1 2 3 4 5 Abstract methods and
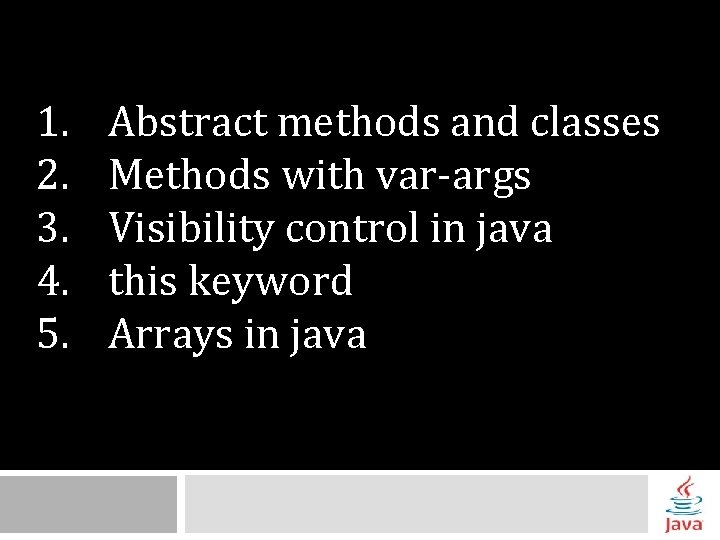
1. 2. 3. 4. 5. Abstract methods and classes Methods with var-args Visibility control in java this keyword Arrays in java
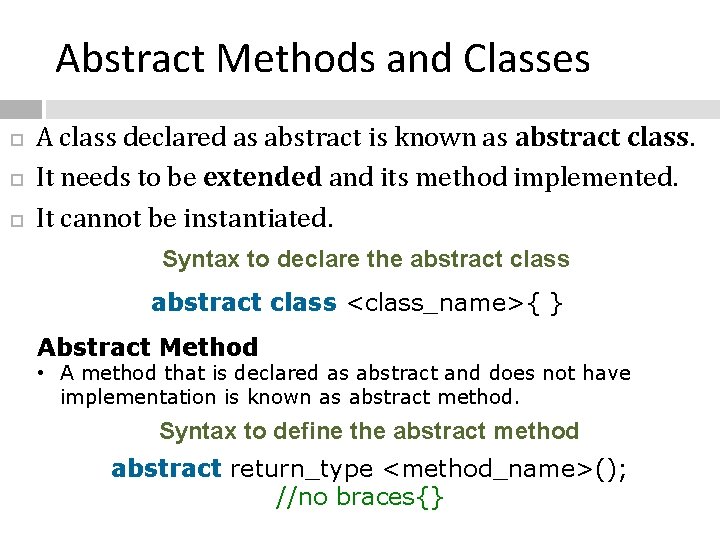
Abstract Methods and Classes A class declared as abstract is known as abstract class. It needs to be extended and its method implemented. It cannot be instantiated. Syntax to declare the abstract class <class_name>{ } Abstract Method • A method that is declared as abstract and does not have implementation is known as abstract method. Syntax to define the abstract method abstract return_type <method_name>(); //no braces{}
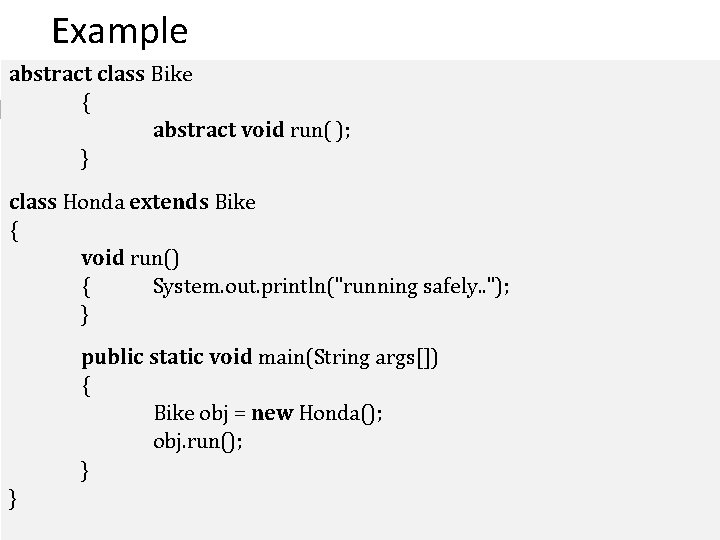
Example abstract class Bike { abstract void run( ); } class Honda extends Bike { void run() { System. out. println("running safely. . "); } } public static void main(String args[]) { Bike obj = new Honda(); obj. run(); }
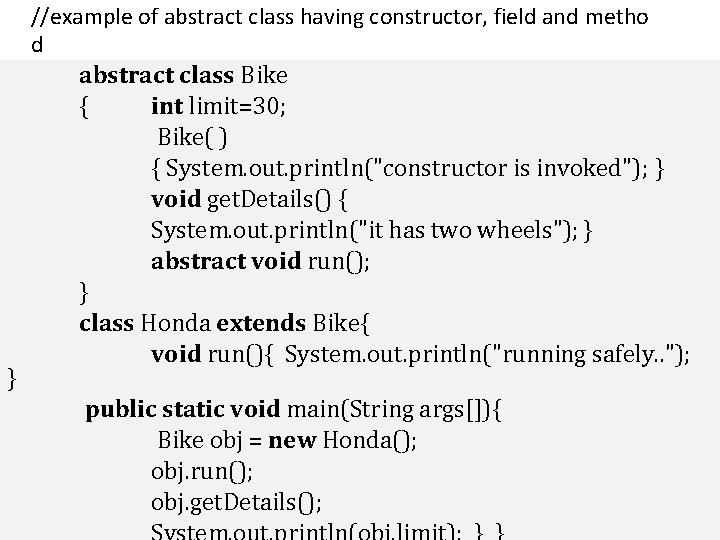
//example of abstract class having constructor, field and metho d abstract class Bike { int limit=30; Bike( ) { System. out. println("constructor is invoked"); } void get. Details() { System. out. println("it has two wheels"); } abstract void run(); } class Honda extends Bike{ void run(){ System. out. println("running safely. . "); } public static void main(String args[]){ Bike obj = new Honda(); obj. run(); obj. get. Details();
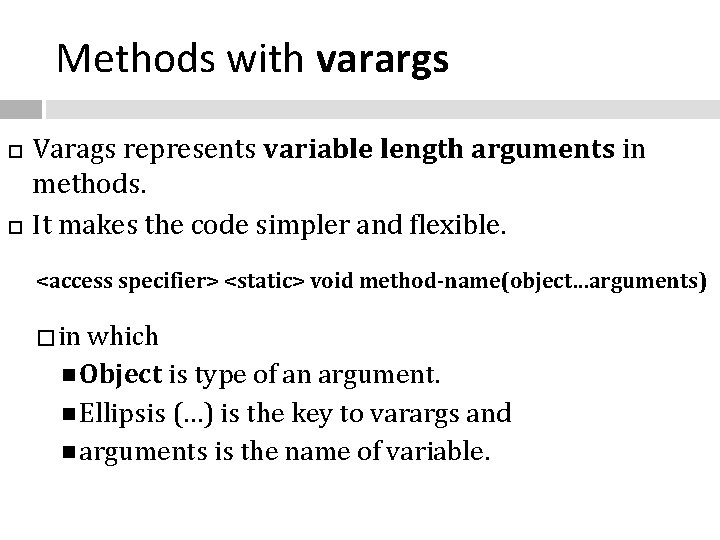
Methods with varargs Varags represents variable length arguments in methods. It makes the code simpler and flexible. <access specifier> <static> void method-name(object…arguments) � in which Object is type of an argument. Ellipsis (…) is the key to varargs and arguments is the name of variable.
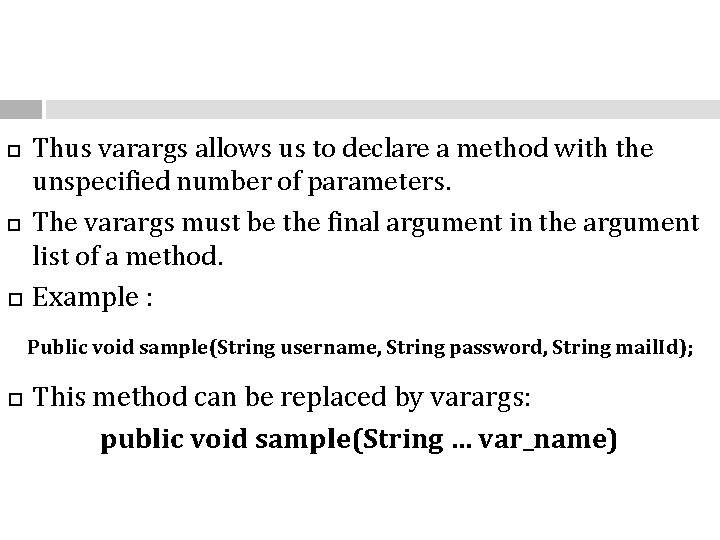
Thus varargs allows us to declare a method with the unspecified number of parameters. The varargs must be the final argument in the argument list of a method. Example : Public void sample(String username, String password, String mail. Id); This method can be replaced by varargs: public void sample(String … var_name)
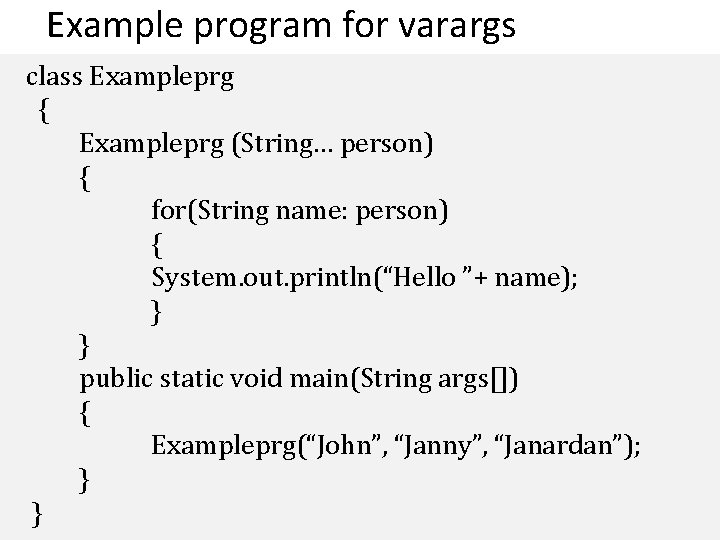
Example program for varargs class Exampleprg { Exampleprg (String… person) { for(String name: person) { System. out. println(“Hello ”+ name); } } public static void main(String args[]) { Exampleprg(“John”, “Janny”, “Janardan”); } }
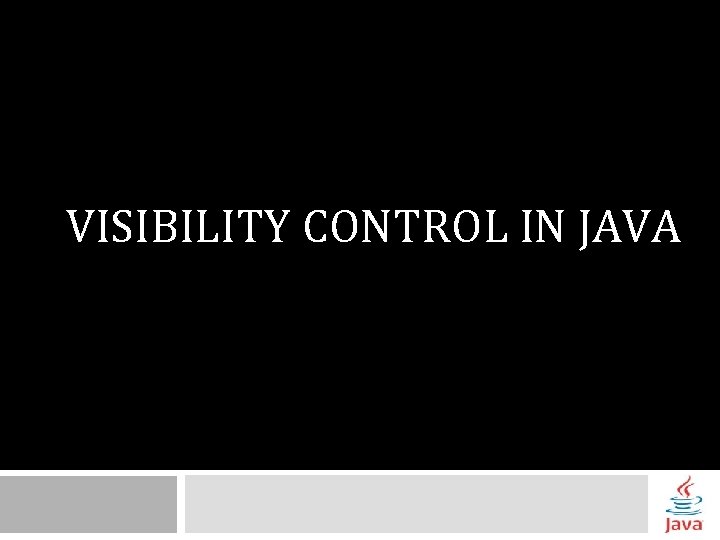
VISIBILITY CONTROL IN JAVA
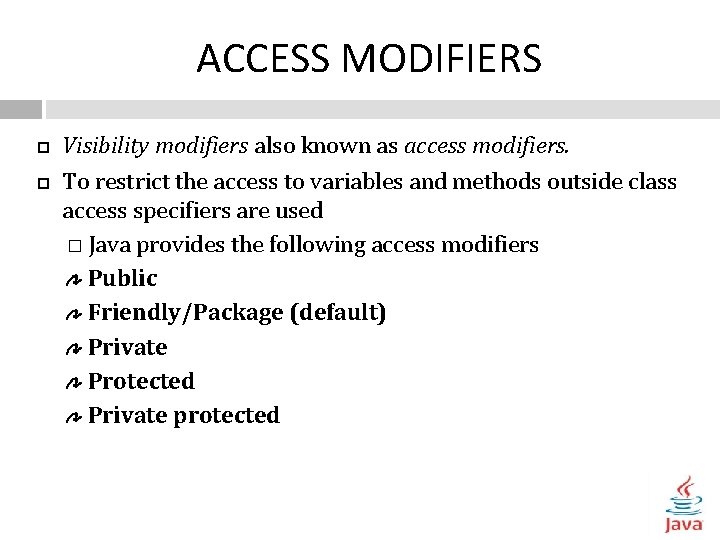
ACCESS MODIFIERS Visibility modifiers also known as access modifiers. To restrict the access to variables and methods outside class access specifiers are used � Java provides the following access modifiers Public Friendly/Package (default) Private Protected Private protected
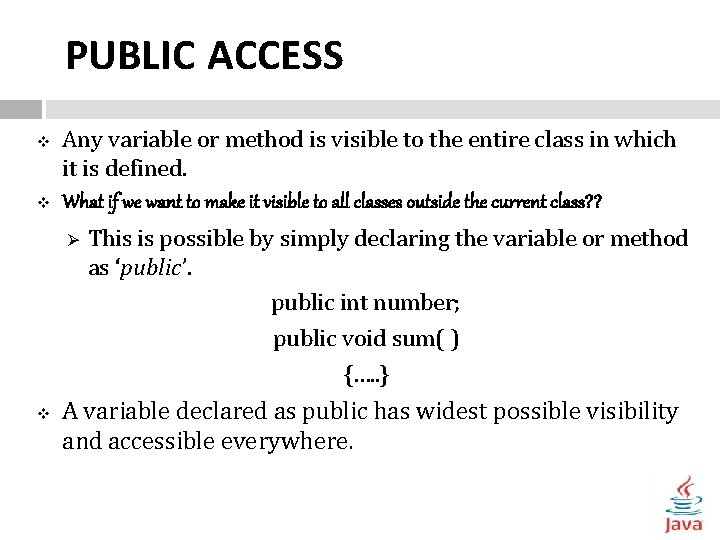
PUBLIC ACCESS v v Any variable or method is visible to the entire class in which it is defined. What if we want to make it visible to all classes outside the current class? ? Ø v This is possible by simply declaring the variable or method as ‘public’. public int number; public void sum( ) {…. . } A variable declared as public has widest possible visibility and accessible everywhere.
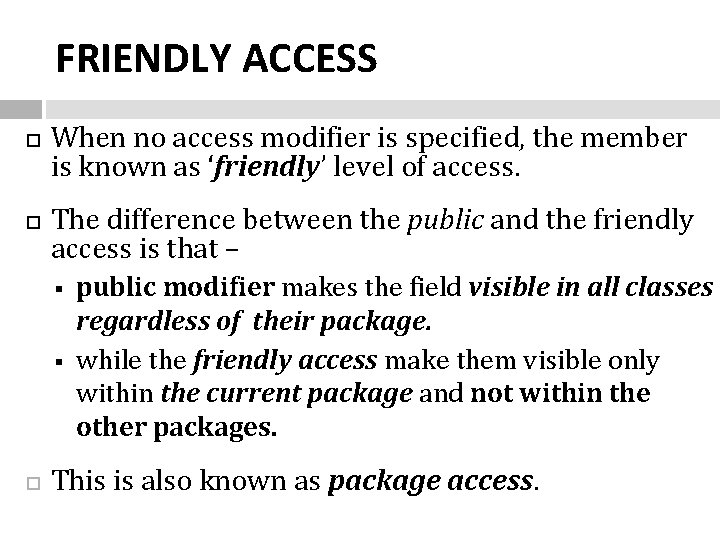
FRIENDLY ACCESS When no access modifier is specified, the member is known as ‘friendly’ level of access. The difference between the public and the friendly access is that – § § public modifier makes the field visible in all classes regardless of their package. while the friendly access make them visible only within the current package and not within the other packages. This is also known as package access.
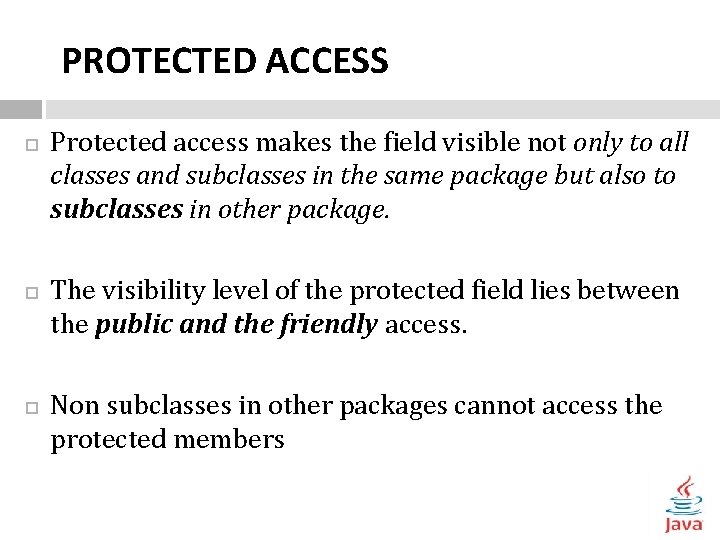
PROTECTED ACCESS Protected access makes the field visible not only to all classes and subclasses in the same package but also to subclasses in other package. The visibility level of the protected field lies between the public and the friendly access. Non subclasses in other packages cannot access the protected members
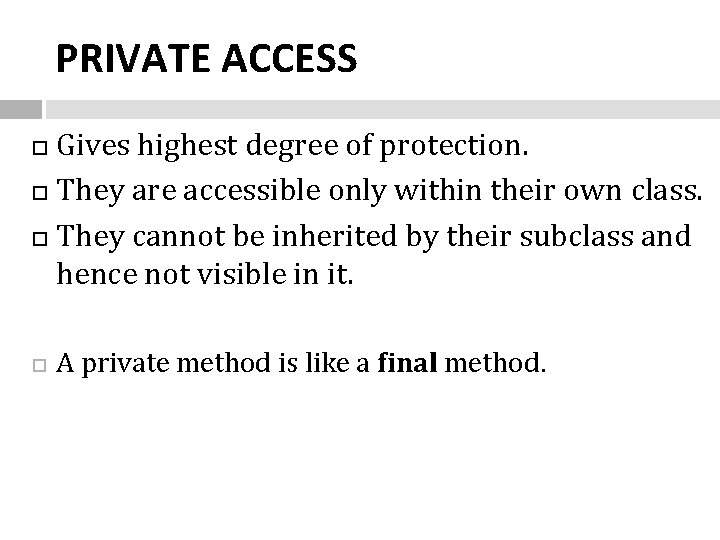
PRIVATE ACCESS Gives highest degree of protection. They are accessible only within their own class. They cannot be inherited by their subclass and hence not visible in it. A private method is like a final method.
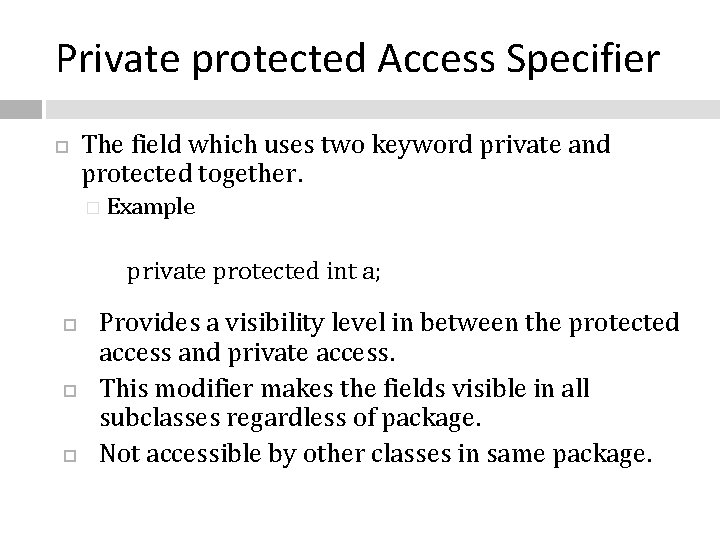
Private protected Access Specifier The field which uses two keyword private and protected together. � Example private protected int a; Provides a visibility level in between the protected access and private access. This modifier makes the fields visible in all subclasses regardless of package. Not accessible by other classes in same package.
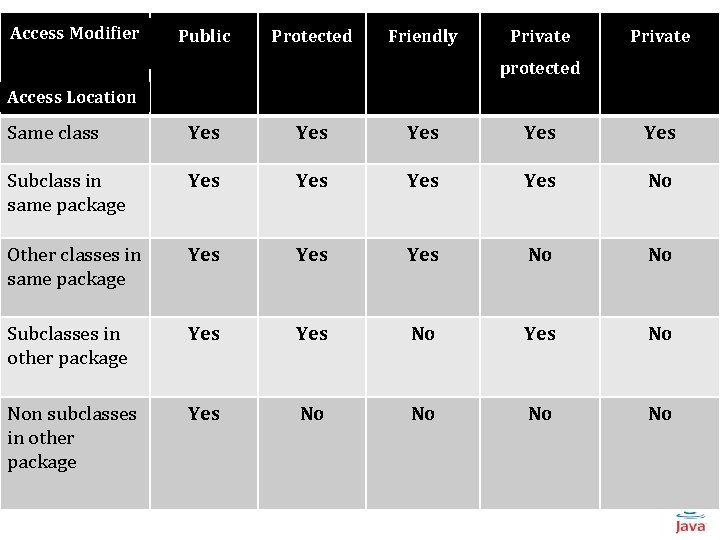
Access Modifier Public Protected Friendly Private protected Access Location Same class Yes Yes Yes Subclass in same package Yes Yes No Other classes in same package Yes Yes No No Subclasses in other package Yes No Non subclasses in other package Yes No No
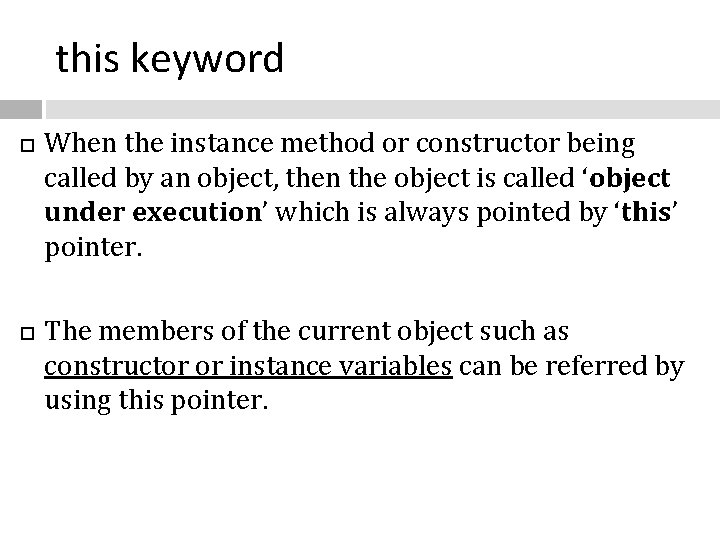
this keyword When the instance method or constructor being called by an object, then the object is called ‘object under execution’ which is always pointed by ‘this’ pointer. The members of the current object such as constructor or instance variables can be referred by using this pointer.
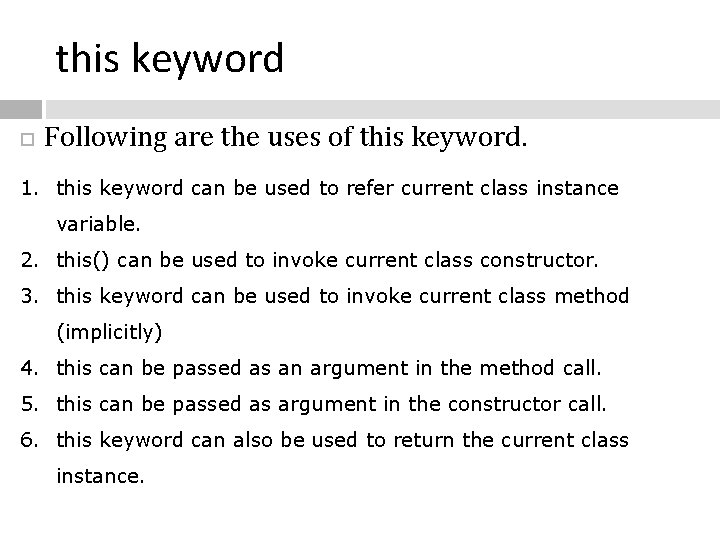
this keyword Following are the uses of this keyword. 1. this keyword can be used to refer current class instance variable. 2. this() can be used to invoke current class constructor. 3. this keyword can be used to invoke current class method (implicitly) 4. this can be passed as an argument in the method call. 5. this can be passed as argument in the constructor call. 6. this keyword can also be used to return the current class instance.
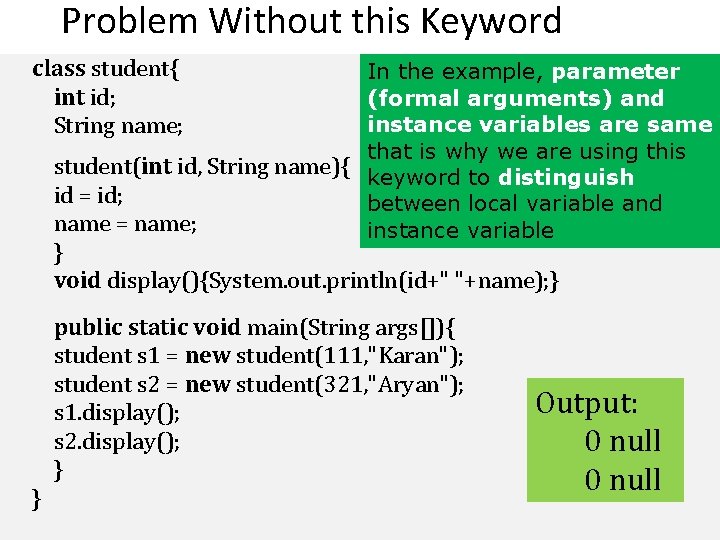
Problem Without this Keyword class student{ int id; String name; In the example, parameter (formal arguments) and instance variables are same that is why we are using this student(int id, String name){ keyword to distinguish id = id; between local variable and name = name; instance variable } void display(){System. out. println(id+" "+name); } public static void main(String args[]){ student s 1 = new student(111, "Karan"); student s 2 = new student(321, "Aryan"); s 1. display(); s 2. display(); } } Output: 0 null
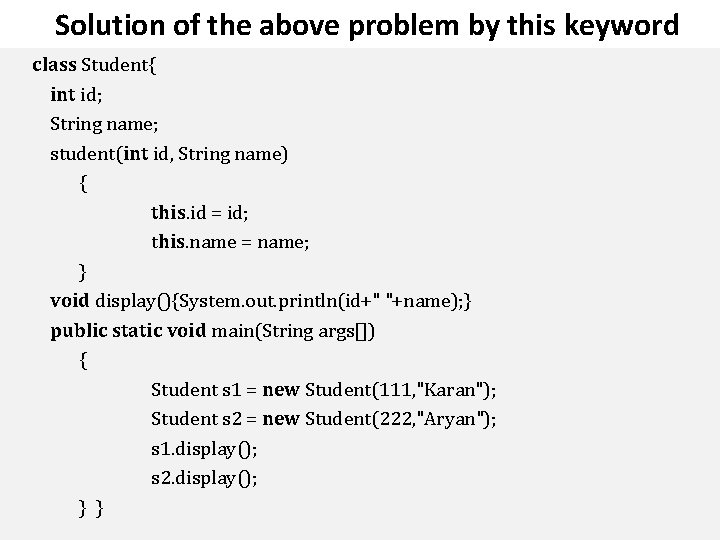
Solution of the above problem by this keyword class Student{ int id; String name; student(int id, String name) { this. id = id; this. name = name; } void display(){System. out. println(id+" "+name); } public static void main(String args[]) { Student s 1 = new Student(111, "Karan"); Student s 2 = new Student(222, "Aryan"); s 1. display(); s 2. display(); } }
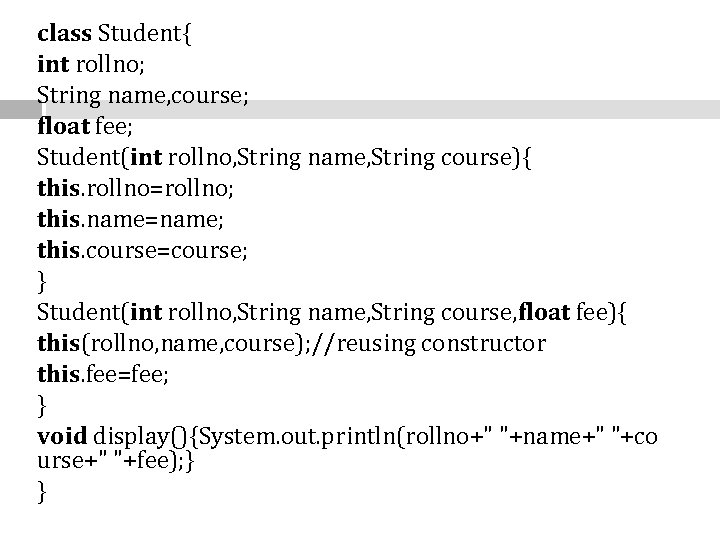
class Student{ int rollno; String name, course; float fee; Student(int rollno, String name, String course){ this. rollno=rollno; this. name=name; this. course=course; } Student(int rollno, String name, String course, float fee){ this(rollno, name, course); //reusing constructor this. fee=fee; } void display(){System. out. println(rollno+" "+name+" "+co urse+" "+fee); } }
![class Test. This{ public static void main(String args[]){ Student s 1=new Student(111, "ankit", "java"); class Test. This{ public static void main(String args[]){ Student s 1=new Student(111, "ankit", "java");](http://slidetodoc.com/presentation_image_h/046df2ce0367c31fa3f00706c67330a7/image-21.jpg)
class Test. This{ public static void main(String args[]){ Student s 1=new Student(111, "ankit", "java"); Student s 2=new Student(112, "sumit", "java", 6000 f ); s 1. display(); s 2. display(); }}
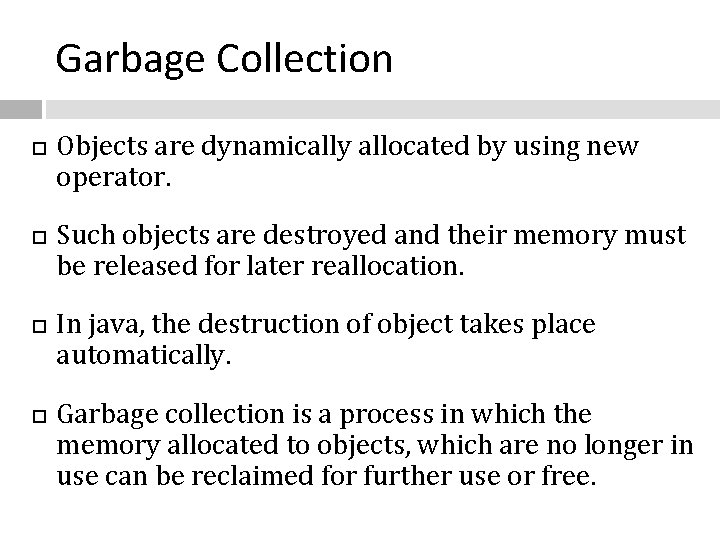
Garbage Collection Objects are dynamically allocated by using new operator. Such objects are destroyed and their memory must be released for later reallocation. In java, the destruction of object takes place automatically. Garbage collection is a process in which the memory allocated to objects, which are no longer in use can be reclaimed for further use or free.
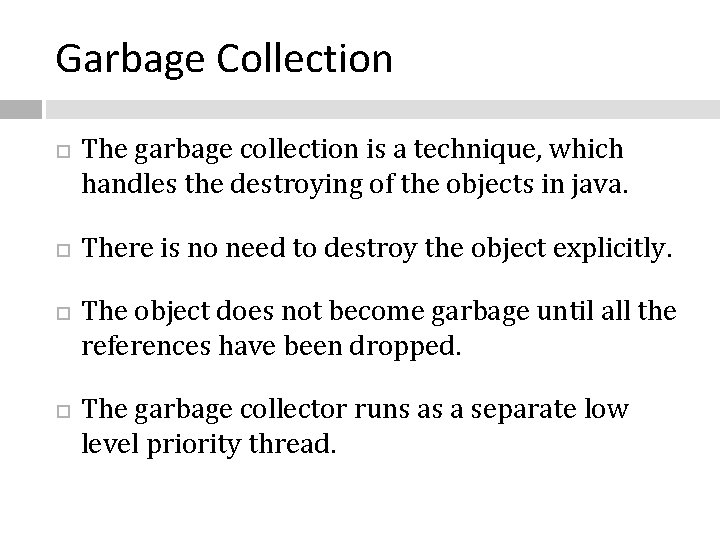
Garbage Collection The garbage collection is a technique, which handles the destroying of the objects in java. There is no need to destroy the object explicitly. The object does not become garbage until all the references have been dropped. The garbage collector runs as a separate low level priority thread.
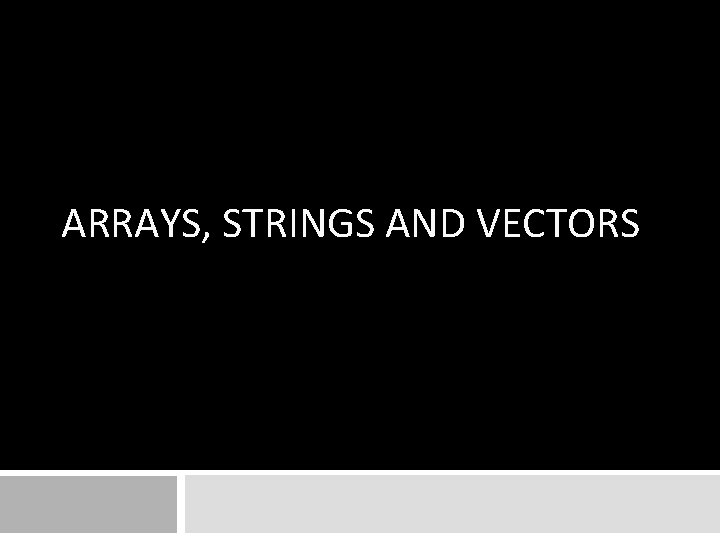
ARRAYS, STRINGS AND VECTORS
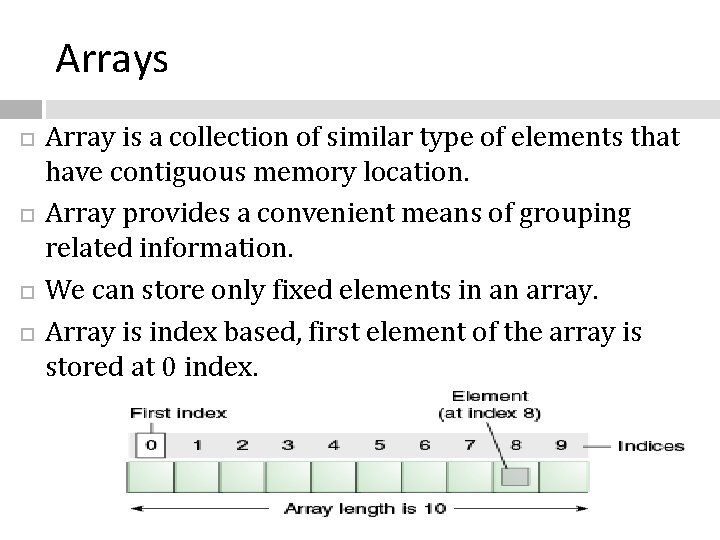
Arrays Array is a collection of similar type of elements that have contiguous memory location. Array provides a convenient means of grouping related information. We can store only fixed elements in an array. Array is index based, first element of the array is stored at 0 index.
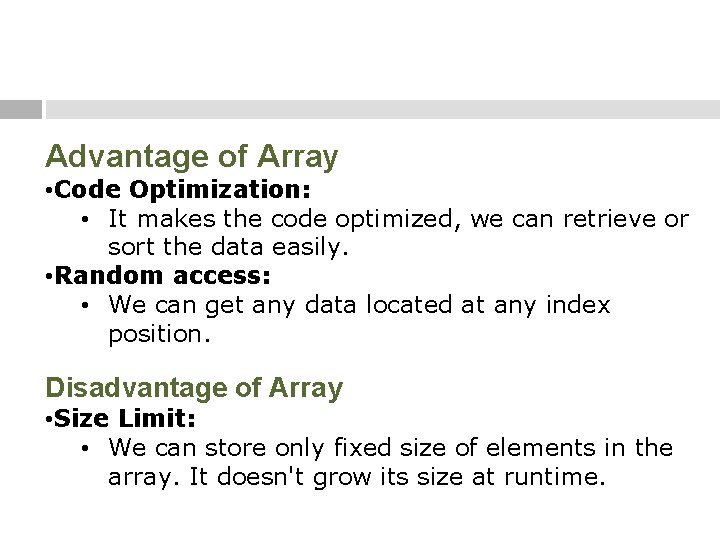
Advantage of Array • Code Optimization: • It makes the code optimized, we can retrieve or sort the data easily. • Random access: • We can get any data located at any index position. Disadvantage of Array • Size Limit: • We can store only fixed size of elements in the array. It doesn't grow its size at runtime.
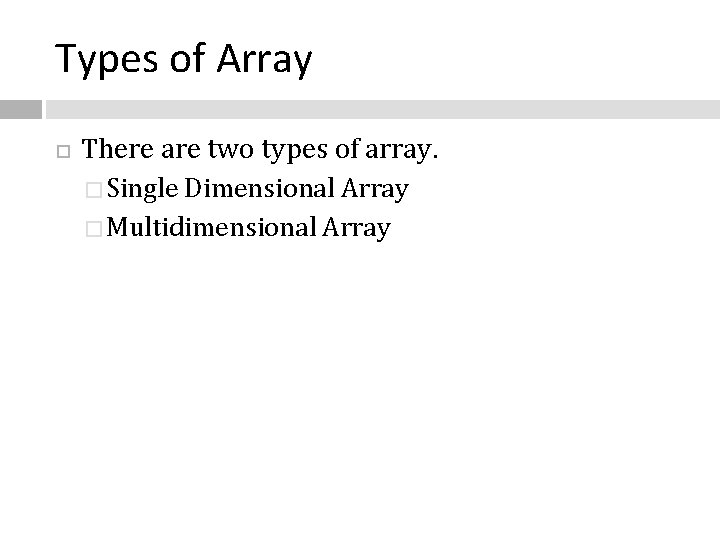
Types of Array There are two types of array. � Single Dimensional Array � Multidimensional Array
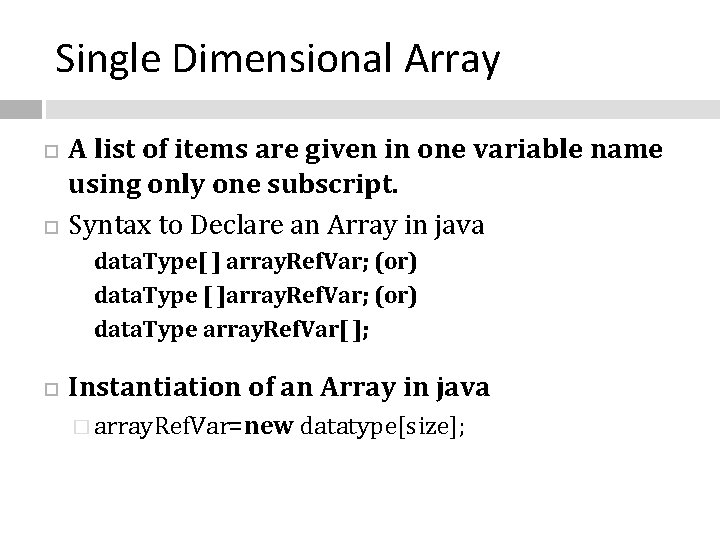
Single Dimensional Array A list of items are given in one variable name using only one subscript. Syntax to Declare an Array in java data. Type[ ] array. Ref. Var; (or) data. Type [ ]array. Ref. Var; (or) data. Type array. Ref. Var[ ]; Instantiation of an Array in java � array. Ref. Var=new datatype[size];
![Example of single dimensional java array class B { public static void main(String args[]) Example of single dimensional java array class B { public static void main(String args[])](http://slidetodoc.com/presentation_image_h/046df2ce0367c31fa3f00706c67330a7/image-29.jpg)
Example of single dimensional java array class B { public static void main(String args[]) { int a[]=new int[5]; //declaration and instantiation a[0]=10; //initialization a[1]=20; a[2]=70; a[3]=40; a[4]=50; //printing array for(int i=0; i<a. length; i++) //length is the property of array System. out. println(a[i]); }}
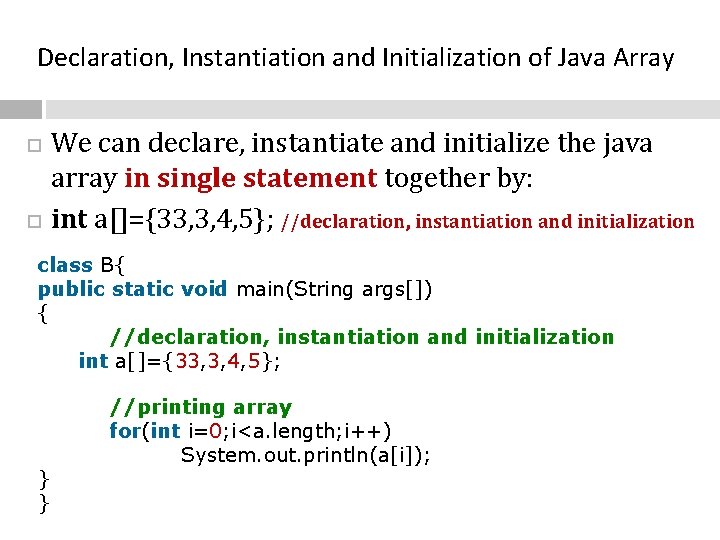
Declaration, Instantiation and Initialization of Java Array We can declare, instantiate and initialize the java array in single statement together by: int a[]={33, 3, 4, 5}; //declaration, instantiation and initialization class B{ public static void main(String args[]) { //declaration, instantiation and initialization int a[]={33, 3, 4, 5}; } } //printing array for(int i=0; i<a. length; i++) System. out. println(a[i]);
![Passing Java Array in the method class B{ static void min(int arr[]) { int Passing Java Array in the method class B{ static void min(int arr[]) { int](http://slidetodoc.com/presentation_image_h/046df2ce0367c31fa3f00706c67330a7/image-31.jpg)
Passing Java Array in the method class B{ static void min(int arr[]) { int min=arr[0]; for(int i=1; i<arr. length; i++) if(min>arr[i]) min=arr[i]; System. out. println(min); } public static void main(String args[]) { int a[]={33, 3, 4, 5}; min(a); //passing array in the method }}
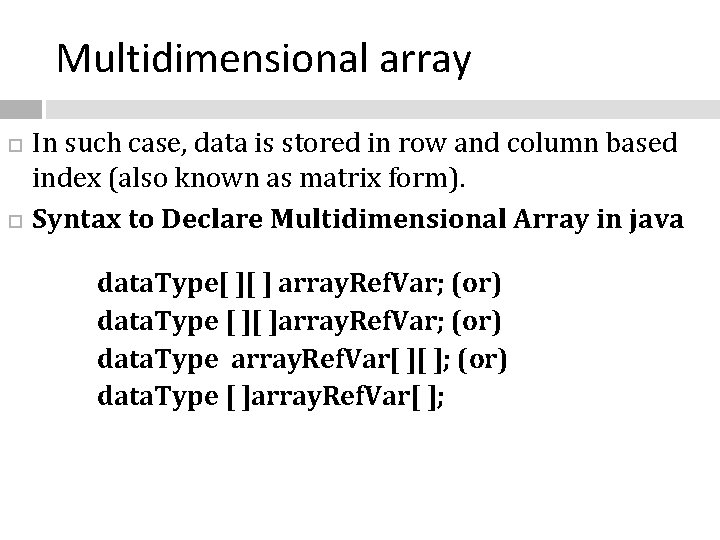
Multidimensional array In such case, data is stored in row and column based index (also known as matrix form). Syntax to Declare Multidimensional Array in java data. Type[ ][ ] array. Ref. Var; (or) data. Type [ ][ ]array. Ref. Var; (or) data. Type array. Ref. Var[ ][ ]; (or) data. Type [ ]array. Ref. Var[ ];
![Example to instantiate Multidimensional Array in java int[ ][ ] arr=new int[3][3]; //3 Example to instantiate Multidimensional Array in java int[ ][ ] arr=new int[3][3]; //3](http://slidetodoc.com/presentation_image_h/046df2ce0367c31fa3f00706c67330a7/image-33.jpg)
Example to instantiate Multidimensional Array in java int[ ][ ] arr=new int[3][3]; //3 row and 3 column Example to initialize Multidimensional Array in java arr[0][0]=1; arr[0][1]=2; arr[0][2]=3; arr[1][0]=4; arr[1][1]=5; arr[1][2]=6; arr[2][0]=7; arr[2][1]=8; arr[2][2]=9;
![Example of Multidimensional java array class B { public static void main(String args[]) { Example of Multidimensional java array class B { public static void main(String args[]) {](http://slidetodoc.com/presentation_image_h/046df2ce0367c31fa3f00706c67330a7/image-34.jpg)
Example of Multidimensional java array class B { public static void main(String args[]) { int arr[][]={{1, 2, 3}, {2, 4, 5}, {4, 4, 5}}; //declaring and initializing 2 D array for(int i=0; i<3; i++) { for(int j=0; j<3; j++) { System. out. print(arr[i][j]+" "); } System. out. println(); } } }
![Addition of 2 matrices class B { public static void main(String args[]) { int Addition of 2 matrices class B { public static void main(String args[]) { int](http://slidetodoc.com/presentation_image_h/046df2ce0367c31fa3f00706c67330a7/image-35.jpg)
Addition of 2 matrices class B { public static void main(String args[]) { int arr[][]={ {1, 2, 3}, {2, 4, 5}, {4, 4, 5} }; for(int i=0; i<3; i++) { for(int j=0; j<3; j++) { System. out. print(arr[i][j]+" "); } System. out. println(); } }
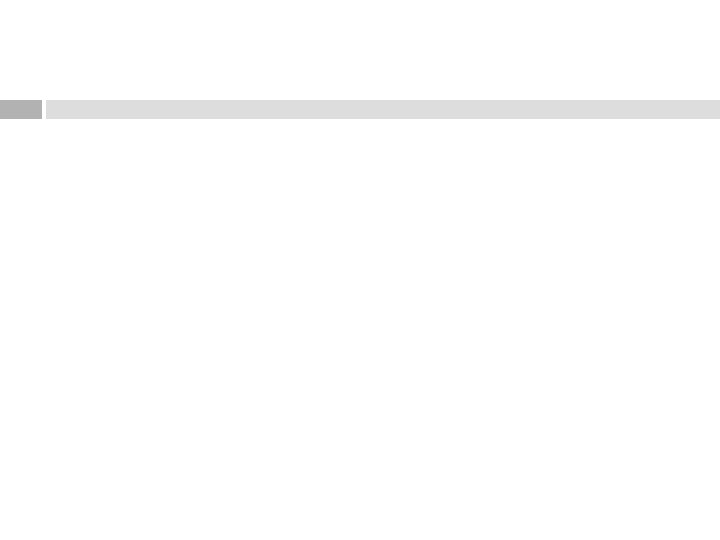
- Slides: 36