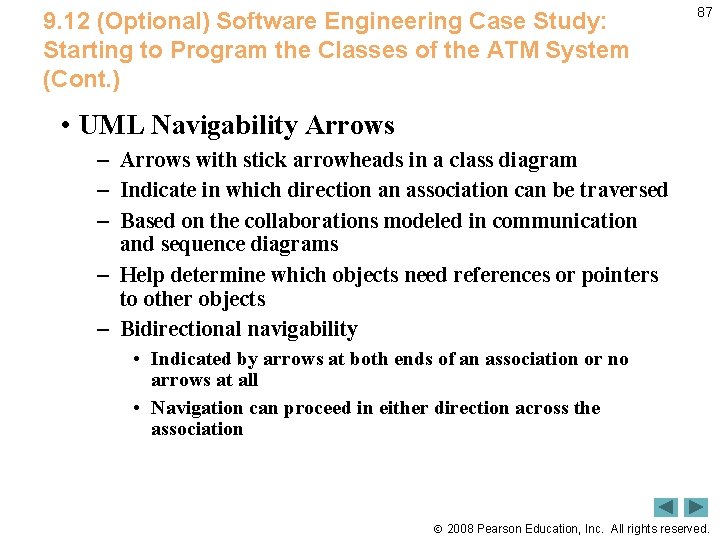
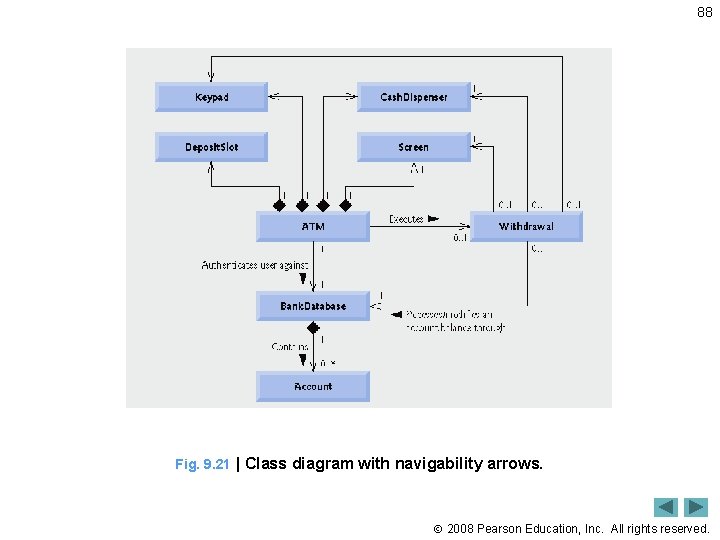
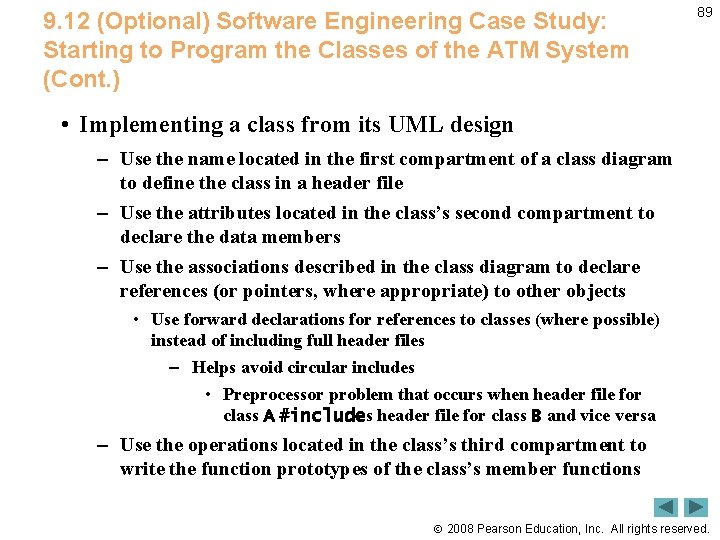
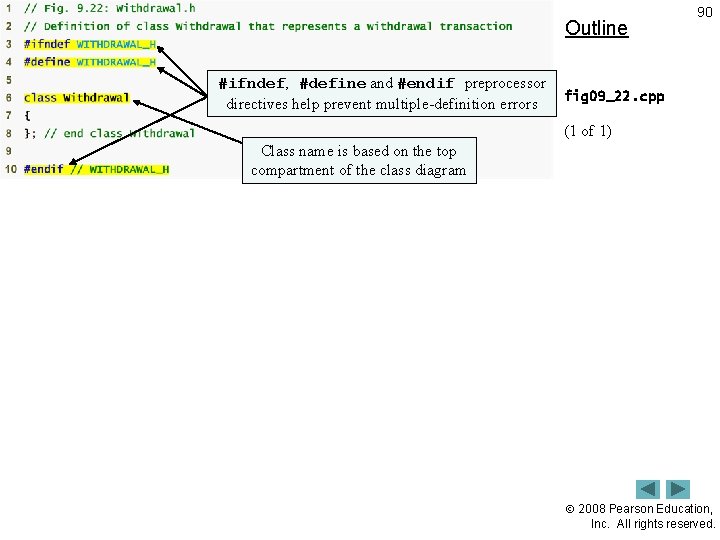
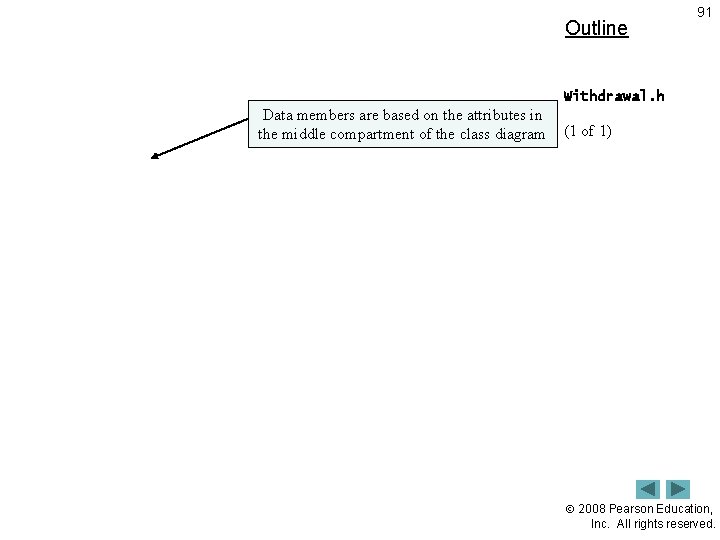
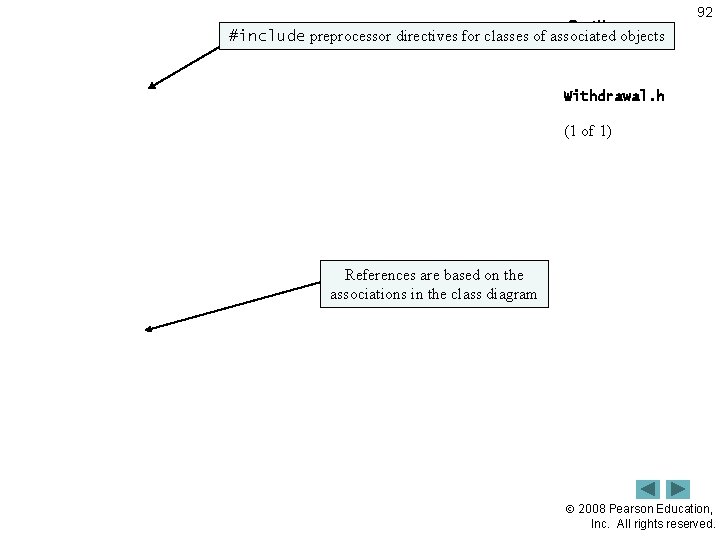
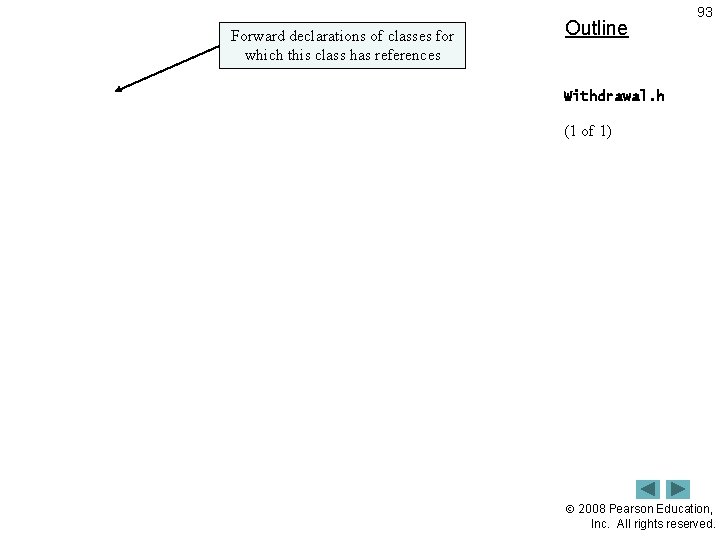
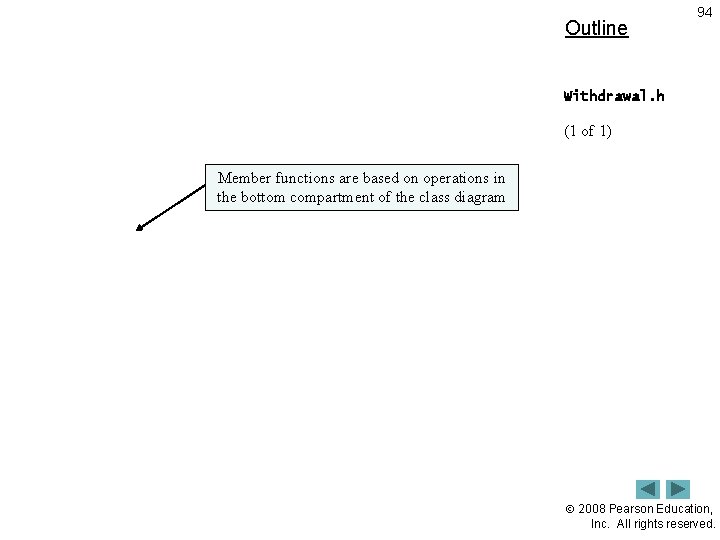
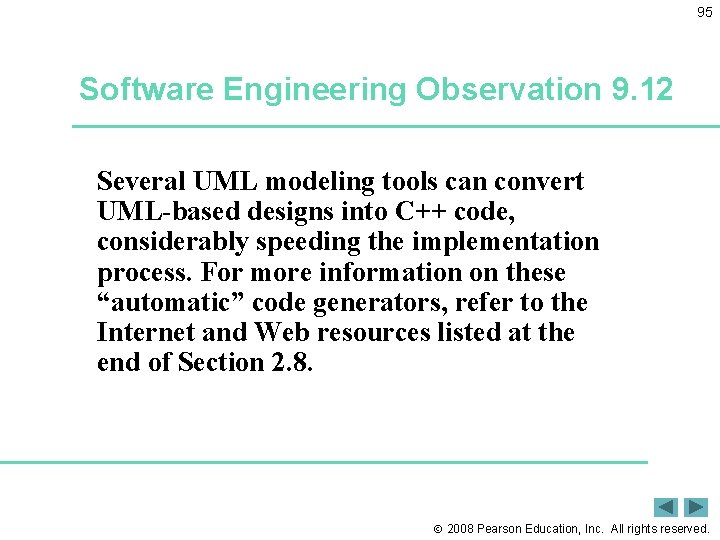
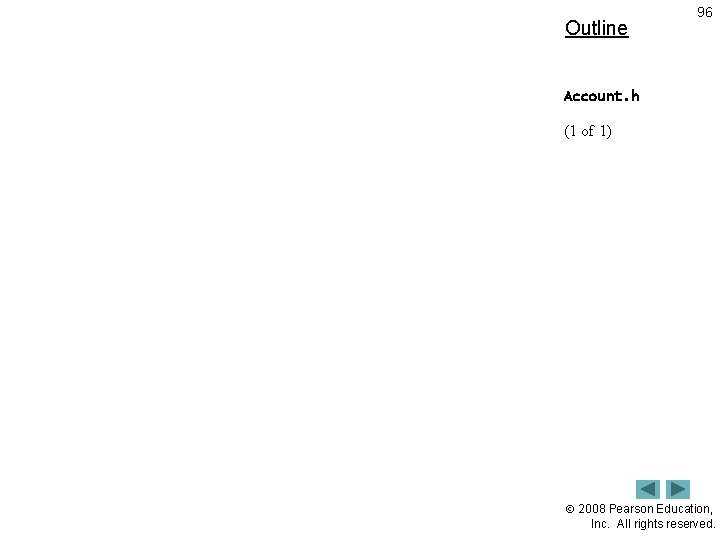
- Slides: 96
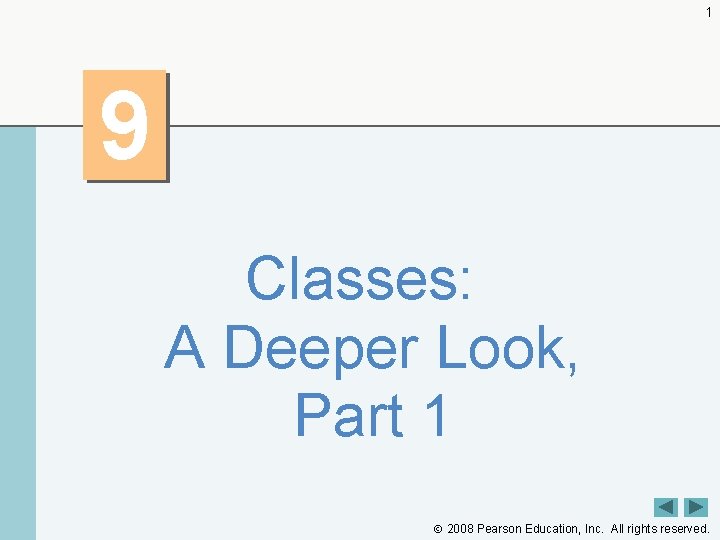
1 9 Classes: A Deeper Look, Part 1 2008 Pearson Education, Inc. All rights reserved.
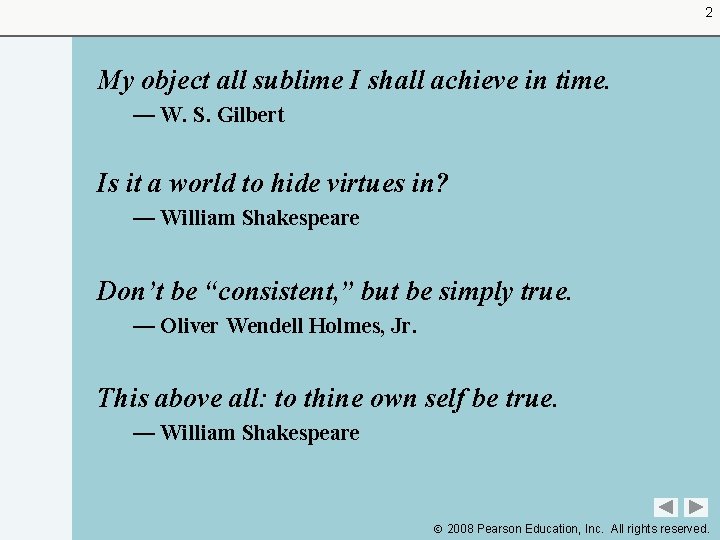
2 My object all sublime I shall achieve in time. — W. S. Gilbert Is it a world to hide virtues in? — William Shakespeare Don’t be “consistent, ” but be simply true. — Oliver Wendell Holmes, Jr. This above all: to thine own self be true. — William Shakespeare 2008 Pearson Education, Inc. All rights reserved.
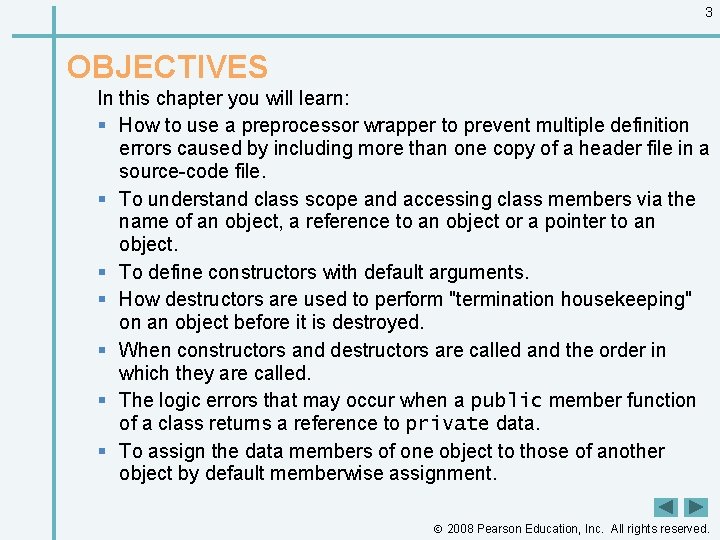
3 OBJECTIVES In this chapter you will learn: § How to use a preprocessor wrapper to prevent multiple definition errors caused by including more than one copy of a header file in a source-code file. § To understand class scope and accessing class members via the name of an object, a reference to an object or a pointer to an object. § To define constructors with default arguments. § How destructors are used to perform "termination housekeeping" on an object before it is destroyed. § When constructors and destructors are called and the order in which they are called. § The logic errors that may occur when a public member function of a class returns a reference to private data. § To assign the data members of one object to those of another object by default memberwise assignment. 2008 Pearson Education, Inc. All rights reserved.
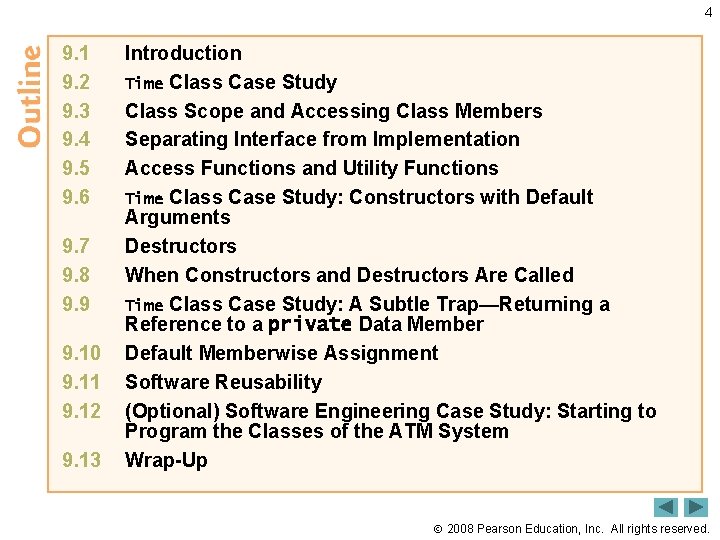
4 9. 1 9. 2 9. 3 9. 4 9. 5 9. 6 9. 7 9. 8 9. 9 9. 10 9. 11 9. 12 9. 13 Introduction Time Class Case Study Class Scope and Accessing Class Members Separating Interface from Implementation Access Functions and Utility Functions Time Class Case Study: Constructors with Default Arguments Destructors When Constructors and Destructors Are Called Time Class Case Study: A Subtle Trap—Returning a Reference to a private Data Member Default Memberwise Assignment Software Reusability (Optional) Software Engineering Case Study: Starting to Program the Classes of the ATM System Wrap-Up 2008 Pearson Education, Inc. All rights reserved.
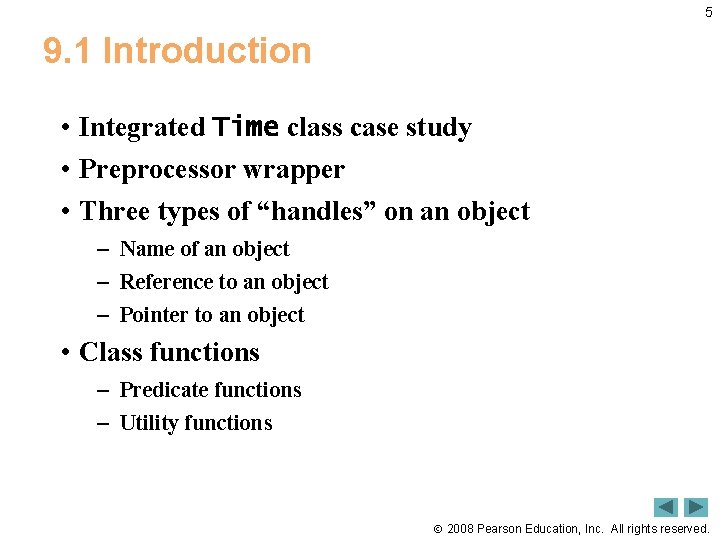
5 9. 1 Introduction • Integrated Time class case study • Preprocessor wrapper • Three types of “handles” on an object – Name of an object – Reference to an object – Pointer to an object • Class functions – Predicate functions – Utility functions 2008 Pearson Education, Inc. All rights reserved.
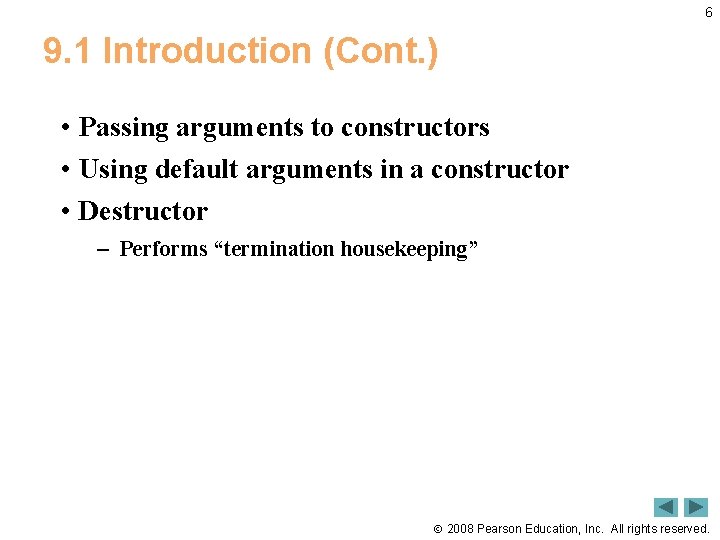
6 9. 1 Introduction (Cont. ) • Passing arguments to constructors • Using default arguments in a constructor • Destructor – Performs “termination housekeeping” 2008 Pearson Education, Inc. All rights reserved.
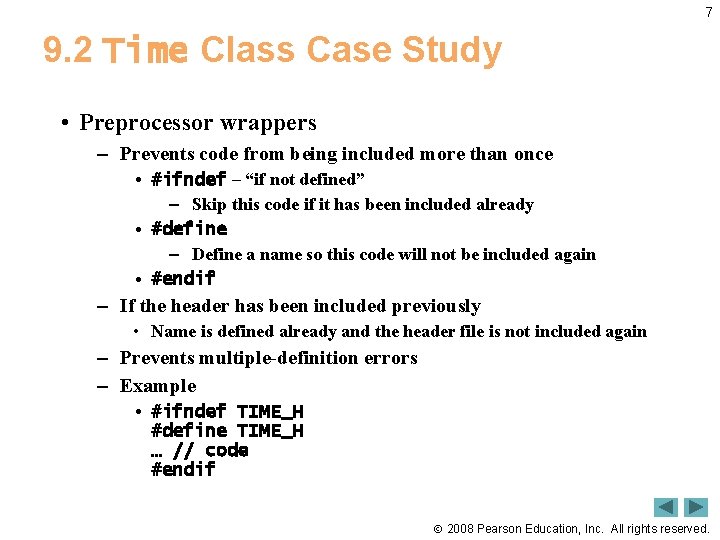
7 9. 2 Time Class Case Study • Preprocessor wrappers – Prevents code from being included more than once • #ifndef – “if not defined” – Skip this code if it has been included already • #define – Define a name so this code will not be included again • #endif – If the header has been included previously • Name is defined already and the header file is not included again – Prevents multiple-definition errors – Example • #ifndef TIME_H #define TIME_H … // code #endif 2008 Pearson Education, Inc. All rights reserved.
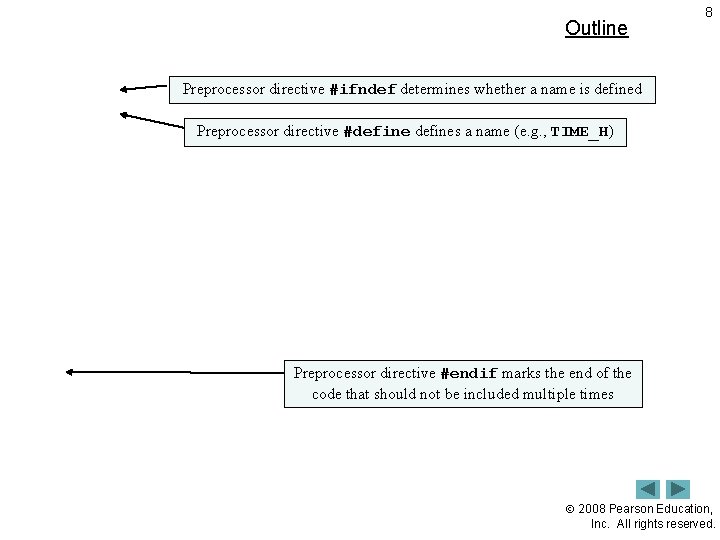
Outline 8 Preprocessor directive #ifndef determines whether a name is defined Time. h (1 of 1) Preprocessor directive #defines a name (e. g. , TIME_H) Preprocessor directive #endif marks the end of the code that should not be included multiple times 2008 Pearson Education, Inc. All rights reserved.
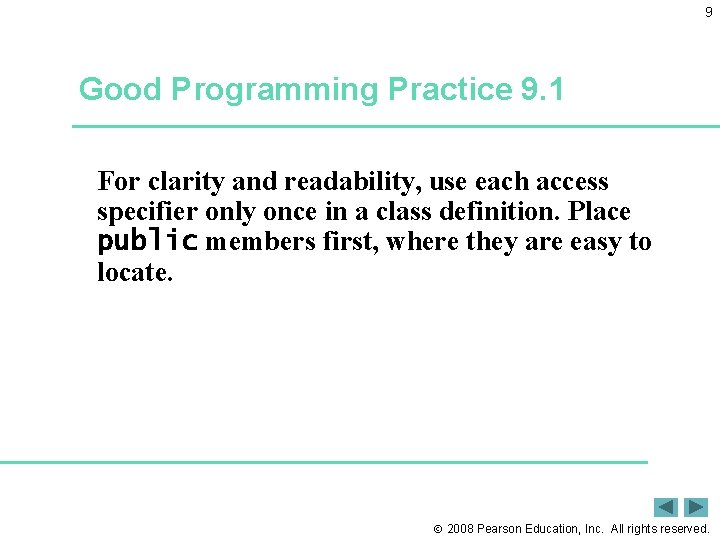
9 Good Programming Practice 9. 1 For clarity and readability, use each access specifier only once in a class definition. Place public members first, where they are easy to locate. 2008 Pearson Education, Inc. All rights reserved.
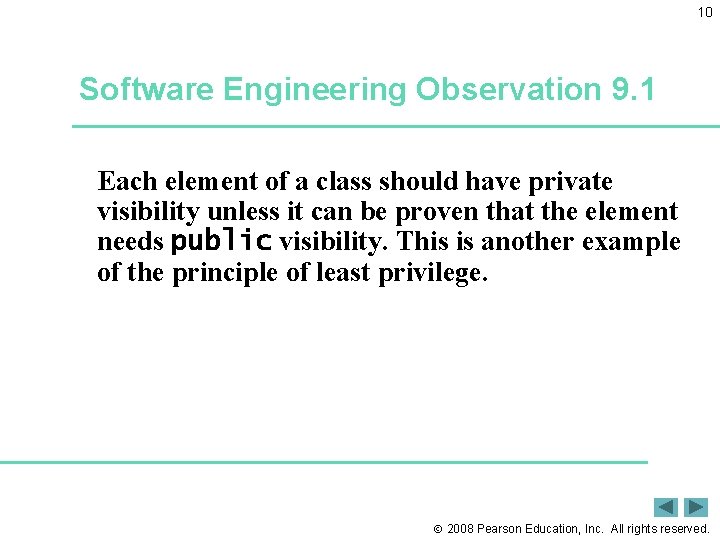
10 Software Engineering Observation 9. 1 Each element of a class should have private visibility unless it can be proven that the element needs public visibility. This is another example of the principle of least privilege. 2008 Pearson Education, Inc. All rights reserved.
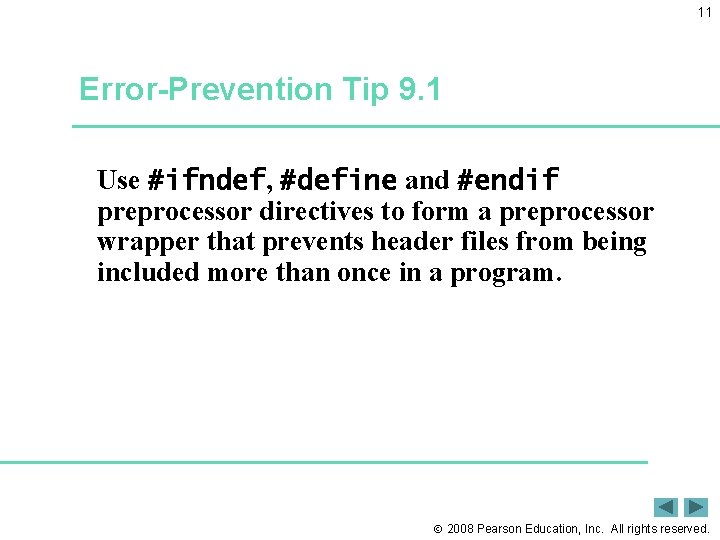
11 Error-Prevention Tip 9. 1 Use #ifndef, #define and #endif preprocessor directives to form a preprocessor wrapper that prevents header files from being included more than once in a program. 2008 Pearson Education, Inc. All rights reserved.
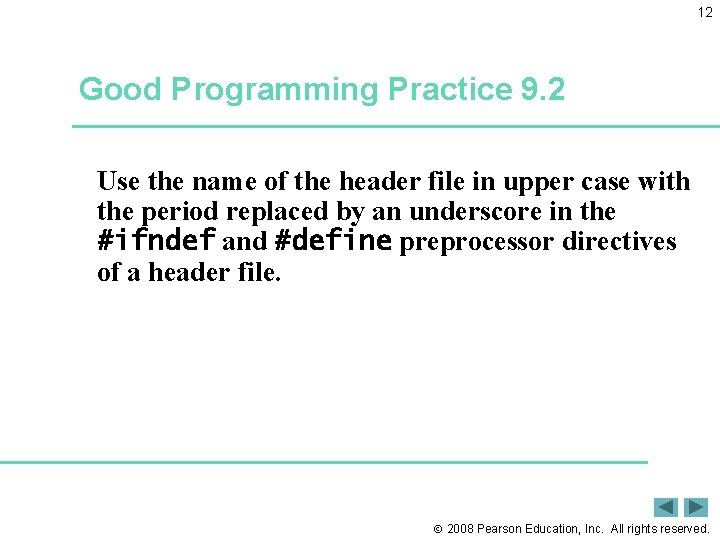
12 Good Programming Practice 9. 2 Use the name of the header file in upper case with the period replaced by an underscore in the #ifndef and #define preprocessor directives of a header file. 2008 Pearson Education, Inc. All rights reserved.
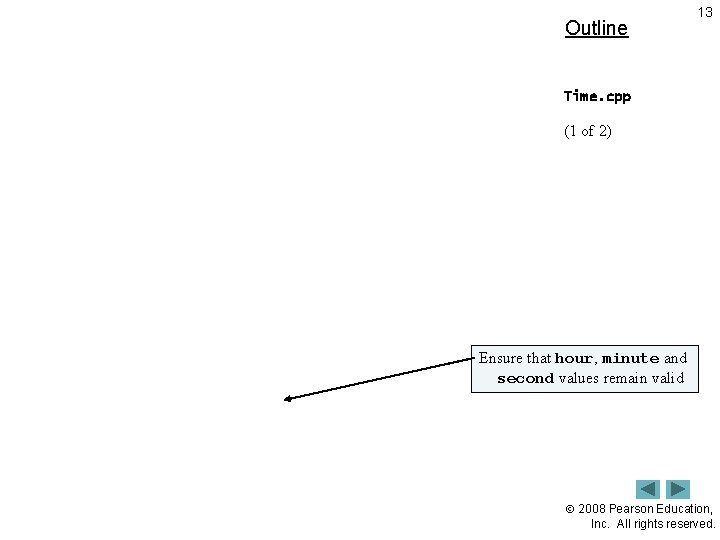
Outline 13 Time. cpp (1 of 2) Ensure that hour, minute and second values remain valid 2008 Pearson Education, Inc. All rights reserved.
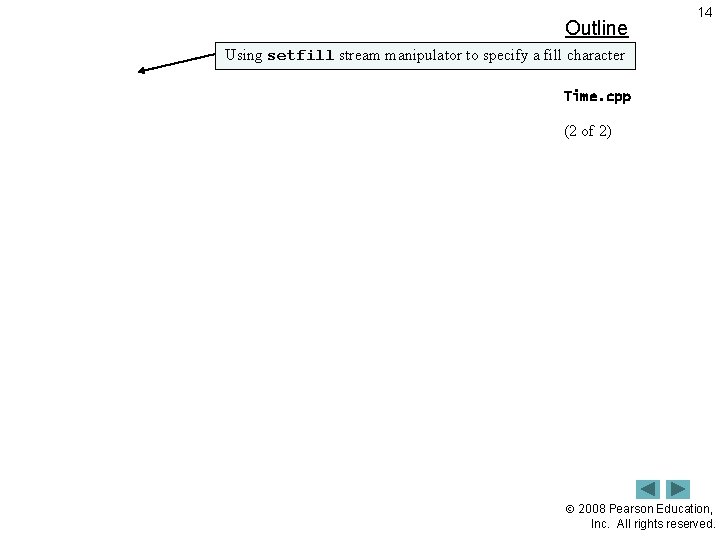
Outline 14 Using setfill stream manipulator to specify a fill character Time. cpp (2 of 2) 2008 Pearson Education, Inc. All rights reserved.
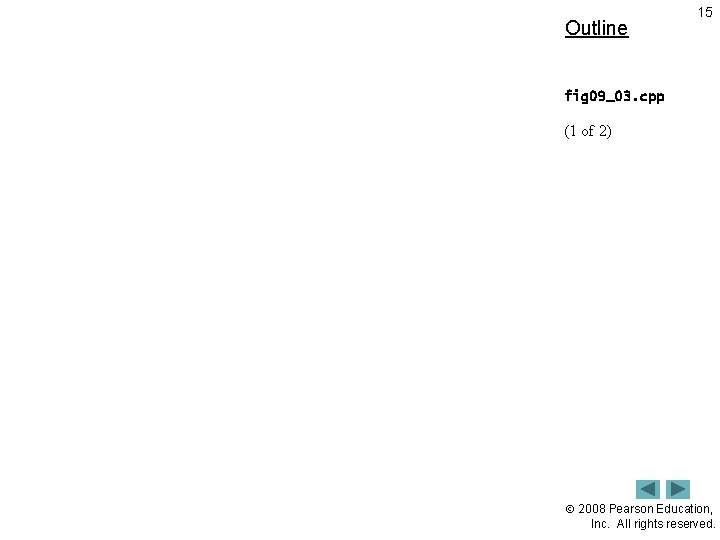
Outline 15 fig 09_03. cpp (1 of 2) 2008 Pearson Education, Inc. All rights reserved.
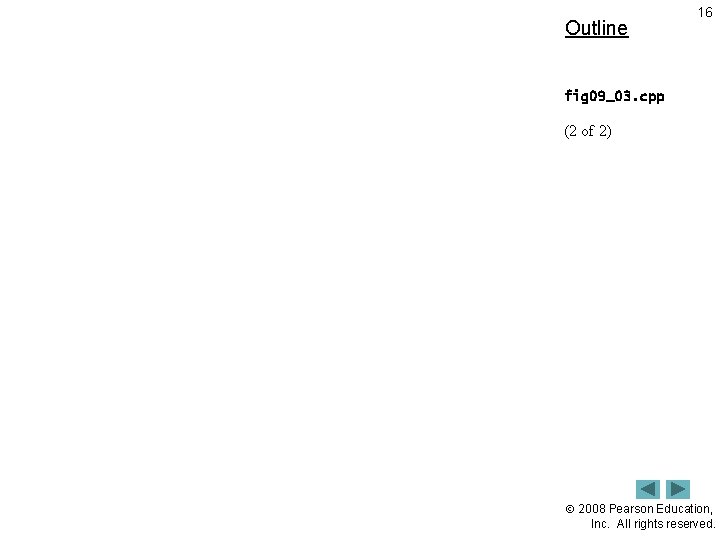
Outline 16 fig 09_03. cpp (2 of 2) 2008 Pearson Education, Inc. All rights reserved.
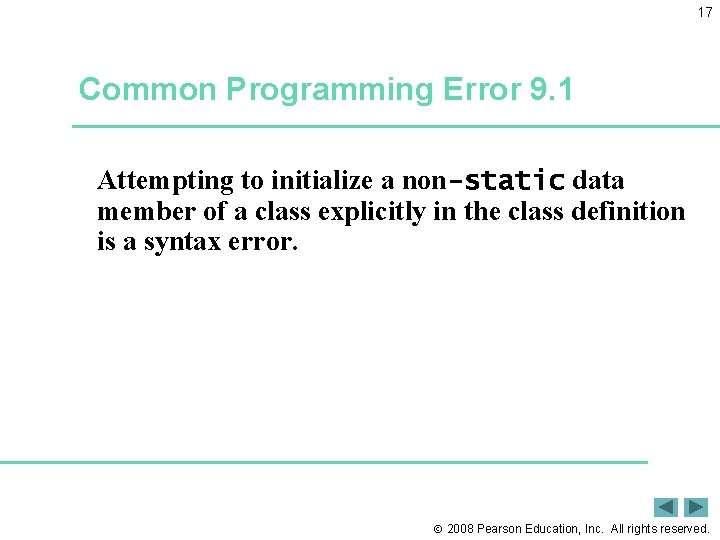
17 Common Programming Error 9. 1 Attempting to initialize a non-static data member of a class explicitly in the class definition is a syntax error. 2008 Pearson Education, Inc. All rights reserved.
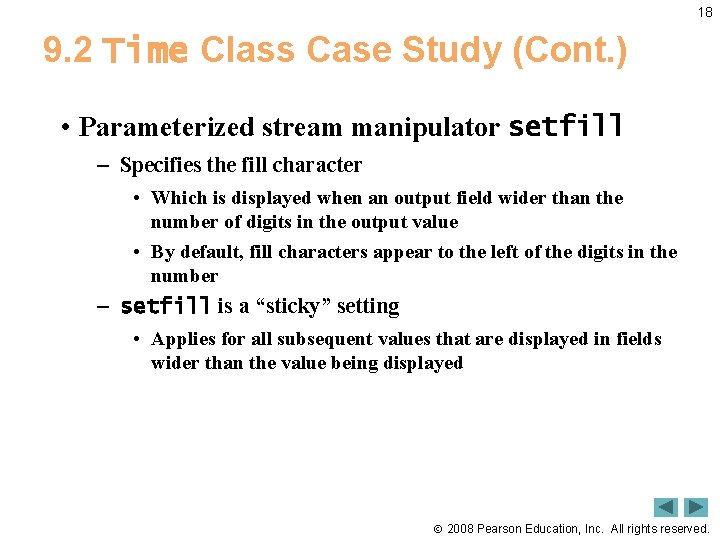
18 9. 2 Time Class Case Study (Cont. ) • Parameterized stream manipulator setfill – Specifies the fill character • Which is displayed when an output field wider than the number of digits in the output value • By default, fill characters appear to the left of the digits in the number – setfill is a “sticky” setting • Applies for all subsequent values that are displayed in fields wider than the value being displayed 2008 Pearson Education, Inc. All rights reserved.
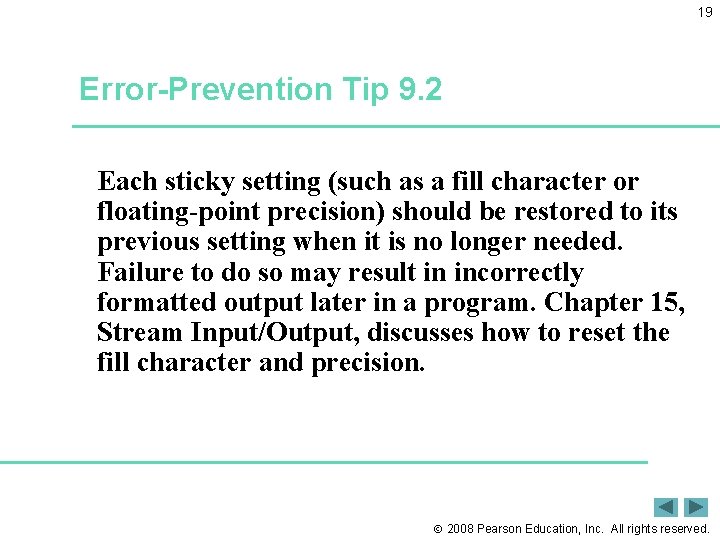
19 Error-Prevention Tip 9. 2 Each sticky setting (such as a fill character or floating-point precision) should be restored to its previous setting when it is no longer needed. Failure to do so may result in incorrectly formatted output later in a program. Chapter 15, Stream Input/Output, discusses how to reset the fill character and precision. 2008 Pearson Education, Inc. All rights reserved.
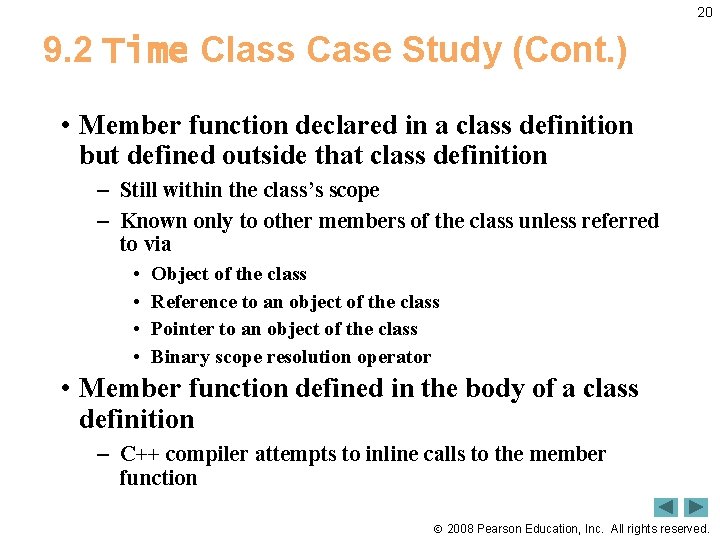
20 9. 2 Time Class Case Study (Cont. ) • Member function declared in a class definition but defined outside that class definition – Still within the class’s scope – Known only to other members of the class unless referred to via • • Object of the class Reference to an object of the class Pointer to an object of the class Binary scope resolution operator • Member function defined in the body of a class definition – C++ compiler attempts to inline calls to the member function 2008 Pearson Education, Inc. All rights reserved.
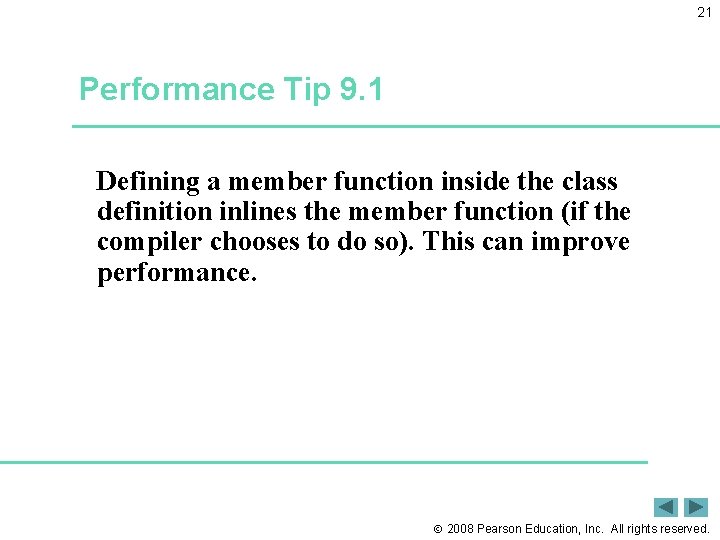
21 Performance Tip 9. 1 Defining a member function inside the class definition inlines the member function (if the compiler chooses to do so). This can improve performance. 2008 Pearson Education, Inc. All rights reserved.
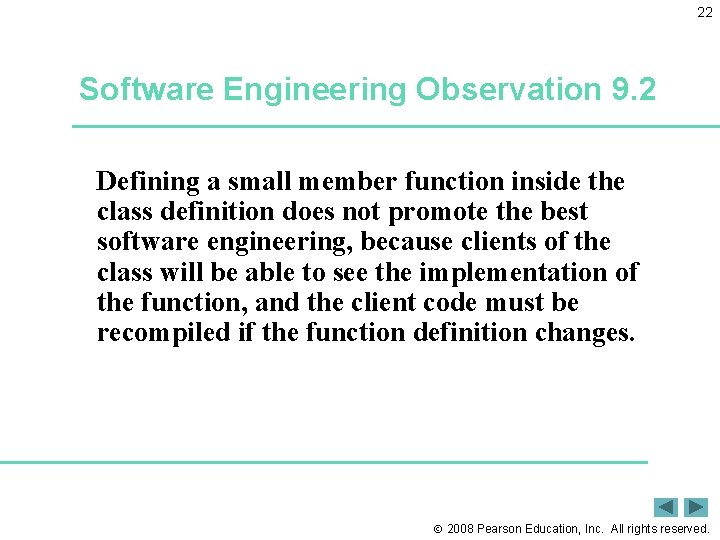
22 Software Engineering Observation 9. 2 Defining a small member function inside the class definition does not promote the best software engineering, because clients of the class will be able to see the implementation of the function, and the client code must be recompiled if the function definition changes. 2008 Pearson Education, Inc. All rights reserved.
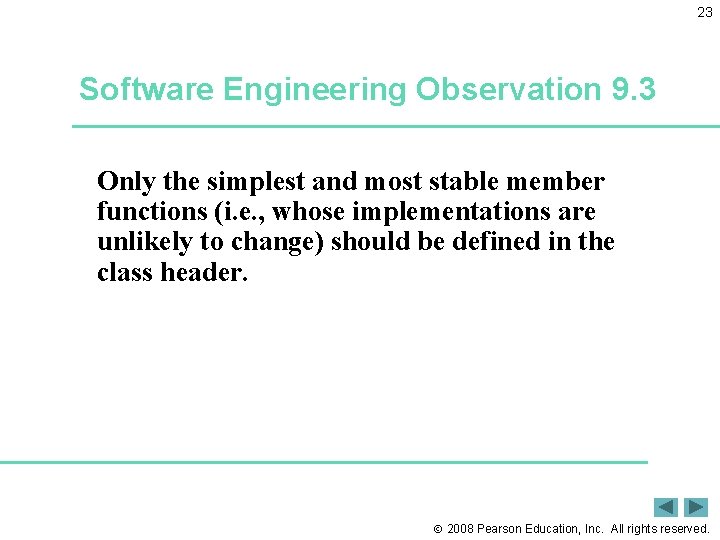
23 Software Engineering Observation 9. 3 Only the simplest and most stable member functions (i. e. , whose implementations are unlikely to change) should be defined in the class header. 2008 Pearson Education, Inc. All rights reserved.
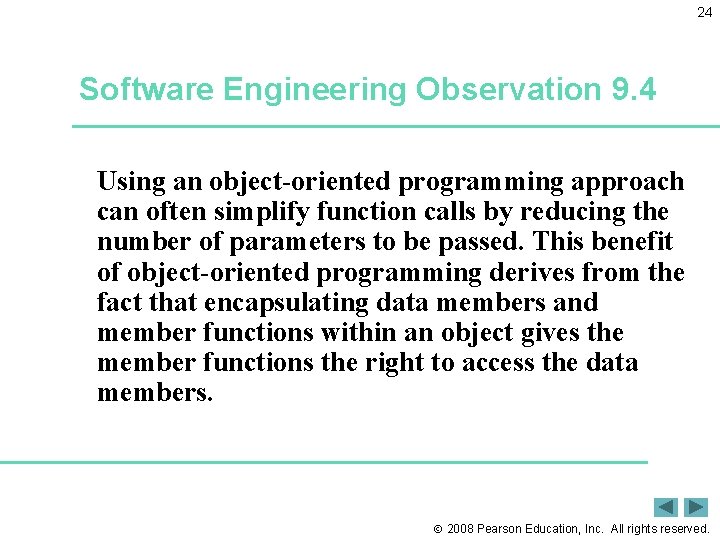
24 Software Engineering Observation 9. 4 Using an object-oriented programming approach can often simplify function calls by reducing the number of parameters to be passed. This benefit of object-oriented programming derives from the fact that encapsulating data members and member functions within an object gives the member functions the right to access the data members. 2008 Pearson Education, Inc. All rights reserved.
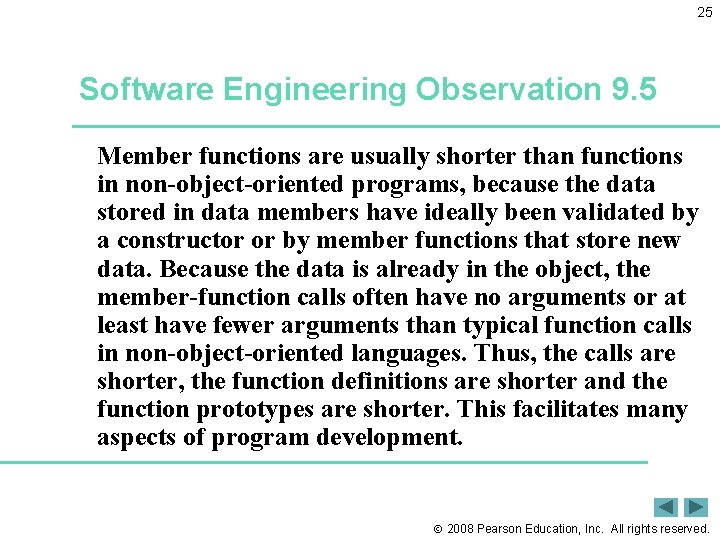
25 Software Engineering Observation 9. 5 Member functions are usually shorter than functions in non-object-oriented programs, because the data stored in data members have ideally been validated by a constructor or by member functions that store new data. Because the data is already in the object, the member-function calls often have no arguments or at least have fewer arguments than typical function calls in non-object-oriented languages. Thus, the calls are shorter, the function definitions are shorter and the function prototypes are shorter. This facilitates many aspects of program development. 2008 Pearson Education, Inc. All rights reserved.
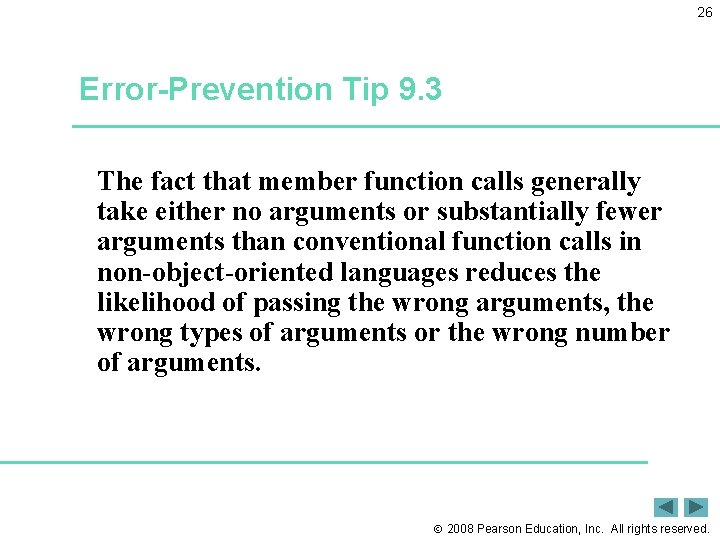
26 Error-Prevention Tip 9. 3 The fact that member function calls generally take either no arguments or substantially fewer arguments than conventional function calls in non-object-oriented languages reduces the likelihood of passing the wrong arguments, the wrong types of arguments or the wrong number of arguments. 2008 Pearson Education, Inc. All rights reserved.
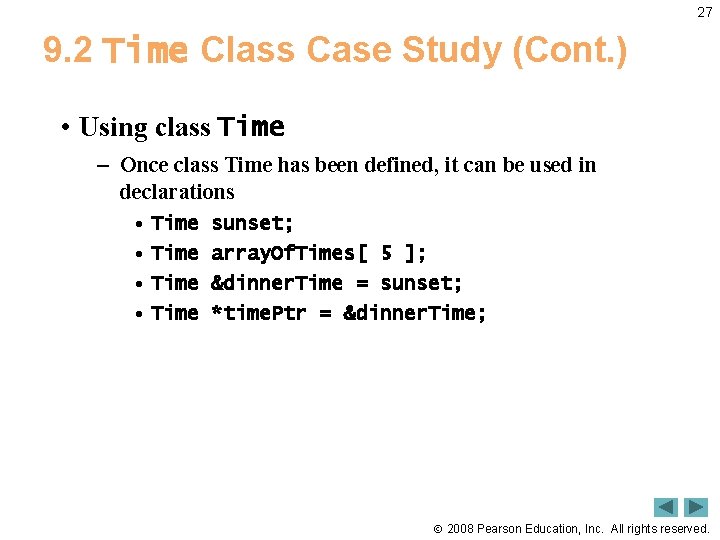
27 9. 2 Time Class Case Study (Cont. ) • Using class Time – Once class Time has been defined, it can be used in declarations • Time sunset; array. Of. Times[ 5 ]; &dinner. Time = sunset; *time. Ptr = &dinner. Time; 2008 Pearson Education, Inc. All rights reserved.
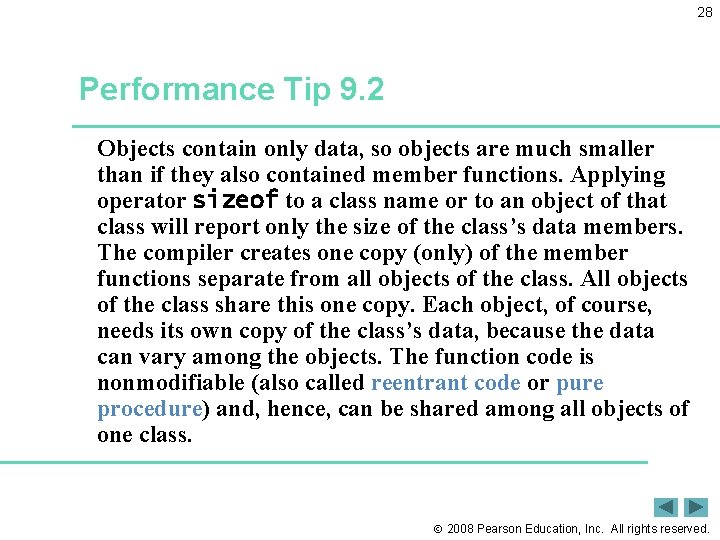
28 Performance Tip 9. 2 Objects contain only data, so objects are much smaller than if they also contained member functions. Applying operator sizeof to a class name or to an object of that class will report only the size of the class’s data members. The compiler creates one copy (only) of the member functions separate from all objects of the class. All objects of the class share this one copy. Each object, of course, needs its own copy of the class’s data, because the data can vary among the objects. The function code is nonmodifiable (also called reentrant code or pure procedure) and, hence, can be shared among all objects of one class. 2008 Pearson Education, Inc. All rights reserved.
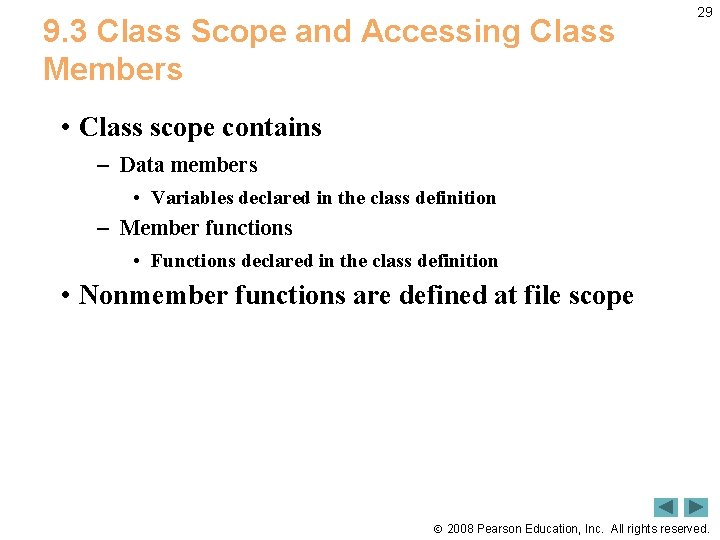
9. 3 Class Scope and Accessing Class Members 29 • Class scope contains – Data members • Variables declared in the class definition – Member functions • Functions declared in the class definition • Nonmember functions are defined at file scope 2008 Pearson Education, Inc. All rights reserved.
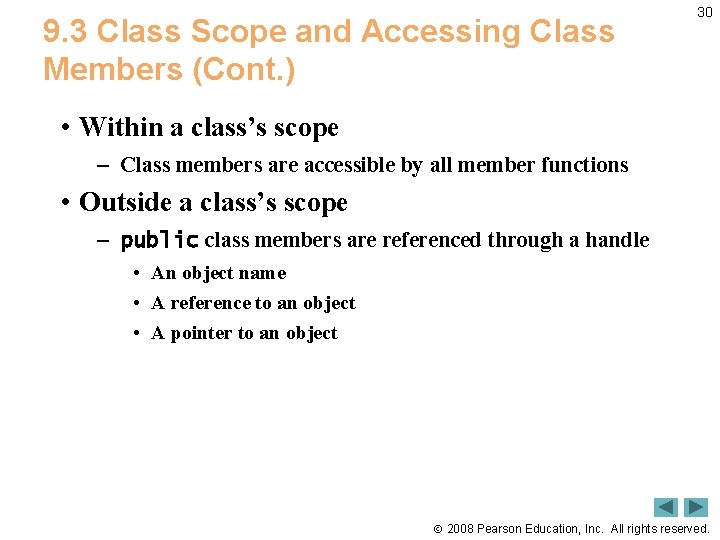
9. 3 Class Scope and Accessing Class Members (Cont. ) 30 • Within a class’s scope – Class members are accessible by all member functions • Outside a class’s scope – public class members are referenced through a handle • An object name • A reference to an object • A pointer to an object 2008 Pearson Education, Inc. All rights reserved.
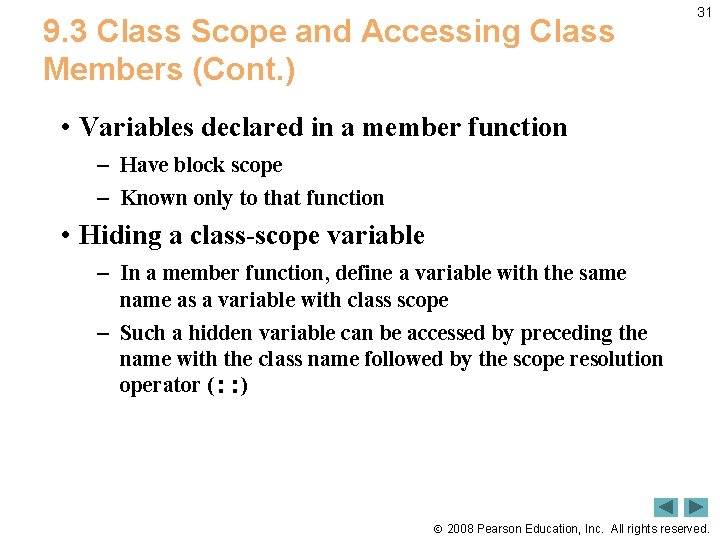
9. 3 Class Scope and Accessing Class Members (Cont. ) 31 • Variables declared in a member function – Have block scope – Known only to that function • Hiding a class-scope variable – In a member function, define a variable with the same name as a variable with class scope – Such a hidden variable can be accessed by preceding the name with the class name followed by the scope resolution operator (: : ) 2008 Pearson Education, Inc. All rights reserved.
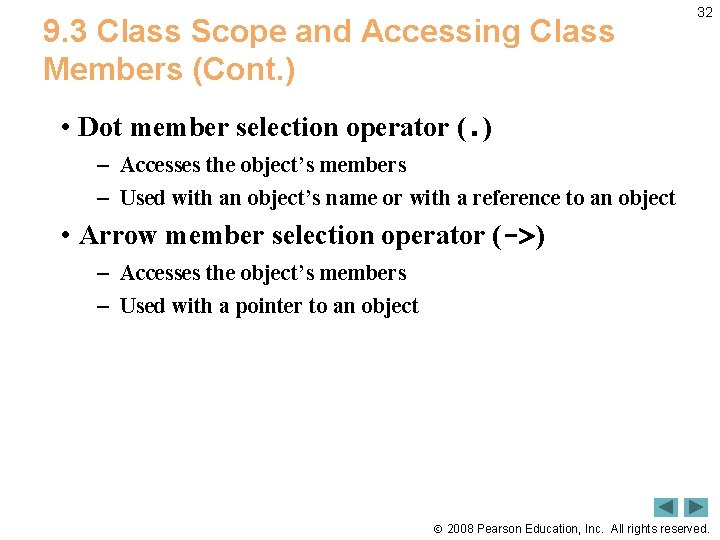
9. 3 Class Scope and Accessing Class Members (Cont. ) 32 • Dot member selection operator (. ) – Accesses the object’s members – Used with an object’s name or with a reference to an object • Arrow member selection operator (->) – Accesses the object’s members – Used with a pointer to an object 2008 Pearson Education, Inc. All rights reserved.
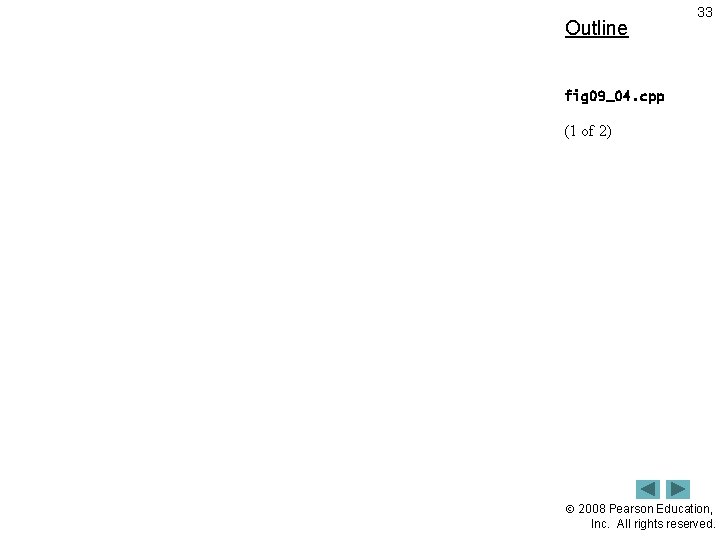
Outline 33 fig 09_04. cpp (1 of 2) 2008 Pearson Education, Inc. All rights reserved.
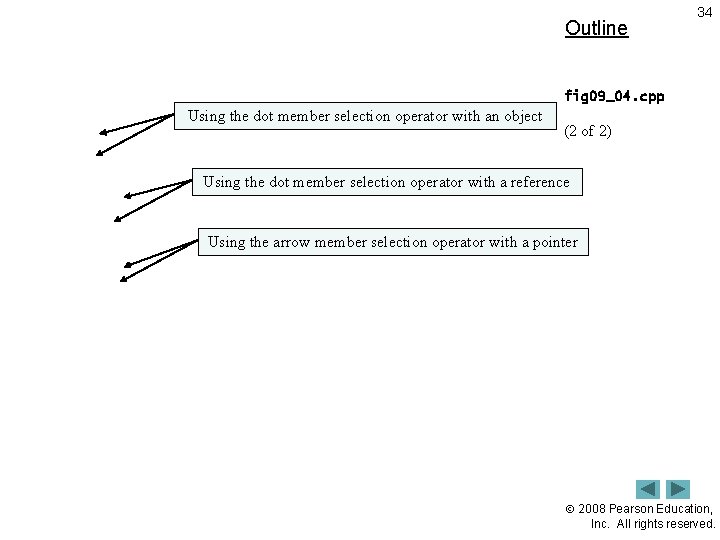
Outline 34 fig 09_04. cpp Using the dot member selection operator with an object (2 of 2) Using the dot member selection operator with a reference Using the arrow member selection operator with a pointer 2008 Pearson Education, Inc. All rights reserved.
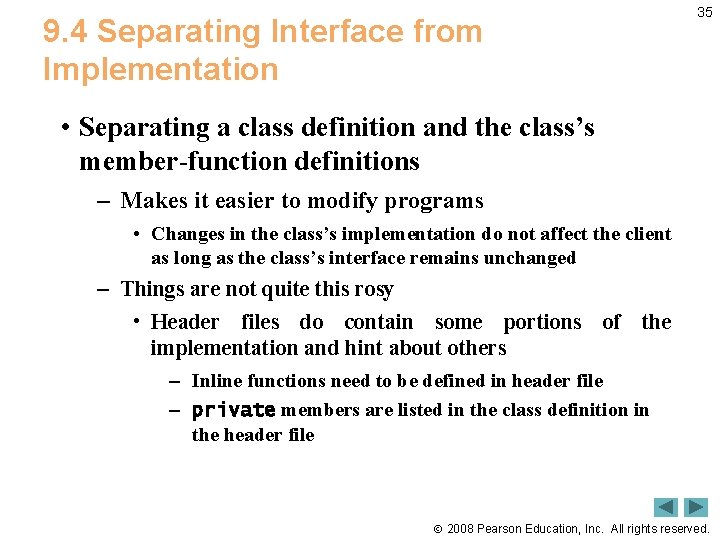
9. 4 Separating Interface from Implementation 35 • Separating a class definition and the class’s member-function definitions – Makes it easier to modify programs • Changes in the class’s implementation do not affect the client as long as the class’s interface remains unchanged – Things are not quite this rosy • Header files do contain some portions of the implementation and hint about others – Inline functions need to be defined in header file – private members are listed in the class definition in the header file 2008 Pearson Education, Inc. All rights reserved.
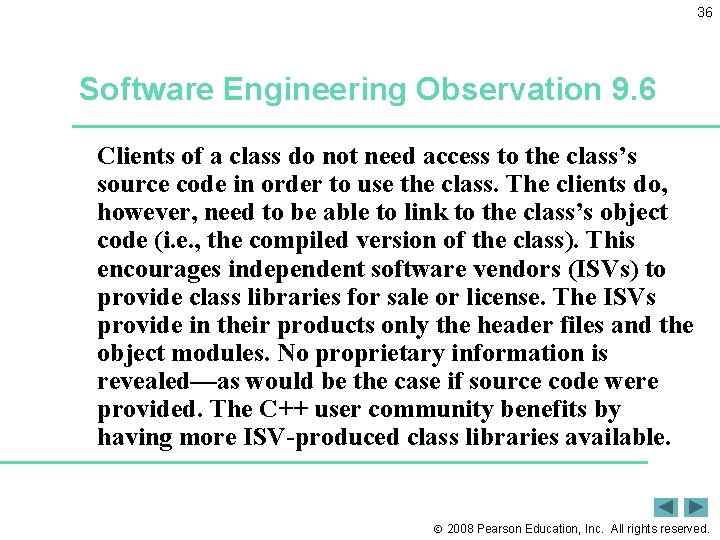
36 Software Engineering Observation 9. 6 Clients of a class do not need access to the class’s source code in order to use the class. The clients do, however, need to be able to link to the class’s object code (i. e. , the compiled version of the class). This encourages independent software vendors (ISVs) to provide class libraries for sale or license. The ISVs provide in their products only the header files and the object modules. No proprietary information is revealed—as would be the case if source code were provided. The C++ user community benefits by having more ISV-produced class libraries available. 2008 Pearson Education, Inc. All rights reserved.
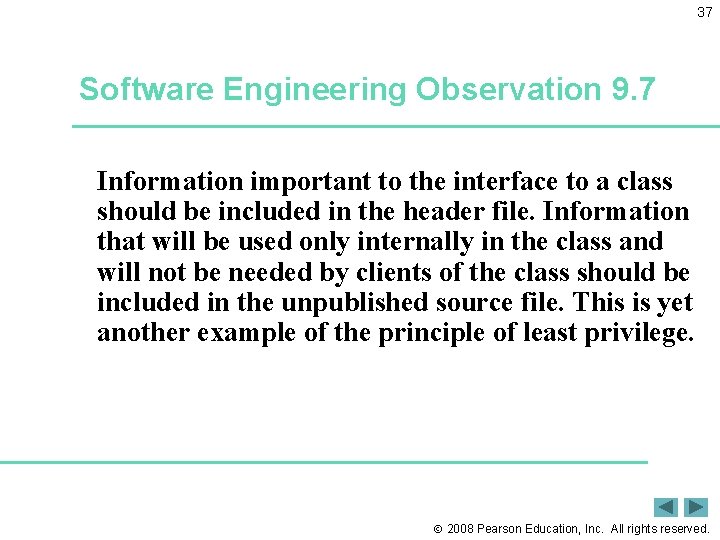
37 Software Engineering Observation 9. 7 Information important to the interface to a class should be included in the header file. Information that will be used only internally in the class and will not be needed by clients of the class should be included in the unpublished source file. This is yet another example of the principle of least privilege. 2008 Pearson Education, Inc. All rights reserved.
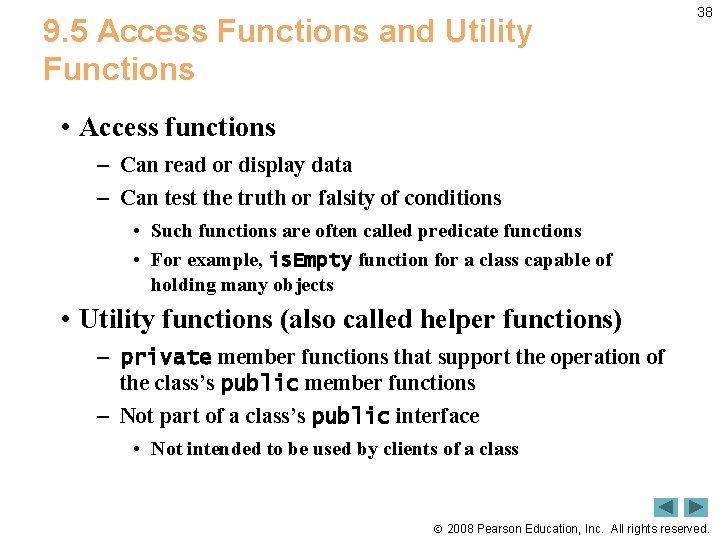
9. 5 Access Functions and Utility Functions 38 • Access functions – Can read or display data – Can test the truth or falsity of conditions • Such functions are often called predicate functions • For example, is. Empty function for a class capable of holding many objects • Utility functions (also called helper functions) – private member functions that support the operation of the class’s public member functions – Not part of a class’s public interface • Not intended to be used by clients of a class 2008 Pearson Education, Inc. All rights reserved.
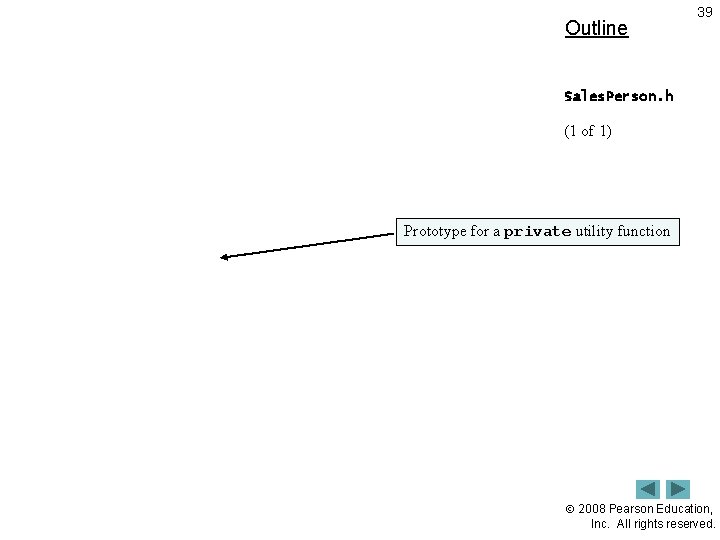
Outline 39 Sales. Person. h (1 of 1) Prototype for a private utility function 2008 Pearson Education, Inc. All rights reserved.
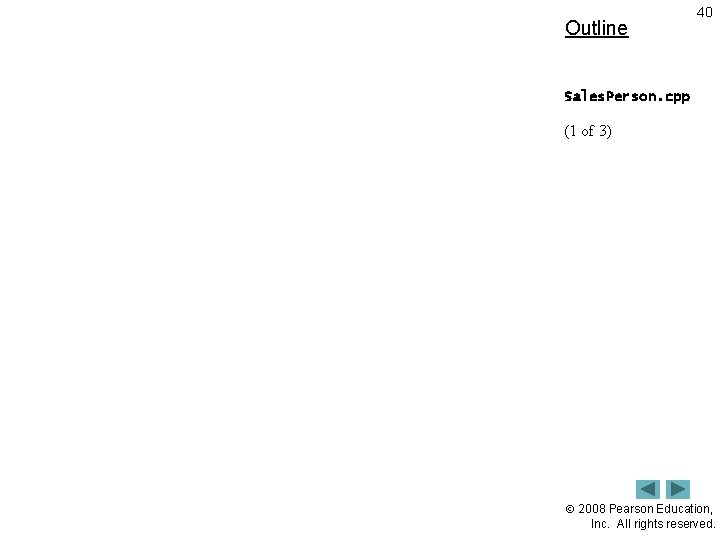
Outline 40 Sales. Person. cpp (1 of 3) 2008 Pearson Education, Inc. All rights reserved.
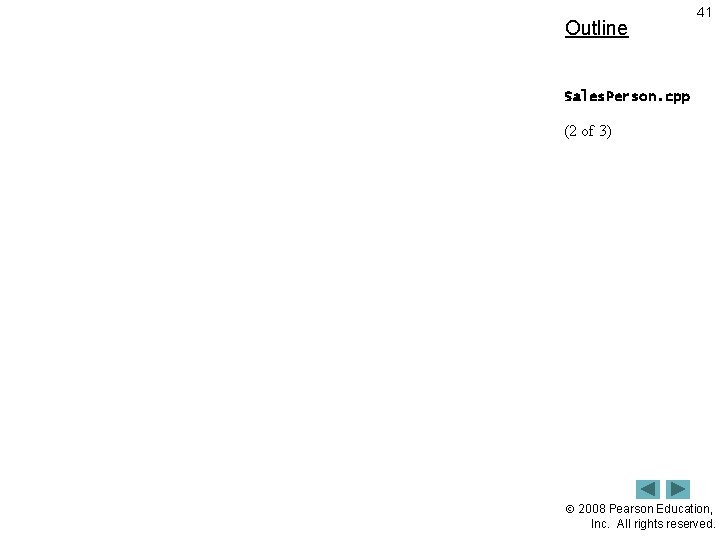
Outline 41 Sales. Person. cpp (2 of 3) 2008 Pearson Education, Inc. All rights reserved.
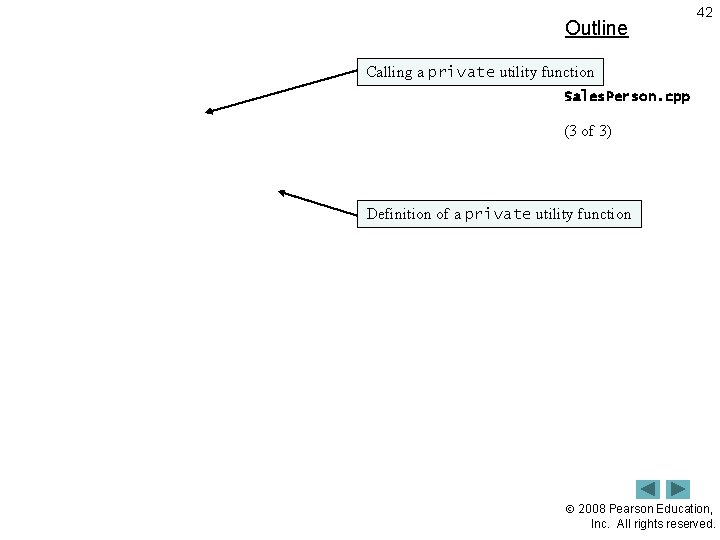
Outline 42 Calling a private utility function Sales. Person. cpp (3 of 3) Definition of a private utility function 2008 Pearson Education, Inc. All rights reserved.
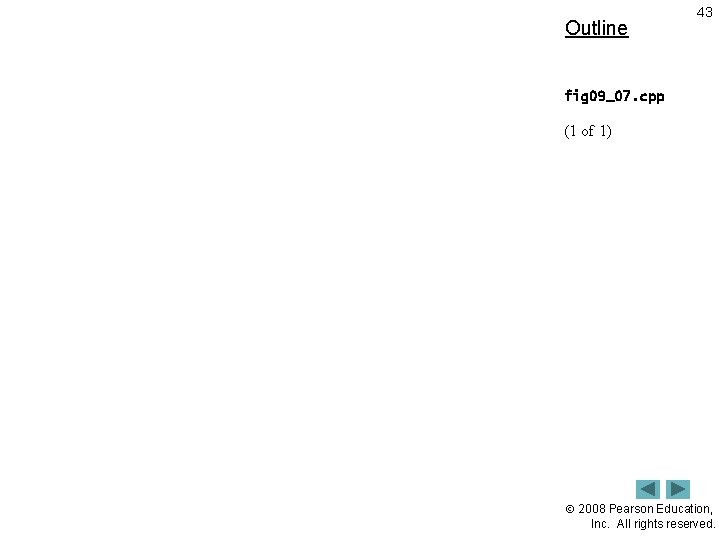
Outline 43 fig 09_07. cpp (1 of 1) 2008 Pearson Education, Inc. All rights reserved.
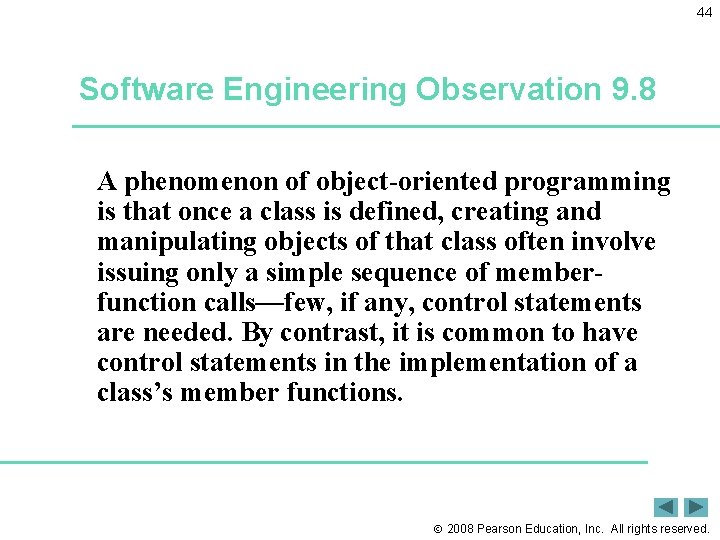
44 Software Engineering Observation 9. 8 A phenomenon of object-oriented programming is that once a class is defined, creating and manipulating objects of that class often involve issuing only a simple sequence of memberfunction calls—few, if any, control statements are needed. By contrast, it is common to have control statements in the implementation of a class’s member functions. 2008 Pearson Education, Inc. All rights reserved.
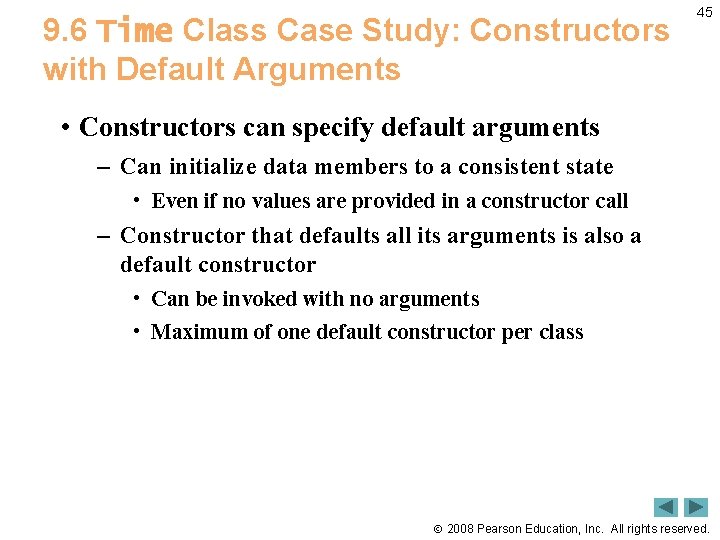
9. 6 Time Class Case Study: Constructors with Default Arguments 45 • Constructors can specify default arguments – Can initialize data members to a consistent state • Even if no values are provided in a constructor call – Constructor that defaults all its arguments is also a default constructor • Can be invoked with no arguments • Maximum of one default constructor per class 2008 Pearson Education, Inc. All rights reserved.
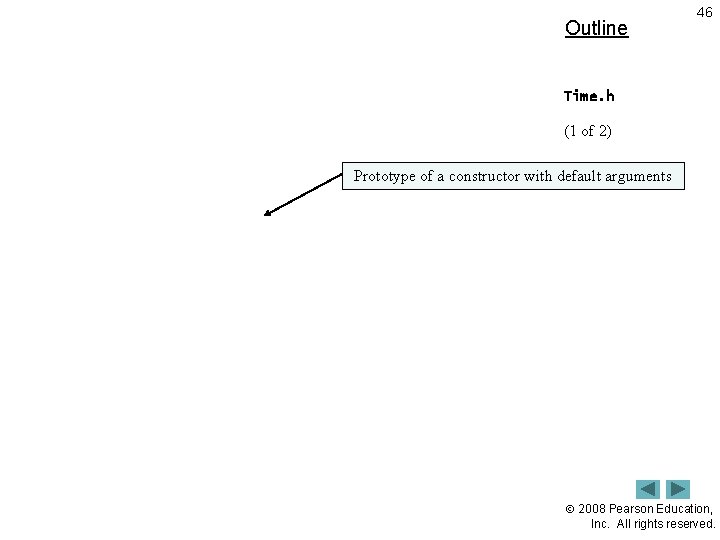
Outline 46 Time. h (1 of 2) Prototype of a constructor with default arguments 2008 Pearson Education, Inc. All rights reserved.
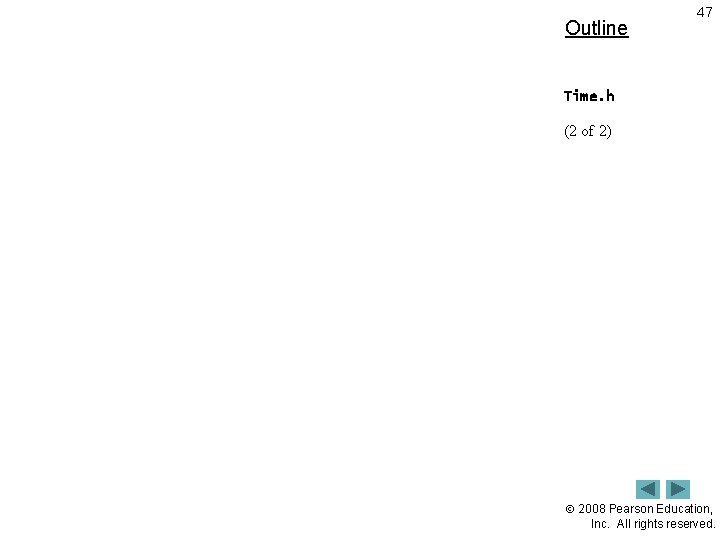
Outline 47 Time. h (2 of 2) 2008 Pearson Education, Inc. All rights reserved.
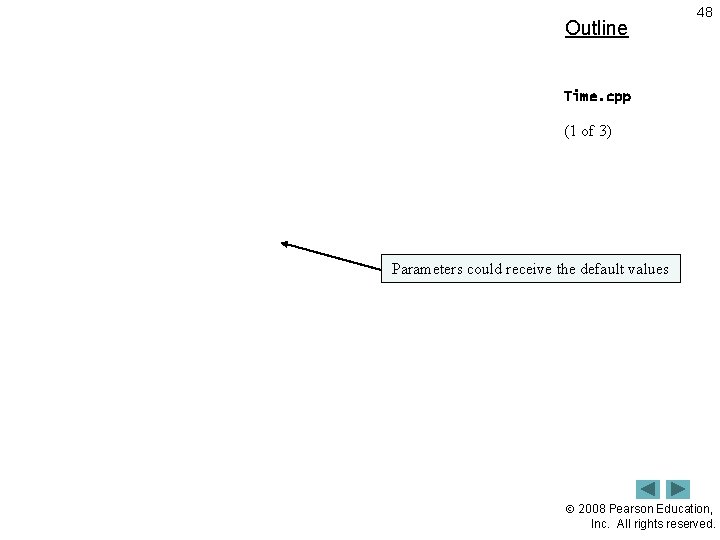
Outline 48 Time. cpp (1 of 3) Parameters could receive the default values 2008 Pearson Education, Inc. All rights reserved.
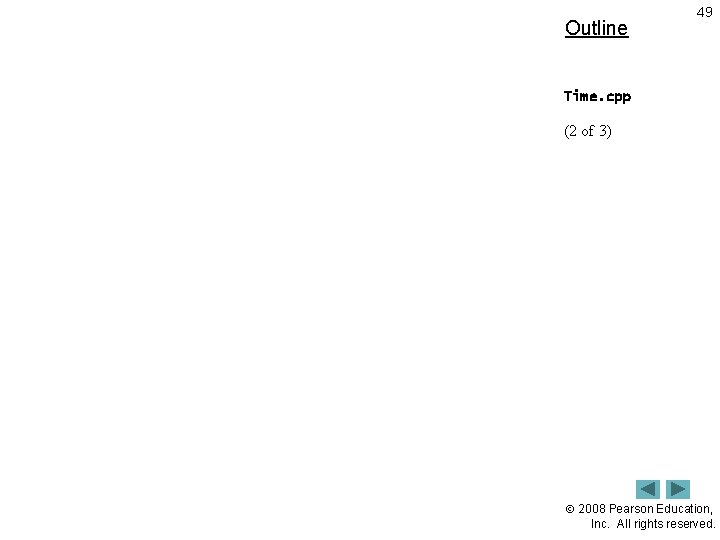
Outline 49 Time. cpp (2 of 3) 2008 Pearson Education, Inc. All rights reserved.
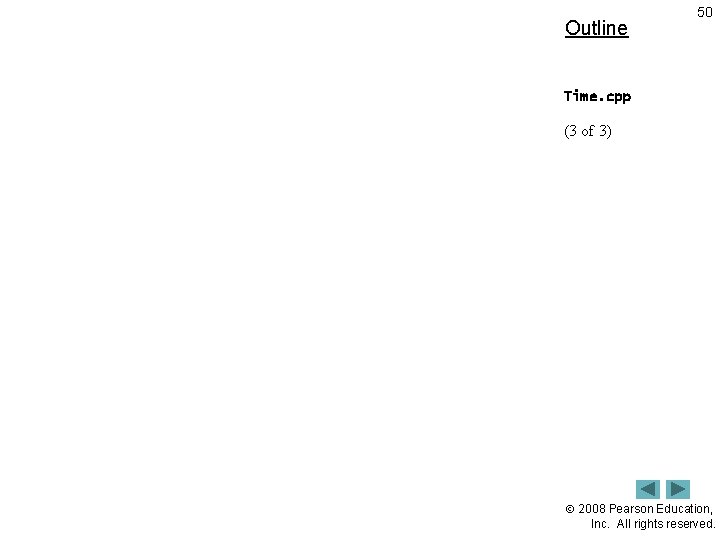
Outline 50 Time. cpp (3 of 3) 2008 Pearson Education, Inc. All rights reserved.
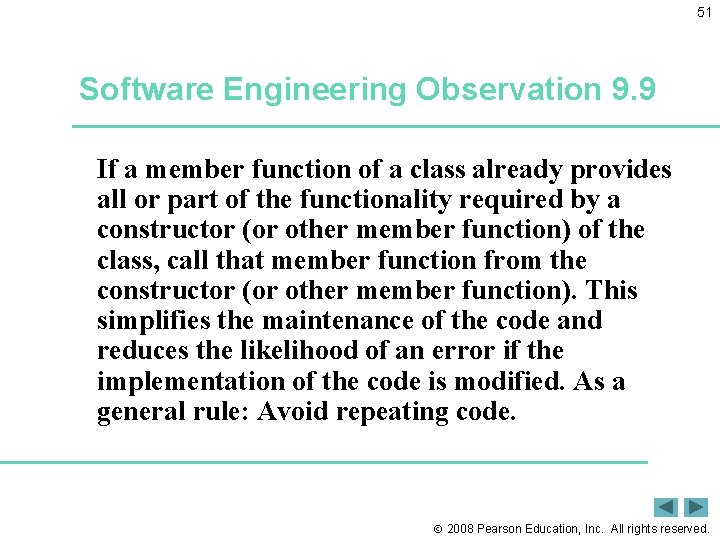
51 Software Engineering Observation 9. 9 If a member function of a class already provides all or part of the functionality required by a constructor (or other member function) of the class, call that member function from the constructor (or other member function). This simplifies the maintenance of the code and reduces the likelihood of an error if the implementation of the code is modified. As a general rule: Avoid repeating code. 2008 Pearson Education, Inc. All rights reserved.
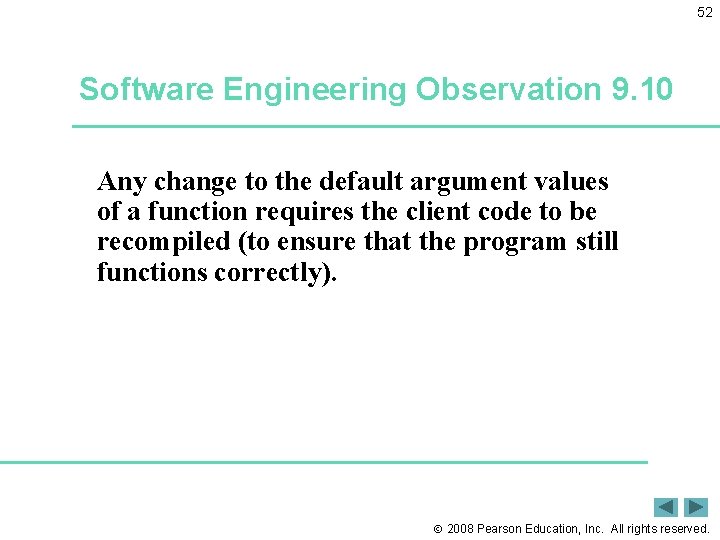
52 Software Engineering Observation 9. 10 Any change to the default argument values of a function requires the client code to be recompiled (to ensure that the program still functions correctly). 2008 Pearson Education, Inc. All rights reserved.
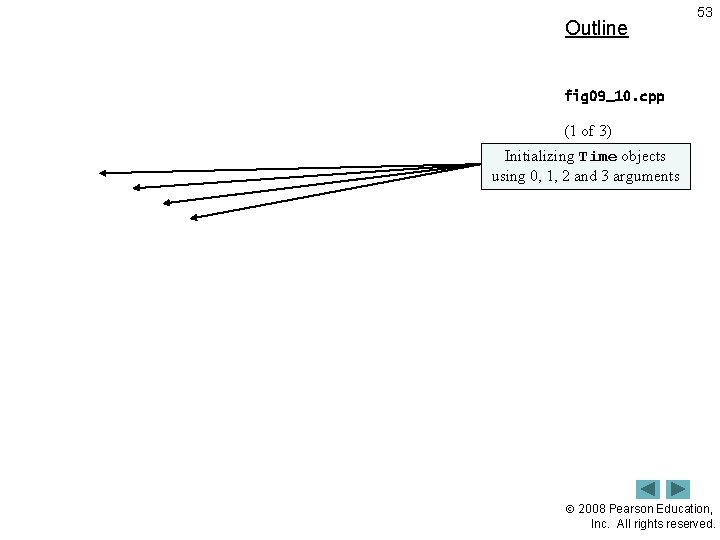
Outline 53 fig 09_10. cpp (1 of 3) Initializing Time objects using 0, 1, 2 and 3 arguments 2008 Pearson Education, Inc. All rights reserved.
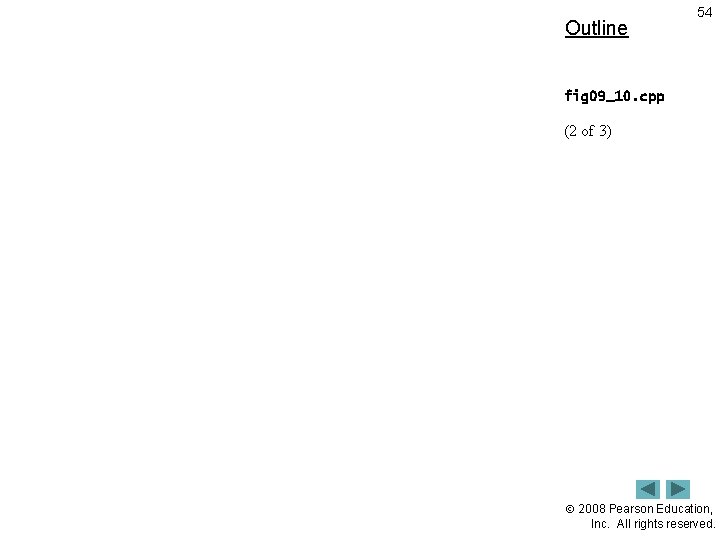
Outline 54 fig 09_10. cpp (2 of 3) 2008 Pearson Education, Inc. All rights reserved.
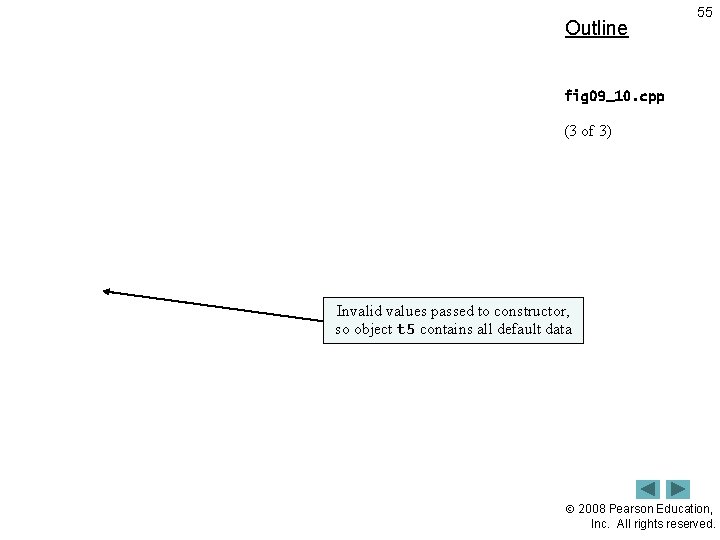
Outline 55 fig 09_10. cpp (3 of 3) Invalid values passed to constructor, so object t 5 contains all default data 2008 Pearson Education, Inc. All rights reserved.
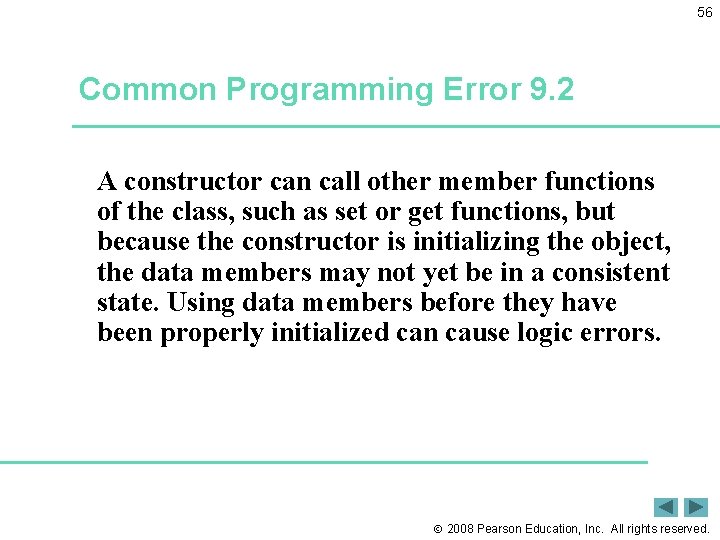
56 Common Programming Error 9. 2 A constructor can call other member functions of the class, such as set or get functions, but because the constructor is initializing the object, the data members may not yet be in a consistent state. Using data members before they have been properly initialized can cause logic errors. 2008 Pearson Education, Inc. All rights reserved.
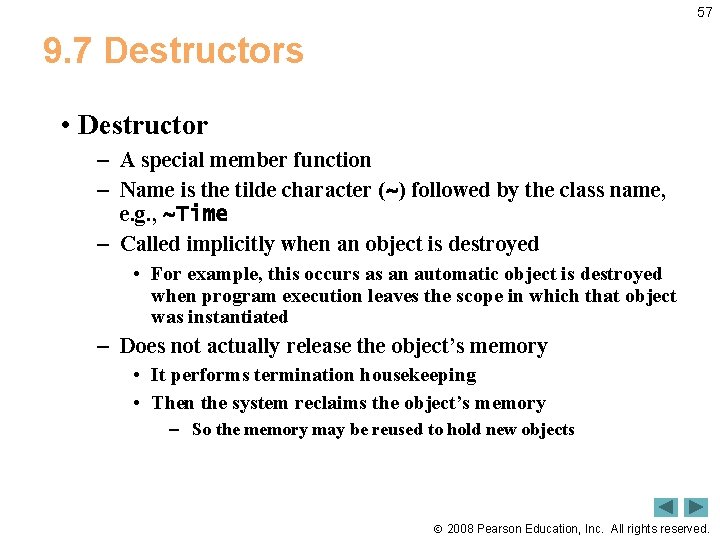
57 9. 7 Destructors • Destructor – A special member function – Name is the tilde character (~) followed by the class name, e. g. , ~Time – Called implicitly when an object is destroyed • For example, this occurs as an automatic object is destroyed when program execution leaves the scope in which that object was instantiated – Does not actually release the object’s memory • It performs termination housekeeping • Then the system reclaims the object’s memory – So the memory may be reused to hold new objects 2008 Pearson Education, Inc. All rights reserved.
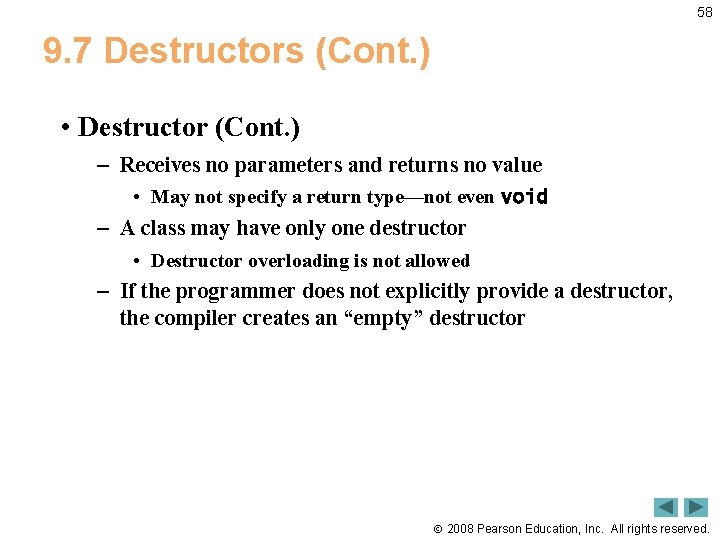
58 9. 7 Destructors (Cont. ) • Destructor (Cont. ) – Receives no parameters and returns no value • May not specify a return type—not even void – A class may have only one destructor • Destructor overloading is not allowed – If the programmer does not explicitly provide a destructor, the compiler creates an “empty” destructor 2008 Pearson Education, Inc. All rights reserved.
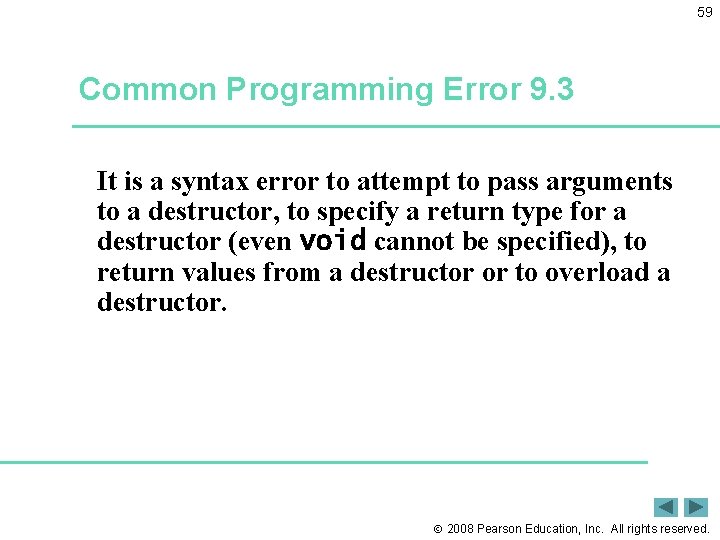
59 Common Programming Error 9. 3 It is a syntax error to attempt to pass arguments to a destructor, to specify a return type for a destructor (even void cannot be specified), to return values from a destructor or to overload a destructor. 2008 Pearson Education, Inc. All rights reserved.
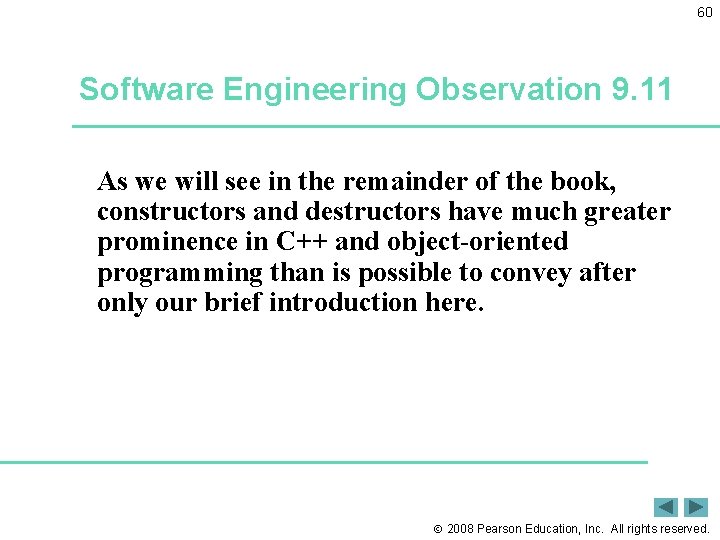
60 Software Engineering Observation 9. 11 As we will see in the remainder of the book, constructors and destructors have much greater prominence in C++ and object-oriented programming than is possible to convey after only our brief introduction here. 2008 Pearson Education, Inc. All rights reserved.
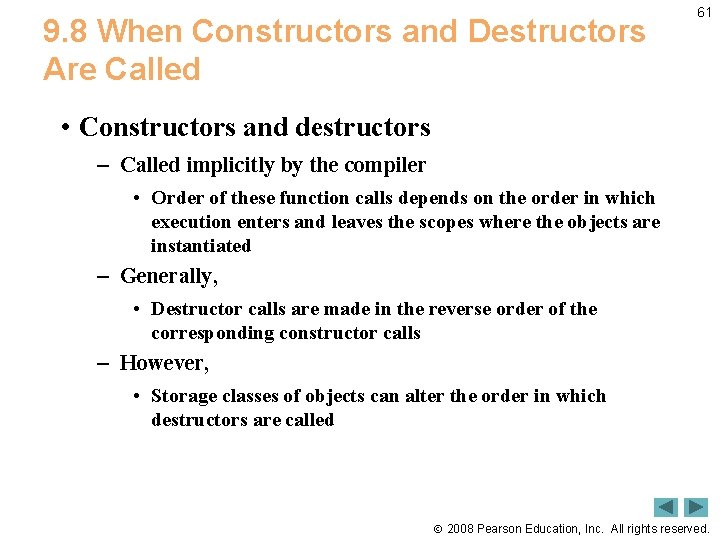
9. 8 When Constructors and Destructors Are Called 61 • Constructors and destructors – Called implicitly by the compiler • Order of these function calls depends on the order in which execution enters and leaves the scopes where the objects are instantiated – Generally, • Destructor calls are made in the reverse order of the corresponding constructor calls – However, • Storage classes of objects can alter the order in which destructors are called 2008 Pearson Education, Inc. All rights reserved.
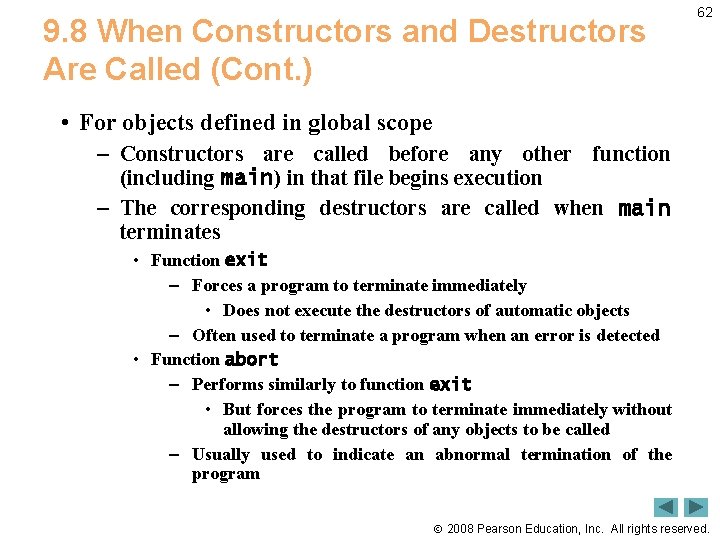
9. 8 When Constructors and Destructors Are Called (Cont. ) 62 • For objects defined in global scope – Constructors are called before any other function (including main) in that file begins execution – The corresponding destructors are called when main terminates • Function exit – Forces a program to terminate immediately • Does not execute the destructors of automatic objects – Often used to terminate a program when an error is detected • Function abort – Performs similarly to function exit • But forces the program to terminate immediately without allowing the destructors of any objects to be called – Usually used to indicate an abnormal termination of the program 2008 Pearson Education, Inc. All rights reserved.
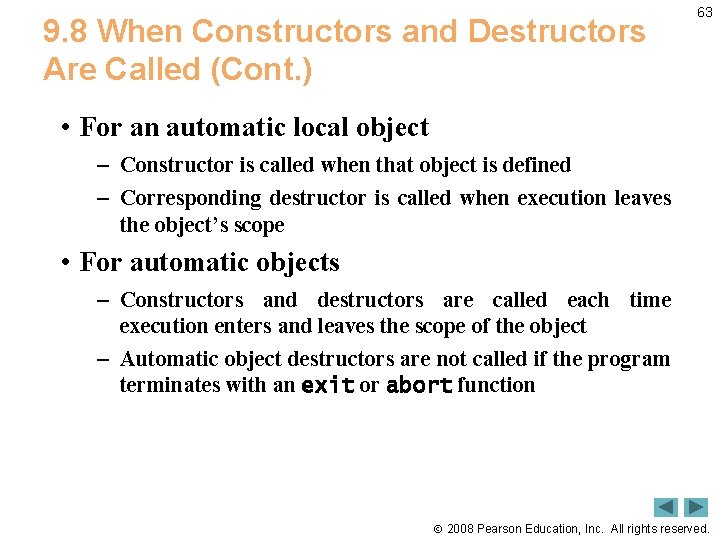
9. 8 When Constructors and Destructors Are Called (Cont. ) 63 • For an automatic local object – Constructor is called when that object is defined – Corresponding destructor is called when execution leaves the object’s scope • For automatic objects – Constructors and destructors are called each time execution enters and leaves the scope of the object – Automatic object destructors are not called if the program terminates with an exit or abort function 2008 Pearson Education, Inc. All rights reserved.
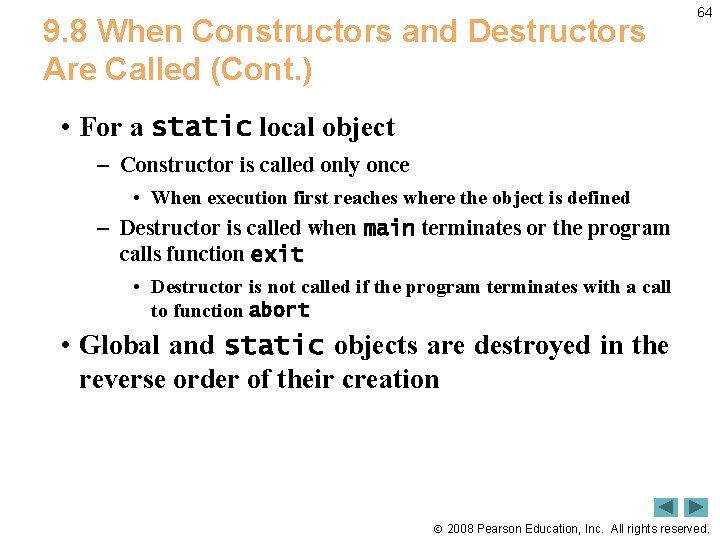
9. 8 When Constructors and Destructors Are Called (Cont. ) 64 • For a static local object – Constructor is called only once • When execution first reaches where the object is defined – Destructor is called when main terminates or the program calls function exit • Destructor is not called if the program terminates with a call to function abort • Global and static objects are destroyed in the reverse order of their creation 2008 Pearson Education, Inc. All rights reserved.
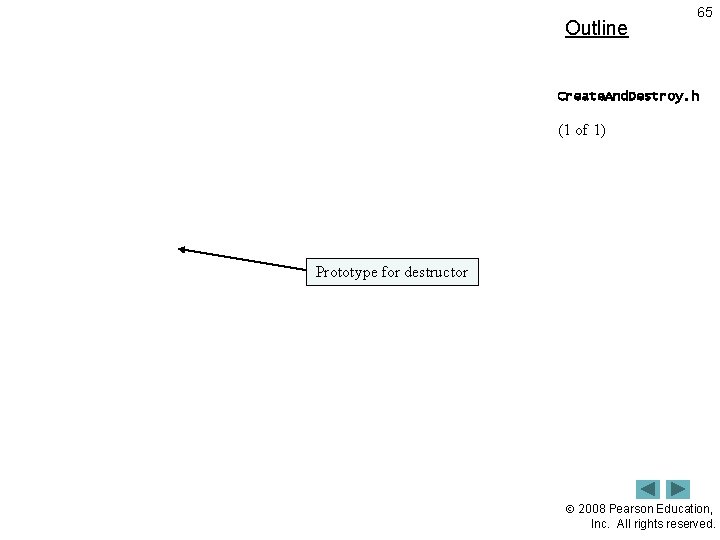
Outline 65 Create. And. Destroy. h (1 of 1) Prototype for destructor 2008 Pearson Education, Inc. All rights reserved.
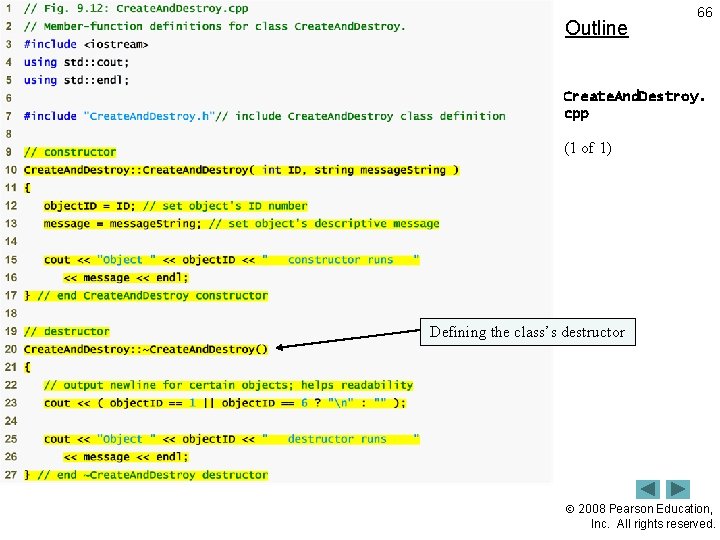
Outline 66 Create. And. Destroy. cpp (1 of 1) Defining the class’s destructor 2008 Pearson Education, Inc. All rights reserved.
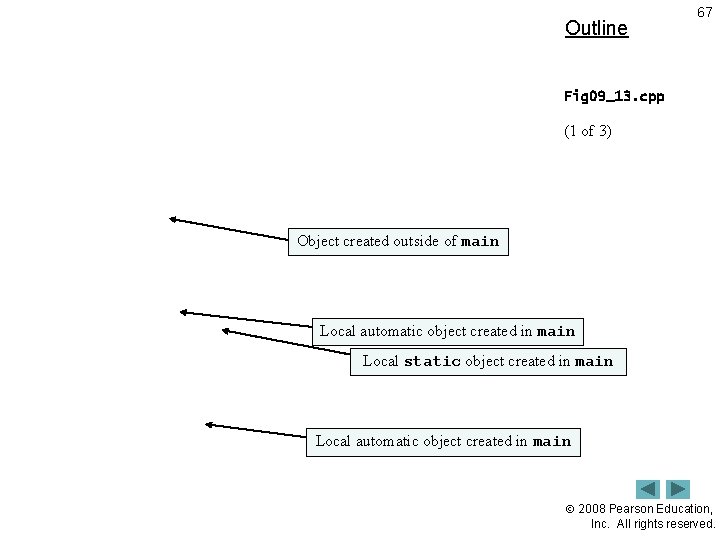
Outline 67 Fig 09_13. cpp (1 of 3) Object created outside of main Local automatic object created in main Local static object created in main Local automatic object created in main 2008 Pearson Education, Inc. All rights reserved.
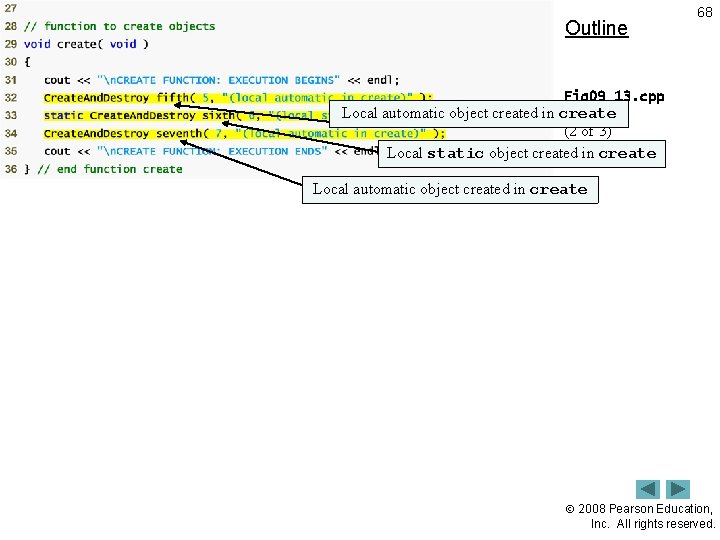
Outline 68 Fig 09_13. cpp Local automatic object created in create (2 of 3) Local static object created in create Local automatic object created in create 2008 Pearson Education, Inc. All rights reserved.
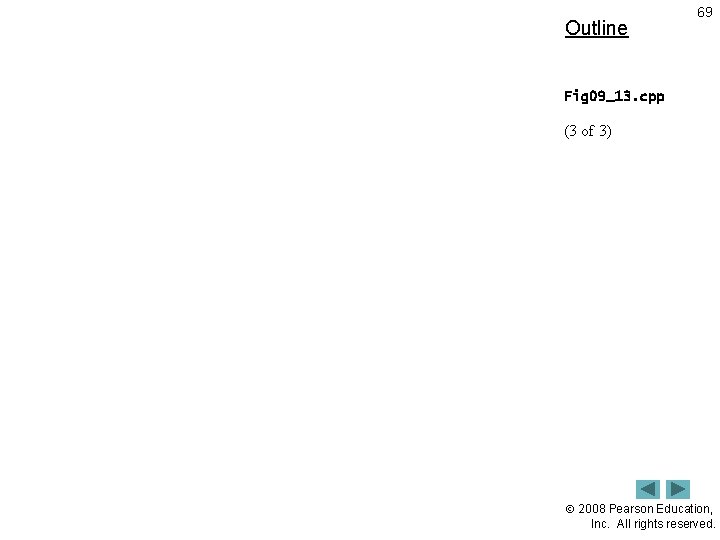
Outline 69 Fig 09_13. cpp (3 of 3) 2008 Pearson Education, Inc. All rights reserved.
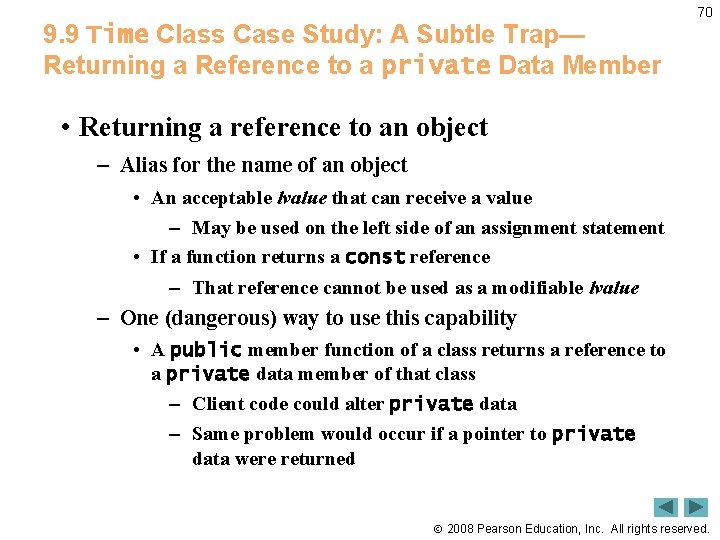
9. 9 Time Class Case Study: A Subtle Trap— Returning a Reference to a private Data Member 70 • Returning a reference to an object – Alias for the name of an object • An acceptable lvalue that can receive a value – May be used on the left side of an assignment statement • If a function returns a const reference – That reference cannot be used as a modifiable lvalue – One (dangerous) way to use this capability • A public member function of a class returns a reference to a private data member of that class – Client code could alter private data – Same problem would occur if a pointer to private data were returned 2008 Pearson Education, Inc. All rights reserved.
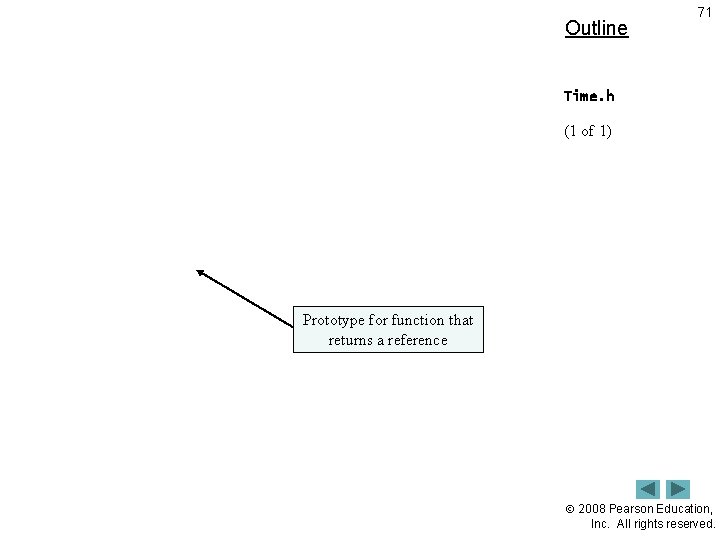
Outline 71 Time. h (1 of 1) Prototype for function that returns a reference 2008 Pearson Education, Inc. All rights reserved.
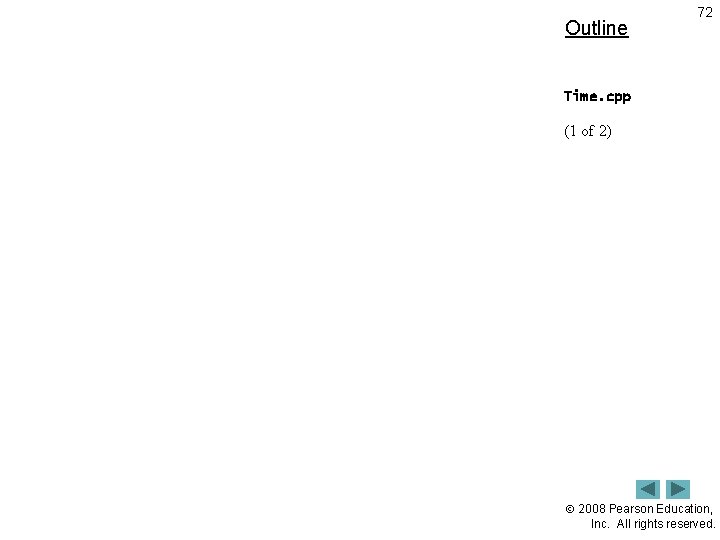
Outline 72 Time. cpp (1 of 2) 2008 Pearson Education, Inc. All rights reserved.
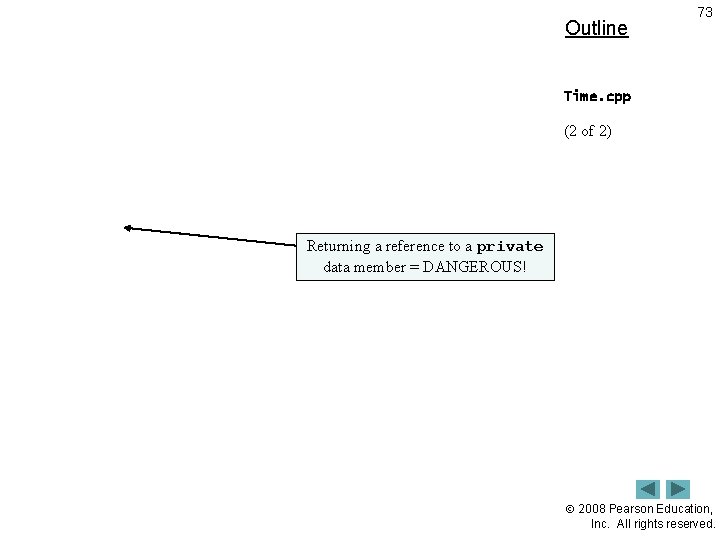
Outline 73 Time. cpp (2 of 2) Returning a reference to a private data member = DANGEROUS! 2008 Pearson Education, Inc. All rights reserved.
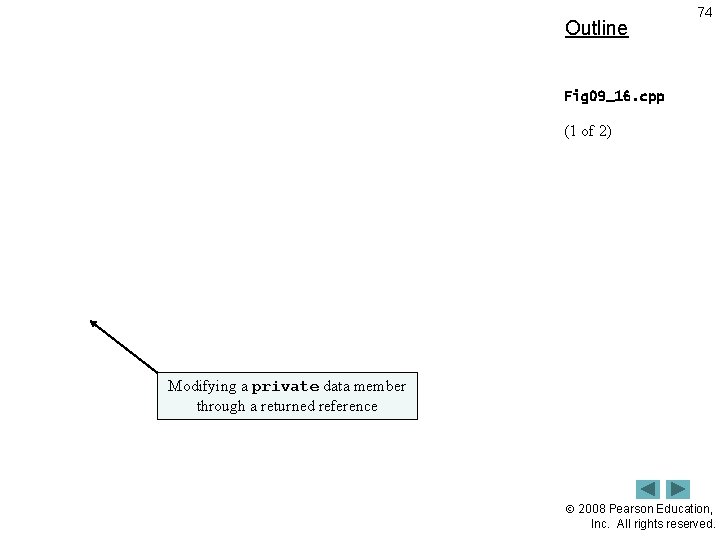
Outline 74 Fig 09_16. cpp (1 of 2) Modifying a private data member through a returned reference 2008 Pearson Education, Inc. All rights reserved.
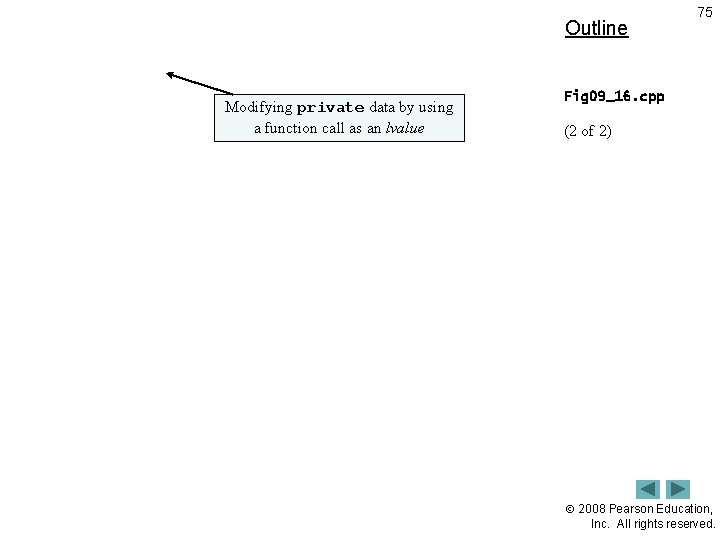
Outline Modifying private data by using a function call as an lvalue 75 Fig 09_16. cpp (2 of 2) 2008 Pearson Education, Inc. All rights reserved.
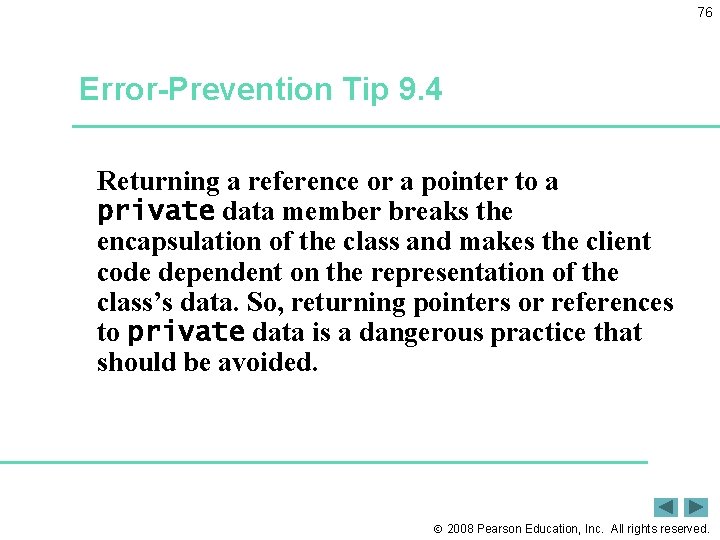
76 Error-Prevention Tip 9. 4 Returning a reference or a pointer to a private data member breaks the encapsulation of the class and makes the client code dependent on the representation of the class’s data. So, returning pointers or references to private data is a dangerous practice that should be avoided. 2008 Pearson Education, Inc. All rights reserved.
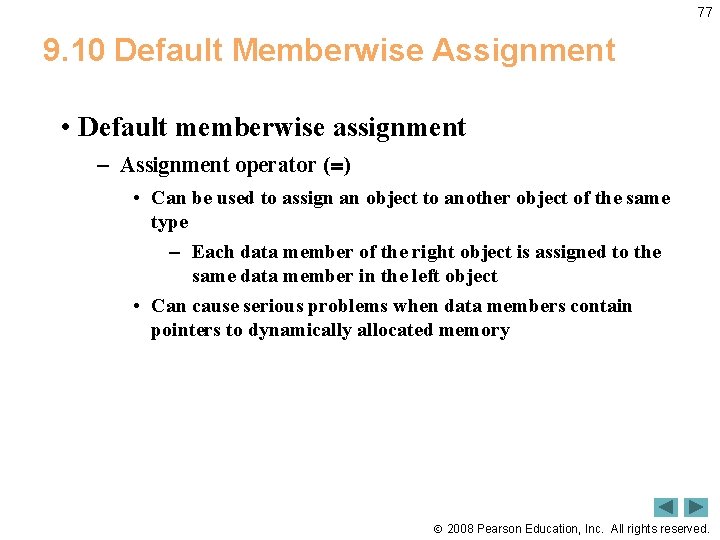
77 9. 10 Default Memberwise Assignment • Default memberwise assignment – Assignment operator (=) • Can be used to assign an object to another object of the same type – Each data member of the right object is assigned to the same data member in the left object • Can cause serious problems when data members contain pointers to dynamically allocated memory 2008 Pearson Education, Inc. All rights reserved.
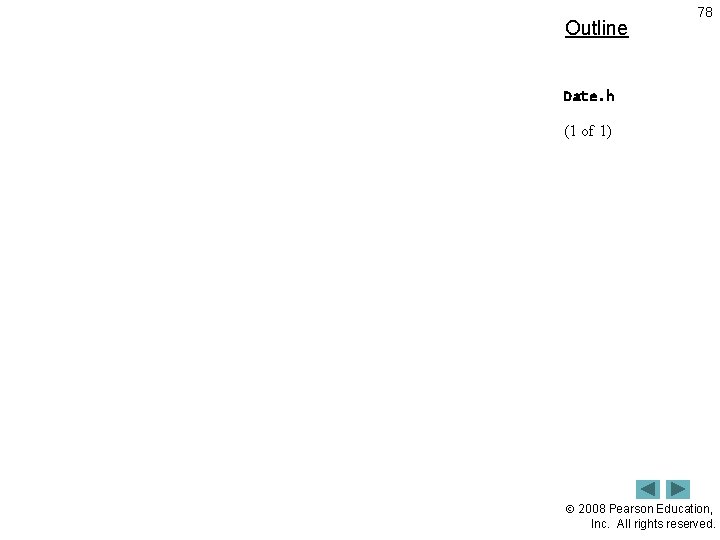
Outline 78 Date. h (1 of 1) 2008 Pearson Education, Inc. All rights reserved.
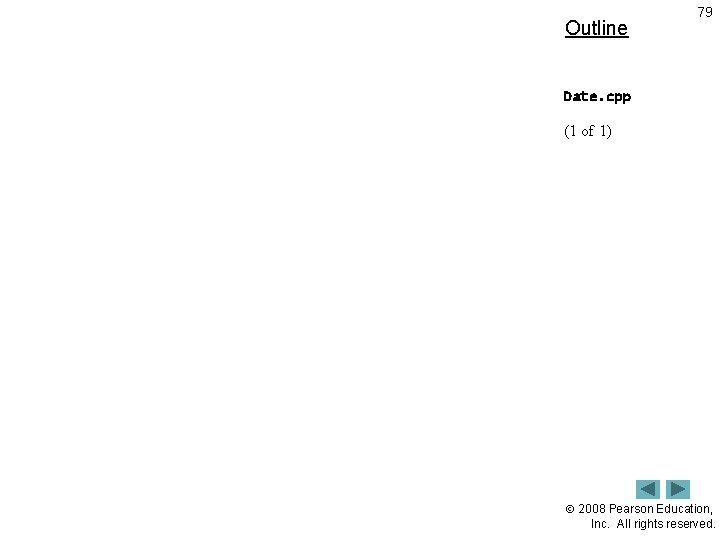
Outline 79 Date. cpp (1 of 1) 2008 Pearson Education, Inc. All rights reserved.
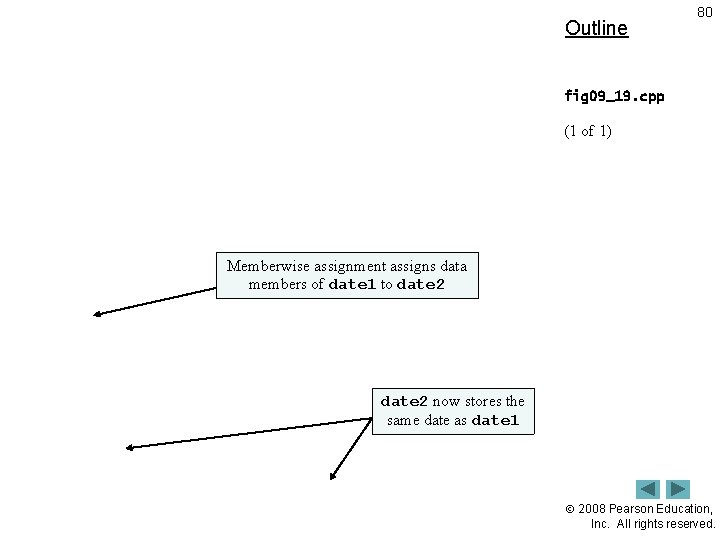
Outline 80 fig 09_19. cpp (1 of 1) Memberwise assignment assigns data members of date 1 to date 2 now stores the same date as date 1 2008 Pearson Education, Inc. All rights reserved.
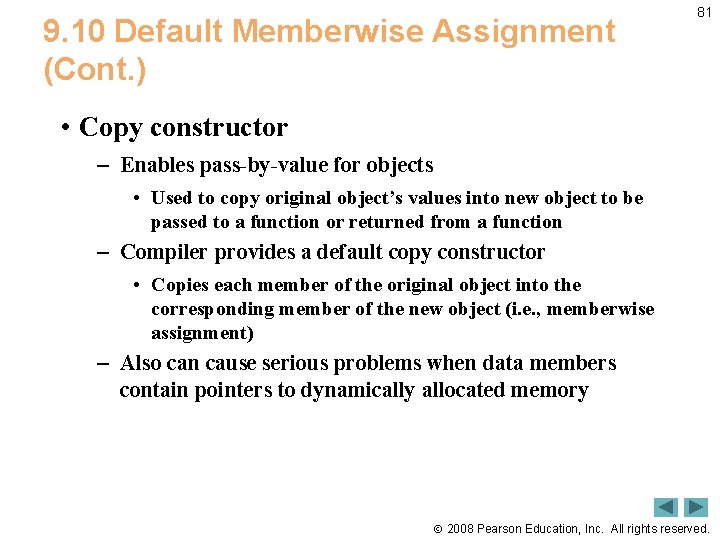
9. 10 Default Memberwise Assignment (Cont. ) 81 • Copy constructor – Enables pass-by-value for objects • Used to copy original object’s values into new object to be passed to a function or returned from a function – Compiler provides a default copy constructor • Copies each member of the original object into the corresponding member of the new object (i. e. , memberwise assignment) – Also can cause serious problems when data members contain pointers to dynamically allocated memory 2008 Pearson Education, Inc. All rights reserved.
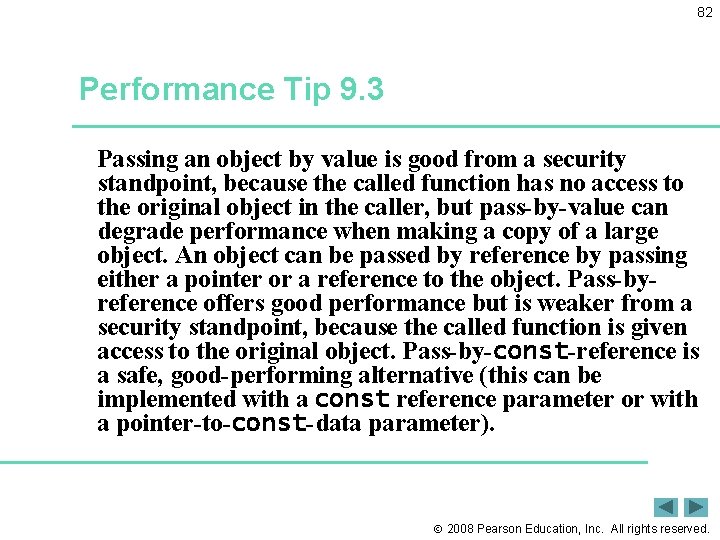
82 Performance Tip 9. 3 Passing an object by value is good from a security standpoint, because the called function has no access to the original object in the caller, but pass-by-value can degrade performance when making a copy of a large object. An object can be passed by reference by passing either a pointer or a reference to the object. Pass-byreference offers good performance but is weaker from a security standpoint, because the called function is given access to the original object. Pass-by-const-reference is a safe, good-performing alternative (this can be implemented with a const reference parameter or with a pointer-to-const-data parameter). 2008 Pearson Education, Inc. All rights reserved.
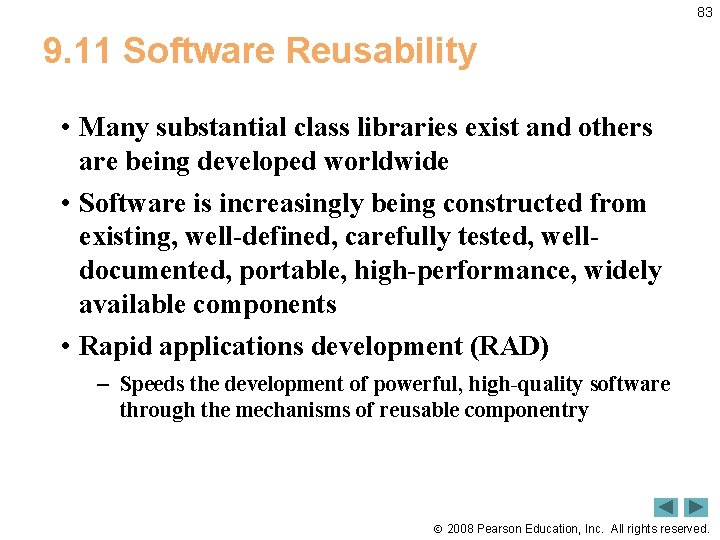
83 9. 11 Software Reusability • Many substantial class libraries exist and others are being developed worldwide • Software is increasingly being constructed from existing, well-defined, carefully tested, welldocumented, portable, high-performance, widely available components • Rapid applications development (RAD) – Speeds the development of powerful, high-quality software through the mechanisms of reusable componentry 2008 Pearson Education, Inc. All rights reserved.
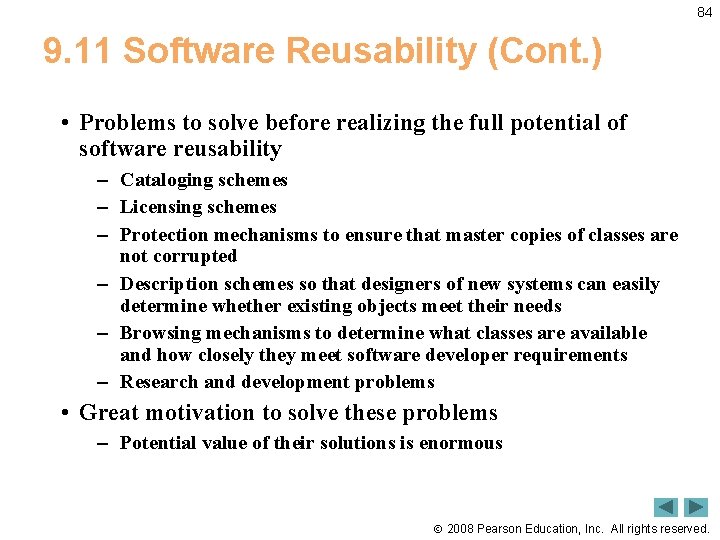
84 9. 11 Software Reusability (Cont. ) • Problems to solve before realizing the full potential of software reusability – Cataloging schemes – Licensing schemes – Protection mechanisms to ensure that master copies of classes are not corrupted – Description schemes so that designers of new systems can easily determine whether existing objects meet their needs – Browsing mechanisms to determine what classes are available and how closely they meet software developer requirements – Research and development problems • Great motivation to solve these problems – Potential value of their solutions is enormous 2008 Pearson Education, Inc. All rights reserved.
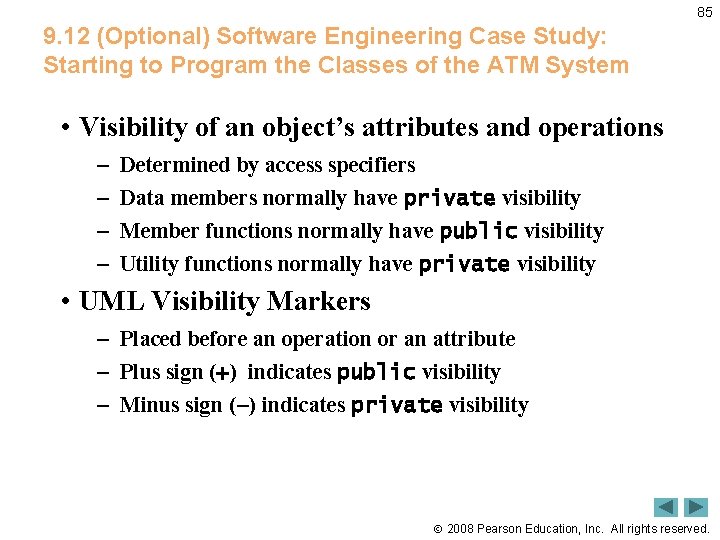
85 9. 12 (Optional) Software Engineering Case Study: Starting to Program the Classes of the ATM System • Visibility of an object’s attributes and operations – – Determined by access specifiers Data members normally have private visibility Member functions normally have public visibility Utility functions normally have private visibility • UML Visibility Markers – Placed before an operation or an attribute – Plus sign (+) indicates public visibility – Minus sign (–) indicates private visibility 2008 Pearson Education, Inc. All rights reserved.
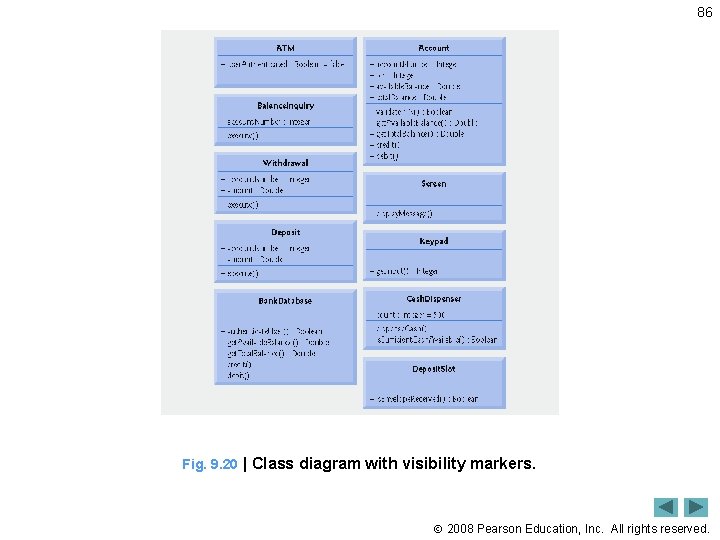
86 Fig. 9. 20 | Class diagram with visibility markers. 2008 Pearson Education, Inc. All rights reserved.
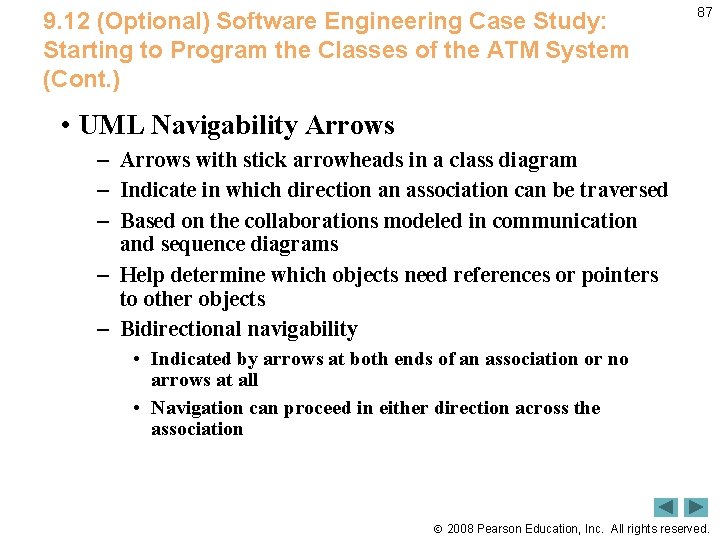
9. 12 (Optional) Software Engineering Case Study: Starting to Program the Classes of the ATM System (Cont. ) 87 • UML Navigability Arrows – Arrows with stick arrowheads in a class diagram – Indicate in which direction an association can be traversed – Based on the collaborations modeled in communication and sequence diagrams – Help determine which objects need references or pointers to other objects – Bidirectional navigability • Indicated by arrows at both ends of an association or no arrows at all • Navigation can proceed in either direction across the association 2008 Pearson Education, Inc. All rights reserved.
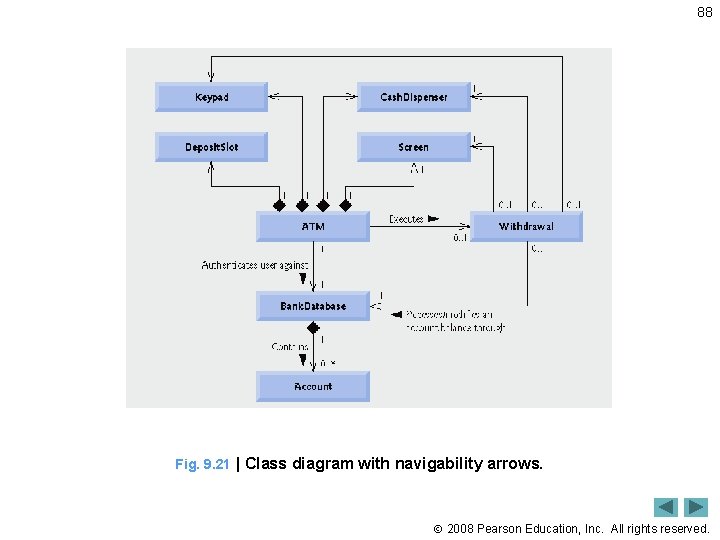
88 Fig. 9. 21 | Class diagram with navigability arrows. 2008 Pearson Education, Inc. All rights reserved.
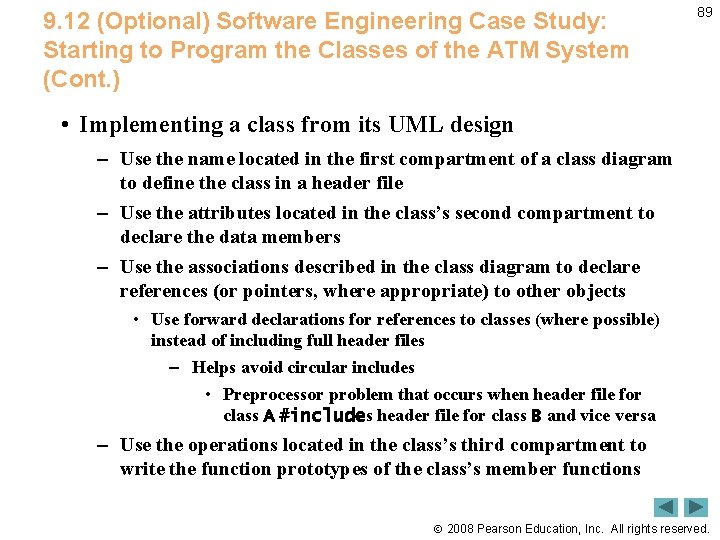
9. 12 (Optional) Software Engineering Case Study: Starting to Program the Classes of the ATM System (Cont. ) 89 • Implementing a class from its UML design – Use the name located in the first compartment of a class diagram to define the class in a header file – Use the attributes located in the class’s second compartment to declare the data members – Use the associations described in the class diagram to declare references (or pointers, where appropriate) to other objects • Use forward declarations for references to classes (where possible) instead of including full header files – Helps avoid circular includes • Preprocessor problem that occurs when header file for class A #includes header file for class B and vice versa – Use the operations located in the class’s third compartment to write the function prototypes of the class’s member functions 2008 Pearson Education, Inc. All rights reserved.
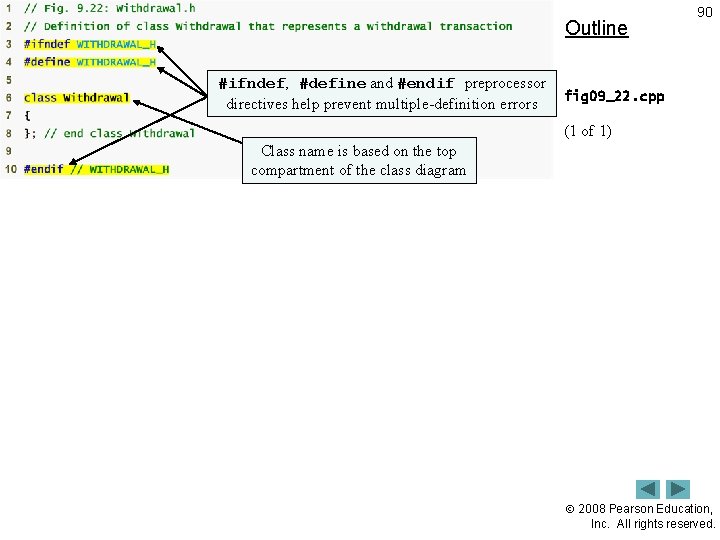
Outline #ifndef, #define and #endif preprocessor directives help prevent multiple-definition errors 90 fig 09_22. cpp (1 of 1) Class name is based on the top compartment of the class diagram 2008 Pearson Education, Inc. All rights reserved.
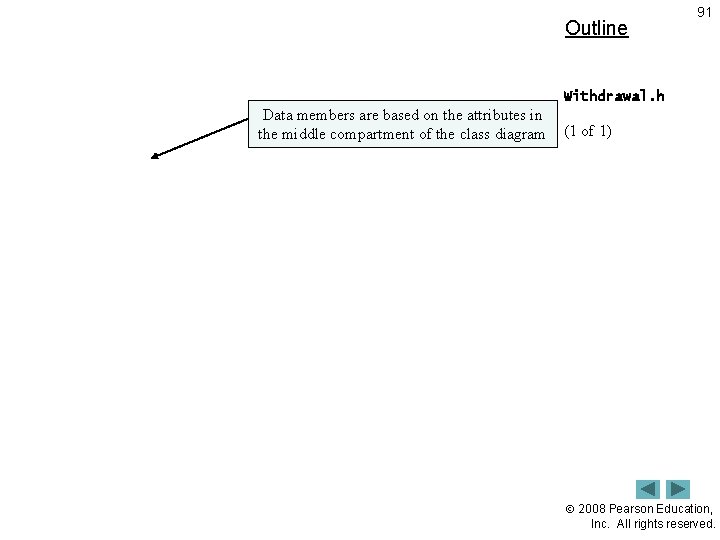
Outline 91 Withdrawal. h Data members are based on the attributes in the middle compartment of the class diagram (1 of 1) 2008 Pearson Education, Inc. All rights reserved.
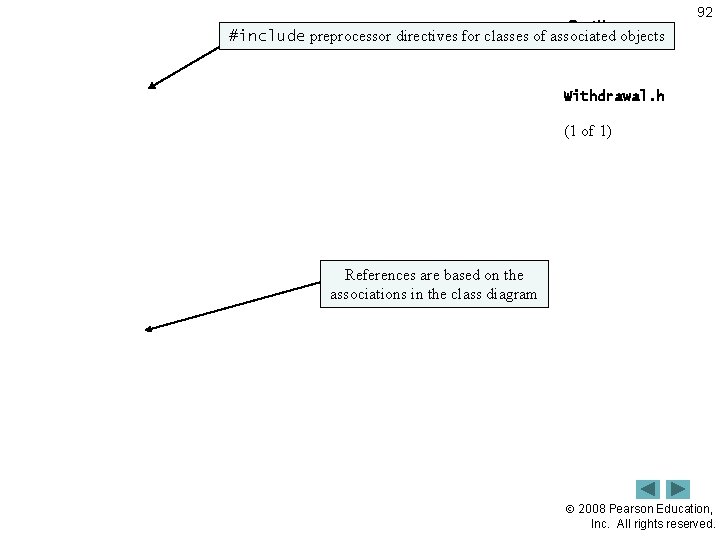
Outlineobjects #include preprocessor directives for classes of associated 92 Withdrawal. h (1 of 1) References are based on the associations in the class diagram 2008 Pearson Education, Inc. All rights reserved.
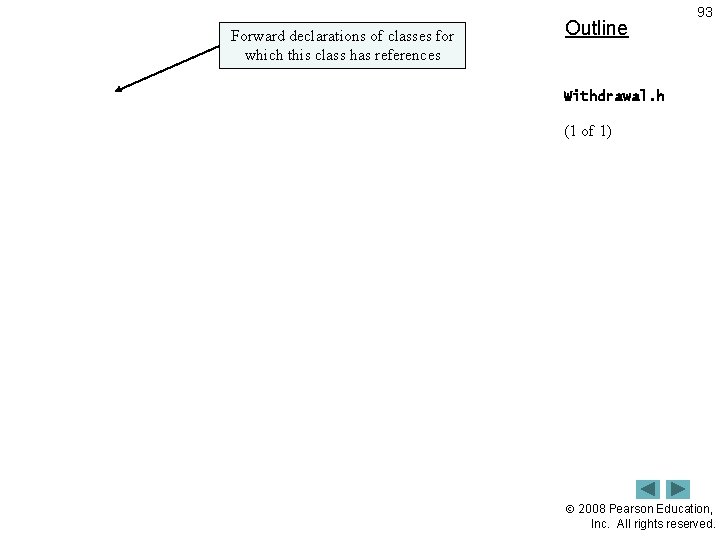
Forward declarations of classes for which this class has references Outline 93 Withdrawal. h (1 of 1) 2008 Pearson Education, Inc. All rights reserved.
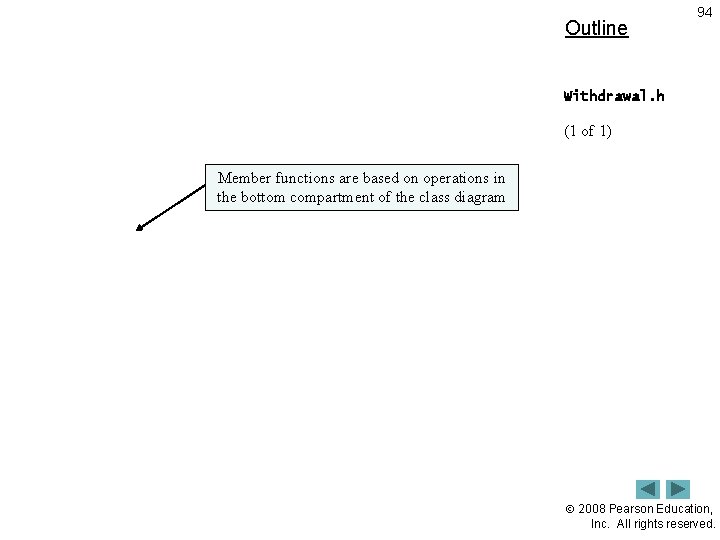
Outline 94 Withdrawal. h (1 of 1) Member functions are based on operations in the bottom compartment of the class diagram 2008 Pearson Education, Inc. All rights reserved.
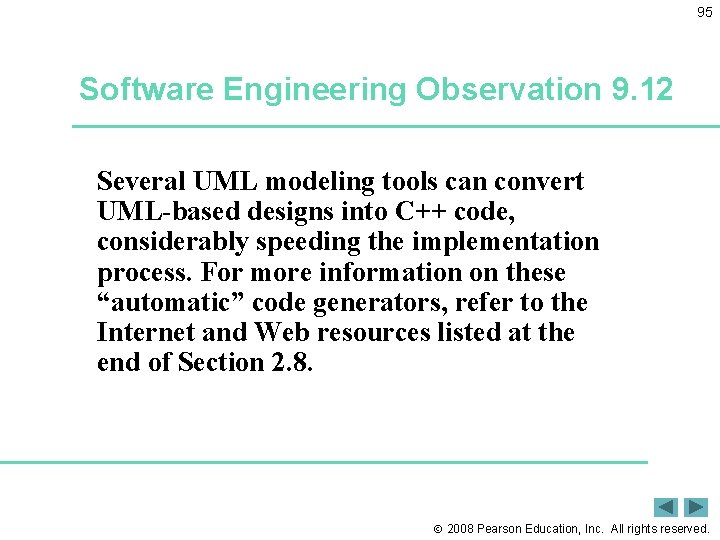
95 Software Engineering Observation 9. 12 Several UML modeling tools can convert UML-based designs into C++ code, considerably speeding the implementation process. For more information on these “automatic” code generators, refer to the Internet and Web resources listed at the end of Section 2. 8. 2008 Pearson Education, Inc. All rights reserved.
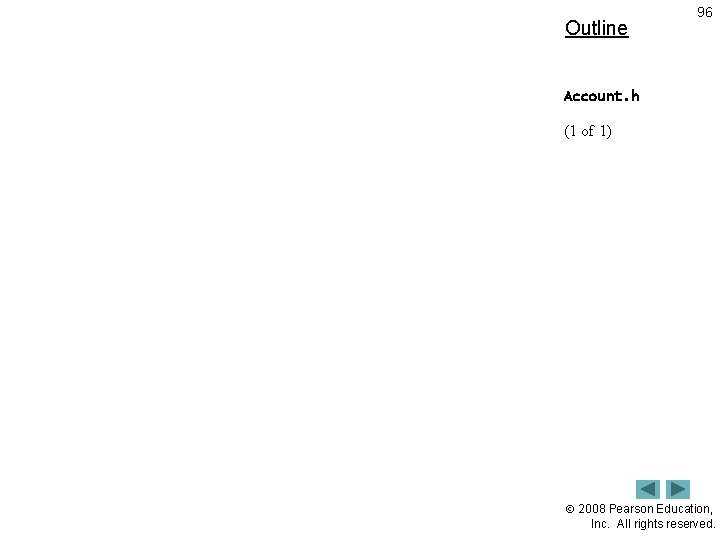
Outline 96 Account. h (1 of 1) 2008 Pearson Education, Inc. All rights reserved.