1 2 3 Fig 4 3 fig 0403
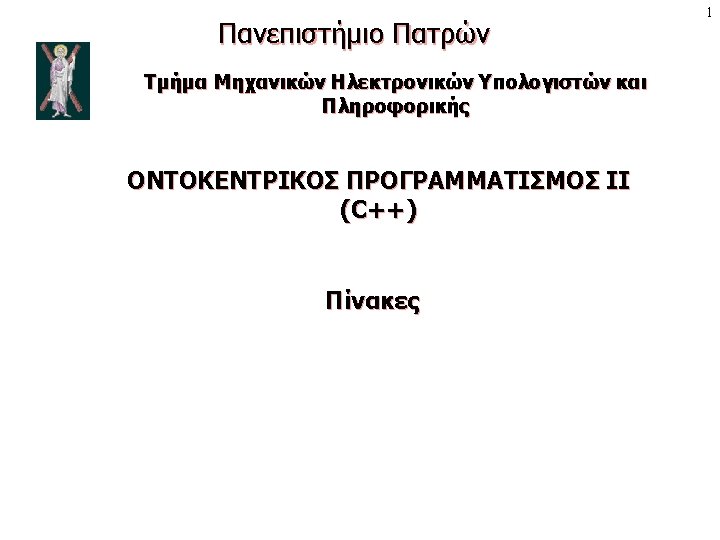
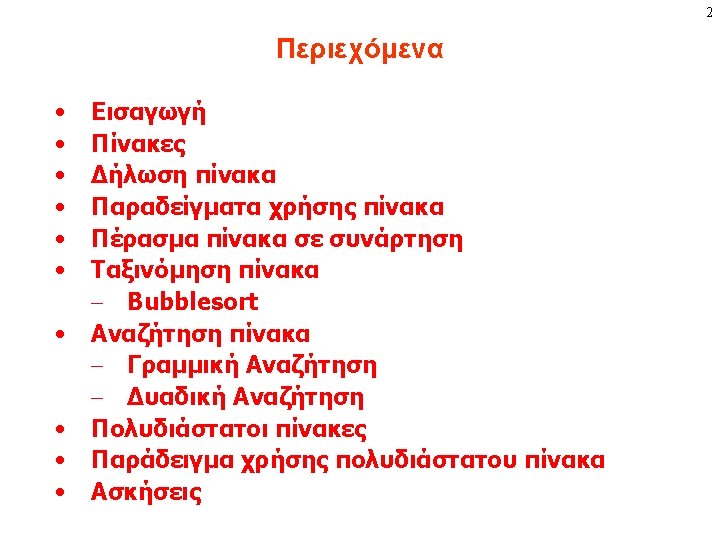
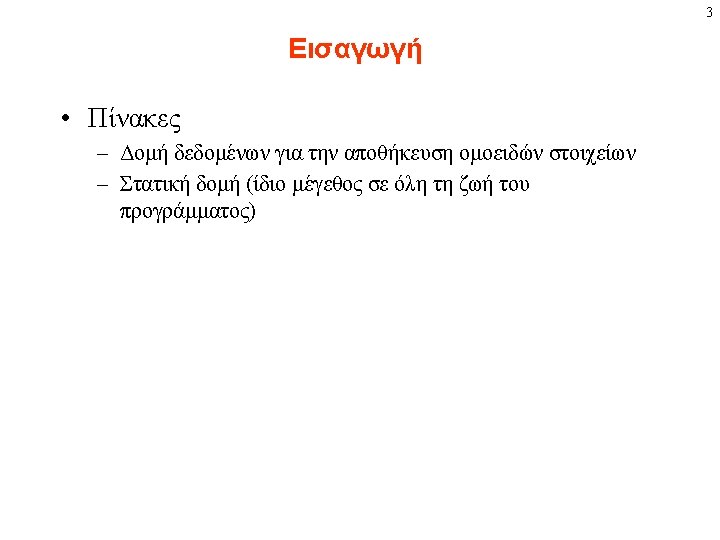
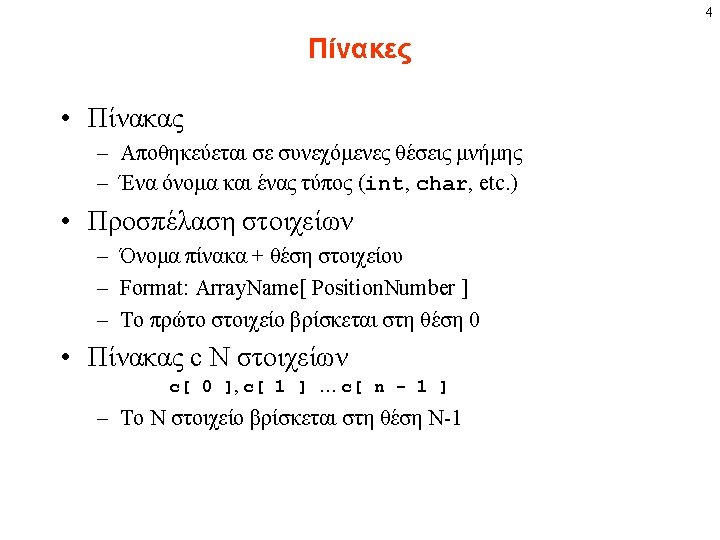
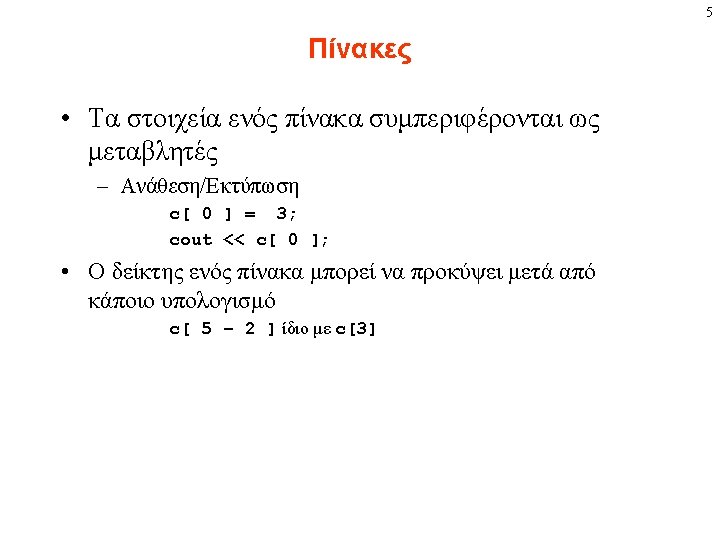
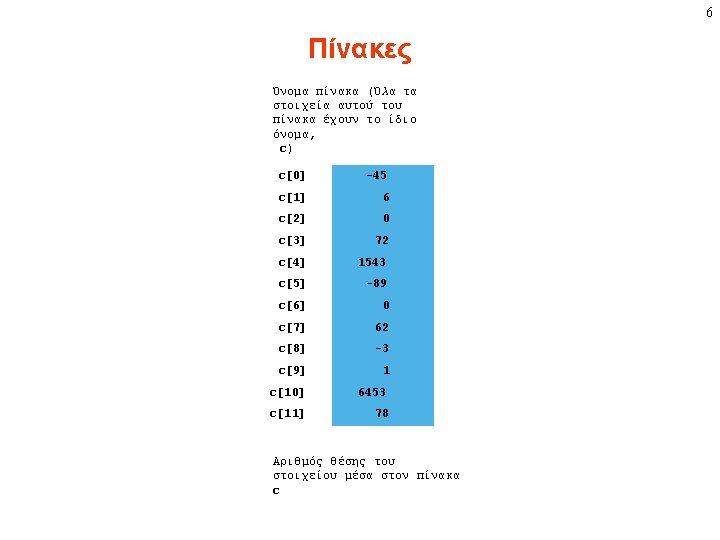
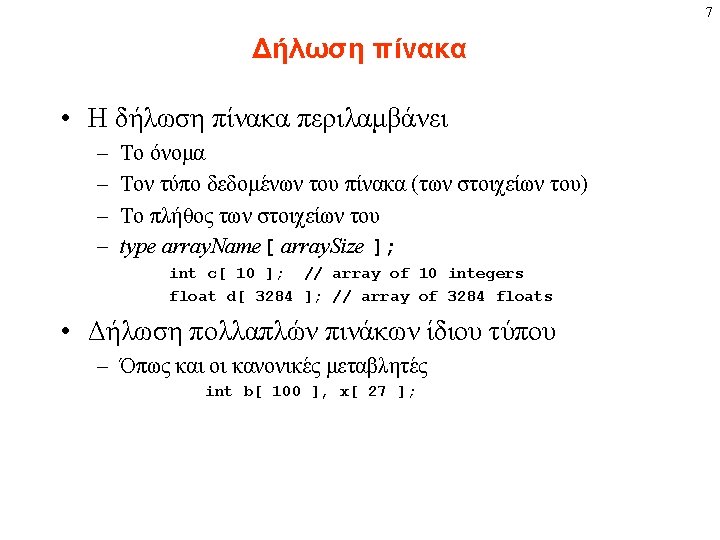
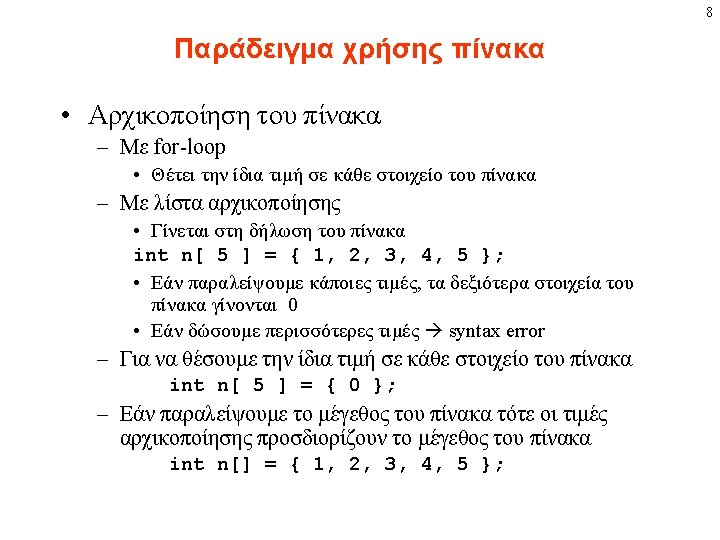
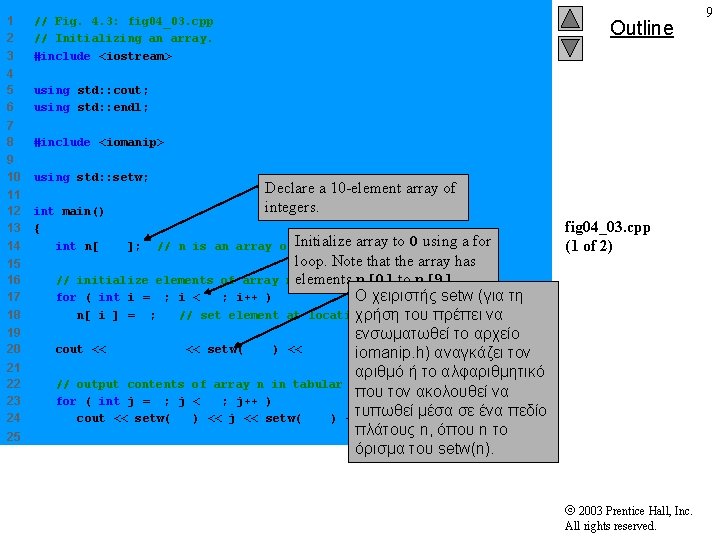
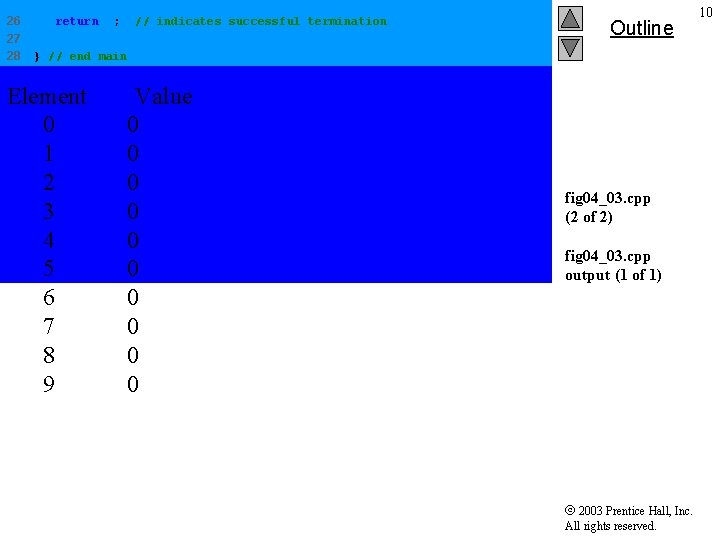
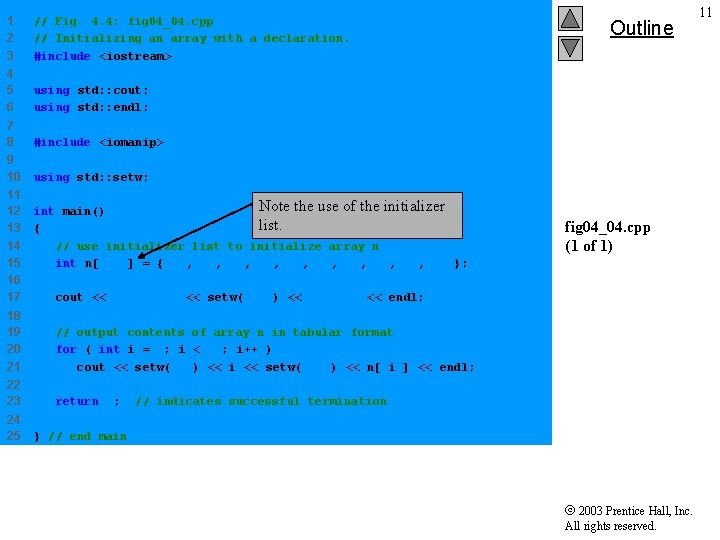
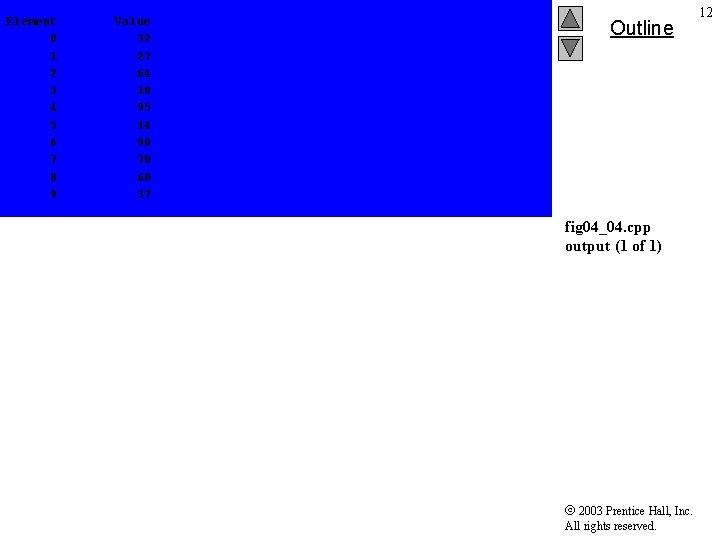
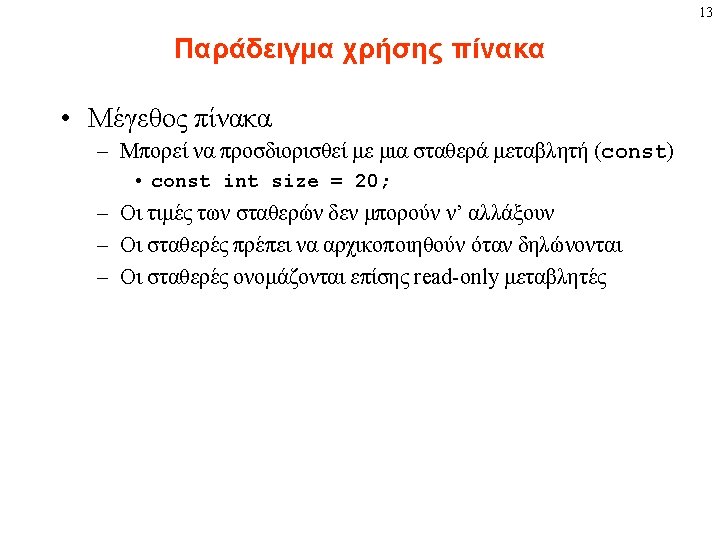
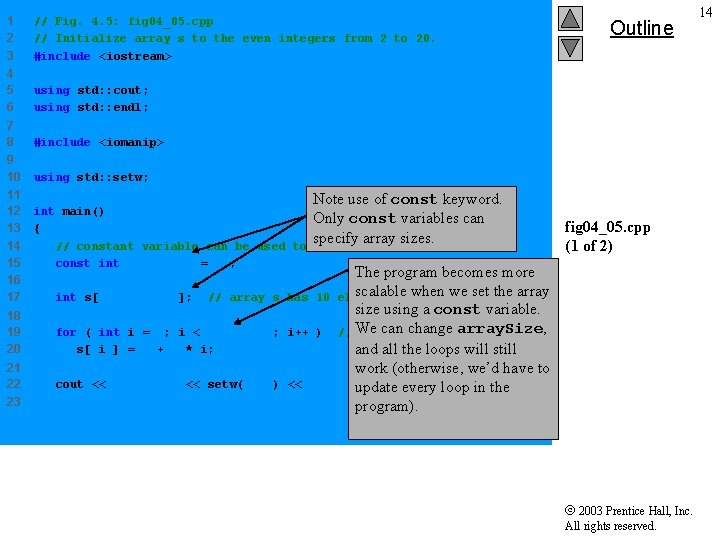
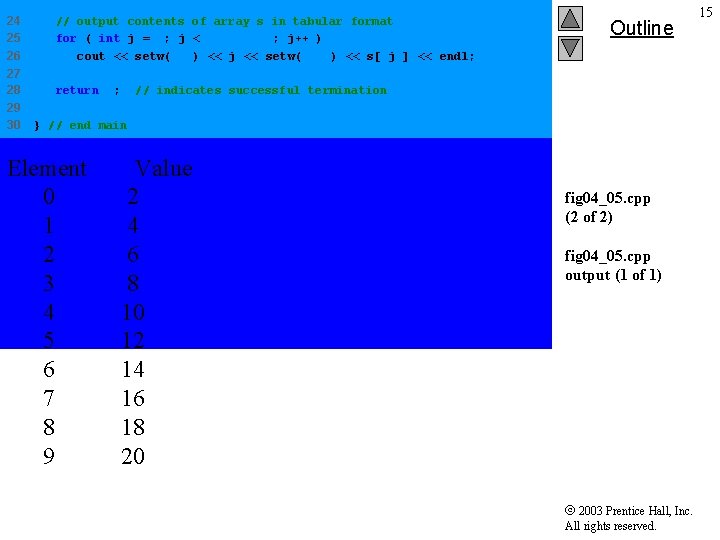
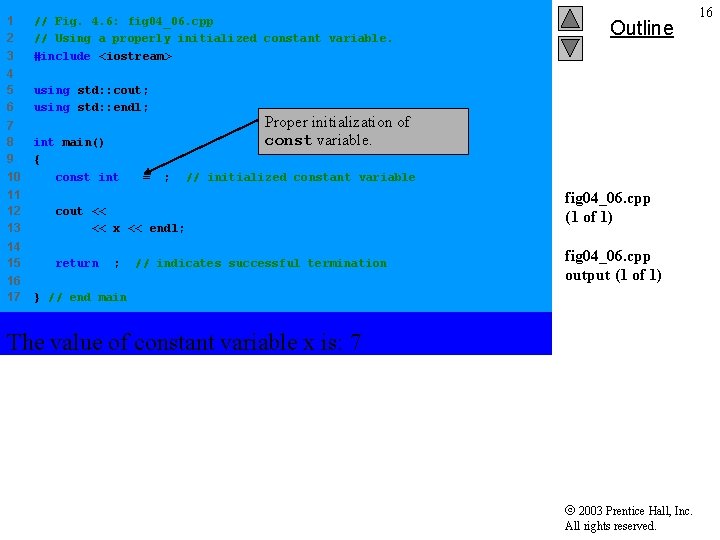
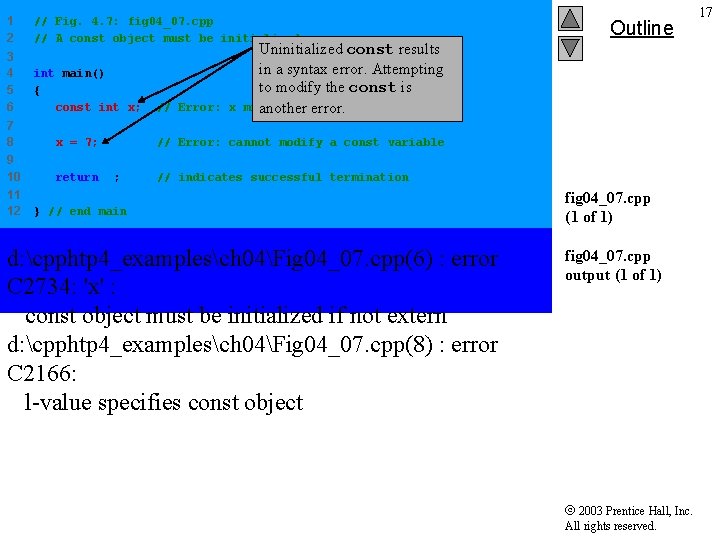
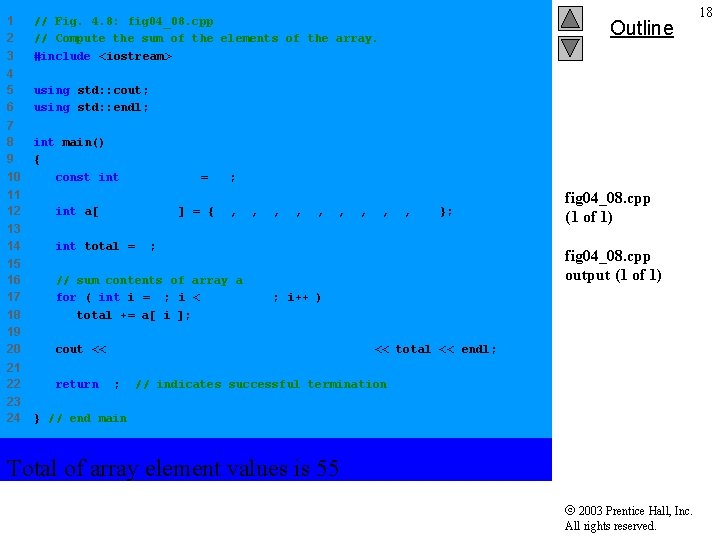
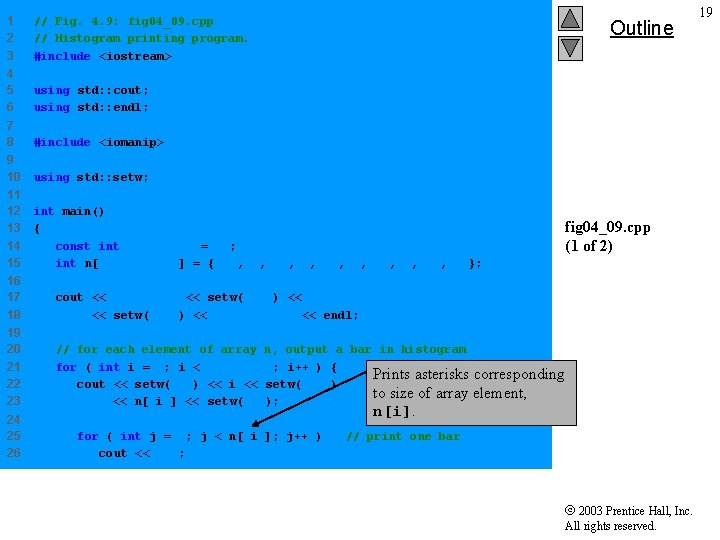
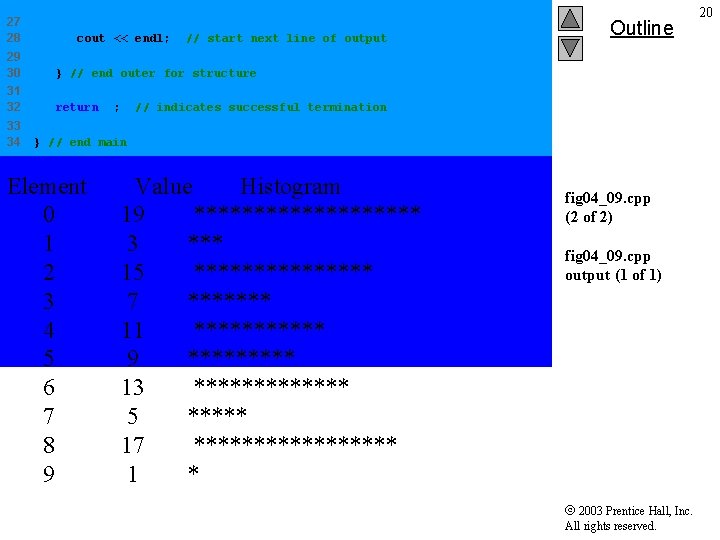
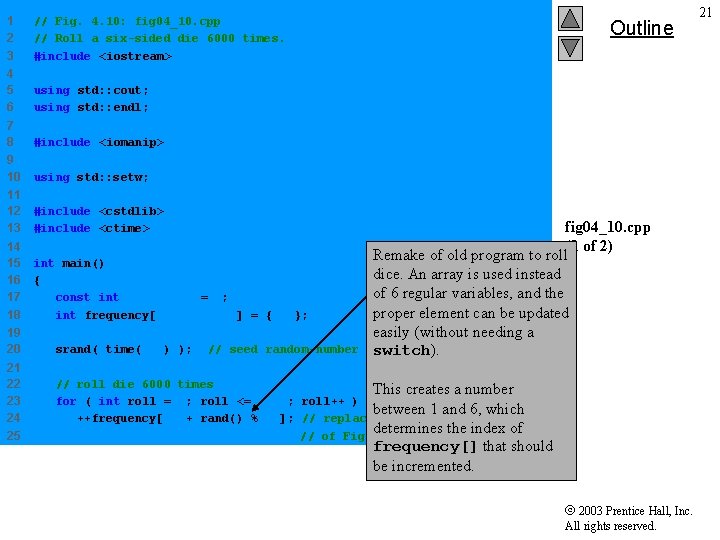
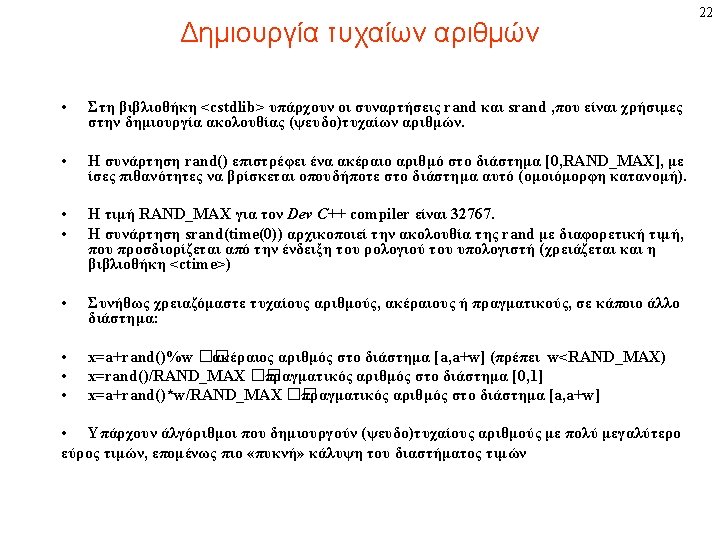
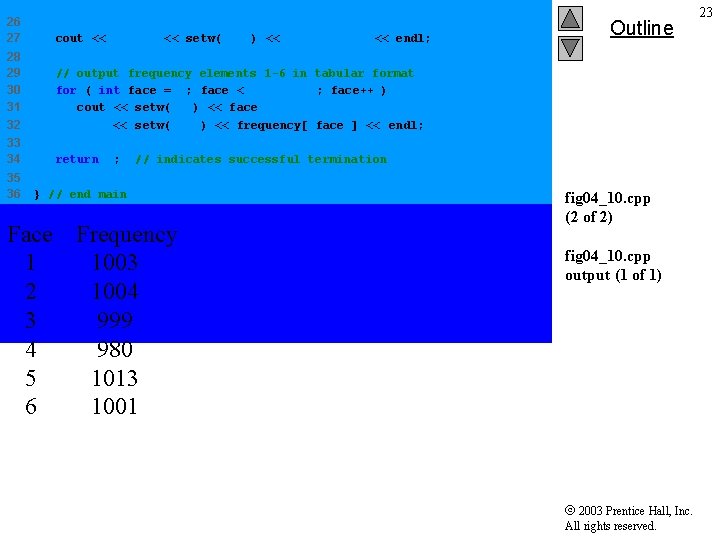
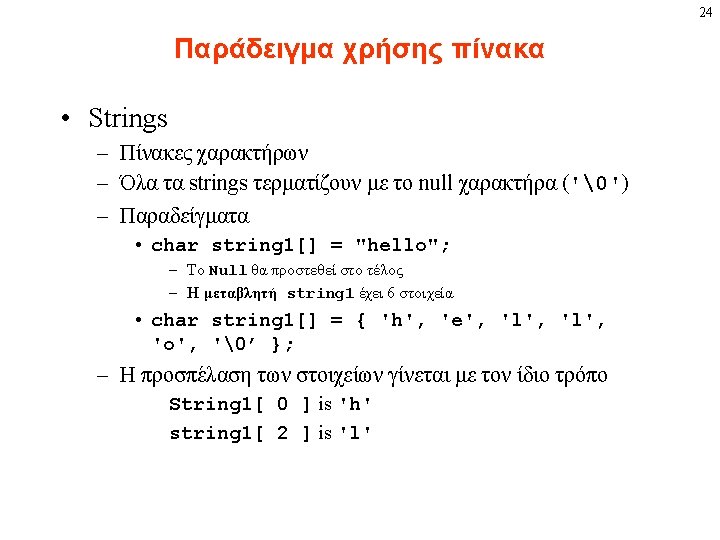
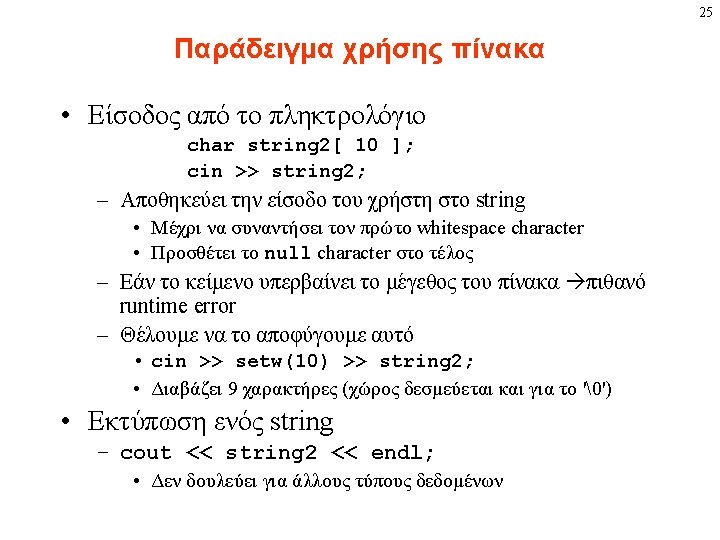
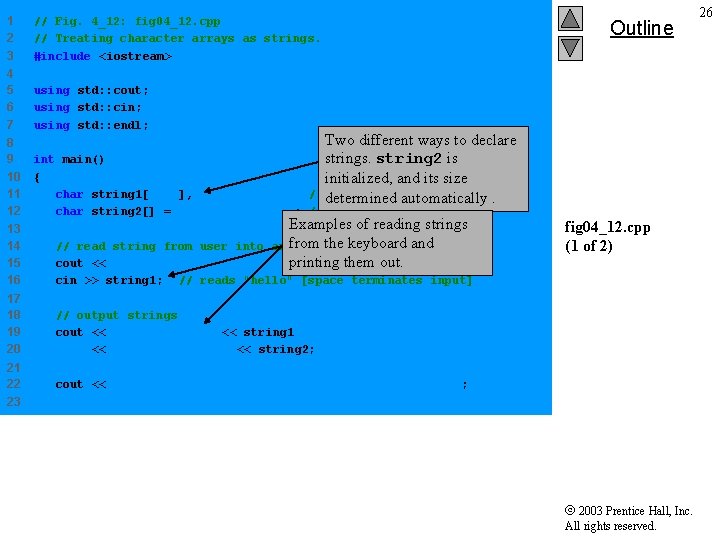
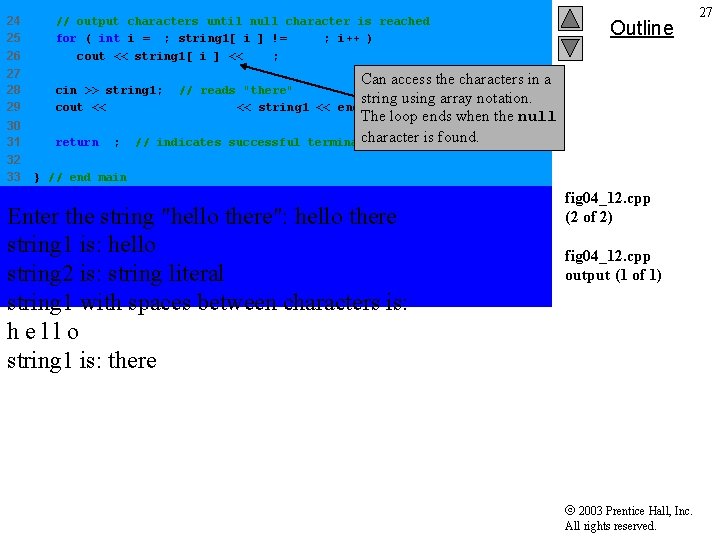
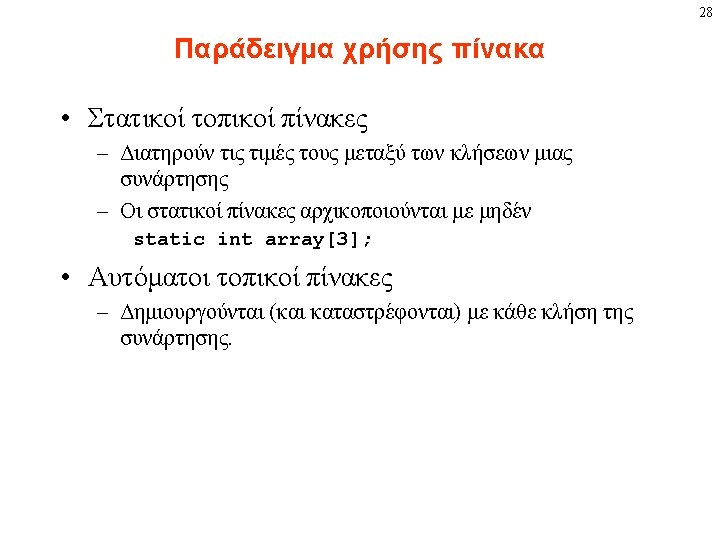
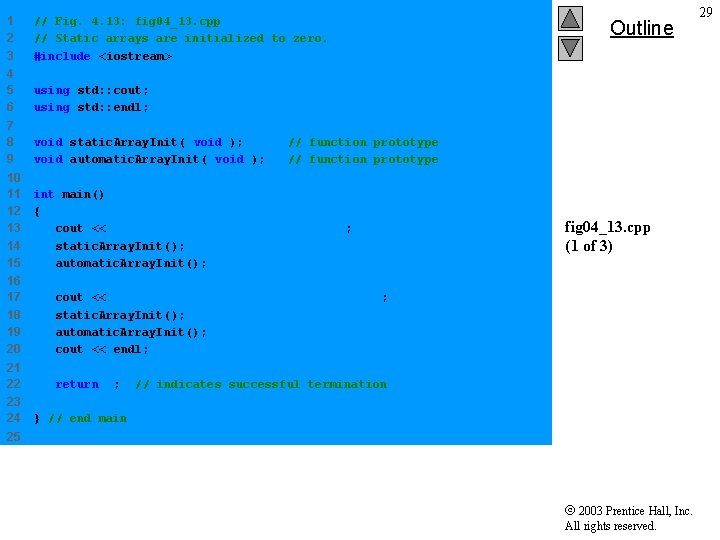
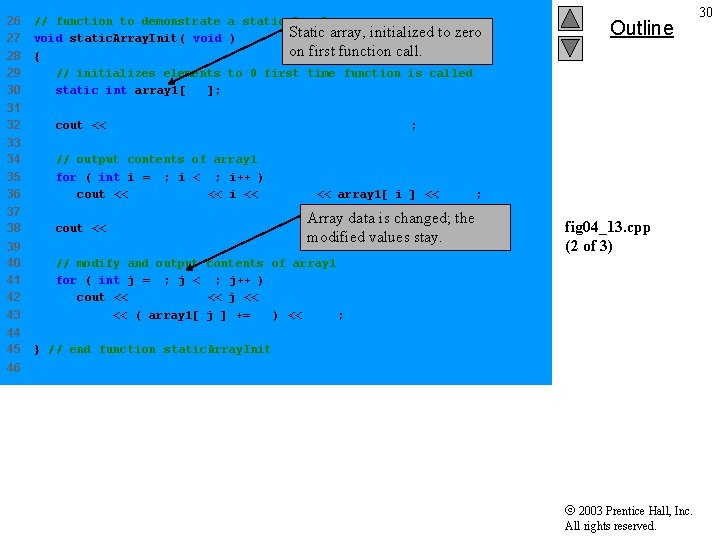
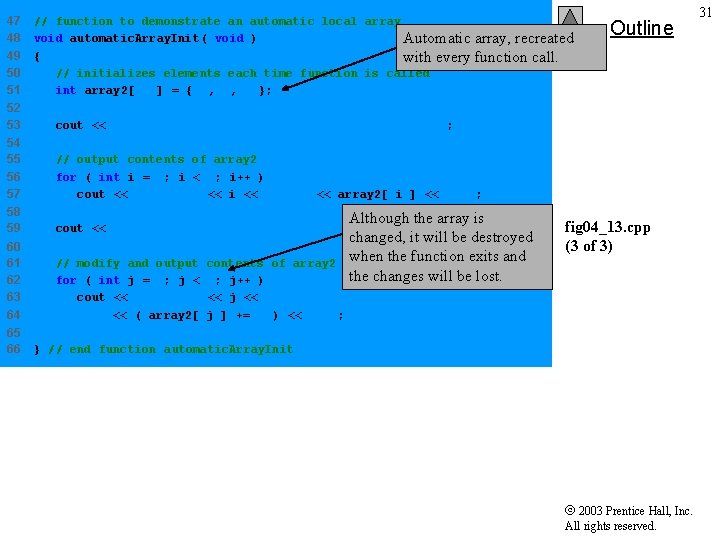
![First call to each function: Values on entering static. Array. Init: array 1[0] = First call to each function: Values on entering static. Array. Init: array 1[0] =](https://slidetodoc.com/presentation_image_h/30709c6f2f777c7d47f52e1a25567873/image-32.jpg)
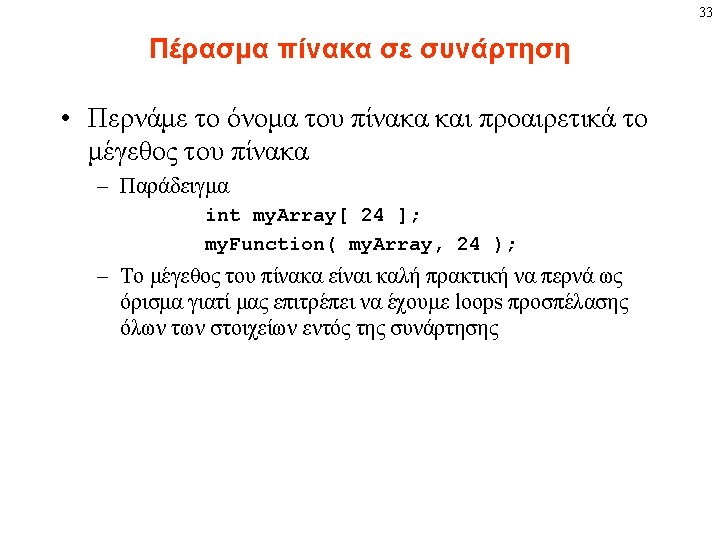
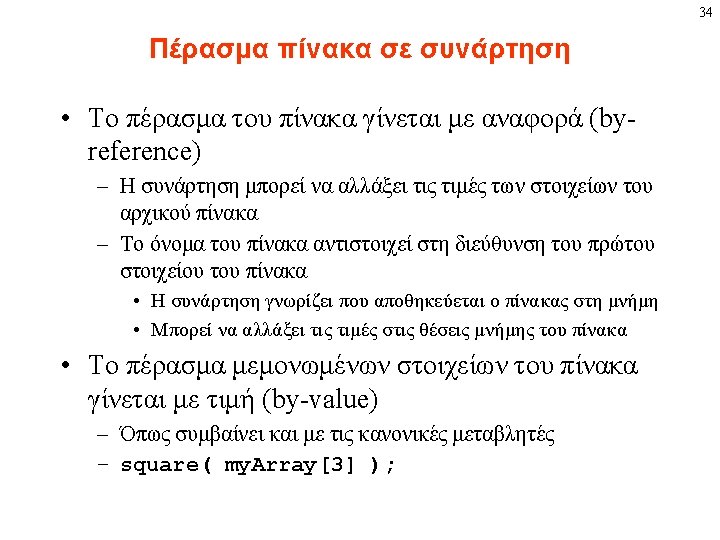
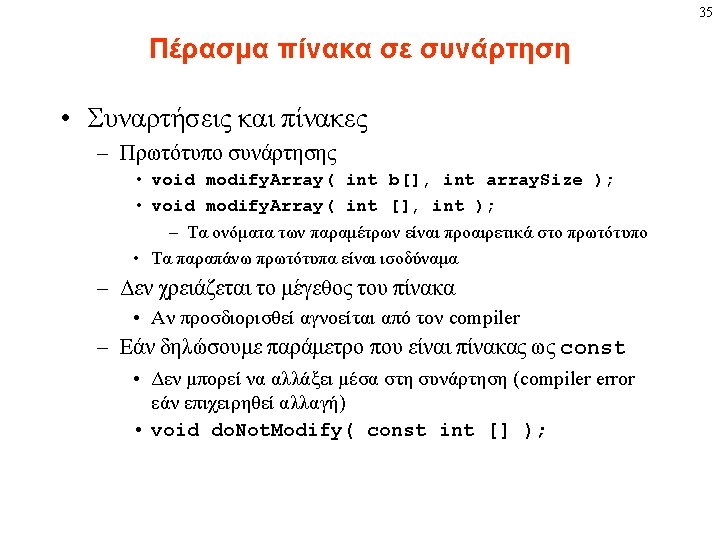
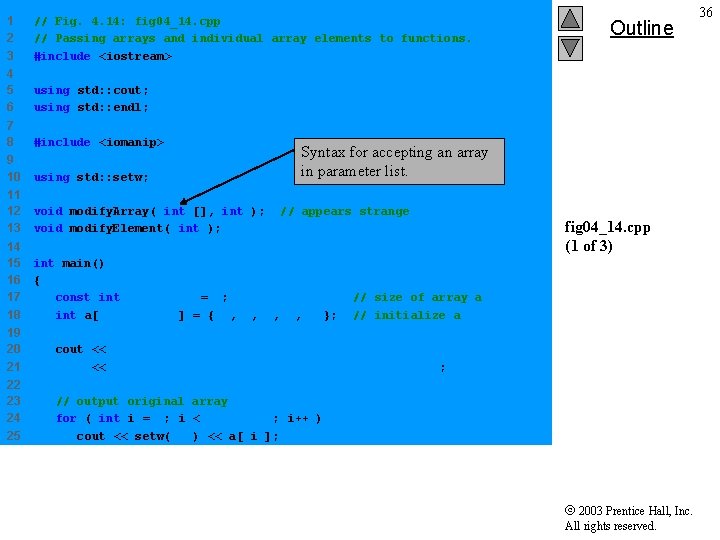
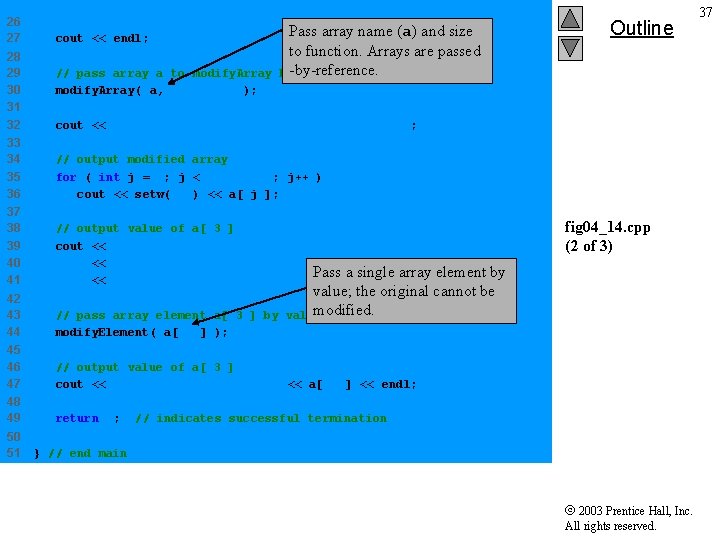
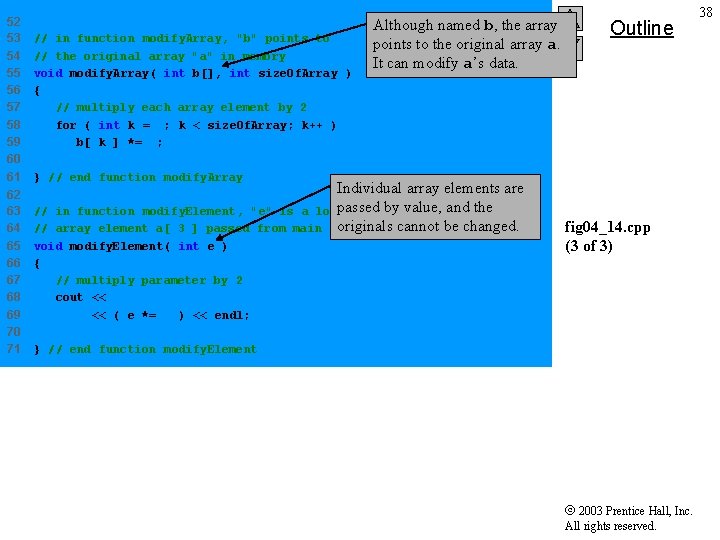
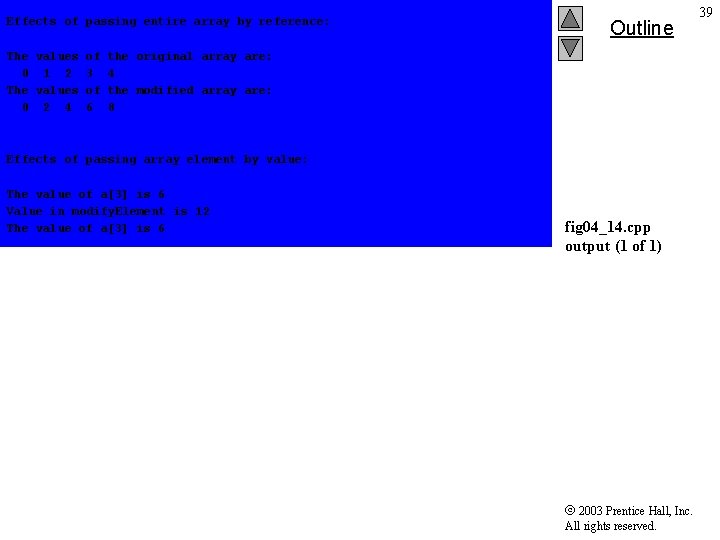
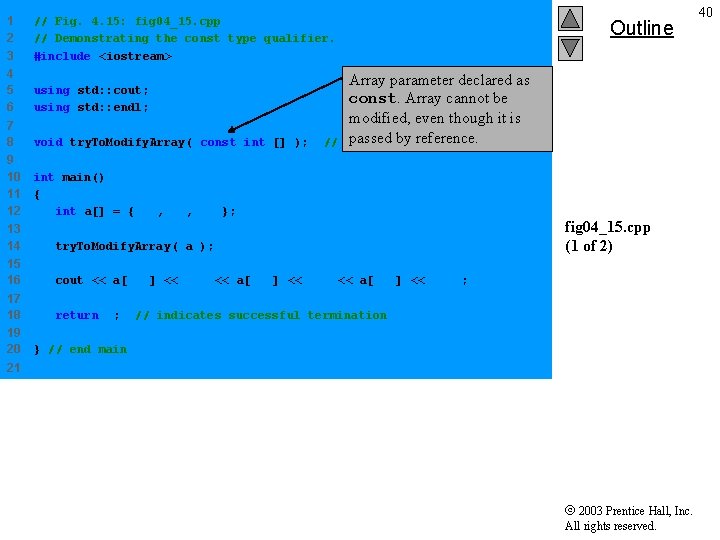
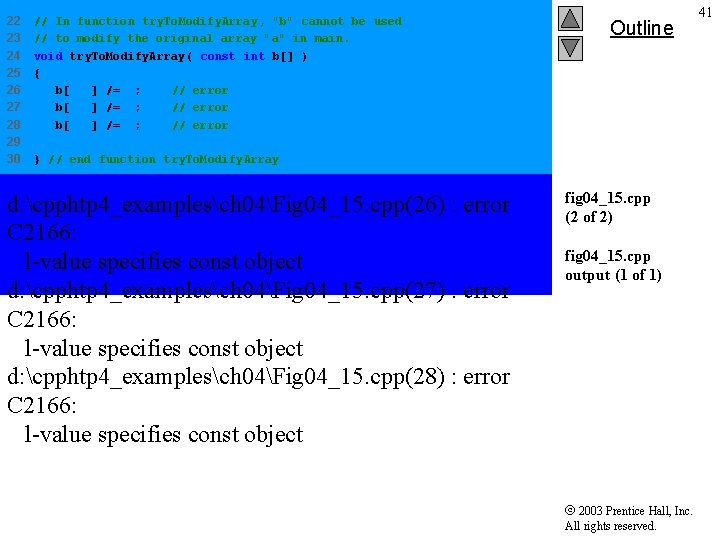
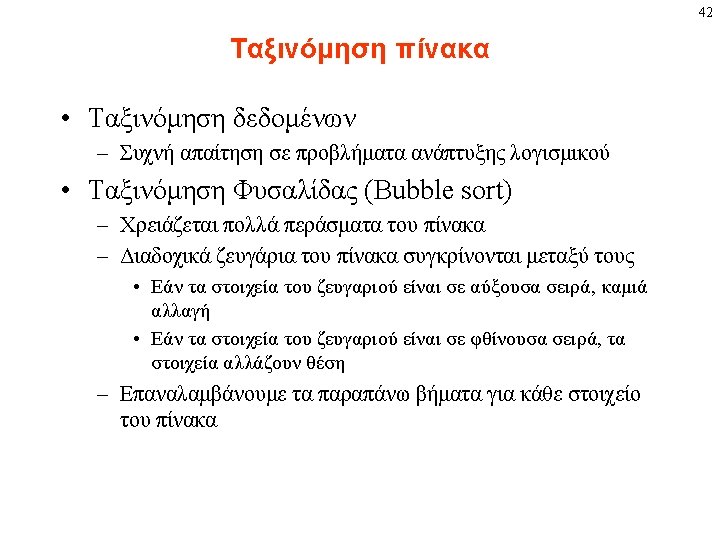
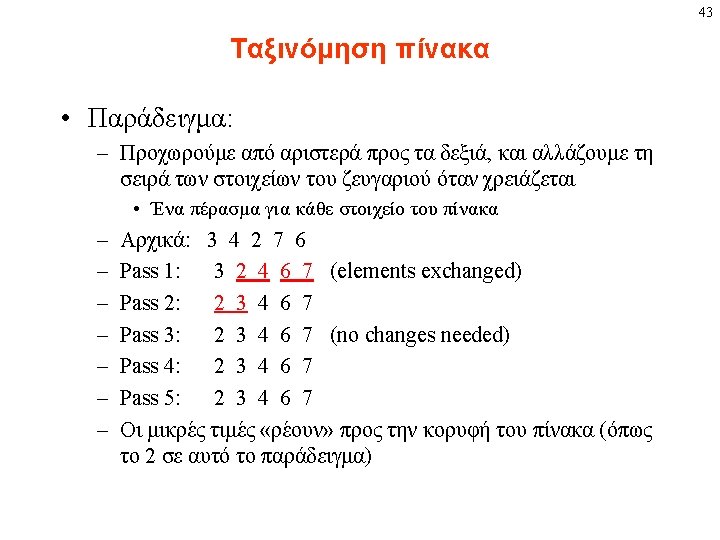
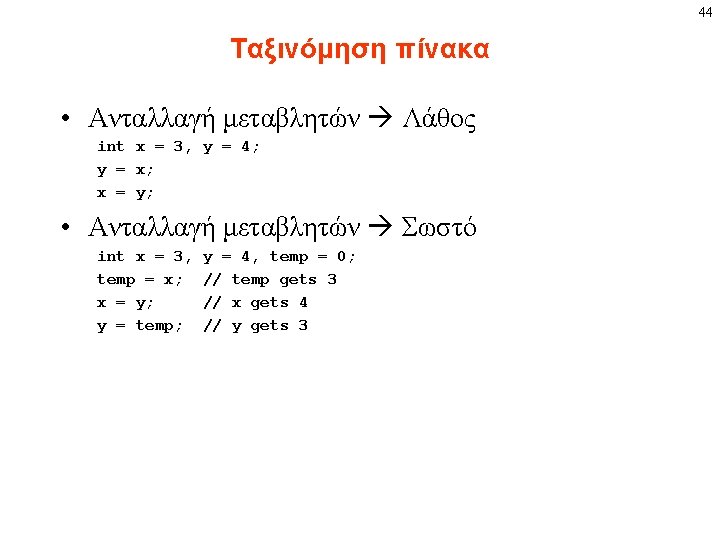
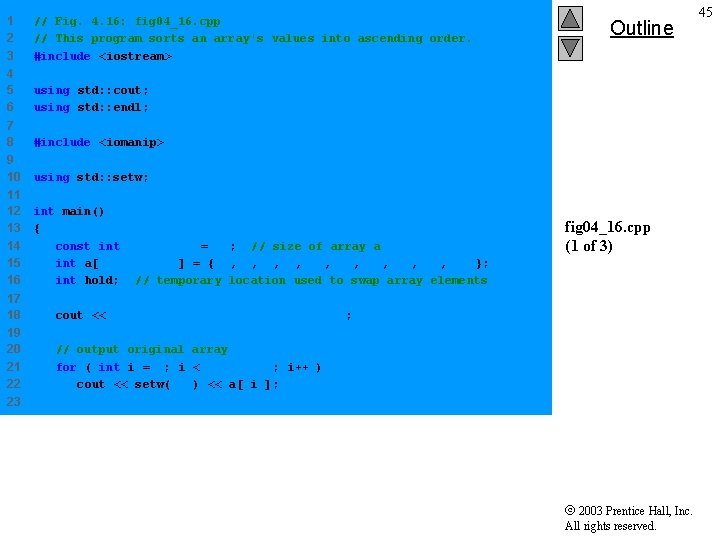
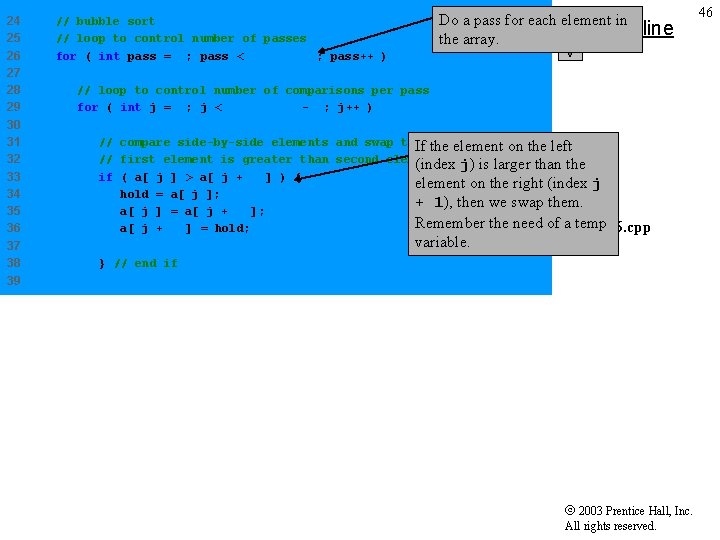
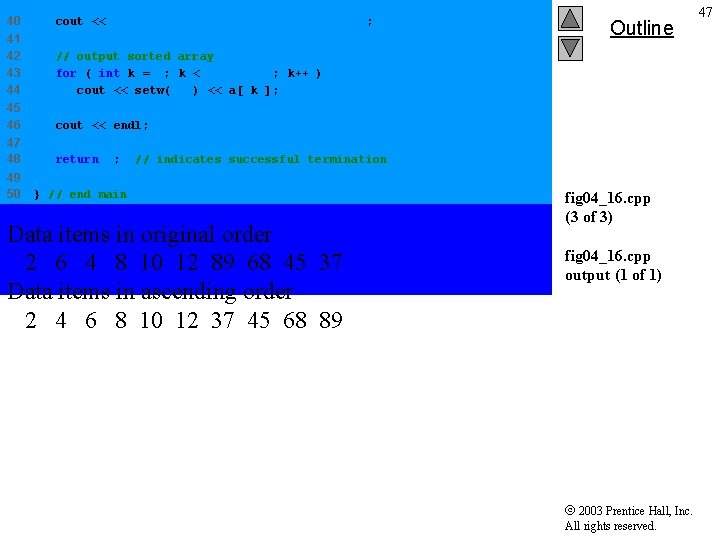
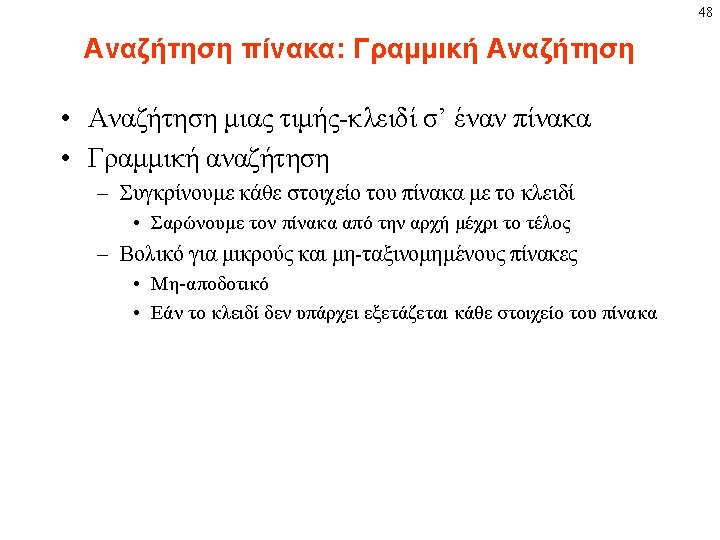
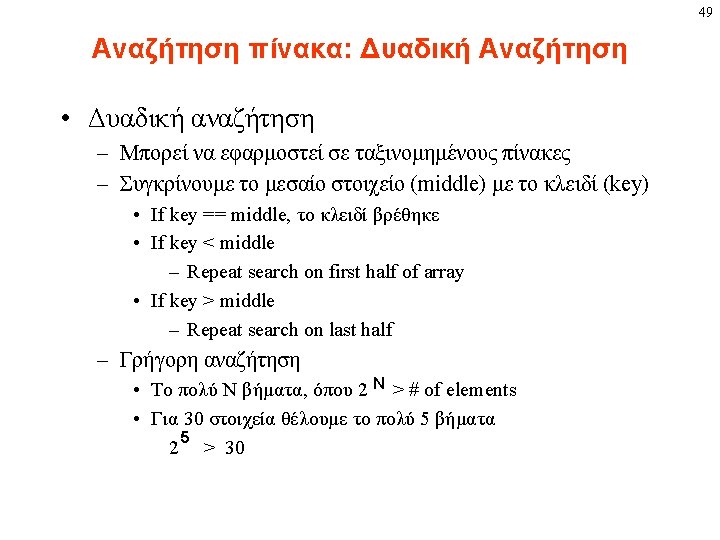
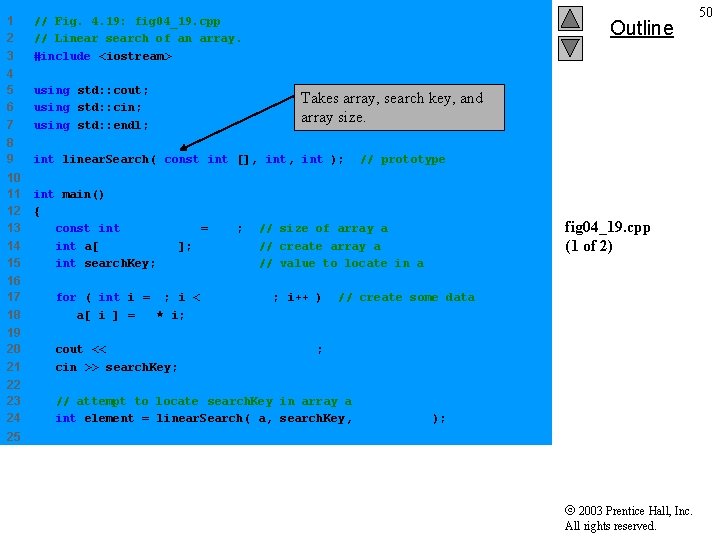
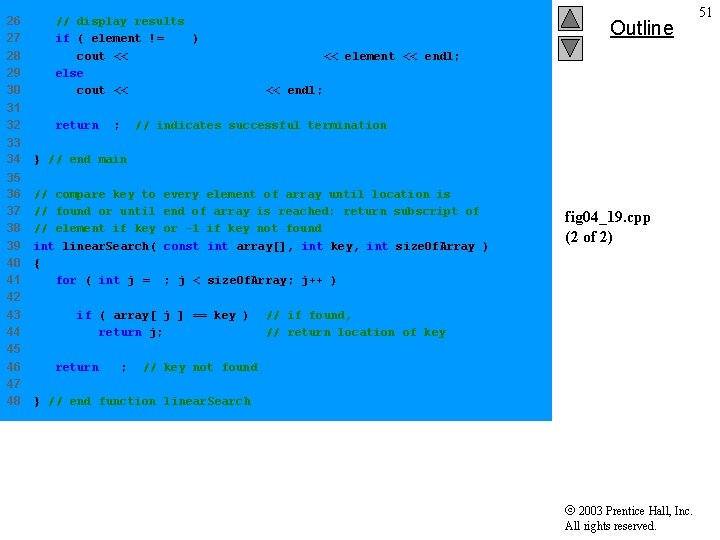
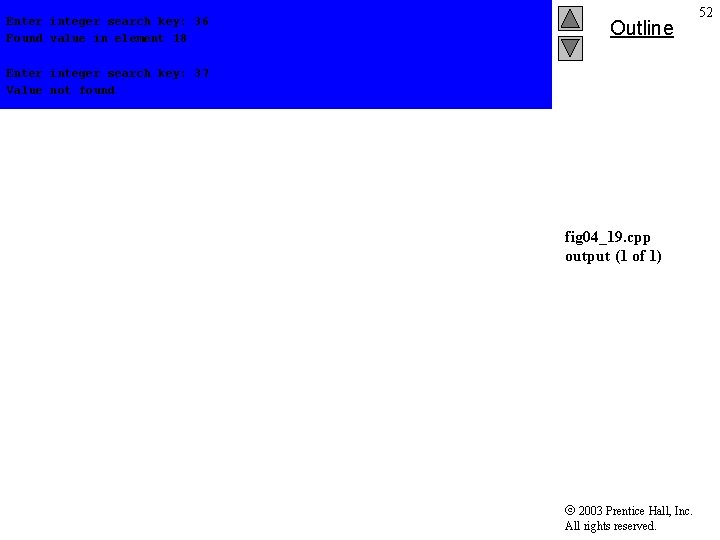
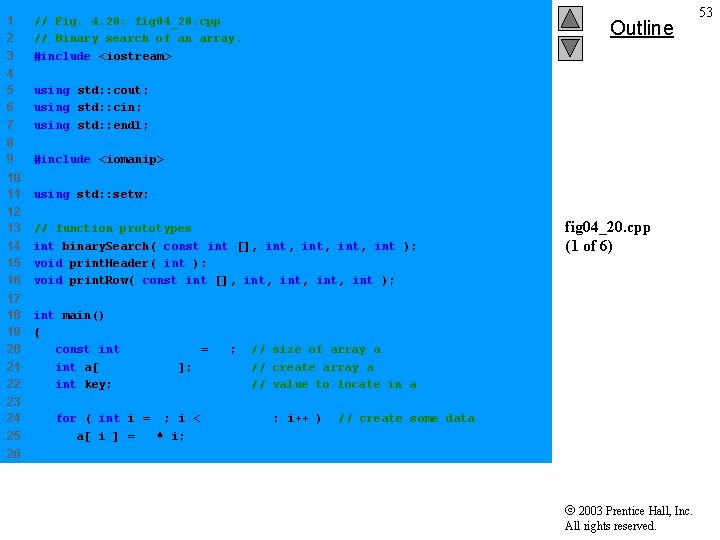
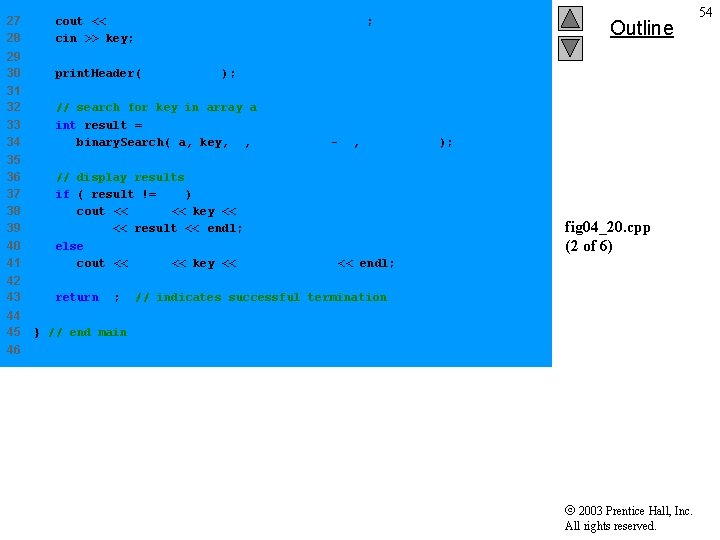
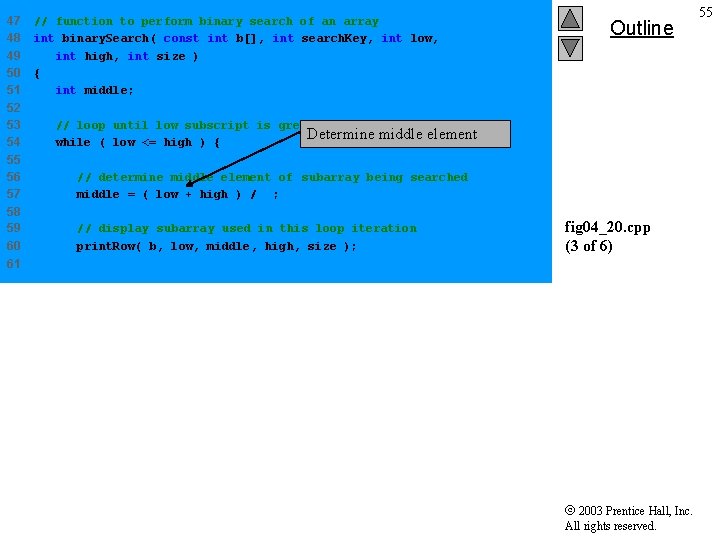
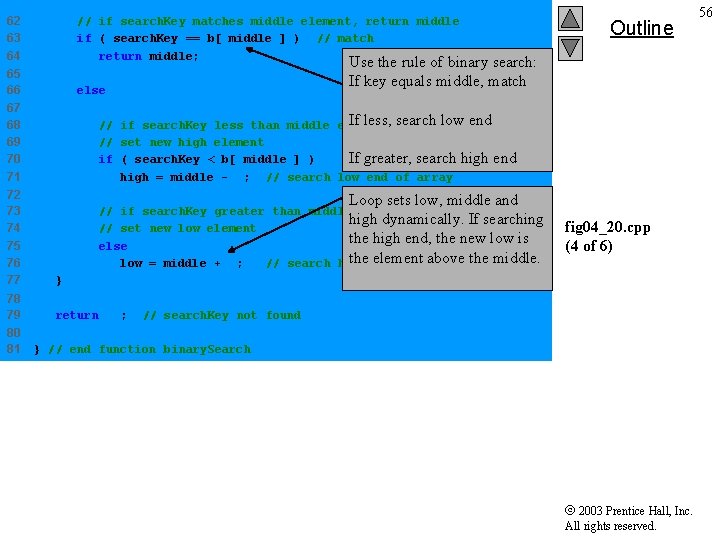
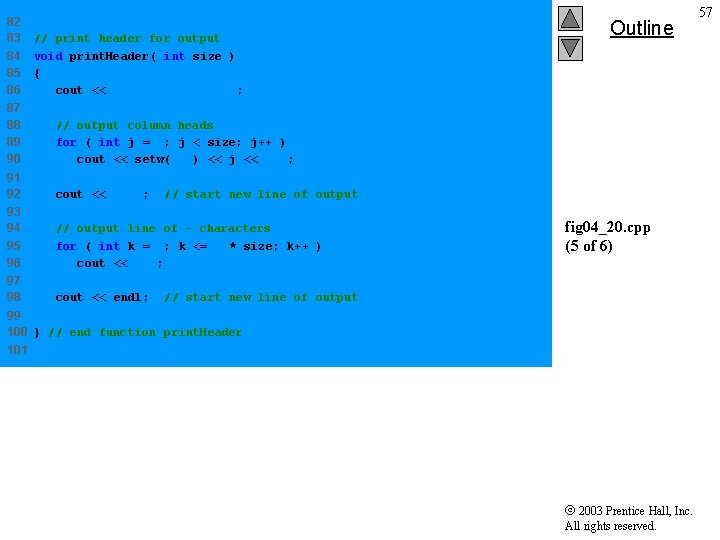
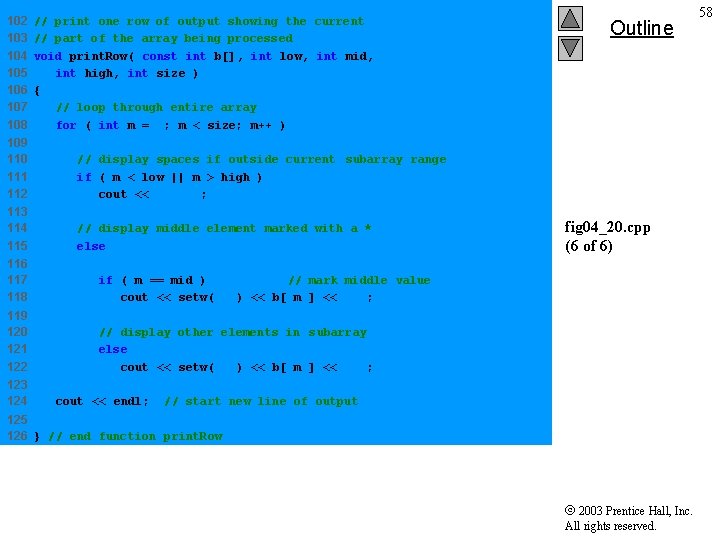
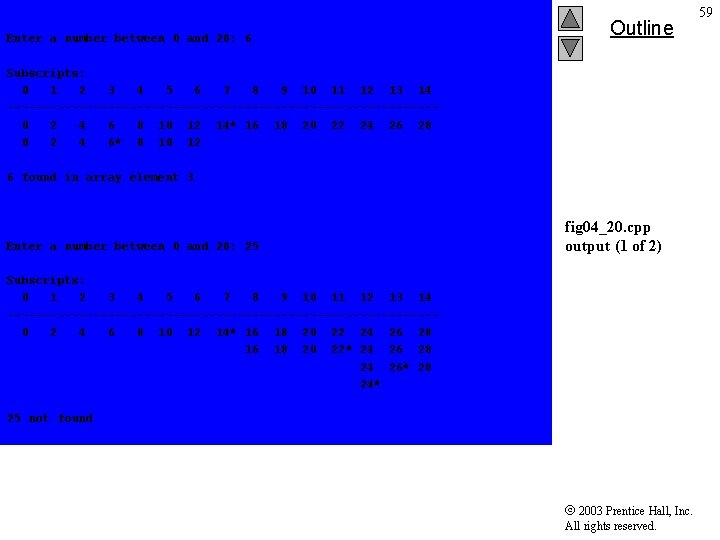
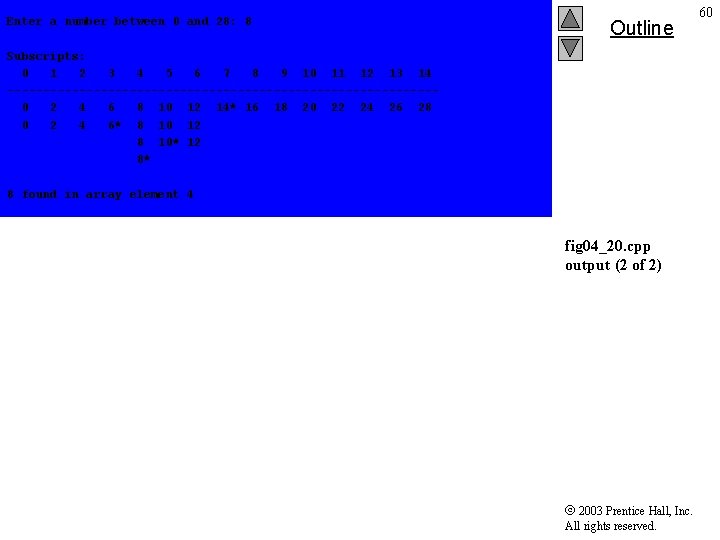
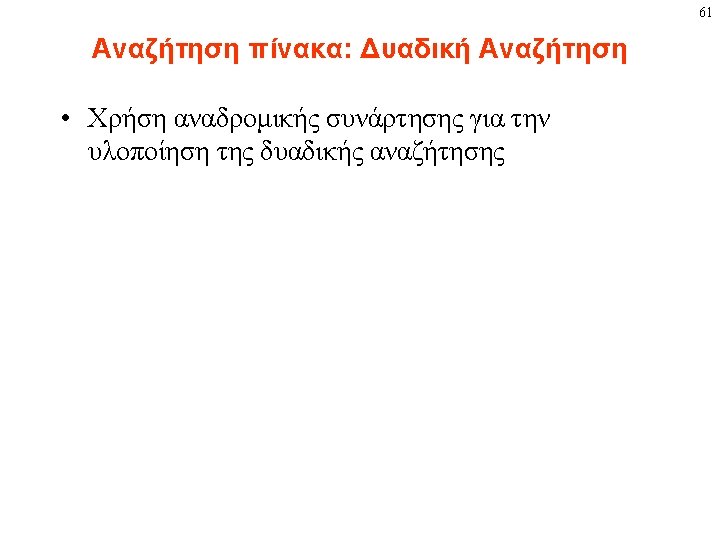
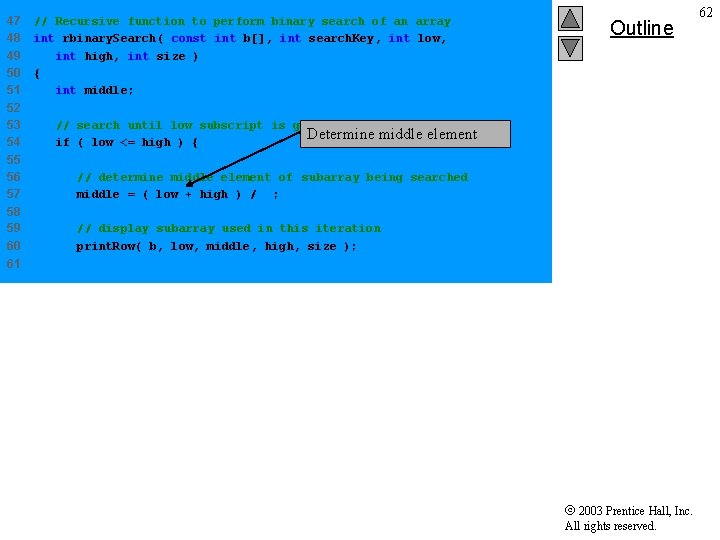
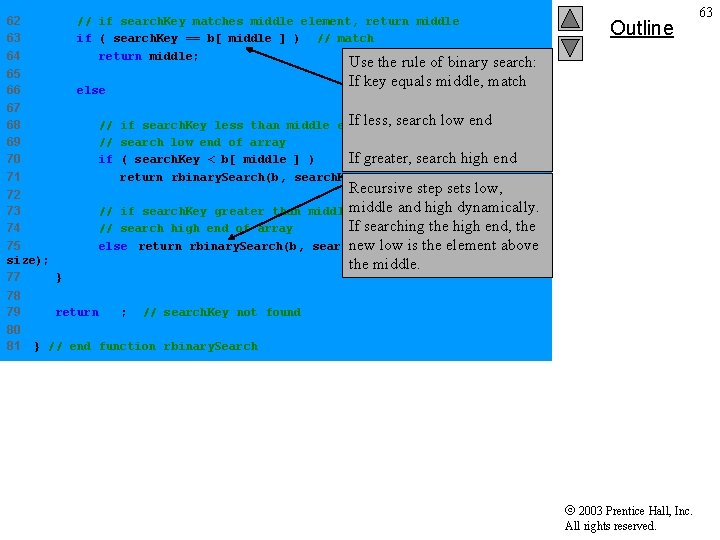
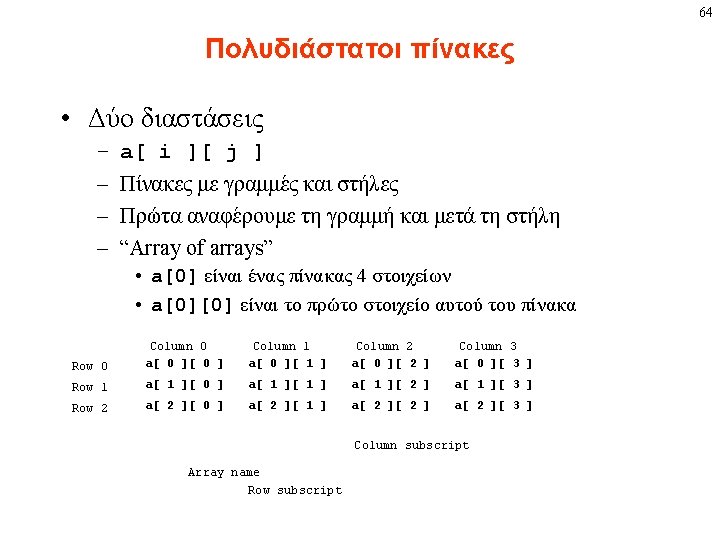
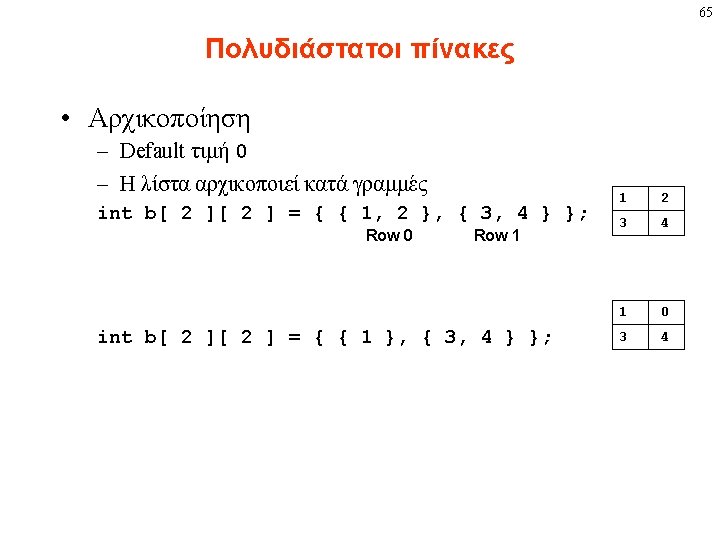
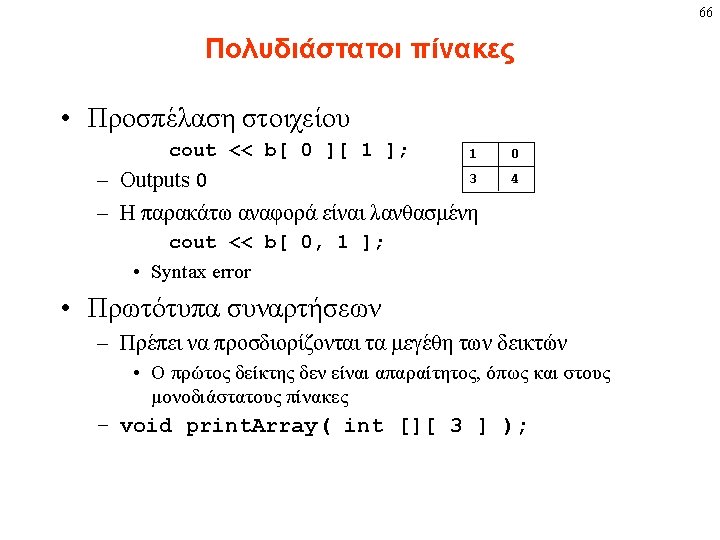
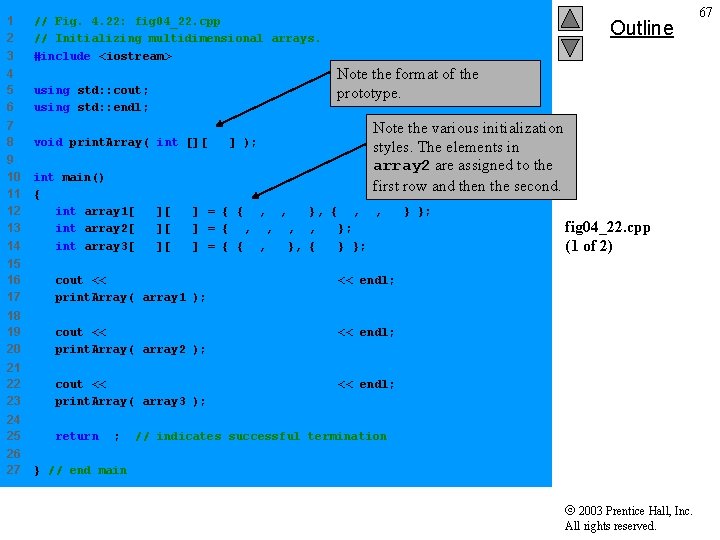
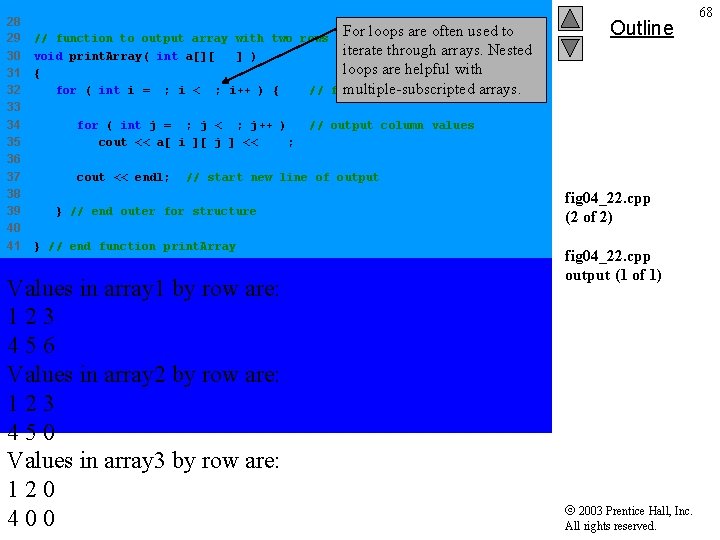
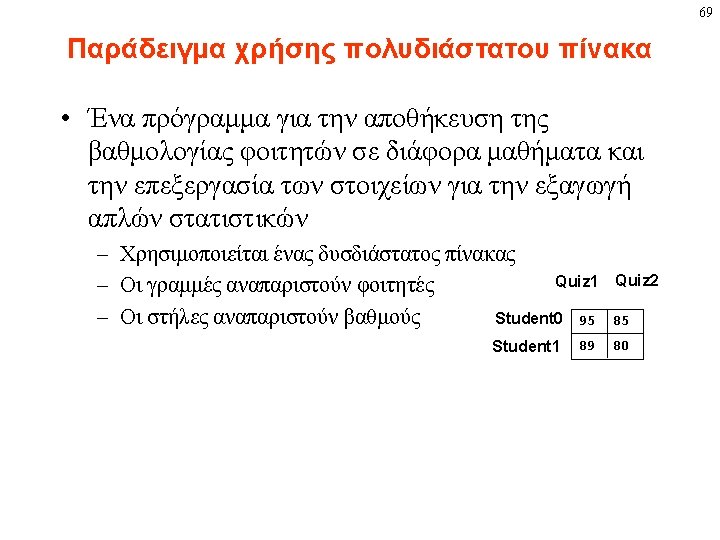
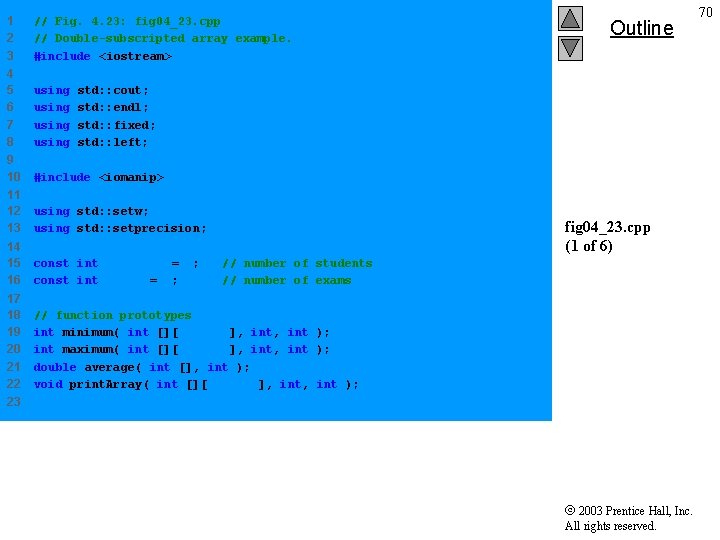
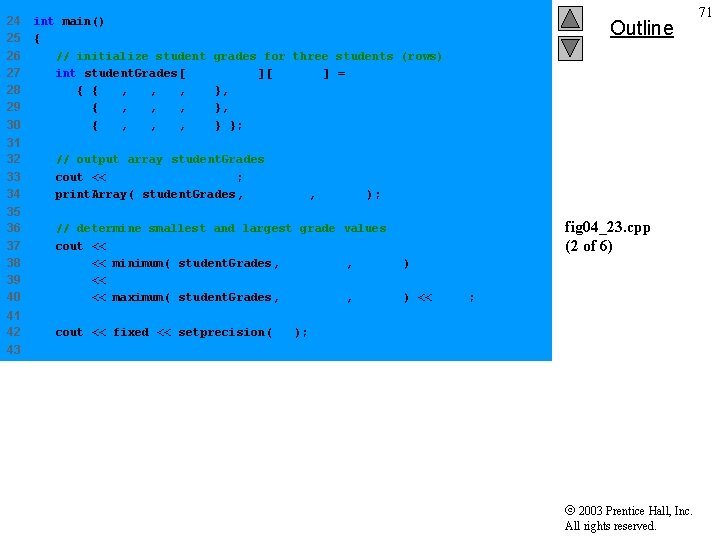
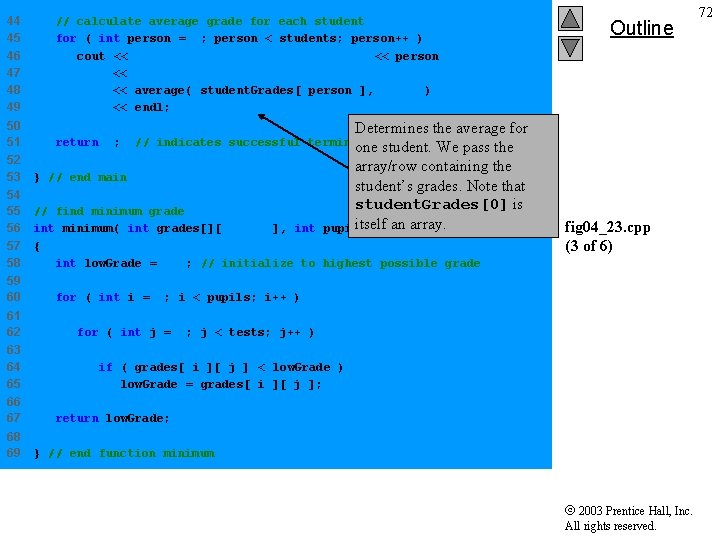
![70 71 72 73 74 // find maximum grade int maximum( int grades[][ exams 70 71 72 73 74 // find maximum grade int maximum( int grades[][ exams](https://slidetodoc.com/presentation_image_h/30709c6f2f777c7d47f52e1a25567873/image-73.jpg)
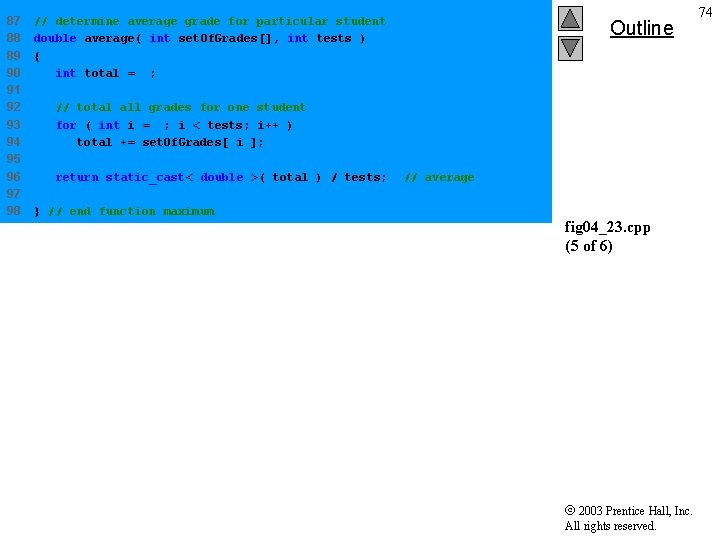
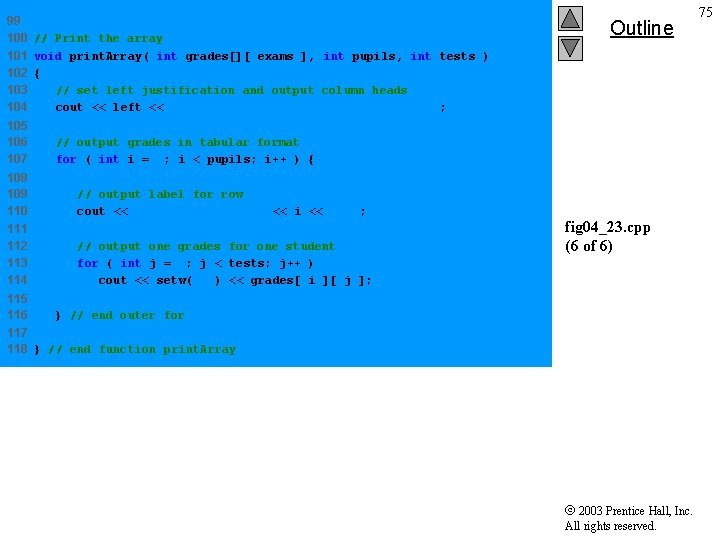
![The array is: [0] [1] [2] [3] student. Grades[0] 77 68 86 73 student. The array is: [0] [1] [2] [3] student. Grades[0] 77 68 86 73 student.](https://slidetodoc.com/presentation_image_h/30709c6f2f777c7d47f52e1a25567873/image-76.jpg)
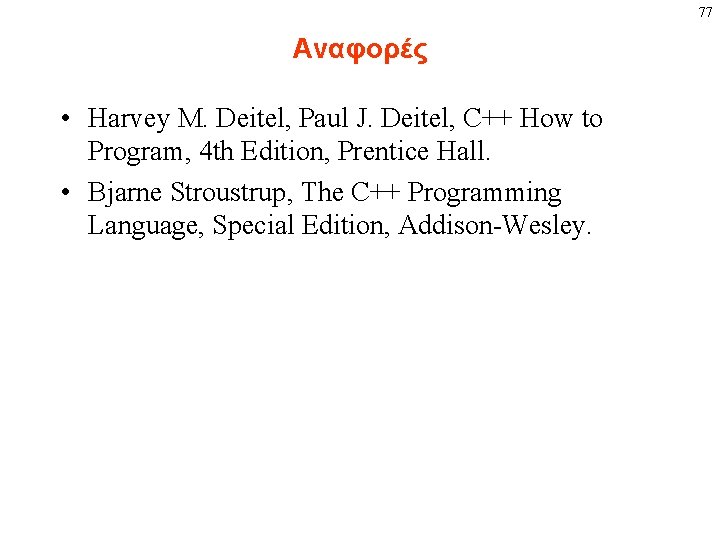
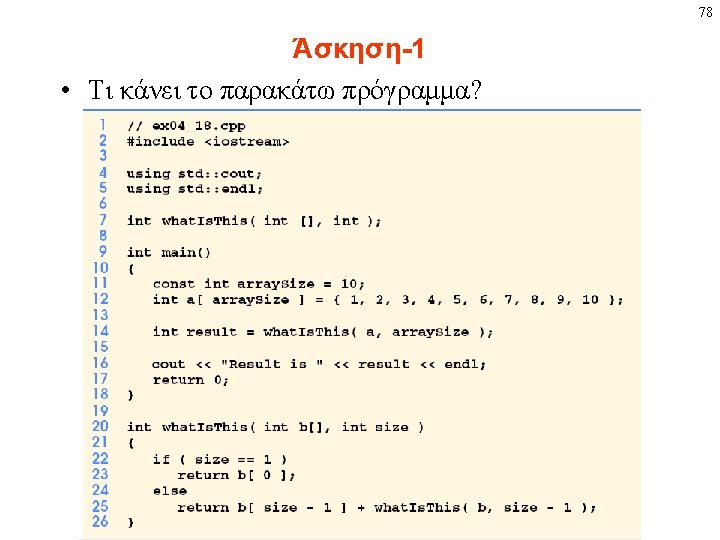
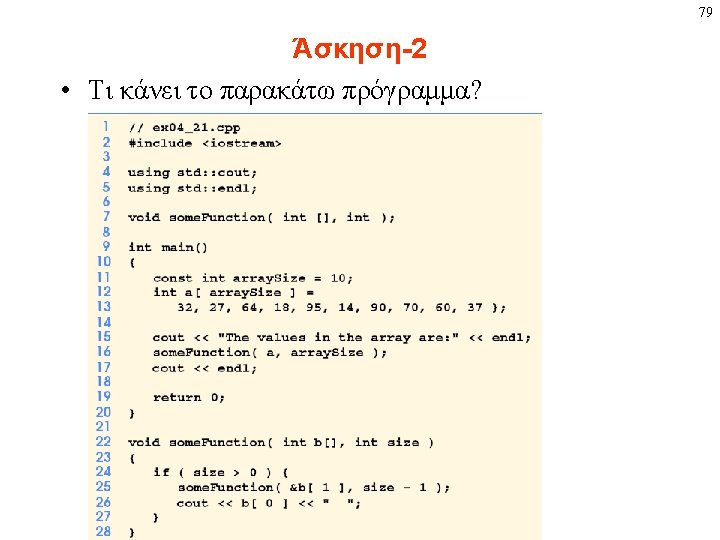
- Slides: 79
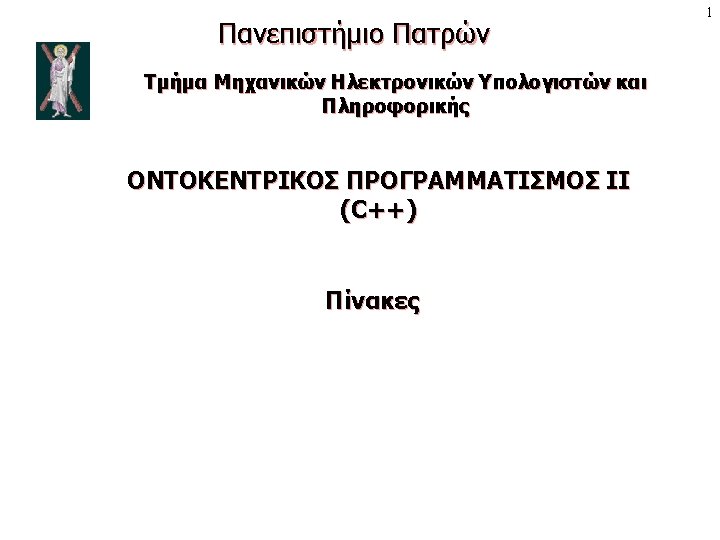
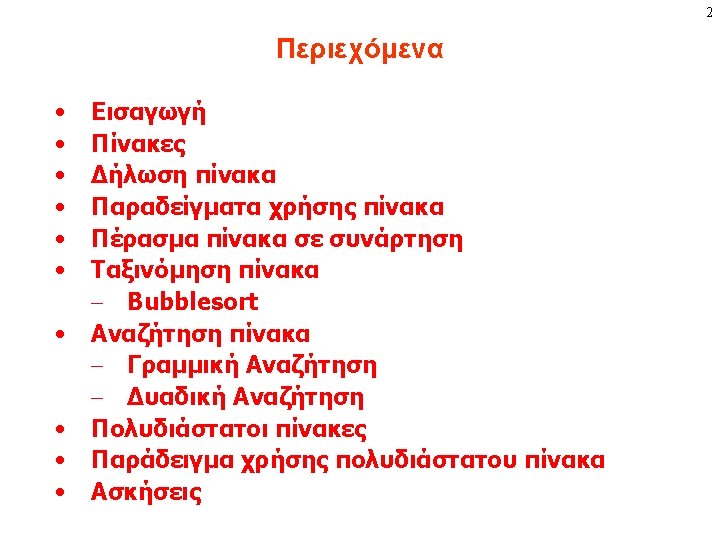
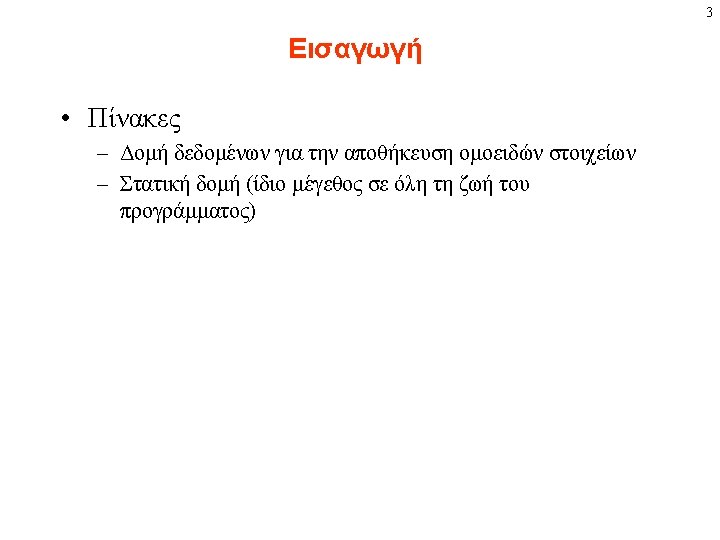
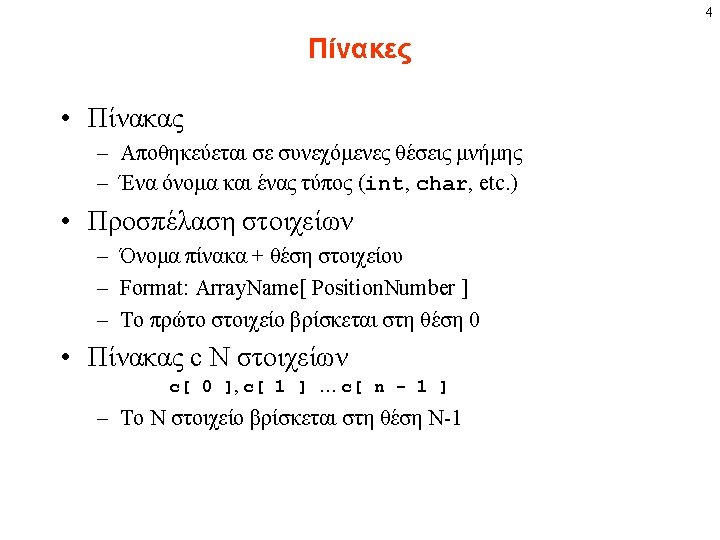
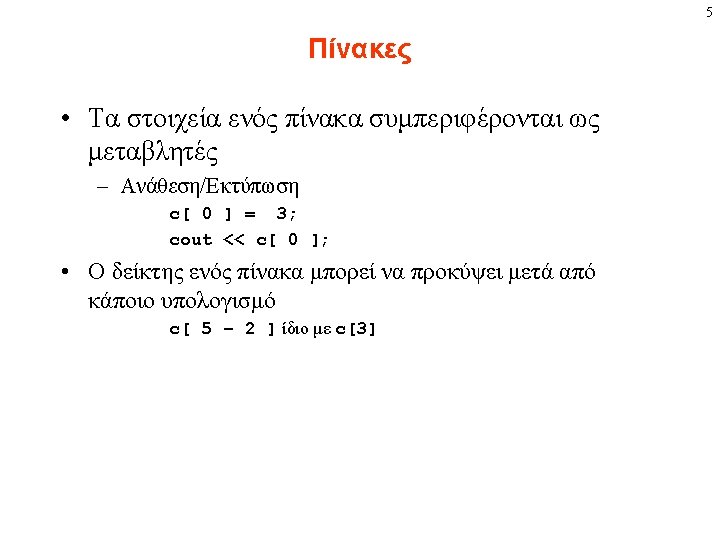
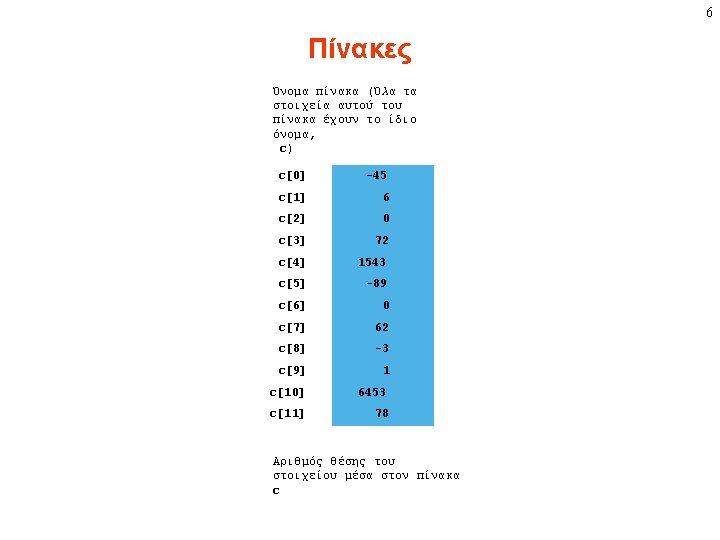
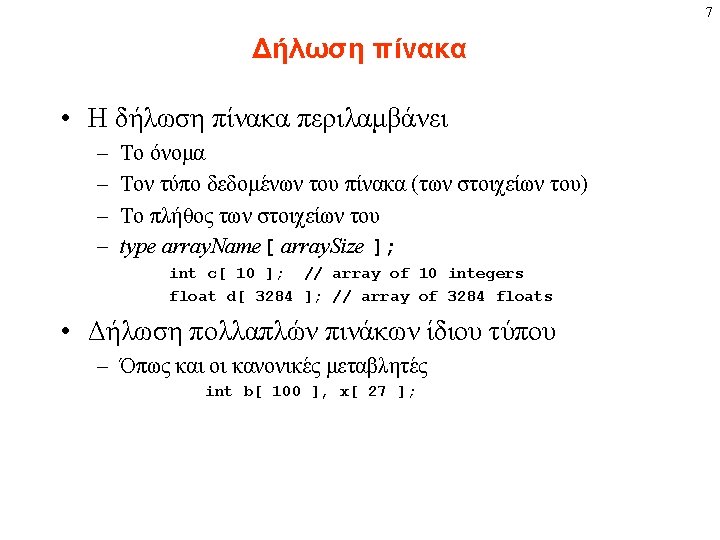
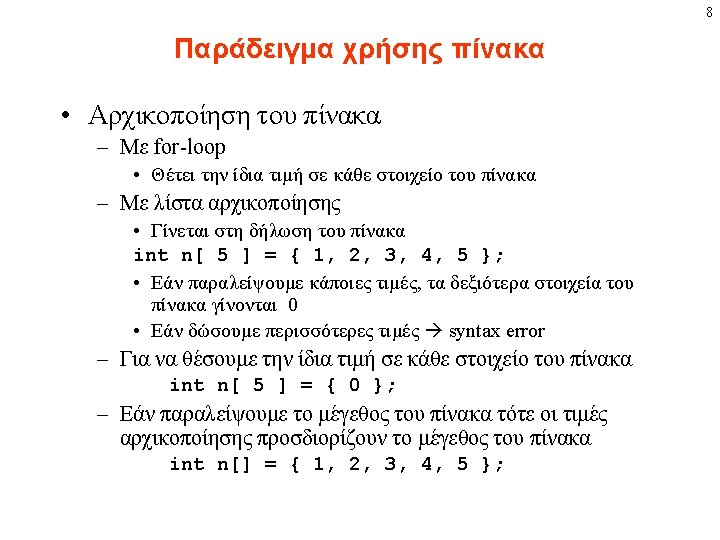
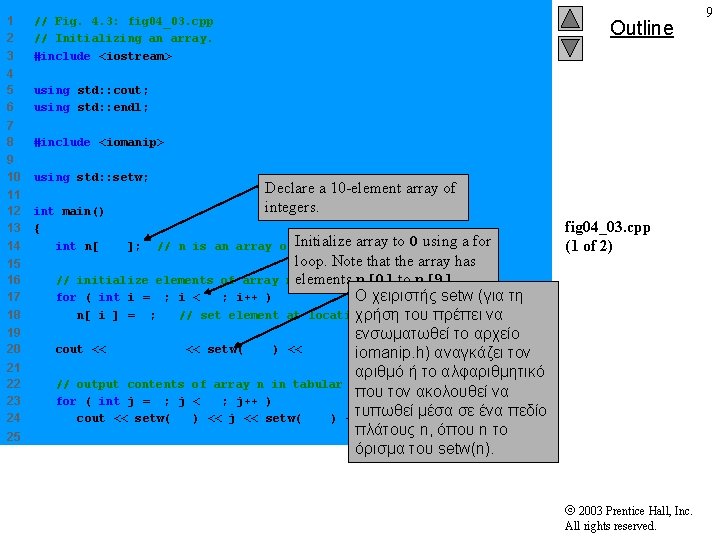
1 2 3 // Fig. 4. 3: fig 04_03. cpp // Initializing an array. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 #include <iomanip> 9 10 using std: : setw; 11 12 13 14 int main() { Initialize array to 0 using a for int n[ 10 ]; // n is an array of 10 integers 15 16 17 18 19 20 21 22 23 24 25 Outline Declare a 10 -element array of integers. loop. Note that the array has elements n[0] to n[9]. // initialize elements of array n to 0 Ο χειριστής setw (για τη for ( int i = 0; i < 10; i++ ) n[ i ] = 0; // set element at location i to 0 χρήση του πρέπει να ενσωματωθεί το αρχείο cout << "Element" << setw( 13 ) << "Value" << endl; iomanip. h) αναγκάζει τον αριθμό ή το αλφαριθμητικό // output contents of array n in tabular format που τον ακολουθεί να for ( int j = 0; j < 10; j++ ) τυπωθεί μέσα σε ένα πεδίο cout << setw( 7 ) << j << setw( 13 ) << n[ j ] << endl; πλάτους n, όπου n το όρισμα του setw(n). fig 04_03. cpp (1 of 2) 2003 Prentice Hall, Inc. All rights reserved. 9
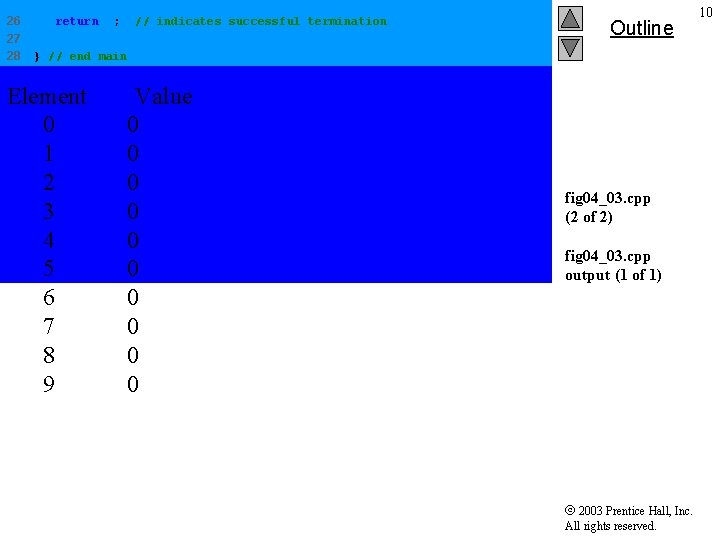
26 return 0; // indicates successful termination 27 28 } // end main Element Value 0 0 1 0 2 0 3 0 4 0 5 0 6 0 7 0 8 0 9 0 Outline fig 04_03. cpp (2 of 2) fig 04_03. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 10
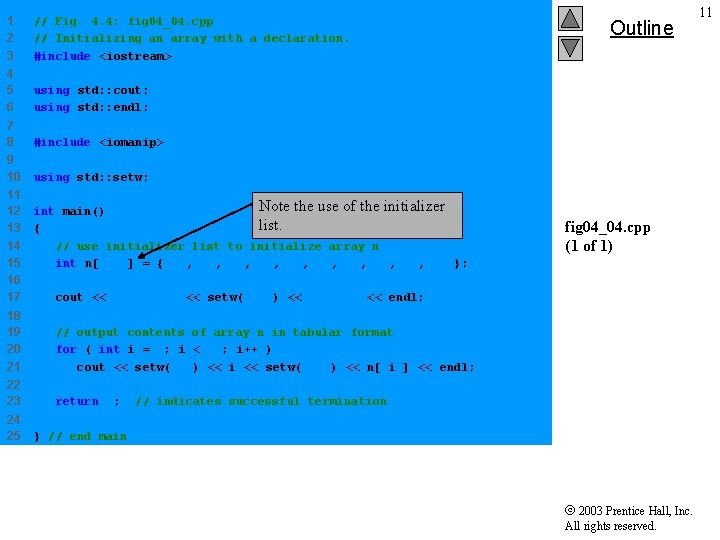
1 2 3 // Fig. 4. 4: fig 04_04. cpp // Initializing an array with a declaration. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 #include <iomanip> 9 10 using std: : setw; 11 12 13 14 15 16 17 Note the use of the initializer int main() list. { // use initializer list to initialize array n int n[ 10 ] = { 32, 27, 64, 18, 95, 14, 90, 70, 60, 37 }; cout << "Element" << setw( 13 ) << "Value" << endl; 18 19 20 21 // output contents of array n in tabular format for ( int i = 0; i < 10; i++ ) cout << setw( 7 ) << i << setw( 13 ) << n[ i ] << endl; 22 23 return 0; // indicates successful termination 24 25 } // end main Outline fig 04_04. cpp (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 11
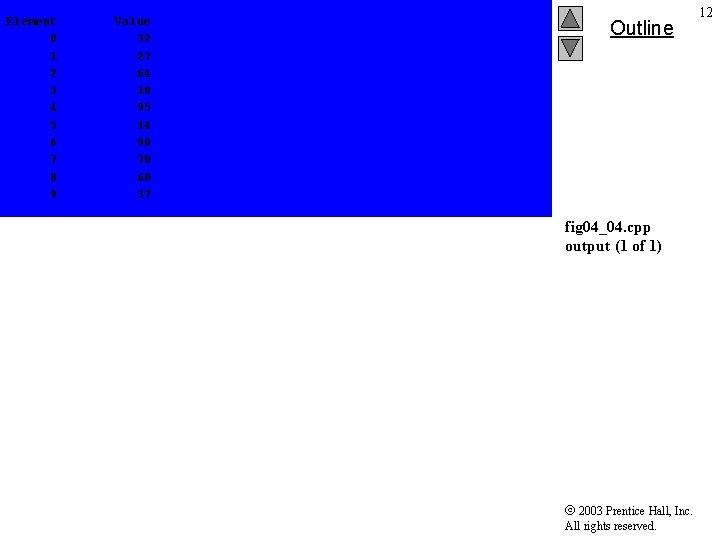
Element Value 0 32 1 27 2 64 3 18 4 95 5 14 6 90 7 70 8 60 9 37 Outline fig 04_04. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 12
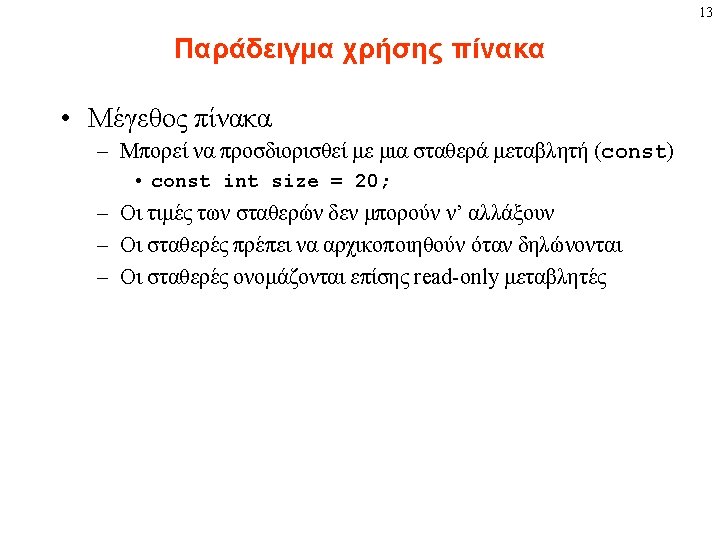
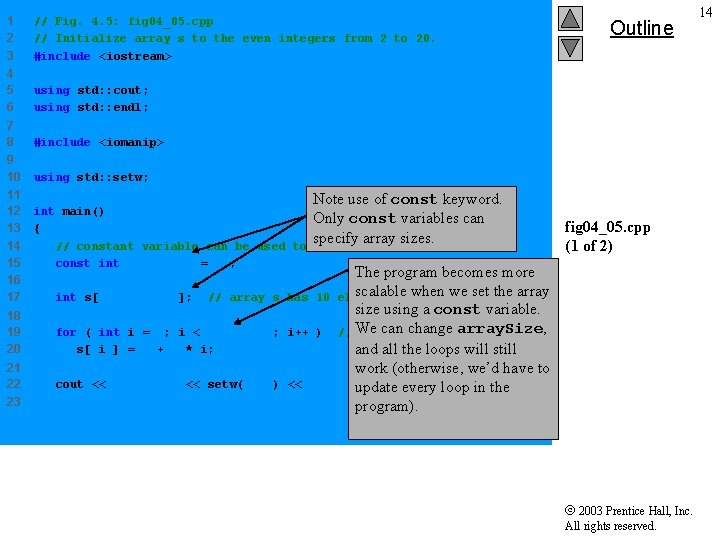
1 2 3 // Fig. 4. 5: fig 04_05. cpp // Initialize array s to the even integers from 2 to 20. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 #include <iomanip> 9 10 using std: : setw; 11 12 13 14 15 16 17 int main() Only const variables can { specify array sizes. // constant variable can be used to specify array size const int array. Size = 10; The program becomes more scalable when we set the array int s[ array. Size ]; // array s has 10 elements 18 19 20 21 22 23 Outline Note use of const keyword. fig 04_05. cpp (1 of 2) size using a const variable. We can change array. Size, for ( int i = 0; i < array. Size; i++ ) // set the values s[ i ] = 2 + 2 * i; and all the loops will still work (otherwise, we’d have to cout << "Element" << setw( 13 ) << "Value" << endl; update every loop in the program). 2003 Prentice Hall, Inc. All rights reserved. 14
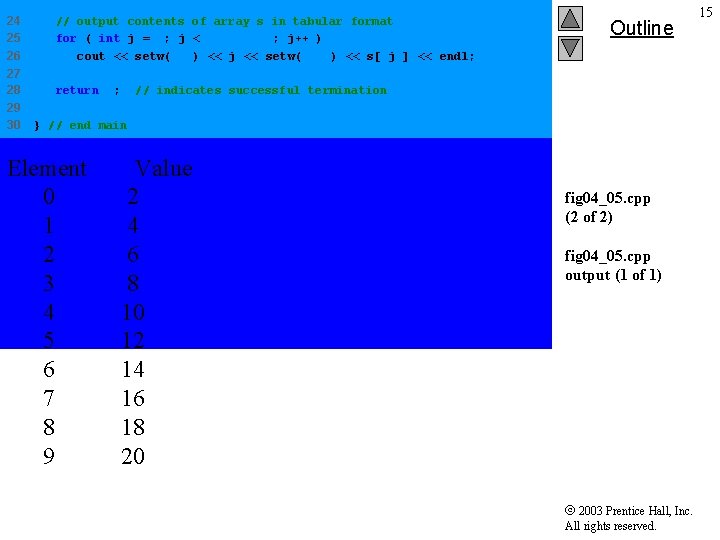
24 25 26 // output contents of array s in tabular format for ( int j = 0; j < array. Size; j++ ) cout << setw( 7 ) << j << setw( 13 ) << s[ j ] << endl; 27 28 return 0; // indicates successful termination 29 30 } // end main Element Value 0 2 1 4 2 6 3 8 4 10 5 12 6 14 7 16 8 18 9 20 Outline fig 04_05. cpp (2 of 2) fig 04_05. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 15
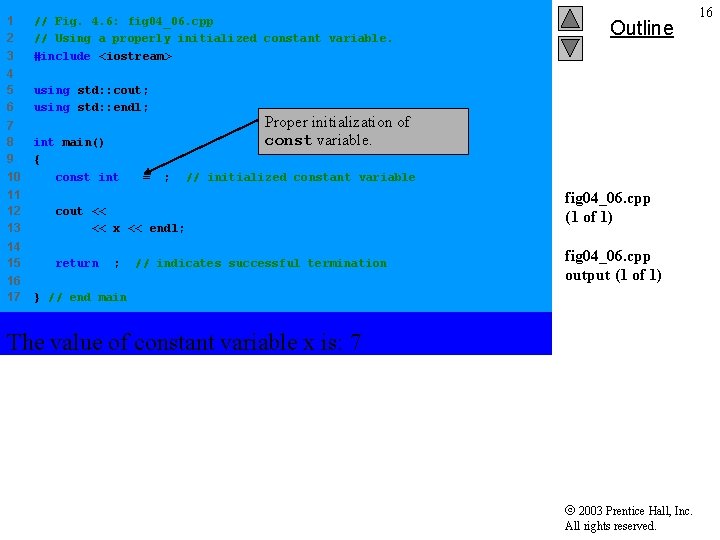
1 2 3 // Fig. 4. 6: fig 04_06. cpp // Using a properly initialized constant variable. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 int main() { const int x = 7; // initialized constant variable 11 12 13 cout << "The value of constant variable x is: " << x << endl; 14 15 return 0; // indicates successful termination 16 17 } // end main Outline Proper initialization of const variable. fig 04_06. cpp (1 of 1) fig 04_06. cpp output (1 of 1) The value of constant variable x is: 7 2003 Prentice Hall, Inc. All rights reserved. 16
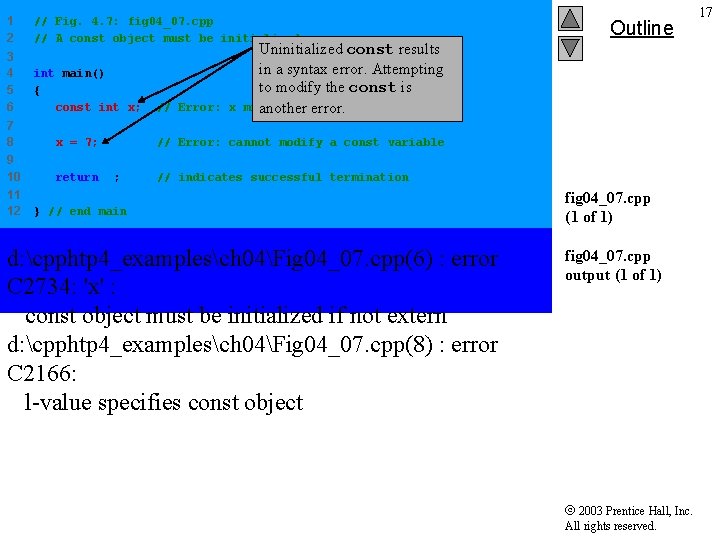
1 2 // Fig. 4. 7: fig 04_07. cpp // A const object must be initialized. 3 4 5 6 Uninitialized const results in a syntax error. Attempting int main() to modify the const is { const int x; // Error: x must be initialized another error. 7 8 x = 7; // Error: cannot modify a const variable 9 10 return 0; // indicates successful termination 11 12 } // end main d: cpphtp 4_examplesch 04Fig 04_07. cpp(6) : error C 2734: 'x' : const object must be initialized if not extern d: cpphtp 4_examplesch 04Fig 04_07. cpp(8) : error C 2166: l-value specifies const object Outline fig 04_07. cpp (1 of 1) fig 04_07. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 17
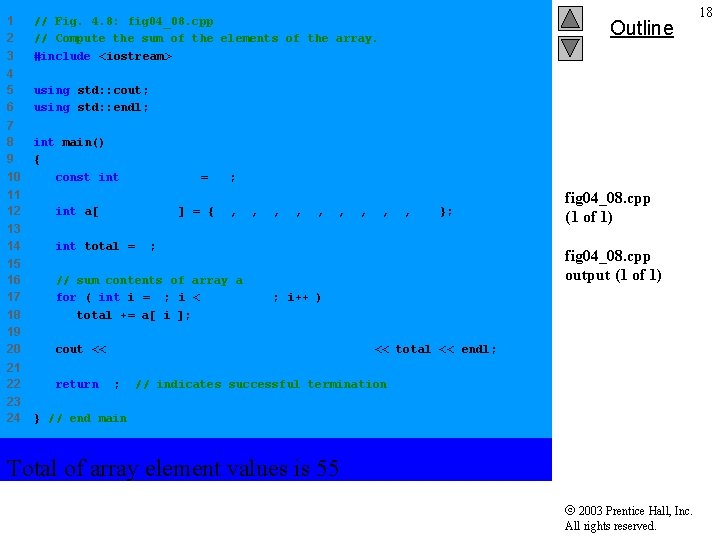
1 2 3 // Fig. 4. 8: fig 04_08. cpp // Compute the sum of the elements of the array. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 int main() { const int array. Size = 10; 11 12 int a[ array. Size ] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; 13 14 int total = 0; 15 16 17 18 19 20 // sum contents of array a for ( int i = 0; i < array. Size; i++ ) total += a[ i ]; cout << "Total of array element values is " << total << endl; 21 22 return 0; // indicates successful termination 23 24 } // end main Outline fig 04_08. cpp (1 of 1) fig 04_08. cpp output (1 of 1) Total of array element values is 55 2003 Prentice Hall, Inc. All rights reserved. 18
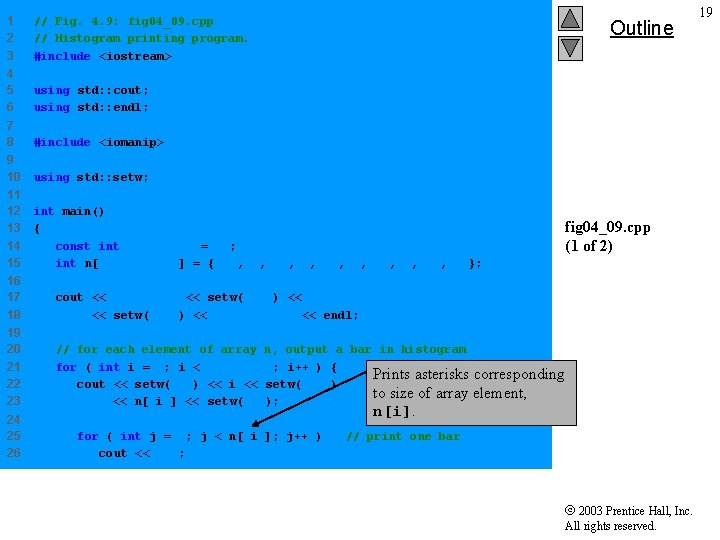
1 2 3 // Fig. 4. 9: fig 04_09. cpp // Histogram printing program. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 #include <iomanip> 9 10 using std: : setw; 11 12 13 14 15 int main() { const int array. Size = 10; int n[ array. Size ] = { 19, 3, 15, 7, 11, 9, 13, 5, 17, 1 }; 16 17 18 cout << "Element" << setw( 13 ) << "Value" << setw( 17 ) << "Histogram" << endl; 19 20 21 22 23 // for each element of array n, output a bar in histogram for ( int i = 0; i < array. Size; i++ ) { Prints asterisks corresponding cout << setw( 7 ) << i << setw( 13 ) to size of array element, << n[ i ] << setw( 9 ); 24 25 26 for ( int j = 0; j < n[ i ]; j++ ) // print one bar cout << '*'; Outline fig 04_09. cpp (1 of 2) n[i]. 2003 Prentice Hall, Inc. All rights reserved. 19
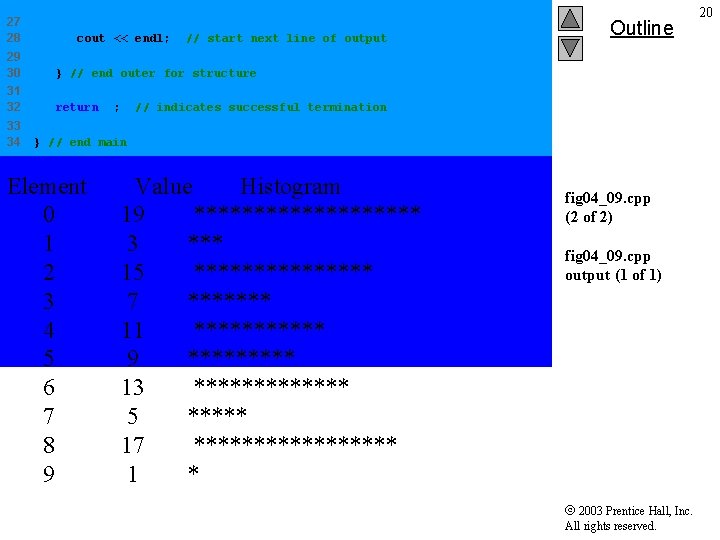
27 28 cout << endl; // start next line of output 29 30 } // end outer for structure 31 32 return 0; // indicates successful termination 33 34 } // end main Element Value Histogram 0 19 ********** 1 3 *** 2 15 ******** 3 7 ******* 4 11 ****** 5 9 ***** 6 13 ******* 7 5 ***** 8 17 ********* 9 1 * Outline fig 04_09. cpp (2 of 2) fig 04_09. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 20
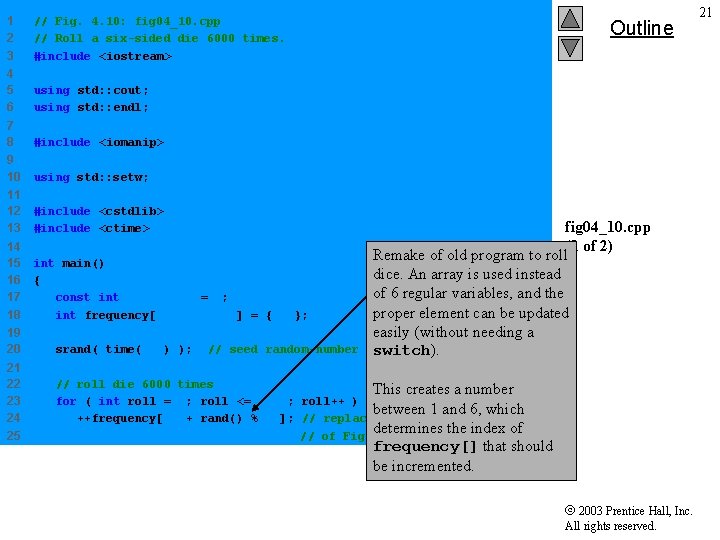
1 2 3 // Fig. 4. 10: fig 04_10. cpp // Roll a six-sided die 6000 times. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 #include <iomanip> 9 10 using std: : setw; 11 12 13 #include <cstdlib> #include <ctime> Outline 19 20 fig 04_10. cpp (1 of 2) Remake of old program to roll int main() dice. An array is used instead { of 6 regular variables, and the const int array. Size = 7; proper element can be updated int frequency[ array. Size ] = { 0 }; easily (without needing a srand( time( 0 ) ); // seed random-number generator switch). 21 22 23 24 25 // roll die 6000 times This creates a number for ( int roll = 1; roll <= 6000; roll++ ) between 1 and 6, which ++frequency[ 1 + rand() % 6 ]; // replaces 20 -line switch determines the index of // of Fig. 3. 8 14 15 16 17 18 frequency[] that should be incremented. 2003 Prentice Hall, Inc. All rights reserved. 21
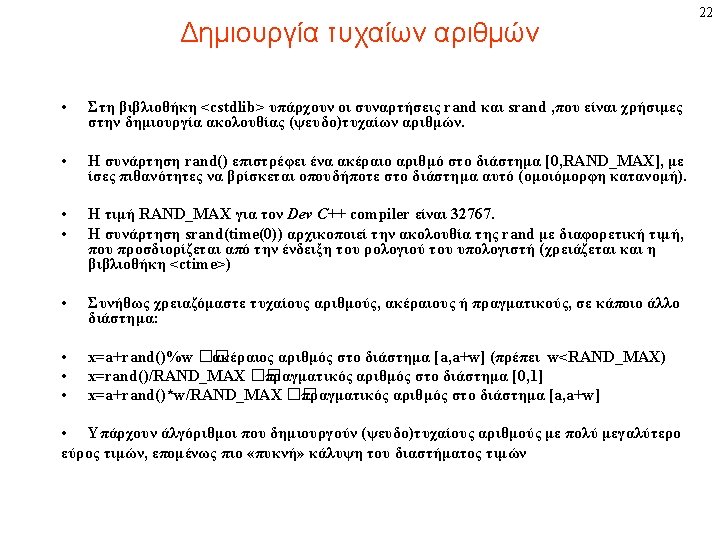
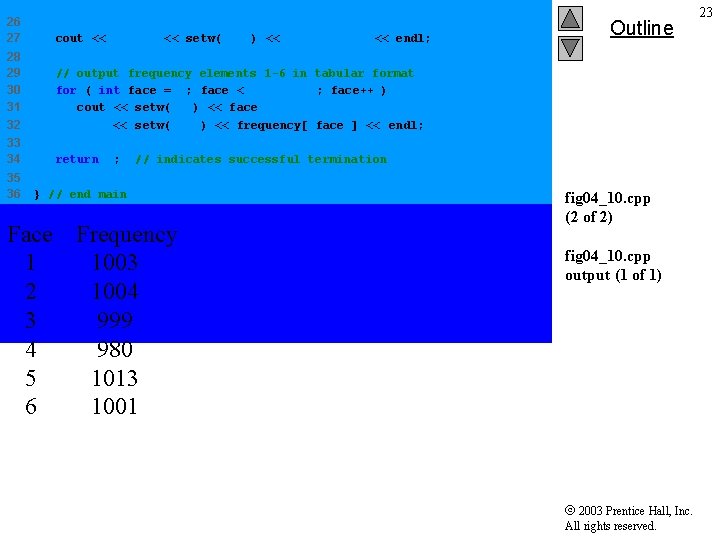
26 27 cout << "Face" << setw( 13 ) << "Frequency" << endl; 28 29 30 31 32 // output frequency elements 1 -6 in tabular format for ( int face = 1; face < array. Size; face++ ) cout << setw( 4 ) << face << setw( 13 ) << frequency[ face ] << endl; 33 34 return 0; // indicates successful termination 35 36 } // end main Face Frequency 1 1003 2 1004 3 999 4 980 5 1013 6 1001 Outline fig 04_10. cpp (2 of 2) fig 04_10. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 23
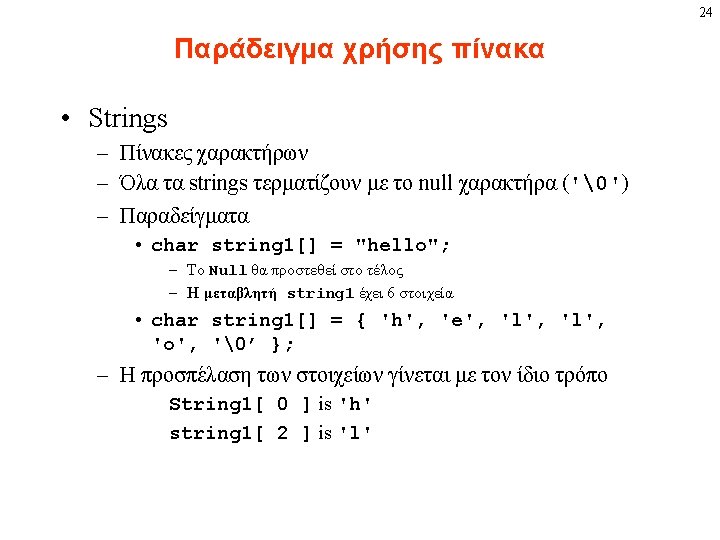
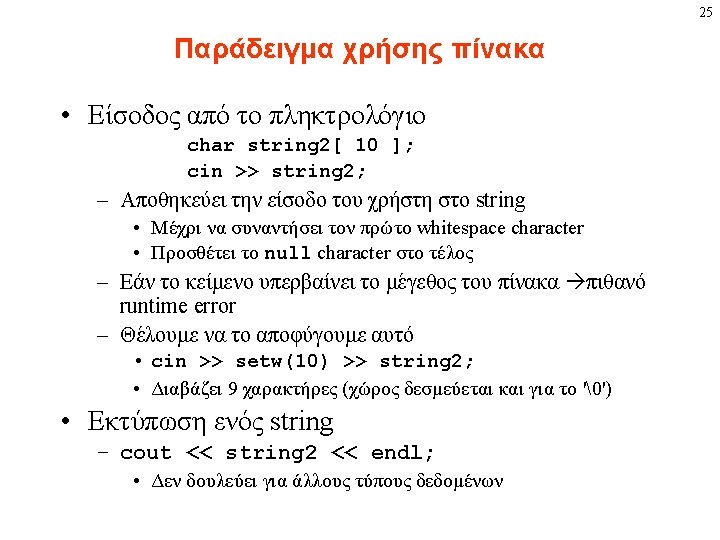
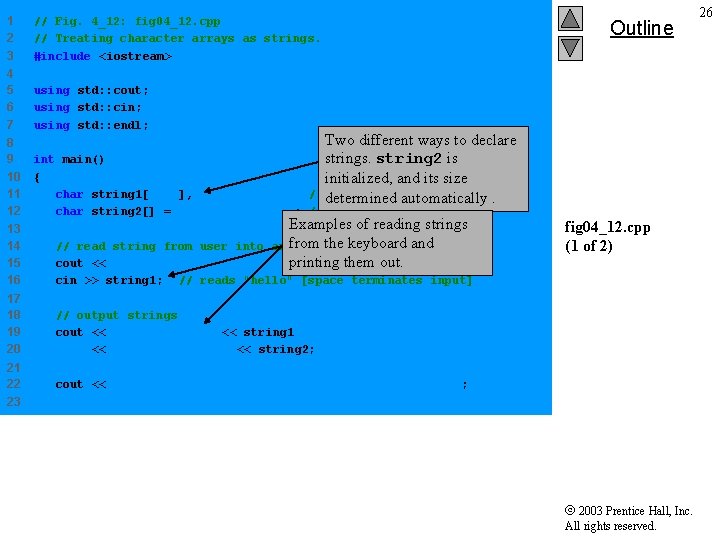
1 2 3 // Fig. 4_12: fig 04_12. cpp // Treating character arrays as strings. #include <iostream> 4 5 6 7 using std: : cout; using std: : cin; using std: : endl; 8 9 10 11 12 13 14 15 16 Outline Two different ways to declare strings. string 2 is int main() { initialized, and its size char string 1[ 20 ], // reserves 20 characters determined automatically. char string 2[] = "string literal"; // reserves 15 characters Examples of reading strings from the keyboard and // read string from user into array string 2 cout << "Enter the string "hello there": " ; printing them out. fig 04_12. cpp (1 of 2) cin >> string 1; // reads "hello" [space terminates input] 17 18 19 20 // output strings cout << "string 1 is: " << string 1 << "nstring 2 is: " << string 2; 21 22 cout << "nstring 1 with spaces between characters is: n" ; 23 2003 Prentice Hall, Inc. All rights reserved. 26
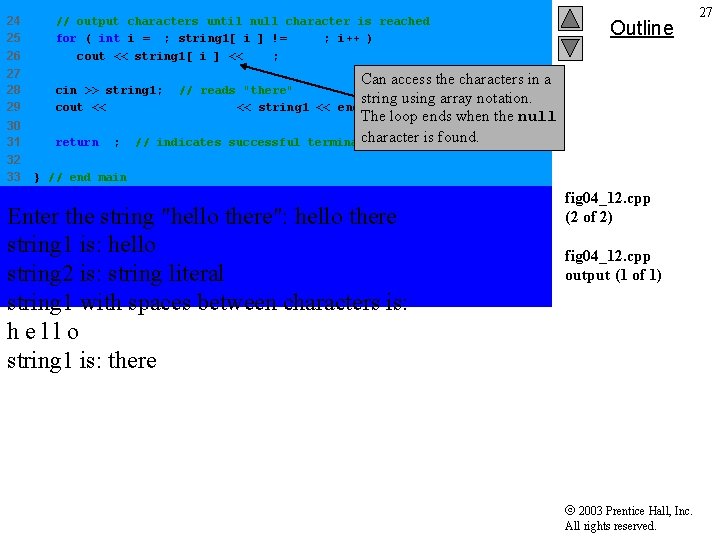
24 25 26 // output characters until null character is reached for ( int i = 0; string 1[ i ] != '